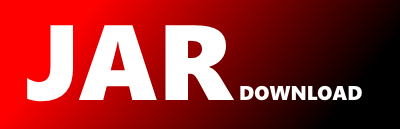
com.amazonaws.services.pinpointsmsvoicev2.AmazonPinpointSMSVoiceV2 Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.pinpointsmsvoicev2.model.*;
/**
* Interface for accessing Amazon Pinpoint SMS Voice V2.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.pinpointsmsvoicev2.AbstractAmazonPinpointSMSVoiceV2} instead.
*
*
*
* Welcome to the AWS End User Messaging SMS and Voice, version 2 API Reference. This guide provides information
* about AWS End User Messaging SMS and Voice, version 2 API resources, including supported HTTP methods, parameters,
* and schemas.
*
*
* Amazon Pinpoint is an Amazon Web Services service that you can use to engage with your recipients across multiple
* messaging channels. The AWS End User Messaging SMS and Voice, version 2 API provides programmatic access to options
* that are unique to the SMS and voice channels. AWS End User Messaging SMS and Voice, version 2 resources such as
* phone numbers, sender IDs, and opt-out lists can be used by the Amazon Pinpoint API.
*
*
* If you're new to AWS End User Messaging SMS and Voice, it's also helpful to review the AWS End User Messaging SMS User
* Guide. The AWS End User Messaging SMS User Guide provides tutorials, code samples, and procedures that
* demonstrate how to use AWS End User Messaging SMS and Voice features programmatically and how to integrate
* functionality into mobile apps and other types of applications. The guide also provides key information, such as AWS
* End User Messaging SMS and Voice integration with other Amazon Web Services services, and the quotas that apply to
* use of the service.
*
*
* Regional availability
*
*
* The AWS End User Messaging SMS and Voice version 2 API Reference is available in several Amazon Web Services
* Regions and it provides an endpoint for each of these Regions. For a list of all the Regions and endpoints where the
* API is currently available, see Amazon Web Services Service
* Endpoints and Amazon Pinpoint endpoints and
* quotas in the Amazon Web Services General Reference. To learn more about Amazon Web Services Regions, see Managing Amazon Web Services Regions in
* the Amazon Web Services General Reference.
*
*
* In each Region, Amazon Web Services maintains multiple Availability Zones. These Availability Zones are physically
* isolated from each other, but are united by private, low-latency, high-throughput, and highly redundant network
* connections. These Availability Zones enable us to provide very high levels of availability and redundancy, while
* also minimizing latency. To learn more about the number of Availability Zones that are available in each Region, see
* Amazon Web Services Global Infrastructure.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonPinpointSMSVoiceV2 {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "sms-voice";
/**
*
* Associates the specified origination identity with a pool.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* If the origination identity configuration doesn't match the pool's configuration, an error is returned.
*
*
* @param associateOriginationIdentityRequest
* @return Result of the AssociateOriginationIdentity operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.AssociateOriginationIdentity
* @see AWS API Documentation
*/
AssociateOriginationIdentityResult associateOriginationIdentity(AssociateOriginationIdentityRequest associateOriginationIdentityRequest);
/**
*
* Associate a protect configuration with a configuration set. This replaces the configuration sets current protect
* configuration. A configuration set can only be associated with one protect configuration at a time. A protect
* configuration can be associated with multiple configuration sets.
*
*
* @param associateProtectConfigurationRequest
* @return Result of the AssociateProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.AssociateProtectConfiguration
* @see AWS API Documentation
*/
AssociateProtectConfigurationResult associateProtectConfiguration(AssociateProtectConfigurationRequest associateProtectConfigurationRequest);
/**
*
* Creates a new configuration set. After you create the configuration set, you can add one or more event
* destinations to it.
*
*
* A configuration set is a set of rules that you apply to the SMS and voice messages that you send.
*
*
* When you send a message, you can optionally specify a single configuration set.
*
*
* @param createConfigurationSetRequest
* @return Result of the CreateConfigurationSet operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateConfigurationSet
* @see AWS API Documentation
*/
CreateConfigurationSetResult createConfigurationSet(CreateConfigurationSetRequest createConfigurationSetRequest);
/**
*
* Creates a new event destination in a configuration set.
*
*
* An event destination is a location where you send message events. The event options are Amazon CloudWatch, Amazon
* Data Firehose, or Amazon SNS. For example, when a message is delivered successfully, you can send information
* about that event to an event destination, or send notifications to endpoints that are subscribed to an Amazon SNS
* topic.
*
*
* Each configuration set can contain between 0 and 5 event destinations. Each event destination can contain a
* reference to a single destination, such as a CloudWatch or Firehose destination.
*
*
* @param createEventDestinationRequest
* @return Result of the CreateEventDestination operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateEventDestination
* @see AWS API Documentation
*/
CreateEventDestinationResult createEventDestination(CreateEventDestinationRequest createEventDestinationRequest);
/**
*
* Creates a new opt-out list.
*
*
* If the opt-out list name already exists, an error is returned.
*
*
* An opt-out list is a list of phone numbers that are opted out, meaning you can't send SMS or voice messages to
* them. If end user replies with the keyword "STOP," an entry for the phone number is added to the opt-out list. In
* addition to STOP, your recipients can use any supported opt-out keyword, such as CANCEL or OPTOUT. For a list of
* supported opt-out keywords, see SMS
* opt out in the AWS End User Messaging SMS User Guide.
*
*
* @param createOptOutListRequest
* @return Result of the CreateOptOutList operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateOptOutList
* @see AWS API Documentation
*/
CreateOptOutListResult createOptOutList(CreateOptOutListRequest createOptOutListRequest);
/**
*
* Creates a new pool and associates the specified origination identity to the pool. A pool can include one or more
* phone numbers and SenderIds that are associated with your Amazon Web Services account.
*
*
* The new pool inherits its configuration from the specified origination identity. This includes keywords, message
* type, opt-out list, two-way configuration, and self-managed opt-out configuration. Deletion protection isn't
* inherited from the origination identity and defaults to false.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* @param createPoolRequest
* @return Result of the CreatePool operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreatePool
* @see AWS API Documentation
*/
CreatePoolResult createPool(CreatePoolRequest createPoolRequest);
/**
*
* Create a new protect configuration. By default all country rule sets for each capability are set to
* ALLOW
. Update the country rule sets using UpdateProtectConfigurationCountryRuleSet
. A
* protect configurations name is stored as a Tag with the key set to Name
and value as the name of the
* protect configuration.
*
*
* @param createProtectConfigurationRequest
* @return Result of the CreateProtectConfiguration operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateProtectConfiguration
* @see AWS API Documentation
*/
CreateProtectConfigurationResult createProtectConfiguration(CreateProtectConfigurationRequest createProtectConfigurationRequest);
/**
*
* Creates a new registration based on the RegistrationType field.
*
*
* @param createRegistrationRequest
* @return Result of the CreateRegistration operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateRegistration
* @see AWS API Documentation
*/
CreateRegistrationResult createRegistration(CreateRegistrationRequest createRegistrationRequest);
/**
*
* Associate the registration with an origination identity such as a phone number or sender ID.
*
*
* @param createRegistrationAssociationRequest
* @return Result of the CreateRegistrationAssociation operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateRegistrationAssociation
* @see AWS API Documentation
*/
CreateRegistrationAssociationResult createRegistrationAssociation(CreateRegistrationAssociationRequest createRegistrationAssociationRequest);
/**
*
* Create a new registration attachment to use for uploading a file or a URL to a file. The maximum file size is
* 1MiB and valid file extensions are PDF, JPEG and PNG. For example, many sender ID registrations require a signed
* “letter of authorization” (LOA) to be submitted.
*
*
* @param createRegistrationAttachmentRequest
* @return Result of the CreateRegistrationAttachment operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateRegistrationAttachment
* @see AWS API Documentation
*/
CreateRegistrationAttachmentResult createRegistrationAttachment(CreateRegistrationAttachmentRequest createRegistrationAttachmentRequest);
/**
*
* Create a new version of the registration and increase the VersionNumber. The previous version of the
* registration becomes read-only.
*
*
* @param createRegistrationVersionRequest
* @return Result of the CreateRegistrationVersion operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateRegistrationVersion
* @see AWS API Documentation
*/
CreateRegistrationVersionResult createRegistrationVersion(CreateRegistrationVersionRequest createRegistrationVersionRequest);
/**
*
* You can only send messages to verified destination numbers when your account is in the sandbox. You can add up to
* 10 verified destination numbers.
*
*
* @param createVerifiedDestinationNumberRequest
* @return Result of the CreateVerifiedDestinationNumber operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.CreateVerifiedDestinationNumber
* @see AWS API Documentation
*/
CreateVerifiedDestinationNumberResult createVerifiedDestinationNumber(CreateVerifiedDestinationNumberRequest createVerifiedDestinationNumberRequest);
/**
*
* Removes the current account default protect configuration.
*
*
* @param deleteAccountDefaultProtectConfigurationRequest
* @return Result of the DeleteAccountDefaultProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
DeleteAccountDefaultProtectConfigurationResult deleteAccountDefaultProtectConfiguration(
DeleteAccountDefaultProtectConfigurationRequest deleteAccountDefaultProtectConfigurationRequest);
/**
*
* Deletes an existing configuration set.
*
*
* A configuration set is a set of rules that you apply to voice and SMS messages that you send. In a configuration
* set, you can specify a destination for specific types of events related to voice and SMS messages.
*
*
* @param deleteConfigurationSetRequest
* @return Result of the DeleteConfigurationSet operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteConfigurationSet
* @see AWS API Documentation
*/
DeleteConfigurationSetResult deleteConfigurationSet(DeleteConfigurationSetRequest deleteConfigurationSetRequest);
/**
*
* Deletes an existing default message type on a configuration set.
*
*
* A message type is a type of messages that you plan to send. If you send account-related messages or
* time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param deleteDefaultMessageTypeRequest
* @return Result of the DeleteDefaultMessageType operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteDefaultMessageType
* @see AWS API Documentation
*/
DeleteDefaultMessageTypeResult deleteDefaultMessageType(DeleteDefaultMessageTypeRequest deleteDefaultMessageTypeRequest);
/**
*
* Deletes an existing default sender ID on a configuration set.
*
*
* A default sender ID is the identity that appears on recipients' devices when they receive SMS messages. Support
* for sender ID capabilities varies by country or region.
*
*
* @param deleteDefaultSenderIdRequest
* @return Result of the DeleteDefaultSenderId operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteDefaultSenderId
* @see AWS API Documentation
*/
DeleteDefaultSenderIdResult deleteDefaultSenderId(DeleteDefaultSenderIdRequest deleteDefaultSenderIdRequest);
/**
*
* Deletes an existing event destination.
*
*
* An event destination is a location where you send response information about the messages that you send. For
* example, when a message is delivered successfully, you can send information about that event to an Amazon
* CloudWatch destination, or send notifications to endpoints that are subscribed to an Amazon SNS topic.
*
*
* @param deleteEventDestinationRequest
* @return Result of the DeleteEventDestination operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteEventDestination
* @see AWS API Documentation
*/
DeleteEventDestinationResult deleteEventDestination(DeleteEventDestinationRequest deleteEventDestinationRequest);
/**
*
* Deletes an existing keyword from an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* Keywords "HELP" and "STOP" can't be deleted or modified.
*
*
* @param deleteKeywordRequest
* @return Result of the DeleteKeyword operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteKeyword
* @see AWS API Documentation
*/
DeleteKeywordResult deleteKeyword(DeleteKeywordRequest deleteKeywordRequest);
/**
*
* Deletes an account-level monthly spending limit override for sending multimedia messages (MMS). Deleting a spend
* limit override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas for Server Migration Service
* in the Server Migration Service User Guide.
*
*
* @param deleteMediaMessageSpendLimitOverrideRequest
* @return Result of the DeleteMediaMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
DeleteMediaMessageSpendLimitOverrideResult deleteMediaMessageSpendLimitOverride(
DeleteMediaMessageSpendLimitOverrideRequest deleteMediaMessageSpendLimitOverrideRequest);
/**
*
* Deletes an existing opt-out list. All opted out phone numbers in the opt-out list are deleted.
*
*
* If the specified opt-out list name doesn't exist or is in-use by an origination phone number or pool, an error is
* returned.
*
*
* @param deleteOptOutListRequest
* @return Result of the DeleteOptOutList operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteOptOutList
* @see AWS API Documentation
*/
DeleteOptOutListResult deleteOptOutList(DeleteOptOutListRequest deleteOptOutListRequest);
/**
*
* Deletes an existing opted out destination phone number from the specified opt-out list.
*
*
* Each destination phone number can only be deleted once every 30 days.
*
*
* If the specified destination phone number doesn't exist or if the opt-out list doesn't exist, an error is
* returned.
*
*
* @param deleteOptedOutNumberRequest
* @return Result of the DeleteOptedOutNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteOptedOutNumber
* @see AWS API Documentation
*/
DeleteOptedOutNumberResult deleteOptedOutNumber(DeleteOptedOutNumberRequest deleteOptedOutNumberRequest);
/**
*
* Deletes an existing pool. Deleting a pool disassociates all origination identities from that pool.
*
*
* If the pool status isn't active or if deletion protection is enabled, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param deletePoolRequest
* @return Result of the DeletePool operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeletePool
* @see AWS API Documentation
*/
DeletePoolResult deletePool(DeletePoolRequest deletePoolRequest);
/**
*
* Permanently delete the protect configuration. The protect configuration must have deletion protection disabled
* and must not be associated as the account default protect configuration or associated with a configuration set.
*
*
* @param deleteProtectConfigurationRequest
* @return Result of the DeleteProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteProtectConfiguration
* @see AWS API Documentation
*/
DeleteProtectConfigurationResult deleteProtectConfiguration(DeleteProtectConfigurationRequest deleteProtectConfigurationRequest);
/**
*
* Permanently delete an existing registration from your account.
*
*
* @param deleteRegistrationRequest
* @return Result of the DeleteRegistration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteRegistration
* @see AWS API Documentation
*/
DeleteRegistrationResult deleteRegistration(DeleteRegistrationRequest deleteRegistrationRequest);
/**
*
* Permanently delete the specified registration attachment.
*
*
* @param deleteRegistrationAttachmentRequest
* @return Result of the DeleteRegistrationAttachment operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteRegistrationAttachment
* @see AWS API Documentation
*/
DeleteRegistrationAttachmentResult deleteRegistrationAttachment(DeleteRegistrationAttachmentRequest deleteRegistrationAttachmentRequest);
/**
*
* Delete the value in a registration form field.
*
*
* @param deleteRegistrationFieldValueRequest
* @return Result of the DeleteRegistrationFieldValue operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteRegistrationFieldValue
* @see AWS API Documentation
*/
DeleteRegistrationFieldValueResult deleteRegistrationFieldValue(DeleteRegistrationFieldValueRequest deleteRegistrationFieldValueRequest);
/**
*
* Deletes an account-level monthly spending limit override for sending text messages. Deleting a spend limit
* override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteTextMessageSpendLimitOverrideRequest
* @return Result of the DeleteTextMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
DeleteTextMessageSpendLimitOverrideResult deleteTextMessageSpendLimitOverride(
DeleteTextMessageSpendLimitOverrideRequest deleteTextMessageSpendLimitOverrideRequest);
/**
*
* Delete a verified destination phone number.
*
*
* @param deleteVerifiedDestinationNumberRequest
* @return Result of the DeleteVerifiedDestinationNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteVerifiedDestinationNumber
* @see AWS API Documentation
*/
DeleteVerifiedDestinationNumberResult deleteVerifiedDestinationNumber(DeleteVerifiedDestinationNumberRequest deleteVerifiedDestinationNumberRequest);
/**
*
* Deletes an account level monthly spend limit override for sending voice messages. Deleting a spend limit override
* sets the EnforcedLimit
equal to the MaxLimit
, which is controlled by Amazon Web
* Services. For more information on spending limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteVoiceMessageSpendLimitOverrideRequest
* @return Result of the DeleteVoiceMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DeleteVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
DeleteVoiceMessageSpendLimitOverrideResult deleteVoiceMessageSpendLimitOverride(
DeleteVoiceMessageSpendLimitOverrideRequest deleteVoiceMessageSpendLimitOverrideRequest);
/**
*
* Describes attributes of your Amazon Web Services account. The supported account attributes include account tier,
* which indicates whether your account is in the sandbox or production environment. When you're ready to move your
* account out of the sandbox, create an Amazon Web Services Support case for a service limit increase request.
*
*
* New accounts are placed into an SMS or voice sandbox. The sandbox protects both Amazon Web Services end
* recipients and SMS or voice recipients from fraud and abuse.
*
*
* @param describeAccountAttributesRequest
* @return Result of the DescribeAccountAttributes operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeAccountAttributes
* @see AWS API Documentation
*/
DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Describes the current AWS End User Messaging SMS and Voice SMS Voice V2 resource quotas for your account. The
* description for a quota includes the quota name, current usage toward that quota, and the quota's maximum value.
*
*
* When you establish an Amazon Web Services account, the account has initial quotas on the maximum number of
* configuration sets, opt-out lists, phone numbers, and pools that you can create in a given Region. For more
* information see Quotas in the
* AWS End User Messaging SMS User Guide.
*
*
* @param describeAccountLimitsRequest
* @return Result of the DescribeAccountLimits operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeAccountLimits
* @see AWS API Documentation
*/
DescribeAccountLimitsResult describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Describes the specified configuration sets or all in your account.
*
*
* If you specify configuration set names, the output includes information for only the specified configuration
* sets. If you specify filters, the output includes information for only those configuration sets that meet the
* filter criteria. If you don't specify configuration set names or filters, the output includes information for all
* configuration sets.
*
*
* If you specify a configuration set name that isn't valid, an error is returned.
*
*
* @param describeConfigurationSetsRequest
* @return Result of the DescribeConfigurationSets operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeConfigurationSets
* @see AWS API Documentation
*/
DescribeConfigurationSetsResult describeConfigurationSets(DescribeConfigurationSetsRequest describeConfigurationSetsRequest);
/**
*
* Describes the specified keywords or all keywords on your origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param describeKeywordsRequest
* @return Result of the DescribeKeywords operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeKeywords
* @see AWS API Documentation
*/
DescribeKeywordsResult describeKeywords(DescribeKeywordsRequest describeKeywordsRequest);
/**
*
* Describes the specified opt-out list or all opt-out lists in your account.
*
*
* If you specify opt-out list names, the output includes information for only the specified opt-out lists. Opt-out
* lists include only those that meet the filter criteria. If you don't specify opt-out list names or filters, the
* output includes information for all opt-out lists.
*
*
* If you specify an opt-out list name that isn't valid, an error is returned.
*
*
* @param describeOptOutListsRequest
* @return Result of the DescribeOptOutLists operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeOptOutLists
* @see AWS API Documentation
*/
DescribeOptOutListsResult describeOptOutLists(DescribeOptOutListsRequest describeOptOutListsRequest);
/**
*
* Describes the specified opted out destination numbers or all opted out destination numbers in an opt-out list.
*
*
* If you specify opted out numbers, the output includes information for only the specified opted out numbers. If
* you specify filters, the output includes information for only those opted out numbers that meet the filter
* criteria. If you don't specify opted out numbers or filters, the output includes information for all opted out
* destination numbers in your opt-out list.
*
*
* If you specify an opted out number that isn't valid, an error is returned.
*
*
* @param describeOptedOutNumbersRequest
* @return Result of the DescribeOptedOutNumbers operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeOptedOutNumbers
* @see AWS API Documentation
*/
DescribeOptedOutNumbersResult describeOptedOutNumbers(DescribeOptedOutNumbersRequest describeOptedOutNumbersRequest);
/**
*
* Describes the specified origination phone number, or all the phone numbers in your account.
*
*
* If you specify phone number IDs, the output includes information for only the specified phone numbers. If you
* specify filters, the output includes information for only those phone numbers that meet the filter criteria. If
* you don't specify phone number IDs or filters, the output includes information for all phone numbers.
*
*
* If you specify a phone number ID that isn't valid, an error is returned.
*
*
* @param describePhoneNumbersRequest
* @return Result of the DescribePhoneNumbers operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribePhoneNumbers
* @see AWS API Documentation
*/
DescribePhoneNumbersResult describePhoneNumbers(DescribePhoneNumbersRequest describePhoneNumbersRequest);
/**
*
* Retrieves the specified pools or all pools associated with your Amazon Web Services account.
*
*
* If you specify pool IDs, the output includes information for only the specified pools. If you specify filters,
* the output includes information for only those pools that meet the filter criteria. If you don't specify pool IDs
* or filters, the output includes information for all pools.
*
*
* If you specify a pool ID that isn't valid, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param describePoolsRequest
* @return Result of the DescribePools operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribePools
* @see AWS API Documentation
*/
DescribePoolsResult describePools(DescribePoolsRequest describePoolsRequest);
/**
*
* Retrieves the protect configurations that match any of filters. If a filter isn’t provided then all protect
* configurations are returned.
*
*
* @param describeProtectConfigurationsRequest
* @return Result of the DescribeProtectConfigurations operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeProtectConfigurations
* @see AWS API Documentation
*/
DescribeProtectConfigurationsResult describeProtectConfigurations(DescribeProtectConfigurationsRequest describeProtectConfigurationsRequest);
/**
*
* Retrieves the specified registration attachments or all registration attachments associated with your Amazon Web
* Services account.
*
*
* @param describeRegistrationAttachmentsRequest
* @return Result of the DescribeRegistrationAttachments operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationAttachments
* @see AWS API Documentation
*/
DescribeRegistrationAttachmentsResult describeRegistrationAttachments(DescribeRegistrationAttachmentsRequest describeRegistrationAttachmentsRequest);
/**
*
* Retrieves the specified registration type field definitions. You can use DescribeRegistrationFieldDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationFieldDefinitionsRequest
* @return Result of the DescribeRegistrationFieldDefinitions operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationFieldDefinitions
* @see AWS API Documentation
*/
DescribeRegistrationFieldDefinitionsResult describeRegistrationFieldDefinitions(
DescribeRegistrationFieldDefinitionsRequest describeRegistrationFieldDefinitionsRequest);
/**
*
* Retrieves the specified registration field values.
*
*
* @param describeRegistrationFieldValuesRequest
* @return Result of the DescribeRegistrationFieldValues operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationFieldValues
* @see AWS API Documentation
*/
DescribeRegistrationFieldValuesResult describeRegistrationFieldValues(DescribeRegistrationFieldValuesRequest describeRegistrationFieldValuesRequest);
/**
*
* Retrieves the specified registration section definitions. You can use DescribeRegistrationSectionDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationSectionDefinitionsRequest
* @return Result of the DescribeRegistrationSectionDefinitions operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationSectionDefinitions
* @see AWS API Documentation
*/
DescribeRegistrationSectionDefinitionsResult describeRegistrationSectionDefinitions(
DescribeRegistrationSectionDefinitionsRequest describeRegistrationSectionDefinitionsRequest);
/**
*
* Retrieves the specified registration type definitions. You can use DescribeRegistrationTypeDefinitions to view
* the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationTypeDefinitionsRequest
* @return Result of the DescribeRegistrationTypeDefinitions operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationTypeDefinitions
* @see AWS API Documentation
*/
DescribeRegistrationTypeDefinitionsResult describeRegistrationTypeDefinitions(
DescribeRegistrationTypeDefinitionsRequest describeRegistrationTypeDefinitionsRequest);
/**
*
* Retrieves the specified registration version.
*
*
* @param describeRegistrationVersionsRequest
* @return Result of the DescribeRegistrationVersions operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrationVersions
* @see AWS API Documentation
*/
DescribeRegistrationVersionsResult describeRegistrationVersions(DescribeRegistrationVersionsRequest describeRegistrationVersionsRequest);
/**
*
* Retrieves the specified registrations.
*
*
* @param describeRegistrationsRequest
* @return Result of the DescribeRegistrations operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeRegistrations
* @see AWS API Documentation
*/
DescribeRegistrationsResult describeRegistrations(DescribeRegistrationsRequest describeRegistrationsRequest);
/**
*
* Describes the specified SenderIds or all SenderIds associated with your Amazon Web Services account.
*
*
* If you specify SenderIds, the output includes information for only the specified SenderIds. If you specify
* filters, the output includes information for only those SenderIds that meet the filter criteria. If you don't
* specify SenderIds or filters, the output includes information for all SenderIds.
*
*
* f you specify a sender ID that isn't valid, an error is returned.
*
*
* @param describeSenderIdsRequest
* @return Result of the DescribeSenderIds operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeSenderIds
* @see AWS API Documentation
*/
DescribeSenderIdsResult describeSenderIds(DescribeSenderIdsRequest describeSenderIdsRequest);
/**
*
* Describes the current monthly spend limits for sending voice and text messages.
*
*
* When you establish an Amazon Web Services account, the account has initial monthly spend limit in a given Region.
* For more information on increasing your monthly spend limit, see Requesting
* increases to your monthly SMS, MMS, or Voice spending quota in the AWS End User Messaging SMS User
* Guide.
*
*
* @param describeSpendLimitsRequest
* @return Result of the DescribeSpendLimits operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeSpendLimits
* @see AWS API Documentation
*/
DescribeSpendLimitsResult describeSpendLimits(DescribeSpendLimitsRequest describeSpendLimitsRequest);
/**
*
* Retrieves the specified verified destiona numbers.
*
*
* @param describeVerifiedDestinationNumbersRequest
* @return Result of the DescribeVerifiedDestinationNumbers operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DescribeVerifiedDestinationNumbers
* @see AWS API Documentation
*/
DescribeVerifiedDestinationNumbersResult describeVerifiedDestinationNumbers(
DescribeVerifiedDestinationNumbersRequest describeVerifiedDestinationNumbersRequest);
/**
*
* Removes the specified origination identity from an existing pool.
*
*
* If the origination identity isn't associated with the specified pool, an error is returned.
*
*
* @param disassociateOriginationIdentityRequest
* @return Result of the DisassociateOriginationIdentity operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DisassociateOriginationIdentity
* @see AWS API Documentation
*/
DisassociateOriginationIdentityResult disassociateOriginationIdentity(DisassociateOriginationIdentityRequest disassociateOriginationIdentityRequest);
/**
*
* Disassociate a protect configuration from a configuration set.
*
*
* @param disassociateProtectConfigurationRequest
* @return Result of the DisassociateProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DisassociateProtectConfiguration
* @see AWS API Documentation
*/
DisassociateProtectConfigurationResult disassociateProtectConfiguration(DisassociateProtectConfigurationRequest disassociateProtectConfigurationRequest);
/**
*
* Discard the current version of the registration.
*
*
* @param discardRegistrationVersionRequest
* @return Result of the DiscardRegistrationVersion operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.DiscardRegistrationVersion
* @see AWS API Documentation
*/
DiscardRegistrationVersionResult discardRegistrationVersion(DiscardRegistrationVersionRequest discardRegistrationVersionRequest);
/**
*
* Retrieve the CountryRuleSet for the specified NumberCapability from a protect configuration.
*
*
* @param getProtectConfigurationCountryRuleSetRequest
* @return Result of the GetProtectConfigurationCountryRuleSet operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.GetProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
GetProtectConfigurationCountryRuleSetResult getProtectConfigurationCountryRuleSet(
GetProtectConfigurationCountryRuleSetRequest getProtectConfigurationCountryRuleSetRequest);
/**
*
* Lists all associated origination identities in your pool.
*
*
* If you specify filters, the output includes information for only those origination identities that meet the
* filter criteria.
*
*
* @param listPoolOriginationIdentitiesRequest
* @return Result of the ListPoolOriginationIdentities operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.ListPoolOriginationIdentities
* @see AWS API Documentation
*/
ListPoolOriginationIdentitiesResult listPoolOriginationIdentities(ListPoolOriginationIdentitiesRequest listPoolOriginationIdentitiesRequest);
/**
*
* Retreive all of the origination identies that are associated with a registration.
*
*
* @param listRegistrationAssociationsRequest
* @return Result of the ListRegistrationAssociations operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.ListRegistrationAssociations
* @see AWS API Documentation
*/
ListRegistrationAssociationsResult listRegistrationAssociations(ListRegistrationAssociationsRequest listRegistrationAssociationsRequest);
/**
*
* List all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Creates or updates a keyword configuration on an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param putKeywordRequest
* @return Result of the PutKeyword operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.PutKeyword
* @see AWS API Documentation
*/
PutKeywordResult putKeyword(PutKeywordRequest putKeywordRequest);
/**
*
* Creates an opted out destination phone number in the opt-out list.
*
*
* If the destination phone number isn't valid or if the specified opt-out list doesn't exist, an error is returned.
*
*
* @param putOptedOutNumberRequest
* @return Result of the PutOptedOutNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.PutOptedOutNumber
* @see AWS API Documentation
*/
PutOptedOutNumberResult putOptedOutNumber(PutOptedOutNumberRequest putOptedOutNumberRequest);
/**
*
* Creates or updates a field value for a registration.
*
*
* @param putRegistrationFieldValueRequest
* @return Result of the PutRegistrationFieldValue operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.PutRegistrationFieldValue
* @see AWS API Documentation
*/
PutRegistrationFieldValueResult putRegistrationFieldValue(PutRegistrationFieldValueRequest putRegistrationFieldValueRequest);
/**
*
* Releases an existing origination phone number in your account. Once released, a phone number is no longer
* available for sending messages.
*
*
* If the origination phone number has deletion protection enabled or is associated with a pool, an error is
* returned.
*
*
* @param releasePhoneNumberRequest
* @return Result of the ReleasePhoneNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.ReleasePhoneNumber
* @see AWS API Documentation
*/
ReleasePhoneNumberResult releasePhoneNumber(ReleasePhoneNumberRequest releasePhoneNumberRequest);
/**
*
* Releases an existing sender ID in your account.
*
*
* @param releaseSenderIdRequest
* @return Result of the ReleaseSenderId operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.ReleaseSenderId
* @see AWS API Documentation
*/
ReleaseSenderIdResult releaseSenderId(ReleaseSenderIdRequest releaseSenderIdRequest);
/**
*
* Request an origination phone number for use in your account. For more information on phone number request see Request a phone
* number in the AWS End User Messaging SMS User Guide.
*
*
* @param requestPhoneNumberRequest
* @return Result of the RequestPhoneNumber operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.RequestPhoneNumber
* @see AWS API Documentation
*/
RequestPhoneNumberResult requestPhoneNumber(RequestPhoneNumberRequest requestPhoneNumberRequest);
/**
*
* Request a new sender ID that doesn't require registration.
*
*
* @param requestSenderIdRequest
* @return Result of the RequestSenderId operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.RequestSenderId
* @see AWS API Documentation
*/
RequestSenderIdResult requestSenderId(RequestSenderIdRequest requestSenderIdRequest);
/**
*
* Before you can send test messages to a verified destination phone number you need to opt-in the verified
* destination phone number. Creates a new text message with a verification code and send it to a verified
* destination phone number. Once you have the verification code use VerifyDestinationNumber to opt-in the
* verified destination phone number to receive messages.
*
*
* @param sendDestinationNumberVerificationCodeRequest
* @return Result of the SendDestinationNumberVerificationCode operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SendDestinationNumberVerificationCode
* @see AWS API Documentation
*/
SendDestinationNumberVerificationCodeResult sendDestinationNumberVerificationCode(
SendDestinationNumberVerificationCodeRequest sendDestinationNumberVerificationCodeRequest);
/**
*
* Creates a new multimedia message (MMS) and sends it to a recipient's phone number.
*
*
* @param sendMediaMessageRequest
* @return Result of the SendMediaMessage operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SendMediaMessage
* @see AWS API Documentation
*/
SendMediaMessageResult sendMediaMessage(SendMediaMessageRequest sendMediaMessageRequest);
/**
*
* Creates a new text message and sends it to a recipient's phone number. SendTextMessage only sends an SMS message
* to one recipient each time it is invoked.
*
*
* SMS throughput limits are measured in Message Parts per Second (MPS). Your MPS limit depends on the destination
* country of your messages, as well as the type of phone number (origination number) that you use to send the
* message. For more information about MPS, see Message Parts per Second
* (MPS) limits in the AWS End User Messaging SMS User Guide.
*
*
* @param sendTextMessageRequest
* @return Result of the SendTextMessage operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SendTextMessage
* @see AWS API Documentation
*/
SendTextMessageResult sendTextMessage(SendTextMessageRequest sendTextMessageRequest);
/**
*
* Allows you to send a request that sends a voice message. This operation uses Amazon Polly to convert a text script into a voice message.
*
*
* @param sendVoiceMessageRequest
* @return Result of the SendVoiceMessage operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SendVoiceMessage
* @see AWS API Documentation
*/
SendVoiceMessageResult sendVoiceMessage(SendVoiceMessageRequest sendVoiceMessageRequest);
/**
*
* Set a protect configuration as your account default. You can only have one account default protect configuration
* at a time. The current account default protect configuration is replaced with the provided protect configuration.
*
*
* @param setAccountDefaultProtectConfigurationRequest
* @return Result of the SetAccountDefaultProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
SetAccountDefaultProtectConfigurationResult setAccountDefaultProtectConfiguration(
SetAccountDefaultProtectConfigurationRequest setAccountDefaultProtectConfigurationRequest);
/**
*
* Sets the default message type on a configuration set.
*
*
* Choose the category of SMS messages that you plan to send from this account. If you send account-related messages
* or time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param setDefaultMessageTypeRequest
* @return Result of the SetDefaultMessageType operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetDefaultMessageType
* @see AWS API Documentation
*/
SetDefaultMessageTypeResult setDefaultMessageType(SetDefaultMessageTypeRequest setDefaultMessageTypeRequest);
/**
*
* Sets default sender ID on a configuration set.
*
*
* When sending a text message to a destination country that supports sender IDs, the default sender ID on the
* configuration set specified will be used if no dedicated origination phone numbers or registered sender IDs are
* available in your account.
*
*
* @param setDefaultSenderIdRequest
* @return Result of the SetDefaultSenderId operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetDefaultSenderId
* @see AWS API Documentation
*/
SetDefaultSenderIdResult setDefaultSenderId(SetDefaultSenderIdRequest setDefaultSenderIdRequest);
/**
*
* Sets an account level monthly spend limit override for sending MMS messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setMediaMessageSpendLimitOverrideRequest
* @return Result of the SetMediaMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
SetMediaMessageSpendLimitOverrideResult setMediaMessageSpendLimitOverride(SetMediaMessageSpendLimitOverrideRequest setMediaMessageSpendLimitOverrideRequest);
/**
*
* Sets an account level monthly spend limit override for sending text messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setTextMessageSpendLimitOverrideRequest
* @return Result of the SetTextMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
SetTextMessageSpendLimitOverrideResult setTextMessageSpendLimitOverride(SetTextMessageSpendLimitOverrideRequest setTextMessageSpendLimitOverrideRequest);
/**
*
* Sets an account level monthly spend limit override for sending voice messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setVoiceMessageSpendLimitOverrideRequest
* @return Result of the SetVoiceMessageSpendLimitOverride operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SetVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
SetVoiceMessageSpendLimitOverrideResult setVoiceMessageSpendLimitOverride(SetVoiceMessageSpendLimitOverrideRequest setVoiceMessageSpendLimitOverrideRequest);
/**
*
* Submit the specified registration for review and approval.
*
*
* @param submitRegistrationVersionRequest
* @return Result of the SubmitRegistrationVersion operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.SubmitRegistrationVersion
* @see AWS API Documentation
*/
SubmitRegistrationVersionResult submitRegistrationVersion(SubmitRegistrationVersionRequest submitRegistrationVersionRequest);
/**
*
* Adds or overwrites only the specified tags for the specified resource. When you specify an existing tag key, the
* value is overwritten with the new value. Each resource can have a maximum of 50 tags. Each tag consists of a key
* and an optional value. Tag keys must be unique per resource. For more information about tags, see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ServiceQuotaExceededException
* The request would cause a service quota to be exceeded.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes the association of the specified tags from a resource. For more information on tags see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an existing event destination in a configuration set. You can update the IAM role ARN for CloudWatch Logs
* and Firehose. You can also enable or disable the event destination.
*
*
* You may want to update an event destination to change its matching event types or updating the destination
* resource ARN. You can't change an event destination's type between CloudWatch Logs, Firehose, and Amazon SNS.
*
*
* @param updateEventDestinationRequest
* @return Result of the UpdateEventDestination operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdateEventDestination
* @see AWS API Documentation
*/
UpdateEventDestinationResult updateEventDestination(UpdateEventDestinationRequest updateEventDestinationRequest);
/**
*
* Updates the configuration of an existing origination phone number. You can update the opt-out list, enable or
* disable two-way messaging, change the TwoWayChannelArn, enable or disable self-managed opt-outs, and enable or
* disable deletion protection.
*
*
* If the origination phone number is associated with a pool, an error is returned.
*
*
* @param updatePhoneNumberRequest
* @return Result of the UpdatePhoneNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdatePhoneNumber
* @see AWS API Documentation
*/
UpdatePhoneNumberResult updatePhoneNumber(UpdatePhoneNumberRequest updatePhoneNumberRequest);
/**
*
* Updates the configuration of an existing pool. You can update the opt-out list, enable or disable two-way
* messaging, change the TwoWayChannelArn
, enable or disable self-managed opt-outs, enable or disable
* deletion protection, and enable or disable shared routes.
*
*
* @param updatePoolRequest
* @return Result of the UpdatePool operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdatePool
* @see AWS API Documentation
*/
UpdatePoolResult updatePool(UpdatePoolRequest updatePoolRequest);
/**
*
* Update the setting for an existing protect configuration.
*
*
* @param updateProtectConfigurationRequest
* @return Result of the UpdateProtectConfiguration operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdateProtectConfiguration
* @see AWS API Documentation
*/
UpdateProtectConfigurationResult updateProtectConfiguration(UpdateProtectConfigurationRequest updateProtectConfigurationRequest);
/**
*
* Update a country rule set to ALLOW
or BLOCK
messages to be sent to the specified
* destination counties. You can update one or multiple countries at a time. The updates are only applied to the
* specified NumberCapability type.
*
*
* @param updateProtectConfigurationCountryRuleSetRequest
* @return Result of the UpdateProtectConfigurationCountryRuleSet operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdateProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
UpdateProtectConfigurationCountryRuleSetResult updateProtectConfigurationCountryRuleSet(
UpdateProtectConfigurationCountryRuleSetRequest updateProtectConfigurationCountryRuleSetRequest);
/**
*
* Updates the configuration of an existing sender ID.
*
*
* @param updateSenderIdRequest
* @return Result of the UpdateSenderId operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.UpdateSenderId
* @see AWS API Documentation
*/
UpdateSenderIdResult updateSenderId(UpdateSenderIdRequest updateSenderIdRequest);
/**
*
* Use the verification code that was received by the verified destination phone number to opt-in the verified
* destination phone number to receive more messages.
*
*
* @param verifyDestinationNumberRequest
* @return Result of the VerifyDestinationNumber operation returned by the service.
* @throws ThrottlingException
* An error that occurred because too many requests were sent during a certain amount of time.
* @throws AccessDeniedException
* The request was denied because you don't have sufficient permissions to access the resource.
* @throws ResourceNotFoundException
* A requested resource couldn't be found.
* @throws ValidationException
* A validation exception for a field.
* @throws ConflictException
* Your request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time or it could be that the requested action isn't valid for
* the current state or configuration of the resource.
* @throws InternalServerException
* The API encountered an unexpected error and couldn't complete the request. You might be able to
* successfully issue the request again in the future.
* @sample AmazonPinpointSMSVoiceV2.VerifyDestinationNumber
* @see AWS API Documentation
*/
VerifyDestinationNumberResult verifyDestinationNumber(VerifyDestinationNumberRequest verifyDestinationNumberRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}