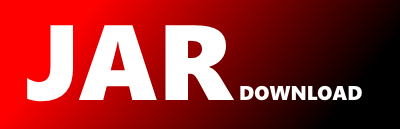
com.amazonaws.services.pinpointsmsvoicev2.AmazonPinpointSMSVoiceV2Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2;
import javax.annotation.Generated;
import com.amazonaws.services.pinpointsmsvoicev2.model.*;
/**
* Interface for accessing Amazon Pinpoint SMS Voice V2 asynchronously. Each asynchronous method will return a Java
* Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.pinpointsmsvoicev2.AbstractAmazonPinpointSMSVoiceV2Async} instead.
*
*
*
* Welcome to the AWS End User Messaging SMS and Voice, version 2 API Reference. This guide provides information
* about AWS End User Messaging SMS and Voice, version 2 API resources, including supported HTTP methods, parameters,
* and schemas.
*
*
* Amazon Pinpoint is an Amazon Web Services service that you can use to engage with your recipients across multiple
* messaging channels. The AWS End User Messaging SMS and Voice, version 2 API provides programmatic access to options
* that are unique to the SMS and voice channels. AWS End User Messaging SMS and Voice, version 2 resources such as
* phone numbers, sender IDs, and opt-out lists can be used by the Amazon Pinpoint API.
*
*
* If you're new to AWS End User Messaging SMS and Voice, it's also helpful to review the AWS End User Messaging SMS User
* Guide. The AWS End User Messaging SMS User Guide provides tutorials, code samples, and procedures that
* demonstrate how to use AWS End User Messaging SMS and Voice features programmatically and how to integrate
* functionality into mobile apps and other types of applications. The guide also provides key information, such as AWS
* End User Messaging SMS and Voice integration with other Amazon Web Services services, and the quotas that apply to
* use of the service.
*
*
* Regional availability
*
*
* The AWS End User Messaging SMS and Voice version 2 API Reference is available in several Amazon Web Services
* Regions and it provides an endpoint for each of these Regions. For a list of all the Regions and endpoints where the
* API is currently available, see Amazon Web Services Service
* Endpoints and Amazon Pinpoint endpoints and
* quotas in the Amazon Web Services General Reference. To learn more about Amazon Web Services Regions, see Managing Amazon Web Services Regions in
* the Amazon Web Services General Reference.
*
*
* In each Region, Amazon Web Services maintains multiple Availability Zones. These Availability Zones are physically
* isolated from each other, but are united by private, low-latency, high-throughput, and highly redundant network
* connections. These Availability Zones enable us to provide very high levels of availability and redundancy, while
* also minimizing latency. To learn more about the number of Availability Zones that are available in each Region, see
* Amazon Web Services Global Infrastructure.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonPinpointSMSVoiceV2Async extends AmazonPinpointSMSVoiceV2 {
/**
*
* Associates the specified origination identity with a pool.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* If the origination identity configuration doesn't match the pool's configuration, an error is returned.
*
*
* @param associateOriginationIdentityRequest
* @return A Java Future containing the result of the AssociateOriginationIdentity operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.AssociateOriginationIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future associateOriginationIdentityAsync(
AssociateOriginationIdentityRequest associateOriginationIdentityRequest);
/**
*
* Associates the specified origination identity with a pool.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* If the origination identity configuration doesn't match the pool's configuration, an error is returned.
*
*
* @param associateOriginationIdentityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateOriginationIdentity operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.AssociateOriginationIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future associateOriginationIdentityAsync(
AssociateOriginationIdentityRequest associateOriginationIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate a protect configuration with a configuration set. This replaces the configuration sets current protect
* configuration. A configuration set can only be associated with one protect configuration at a time. A protect
* configuration can be associated with multiple configuration sets.
*
*
* @param associateProtectConfigurationRequest
* @return A Java Future containing the result of the AssociateProtectConfiguration operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.AssociateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future associateProtectConfigurationAsync(
AssociateProtectConfigurationRequest associateProtectConfigurationRequest);
/**
*
* Associate a protect configuration with a configuration set. This replaces the configuration sets current protect
* configuration. A configuration set can only be associated with one protect configuration at a time. A protect
* configuration can be associated with multiple configuration sets.
*
*
* @param associateProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateProtectConfiguration operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.AssociateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future associateProtectConfigurationAsync(
AssociateProtectConfigurationRequest associateProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new configuration set. After you create the configuration set, you can add one or more event
* destinations to it.
*
*
* A configuration set is a set of rules that you apply to the SMS and voice messages that you send.
*
*
* When you send a message, you can optionally specify a single configuration set.
*
*
* @param createConfigurationSetRequest
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest);
/**
*
* Creates a new configuration set. After you create the configuration set, you can add one or more event
* destinations to it.
*
*
* A configuration set is a set of rules that you apply to the SMS and voice messages that you send.
*
*
* When you send a message, you can optionally specify a single configuration set.
*
*
* @param createConfigurationSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future createConfigurationSetAsync(CreateConfigurationSetRequest createConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new event destination in a configuration set.
*
*
* An event destination is a location where you send message events. The event options are Amazon CloudWatch, Amazon
* Data Firehose, or Amazon SNS. For example, when a message is delivered successfully, you can send information
* about that event to an event destination, or send notifications to endpoints that are subscribed to an Amazon SNS
* topic.
*
*
* Each configuration set can contain between 0 and 5 event destinations. Each event destination can contain a
* reference to a single destination, such as a CloudWatch or Firehose destination.
*
*
* @param createEventDestinationRequest
* @return A Java Future containing the result of the CreateEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventDestinationAsync(CreateEventDestinationRequest createEventDestinationRequest);
/**
*
* Creates a new event destination in a configuration set.
*
*
* An event destination is a location where you send message events. The event options are Amazon CloudWatch, Amazon
* Data Firehose, or Amazon SNS. For example, when a message is delivered successfully, you can send information
* about that event to an event destination, or send notifications to endpoints that are subscribed to an Amazon SNS
* topic.
*
*
* Each configuration set can contain between 0 and 5 event destinations. Each event destination can contain a
* reference to a single destination, such as a CloudWatch or Firehose destination.
*
*
* @param createEventDestinationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventDestinationAsync(CreateEventDestinationRequest createEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new opt-out list.
*
*
* If the opt-out list name already exists, an error is returned.
*
*
* An opt-out list is a list of phone numbers that are opted out, meaning you can't send SMS or voice messages to
* them. If end user replies with the keyword "STOP," an entry for the phone number is added to the opt-out list. In
* addition to STOP, your recipients can use any supported opt-out keyword, such as CANCEL or OPTOUT. For a list of
* supported opt-out keywords, see SMS
* opt out in the AWS End User Messaging SMS User Guide.
*
*
* @param createOptOutListRequest
* @return A Java Future containing the result of the CreateOptOutList operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateOptOutList
* @see AWS API Documentation
*/
java.util.concurrent.Future createOptOutListAsync(CreateOptOutListRequest createOptOutListRequest);
/**
*
* Creates a new opt-out list.
*
*
* If the opt-out list name already exists, an error is returned.
*
*
* An opt-out list is a list of phone numbers that are opted out, meaning you can't send SMS or voice messages to
* them. If end user replies with the keyword "STOP," an entry for the phone number is added to the opt-out list. In
* addition to STOP, your recipients can use any supported opt-out keyword, such as CANCEL or OPTOUT. For a list of
* supported opt-out keywords, see SMS
* opt out in the AWS End User Messaging SMS User Guide.
*
*
* @param createOptOutListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOptOutList operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateOptOutList
* @see AWS API Documentation
*/
java.util.concurrent.Future createOptOutListAsync(CreateOptOutListRequest createOptOutListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new pool and associates the specified origination identity to the pool. A pool can include one or more
* phone numbers and SenderIds that are associated with your Amazon Web Services account.
*
*
* The new pool inherits its configuration from the specified origination identity. This includes keywords, message
* type, opt-out list, two-way configuration, and self-managed opt-out configuration. Deletion protection isn't
* inherited from the origination identity and defaults to false.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* @param createPoolRequest
* @return A Java Future containing the result of the CreatePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreatePool
* @see AWS API Documentation
*/
java.util.concurrent.Future createPoolAsync(CreatePoolRequest createPoolRequest);
/**
*
* Creates a new pool and associates the specified origination identity to the pool. A pool can include one or more
* phone numbers and SenderIds that are associated with your Amazon Web Services account.
*
*
* The new pool inherits its configuration from the specified origination identity. This includes keywords, message
* type, opt-out list, two-way configuration, and self-managed opt-out configuration. Deletion protection isn't
* inherited from the origination identity and defaults to false.
*
*
* If the origination identity is a phone number and is already associated with another pool, an error is returned.
* A sender ID can be associated with multiple pools.
*
*
* @param createPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreatePool
* @see AWS API Documentation
*/
java.util.concurrent.Future createPoolAsync(CreatePoolRequest createPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new protect configuration. By default all country rule sets for each capability are set to
* ALLOW
. Update the country rule sets using UpdateProtectConfigurationCountryRuleSet
. A
* protect configurations name is stored as a Tag with the key set to Name
and value as the name of the
* protect configuration.
*
*
* @param createProtectConfigurationRequest
* @return A Java Future containing the result of the CreateProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createProtectConfigurationAsync(
CreateProtectConfigurationRequest createProtectConfigurationRequest);
/**
*
* Create a new protect configuration. By default all country rule sets for each capability are set to
* ALLOW
. Update the country rule sets using UpdateProtectConfigurationCountryRuleSet
. A
* protect configurations name is stored as a Tag with the key set to Name
and value as the name of the
* protect configuration.
*
*
* @param createProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createProtectConfigurationAsync(
CreateProtectConfigurationRequest createProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new registration based on the RegistrationType field.
*
*
* @param createRegistrationRequest
* @return A Java Future containing the result of the CreateRegistration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAsync(CreateRegistrationRequest createRegistrationRequest);
/**
*
* Creates a new registration based on the RegistrationType field.
*
*
* @param createRegistrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRegistration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAsync(CreateRegistrationRequest createRegistrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate the registration with an origination identity such as a phone number or sender ID.
*
*
* @param createRegistrationAssociationRequest
* @return A Java Future containing the result of the CreateRegistrationAssociation operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateRegistrationAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAssociationAsync(
CreateRegistrationAssociationRequest createRegistrationAssociationRequest);
/**
*
* Associate the registration with an origination identity such as a phone number or sender ID.
*
*
* @param createRegistrationAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRegistrationAssociation operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateRegistrationAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAssociationAsync(
CreateRegistrationAssociationRequest createRegistrationAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new registration attachment to use for uploading a file or a URL to a file. The maximum file size is
* 1MiB and valid file extensions are PDF, JPEG and PNG. For example, many sender ID registrations require a signed
* “letter of authorization” (LOA) to be submitted.
*
*
* @param createRegistrationAttachmentRequest
* @return A Java Future containing the result of the CreateRegistrationAttachment operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateRegistrationAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAttachmentAsync(
CreateRegistrationAttachmentRequest createRegistrationAttachmentRequest);
/**
*
* Create a new registration attachment to use for uploading a file or a URL to a file. The maximum file size is
* 1MiB and valid file extensions are PDF, JPEG and PNG. For example, many sender ID registrations require a signed
* “letter of authorization” (LOA) to be submitted.
*
*
* @param createRegistrationAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRegistrationAttachment operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateRegistrationAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationAttachmentAsync(
CreateRegistrationAttachmentRequest createRegistrationAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a new version of the registration and increase the VersionNumber. The previous version of the
* registration becomes read-only.
*
*
* @param createRegistrationVersionRequest
* @return A Java Future containing the result of the CreateRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationVersionAsync(
CreateRegistrationVersionRequest createRegistrationVersionRequest);
/**
*
* Create a new version of the registration and increase the VersionNumber. The previous version of the
* registration becomes read-only.
*
*
* @param createRegistrationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createRegistrationVersionAsync(
CreateRegistrationVersionRequest createRegistrationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* You can only send messages to verified destination numbers when your account is in the sandbox. You can add up to
* 10 verified destination numbers.
*
*
* @param createVerifiedDestinationNumberRequest
* @return A Java Future containing the result of the CreateVerifiedDestinationNumber operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.CreateVerifiedDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future createVerifiedDestinationNumberAsync(
CreateVerifiedDestinationNumberRequest createVerifiedDestinationNumberRequest);
/**
*
* You can only send messages to verified destination numbers when your account is in the sandbox. You can add up to
* 10 verified destination numbers.
*
*
* @param createVerifiedDestinationNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVerifiedDestinationNumber operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.CreateVerifiedDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future createVerifiedDestinationNumberAsync(
CreateVerifiedDestinationNumberRequest createVerifiedDestinationNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the current account default protect configuration.
*
*
* @param deleteAccountDefaultProtectConfigurationRequest
* @return A Java Future containing the result of the DeleteAccountDefaultProtectConfiguration operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountDefaultProtectConfigurationAsync(
DeleteAccountDefaultProtectConfigurationRequest deleteAccountDefaultProtectConfigurationRequest);
/**
*
* Removes the current account default protect configuration.
*
*
* @param deleteAccountDefaultProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccountDefaultProtectConfiguration operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountDefaultProtectConfigurationAsync(
DeleteAccountDefaultProtectConfigurationRequest deleteAccountDefaultProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing configuration set.
*
*
* A configuration set is a set of rules that you apply to voice and SMS messages that you send. In a configuration
* set, you can specify a destination for specific types of events related to voice and SMS messages.
*
*
* @param deleteConfigurationSetRequest
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest);
/**
*
* Deletes an existing configuration set.
*
*
* A configuration set is a set of rules that you apply to voice and SMS messages that you send. In a configuration
* set, you can specify a destination for specific types of events related to voice and SMS messages.
*
*
* @param deleteConfigurationSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteConfigurationSet
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteConfigurationSetAsync(DeleteConfigurationSetRequest deleteConfigurationSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing default message type on a configuration set.
*
*
* A message type is a type of messages that you plan to send. If you send account-related messages or
* time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param deleteDefaultMessageTypeRequest
* @return A Java Future containing the result of the DeleteDefaultMessageType operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteDefaultMessageType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDefaultMessageTypeAsync(DeleteDefaultMessageTypeRequest deleteDefaultMessageTypeRequest);
/**
*
* Deletes an existing default message type on a configuration set.
*
*
* A message type is a type of messages that you plan to send. If you send account-related messages or
* time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param deleteDefaultMessageTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDefaultMessageType operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteDefaultMessageType
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDefaultMessageTypeAsync(DeleteDefaultMessageTypeRequest deleteDefaultMessageTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing default sender ID on a configuration set.
*
*
* A default sender ID is the identity that appears on recipients' devices when they receive SMS messages. Support
* for sender ID capabilities varies by country or region.
*
*
* @param deleteDefaultSenderIdRequest
* @return A Java Future containing the result of the DeleteDefaultSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteDefaultSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDefaultSenderIdAsync(DeleteDefaultSenderIdRequest deleteDefaultSenderIdRequest);
/**
*
* Deletes an existing default sender ID on a configuration set.
*
*
* A default sender ID is the identity that appears on recipients' devices when they receive SMS messages. Support
* for sender ID capabilities varies by country or region.
*
*
* @param deleteDefaultSenderIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDefaultSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteDefaultSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDefaultSenderIdAsync(DeleteDefaultSenderIdRequest deleteDefaultSenderIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing event destination.
*
*
* An event destination is a location where you send response information about the messages that you send. For
* example, when a message is delivered successfully, you can send information about that event to an Amazon
* CloudWatch destination, or send notifications to endpoints that are subscribed to an Amazon SNS topic.
*
*
* @param deleteEventDestinationRequest
* @return A Java Future containing the result of the DeleteEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventDestinationAsync(DeleteEventDestinationRequest deleteEventDestinationRequest);
/**
*
* Deletes an existing event destination.
*
*
* An event destination is a location where you send response information about the messages that you send. For
* example, when a message is delivered successfully, you can send information about that event to an Amazon
* CloudWatch destination, or send notifications to endpoints that are subscribed to an Amazon SNS topic.
*
*
* @param deleteEventDestinationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventDestinationAsync(DeleteEventDestinationRequest deleteEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing keyword from an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* Keywords "HELP" and "STOP" can't be deleted or modified.
*
*
* @param deleteKeywordRequest
* @return A Java Future containing the result of the DeleteKeyword operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteKeyword
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteKeywordAsync(DeleteKeywordRequest deleteKeywordRequest);
/**
*
* Deletes an existing keyword from an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* Keywords "HELP" and "STOP" can't be deleted or modified.
*
*
* @param deleteKeywordRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteKeyword operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteKeyword
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteKeywordAsync(DeleteKeywordRequest deleteKeywordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an account-level monthly spending limit override for sending multimedia messages (MMS). Deleting a spend
* limit override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas for Server Migration Service
* in the Server Migration Service User Guide.
*
*
* @param deleteMediaMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the DeleteMediaMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaMessageSpendLimitOverrideAsync(
DeleteMediaMessageSpendLimitOverrideRequest deleteMediaMessageSpendLimitOverrideRequest);
/**
*
* Deletes an account-level monthly spending limit override for sending multimedia messages (MMS). Deleting a spend
* limit override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas for Server Migration Service
* in the Server Migration Service User Guide.
*
*
* @param deleteMediaMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaMessageSpendLimitOverrideAsync(
DeleteMediaMessageSpendLimitOverrideRequest deleteMediaMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing opt-out list. All opted out phone numbers in the opt-out list are deleted.
*
*
* If the specified opt-out list name doesn't exist or is in-use by an origination phone number or pool, an error is
* returned.
*
*
* @param deleteOptOutListRequest
* @return A Java Future containing the result of the DeleteOptOutList operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteOptOutList
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOptOutListAsync(DeleteOptOutListRequest deleteOptOutListRequest);
/**
*
* Deletes an existing opt-out list. All opted out phone numbers in the opt-out list are deleted.
*
*
* If the specified opt-out list name doesn't exist or is in-use by an origination phone number or pool, an error is
* returned.
*
*
* @param deleteOptOutListRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOptOutList operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteOptOutList
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOptOutListAsync(DeleteOptOutListRequest deleteOptOutListRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing opted out destination phone number from the specified opt-out list.
*
*
* Each destination phone number can only be deleted once every 30 days.
*
*
* If the specified destination phone number doesn't exist or if the opt-out list doesn't exist, an error is
* returned.
*
*
* @param deleteOptedOutNumberRequest
* @return A Java Future containing the result of the DeleteOptedOutNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteOptedOutNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOptedOutNumberAsync(DeleteOptedOutNumberRequest deleteOptedOutNumberRequest);
/**
*
* Deletes an existing opted out destination phone number from the specified opt-out list.
*
*
* Each destination phone number can only be deleted once every 30 days.
*
*
* If the specified destination phone number doesn't exist or if the opt-out list doesn't exist, an error is
* returned.
*
*
* @param deleteOptedOutNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOptedOutNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteOptedOutNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteOptedOutNumberAsync(DeleteOptedOutNumberRequest deleteOptedOutNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing pool. Deleting a pool disassociates all origination identities from that pool.
*
*
* If the pool status isn't active or if deletion protection is enabled, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param deletePoolRequest
* @return A Java Future containing the result of the DeletePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeletePool
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePoolAsync(DeletePoolRequest deletePoolRequest);
/**
*
* Deletes an existing pool. Deleting a pool disassociates all origination identities from that pool.
*
*
* If the pool status isn't active or if deletion protection is enabled, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param deletePoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeletePool
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePoolAsync(DeletePoolRequest deletePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently delete the protect configuration. The protect configuration must have deletion protection disabled
* and must not be associated as the account default protect configuration or associated with a configuration set.
*
*
* @param deleteProtectConfigurationRequest
* @return A Java Future containing the result of the DeleteProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProtectConfigurationAsync(
DeleteProtectConfigurationRequest deleteProtectConfigurationRequest);
/**
*
* Permanently delete the protect configuration. The protect configuration must have deletion protection disabled
* and must not be associated as the account default protect configuration or associated with a configuration set.
*
*
* @param deleteProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteProtectConfigurationAsync(
DeleteProtectConfigurationRequest deleteProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently delete an existing registration from your account.
*
*
* @param deleteRegistrationRequest
* @return A Java Future containing the result of the DeleteRegistration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationAsync(DeleteRegistrationRequest deleteRegistrationRequest);
/**
*
* Permanently delete an existing registration from your account.
*
*
* @param deleteRegistrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRegistration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationAsync(DeleteRegistrationRequest deleteRegistrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently delete the specified registration attachment.
*
*
* @param deleteRegistrationAttachmentRequest
* @return A Java Future containing the result of the DeleteRegistrationAttachment operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteRegistrationAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationAttachmentAsync(
DeleteRegistrationAttachmentRequest deleteRegistrationAttachmentRequest);
/**
*
* Permanently delete the specified registration attachment.
*
*
* @param deleteRegistrationAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRegistrationAttachment operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteRegistrationAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationAttachmentAsync(
DeleteRegistrationAttachmentRequest deleteRegistrationAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete the value in a registration form field.
*
*
* @param deleteRegistrationFieldValueRequest
* @return A Java Future containing the result of the DeleteRegistrationFieldValue operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteRegistrationFieldValue
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationFieldValueAsync(
DeleteRegistrationFieldValueRequest deleteRegistrationFieldValueRequest);
/**
*
* Delete the value in a registration form field.
*
*
* @param deleteRegistrationFieldValueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRegistrationFieldValue operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteRegistrationFieldValue
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRegistrationFieldValueAsync(
DeleteRegistrationFieldValueRequest deleteRegistrationFieldValueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an account-level monthly spending limit override for sending text messages. Deleting a spend limit
* override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteTextMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the DeleteTextMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTextMessageSpendLimitOverrideAsync(
DeleteTextMessageSpendLimitOverrideRequest deleteTextMessageSpendLimitOverrideRequest);
/**
*
* Deletes an account-level monthly spending limit override for sending text messages. Deleting a spend limit
* override will set the EnforcedLimit
to equal the MaxLimit
, which is controlled by
* Amazon Web Services. For more information on spend limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteTextMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTextMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTextMessageSpendLimitOverrideAsync(
DeleteTextMessageSpendLimitOverrideRequest deleteTextMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a verified destination phone number.
*
*
* @param deleteVerifiedDestinationNumberRequest
* @return A Java Future containing the result of the DeleteVerifiedDestinationNumber operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteVerifiedDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVerifiedDestinationNumberAsync(
DeleteVerifiedDestinationNumberRequest deleteVerifiedDestinationNumberRequest);
/**
*
* Delete a verified destination phone number.
*
*
* @param deleteVerifiedDestinationNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVerifiedDestinationNumber operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteVerifiedDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVerifiedDestinationNumberAsync(
DeleteVerifiedDestinationNumberRequest deleteVerifiedDestinationNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an account level monthly spend limit override for sending voice messages. Deleting a spend limit override
* sets the EnforcedLimit
equal to the MaxLimit
, which is controlled by Amazon Web
* Services. For more information on spending limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteVoiceMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the DeleteVoiceMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DeleteVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVoiceMessageSpendLimitOverrideAsync(
DeleteVoiceMessageSpendLimitOverrideRequest deleteVoiceMessageSpendLimitOverrideRequest);
/**
*
* Deletes an account level monthly spend limit override for sending voice messages. Deleting a spend limit override
* sets the EnforcedLimit
equal to the MaxLimit
, which is controlled by Amazon Web
* Services. For more information on spending limits (quotas) see Quotas in the AWS End User
* Messaging SMS User Guide.
*
*
* @param deleteVoiceMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DeleteVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVoiceMessageSpendLimitOverrideAsync(
DeleteVoiceMessageSpendLimitOverrideRequest deleteVoiceMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes attributes of your Amazon Web Services account. The supported account attributes include account tier,
* which indicates whether your account is in the sandbox or production environment. When you're ready to move your
* account out of the sandbox, create an Amazon Web Services Support case for a service limit increase request.
*
*
* New accounts are placed into an SMS or voice sandbox. The sandbox protects both Amazon Web Services end
* recipients and SMS or voice recipients from fraud and abuse.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeAccountAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Describes attributes of your Amazon Web Services account. The supported account attributes include account tier,
* which indicates whether your account is in the sandbox or production environment. When you're ready to move your
* account out of the sandbox, create an Amazon Web Services Support case for a service limit increase request.
*
*
* New accounts are placed into an SMS or voice sandbox. The sandbox protects both Amazon Web Services end
* recipients and SMS or voice recipients from fraud and abuse.
*
*
* @param describeAccountAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeAccountAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the current AWS End User Messaging SMS and Voice SMS Voice V2 resource quotas for your account. The
* description for a quota includes the quota name, current usage toward that quota, and the quota's maximum value.
*
*
* When you establish an Amazon Web Services account, the account has initial quotas on the maximum number of
* configuration sets, opt-out lists, phone numbers, and pools that you can create in a given Region. For more
* information see Quotas in the
* AWS End User Messaging SMS User Guide.
*
*
* @param describeAccountLimitsRequest
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeAccountLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountLimitsAsync(DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Describes the current AWS End User Messaging SMS and Voice SMS Voice V2 resource quotas for your account. The
* description for a quota includes the quota name, current usage toward that quota, and the quota's maximum value.
*
*
* When you establish an Amazon Web Services account, the account has initial quotas on the maximum number of
* configuration sets, opt-out lists, phone numbers, and pools that you can create in a given Region. For more
* information see Quotas in the
* AWS End User Messaging SMS User Guide.
*
*
* @param describeAccountLimitsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeAccountLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountLimitsAsync(DescribeAccountLimitsRequest describeAccountLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified configuration sets or all in your account.
*
*
* If you specify configuration set names, the output includes information for only the specified configuration
* sets. If you specify filters, the output includes information for only those configuration sets that meet the
* filter criteria. If you don't specify configuration set names or filters, the output includes information for all
* configuration sets.
*
*
* If you specify a configuration set name that isn't valid, an error is returned.
*
*
* @param describeConfigurationSetsRequest
* @return A Java Future containing the result of the DescribeConfigurationSets operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeConfigurationSets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConfigurationSetsAsync(
DescribeConfigurationSetsRequest describeConfigurationSetsRequest);
/**
*
* Describes the specified configuration sets or all in your account.
*
*
* If you specify configuration set names, the output includes information for only the specified configuration
* sets. If you specify filters, the output includes information for only those configuration sets that meet the
* filter criteria. If you don't specify configuration set names or filters, the output includes information for all
* configuration sets.
*
*
* If you specify a configuration set name that isn't valid, an error is returned.
*
*
* @param describeConfigurationSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConfigurationSets operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeConfigurationSets
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConfigurationSetsAsync(
DescribeConfigurationSetsRequest describeConfigurationSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified keywords or all keywords on your origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param describeKeywordsRequest
* @return A Java Future containing the result of the DescribeKeywords operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeKeywords
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeywordsAsync(DescribeKeywordsRequest describeKeywordsRequest);
/**
*
* Describes the specified keywords or all keywords on your origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param describeKeywordsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeKeywords operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeKeywords
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeywordsAsync(DescribeKeywordsRequest describeKeywordsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified opt-out list or all opt-out lists in your account.
*
*
* If you specify opt-out list names, the output includes information for only the specified opt-out lists. Opt-out
* lists include only those that meet the filter criteria. If you don't specify opt-out list names or filters, the
* output includes information for all opt-out lists.
*
*
* If you specify an opt-out list name that isn't valid, an error is returned.
*
*
* @param describeOptOutListsRequest
* @return A Java Future containing the result of the DescribeOptOutLists operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeOptOutLists
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOptOutListsAsync(DescribeOptOutListsRequest describeOptOutListsRequest);
/**
*
* Describes the specified opt-out list or all opt-out lists in your account.
*
*
* If you specify opt-out list names, the output includes information for only the specified opt-out lists. Opt-out
* lists include only those that meet the filter criteria. If you don't specify opt-out list names or filters, the
* output includes information for all opt-out lists.
*
*
* If you specify an opt-out list name that isn't valid, an error is returned.
*
*
* @param describeOptOutListsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOptOutLists operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeOptOutLists
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOptOutListsAsync(DescribeOptOutListsRequest describeOptOutListsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified opted out destination numbers or all opted out destination numbers in an opt-out list.
*
*
* If you specify opted out numbers, the output includes information for only the specified opted out numbers. If
* you specify filters, the output includes information for only those opted out numbers that meet the filter
* criteria. If you don't specify opted out numbers or filters, the output includes information for all opted out
* destination numbers in your opt-out list.
*
*
* If you specify an opted out number that isn't valid, an error is returned.
*
*
* @param describeOptedOutNumbersRequest
* @return A Java Future containing the result of the DescribeOptedOutNumbers operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeOptedOutNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOptedOutNumbersAsync(DescribeOptedOutNumbersRequest describeOptedOutNumbersRequest);
/**
*
* Describes the specified opted out destination numbers or all opted out destination numbers in an opt-out list.
*
*
* If you specify opted out numbers, the output includes information for only the specified opted out numbers. If
* you specify filters, the output includes information for only those opted out numbers that meet the filter
* criteria. If you don't specify opted out numbers or filters, the output includes information for all opted out
* destination numbers in your opt-out list.
*
*
* If you specify an opted out number that isn't valid, an error is returned.
*
*
* @param describeOptedOutNumbersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOptedOutNumbers operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeOptedOutNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOptedOutNumbersAsync(DescribeOptedOutNumbersRequest describeOptedOutNumbersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified origination phone number, or all the phone numbers in your account.
*
*
* If you specify phone number IDs, the output includes information for only the specified phone numbers. If you
* specify filters, the output includes information for only those phone numbers that meet the filter criteria. If
* you don't specify phone number IDs or filters, the output includes information for all phone numbers.
*
*
* If you specify a phone number ID that isn't valid, an error is returned.
*
*
* @param describePhoneNumbersRequest
* @return A Java Future containing the result of the DescribePhoneNumbers operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribePhoneNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describePhoneNumbersAsync(DescribePhoneNumbersRequest describePhoneNumbersRequest);
/**
*
* Describes the specified origination phone number, or all the phone numbers in your account.
*
*
* If you specify phone number IDs, the output includes information for only the specified phone numbers. If you
* specify filters, the output includes information for only those phone numbers that meet the filter criteria. If
* you don't specify phone number IDs or filters, the output includes information for all phone numbers.
*
*
* If you specify a phone number ID that isn't valid, an error is returned.
*
*
* @param describePhoneNumbersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePhoneNumbers operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribePhoneNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describePhoneNumbersAsync(DescribePhoneNumbersRequest describePhoneNumbersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified pools or all pools associated with your Amazon Web Services account.
*
*
* If you specify pool IDs, the output includes information for only the specified pools. If you specify filters,
* the output includes information for only those pools that meet the filter criteria. If you don't specify pool IDs
* or filters, the output includes information for all pools.
*
*
* If you specify a pool ID that isn't valid, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param describePoolsRequest
* @return A Java Future containing the result of the DescribePools operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribePools
* @see AWS API Documentation
*/
java.util.concurrent.Future describePoolsAsync(DescribePoolsRequest describePoolsRequest);
/**
*
* Retrieves the specified pools or all pools associated with your Amazon Web Services account.
*
*
* If you specify pool IDs, the output includes information for only the specified pools. If you specify filters,
* the output includes information for only those pools that meet the filter criteria. If you don't specify pool IDs
* or filters, the output includes information for all pools.
*
*
* If you specify a pool ID that isn't valid, an error is returned.
*
*
* A pool is a collection of phone numbers and SenderIds. A pool can include one or more phone numbers and SenderIds
* that are associated with your Amazon Web Services account.
*
*
* @param describePoolsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePools operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribePools
* @see AWS API Documentation
*/
java.util.concurrent.Future describePoolsAsync(DescribePoolsRequest describePoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the protect configurations that match any of filters. If a filter isn’t provided then all protect
* configurations are returned.
*
*
* @param describeProtectConfigurationsRequest
* @return A Java Future containing the result of the DescribeProtectConfigurations operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeProtectConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProtectConfigurationsAsync(
DescribeProtectConfigurationsRequest describeProtectConfigurationsRequest);
/**
*
* Retrieves the protect configurations that match any of filters. If a filter isn’t provided then all protect
* configurations are returned.
*
*
* @param describeProtectConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeProtectConfigurations operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeProtectConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeProtectConfigurationsAsync(
DescribeProtectConfigurationsRequest describeProtectConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration attachments or all registration attachments associated with your Amazon Web
* Services account.
*
*
* @param describeRegistrationAttachmentsRequest
* @return A Java Future containing the result of the DescribeRegistrationAttachments operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationAttachments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationAttachmentsAsync(
DescribeRegistrationAttachmentsRequest describeRegistrationAttachmentsRequest);
/**
*
* Retrieves the specified registration attachments or all registration attachments associated with your Amazon Web
* Services account.
*
*
* @param describeRegistrationAttachmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationAttachments operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationAttachments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationAttachmentsAsync(
DescribeRegistrationAttachmentsRequest describeRegistrationAttachmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration type field definitions. You can use DescribeRegistrationFieldDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationFieldDefinitionsRequest
* @return A Java Future containing the result of the DescribeRegistrationFieldDefinitions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationFieldDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationFieldDefinitionsAsync(
DescribeRegistrationFieldDefinitionsRequest describeRegistrationFieldDefinitionsRequest);
/**
*
* Retrieves the specified registration type field definitions. You can use DescribeRegistrationFieldDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationFieldDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationFieldDefinitions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationFieldDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationFieldDefinitionsAsync(
DescribeRegistrationFieldDefinitionsRequest describeRegistrationFieldDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration field values.
*
*
* @param describeRegistrationFieldValuesRequest
* @return A Java Future containing the result of the DescribeRegistrationFieldValues operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationFieldValues
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationFieldValuesAsync(
DescribeRegistrationFieldValuesRequest describeRegistrationFieldValuesRequest);
/**
*
* Retrieves the specified registration field values.
*
*
* @param describeRegistrationFieldValuesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationFieldValues operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationFieldValues
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationFieldValuesAsync(
DescribeRegistrationFieldValuesRequest describeRegistrationFieldValuesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration section definitions. You can use DescribeRegistrationSectionDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationSectionDefinitionsRequest
* @return A Java Future containing the result of the DescribeRegistrationSectionDefinitions operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationSectionDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationSectionDefinitionsAsync(
DescribeRegistrationSectionDefinitionsRequest describeRegistrationSectionDefinitionsRequest);
/**
*
* Retrieves the specified registration section definitions. You can use DescribeRegistrationSectionDefinitions to
* view the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationSectionDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationSectionDefinitions operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationSectionDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationSectionDefinitionsAsync(
DescribeRegistrationSectionDefinitionsRequest describeRegistrationSectionDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration type definitions. You can use DescribeRegistrationTypeDefinitions to view
* the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationTypeDefinitionsRequest
* @return A Java Future containing the result of the DescribeRegistrationTypeDefinitions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationTypeDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationTypeDefinitionsAsync(
DescribeRegistrationTypeDefinitionsRequest describeRegistrationTypeDefinitionsRequest);
/**
*
* Retrieves the specified registration type definitions. You can use DescribeRegistrationTypeDefinitions to view
* the requirements for creating, filling out, and submitting each registration type.
*
*
* @param describeRegistrationTypeDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationTypeDefinitions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationTypeDefinitions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationTypeDefinitionsAsync(
DescribeRegistrationTypeDefinitionsRequest describeRegistrationTypeDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registration version.
*
*
* @param describeRegistrationVersionsRequest
* @return A Java Future containing the result of the DescribeRegistrationVersions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrationVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationVersionsAsync(
DescribeRegistrationVersionsRequest describeRegistrationVersionsRequest);
/**
*
* Retrieves the specified registration version.
*
*
* @param describeRegistrationVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrationVersions operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrationVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationVersionsAsync(
DescribeRegistrationVersionsRequest describeRegistrationVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified registrations.
*
*
* @param describeRegistrationsRequest
* @return A Java Future containing the result of the DescribeRegistrations operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeRegistrations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationsAsync(DescribeRegistrationsRequest describeRegistrationsRequest);
/**
*
* Retrieves the specified registrations.
*
*
* @param describeRegistrationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRegistrations operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeRegistrations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRegistrationsAsync(DescribeRegistrationsRequest describeRegistrationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified SenderIds or all SenderIds associated with your Amazon Web Services account.
*
*
* If you specify SenderIds, the output includes information for only the specified SenderIds. If you specify
* filters, the output includes information for only those SenderIds that meet the filter criteria. If you don't
* specify SenderIds or filters, the output includes information for all SenderIds.
*
*
* f you specify a sender ID that isn't valid, an error is returned.
*
*
* @param describeSenderIdsRequest
* @return A Java Future containing the result of the DescribeSenderIds operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeSenderIds
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSenderIdsAsync(DescribeSenderIdsRequest describeSenderIdsRequest);
/**
*
* Describes the specified SenderIds or all SenderIds associated with your Amazon Web Services account.
*
*
* If you specify SenderIds, the output includes information for only the specified SenderIds. If you specify
* filters, the output includes information for only those SenderIds that meet the filter criteria. If you don't
* specify SenderIds or filters, the output includes information for all SenderIds.
*
*
* f you specify a sender ID that isn't valid, an error is returned.
*
*
* @param describeSenderIdsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSenderIds operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeSenderIds
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSenderIdsAsync(DescribeSenderIdsRequest describeSenderIdsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the current monthly spend limits for sending voice and text messages.
*
*
* When you establish an Amazon Web Services account, the account has initial monthly spend limit in a given Region.
* For more information on increasing your monthly spend limit, see Requesting
* increases to your monthly SMS, MMS, or Voice spending quota in the AWS End User Messaging SMS User
* Guide.
*
*
* @param describeSpendLimitsRequest
* @return A Java Future containing the result of the DescribeSpendLimits operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeSpendLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSpendLimitsAsync(DescribeSpendLimitsRequest describeSpendLimitsRequest);
/**
*
* Describes the current monthly spend limits for sending voice and text messages.
*
*
* When you establish an Amazon Web Services account, the account has initial monthly spend limit in a given Region.
* For more information on increasing your monthly spend limit, see Requesting
* increases to your monthly SMS, MMS, or Voice spending quota in the AWS End User Messaging SMS User
* Guide.
*
*
* @param describeSpendLimitsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSpendLimits operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeSpendLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSpendLimitsAsync(DescribeSpendLimitsRequest describeSpendLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified verified destiona numbers.
*
*
* @param describeVerifiedDestinationNumbersRequest
* @return A Java Future containing the result of the DescribeVerifiedDestinationNumbers operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DescribeVerifiedDestinationNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVerifiedDestinationNumbersAsync(
DescribeVerifiedDestinationNumbersRequest describeVerifiedDestinationNumbersRequest);
/**
*
* Retrieves the specified verified destiona numbers.
*
*
* @param describeVerifiedDestinationNumbersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVerifiedDestinationNumbers operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DescribeVerifiedDestinationNumbers
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVerifiedDestinationNumbersAsync(
DescribeVerifiedDestinationNumbersRequest describeVerifiedDestinationNumbersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified origination identity from an existing pool.
*
*
* If the origination identity isn't associated with the specified pool, an error is returned.
*
*
* @param disassociateOriginationIdentityRequest
* @return A Java Future containing the result of the DisassociateOriginationIdentity operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DisassociateOriginationIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateOriginationIdentityAsync(
DisassociateOriginationIdentityRequest disassociateOriginationIdentityRequest);
/**
*
* Removes the specified origination identity from an existing pool.
*
*
* If the origination identity isn't associated with the specified pool, an error is returned.
*
*
* @param disassociateOriginationIdentityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateOriginationIdentity operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DisassociateOriginationIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateOriginationIdentityAsync(
DisassociateOriginationIdentityRequest disassociateOriginationIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociate a protect configuration from a configuration set.
*
*
* @param disassociateProtectConfigurationRequest
* @return A Java Future containing the result of the DisassociateProtectConfiguration operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.DisassociateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateProtectConfigurationAsync(
DisassociateProtectConfigurationRequest disassociateProtectConfigurationRequest);
/**
*
* Disassociate a protect configuration from a configuration set.
*
*
* @param disassociateProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateProtectConfiguration operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DisassociateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateProtectConfigurationAsync(
DisassociateProtectConfigurationRequest disassociateProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Discard the current version of the registration.
*
*
* @param discardRegistrationVersionRequest
* @return A Java Future containing the result of the DiscardRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.DiscardRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future discardRegistrationVersionAsync(
DiscardRegistrationVersionRequest discardRegistrationVersionRequest);
/**
*
* Discard the current version of the registration.
*
*
* @param discardRegistrationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DiscardRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.DiscardRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future discardRegistrationVersionAsync(
DiscardRegistrationVersionRequest discardRegistrationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieve the CountryRuleSet for the specified NumberCapability from a protect configuration.
*
*
* @param getProtectConfigurationCountryRuleSetRequest
* @return A Java Future containing the result of the GetProtectConfigurationCountryRuleSet operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.GetProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
java.util.concurrent.Future getProtectConfigurationCountryRuleSetAsync(
GetProtectConfigurationCountryRuleSetRequest getProtectConfigurationCountryRuleSetRequest);
/**
*
* Retrieve the CountryRuleSet for the specified NumberCapability from a protect configuration.
*
*
* @param getProtectConfigurationCountryRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetProtectConfigurationCountryRuleSet operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.GetProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
java.util.concurrent.Future getProtectConfigurationCountryRuleSetAsync(
GetProtectConfigurationCountryRuleSetRequest getProtectConfigurationCountryRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all associated origination identities in your pool.
*
*
* If you specify filters, the output includes information for only those origination identities that meet the
* filter criteria.
*
*
* @param listPoolOriginationIdentitiesRequest
* @return A Java Future containing the result of the ListPoolOriginationIdentities operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.ListPoolOriginationIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listPoolOriginationIdentitiesAsync(
ListPoolOriginationIdentitiesRequest listPoolOriginationIdentitiesRequest);
/**
*
* Lists all associated origination identities in your pool.
*
*
* If you specify filters, the output includes information for only those origination identities that meet the
* filter criteria.
*
*
* @param listPoolOriginationIdentitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPoolOriginationIdentities operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.ListPoolOriginationIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listPoolOriginationIdentitiesAsync(
ListPoolOriginationIdentitiesRequest listPoolOriginationIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retreive all of the origination identies that are associated with a registration.
*
*
* @param listRegistrationAssociationsRequest
* @return A Java Future containing the result of the ListRegistrationAssociations operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.ListRegistrationAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listRegistrationAssociationsAsync(
ListRegistrationAssociationsRequest listRegistrationAssociationsRequest);
/**
*
* Retreive all of the origination identies that are associated with a registration.
*
*
* @param listRegistrationAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRegistrationAssociations operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.ListRegistrationAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listRegistrationAssociationsAsync(
ListRegistrationAssociationsRequest listRegistrationAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* List all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a keyword configuration on an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param putKeywordRequest
* @return A Java Future containing the result of the PutKeyword operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.PutKeyword
* @see AWS API Documentation
*/
java.util.concurrent.Future putKeywordAsync(PutKeywordRequest putKeywordRequest);
/**
*
* Creates or updates a keyword configuration on an origination phone number or pool.
*
*
* A keyword is a word that you can search for on a particular phone number or pool. It is also a specific word or
* phrase that an end user can send to your number to elicit a response, such as an informational message or a
* special offer. When your number receives a message that begins with a keyword, AWS End User Messaging SMS and
* Voice responds with a customizable message.
*
*
* If you specify a keyword that isn't valid, an error is returned.
*
*
* @param putKeywordRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutKeyword operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.PutKeyword
* @see AWS API Documentation
*/
java.util.concurrent.Future putKeywordAsync(PutKeywordRequest putKeywordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an opted out destination phone number in the opt-out list.
*
*
* If the destination phone number isn't valid or if the specified opt-out list doesn't exist, an error is returned.
*
*
* @param putOptedOutNumberRequest
* @return A Java Future containing the result of the PutOptedOutNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.PutOptedOutNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future putOptedOutNumberAsync(PutOptedOutNumberRequest putOptedOutNumberRequest);
/**
*
* Creates an opted out destination phone number in the opt-out list.
*
*
* If the destination phone number isn't valid or if the specified opt-out list doesn't exist, an error is returned.
*
*
* @param putOptedOutNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutOptedOutNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.PutOptedOutNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future putOptedOutNumberAsync(PutOptedOutNumberRequest putOptedOutNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a field value for a registration.
*
*
* @param putRegistrationFieldValueRequest
* @return A Java Future containing the result of the PutRegistrationFieldValue operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.PutRegistrationFieldValue
* @see AWS API Documentation
*/
java.util.concurrent.Future putRegistrationFieldValueAsync(
PutRegistrationFieldValueRequest putRegistrationFieldValueRequest);
/**
*
* Creates or updates a field value for a registration.
*
*
* @param putRegistrationFieldValueRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutRegistrationFieldValue operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.PutRegistrationFieldValue
* @see AWS API Documentation
*/
java.util.concurrent.Future putRegistrationFieldValueAsync(
PutRegistrationFieldValueRequest putRegistrationFieldValueRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Releases an existing origination phone number in your account. Once released, a phone number is no longer
* available for sending messages.
*
*
* If the origination phone number has deletion protection enabled or is associated with a pool, an error is
* returned.
*
*
* @param releasePhoneNumberRequest
* @return A Java Future containing the result of the ReleasePhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.ReleasePhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future releasePhoneNumberAsync(ReleasePhoneNumberRequest releasePhoneNumberRequest);
/**
*
* Releases an existing origination phone number in your account. Once released, a phone number is no longer
* available for sending messages.
*
*
* If the origination phone number has deletion protection enabled or is associated with a pool, an error is
* returned.
*
*
* @param releasePhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReleasePhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.ReleasePhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future releasePhoneNumberAsync(ReleasePhoneNumberRequest releasePhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Releases an existing sender ID in your account.
*
*
* @param releaseSenderIdRequest
* @return A Java Future containing the result of the ReleaseSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.ReleaseSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future releaseSenderIdAsync(ReleaseSenderIdRequest releaseSenderIdRequest);
/**
*
* Releases an existing sender ID in your account.
*
*
* @param releaseSenderIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReleaseSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.ReleaseSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future releaseSenderIdAsync(ReleaseSenderIdRequest releaseSenderIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Request an origination phone number for use in your account. For more information on phone number request see Request a phone
* number in the AWS End User Messaging SMS User Guide.
*
*
* @param requestPhoneNumberRequest
* @return A Java Future containing the result of the RequestPhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.RequestPhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future requestPhoneNumberAsync(RequestPhoneNumberRequest requestPhoneNumberRequest);
/**
*
* Request an origination phone number for use in your account. For more information on phone number request see Request a phone
* number in the AWS End User Messaging SMS User Guide.
*
*
* @param requestPhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RequestPhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.RequestPhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future requestPhoneNumberAsync(RequestPhoneNumberRequest requestPhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Request a new sender ID that doesn't require registration.
*
*
* @param requestSenderIdRequest
* @return A Java Future containing the result of the RequestSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.RequestSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future requestSenderIdAsync(RequestSenderIdRequest requestSenderIdRequest);
/**
*
* Request a new sender ID that doesn't require registration.
*
*
* @param requestSenderIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RequestSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.RequestSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future requestSenderIdAsync(RequestSenderIdRequest requestSenderIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Before you can send test messages to a verified destination phone number you need to opt-in the verified
* destination phone number. Creates a new text message with a verification code and send it to a verified
* destination phone number. Once you have the verification code use VerifyDestinationNumber to opt-in the
* verified destination phone number to receive messages.
*
*
* @param sendDestinationNumberVerificationCodeRequest
* @return A Java Future containing the result of the SendDestinationNumberVerificationCode operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.SendDestinationNumberVerificationCode
* @see AWS API Documentation
*/
java.util.concurrent.Future sendDestinationNumberVerificationCodeAsync(
SendDestinationNumberVerificationCodeRequest sendDestinationNumberVerificationCodeRequest);
/**
*
* Before you can send test messages to a verified destination phone number you need to opt-in the verified
* destination phone number. Creates a new text message with a verification code and send it to a verified
* destination phone number. Once you have the verification code use VerifyDestinationNumber to opt-in the
* verified destination phone number to receive messages.
*
*
* @param sendDestinationNumberVerificationCodeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendDestinationNumberVerificationCode operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SendDestinationNumberVerificationCode
* @see AWS API Documentation
*/
java.util.concurrent.Future sendDestinationNumberVerificationCodeAsync(
SendDestinationNumberVerificationCodeRequest sendDestinationNumberVerificationCodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new multimedia message (MMS) and sends it to a recipient's phone number.
*
*
* @param sendMediaMessageRequest
* @return A Java Future containing the result of the SendMediaMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SendMediaMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendMediaMessageAsync(SendMediaMessageRequest sendMediaMessageRequest);
/**
*
* Creates a new multimedia message (MMS) and sends it to a recipient's phone number.
*
*
* @param sendMediaMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendMediaMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SendMediaMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendMediaMessageAsync(SendMediaMessageRequest sendMediaMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new text message and sends it to a recipient's phone number. SendTextMessage only sends an SMS message
* to one recipient each time it is invoked.
*
*
* SMS throughput limits are measured in Message Parts per Second (MPS). Your MPS limit depends on the destination
* country of your messages, as well as the type of phone number (origination number) that you use to send the
* message. For more information about MPS, see Message Parts per Second
* (MPS) limits in the AWS End User Messaging SMS User Guide.
*
*
* @param sendTextMessageRequest
* @return A Java Future containing the result of the SendTextMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SendTextMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendTextMessageAsync(SendTextMessageRequest sendTextMessageRequest);
/**
*
* Creates a new text message and sends it to a recipient's phone number. SendTextMessage only sends an SMS message
* to one recipient each time it is invoked.
*
*
* SMS throughput limits are measured in Message Parts per Second (MPS). Your MPS limit depends on the destination
* country of your messages, as well as the type of phone number (origination number) that you use to send the
* message. For more information about MPS, see Message Parts per Second
* (MPS) limits in the AWS End User Messaging SMS User Guide.
*
*
* @param sendTextMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendTextMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SendTextMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendTextMessageAsync(SendTextMessageRequest sendTextMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows you to send a request that sends a voice message. This operation uses Amazon Polly to convert a text script into a voice message.
*
*
* @param sendVoiceMessageRequest
* @return A Java Future containing the result of the SendVoiceMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SendVoiceMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendVoiceMessageAsync(SendVoiceMessageRequest sendVoiceMessageRequest);
/**
*
* Allows you to send a request that sends a voice message. This operation uses Amazon Polly to convert a text script into a voice message.
*
*
* @param sendVoiceMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SendVoiceMessage operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SendVoiceMessage
* @see AWS API Documentation
*/
java.util.concurrent.Future sendVoiceMessageAsync(SendVoiceMessageRequest sendVoiceMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Set a protect configuration as your account default. You can only have one account default protect configuration
* at a time. The current account default protect configuration is replaced with the provided protect configuration.
*
*
* @param setAccountDefaultProtectConfigurationRequest
* @return A Java Future containing the result of the SetAccountDefaultProtectConfiguration operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.SetAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future setAccountDefaultProtectConfigurationAsync(
SetAccountDefaultProtectConfigurationRequest setAccountDefaultProtectConfigurationRequest);
/**
*
* Set a protect configuration as your account default. You can only have one account default protect configuration
* at a time. The current account default protect configuration is replaced with the provided protect configuration.
*
*
* @param setAccountDefaultProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetAccountDefaultProtectConfiguration operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetAccountDefaultProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future setAccountDefaultProtectConfigurationAsync(
SetAccountDefaultProtectConfigurationRequest setAccountDefaultProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the default message type on a configuration set.
*
*
* Choose the category of SMS messages that you plan to send from this account. If you send account-related messages
* or time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param setDefaultMessageTypeRequest
* @return A Java Future containing the result of the SetDefaultMessageType operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SetDefaultMessageType
* @see AWS API Documentation
*/
java.util.concurrent.Future setDefaultMessageTypeAsync(SetDefaultMessageTypeRequest setDefaultMessageTypeRequest);
/**
*
* Sets the default message type on a configuration set.
*
*
* Choose the category of SMS messages that you plan to send from this account. If you send account-related messages
* or time-sensitive messages such as one-time passcodes, choose Transactional. If you plan to send messages
* that contain marketing material or other promotional content, choose Promotional. This setting applies to
* your entire Amazon Web Services account.
*
*
* @param setDefaultMessageTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetDefaultMessageType operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetDefaultMessageType
* @see AWS API Documentation
*/
java.util.concurrent.Future setDefaultMessageTypeAsync(SetDefaultMessageTypeRequest setDefaultMessageTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets default sender ID on a configuration set.
*
*
* When sending a text message to a destination country that supports sender IDs, the default sender ID on the
* configuration set specified will be used if no dedicated origination phone numbers or registered sender IDs are
* available in your account.
*
*
* @param setDefaultSenderIdRequest
* @return A Java Future containing the result of the SetDefaultSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SetDefaultSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future setDefaultSenderIdAsync(SetDefaultSenderIdRequest setDefaultSenderIdRequest);
/**
*
* Sets default sender ID on a configuration set.
*
*
* When sending a text message to a destination country that supports sender IDs, the default sender ID on the
* configuration set specified will be used if no dedicated origination phone numbers or registered sender IDs are
* available in your account.
*
*
* @param setDefaultSenderIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetDefaultSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetDefaultSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future setDefaultSenderIdAsync(SetDefaultSenderIdRequest setDefaultSenderIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets an account level monthly spend limit override for sending MMS messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setMediaMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the SetMediaMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.SetMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setMediaMessageSpendLimitOverrideAsync(
SetMediaMessageSpendLimitOverrideRequest setMediaMessageSpendLimitOverrideRequest);
/**
*
* Sets an account level monthly spend limit override for sending MMS messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setMediaMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetMediaMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetMediaMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setMediaMessageSpendLimitOverrideAsync(
SetMediaMessageSpendLimitOverrideRequest setMediaMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets an account level monthly spend limit override for sending text messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setTextMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the SetTextMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.SetTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setTextMessageSpendLimitOverrideAsync(
SetTextMessageSpendLimitOverrideRequest setTextMessageSpendLimitOverrideRequest);
/**
*
* Sets an account level monthly spend limit override for sending text messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setTextMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetTextMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetTextMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setTextMessageSpendLimitOverrideAsync(
SetTextMessageSpendLimitOverrideRequest setTextMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets an account level monthly spend limit override for sending voice messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setVoiceMessageSpendLimitOverrideRequest
* @return A Java Future containing the result of the SetVoiceMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2Async.SetVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setVoiceMessageSpendLimitOverrideAsync(
SetVoiceMessageSpendLimitOverrideRequest setVoiceMessageSpendLimitOverrideRequest);
/**
*
* Sets an account level monthly spend limit override for sending voice messages. The requested spend limit must be
* less than or equal to the MaxLimit
, which is set by Amazon Web Services.
*
*
* @param setVoiceMessageSpendLimitOverrideRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetVoiceMessageSpendLimitOverride operation returned by the
* service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SetVoiceMessageSpendLimitOverride
* @see AWS API Documentation
*/
java.util.concurrent.Future setVoiceMessageSpendLimitOverrideAsync(
SetVoiceMessageSpendLimitOverrideRequest setVoiceMessageSpendLimitOverrideRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Submit the specified registration for review and approval.
*
*
* @param submitRegistrationVersionRequest
* @return A Java Future containing the result of the SubmitRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.SubmitRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future submitRegistrationVersionAsync(
SubmitRegistrationVersionRequest submitRegistrationVersionRequest);
/**
*
* Submit the specified registration for review and approval.
*
*
* @param submitRegistrationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SubmitRegistrationVersion operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.SubmitRegistrationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future submitRegistrationVersionAsync(
SubmitRegistrationVersionRequest submitRegistrationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites only the specified tags for the specified resource. When you specify an existing tag key, the
* value is overwritten with the new value. Each resource can have a maximum of 50 tags. Each tag consists of a key
* and an optional value. Tag keys must be unique per resource. For more information about tags, see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or overwrites only the specified tags for the specified resource. When you specify an existing tag key, the
* value is overwritten with the new value. Each resource can have a maximum of 50 tags. Each tag consists of a key
* and an optional value. Tag keys must be unique per resource. For more information about tags, see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the association of the specified tags from a resource. For more information on tags see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the association of the specified tags from a resource. For more information on tags see Tags in the AWS End
* User Messaging SMS User Guide.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing event destination in a configuration set. You can update the IAM role ARN for CloudWatch Logs
* and Firehose. You can also enable or disable the event destination.
*
*
* You may want to update an event destination to change its matching event types or updating the destination
* resource ARN. You can't change an event destination's type between CloudWatch Logs, Firehose, and Amazon SNS.
*
*
* @param updateEventDestinationRequest
* @return A Java Future containing the result of the UpdateEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdateEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEventDestinationAsync(UpdateEventDestinationRequest updateEventDestinationRequest);
/**
*
* Updates an existing event destination in a configuration set. You can update the IAM role ARN for CloudWatch Logs
* and Firehose. You can also enable or disable the event destination.
*
*
* You may want to update an event destination to change its matching event types or updating the destination
* resource ARN. You can't change an event destination's type between CloudWatch Logs, Firehose, and Amazon SNS.
*
*
* @param updateEventDestinationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEventDestination operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdateEventDestination
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEventDestinationAsync(UpdateEventDestinationRequest updateEventDestinationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing origination phone number. You can update the opt-out list, enable or
* disable two-way messaging, change the TwoWayChannelArn, enable or disable self-managed opt-outs, and enable or
* disable deletion protection.
*
*
* If the origination phone number is associated with a pool, an error is returned.
*
*
* @param updatePhoneNumberRequest
* @return A Java Future containing the result of the UpdatePhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdatePhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePhoneNumberAsync(UpdatePhoneNumberRequest updatePhoneNumberRequest);
/**
*
* Updates the configuration of an existing origination phone number. You can update the opt-out list, enable or
* disable two-way messaging, change the TwoWayChannelArn, enable or disable self-managed opt-outs, and enable or
* disable deletion protection.
*
*
* If the origination phone number is associated with a pool, an error is returned.
*
*
* @param updatePhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePhoneNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdatePhoneNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePhoneNumberAsync(UpdatePhoneNumberRequest updatePhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing pool. You can update the opt-out list, enable or disable two-way
* messaging, change the TwoWayChannelArn
, enable or disable self-managed opt-outs, enable or disable
* deletion protection, and enable or disable shared routes.
*
*
* @param updatePoolRequest
* @return A Java Future containing the result of the UpdatePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdatePool
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePoolAsync(UpdatePoolRequest updatePoolRequest);
/**
*
* Updates the configuration of an existing pool. You can update the opt-out list, enable or disable two-way
* messaging, change the TwoWayChannelArn
, enable or disable self-managed opt-outs, enable or disable
* deletion protection, and enable or disable shared routes.
*
*
* @param updatePoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePool operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdatePool
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePoolAsync(UpdatePoolRequest updatePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update the setting for an existing protect configuration.
*
*
* @param updateProtectConfigurationRequest
* @return A Java Future containing the result of the UpdateProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProtectConfigurationAsync(
UpdateProtectConfigurationRequest updateProtectConfigurationRequest);
/**
*
* Update the setting for an existing protect configuration.
*
*
* @param updateProtectConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProtectConfiguration operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdateProtectConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProtectConfigurationAsync(
UpdateProtectConfigurationRequest updateProtectConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update a country rule set to ALLOW
or BLOCK
messages to be sent to the specified
* destination counties. You can update one or multiple countries at a time. The updates are only applied to the
* specified NumberCapability type.
*
*
* @param updateProtectConfigurationCountryRuleSetRequest
* @return A Java Future containing the result of the UpdateProtectConfigurationCountryRuleSet operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdateProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProtectConfigurationCountryRuleSetAsync(
UpdateProtectConfigurationCountryRuleSetRequest updateProtectConfigurationCountryRuleSetRequest);
/**
*
* Update a country rule set to ALLOW
or BLOCK
messages to be sent to the specified
* destination counties. You can update one or multiple countries at a time. The updates are only applied to the
* specified NumberCapability type.
*
*
* @param updateProtectConfigurationCountryRuleSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateProtectConfigurationCountryRuleSet operation returned by
* the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdateProtectConfigurationCountryRuleSet
* @see AWS API Documentation
*/
java.util.concurrent.Future updateProtectConfigurationCountryRuleSetAsync(
UpdateProtectConfigurationCountryRuleSetRequest updateProtectConfigurationCountryRuleSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration of an existing sender ID.
*
*
* @param updateSenderIdRequest
* @return A Java Future containing the result of the UpdateSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.UpdateSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSenderIdAsync(UpdateSenderIdRequest updateSenderIdRequest);
/**
*
* Updates the configuration of an existing sender ID.
*
*
* @param updateSenderIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSenderId operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.UpdateSenderId
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSenderIdAsync(UpdateSenderIdRequest updateSenderIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the verification code that was received by the verified destination phone number to opt-in the verified
* destination phone number to receive more messages.
*
*
* @param verifyDestinationNumberRequest
* @return A Java Future containing the result of the VerifyDestinationNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2Async.VerifyDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future verifyDestinationNumberAsync(VerifyDestinationNumberRequest verifyDestinationNumberRequest);
/**
*
* Use the verification code that was received by the verified destination phone number to opt-in the verified
* destination phone number to receive more messages.
*
*
* @param verifyDestinationNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the VerifyDestinationNumber operation returned by the service.
* @sample AmazonPinpointSMSVoiceV2AsyncHandler.VerifyDestinationNumber
* @see AWS API Documentation
*/
java.util.concurrent.Future verifyDestinationNumberAsync(VerifyDestinationNumberRequest verifyDestinationNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}