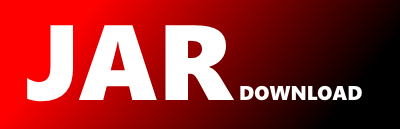
com.amazonaws.services.pinpointsmsvoicev2.model.CreateRegistrationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateRegistrationResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*/
private String registrationArn;
/**
*
* The unique identifier for the registration.
*
*/
private String registrationId;
/**
*
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*/
private String registrationType;
/**
*
* The status of the registration.
*
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the registration for
* the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
*
*/
private String registrationStatus;
/**
*
* The current version number of the registration.
*
*/
private Long currentVersionNumber;
/**
*
* Metadata about a given registration which is specific to that registration type.
*
*/
private java.util.Map additionalAttributes;
/**
*
* An array of tags (key and value pairs) to associate with the registration.
*
*/
private java.util.List tags;
/**
*
* The time when the registration was created, in UNIX epoch time
* format.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
*/
public void setRegistrationArn(String registrationArn) {
this.registrationArn = registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @return The Amazon Resource Name (ARN) for the registration.
*/
public String getRegistrationArn() {
return this.registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withRegistrationArn(String registrationArn) {
setRegistrationArn(registrationArn);
return this;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
*/
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @return The unique identifier for the registration.
*/
public String getRegistrationId() {
return this.registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withRegistrationId(String registrationId) {
setRegistrationId(registrationId);
return this;
}
/**
*
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public void setRegistrationType(String registrationType) {
this.registrationType = registrationType;
}
/**
*
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @return The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public String getRegistrationType() {
return this.registrationType;
}
/**
*
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form to create. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withRegistrationType(String registrationType) {
setRegistrationType(registrationType);
return this;
}
/**
*
* The status of the registration.
*
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the registration for
* the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
*
*
* @param registrationStatus
* The status of the registration.
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being
* created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been
* created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the
* registration for the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
* @see RegistrationStatus
*/
public void setRegistrationStatus(String registrationStatus) {
this.registrationStatus = registrationStatus;
}
/**
*
* The status of the registration.
*
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the registration for
* the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
*
*
* @return The status of the registration.
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being
* created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been
* created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the
* registration for the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
* @see RegistrationStatus
*/
public String getRegistrationStatus() {
return this.registrationStatus;
}
/**
*
* The status of the registration.
*
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the registration for
* the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
*
*
* @param registrationStatus
* The status of the registration.
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being
* created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been
* created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the
* registration for the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RegistrationStatus
*/
public CreateRegistrationResult withRegistrationStatus(String registrationStatus) {
setRegistrationStatus(registrationStatus);
return this;
}
/**
*
* The status of the registration.
*
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the registration for
* the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
*
*
* @param registrationStatus
* The status of the registration.
*
* -
*
* CREATED
: Your registration is created but not submitted.
*
*
* -
*
* SUBMITTED
: Your registration has been submitted and is awaiting review.
*
*
* -
*
* REVIEWING
: Your registration has been accepted and is being reviewed.
*
*
* -
*
* PROVISIONING
: Your registration has been approved and your origination identity is being
* created.
*
*
* -
*
* COMPLETE
: Your registration has been approved and and your origination identity has been
* created.
*
*
* -
*
* REQUIRES_UPDATES
: You must fix your registration and resubmit it.
*
*
* -
*
* CLOSED
: The phone number or sender ID has been deleted and you must also delete the
* registration for the number.
*
*
* -
*
* DELETED
: The registration has been deleted.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RegistrationStatus
*/
public CreateRegistrationResult withRegistrationStatus(RegistrationStatus registrationStatus) {
this.registrationStatus = registrationStatus.toString();
return this;
}
/**
*
* The current version number of the registration.
*
*
* @param currentVersionNumber
* The current version number of the registration.
*/
public void setCurrentVersionNumber(Long currentVersionNumber) {
this.currentVersionNumber = currentVersionNumber;
}
/**
*
* The current version number of the registration.
*
*
* @return The current version number of the registration.
*/
public Long getCurrentVersionNumber() {
return this.currentVersionNumber;
}
/**
*
* The current version number of the registration.
*
*
* @param currentVersionNumber
* The current version number of the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withCurrentVersionNumber(Long currentVersionNumber) {
setCurrentVersionNumber(currentVersionNumber);
return this;
}
/**
*
* Metadata about a given registration which is specific to that registration type.
*
*
* @return Metadata about a given registration which is specific to that registration type.
*/
public java.util.Map getAdditionalAttributes() {
return additionalAttributes;
}
/**
*
* Metadata about a given registration which is specific to that registration type.
*
*
* @param additionalAttributes
* Metadata about a given registration which is specific to that registration type.
*/
public void setAdditionalAttributes(java.util.Map additionalAttributes) {
this.additionalAttributes = additionalAttributes;
}
/**
*
* Metadata about a given registration which is specific to that registration type.
*
*
* @param additionalAttributes
* Metadata about a given registration which is specific to that registration type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withAdditionalAttributes(java.util.Map additionalAttributes) {
setAdditionalAttributes(additionalAttributes);
return this;
}
/**
* Add a single AdditionalAttributes entry
*
* @see CreateRegistrationResult#withAdditionalAttributes
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult addAdditionalAttributesEntry(String key, String value) {
if (null == this.additionalAttributes) {
this.additionalAttributes = new java.util.HashMap();
}
if (this.additionalAttributes.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.additionalAttributes.put(key, value);
return this;
}
/**
* Removes all the entries added into AdditionalAttributes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult clearAdditionalAttributesEntries() {
this.additionalAttributes = null;
return this;
}
/**
*
* An array of tags (key and value pairs) to associate with the registration.
*
*
* @return An array of tags (key and value pairs) to associate with the registration.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of tags (key and value pairs) to associate with the registration.
*
*
* @param tags
* An array of tags (key and value pairs) to associate with the registration.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of tags (key and value pairs) to associate with the registration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of tags (key and value pairs) to associate with the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of tags (key and value pairs) to associate with the registration.
*
*
* @param tags
* An array of tags (key and value pairs) to associate with the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The time when the registration was created, in UNIX epoch time
* format.
*
*
* @param createdTimestamp
* The time when the registration was created, in UNIX epoch
* time format.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The time when the registration was created, in UNIX epoch time
* format.
*
*
* @return The time when the registration was created, in UNIX epoch
* time format.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The time when the registration was created, in UNIX epoch time
* format.
*
*
* @param createdTimestamp
* The time when the registration was created, in UNIX epoch
* time format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateRegistrationResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegistrationArn() != null)
sb.append("RegistrationArn: ").append(getRegistrationArn()).append(",");
if (getRegistrationId() != null)
sb.append("RegistrationId: ").append(getRegistrationId()).append(",");
if (getRegistrationType() != null)
sb.append("RegistrationType: ").append(getRegistrationType()).append(",");
if (getRegistrationStatus() != null)
sb.append("RegistrationStatus: ").append(getRegistrationStatus()).append(",");
if (getCurrentVersionNumber() != null)
sb.append("CurrentVersionNumber: ").append(getCurrentVersionNumber()).append(",");
if (getAdditionalAttributes() != null)
sb.append("AdditionalAttributes: ").append(getAdditionalAttributes()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateRegistrationResult == false)
return false;
CreateRegistrationResult other = (CreateRegistrationResult) obj;
if (other.getRegistrationArn() == null ^ this.getRegistrationArn() == null)
return false;
if (other.getRegistrationArn() != null && other.getRegistrationArn().equals(this.getRegistrationArn()) == false)
return false;
if (other.getRegistrationId() == null ^ this.getRegistrationId() == null)
return false;
if (other.getRegistrationId() != null && other.getRegistrationId().equals(this.getRegistrationId()) == false)
return false;
if (other.getRegistrationType() == null ^ this.getRegistrationType() == null)
return false;
if (other.getRegistrationType() != null && other.getRegistrationType().equals(this.getRegistrationType()) == false)
return false;
if (other.getRegistrationStatus() == null ^ this.getRegistrationStatus() == null)
return false;
if (other.getRegistrationStatus() != null && other.getRegistrationStatus().equals(this.getRegistrationStatus()) == false)
return false;
if (other.getCurrentVersionNumber() == null ^ this.getCurrentVersionNumber() == null)
return false;
if (other.getCurrentVersionNumber() != null && other.getCurrentVersionNumber().equals(this.getCurrentVersionNumber()) == false)
return false;
if (other.getAdditionalAttributes() == null ^ this.getAdditionalAttributes() == null)
return false;
if (other.getAdditionalAttributes() != null && other.getAdditionalAttributes().equals(this.getAdditionalAttributes()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegistrationArn() == null) ? 0 : getRegistrationArn().hashCode());
hashCode = prime * hashCode + ((getRegistrationId() == null) ? 0 : getRegistrationId().hashCode());
hashCode = prime * hashCode + ((getRegistrationType() == null) ? 0 : getRegistrationType().hashCode());
hashCode = prime * hashCode + ((getRegistrationStatus() == null) ? 0 : getRegistrationStatus().hashCode());
hashCode = prime * hashCode + ((getCurrentVersionNumber() == null) ? 0 : getCurrentVersionNumber().hashCode());
hashCode = prime * hashCode + ((getAdditionalAttributes() == null) ? 0 : getAdditionalAttributes().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
return hashCode;
}
@Override
public CreateRegistrationResult clone() {
try {
return (CreateRegistrationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}