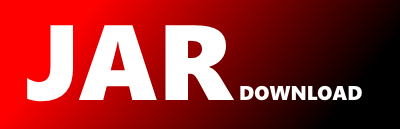
com.amazonaws.services.pinpointsmsvoicev2.model.DeleteRegistrationFieldValueResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DeleteRegistrationFieldValueResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*/
private String registrationArn;
/**
*
* The unique identifier for the registration.
*
*/
private String registrationId;
/**
*
* The version number of the registration.
*
*/
private Long versionNumber;
/**
*
* The path to the registration form field.
*
*/
private String fieldPath;
/**
*
* An array of values for the form field.
*
*/
private java.util.List selectChoices;
/**
*
* The text data for a free form field.
*
*/
private String textValue;
/**
*
* The unique identifier for the registration attachment.
*
*/
private String registrationAttachmentId;
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
*/
public void setRegistrationArn(String registrationArn) {
this.registrationArn = registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @return The Amazon Resource Name (ARN) for the registration.
*/
public String getRegistrationArn() {
return this.registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withRegistrationArn(String registrationArn) {
setRegistrationArn(registrationArn);
return this;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
*/
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @return The unique identifier for the registration.
*/
public String getRegistrationId() {
return this.registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withRegistrationId(String registrationId) {
setRegistrationId(registrationId);
return this;
}
/**
*
* The version number of the registration.
*
*
* @param versionNumber
* The version number of the registration.
*/
public void setVersionNumber(Long versionNumber) {
this.versionNumber = versionNumber;
}
/**
*
* The version number of the registration.
*
*
* @return The version number of the registration.
*/
public Long getVersionNumber() {
return this.versionNumber;
}
/**
*
* The version number of the registration.
*
*
* @param versionNumber
* The version number of the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withVersionNumber(Long versionNumber) {
setVersionNumber(versionNumber);
return this;
}
/**
*
* The path to the registration form field.
*
*
* @param fieldPath
* The path to the registration form field.
*/
public void setFieldPath(String fieldPath) {
this.fieldPath = fieldPath;
}
/**
*
* The path to the registration form field.
*
*
* @return The path to the registration form field.
*/
public String getFieldPath() {
return this.fieldPath;
}
/**
*
* The path to the registration form field.
*
*
* @param fieldPath
* The path to the registration form field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withFieldPath(String fieldPath) {
setFieldPath(fieldPath);
return this;
}
/**
*
* An array of values for the form field.
*
*
* @return An array of values for the form field.
*/
public java.util.List getSelectChoices() {
return selectChoices;
}
/**
*
* An array of values for the form field.
*
*
* @param selectChoices
* An array of values for the form field.
*/
public void setSelectChoices(java.util.Collection selectChoices) {
if (selectChoices == null) {
this.selectChoices = null;
return;
}
this.selectChoices = new java.util.ArrayList(selectChoices);
}
/**
*
* An array of values for the form field.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSelectChoices(java.util.Collection)} or {@link #withSelectChoices(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param selectChoices
* An array of values for the form field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withSelectChoices(String... selectChoices) {
if (this.selectChoices == null) {
setSelectChoices(new java.util.ArrayList(selectChoices.length));
}
for (String ele : selectChoices) {
this.selectChoices.add(ele);
}
return this;
}
/**
*
* An array of values for the form field.
*
*
* @param selectChoices
* An array of values for the form field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withSelectChoices(java.util.Collection selectChoices) {
setSelectChoices(selectChoices);
return this;
}
/**
*
* The text data for a free form field.
*
*
* @param textValue
* The text data for a free form field.
*/
public void setTextValue(String textValue) {
this.textValue = textValue;
}
/**
*
* The text data for a free form field.
*
*
* @return The text data for a free form field.
*/
public String getTextValue() {
return this.textValue;
}
/**
*
* The text data for a free form field.
*
*
* @param textValue
* The text data for a free form field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withTextValue(String textValue) {
setTextValue(textValue);
return this;
}
/**
*
* The unique identifier for the registration attachment.
*
*
* @param registrationAttachmentId
* The unique identifier for the registration attachment.
*/
public void setRegistrationAttachmentId(String registrationAttachmentId) {
this.registrationAttachmentId = registrationAttachmentId;
}
/**
*
* The unique identifier for the registration attachment.
*
*
* @return The unique identifier for the registration attachment.
*/
public String getRegistrationAttachmentId() {
return this.registrationAttachmentId;
}
/**
*
* The unique identifier for the registration attachment.
*
*
* @param registrationAttachmentId
* The unique identifier for the registration attachment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteRegistrationFieldValueResult withRegistrationAttachmentId(String registrationAttachmentId) {
setRegistrationAttachmentId(registrationAttachmentId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegistrationArn() != null)
sb.append("RegistrationArn: ").append(getRegistrationArn()).append(",");
if (getRegistrationId() != null)
sb.append("RegistrationId: ").append(getRegistrationId()).append(",");
if (getVersionNumber() != null)
sb.append("VersionNumber: ").append(getVersionNumber()).append(",");
if (getFieldPath() != null)
sb.append("FieldPath: ").append(getFieldPath()).append(",");
if (getSelectChoices() != null)
sb.append("SelectChoices: ").append(getSelectChoices()).append(",");
if (getTextValue() != null)
sb.append("TextValue: ").append(getTextValue()).append(",");
if (getRegistrationAttachmentId() != null)
sb.append("RegistrationAttachmentId: ").append(getRegistrationAttachmentId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DeleteRegistrationFieldValueResult == false)
return false;
DeleteRegistrationFieldValueResult other = (DeleteRegistrationFieldValueResult) obj;
if (other.getRegistrationArn() == null ^ this.getRegistrationArn() == null)
return false;
if (other.getRegistrationArn() != null && other.getRegistrationArn().equals(this.getRegistrationArn()) == false)
return false;
if (other.getRegistrationId() == null ^ this.getRegistrationId() == null)
return false;
if (other.getRegistrationId() != null && other.getRegistrationId().equals(this.getRegistrationId()) == false)
return false;
if (other.getVersionNumber() == null ^ this.getVersionNumber() == null)
return false;
if (other.getVersionNumber() != null && other.getVersionNumber().equals(this.getVersionNumber()) == false)
return false;
if (other.getFieldPath() == null ^ this.getFieldPath() == null)
return false;
if (other.getFieldPath() != null && other.getFieldPath().equals(this.getFieldPath()) == false)
return false;
if (other.getSelectChoices() == null ^ this.getSelectChoices() == null)
return false;
if (other.getSelectChoices() != null && other.getSelectChoices().equals(this.getSelectChoices()) == false)
return false;
if (other.getTextValue() == null ^ this.getTextValue() == null)
return false;
if (other.getTextValue() != null && other.getTextValue().equals(this.getTextValue()) == false)
return false;
if (other.getRegistrationAttachmentId() == null ^ this.getRegistrationAttachmentId() == null)
return false;
if (other.getRegistrationAttachmentId() != null && other.getRegistrationAttachmentId().equals(this.getRegistrationAttachmentId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegistrationArn() == null) ? 0 : getRegistrationArn().hashCode());
hashCode = prime * hashCode + ((getRegistrationId() == null) ? 0 : getRegistrationId().hashCode());
hashCode = prime * hashCode + ((getVersionNumber() == null) ? 0 : getVersionNumber().hashCode());
hashCode = prime * hashCode + ((getFieldPath() == null) ? 0 : getFieldPath().hashCode());
hashCode = prime * hashCode + ((getSelectChoices() == null) ? 0 : getSelectChoices().hashCode());
hashCode = prime * hashCode + ((getTextValue() == null) ? 0 : getTextValue().hashCode());
hashCode = prime * hashCode + ((getRegistrationAttachmentId() == null) ? 0 : getRegistrationAttachmentId().hashCode());
return hashCode;
}
@Override
public DeleteRegistrationFieldValueResult clone() {
try {
return (DeleteRegistrationFieldValueResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}