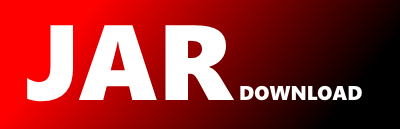
com.amazonaws.services.pinpointsmsvoicev2.model.DescribeOptedOutNumbersResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeOptedOutNumbersResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the OptOutList.
*
*/
private String optOutListArn;
/**
*
* The name of the OptOutList.
*
*/
private String optOutListName;
/**
*
* An array of OptedOutNumbersInformation objects that provide information about the requested OptedOutNumbers.
*
*/
private java.util.List optedOutNumbers;
/**
*
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*
*/
private String nextToken;
/**
*
* The Amazon Resource Name (ARN) of the OptOutList.
*
*
* @param optOutListArn
* The Amazon Resource Name (ARN) of the OptOutList.
*/
public void setOptOutListArn(String optOutListArn) {
this.optOutListArn = optOutListArn;
}
/**
*
* The Amazon Resource Name (ARN) of the OptOutList.
*
*
* @return The Amazon Resource Name (ARN) of the OptOutList.
*/
public String getOptOutListArn() {
return this.optOutListArn;
}
/**
*
* The Amazon Resource Name (ARN) of the OptOutList.
*
*
* @param optOutListArn
* The Amazon Resource Name (ARN) of the OptOutList.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeOptedOutNumbersResult withOptOutListArn(String optOutListArn) {
setOptOutListArn(optOutListArn);
return this;
}
/**
*
* The name of the OptOutList.
*
*
* @param optOutListName
* The name of the OptOutList.
*/
public void setOptOutListName(String optOutListName) {
this.optOutListName = optOutListName;
}
/**
*
* The name of the OptOutList.
*
*
* @return The name of the OptOutList.
*/
public String getOptOutListName() {
return this.optOutListName;
}
/**
*
* The name of the OptOutList.
*
*
* @param optOutListName
* The name of the OptOutList.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeOptedOutNumbersResult withOptOutListName(String optOutListName) {
setOptOutListName(optOutListName);
return this;
}
/**
*
* An array of OptedOutNumbersInformation objects that provide information about the requested OptedOutNumbers.
*
*
* @return An array of OptedOutNumbersInformation objects that provide information about the requested
* OptedOutNumbers.
*/
public java.util.List getOptedOutNumbers() {
return optedOutNumbers;
}
/**
*
* An array of OptedOutNumbersInformation objects that provide information about the requested OptedOutNumbers.
*
*
* @param optedOutNumbers
* An array of OptedOutNumbersInformation objects that provide information about the requested
* OptedOutNumbers.
*/
public void setOptedOutNumbers(java.util.Collection optedOutNumbers) {
if (optedOutNumbers == null) {
this.optedOutNumbers = null;
return;
}
this.optedOutNumbers = new java.util.ArrayList(optedOutNumbers);
}
/**
*
* An array of OptedOutNumbersInformation objects that provide information about the requested OptedOutNumbers.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOptedOutNumbers(java.util.Collection)} or {@link #withOptedOutNumbers(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param optedOutNumbers
* An array of OptedOutNumbersInformation objects that provide information about the requested
* OptedOutNumbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeOptedOutNumbersResult withOptedOutNumbers(OptedOutNumberInformation... optedOutNumbers) {
if (this.optedOutNumbers == null) {
setOptedOutNumbers(new java.util.ArrayList(optedOutNumbers.length));
}
for (OptedOutNumberInformation ele : optedOutNumbers) {
this.optedOutNumbers.add(ele);
}
return this;
}
/**
*
* An array of OptedOutNumbersInformation objects that provide information about the requested OptedOutNumbers.
*
*
* @param optedOutNumbers
* An array of OptedOutNumbersInformation objects that provide information about the requested
* OptedOutNumbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeOptedOutNumbersResult withOptedOutNumbers(java.util.Collection optedOutNumbers) {
setOptedOutNumbers(optedOutNumbers);
return this;
}
/**
*
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*
*
* @return The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. If this field is empty then there are no more
* results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeOptedOutNumbersResult withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOptOutListArn() != null)
sb.append("OptOutListArn: ").append(getOptOutListArn()).append(",");
if (getOptOutListName() != null)
sb.append("OptOutListName: ").append(getOptOutListName()).append(",");
if (getOptedOutNumbers() != null)
sb.append("OptedOutNumbers: ").append(getOptedOutNumbers()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeOptedOutNumbersResult == false)
return false;
DescribeOptedOutNumbersResult other = (DescribeOptedOutNumbersResult) obj;
if (other.getOptOutListArn() == null ^ this.getOptOutListArn() == null)
return false;
if (other.getOptOutListArn() != null && other.getOptOutListArn().equals(this.getOptOutListArn()) == false)
return false;
if (other.getOptOutListName() == null ^ this.getOptOutListName() == null)
return false;
if (other.getOptOutListName() != null && other.getOptOutListName().equals(this.getOptOutListName()) == false)
return false;
if (other.getOptedOutNumbers() == null ^ this.getOptedOutNumbers() == null)
return false;
if (other.getOptedOutNumbers() != null && other.getOptedOutNumbers().equals(this.getOptedOutNumbers()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOptOutListArn() == null) ? 0 : getOptOutListArn().hashCode());
hashCode = prime * hashCode + ((getOptOutListName() == null) ? 0 : getOptOutListName().hashCode());
hashCode = prime * hashCode + ((getOptedOutNumbers() == null) ? 0 : getOptedOutNumbers().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public DescribeOptedOutNumbersResult clone() {
try {
return (DescribeOptedOutNumbersResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}