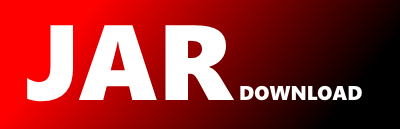
com.amazonaws.services.pinpointsmsvoicev2.model.DescribeRegistrationSectionDefinitionsResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeRegistrationSectionDefinitionsResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*/
private String registrationType;
/**
*
* An array of RegistrationSectionDefinition objects.
*
*/
private java.util.List registrationSectionDefinitions;
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*/
private String nextToken;
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public void setRegistrationType(String registrationType) {
this.registrationType = registrationType;
}
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @return The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public String getRegistrationType() {
return this.registrationType;
}
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeRegistrationSectionDefinitionsResult withRegistrationType(String registrationType) {
setRegistrationType(registrationType);
return this;
}
/**
*
* An array of RegistrationSectionDefinition objects.
*
*
* @return An array of RegistrationSectionDefinition objects.
*/
public java.util.List getRegistrationSectionDefinitions() {
return registrationSectionDefinitions;
}
/**
*
* An array of RegistrationSectionDefinition objects.
*
*
* @param registrationSectionDefinitions
* An array of RegistrationSectionDefinition objects.
*/
public void setRegistrationSectionDefinitions(java.util.Collection registrationSectionDefinitions) {
if (registrationSectionDefinitions == null) {
this.registrationSectionDefinitions = null;
return;
}
this.registrationSectionDefinitions = new java.util.ArrayList(registrationSectionDefinitions);
}
/**
*
* An array of RegistrationSectionDefinition objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegistrationSectionDefinitions(java.util.Collection)} or
* {@link #withRegistrationSectionDefinitions(java.util.Collection)} if you want to override the existing values.
*
*
* @param registrationSectionDefinitions
* An array of RegistrationSectionDefinition objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeRegistrationSectionDefinitionsResult withRegistrationSectionDefinitions(RegistrationSectionDefinition... registrationSectionDefinitions) {
if (this.registrationSectionDefinitions == null) {
setRegistrationSectionDefinitions(new java.util.ArrayList(registrationSectionDefinitions.length));
}
for (RegistrationSectionDefinition ele : registrationSectionDefinitions) {
this.registrationSectionDefinitions.add(ele);
}
return this;
}
/**
*
* An array of RegistrationSectionDefinition objects.
*
*
* @param registrationSectionDefinitions
* An array of RegistrationSectionDefinition objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeRegistrationSectionDefinitionsResult withRegistrationSectionDefinitions(
java.util.Collection registrationSectionDefinitions) {
setRegistrationSectionDefinitions(registrationSectionDefinitions);
return this;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @return The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeRegistrationSectionDefinitionsResult withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegistrationType() != null)
sb.append("RegistrationType: ").append(getRegistrationType()).append(",");
if (getRegistrationSectionDefinitions() != null)
sb.append("RegistrationSectionDefinitions: ").append(getRegistrationSectionDefinitions()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeRegistrationSectionDefinitionsResult == false)
return false;
DescribeRegistrationSectionDefinitionsResult other = (DescribeRegistrationSectionDefinitionsResult) obj;
if (other.getRegistrationType() == null ^ this.getRegistrationType() == null)
return false;
if (other.getRegistrationType() != null && other.getRegistrationType().equals(this.getRegistrationType()) == false)
return false;
if (other.getRegistrationSectionDefinitions() == null ^ this.getRegistrationSectionDefinitions() == null)
return false;
if (other.getRegistrationSectionDefinitions() != null
&& other.getRegistrationSectionDefinitions().equals(this.getRegistrationSectionDefinitions()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegistrationType() == null) ? 0 : getRegistrationType().hashCode());
hashCode = prime * hashCode + ((getRegistrationSectionDefinitions() == null) ? 0 : getRegistrationSectionDefinitions().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public DescribeRegistrationSectionDefinitionsResult clone() {
try {
return (DescribeRegistrationSectionDefinitionsResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}