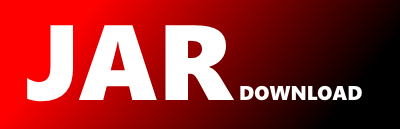
com.amazonaws.services.pinpointsmsvoicev2.model.ListRegistrationAssociationsResult Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListRegistrationAssociationsResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*/
private String registrationArn;
/**
*
* The unique identifier for the registration.
*
*/
private String registrationId;
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*/
private String registrationType;
/**
*
* An array of RegistrationAssociationMetadata objects.
*
*/
private java.util.List registrationAssociations;
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*/
private String nextToken;
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
*/
public void setRegistrationArn(String registrationArn) {
this.registrationArn = registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @return The Amazon Resource Name (ARN) for the registration.
*/
public String getRegistrationArn() {
return this.registrationArn;
}
/**
*
* The Amazon Resource Name (ARN) for the registration.
*
*
* @param registrationArn
* The Amazon Resource Name (ARN) for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withRegistrationArn(String registrationArn) {
setRegistrationArn(registrationArn);
return this;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
*/
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @return The unique identifier for the registration.
*/
public String getRegistrationId() {
return this.registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withRegistrationId(String registrationId) {
setRegistrationId(registrationId);
return this;
}
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public void setRegistrationType(String registrationType) {
this.registrationType = registrationType;
}
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @return The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*/
public String getRegistrationType() {
return this.registrationType;
}
/**
*
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
*
*
* @param registrationType
* The type of registration form. The list of RegistrationTypes can be found using the
* DescribeRegistrationTypeDefinitions action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withRegistrationType(String registrationType) {
setRegistrationType(registrationType);
return this;
}
/**
*
* An array of RegistrationAssociationMetadata objects.
*
*
* @return An array of RegistrationAssociationMetadata objects.
*/
public java.util.List getRegistrationAssociations() {
return registrationAssociations;
}
/**
*
* An array of RegistrationAssociationMetadata objects.
*
*
* @param registrationAssociations
* An array of RegistrationAssociationMetadata objects.
*/
public void setRegistrationAssociations(java.util.Collection registrationAssociations) {
if (registrationAssociations == null) {
this.registrationAssociations = null;
return;
}
this.registrationAssociations = new java.util.ArrayList(registrationAssociations);
}
/**
*
* An array of RegistrationAssociationMetadata objects.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegistrationAssociations(java.util.Collection)} or
* {@link #withRegistrationAssociations(java.util.Collection)} if you want to override the existing values.
*
*
* @param registrationAssociations
* An array of RegistrationAssociationMetadata objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withRegistrationAssociations(RegistrationAssociationMetadata... registrationAssociations) {
if (this.registrationAssociations == null) {
setRegistrationAssociations(new java.util.ArrayList(registrationAssociations.length));
}
for (RegistrationAssociationMetadata ele : registrationAssociations) {
this.registrationAssociations.add(ele);
}
return this;
}
/**
*
* An array of RegistrationAssociationMetadata objects.
*
*
* @param registrationAssociations
* An array of RegistrationAssociationMetadata objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withRegistrationAssociations(java.util.Collection registrationAssociations) {
setRegistrationAssociations(registrationAssociations);
return this;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @return The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token to be used for the next set of paginated results. You don't need to supply a value for this field in
* the initial request.
*
*
* @param nextToken
* The token to be used for the next set of paginated results. You don't need to supply a value for this
* field in the initial request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListRegistrationAssociationsResult withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegistrationArn() != null)
sb.append("RegistrationArn: ").append(getRegistrationArn()).append(",");
if (getRegistrationId() != null)
sb.append("RegistrationId: ").append(getRegistrationId()).append(",");
if (getRegistrationType() != null)
sb.append("RegistrationType: ").append(getRegistrationType()).append(",");
if (getRegistrationAssociations() != null)
sb.append("RegistrationAssociations: ").append(getRegistrationAssociations()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListRegistrationAssociationsResult == false)
return false;
ListRegistrationAssociationsResult other = (ListRegistrationAssociationsResult) obj;
if (other.getRegistrationArn() == null ^ this.getRegistrationArn() == null)
return false;
if (other.getRegistrationArn() != null && other.getRegistrationArn().equals(this.getRegistrationArn()) == false)
return false;
if (other.getRegistrationId() == null ^ this.getRegistrationId() == null)
return false;
if (other.getRegistrationId() != null && other.getRegistrationId().equals(this.getRegistrationId()) == false)
return false;
if (other.getRegistrationType() == null ^ this.getRegistrationType() == null)
return false;
if (other.getRegistrationType() != null && other.getRegistrationType().equals(this.getRegistrationType()) == false)
return false;
if (other.getRegistrationAssociations() == null ^ this.getRegistrationAssociations() == null)
return false;
if (other.getRegistrationAssociations() != null && other.getRegistrationAssociations().equals(this.getRegistrationAssociations()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegistrationArn() == null) ? 0 : getRegistrationArn().hashCode());
hashCode = prime * hashCode + ((getRegistrationId() == null) ? 0 : getRegistrationId().hashCode());
hashCode = prime * hashCode + ((getRegistrationType() == null) ? 0 : getRegistrationType().hashCode());
hashCode = prime * hashCode + ((getRegistrationAssociations() == null) ? 0 : getRegistrationAssociations().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
return hashCode;
}
@Override
public ListRegistrationAssociationsResult clone() {
try {
return (ListRegistrationAssociationsResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}