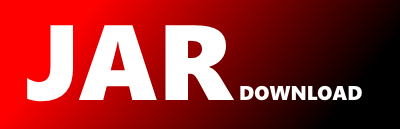
com.amazonaws.services.pinpointsmsvoicev2.model.PoolInformation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pinpointsmsvoicev2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The information for a pool in an Amazon Web Services account.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PoolInformation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) for the pool.
*
*/
private String poolArn;
/**
*
* The unique identifier for the pool.
*
*/
private String poolId;
/**
*
* The current status of the pool.
*
*/
private String status;
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*/
private String messageType;
/**
*
* When set to true you can receive incoming text messages from your end recipients using the TwoWayChannelArn.
*
*/
private Boolean twoWayEnabled;
/**
*
* The Amazon Resource Name (ARN) of the two way channel.
*
*/
private String twoWayChannelArn;
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*/
private String twoWayChannelRole;
/**
*
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds the end
* recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP requests. You're
* also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*
*/
private Boolean selfManagedOptOutsEnabled;
/**
*
* The name of the OptOutList associated with the pool.
*
*/
private String optOutListName;
/**
*
* Allows you to enable shared routes on your pool.
*
*
* By default, this is set to False
. If you set this value to True
, your messages are sent
* using phone numbers or sender IDs (depending on the country) that are shared with other users. In some countries,
* such as the United States, senders aren't allowed to use shared routes and must use a dedicated phone number or
* short code.
*
*/
private Boolean sharedRoutesEnabled;
/**
*
* When set to true the pool can't be deleted.
*
*/
private Boolean deletionProtectionEnabled;
/**
*
* The time when the pool was created, in UNIX epoch time format.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The Amazon Resource Name (ARN) for the pool.
*
*
* @param poolArn
* The Amazon Resource Name (ARN) for the pool.
*/
public void setPoolArn(String poolArn) {
this.poolArn = poolArn;
}
/**
*
* The Amazon Resource Name (ARN) for the pool.
*
*
* @return The Amazon Resource Name (ARN) for the pool.
*/
public String getPoolArn() {
return this.poolArn;
}
/**
*
* The Amazon Resource Name (ARN) for the pool.
*
*
* @param poolArn
* The Amazon Resource Name (ARN) for the pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withPoolArn(String poolArn) {
setPoolArn(poolArn);
return this;
}
/**
*
* The unique identifier for the pool.
*
*
* @param poolId
* The unique identifier for the pool.
*/
public void setPoolId(String poolId) {
this.poolId = poolId;
}
/**
*
* The unique identifier for the pool.
*
*
* @return The unique identifier for the pool.
*/
public String getPoolId() {
return this.poolId;
}
/**
*
* The unique identifier for the pool.
*
*
* @param poolId
* The unique identifier for the pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withPoolId(String poolId) {
setPoolId(poolId);
return this;
}
/**
*
* The current status of the pool.
*
*
* @param status
* The current status of the pool.
* @see PoolStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the pool.
*
*
* @return The current status of the pool.
* @see PoolStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the pool.
*
*
* @param status
* The current status of the pool.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PoolStatus
*/
public PoolInformation withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the pool.
*
*
* @param status
* The current status of the pool.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PoolStatus
*/
public PoolInformation withStatus(PoolStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @see MessageType
*/
public void setMessageType(String messageType) {
this.messageType = messageType;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @return The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @see MessageType
*/
public String getMessageType() {
return this.messageType;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public PoolInformation withMessageType(String messageType) {
setMessageType(messageType);
return this;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public PoolInformation withMessageType(MessageType messageType) {
this.messageType = messageType.toString();
return this;
}
/**
*
* When set to true you can receive incoming text messages from your end recipients using the TwoWayChannelArn.
*
*
* @param twoWayEnabled
* When set to true you can receive incoming text messages from your end recipients using the
* TwoWayChannelArn.
*/
public void setTwoWayEnabled(Boolean twoWayEnabled) {
this.twoWayEnabled = twoWayEnabled;
}
/**
*
* When set to true you can receive incoming text messages from your end recipients using the TwoWayChannelArn.
*
*
* @return When set to true you can receive incoming text messages from your end recipients using the
* TwoWayChannelArn.
*/
public Boolean getTwoWayEnabled() {
return this.twoWayEnabled;
}
/**
*
* When set to true you can receive incoming text messages from your end recipients using the TwoWayChannelArn.
*
*
* @param twoWayEnabled
* When set to true you can receive incoming text messages from your end recipients using the
* TwoWayChannelArn.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withTwoWayEnabled(Boolean twoWayEnabled) {
setTwoWayEnabled(twoWayEnabled);
return this;
}
/**
*
* When set to true you can receive incoming text messages from your end recipients using the TwoWayChannelArn.
*
*
* @return When set to true you can receive incoming text messages from your end recipients using the
* TwoWayChannelArn.
*/
public Boolean isTwoWayEnabled() {
return this.twoWayEnabled;
}
/**
*
* The Amazon Resource Name (ARN) of the two way channel.
*
*
* @param twoWayChannelArn
* The Amazon Resource Name (ARN) of the two way channel.
*/
public void setTwoWayChannelArn(String twoWayChannelArn) {
this.twoWayChannelArn = twoWayChannelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the two way channel.
*
*
* @return The Amazon Resource Name (ARN) of the two way channel.
*/
public String getTwoWayChannelArn() {
return this.twoWayChannelArn;
}
/**
*
* The Amazon Resource Name (ARN) of the two way channel.
*
*
* @param twoWayChannelArn
* The Amazon Resource Name (ARN) of the two way channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withTwoWayChannelArn(String twoWayChannelArn) {
setTwoWayChannelArn(twoWayChannelArn);
return this;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @param twoWayChannelRole
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*/
public void setTwoWayChannelRole(String twoWayChannelRole) {
this.twoWayChannelRole = twoWayChannelRole;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @return An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*/
public String getTwoWayChannelRole() {
return this.twoWayChannelRole;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @param twoWayChannelRole
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withTwoWayChannelRole(String twoWayChannelRole) {
setTwoWayChannelRole(twoWayChannelRole);
return this;
}
/**
*
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds the end
* recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP requests. You're
* also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*
*
* @param selfManagedOptOutsEnabled
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds
* the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*/
public void setSelfManagedOptOutsEnabled(Boolean selfManagedOptOutsEnabled) {
this.selfManagedOptOutsEnabled = selfManagedOptOutsEnabled;
}
/**
*
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds the end
* recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP requests. You're
* also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*
*
* @return When set to false, an end recipient sends a message that begins with HELP or STOP to one of your
* dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP
* and STOP requests. You're also responsible for tracking and honoring opt-out requests. For more
* information see Self-managed opt-outs
*/
public Boolean getSelfManagedOptOutsEnabled() {
return this.selfManagedOptOutsEnabled;
}
/**
*
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds the end
* recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP requests. You're
* also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*
*
* @param selfManagedOptOutsEnabled
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds
* the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withSelfManagedOptOutsEnabled(Boolean selfManagedOptOutsEnabled) {
setSelfManagedOptOutsEnabled(selfManagedOptOutsEnabled);
return this;
}
/**
*
* When set to false, an end recipient sends a message that begins with HELP or STOP to one of your dedicated
* numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message and adds the end
* recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP requests. You're
* also responsible for tracking and honoring opt-out requests. For more information see Self-managed opt-outs
*
*
* @return When set to false, an end recipient sends a message that begins with HELP or STOP to one of your
* dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP
* and STOP requests. You're also responsible for tracking and honoring opt-out requests. For more
* information see Self-managed opt-outs
*/
public Boolean isSelfManagedOptOutsEnabled() {
return this.selfManagedOptOutsEnabled;
}
/**
*
* The name of the OptOutList associated with the pool.
*
*
* @param optOutListName
* The name of the OptOutList associated with the pool.
*/
public void setOptOutListName(String optOutListName) {
this.optOutListName = optOutListName;
}
/**
*
* The name of the OptOutList associated with the pool.
*
*
* @return The name of the OptOutList associated with the pool.
*/
public String getOptOutListName() {
return this.optOutListName;
}
/**
*
* The name of the OptOutList associated with the pool.
*
*
* @param optOutListName
* The name of the OptOutList associated with the pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withOptOutListName(String optOutListName) {
setOptOutListName(optOutListName);
return this;
}
/**
*
* Allows you to enable shared routes on your pool.
*
*
* By default, this is set to False
. If you set this value to True
, your messages are sent
* using phone numbers or sender IDs (depending on the country) that are shared with other users. In some countries,
* such as the United States, senders aren't allowed to use shared routes and must use a dedicated phone number or
* short code.
*
*
* @param sharedRoutesEnabled
* Allows you to enable shared routes on your pool.
*
* By default, this is set to False
. If you set this value to True
, your messages
* are sent using phone numbers or sender IDs (depending on the country) that are shared with other users. In
* some countries, such as the United States, senders aren't allowed to use shared routes and must use a
* dedicated phone number or short code.
*/
public void setSharedRoutesEnabled(Boolean sharedRoutesEnabled) {
this.sharedRoutesEnabled = sharedRoutesEnabled;
}
/**
*
* Allows you to enable shared routes on your pool.
*
*
* By default, this is set to False
. If you set this value to True
, your messages are sent
* using phone numbers or sender IDs (depending on the country) that are shared with other users. In some countries,
* such as the United States, senders aren't allowed to use shared routes and must use a dedicated phone number or
* short code.
*
*
* @return Allows you to enable shared routes on your pool.
*
* By default, this is set to False
. If you set this value to True
, your messages
* are sent using phone numbers or sender IDs (depending on the country) that are shared with other users.
* In some countries, such as the United States, senders aren't allowed to use shared routes and must use a
* dedicated phone number or short code.
*/
public Boolean getSharedRoutesEnabled() {
return this.sharedRoutesEnabled;
}
/**
*
* Allows you to enable shared routes on your pool.
*
*
* By default, this is set to False
. If you set this value to True
, your messages are sent
* using phone numbers or sender IDs (depending on the country) that are shared with other users. In some countries,
* such as the United States, senders aren't allowed to use shared routes and must use a dedicated phone number or
* short code.
*
*
* @param sharedRoutesEnabled
* Allows you to enable shared routes on your pool.
*
* By default, this is set to False
. If you set this value to True
, your messages
* are sent using phone numbers or sender IDs (depending on the country) that are shared with other users. In
* some countries, such as the United States, senders aren't allowed to use shared routes and must use a
* dedicated phone number or short code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withSharedRoutesEnabled(Boolean sharedRoutesEnabled) {
setSharedRoutesEnabled(sharedRoutesEnabled);
return this;
}
/**
*
* Allows you to enable shared routes on your pool.
*
*
* By default, this is set to False
. If you set this value to True
, your messages are sent
* using phone numbers or sender IDs (depending on the country) that are shared with other users. In some countries,
* such as the United States, senders aren't allowed to use shared routes and must use a dedicated phone number or
* short code.
*
*
* @return Allows you to enable shared routes on your pool.
*
* By default, this is set to False
. If you set this value to True
, your messages
* are sent using phone numbers or sender IDs (depending on the country) that are shared with other users.
* In some countries, such as the United States, senders aren't allowed to use shared routes and must use a
* dedicated phone number or short code.
*/
public Boolean isSharedRoutesEnabled() {
return this.sharedRoutesEnabled;
}
/**
*
* When set to true the pool can't be deleted.
*
*
* @param deletionProtectionEnabled
* When set to true the pool can't be deleted.
*/
public void setDeletionProtectionEnabled(Boolean deletionProtectionEnabled) {
this.deletionProtectionEnabled = deletionProtectionEnabled;
}
/**
*
* When set to true the pool can't be deleted.
*
*
* @return When set to true the pool can't be deleted.
*/
public Boolean getDeletionProtectionEnabled() {
return this.deletionProtectionEnabled;
}
/**
*
* When set to true the pool can't be deleted.
*
*
* @param deletionProtectionEnabled
* When set to true the pool can't be deleted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withDeletionProtectionEnabled(Boolean deletionProtectionEnabled) {
setDeletionProtectionEnabled(deletionProtectionEnabled);
return this;
}
/**
*
* When set to true the pool can't be deleted.
*
*
* @return When set to true the pool can't be deleted.
*/
public Boolean isDeletionProtectionEnabled() {
return this.deletionProtectionEnabled;
}
/**
*
* The time when the pool was created, in UNIX epoch time format.
*
*
* @param createdTimestamp
* The time when the pool was created, in UNIX epoch time
* format.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The time when the pool was created, in UNIX epoch time format.
*
*
* @return The time when the pool was created, in UNIX epoch time
* format.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The time when the pool was created, in UNIX epoch time format.
*
*
* @param createdTimestamp
* The time when the pool was created, in UNIX epoch time
* format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PoolInformation withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPoolArn() != null)
sb.append("PoolArn: ").append(getPoolArn()).append(",");
if (getPoolId() != null)
sb.append("PoolId: ").append(getPoolId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getMessageType() != null)
sb.append("MessageType: ").append(getMessageType()).append(",");
if (getTwoWayEnabled() != null)
sb.append("TwoWayEnabled: ").append(getTwoWayEnabled()).append(",");
if (getTwoWayChannelArn() != null)
sb.append("TwoWayChannelArn: ").append(getTwoWayChannelArn()).append(",");
if (getTwoWayChannelRole() != null)
sb.append("TwoWayChannelRole: ").append(getTwoWayChannelRole()).append(",");
if (getSelfManagedOptOutsEnabled() != null)
sb.append("SelfManagedOptOutsEnabled: ").append(getSelfManagedOptOutsEnabled()).append(",");
if (getOptOutListName() != null)
sb.append("OptOutListName: ").append(getOptOutListName()).append(",");
if (getSharedRoutesEnabled() != null)
sb.append("SharedRoutesEnabled: ").append(getSharedRoutesEnabled()).append(",");
if (getDeletionProtectionEnabled() != null)
sb.append("DeletionProtectionEnabled: ").append(getDeletionProtectionEnabled()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PoolInformation == false)
return false;
PoolInformation other = (PoolInformation) obj;
if (other.getPoolArn() == null ^ this.getPoolArn() == null)
return false;
if (other.getPoolArn() != null && other.getPoolArn().equals(this.getPoolArn()) == false)
return false;
if (other.getPoolId() == null ^ this.getPoolId() == null)
return false;
if (other.getPoolId() != null && other.getPoolId().equals(this.getPoolId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getMessageType() == null ^ this.getMessageType() == null)
return false;
if (other.getMessageType() != null && other.getMessageType().equals(this.getMessageType()) == false)
return false;
if (other.getTwoWayEnabled() == null ^ this.getTwoWayEnabled() == null)
return false;
if (other.getTwoWayEnabled() != null && other.getTwoWayEnabled().equals(this.getTwoWayEnabled()) == false)
return false;
if (other.getTwoWayChannelArn() == null ^ this.getTwoWayChannelArn() == null)
return false;
if (other.getTwoWayChannelArn() != null && other.getTwoWayChannelArn().equals(this.getTwoWayChannelArn()) == false)
return false;
if (other.getTwoWayChannelRole() == null ^ this.getTwoWayChannelRole() == null)
return false;
if (other.getTwoWayChannelRole() != null && other.getTwoWayChannelRole().equals(this.getTwoWayChannelRole()) == false)
return false;
if (other.getSelfManagedOptOutsEnabled() == null ^ this.getSelfManagedOptOutsEnabled() == null)
return false;
if (other.getSelfManagedOptOutsEnabled() != null && other.getSelfManagedOptOutsEnabled().equals(this.getSelfManagedOptOutsEnabled()) == false)
return false;
if (other.getOptOutListName() == null ^ this.getOptOutListName() == null)
return false;
if (other.getOptOutListName() != null && other.getOptOutListName().equals(this.getOptOutListName()) == false)
return false;
if (other.getSharedRoutesEnabled() == null ^ this.getSharedRoutesEnabled() == null)
return false;
if (other.getSharedRoutesEnabled() != null && other.getSharedRoutesEnabled().equals(this.getSharedRoutesEnabled()) == false)
return false;
if (other.getDeletionProtectionEnabled() == null ^ this.getDeletionProtectionEnabled() == null)
return false;
if (other.getDeletionProtectionEnabled() != null && other.getDeletionProtectionEnabled().equals(this.getDeletionProtectionEnabled()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPoolArn() == null) ? 0 : getPoolArn().hashCode());
hashCode = prime * hashCode + ((getPoolId() == null) ? 0 : getPoolId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getMessageType() == null) ? 0 : getMessageType().hashCode());
hashCode = prime * hashCode + ((getTwoWayEnabled() == null) ? 0 : getTwoWayEnabled().hashCode());
hashCode = prime * hashCode + ((getTwoWayChannelArn() == null) ? 0 : getTwoWayChannelArn().hashCode());
hashCode = prime * hashCode + ((getTwoWayChannelRole() == null) ? 0 : getTwoWayChannelRole().hashCode());
hashCode = prime * hashCode + ((getSelfManagedOptOutsEnabled() == null) ? 0 : getSelfManagedOptOutsEnabled().hashCode());
hashCode = prime * hashCode + ((getOptOutListName() == null) ? 0 : getOptOutListName().hashCode());
hashCode = prime * hashCode + ((getSharedRoutesEnabled() == null) ? 0 : getSharedRoutesEnabled().hashCode());
hashCode = prime * hashCode + ((getDeletionProtectionEnabled() == null) ? 0 : getDeletionProtectionEnabled().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
return hashCode;
}
@Override
public PoolInformation clone() {
try {
return (PoolInformation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.pinpointsmsvoicev2.model.transform.PoolInformationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}