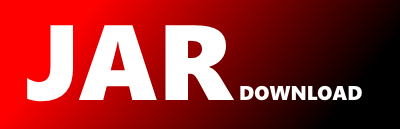
com.amazonaws.services.pinpointsmsvoicev2.model.RequestPhoneNumberResult Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pinpointsmsvoicev2.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RequestPhoneNumberResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the requested phone number.
*
*/
private String phoneNumberArn;
/**
*
* The unique identifier of the new phone number.
*
*/
private String phoneNumberId;
/**
*
* The new phone number that was requested.
*
*/
private String phoneNumber;
/**
*
* The current status of the request.
*
*/
private String status;
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*
*/
private String isoCountryCode;
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*/
private String messageType;
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*/
private java.util.List numberCapabilities;
/**
*
* The type of number that was released.
*
*/
private String numberType;
/**
*
* The monthly price, in US dollars, to lease the phone number.
*
*/
private String monthlyLeasingPrice;
/**
*
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*
*/
private Boolean twoWayEnabled;
/**
*
* The ARN used to identify the two way channel.
*
*/
private String twoWayChannelArn;
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*/
private String twoWayChannelRole;
/**
*
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to one of
* your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests.
*
*/
private Boolean selfManagedOptOutsEnabled;
/**
*
* The name of the OptOutList that is associated with the requested phone number.
*
*/
private String optOutListName;
/**
*
* By default this is set to false. When set to true the phone number can't be deleted.
*
*/
private Boolean deletionProtectionEnabled;
/**
*
* The unique identifier of the pool associated with the phone number
*
*/
private String poolId;
/**
*
* The unique identifier for the registration.
*
*/
private String registrationId;
/**
*
* An array of key and value pair tags that are associated with the phone number.
*
*/
private java.util.List tags;
/**
*
* The time when the phone number was created, in UNIX epoch time
* format.
*
*/
private java.util.Date createdTimestamp;
/**
*
* The Amazon Resource Name (ARN) of the requested phone number.
*
*
* @param phoneNumberArn
* The Amazon Resource Name (ARN) of the requested phone number.
*/
public void setPhoneNumberArn(String phoneNumberArn) {
this.phoneNumberArn = phoneNumberArn;
}
/**
*
* The Amazon Resource Name (ARN) of the requested phone number.
*
*
* @return The Amazon Resource Name (ARN) of the requested phone number.
*/
public String getPhoneNumberArn() {
return this.phoneNumberArn;
}
/**
*
* The Amazon Resource Name (ARN) of the requested phone number.
*
*
* @param phoneNumberArn
* The Amazon Resource Name (ARN) of the requested phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withPhoneNumberArn(String phoneNumberArn) {
setPhoneNumberArn(phoneNumberArn);
return this;
}
/**
*
* The unique identifier of the new phone number.
*
*
* @param phoneNumberId
* The unique identifier of the new phone number.
*/
public void setPhoneNumberId(String phoneNumberId) {
this.phoneNumberId = phoneNumberId;
}
/**
*
* The unique identifier of the new phone number.
*
*
* @return The unique identifier of the new phone number.
*/
public String getPhoneNumberId() {
return this.phoneNumberId;
}
/**
*
* The unique identifier of the new phone number.
*
*
* @param phoneNumberId
* The unique identifier of the new phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withPhoneNumberId(String phoneNumberId) {
setPhoneNumberId(phoneNumberId);
return this;
}
/**
*
* The new phone number that was requested.
*
*
* @param phoneNumber
* The new phone number that was requested.
*/
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
/**
*
* The new phone number that was requested.
*
*
* @return The new phone number that was requested.
*/
public String getPhoneNumber() {
return this.phoneNumber;
}
/**
*
* The new phone number that was requested.
*
*
* @param phoneNumber
* The new phone number that was requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withPhoneNumber(String phoneNumber) {
setPhoneNumber(phoneNumber);
return this;
}
/**
*
* The current status of the request.
*
*
* @param status
* The current status of the request.
* @see NumberStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the request.
*
*
* @return The current status of the request.
* @see NumberStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the request.
*
*
* @param status
* The current status of the request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NumberStatus
*/
public RequestPhoneNumberResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the request.
*
*
* @param status
* The current status of the request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NumberStatus
*/
public RequestPhoneNumberResult withStatus(NumberStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*
*
* @param isoCountryCode
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*/
public void setIsoCountryCode(String isoCountryCode) {
this.isoCountryCode = isoCountryCode;
}
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*
*
* @return The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*/
public String getIsoCountryCode() {
return this.isoCountryCode;
}
/**
*
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
*
*
* @param isoCountryCode
* The two-character code, in ISO 3166-1 alpha-2 format, for the country or region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withIsoCountryCode(String isoCountryCode) {
setIsoCountryCode(isoCountryCode);
return this;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @see MessageType
*/
public void setMessageType(String messageType) {
this.messageType = messageType;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @return The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @see MessageType
*/
public String getMessageType() {
return this.messageType;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public RequestPhoneNumberResult withMessageType(String messageType) {
setMessageType(messageType);
return this;
}
/**
*
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
*
*
* @param messageType
* The type of message. Valid values are TRANSACTIONAL for messages that are critical or time-sensitive and
* PROMOTIONAL for messages that aren't critical or time-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MessageType
*/
public RequestPhoneNumberResult withMessageType(MessageType messageType) {
this.messageType = messageType.toString();
return this;
}
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*
* @return Indicates if the phone number will be used for text messages, voice messages or both.
* @see NumberCapability
*/
public java.util.List getNumberCapabilities() {
return numberCapabilities;
}
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*
* @param numberCapabilities
* Indicates if the phone number will be used for text messages, voice messages or both.
* @see NumberCapability
*/
public void setNumberCapabilities(java.util.Collection numberCapabilities) {
if (numberCapabilities == null) {
this.numberCapabilities = null;
return;
}
this.numberCapabilities = new java.util.ArrayList(numberCapabilities);
}
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNumberCapabilities(java.util.Collection)} or {@link #withNumberCapabilities(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param numberCapabilities
* Indicates if the phone number will be used for text messages, voice messages or both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NumberCapability
*/
public RequestPhoneNumberResult withNumberCapabilities(String... numberCapabilities) {
if (this.numberCapabilities == null) {
setNumberCapabilities(new java.util.ArrayList(numberCapabilities.length));
}
for (String ele : numberCapabilities) {
this.numberCapabilities.add(ele);
}
return this;
}
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*
* @param numberCapabilities
* Indicates if the phone number will be used for text messages, voice messages or both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NumberCapability
*/
public RequestPhoneNumberResult withNumberCapabilities(java.util.Collection numberCapabilities) {
setNumberCapabilities(numberCapabilities);
return this;
}
/**
*
* Indicates if the phone number will be used for text messages, voice messages or both.
*
*
* @param numberCapabilities
* Indicates if the phone number will be used for text messages, voice messages or both.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NumberCapability
*/
public RequestPhoneNumberResult withNumberCapabilities(NumberCapability... numberCapabilities) {
java.util.ArrayList numberCapabilitiesCopy = new java.util.ArrayList(numberCapabilities.length);
for (NumberCapability value : numberCapabilities) {
numberCapabilitiesCopy.add(value.toString());
}
if (getNumberCapabilities() == null) {
setNumberCapabilities(numberCapabilitiesCopy);
} else {
getNumberCapabilities().addAll(numberCapabilitiesCopy);
}
return this;
}
/**
*
* The type of number that was released.
*
*
* @param numberType
* The type of number that was released.
* @see RequestableNumberType
*/
public void setNumberType(String numberType) {
this.numberType = numberType;
}
/**
*
* The type of number that was released.
*
*
* @return The type of number that was released.
* @see RequestableNumberType
*/
public String getNumberType() {
return this.numberType;
}
/**
*
* The type of number that was released.
*
*
* @param numberType
* The type of number that was released.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RequestableNumberType
*/
public RequestPhoneNumberResult withNumberType(String numberType) {
setNumberType(numberType);
return this;
}
/**
*
* The type of number that was released.
*
*
* @param numberType
* The type of number that was released.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RequestableNumberType
*/
public RequestPhoneNumberResult withNumberType(RequestableNumberType numberType) {
this.numberType = numberType.toString();
return this;
}
/**
*
* The monthly price, in US dollars, to lease the phone number.
*
*
* @param monthlyLeasingPrice
* The monthly price, in US dollars, to lease the phone number.
*/
public void setMonthlyLeasingPrice(String monthlyLeasingPrice) {
this.monthlyLeasingPrice = monthlyLeasingPrice;
}
/**
*
* The monthly price, in US dollars, to lease the phone number.
*
*
* @return The monthly price, in US dollars, to lease the phone number.
*/
public String getMonthlyLeasingPrice() {
return this.monthlyLeasingPrice;
}
/**
*
* The monthly price, in US dollars, to lease the phone number.
*
*
* @param monthlyLeasingPrice
* The monthly price, in US dollars, to lease the phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withMonthlyLeasingPrice(String monthlyLeasingPrice) {
setMonthlyLeasingPrice(monthlyLeasingPrice);
return this;
}
/**
*
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*
*
* @param twoWayEnabled
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*/
public void setTwoWayEnabled(Boolean twoWayEnabled) {
this.twoWayEnabled = twoWayEnabled;
}
/**
*
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*
*
* @return By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*/
public Boolean getTwoWayEnabled() {
return this.twoWayEnabled;
}
/**
*
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*
*
* @param twoWayEnabled
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withTwoWayEnabled(Boolean twoWayEnabled) {
setTwoWayEnabled(twoWayEnabled);
return this;
}
/**
*
* By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*
*
* @return By default this is set to false. When set to true you can receive incoming text messages from your end
* recipients.
*/
public Boolean isTwoWayEnabled() {
return this.twoWayEnabled;
}
/**
*
* The ARN used to identify the two way channel.
*
*
* @param twoWayChannelArn
* The ARN used to identify the two way channel.
*/
public void setTwoWayChannelArn(String twoWayChannelArn) {
this.twoWayChannelArn = twoWayChannelArn;
}
/**
*
* The ARN used to identify the two way channel.
*
*
* @return The ARN used to identify the two way channel.
*/
public String getTwoWayChannelArn() {
return this.twoWayChannelArn;
}
/**
*
* The ARN used to identify the two way channel.
*
*
* @param twoWayChannelArn
* The ARN used to identify the two way channel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withTwoWayChannelArn(String twoWayChannelArn) {
setTwoWayChannelArn(twoWayChannelArn);
return this;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @param twoWayChannelRole
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*/
public void setTwoWayChannelRole(String twoWayChannelRole) {
this.twoWayChannelRole = twoWayChannelRole;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @return An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*/
public String getTwoWayChannelRole() {
return this.twoWayChannelRole;
}
/**
*
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
*
*
* @param twoWayChannelRole
* An optional IAM Role Arn for a service to assume, to be able to post inbound SMS messages.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withTwoWayChannelRole(String twoWayChannelRole) {
setTwoWayChannelRole(twoWayChannelRole);
return this;
}
/**
*
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to one of
* your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests.
*
*
* @param selfManagedOptOutsEnabled
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to
* one of your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a
* customizable message and adds the end recipient to the OptOutList. When set to true you're responsible for
* responding to HELP and STOP requests. You're also responsible for tracking and honoring opt-out requests.
*/
public void setSelfManagedOptOutsEnabled(Boolean selfManagedOptOutsEnabled) {
this.selfManagedOptOutsEnabled = selfManagedOptOutsEnabled;
}
/**
*
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to one of
* your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests.
*
*
* @return By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to
* one of your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a
* customizable message and adds the end recipient to the OptOutList. When set to true you're responsible
* for responding to HELP and STOP requests. You're also responsible for tracking and honoring opt-out
* requests.
*/
public Boolean getSelfManagedOptOutsEnabled() {
return this.selfManagedOptOutsEnabled;
}
/**
*
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to one of
* your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests.
*
*
* @param selfManagedOptOutsEnabled
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to
* one of your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a
* customizable message and adds the end recipient to the OptOutList. When set to true you're responsible for
* responding to HELP and STOP requests. You're also responsible for tracking and honoring opt-out requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withSelfManagedOptOutsEnabled(Boolean selfManagedOptOutsEnabled) {
setSelfManagedOptOutsEnabled(selfManagedOptOutsEnabled);
return this;
}
/**
*
* By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to one of
* your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a customizable message
* and adds the end recipient to the OptOutList. When set to true you're responsible for responding to HELP and STOP
* requests. You're also responsible for tracking and honoring opt-out requests.
*
*
* @return By default this is set to false. When an end recipient sends a message that begins with HELP or STOP to
* one of your dedicated numbers, AWS End User Messaging SMS and Voice automatically replies with a
* customizable message and adds the end recipient to the OptOutList. When set to true you're responsible
* for responding to HELP and STOP requests. You're also responsible for tracking and honoring opt-out
* requests.
*/
public Boolean isSelfManagedOptOutsEnabled() {
return this.selfManagedOptOutsEnabled;
}
/**
*
* The name of the OptOutList that is associated with the requested phone number.
*
*
* @param optOutListName
* The name of the OptOutList that is associated with the requested phone number.
*/
public void setOptOutListName(String optOutListName) {
this.optOutListName = optOutListName;
}
/**
*
* The name of the OptOutList that is associated with the requested phone number.
*
*
* @return The name of the OptOutList that is associated with the requested phone number.
*/
public String getOptOutListName() {
return this.optOutListName;
}
/**
*
* The name of the OptOutList that is associated with the requested phone number.
*
*
* @param optOutListName
* The name of the OptOutList that is associated with the requested phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withOptOutListName(String optOutListName) {
setOptOutListName(optOutListName);
return this;
}
/**
*
* By default this is set to false. When set to true the phone number can't be deleted.
*
*
* @param deletionProtectionEnabled
* By default this is set to false. When set to true the phone number can't be deleted.
*/
public void setDeletionProtectionEnabled(Boolean deletionProtectionEnabled) {
this.deletionProtectionEnabled = deletionProtectionEnabled;
}
/**
*
* By default this is set to false. When set to true the phone number can't be deleted.
*
*
* @return By default this is set to false. When set to true the phone number can't be deleted.
*/
public Boolean getDeletionProtectionEnabled() {
return this.deletionProtectionEnabled;
}
/**
*
* By default this is set to false. When set to true the phone number can't be deleted.
*
*
* @param deletionProtectionEnabled
* By default this is set to false. When set to true the phone number can't be deleted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withDeletionProtectionEnabled(Boolean deletionProtectionEnabled) {
setDeletionProtectionEnabled(deletionProtectionEnabled);
return this;
}
/**
*
* By default this is set to false. When set to true the phone number can't be deleted.
*
*
* @return By default this is set to false. When set to true the phone number can't be deleted.
*/
public Boolean isDeletionProtectionEnabled() {
return this.deletionProtectionEnabled;
}
/**
*
* The unique identifier of the pool associated with the phone number
*
*
* @param poolId
* The unique identifier of the pool associated with the phone number
*/
public void setPoolId(String poolId) {
this.poolId = poolId;
}
/**
*
* The unique identifier of the pool associated with the phone number
*
*
* @return The unique identifier of the pool associated with the phone number
*/
public String getPoolId() {
return this.poolId;
}
/**
*
* The unique identifier of the pool associated with the phone number
*
*
* @param poolId
* The unique identifier of the pool associated with the phone number
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withPoolId(String poolId) {
setPoolId(poolId);
return this;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
*/
public void setRegistrationId(String registrationId) {
this.registrationId = registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @return The unique identifier for the registration.
*/
public String getRegistrationId() {
return this.registrationId;
}
/**
*
* The unique identifier for the registration.
*
*
* @param registrationId
* The unique identifier for the registration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withRegistrationId(String registrationId) {
setRegistrationId(registrationId);
return this;
}
/**
*
* An array of key and value pair tags that are associated with the phone number.
*
*
* @return An array of key and value pair tags that are associated with the phone number.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key and value pair tags that are associated with the phone number.
*
*
* @param tags
* An array of key and value pair tags that are associated with the phone number.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key and value pair tags that are associated with the phone number.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key and value pair tags that are associated with the phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key and value pair tags that are associated with the phone number.
*
*
* @param tags
* An array of key and value pair tags that are associated with the phone number.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The time when the phone number was created, in UNIX epoch time
* format.
*
*
* @param createdTimestamp
* The time when the phone number was created, in UNIX epoch
* time format.
*/
public void setCreatedTimestamp(java.util.Date createdTimestamp) {
this.createdTimestamp = createdTimestamp;
}
/**
*
* The time when the phone number was created, in UNIX epoch time
* format.
*
*
* @return The time when the phone number was created, in UNIX epoch
* time format.
*/
public java.util.Date getCreatedTimestamp() {
return this.createdTimestamp;
}
/**
*
* The time when the phone number was created, in UNIX epoch time
* format.
*
*
* @param createdTimestamp
* The time when the phone number was created, in UNIX epoch
* time format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RequestPhoneNumberResult withCreatedTimestamp(java.util.Date createdTimestamp) {
setCreatedTimestamp(createdTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPhoneNumberArn() != null)
sb.append("PhoneNumberArn: ").append(getPhoneNumberArn()).append(",");
if (getPhoneNumberId() != null)
sb.append("PhoneNumberId: ").append(getPhoneNumberId()).append(",");
if (getPhoneNumber() != null)
sb.append("PhoneNumber: ").append(getPhoneNumber()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getIsoCountryCode() != null)
sb.append("IsoCountryCode: ").append(getIsoCountryCode()).append(",");
if (getMessageType() != null)
sb.append("MessageType: ").append(getMessageType()).append(",");
if (getNumberCapabilities() != null)
sb.append("NumberCapabilities: ").append(getNumberCapabilities()).append(",");
if (getNumberType() != null)
sb.append("NumberType: ").append(getNumberType()).append(",");
if (getMonthlyLeasingPrice() != null)
sb.append("MonthlyLeasingPrice: ").append(getMonthlyLeasingPrice()).append(",");
if (getTwoWayEnabled() != null)
sb.append("TwoWayEnabled: ").append(getTwoWayEnabled()).append(",");
if (getTwoWayChannelArn() != null)
sb.append("TwoWayChannelArn: ").append(getTwoWayChannelArn()).append(",");
if (getTwoWayChannelRole() != null)
sb.append("TwoWayChannelRole: ").append(getTwoWayChannelRole()).append(",");
if (getSelfManagedOptOutsEnabled() != null)
sb.append("SelfManagedOptOutsEnabled: ").append(getSelfManagedOptOutsEnabled()).append(",");
if (getOptOutListName() != null)
sb.append("OptOutListName: ").append(getOptOutListName()).append(",");
if (getDeletionProtectionEnabled() != null)
sb.append("DeletionProtectionEnabled: ").append(getDeletionProtectionEnabled()).append(",");
if (getPoolId() != null)
sb.append("PoolId: ").append(getPoolId()).append(",");
if (getRegistrationId() != null)
sb.append("RegistrationId: ").append(getRegistrationId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreatedTimestamp() != null)
sb.append("CreatedTimestamp: ").append(getCreatedTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RequestPhoneNumberResult == false)
return false;
RequestPhoneNumberResult other = (RequestPhoneNumberResult) obj;
if (other.getPhoneNumberArn() == null ^ this.getPhoneNumberArn() == null)
return false;
if (other.getPhoneNumberArn() != null && other.getPhoneNumberArn().equals(this.getPhoneNumberArn()) == false)
return false;
if (other.getPhoneNumberId() == null ^ this.getPhoneNumberId() == null)
return false;
if (other.getPhoneNumberId() != null && other.getPhoneNumberId().equals(this.getPhoneNumberId()) == false)
return false;
if (other.getPhoneNumber() == null ^ this.getPhoneNumber() == null)
return false;
if (other.getPhoneNumber() != null && other.getPhoneNumber().equals(this.getPhoneNumber()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getIsoCountryCode() == null ^ this.getIsoCountryCode() == null)
return false;
if (other.getIsoCountryCode() != null && other.getIsoCountryCode().equals(this.getIsoCountryCode()) == false)
return false;
if (other.getMessageType() == null ^ this.getMessageType() == null)
return false;
if (other.getMessageType() != null && other.getMessageType().equals(this.getMessageType()) == false)
return false;
if (other.getNumberCapabilities() == null ^ this.getNumberCapabilities() == null)
return false;
if (other.getNumberCapabilities() != null && other.getNumberCapabilities().equals(this.getNumberCapabilities()) == false)
return false;
if (other.getNumberType() == null ^ this.getNumberType() == null)
return false;
if (other.getNumberType() != null && other.getNumberType().equals(this.getNumberType()) == false)
return false;
if (other.getMonthlyLeasingPrice() == null ^ this.getMonthlyLeasingPrice() == null)
return false;
if (other.getMonthlyLeasingPrice() != null && other.getMonthlyLeasingPrice().equals(this.getMonthlyLeasingPrice()) == false)
return false;
if (other.getTwoWayEnabled() == null ^ this.getTwoWayEnabled() == null)
return false;
if (other.getTwoWayEnabled() != null && other.getTwoWayEnabled().equals(this.getTwoWayEnabled()) == false)
return false;
if (other.getTwoWayChannelArn() == null ^ this.getTwoWayChannelArn() == null)
return false;
if (other.getTwoWayChannelArn() != null && other.getTwoWayChannelArn().equals(this.getTwoWayChannelArn()) == false)
return false;
if (other.getTwoWayChannelRole() == null ^ this.getTwoWayChannelRole() == null)
return false;
if (other.getTwoWayChannelRole() != null && other.getTwoWayChannelRole().equals(this.getTwoWayChannelRole()) == false)
return false;
if (other.getSelfManagedOptOutsEnabled() == null ^ this.getSelfManagedOptOutsEnabled() == null)
return false;
if (other.getSelfManagedOptOutsEnabled() != null && other.getSelfManagedOptOutsEnabled().equals(this.getSelfManagedOptOutsEnabled()) == false)
return false;
if (other.getOptOutListName() == null ^ this.getOptOutListName() == null)
return false;
if (other.getOptOutListName() != null && other.getOptOutListName().equals(this.getOptOutListName()) == false)
return false;
if (other.getDeletionProtectionEnabled() == null ^ this.getDeletionProtectionEnabled() == null)
return false;
if (other.getDeletionProtectionEnabled() != null && other.getDeletionProtectionEnabled().equals(this.getDeletionProtectionEnabled()) == false)
return false;
if (other.getPoolId() == null ^ this.getPoolId() == null)
return false;
if (other.getPoolId() != null && other.getPoolId().equals(this.getPoolId()) == false)
return false;
if (other.getRegistrationId() == null ^ this.getRegistrationId() == null)
return false;
if (other.getRegistrationId() != null && other.getRegistrationId().equals(this.getRegistrationId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreatedTimestamp() == null ^ this.getCreatedTimestamp() == null)
return false;
if (other.getCreatedTimestamp() != null && other.getCreatedTimestamp().equals(this.getCreatedTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPhoneNumberArn() == null) ? 0 : getPhoneNumberArn().hashCode());
hashCode = prime * hashCode + ((getPhoneNumberId() == null) ? 0 : getPhoneNumberId().hashCode());
hashCode = prime * hashCode + ((getPhoneNumber() == null) ? 0 : getPhoneNumber().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getIsoCountryCode() == null) ? 0 : getIsoCountryCode().hashCode());
hashCode = prime * hashCode + ((getMessageType() == null) ? 0 : getMessageType().hashCode());
hashCode = prime * hashCode + ((getNumberCapabilities() == null) ? 0 : getNumberCapabilities().hashCode());
hashCode = prime * hashCode + ((getNumberType() == null) ? 0 : getNumberType().hashCode());
hashCode = prime * hashCode + ((getMonthlyLeasingPrice() == null) ? 0 : getMonthlyLeasingPrice().hashCode());
hashCode = prime * hashCode + ((getTwoWayEnabled() == null) ? 0 : getTwoWayEnabled().hashCode());
hashCode = prime * hashCode + ((getTwoWayChannelArn() == null) ? 0 : getTwoWayChannelArn().hashCode());
hashCode = prime * hashCode + ((getTwoWayChannelRole() == null) ? 0 : getTwoWayChannelRole().hashCode());
hashCode = prime * hashCode + ((getSelfManagedOptOutsEnabled() == null) ? 0 : getSelfManagedOptOutsEnabled().hashCode());
hashCode = prime * hashCode + ((getOptOutListName() == null) ? 0 : getOptOutListName().hashCode());
hashCode = prime * hashCode + ((getDeletionProtectionEnabled() == null) ? 0 : getDeletionProtectionEnabled().hashCode());
hashCode = prime * hashCode + ((getPoolId() == null) ? 0 : getPoolId().hashCode());
hashCode = prime * hashCode + ((getRegistrationId() == null) ? 0 : getRegistrationId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreatedTimestamp() == null) ? 0 : getCreatedTimestamp().hashCode());
return hashCode;
}
@Override
public RequestPhoneNumberResult clone() {
try {
return (RequestPhoneNumberResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}