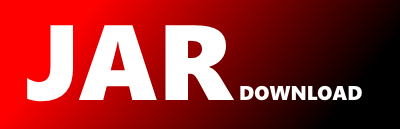
com.amazonaws.services.pipes.AmazonPipes Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pipes Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pipes;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.pipes.model.*;
/**
* Interface for accessing Amazon EventBridge Pipes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.pipes.AbstractAmazonPipes} instead.
*
*
*
* Amazon EventBridge Pipes connects event sources to targets. Pipes reduces the need for specialized knowledge and
* integration code when developing event driven architectures. This helps ensures consistency across your company’s
* applications. With Pipes, the target can be any available EventBridge target. To set up a pipe, you select the event
* source, add optional event filtering, define optional enrichment, and select the target for the event data.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonPipes {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "pipes";
/**
*
* Create a pipe. Amazon EventBridge Pipes connect event sources to targets and reduces the need for specialized
* knowledge and integration code.
*
*
* @param createPipeRequest
* @return Result of the CreatePipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @throws ConflictException
* An action you attempted resulted in an exception.
* @throws ServiceQuotaExceededException
* A quota has been exceeded.
* @sample AmazonPipes.CreatePipe
* @see AWS API
* Documentation
*/
CreatePipeResult createPipe(CreatePipeRequest createPipeRequest);
/**
*
* Delete an existing pipe. For more information about pipes, see Amazon EventBridge Pipes in the
* Amazon EventBridge User Guide.
*
*
* @param deletePipeRequest
* @return Result of the DeletePipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @throws ConflictException
* An action you attempted resulted in an exception.
* @sample AmazonPipes.DeletePipe
* @see AWS API
* Documentation
*/
DeletePipeResult deletePipe(DeletePipeRequest deletePipeRequest);
/**
*
* Get the information about an existing pipe. For more information about pipes, see Amazon EventBridge Pipes in the
* Amazon EventBridge User Guide.
*
*
* @param describePipeRequest
* @return Result of the DescribePipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @sample AmazonPipes.DescribePipe
* @see AWS API
* Documentation
*/
DescribePipeResult describePipe(DescribePipeRequest describePipeRequest);
/**
*
* Get the pipes associated with this account. For more information about pipes, see Amazon EventBridge Pipes in the
* Amazon EventBridge User Guide.
*
*
* @param listPipesRequest
* @return Result of the ListPipes operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @sample AmazonPipes.ListPipes
* @see AWS API
* Documentation
*/
ListPipesResult listPipes(ListPipesRequest listPipesRequest);
/**
*
* Displays the tags associated with a pipe.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws NotFoundException
* An entity that you specified does not exist.
* @sample AmazonPipes.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Start an existing pipe.
*
*
* @param startPipeRequest
* @return Result of the StartPipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @throws ConflictException
* An action you attempted resulted in an exception.
* @sample AmazonPipes.StartPipe
* @see AWS API
* Documentation
*/
StartPipeResult startPipe(StartPipeRequest startPipeRequest);
/**
*
* Stop an existing pipe.
*
*
* @param stopPipeRequest
* @return Result of the StopPipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @throws ConflictException
* An action you attempted resulted in an exception.
* @sample AmazonPipes.StopPipe
* @see AWS API
* Documentation
*/
StopPipeResult stopPipe(StopPipeRequest stopPipeRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified pipe. Tags can help you organize and categorize your
* resources. You can also use them to scope user permissions by granting a user permission to access or change only
* resources with certain tag values.
*
*
* Tags don't have any semantic meaning to Amazon Web Services and are interpreted strictly as strings of
* characters.
*
*
* You can use the TagResource
action with a pipe that already has tags. If you specify a new tag key,
* this tag is appended to the list of tags associated with the pipe. If you specify a tag key that is already
* associated with the pipe, the new tag value that you specify replaces the previous value for that tag.
*
*
* You can associate as many as 50 tags with a pipe.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws NotFoundException
* An entity that you specified does not exist.
* @sample AmazonPipes.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes one or more tags from the specified pipes.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws NotFoundException
* An entity that you specified does not exist.
* @sample AmazonPipes.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Update an existing pipe. When you call UpdatePipe
, EventBridge only the updates fields you have
* specified in the request; the rest remain unchanged. The exception to this is if you modify any Amazon Web
* Services-service specific fields in the SourceParameters
, EnrichmentParameters
, or
* TargetParameters
objects. For example, DynamoDBStreamParameters
or
* EventBridgeEventBusParameters
. EventBridge updates the fields in these objects atomically as one and
* overrides existing values. This is by design, and means that if you don't specify an optional field in one of
* these Parameters
objects, EventBridge sets that field to its system-default value during the update.
*
*
* For more information about pipes, see Amazon EventBridge Pipes in
* the Amazon EventBridge User Guide.
*
*
* @param updatePipeRequest
* @return Result of the UpdatePipe operation returned by the service.
* @throws InternalException
* This exception occurs due to unexpected causes.
* @throws ValidationException
* Indicates that an error has occurred while performing a validate operation.
* @throws ThrottlingException
* An action was throttled.
* @throws NotFoundException
* An entity that you specified does not exist.
* @throws ConflictException
* An action you attempted resulted in an exception.
* @sample AmazonPipes.UpdatePipe
* @see AWS API
* Documentation
*/
UpdatePipeResult updatePipe(UpdatePipeRequest updatePipeRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}