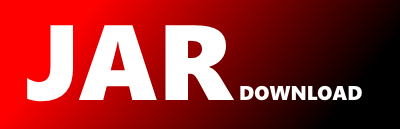
com.amazonaws.services.pipes.model.PipeLogConfigurationParameters Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pipes Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pipes.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Specifies the logging configuration settings for the pipe.
*
*
* When you call UpdatePipe
, EventBridge updates the fields in the
* PipeLogConfigurationParameters
object atomically as one and overrides existing values. This is by
* design. If you don't specify an optional field in any of the Amazon Web Services service parameters objects (
* CloudwatchLogsLogDestinationParameters
, FirehoseLogDestinationParameters
, or
* S3LogDestinationParameters
), EventBridge sets that field to its system-default value during the update.
*
*
* For example, suppose when you created the pipe you specified a Firehose stream log destination. You then update the
* pipe to add an Amazon S3 log destination. In addition to specifying the S3LogDestinationParameters
for
* the new log destination, you must also specify the fields in the FirehoseLogDestinationParameters
object
* in order to retain the Firehose stream log destination.
*
*
* For more information on generating pipe log records, see Log EventBridge Pipes in the Amazon EventBridge User
* Guide.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PipeLogConfigurationParameters implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon S3 logging configuration settings for the pipe.
*
*/
private S3LogDestinationParameters s3LogDestination;
/**
*
* The Amazon Data Firehose logging configuration settings for the pipe.
*
*/
private FirehoseLogDestinationParameters firehoseLogDestination;
/**
*
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
*
*/
private CloudwatchLogsLogDestinationParameters cloudwatchLogsLogDestination;
/**
*
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
*
* For more information, see Specifying
* EventBridge Pipes log level in the Amazon EventBridge User Guide.
*
*/
private String level;
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*/
private java.util.List includeExecutionData;
/**
*
* The Amazon S3 logging configuration settings for the pipe.
*
*
* @param s3LogDestination
* The Amazon S3 logging configuration settings for the pipe.
*/
public void setS3LogDestination(S3LogDestinationParameters s3LogDestination) {
this.s3LogDestination = s3LogDestination;
}
/**
*
* The Amazon S3 logging configuration settings for the pipe.
*
*
* @return The Amazon S3 logging configuration settings for the pipe.
*/
public S3LogDestinationParameters getS3LogDestination() {
return this.s3LogDestination;
}
/**
*
* The Amazon S3 logging configuration settings for the pipe.
*
*
* @param s3LogDestination
* The Amazon S3 logging configuration settings for the pipe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeLogConfigurationParameters withS3LogDestination(S3LogDestinationParameters s3LogDestination) {
setS3LogDestination(s3LogDestination);
return this;
}
/**
*
* The Amazon Data Firehose logging configuration settings for the pipe.
*
*
* @param firehoseLogDestination
* The Amazon Data Firehose logging configuration settings for the pipe.
*/
public void setFirehoseLogDestination(FirehoseLogDestinationParameters firehoseLogDestination) {
this.firehoseLogDestination = firehoseLogDestination;
}
/**
*
* The Amazon Data Firehose logging configuration settings for the pipe.
*
*
* @return The Amazon Data Firehose logging configuration settings for the pipe.
*/
public FirehoseLogDestinationParameters getFirehoseLogDestination() {
return this.firehoseLogDestination;
}
/**
*
* The Amazon Data Firehose logging configuration settings for the pipe.
*
*
* @param firehoseLogDestination
* The Amazon Data Firehose logging configuration settings for the pipe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeLogConfigurationParameters withFirehoseLogDestination(FirehoseLogDestinationParameters firehoseLogDestination) {
setFirehoseLogDestination(firehoseLogDestination);
return this;
}
/**
*
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
*
*
* @param cloudwatchLogsLogDestination
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
*/
public void setCloudwatchLogsLogDestination(CloudwatchLogsLogDestinationParameters cloudwatchLogsLogDestination) {
this.cloudwatchLogsLogDestination = cloudwatchLogsLogDestination;
}
/**
*
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
*
*
* @return The Amazon CloudWatch Logs logging configuration settings for the pipe.
*/
public CloudwatchLogsLogDestinationParameters getCloudwatchLogsLogDestination() {
return this.cloudwatchLogsLogDestination;
}
/**
*
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
*
*
* @param cloudwatchLogsLogDestination
* The Amazon CloudWatch Logs logging configuration settings for the pipe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeLogConfigurationParameters withCloudwatchLogsLogDestination(CloudwatchLogsLogDestinationParameters cloudwatchLogsLogDestination) {
setCloudwatchLogsLogDestination(cloudwatchLogsLogDestination);
return this;
}
/**
*
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
*
* For more information, see Specifying
* EventBridge Pipes log level in the Amazon EventBridge User Guide.
*
*
* @param level
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
* For more information, see Specifying EventBridge Pipes log level in the Amazon EventBridge User Guide.
* @see LogLevel
*/
public void setLevel(String level) {
this.level = level;
}
/**
*
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
*
* For more information, see Specifying
* EventBridge Pipes log level in the Amazon EventBridge User Guide.
*
*
* @return The level of logging detail to include. This applies to all log destinations for the pipe.
*
* For more information, see Specifying EventBridge Pipes log level in the Amazon EventBridge User Guide.
* @see LogLevel
*/
public String getLevel() {
return this.level;
}
/**
*
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
*
* For more information, see Specifying
* EventBridge Pipes log level in the Amazon EventBridge User Guide.
*
*
* @param level
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
* For more information, see Specifying EventBridge Pipes log level in the Amazon EventBridge User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LogLevel
*/
public PipeLogConfigurationParameters withLevel(String level) {
setLevel(level);
return this;
}
/**
*
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
*
* For more information, see Specifying
* EventBridge Pipes log level in the Amazon EventBridge User Guide.
*
*
* @param level
* The level of logging detail to include. This applies to all log destinations for the pipe.
*
* For more information, see Specifying EventBridge Pipes log level in the Amazon EventBridge User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LogLevel
*/
public PipeLogConfigurationParameters withLevel(LogLevel level) {
this.level = level.toString();
return this;
}
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*
* @return Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
* @see IncludeExecutionDataOption
*/
public java.util.List getIncludeExecutionData() {
return includeExecutionData;
}
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*
* @param includeExecutionData
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
* @see IncludeExecutionDataOption
*/
public void setIncludeExecutionData(java.util.Collection includeExecutionData) {
if (includeExecutionData == null) {
this.includeExecutionData = null;
return;
}
this.includeExecutionData = new java.util.ArrayList(includeExecutionData);
}
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludeExecutionData(java.util.Collection)} or {@link #withIncludeExecutionData(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param includeExecutionData
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IncludeExecutionDataOption
*/
public PipeLogConfigurationParameters withIncludeExecutionData(String... includeExecutionData) {
if (this.includeExecutionData == null) {
setIncludeExecutionData(new java.util.ArrayList(includeExecutionData.length));
}
for (String ele : includeExecutionData) {
this.includeExecutionData.add(ele);
}
return this;
}
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*
* @param includeExecutionData
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IncludeExecutionDataOption
*/
public PipeLogConfigurationParameters withIncludeExecutionData(java.util.Collection includeExecutionData) {
setIncludeExecutionData(includeExecutionData);
return this;
}
/**
*
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
*
*
* @param includeExecutionData
* Specify ALL
to include the execution data (specifically, the payload
,
* awsRequest
, and awsResponse
fields) in the log messages for this pipe.
*
* This applies to all log destinations for the pipe.
*
*
* For more information, see Including execution data in logs in the Amazon EventBridge User Guide.
*
*
* By default, execution data is not included.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IncludeExecutionDataOption
*/
public PipeLogConfigurationParameters withIncludeExecutionData(IncludeExecutionDataOption... includeExecutionData) {
java.util.ArrayList includeExecutionDataCopy = new java.util.ArrayList(includeExecutionData.length);
for (IncludeExecutionDataOption value : includeExecutionData) {
includeExecutionDataCopy.add(value.toString());
}
if (getIncludeExecutionData() == null) {
setIncludeExecutionData(includeExecutionDataCopy);
} else {
getIncludeExecutionData().addAll(includeExecutionDataCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getS3LogDestination() != null)
sb.append("S3LogDestination: ").append(getS3LogDestination()).append(",");
if (getFirehoseLogDestination() != null)
sb.append("FirehoseLogDestination: ").append(getFirehoseLogDestination()).append(",");
if (getCloudwatchLogsLogDestination() != null)
sb.append("CloudwatchLogsLogDestination: ").append(getCloudwatchLogsLogDestination()).append(",");
if (getLevel() != null)
sb.append("Level: ").append(getLevel()).append(",");
if (getIncludeExecutionData() != null)
sb.append("IncludeExecutionData: ").append(getIncludeExecutionData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PipeLogConfigurationParameters == false)
return false;
PipeLogConfigurationParameters other = (PipeLogConfigurationParameters) obj;
if (other.getS3LogDestination() == null ^ this.getS3LogDestination() == null)
return false;
if (other.getS3LogDestination() != null && other.getS3LogDestination().equals(this.getS3LogDestination()) == false)
return false;
if (other.getFirehoseLogDestination() == null ^ this.getFirehoseLogDestination() == null)
return false;
if (other.getFirehoseLogDestination() != null && other.getFirehoseLogDestination().equals(this.getFirehoseLogDestination()) == false)
return false;
if (other.getCloudwatchLogsLogDestination() == null ^ this.getCloudwatchLogsLogDestination() == null)
return false;
if (other.getCloudwatchLogsLogDestination() != null && other.getCloudwatchLogsLogDestination().equals(this.getCloudwatchLogsLogDestination()) == false)
return false;
if (other.getLevel() == null ^ this.getLevel() == null)
return false;
if (other.getLevel() != null && other.getLevel().equals(this.getLevel()) == false)
return false;
if (other.getIncludeExecutionData() == null ^ this.getIncludeExecutionData() == null)
return false;
if (other.getIncludeExecutionData() != null && other.getIncludeExecutionData().equals(this.getIncludeExecutionData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getS3LogDestination() == null) ? 0 : getS3LogDestination().hashCode());
hashCode = prime * hashCode + ((getFirehoseLogDestination() == null) ? 0 : getFirehoseLogDestination().hashCode());
hashCode = prime * hashCode + ((getCloudwatchLogsLogDestination() == null) ? 0 : getCloudwatchLogsLogDestination().hashCode());
hashCode = prime * hashCode + ((getLevel() == null) ? 0 : getLevel().hashCode());
hashCode = prime * hashCode + ((getIncludeExecutionData() == null) ? 0 : getIncludeExecutionData().hashCode());
return hashCode;
}
@Override
public PipeLogConfigurationParameters clone() {
try {
return (PipeLogConfigurationParameters) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.pipes.model.transform.PipeLogConfigurationParametersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}