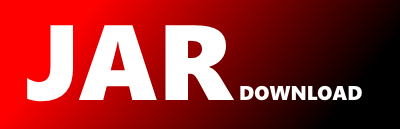
com.amazonaws.services.pipes.model.PipeTargetTimestreamParameters Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pipes Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pipes.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The parameters for using a Timestream for LiveAnalytics table as a target.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PipeTargetTimestreamParameters implements Serializable, Cloneable, StructuredPojo {
/**
*
* Dynamic path to the source data field that represents the time value for your data.
*
*/
private String timeValue;
/**
*
* The granularity of the time units used. Default is MILLISECONDS
.
*
*
* Required if TimeFieldType
is specified as EPOCH
.
*
*/
private String epochTimeUnit;
/**
*
* The type of time value used.
*
*
* The default is EPOCH
.
*
*/
private String timeFieldType;
/**
*
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*
*/
private String timestampFormat;
/**
*
* 64 bit version value or source data field that represents the version value for your data.
*
*
* Write requests with a higher version number will update the existing measure values of the record and version. In
* cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default value
* is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a ValidationException
* error.
*
*
*/
private String versionValue;
/**
*
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*
*/
private java.util.List dimensionMappings;
/**
*
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics table.
*
*/
private java.util.List singleMeasureMappings;
/**
*
* Maps multiple measures from the source event to the same record in the specified Timestream for LiveAnalytics
* table.
*
*/
private java.util.List multiMeasureMappings;
/**
*
* Dynamic path to the source data field that represents the time value for your data.
*
*
* @param timeValue
* Dynamic path to the source data field that represents the time value for your data.
*/
public void setTimeValue(String timeValue) {
this.timeValue = timeValue;
}
/**
*
* Dynamic path to the source data field that represents the time value for your data.
*
*
* @return Dynamic path to the source data field that represents the time value for your data.
*/
public String getTimeValue() {
return this.timeValue;
}
/**
*
* Dynamic path to the source data field that represents the time value for your data.
*
*
* @param timeValue
* Dynamic path to the source data field that represents the time value for your data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withTimeValue(String timeValue) {
setTimeValue(timeValue);
return this;
}
/**
*
* The granularity of the time units used. Default is MILLISECONDS
.
*
*
* Required if TimeFieldType
is specified as EPOCH
.
*
*
* @param epochTimeUnit
* The granularity of the time units used. Default is MILLISECONDS
.
*
* Required if TimeFieldType
is specified as EPOCH
.
* @see EpochTimeUnit
*/
public void setEpochTimeUnit(String epochTimeUnit) {
this.epochTimeUnit = epochTimeUnit;
}
/**
*
* The granularity of the time units used. Default is MILLISECONDS
.
*
*
* Required if TimeFieldType
is specified as EPOCH
.
*
*
* @return The granularity of the time units used. Default is MILLISECONDS
.
*
* Required if TimeFieldType
is specified as EPOCH
.
* @see EpochTimeUnit
*/
public String getEpochTimeUnit() {
return this.epochTimeUnit;
}
/**
*
* The granularity of the time units used. Default is MILLISECONDS
.
*
*
* Required if TimeFieldType
is specified as EPOCH
.
*
*
* @param epochTimeUnit
* The granularity of the time units used. Default is MILLISECONDS
.
*
* Required if TimeFieldType
is specified as EPOCH
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EpochTimeUnit
*/
public PipeTargetTimestreamParameters withEpochTimeUnit(String epochTimeUnit) {
setEpochTimeUnit(epochTimeUnit);
return this;
}
/**
*
* The granularity of the time units used. Default is MILLISECONDS
.
*
*
* Required if TimeFieldType
is specified as EPOCH
.
*
*
* @param epochTimeUnit
* The granularity of the time units used. Default is MILLISECONDS
.
*
* Required if TimeFieldType
is specified as EPOCH
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EpochTimeUnit
*/
public PipeTargetTimestreamParameters withEpochTimeUnit(EpochTimeUnit epochTimeUnit) {
this.epochTimeUnit = epochTimeUnit.toString();
return this;
}
/**
*
* The type of time value used.
*
*
* The default is EPOCH
.
*
*
* @param timeFieldType
* The type of time value used.
*
* The default is EPOCH
.
* @see TimeFieldType
*/
public void setTimeFieldType(String timeFieldType) {
this.timeFieldType = timeFieldType;
}
/**
*
* The type of time value used.
*
*
* The default is EPOCH
.
*
*
* @return The type of time value used.
*
* The default is EPOCH
.
* @see TimeFieldType
*/
public String getTimeFieldType() {
return this.timeFieldType;
}
/**
*
* The type of time value used.
*
*
* The default is EPOCH
.
*
*
* @param timeFieldType
* The type of time value used.
*
* The default is EPOCH
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TimeFieldType
*/
public PipeTargetTimestreamParameters withTimeFieldType(String timeFieldType) {
setTimeFieldType(timeFieldType);
return this;
}
/**
*
* The type of time value used.
*
*
* The default is EPOCH
.
*
*
* @param timeFieldType
* The type of time value used.
*
* The default is EPOCH
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TimeFieldType
*/
public PipeTargetTimestreamParameters withTimeFieldType(TimeFieldType timeFieldType) {
this.timeFieldType = timeFieldType.toString();
return this;
}
/**
*
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*
*
* @param timestampFormat
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*/
public void setTimestampFormat(String timestampFormat) {
this.timestampFormat = timestampFormat;
}
/**
*
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*
*
* @return How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*/
public String getTimestampFormat() {
return this.timestampFormat;
}
/**
*
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
*
*
* @param timestampFormat
* How to format the timestamps. For example, YYYY-MM-DDThh:mm:ss.sssTZD
.
*
* Required if TimeFieldType
is specified as TIMESTAMP_FORMAT
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withTimestampFormat(String timestampFormat) {
setTimestampFormat(timestampFormat);
return this;
}
/**
*
* 64 bit version value or source data field that represents the version value for your data.
*
*
* Write requests with a higher version number will update the existing measure values of the record and version. In
* cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default value
* is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a ValidationException
* error.
*
*
*
* @param versionValue
* 64 bit version value or source data field that represents the version value for your data.
*
* Write requests with a higher version number will update the existing measure values of the record and
* version. In cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default
* value is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a
* ValidationException
error.
*
*/
public void setVersionValue(String versionValue) {
this.versionValue = versionValue;
}
/**
*
* 64 bit version value or source data field that represents the version value for your data.
*
*
* Write requests with a higher version number will update the existing measure values of the record and version. In
* cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default value
* is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a ValidationException
* error.
*
*
*
* @return 64 bit version value or source data field that represents the version value for your data.
*
* Write requests with a higher version number will update the existing measure values of the record and
* version. In cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated.
* Default value is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a
* ValidationException
error.
*
*/
public String getVersionValue() {
return this.versionValue;
}
/**
*
* 64 bit version value or source data field that represents the version value for your data.
*
*
* Write requests with a higher version number will update the existing measure values of the record and version. In
* cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default value
* is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a ValidationException
* error.
*
*
*
* @param versionValue
* 64 bit version value or source data field that represents the version value for your data.
*
* Write requests with a higher version number will update the existing measure values of the record and
* version. In cases where the measure value is the same, the version will still be updated.
*
*
* Default value is 1.
*
*
* Timestream for LiveAnalytics does not support updating partial measure values in a record.
*
*
* Write requests for duplicate data with a higher version number will update the existing measure value and
* version. In cases where the measure value is the same, Version
will still be updated. Default
* value is 1
.
*
*
*
* Version
must be 1
or greater, or you will receive a
* ValidationException
error.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withVersionValue(String versionValue) {
setVersionValue(versionValue);
return this;
}
/**
*
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*
*
* @return Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*/
public java.util.List getDimensionMappings() {
return dimensionMappings;
}
/**
*
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*
*
* @param dimensionMappings
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*/
public void setDimensionMappings(java.util.Collection dimensionMappings) {
if (dimensionMappings == null) {
this.dimensionMappings = null;
return;
}
this.dimensionMappings = new java.util.ArrayList(dimensionMappings);
}
/**
*
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDimensionMappings(java.util.Collection)} or {@link #withDimensionMappings(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param dimensionMappings
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withDimensionMappings(DimensionMapping... dimensionMappings) {
if (this.dimensionMappings == null) {
setDimensionMappings(new java.util.ArrayList(dimensionMappings.length));
}
for (DimensionMapping ele : dimensionMappings) {
this.dimensionMappings.add(ele);
}
return this;
}
/**
*
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
*
*
* @param dimensionMappings
* Map source data to dimensions in the target Timestream for LiveAnalytics table.
*
* For more information, see Amazon Timestream for
* LiveAnalytics concepts
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withDimensionMappings(java.util.Collection dimensionMappings) {
setDimensionMappings(dimensionMappings);
return this;
}
/**
*
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics table.
*
*
* @return Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics
* table.
*/
public java.util.List getSingleMeasureMappings() {
return singleMeasureMappings;
}
/**
*
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics table.
*
*
* @param singleMeasureMappings
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics
* table.
*/
public void setSingleMeasureMappings(java.util.Collection singleMeasureMappings) {
if (singleMeasureMappings == null) {
this.singleMeasureMappings = null;
return;
}
this.singleMeasureMappings = new java.util.ArrayList(singleMeasureMappings);
}
/**
*
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics table.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSingleMeasureMappings(java.util.Collection)} or
* {@link #withSingleMeasureMappings(java.util.Collection)} if you want to override the existing values.
*
*
* @param singleMeasureMappings
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics
* table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withSingleMeasureMappings(SingleMeasureMapping... singleMeasureMappings) {
if (this.singleMeasureMappings == null) {
setSingleMeasureMappings(new java.util.ArrayList(singleMeasureMappings.length));
}
for (SingleMeasureMapping ele : singleMeasureMappings) {
this.singleMeasureMappings.add(ele);
}
return this;
}
/**
*
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics table.
*
*
* @param singleMeasureMappings
* Mappings of single source data fields to individual records in the specified Timestream for LiveAnalytics
* table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withSingleMeasureMappings(java.util.Collection singleMeasureMappings) {
setSingleMeasureMappings(singleMeasureMappings);
return this;
}
/**
*
* Maps multiple measures from the source event to the same record in the specified Timestream for LiveAnalytics
* table.
*
*
* @return Maps multiple measures from the source event to the same record in the specified Timestream for
* LiveAnalytics table.
*/
public java.util.List getMultiMeasureMappings() {
return multiMeasureMappings;
}
/**
*
* Maps multiple measures from the source event to the same record in the specified Timestream for LiveAnalytics
* table.
*
*
* @param multiMeasureMappings
* Maps multiple measures from the source event to the same record in the specified Timestream for
* LiveAnalytics table.
*/
public void setMultiMeasureMappings(java.util.Collection multiMeasureMappings) {
if (multiMeasureMappings == null) {
this.multiMeasureMappings = null;
return;
}
this.multiMeasureMappings = new java.util.ArrayList(multiMeasureMappings);
}
/**
*
* Maps multiple measures from the source event to the same record in the specified Timestream for LiveAnalytics
* table.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMultiMeasureMappings(java.util.Collection)} or {@link #withMultiMeasureMappings(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param multiMeasureMappings
* Maps multiple measures from the source event to the same record in the specified Timestream for
* LiveAnalytics table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withMultiMeasureMappings(MultiMeasureMapping... multiMeasureMappings) {
if (this.multiMeasureMappings == null) {
setMultiMeasureMappings(new java.util.ArrayList(multiMeasureMappings.length));
}
for (MultiMeasureMapping ele : multiMeasureMappings) {
this.multiMeasureMappings.add(ele);
}
return this;
}
/**
*
* Maps multiple measures from the source event to the same record in the specified Timestream for LiveAnalytics
* table.
*
*
* @param multiMeasureMappings
* Maps multiple measures from the source event to the same record in the specified Timestream for
* LiveAnalytics table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipeTargetTimestreamParameters withMultiMeasureMappings(java.util.Collection multiMeasureMappings) {
setMultiMeasureMappings(multiMeasureMappings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTimeValue() != null)
sb.append("TimeValue: ").append(getTimeValue()).append(",");
if (getEpochTimeUnit() != null)
sb.append("EpochTimeUnit: ").append(getEpochTimeUnit()).append(",");
if (getTimeFieldType() != null)
sb.append("TimeFieldType: ").append(getTimeFieldType()).append(",");
if (getTimestampFormat() != null)
sb.append("TimestampFormat: ").append(getTimestampFormat()).append(",");
if (getVersionValue() != null)
sb.append("VersionValue: ").append(getVersionValue()).append(",");
if (getDimensionMappings() != null)
sb.append("DimensionMappings: ").append(getDimensionMappings()).append(",");
if (getSingleMeasureMappings() != null)
sb.append("SingleMeasureMappings: ").append(getSingleMeasureMappings()).append(",");
if (getMultiMeasureMappings() != null)
sb.append("MultiMeasureMappings: ").append(getMultiMeasureMappings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PipeTargetTimestreamParameters == false)
return false;
PipeTargetTimestreamParameters other = (PipeTargetTimestreamParameters) obj;
if (other.getTimeValue() == null ^ this.getTimeValue() == null)
return false;
if (other.getTimeValue() != null && other.getTimeValue().equals(this.getTimeValue()) == false)
return false;
if (other.getEpochTimeUnit() == null ^ this.getEpochTimeUnit() == null)
return false;
if (other.getEpochTimeUnit() != null && other.getEpochTimeUnit().equals(this.getEpochTimeUnit()) == false)
return false;
if (other.getTimeFieldType() == null ^ this.getTimeFieldType() == null)
return false;
if (other.getTimeFieldType() != null && other.getTimeFieldType().equals(this.getTimeFieldType()) == false)
return false;
if (other.getTimestampFormat() == null ^ this.getTimestampFormat() == null)
return false;
if (other.getTimestampFormat() != null && other.getTimestampFormat().equals(this.getTimestampFormat()) == false)
return false;
if (other.getVersionValue() == null ^ this.getVersionValue() == null)
return false;
if (other.getVersionValue() != null && other.getVersionValue().equals(this.getVersionValue()) == false)
return false;
if (other.getDimensionMappings() == null ^ this.getDimensionMappings() == null)
return false;
if (other.getDimensionMappings() != null && other.getDimensionMappings().equals(this.getDimensionMappings()) == false)
return false;
if (other.getSingleMeasureMappings() == null ^ this.getSingleMeasureMappings() == null)
return false;
if (other.getSingleMeasureMappings() != null && other.getSingleMeasureMappings().equals(this.getSingleMeasureMappings()) == false)
return false;
if (other.getMultiMeasureMappings() == null ^ this.getMultiMeasureMappings() == null)
return false;
if (other.getMultiMeasureMappings() != null && other.getMultiMeasureMappings().equals(this.getMultiMeasureMappings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTimeValue() == null) ? 0 : getTimeValue().hashCode());
hashCode = prime * hashCode + ((getEpochTimeUnit() == null) ? 0 : getEpochTimeUnit().hashCode());
hashCode = prime * hashCode + ((getTimeFieldType() == null) ? 0 : getTimeFieldType().hashCode());
hashCode = prime * hashCode + ((getTimestampFormat() == null) ? 0 : getTimestampFormat().hashCode());
hashCode = prime * hashCode + ((getVersionValue() == null) ? 0 : getVersionValue().hashCode());
hashCode = prime * hashCode + ((getDimensionMappings() == null) ? 0 : getDimensionMappings().hashCode());
hashCode = prime * hashCode + ((getSingleMeasureMappings() == null) ? 0 : getSingleMeasureMappings().hashCode());
hashCode = prime * hashCode + ((getMultiMeasureMappings() == null) ? 0 : getMultiMeasureMappings().hashCode());
return hashCode;
}
@Override
public PipeTargetTimestreamParameters clone() {
try {
return (PipeTargetTimestreamParameters) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.pipes.model.transform.PipeTargetTimestreamParametersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}