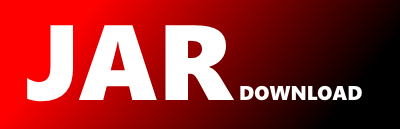
com.amazonaws.services.pipes.model.UpdatePipeSourceParameters Maven / Gradle / Ivy
Show all versions of aws-java-sdk-pipes Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.pipes.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The parameters required to set up a source for your pipe.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdatePipeSourceParameters implements Serializable, Cloneable, StructuredPojo {
/**
*
* The collection of event patterns used to filter events.
*
*
* To remove a filter, specify a FilterCriteria
object with an empty array of Filter
* objects.
*
*
* For more information, see Events and
* Event Patterns in the Amazon EventBridge User Guide.
*
*/
private FilterCriteria filterCriteria;
/**
*
* The parameters for using a Kinesis stream as a source.
*
*/
private UpdatePipeSourceKinesisStreamParameters kinesisStreamParameters;
/**
*
* The parameters for using a DynamoDB stream as a source.
*
*/
private UpdatePipeSourceDynamoDBStreamParameters dynamoDBStreamParameters;
/**
*
* The parameters for using a Amazon SQS stream as a source.
*
*/
private UpdatePipeSourceSqsQueueParameters sqsQueueParameters;
/**
*
* The parameters for using an Active MQ broker as a source.
*
*/
private UpdatePipeSourceActiveMQBrokerParameters activeMQBrokerParameters;
/**
*
* The parameters for using a Rabbit MQ broker as a source.
*
*/
private UpdatePipeSourceRabbitMQBrokerParameters rabbitMQBrokerParameters;
/**
*
* The parameters for using an MSK stream as a source.
*
*/
private UpdatePipeSourceManagedStreamingKafkaParameters managedStreamingKafkaParameters;
/**
*
* The parameters for using a self-managed Apache Kafka stream as a source.
*
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This includes
* both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka,
* or Redpanda. For more information, see Apache Kafka streams as a
* source in the Amazon EventBridge User Guide.
*
*/
private UpdatePipeSourceSelfManagedKafkaParameters selfManagedKafkaParameters;
/**
*
* The collection of event patterns used to filter events.
*
*
* To remove a filter, specify a FilterCriteria
object with an empty array of Filter
* objects.
*
*
* For more information, see Events and
* Event Patterns in the Amazon EventBridge User Guide.
*
*
* @param filterCriteria
* The collection of event patterns used to filter events.
*
* To remove a filter, specify a FilterCriteria
object with an empty array of
* Filter
objects.
*
*
* For more information, see Events
* and Event Patterns in the Amazon EventBridge User Guide.
*/
public void setFilterCriteria(FilterCriteria filterCriteria) {
this.filterCriteria = filterCriteria;
}
/**
*
* The collection of event patterns used to filter events.
*
*
* To remove a filter, specify a FilterCriteria
object with an empty array of Filter
* objects.
*
*
* For more information, see Events and
* Event Patterns in the Amazon EventBridge User Guide.
*
*
* @return The collection of event patterns used to filter events.
*
* To remove a filter, specify a FilterCriteria
object with an empty array of
* Filter
objects.
*
*
* For more information, see Events and Event Patterns in the Amazon EventBridge User Guide.
*/
public FilterCriteria getFilterCriteria() {
return this.filterCriteria;
}
/**
*
* The collection of event patterns used to filter events.
*
*
* To remove a filter, specify a FilterCriteria
object with an empty array of Filter
* objects.
*
*
* For more information, see Events and
* Event Patterns in the Amazon EventBridge User Guide.
*
*
* @param filterCriteria
* The collection of event patterns used to filter events.
*
* To remove a filter, specify a FilterCriteria
object with an empty array of
* Filter
objects.
*
*
* For more information, see Events
* and Event Patterns in the Amazon EventBridge User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withFilterCriteria(FilterCriteria filterCriteria) {
setFilterCriteria(filterCriteria);
return this;
}
/**
*
* The parameters for using a Kinesis stream as a source.
*
*
* @param kinesisStreamParameters
* The parameters for using a Kinesis stream as a source.
*/
public void setKinesisStreamParameters(UpdatePipeSourceKinesisStreamParameters kinesisStreamParameters) {
this.kinesisStreamParameters = kinesisStreamParameters;
}
/**
*
* The parameters for using a Kinesis stream as a source.
*
*
* @return The parameters for using a Kinesis stream as a source.
*/
public UpdatePipeSourceKinesisStreamParameters getKinesisStreamParameters() {
return this.kinesisStreamParameters;
}
/**
*
* The parameters for using a Kinesis stream as a source.
*
*
* @param kinesisStreamParameters
* The parameters for using a Kinesis stream as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withKinesisStreamParameters(UpdatePipeSourceKinesisStreamParameters kinesisStreamParameters) {
setKinesisStreamParameters(kinesisStreamParameters);
return this;
}
/**
*
* The parameters for using a DynamoDB stream as a source.
*
*
* @param dynamoDBStreamParameters
* The parameters for using a DynamoDB stream as a source.
*/
public void setDynamoDBStreamParameters(UpdatePipeSourceDynamoDBStreamParameters dynamoDBStreamParameters) {
this.dynamoDBStreamParameters = dynamoDBStreamParameters;
}
/**
*
* The parameters for using a DynamoDB stream as a source.
*
*
* @return The parameters for using a DynamoDB stream as a source.
*/
public UpdatePipeSourceDynamoDBStreamParameters getDynamoDBStreamParameters() {
return this.dynamoDBStreamParameters;
}
/**
*
* The parameters for using a DynamoDB stream as a source.
*
*
* @param dynamoDBStreamParameters
* The parameters for using a DynamoDB stream as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withDynamoDBStreamParameters(UpdatePipeSourceDynamoDBStreamParameters dynamoDBStreamParameters) {
setDynamoDBStreamParameters(dynamoDBStreamParameters);
return this;
}
/**
*
* The parameters for using a Amazon SQS stream as a source.
*
*
* @param sqsQueueParameters
* The parameters for using a Amazon SQS stream as a source.
*/
public void setSqsQueueParameters(UpdatePipeSourceSqsQueueParameters sqsQueueParameters) {
this.sqsQueueParameters = sqsQueueParameters;
}
/**
*
* The parameters for using a Amazon SQS stream as a source.
*
*
* @return The parameters for using a Amazon SQS stream as a source.
*/
public UpdatePipeSourceSqsQueueParameters getSqsQueueParameters() {
return this.sqsQueueParameters;
}
/**
*
* The parameters for using a Amazon SQS stream as a source.
*
*
* @param sqsQueueParameters
* The parameters for using a Amazon SQS stream as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withSqsQueueParameters(UpdatePipeSourceSqsQueueParameters sqsQueueParameters) {
setSqsQueueParameters(sqsQueueParameters);
return this;
}
/**
*
* The parameters for using an Active MQ broker as a source.
*
*
* @param activeMQBrokerParameters
* The parameters for using an Active MQ broker as a source.
*/
public void setActiveMQBrokerParameters(UpdatePipeSourceActiveMQBrokerParameters activeMQBrokerParameters) {
this.activeMQBrokerParameters = activeMQBrokerParameters;
}
/**
*
* The parameters for using an Active MQ broker as a source.
*
*
* @return The parameters for using an Active MQ broker as a source.
*/
public UpdatePipeSourceActiveMQBrokerParameters getActiveMQBrokerParameters() {
return this.activeMQBrokerParameters;
}
/**
*
* The parameters for using an Active MQ broker as a source.
*
*
* @param activeMQBrokerParameters
* The parameters for using an Active MQ broker as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withActiveMQBrokerParameters(UpdatePipeSourceActiveMQBrokerParameters activeMQBrokerParameters) {
setActiveMQBrokerParameters(activeMQBrokerParameters);
return this;
}
/**
*
* The parameters for using a Rabbit MQ broker as a source.
*
*
* @param rabbitMQBrokerParameters
* The parameters for using a Rabbit MQ broker as a source.
*/
public void setRabbitMQBrokerParameters(UpdatePipeSourceRabbitMQBrokerParameters rabbitMQBrokerParameters) {
this.rabbitMQBrokerParameters = rabbitMQBrokerParameters;
}
/**
*
* The parameters for using a Rabbit MQ broker as a source.
*
*
* @return The parameters for using a Rabbit MQ broker as a source.
*/
public UpdatePipeSourceRabbitMQBrokerParameters getRabbitMQBrokerParameters() {
return this.rabbitMQBrokerParameters;
}
/**
*
* The parameters for using a Rabbit MQ broker as a source.
*
*
* @param rabbitMQBrokerParameters
* The parameters for using a Rabbit MQ broker as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withRabbitMQBrokerParameters(UpdatePipeSourceRabbitMQBrokerParameters rabbitMQBrokerParameters) {
setRabbitMQBrokerParameters(rabbitMQBrokerParameters);
return this;
}
/**
*
* The parameters for using an MSK stream as a source.
*
*
* @param managedStreamingKafkaParameters
* The parameters for using an MSK stream as a source.
*/
public void setManagedStreamingKafkaParameters(UpdatePipeSourceManagedStreamingKafkaParameters managedStreamingKafkaParameters) {
this.managedStreamingKafkaParameters = managedStreamingKafkaParameters;
}
/**
*
* The parameters for using an MSK stream as a source.
*
*
* @return The parameters for using an MSK stream as a source.
*/
public UpdatePipeSourceManagedStreamingKafkaParameters getManagedStreamingKafkaParameters() {
return this.managedStreamingKafkaParameters;
}
/**
*
* The parameters for using an MSK stream as a source.
*
*
* @param managedStreamingKafkaParameters
* The parameters for using an MSK stream as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withManagedStreamingKafkaParameters(UpdatePipeSourceManagedStreamingKafkaParameters managedStreamingKafkaParameters) {
setManagedStreamingKafkaParameters(managedStreamingKafkaParameters);
return this;
}
/**
*
* The parameters for using a self-managed Apache Kafka stream as a source.
*
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This includes
* both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka,
* or Redpanda. For more information, see Apache Kafka streams as a
* source in the Amazon EventBridge User Guide.
*
*
* @param selfManagedKafkaParameters
* The parameters for using a self-managed Apache Kafka stream as a source.
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This
* includes both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka, or Redpanda.
* For more information, see Apache Kafka streams
* as a source in the Amazon EventBridge User Guide.
*/
public void setSelfManagedKafkaParameters(UpdatePipeSourceSelfManagedKafkaParameters selfManagedKafkaParameters) {
this.selfManagedKafkaParameters = selfManagedKafkaParameters;
}
/**
*
* The parameters for using a self-managed Apache Kafka stream as a source.
*
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This includes
* both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka,
* or Redpanda. For more information, see Apache Kafka streams as a
* source in the Amazon EventBridge User Guide.
*
*
* @return The parameters for using a self-managed Apache Kafka stream as a source.
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This
* includes both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka, or Redpanda.
* For more information, see Apache Kafka streams
* as a source in the Amazon EventBridge User Guide.
*/
public UpdatePipeSourceSelfManagedKafkaParameters getSelfManagedKafkaParameters() {
return this.selfManagedKafkaParameters;
}
/**
*
* The parameters for using a self-managed Apache Kafka stream as a source.
*
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This includes
* both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka,
* or Redpanda. For more information, see Apache Kafka streams as a
* source in the Amazon EventBridge User Guide.
*
*
* @param selfManagedKafkaParameters
* The parameters for using a self-managed Apache Kafka stream as a source.
*
* A self managed cluster refers to any Apache Kafka cluster not hosted by Amazon Web Services. This
* includes both clusters you manage yourself, as well as those hosted by a third-party provider, such as Confluent Cloud, CloudKarafka, or Redpanda.
* For more information, see Apache Kafka streams
* as a source in the Amazon EventBridge User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePipeSourceParameters withSelfManagedKafkaParameters(UpdatePipeSourceSelfManagedKafkaParameters selfManagedKafkaParameters) {
setSelfManagedKafkaParameters(selfManagedKafkaParameters);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFilterCriteria() != null)
sb.append("FilterCriteria: ").append(getFilterCriteria()).append(",");
if (getKinesisStreamParameters() != null)
sb.append("KinesisStreamParameters: ").append(getKinesisStreamParameters()).append(",");
if (getDynamoDBStreamParameters() != null)
sb.append("DynamoDBStreamParameters: ").append(getDynamoDBStreamParameters()).append(",");
if (getSqsQueueParameters() != null)
sb.append("SqsQueueParameters: ").append(getSqsQueueParameters()).append(",");
if (getActiveMQBrokerParameters() != null)
sb.append("ActiveMQBrokerParameters: ").append(getActiveMQBrokerParameters()).append(",");
if (getRabbitMQBrokerParameters() != null)
sb.append("RabbitMQBrokerParameters: ").append(getRabbitMQBrokerParameters()).append(",");
if (getManagedStreamingKafkaParameters() != null)
sb.append("ManagedStreamingKafkaParameters: ").append(getManagedStreamingKafkaParameters()).append(",");
if (getSelfManagedKafkaParameters() != null)
sb.append("SelfManagedKafkaParameters: ").append(getSelfManagedKafkaParameters());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdatePipeSourceParameters == false)
return false;
UpdatePipeSourceParameters other = (UpdatePipeSourceParameters) obj;
if (other.getFilterCriteria() == null ^ this.getFilterCriteria() == null)
return false;
if (other.getFilterCriteria() != null && other.getFilterCriteria().equals(this.getFilterCriteria()) == false)
return false;
if (other.getKinesisStreamParameters() == null ^ this.getKinesisStreamParameters() == null)
return false;
if (other.getKinesisStreamParameters() != null && other.getKinesisStreamParameters().equals(this.getKinesisStreamParameters()) == false)
return false;
if (other.getDynamoDBStreamParameters() == null ^ this.getDynamoDBStreamParameters() == null)
return false;
if (other.getDynamoDBStreamParameters() != null && other.getDynamoDBStreamParameters().equals(this.getDynamoDBStreamParameters()) == false)
return false;
if (other.getSqsQueueParameters() == null ^ this.getSqsQueueParameters() == null)
return false;
if (other.getSqsQueueParameters() != null && other.getSqsQueueParameters().equals(this.getSqsQueueParameters()) == false)
return false;
if (other.getActiveMQBrokerParameters() == null ^ this.getActiveMQBrokerParameters() == null)
return false;
if (other.getActiveMQBrokerParameters() != null && other.getActiveMQBrokerParameters().equals(this.getActiveMQBrokerParameters()) == false)
return false;
if (other.getRabbitMQBrokerParameters() == null ^ this.getRabbitMQBrokerParameters() == null)
return false;
if (other.getRabbitMQBrokerParameters() != null && other.getRabbitMQBrokerParameters().equals(this.getRabbitMQBrokerParameters()) == false)
return false;
if (other.getManagedStreamingKafkaParameters() == null ^ this.getManagedStreamingKafkaParameters() == null)
return false;
if (other.getManagedStreamingKafkaParameters() != null
&& other.getManagedStreamingKafkaParameters().equals(this.getManagedStreamingKafkaParameters()) == false)
return false;
if (other.getSelfManagedKafkaParameters() == null ^ this.getSelfManagedKafkaParameters() == null)
return false;
if (other.getSelfManagedKafkaParameters() != null && other.getSelfManagedKafkaParameters().equals(this.getSelfManagedKafkaParameters()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFilterCriteria() == null) ? 0 : getFilterCriteria().hashCode());
hashCode = prime * hashCode + ((getKinesisStreamParameters() == null) ? 0 : getKinesisStreamParameters().hashCode());
hashCode = prime * hashCode + ((getDynamoDBStreamParameters() == null) ? 0 : getDynamoDBStreamParameters().hashCode());
hashCode = prime * hashCode + ((getSqsQueueParameters() == null) ? 0 : getSqsQueueParameters().hashCode());
hashCode = prime * hashCode + ((getActiveMQBrokerParameters() == null) ? 0 : getActiveMQBrokerParameters().hashCode());
hashCode = prime * hashCode + ((getRabbitMQBrokerParameters() == null) ? 0 : getRabbitMQBrokerParameters().hashCode());
hashCode = prime * hashCode + ((getManagedStreamingKafkaParameters() == null) ? 0 : getManagedStreamingKafkaParameters().hashCode());
hashCode = prime * hashCode + ((getSelfManagedKafkaParameters() == null) ? 0 : getSelfManagedKafkaParameters().hashCode());
return hashCode;
}
@Override
public UpdatePipeSourceParameters clone() {
try {
return (UpdatePipeSourceParameters) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.pipes.model.transform.UpdatePipeSourceParametersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}