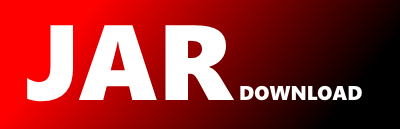
com.amazonaws.services.qapps.AWSQAppsClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-qapps Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.qapps;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.qapps.AWSQAppsClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.qapps.model.*;
import com.amazonaws.services.qapps.model.transform.*;
/**
* Client for accessing QApps. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
*
* The Amazon Q Apps feature capability within Amazon Q Business allows web experience users to create lightweight,
* purpose-built AI apps to fulfill specific tasks from within their web experience. For example, users can create an Q
* Appthat exclusively generates marketing-related content to improve your marketing team's productivity or a Q App for
* marketing content-generation like writing customer emails and creating promotional content using a certain style of
* voice, tone, and branding. For more information, see Amazon Q App in the
* Amazon Q Business User Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSQAppsClient extends AmazonWebServiceClient implements AWSQApps {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSQApps.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "qapps";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ContentTooLargeException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ContentTooLargeExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.UnauthorizedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.qapps.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.qapps.model.AWSQAppsException.class));
public static AWSQAppsClientBuilder builder() {
return AWSQAppsClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on QApps using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSQAppsClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on QApps using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSQAppsClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("data.qapps.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/qapps/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/qapps/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates a rating or review for a library item with the user submitting the request. This increments the rating
* count for the specified library item.
*
*
* @param associateLibraryItemReviewRequest
* @return Result of the AssociateLibraryItemReview operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.AssociateLibraryItemReview
* @see AWS API Documentation
*/
@Override
public AssociateLibraryItemReviewResult associateLibraryItemReview(AssociateLibraryItemReviewRequest request) {
request = beforeClientExecution(request);
return executeAssociateLibraryItemReview(request);
}
@SdkInternalApi
final AssociateLibraryItemReviewResult executeAssociateLibraryItemReview(AssociateLibraryItemReviewRequest associateLibraryItemReviewRequest) {
ExecutionContext executionContext = createExecutionContext(associateLibraryItemReviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateLibraryItemReviewRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateLibraryItemReviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateLibraryItemReview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateLibraryItemReviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This operation creates a link between the user's identity calling the operation and a specific Q App. This is
* useful to mark the Q App as a favorite for the user if the user doesn't own the Amazon Q App so they can
* still run it and see it in their inventory of Q Apps.
*
*
* @param associateQAppWithUserRequest
* @return Result of the AssociateQAppWithUser operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.AssociateQAppWithUser
* @see AWS
* API Documentation
*/
@Override
public AssociateQAppWithUserResult associateQAppWithUser(AssociateQAppWithUserRequest request) {
request = beforeClientExecution(request);
return executeAssociateQAppWithUser(request);
}
@SdkInternalApi
final AssociateQAppWithUserResult executeAssociateQAppWithUser(AssociateQAppWithUserRequest associateQAppWithUserRequest) {
ExecutionContext executionContext = createExecutionContext(associateQAppWithUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateQAppWithUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateQAppWithUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateQAppWithUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateQAppWithUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new library item for an Amazon Q App, allowing it to be discovered and used by other allowed users.
*
*
* @param createLibraryItemRequest
* @return Result of the CreateLibraryItem operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.CreateLibraryItem
* @see AWS API
* Documentation
*/
@Override
public CreateLibraryItemResult createLibraryItem(CreateLibraryItemRequest request) {
request = beforeClientExecution(request);
return executeCreateLibraryItem(request);
}
@SdkInternalApi
final CreateLibraryItemResult executeCreateLibraryItem(CreateLibraryItemRequest createLibraryItemRequest) {
ExecutionContext executionContext = createExecutionContext(createLibraryItemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLibraryItemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createLibraryItemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateLibraryItem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateLibraryItemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Q App based on the provided definition. The Q App definition specifies the cards and flow of
* the Q App. This operation also calculates the dependencies between the cards by inspecting the references in the
* prompts.
*
*
* @param createQAppRequest
* @return Result of the CreateQApp operation returned by the service.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ConflictException
* The requested operation could not be completed due to a conflict with the current state of the resource.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ContentTooLargeException
* The requested operation could not be completed because the content exceeds the maximum allowed size.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.CreateQApp
* @see AWS API
* Documentation
*/
@Override
public CreateQAppResult createQApp(CreateQAppRequest request) {
request = beforeClientExecution(request);
return executeCreateQApp(request);
}
@SdkInternalApi
final CreateQAppResult executeCreateQApp(CreateQAppRequest createQAppRequest) {
ExecutionContext executionContext = createExecutionContext(createQAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateQAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createQAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateQApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateQAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a library item for an Amazon Q App, removing it from the library so it can no longer be discovered or
* used by other users.
*
*
* @param deleteLibraryItemRequest
* @return Result of the DeleteLibraryItem operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.DeleteLibraryItem
* @see AWS API
* Documentation
*/
@Override
public DeleteLibraryItemResult deleteLibraryItem(DeleteLibraryItemRequest request) {
request = beforeClientExecution(request);
return executeDeleteLibraryItem(request);
}
@SdkInternalApi
final DeleteLibraryItemResult executeDeleteLibraryItem(DeleteLibraryItemRequest deleteLibraryItemRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLibraryItemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLibraryItemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteLibraryItemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteLibraryItem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteLibraryItemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Q App owned by the user. If the Q App was previously published to the library, it is also
* removed from the library.
*
*
* @param deleteQAppRequest
* @return Result of the DeleteQApp operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.DeleteQApp
* @see AWS API
* Documentation
*/
@Override
public DeleteQAppResult deleteQApp(DeleteQAppRequest request) {
request = beforeClientExecution(request);
return executeDeleteQApp(request);
}
@SdkInternalApi
final DeleteQAppResult executeDeleteQApp(DeleteQAppRequest deleteQAppRequest) {
ExecutionContext executionContext = createExecutionContext(deleteQAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteQAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteQAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteQApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteQAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a rating or review previously submitted by the user for a library item.
*
*
* @param disassociateLibraryItemReviewRequest
* @return Result of the DisassociateLibraryItemReview operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.DisassociateLibraryItemReview
* @see AWS API Documentation
*/
@Override
public DisassociateLibraryItemReviewResult disassociateLibraryItemReview(DisassociateLibraryItemReviewRequest request) {
request = beforeClientExecution(request);
return executeDisassociateLibraryItemReview(request);
}
@SdkInternalApi
final DisassociateLibraryItemReviewResult executeDisassociateLibraryItemReview(DisassociateLibraryItemReviewRequest disassociateLibraryItemReviewRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateLibraryItemReviewRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateLibraryItemReviewRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateLibraryItemReviewRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateLibraryItemReview");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateLibraryItemReviewResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a Q App from a user removing the user's access to run the Q App.
*
*
* @param disassociateQAppFromUserRequest
* @return Result of the DisassociateQAppFromUser operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.DisassociateQAppFromUser
* @see AWS
* API Documentation
*/
@Override
public DisassociateQAppFromUserResult disassociateQAppFromUser(DisassociateQAppFromUserRequest request) {
request = beforeClientExecution(request);
return executeDisassociateQAppFromUser(request);
}
@SdkInternalApi
final DisassociateQAppFromUserResult executeDisassociateQAppFromUser(DisassociateQAppFromUserRequest disassociateQAppFromUserRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateQAppFromUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateQAppFromUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateQAppFromUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateQAppFromUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateQAppFromUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details about a library item for an Amazon Q App, including its metadata, categories, ratings, and
* usage statistics.
*
*
* @param getLibraryItemRequest
* @return Result of the GetLibraryItem operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.GetLibraryItem
* @see AWS API
* Documentation
*/
@Override
public GetLibraryItemResult getLibraryItem(GetLibraryItemRequest request) {
request = beforeClientExecution(request);
return executeGetLibraryItem(request);
}
@SdkInternalApi
final GetLibraryItemResult executeGetLibraryItem(GetLibraryItemRequest getLibraryItemRequest) {
ExecutionContext executionContext = createExecutionContext(getLibraryItemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetLibraryItemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getLibraryItemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetLibraryItem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetLibraryItemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the full details of an Q App, including its definition specifying the cards and flow.
*
*
* @param getQAppRequest
* @return Result of the GetQApp operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.GetQApp
* @see AWS API
* Documentation
*/
@Override
public GetQAppResult getQApp(GetQAppRequest request) {
request = beforeClientExecution(request);
return executeGetQApp(request);
}
@SdkInternalApi
final GetQAppResult executeGetQApp(GetQAppRequest getQAppRequest) {
ExecutionContext executionContext = createExecutionContext(getQAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetQAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getQAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetQApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetQAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the current state and results for an active session of an Amazon Q App.
*
*
* @param getQAppSessionRequest
* @return Result of the GetQAppSession operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.GetQAppSession
* @see AWS API
* Documentation
*/
@Override
public GetQAppSessionResult getQAppSession(GetQAppSessionRequest request) {
request = beforeClientExecution(request);
return executeGetQAppSession(request);
}
@SdkInternalApi
final GetQAppSessionResult executeGetQAppSession(GetQAppSessionRequest getQAppSessionRequest) {
ExecutionContext executionContext = createExecutionContext(getQAppSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetQAppSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getQAppSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetQAppSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetQAppSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Uploads a file that can then be used either as a default in a FileUploadCard
from Q App definition
* or as a file that is used inside a single Q App run. The purpose of the document is determined by a scope
* parameter that indicates whether it is at the app definition level or at the app session level.
*
*
* @param importDocumentRequest
* @return Result of the ImportDocument operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ContentTooLargeException
* The requested operation could not be completed because the content exceeds the maximum allowed size.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.ImportDocument
* @see AWS API
* Documentation
*/
@Override
public ImportDocumentResult importDocument(ImportDocumentRequest request) {
request = beforeClientExecution(request);
return executeImportDocument(request);
}
@SdkInternalApi
final ImportDocumentResult executeImportDocument(ImportDocumentRequest importDocumentRequest) {
ExecutionContext executionContext = createExecutionContext(importDocumentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ImportDocumentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(importDocumentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ImportDocument");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ImportDocumentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the library items for Amazon Q Apps that are published and available for users in your Amazon Web Services
* account.
*
*
* @param listLibraryItemsRequest
* @return Result of the ListLibraryItems operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.ListLibraryItems
* @see AWS API
* Documentation
*/
@Override
public ListLibraryItemsResult listLibraryItems(ListLibraryItemsRequest request) {
request = beforeClientExecution(request);
return executeListLibraryItems(request);
}
@SdkInternalApi
final ListLibraryItemsResult executeListLibraryItems(ListLibraryItemsRequest listLibraryItemsRequest) {
ExecutionContext executionContext = createExecutionContext(listLibraryItemsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListLibraryItemsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listLibraryItemsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListLibraryItems");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListLibraryItemsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the Amazon Q Apps owned by or associated with the user either because they created it or because they used
* it from the library in the past. The user identity is extracted from the credentials used to invoke this
* operation..
*
*
* @param listQAppsRequest
* @return Result of the ListQApps operation returned by the service.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.ListQApps
* @see AWS API
* Documentation
*/
@Override
public ListQAppsResult listQApps(ListQAppsRequest request) {
request = beforeClientExecution(request);
return executeListQApps(request);
}
@SdkInternalApi
final ListQAppsResult executeListQApps(ListQAppsRequest listQAppsRequest) {
ExecutionContext executionContext = createExecutionContext(listQAppsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListQAppsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listQAppsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListQApps");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListQAppsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags associated with an Amazon Q Apps resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.ListTagsForResource
* @see AWS API
* Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates an Amazon Q App definition based on either a conversation or a problem statement provided as input.The
* resulting app definition can be used to call CreateQApp
. This API doesn't create Amazon Q Apps
* directly.
*
*
* @param predictQAppRequest
* @return Result of the PredictQApp operation returned by the service.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.PredictQApp
* @see AWS API
* Documentation
*/
@Override
public PredictQAppResult predictQApp(PredictQAppRequest request) {
request = beforeClientExecution(request);
return executePredictQApp(request);
}
@SdkInternalApi
final PredictQAppResult executePredictQApp(PredictQAppRequest predictQAppRequest) {
ExecutionContext executionContext = createExecutionContext(predictQAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PredictQAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(predictQAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PredictQApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PredictQAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a new session for an Amazon Q App, allowing inputs to be provided and the app to be run.
*
*
*
* Each Q App session will be condensed into a single conversation in the web experience.
*
*
*
* @param startQAppSessionRequest
* @return Result of the StartQAppSession operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.StartQAppSession
* @see AWS API
* Documentation
*/
@Override
public StartQAppSessionResult startQAppSession(StartQAppSessionRequest request) {
request = beforeClientExecution(request);
return executeStartQAppSession(request);
}
@SdkInternalApi
final StartQAppSessionResult executeStartQAppSession(StartQAppSessionRequest startQAppSessionRequest) {
ExecutionContext executionContext = createExecutionContext(startQAppSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartQAppSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startQAppSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartQAppSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartQAppSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops an active session for an Amazon Q App.This deletes all data related to the session and makes it invalid for
* future uses. The results of the session will be persisted as part of the conversation.
*
*
* @param stopQAppSessionRequest
* @return Result of the StopQAppSession operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.StopQAppSession
* @see AWS API
* Documentation
*/
@Override
public StopQAppSessionResult stopQAppSession(StopQAppSessionRequest request) {
request = beforeClientExecution(request);
return executeStopQAppSession(request);
}
@SdkInternalApi
final StopQAppSessionResult executeStopQAppSession(StopQAppSessionRequest stopQAppSessionRequest) {
ExecutionContext executionContext = createExecutionContext(stopQAppSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopQAppSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopQAppSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopQAppSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StopQAppSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates tags with an Amazon Q Apps resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ConflictException
* The requested operation could not be completed due to a conflict with the current state of the resource.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates tags from an Amazon Q Apps resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the metadata and status of a library item for an Amazon Q App.
*
*
* @param updateLibraryItemRequest
* @return Result of the UpdateLibraryItem operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.UpdateLibraryItem
* @see AWS API
* Documentation
*/
@Override
public UpdateLibraryItemResult updateLibraryItem(UpdateLibraryItemRequest request) {
request = beforeClientExecution(request);
return executeUpdateLibraryItem(request);
}
@SdkInternalApi
final UpdateLibraryItemResult executeUpdateLibraryItem(UpdateLibraryItemRequest updateLibraryItemRequest) {
ExecutionContext executionContext = createExecutionContext(updateLibraryItemRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateLibraryItemRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateLibraryItemRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateLibraryItem");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateLibraryItemResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing Amazon Q App, allowing modifications to its title, description, and definition.
*
*
* @param updateQAppRequest
* @return Result of the UpdateQApp operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ContentTooLargeException
* The requested operation could not be completed because the content exceeds the maximum allowed size.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.UpdateQApp
* @see AWS API
* Documentation
*/
@Override
public UpdateQAppResult updateQApp(UpdateQAppRequest request) {
request = beforeClientExecution(request);
return executeUpdateQApp(request);
}
@SdkInternalApi
final UpdateQAppResult executeUpdateQApp(UpdateQAppRequest updateQAppRequest) {
ExecutionContext executionContext = createExecutionContext(updateQAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateQAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateQAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateQApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateQAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the session for a given Q App sessionId
. This is only valid when at least one card of the
* session is in the WAITING
state. Data for each WAITING
card can be provided as input.
* If inputs are not provided, the call will be accepted but session will not move forward. Inputs for cards that
* are not in the WAITING
status will be ignored.
*
*
* @param updateQAppSessionRequest
* @return Result of the UpdateQAppSession operation returned by the service.
* @throws ResourceNotFoundException
* The requested resource could not be found.
* @throws AccessDeniedException
* The client is not authorized to perform the requested operation.
* @throws ValidationException
* The input failed to satisfy the constraints specified by the service.
* @throws InternalServerException
* An internal service error occurred while processing the request.
* @throws UnauthorizedException
* The client is not authenticated or authorized to perform the requested operation.
* @throws ServiceQuotaExceededException
* The requested operation could not be completed because it would exceed the service's quota or limit.
* @throws ThrottlingException
* The requested operation could not be completed because too many requests were sent at once. Wait a bit
* and try again later.
* @sample AWSQApps.UpdateQAppSession
* @see AWS API
* Documentation
*/
@Override
public UpdateQAppSessionResult updateQAppSession(UpdateQAppSessionRequest request) {
request = beforeClientExecution(request);
return executeUpdateQAppSession(request);
}
@SdkInternalApi
final UpdateQAppSessionResult executeUpdateQAppSession(UpdateQAppSessionRequest updateQAppSessionRequest) {
ExecutionContext executionContext = createExecutionContext(updateQAppSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateQAppSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateQAppSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QApps");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateQAppSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateQAppSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}