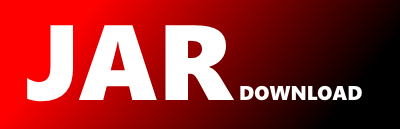
com.amazonaws.services.qapps.AWSQAppsAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.qapps;
import javax.annotation.Generated;
import com.amazonaws.services.qapps.model.*;
/**
* Interface for accessing QApps asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.qapps.AbstractAWSQAppsAsync} instead.
*
*
*
* The Amazon Q Apps feature capability within Amazon Q Business allows web experience users to create lightweight,
* purpose-built AI apps to fulfill specific tasks from within their web experience. For example, users can create an Q
* Appthat exclusively generates marketing-related content to improve your marketing team's productivity or a Q App for
* marketing content-generation like writing customer emails and creating promotional content using a certain style of
* voice, tone, and branding. For more information, see Amazon Q App in the
* Amazon Q Business User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSQAppsAsync extends AWSQApps {
/**
*
* Associates a rating or review for a library item with the user submitting the request. This increments the rating
* count for the specified library item.
*
*
* @param associateLibraryItemReviewRequest
* @return A Java Future containing the result of the AssociateLibraryItemReview operation returned by the service.
* @sample AWSQAppsAsync.AssociateLibraryItemReview
* @see AWS API Documentation
*/
java.util.concurrent.Future associateLibraryItemReviewAsync(
AssociateLibraryItemReviewRequest associateLibraryItemReviewRequest);
/**
*
* Associates a rating or review for a library item with the user submitting the request. This increments the rating
* count for the specified library item.
*
*
* @param associateLibraryItemReviewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateLibraryItemReview operation returned by the service.
* @sample AWSQAppsAsyncHandler.AssociateLibraryItemReview
* @see AWS API Documentation
*/
java.util.concurrent.Future associateLibraryItemReviewAsync(
AssociateLibraryItemReviewRequest associateLibraryItemReviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This operation creates a link between the user's identity calling the operation and a specific Q App. This is
* useful to mark the Q App as a favorite for the user if the user doesn't own the Amazon Q App so they can
* still run it and see it in their inventory of Q Apps.
*
*
* @param associateQAppWithUserRequest
* @return A Java Future containing the result of the AssociateQAppWithUser operation returned by the service.
* @sample AWSQAppsAsync.AssociateQAppWithUser
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateQAppWithUserAsync(AssociateQAppWithUserRequest associateQAppWithUserRequest);
/**
*
* This operation creates a link between the user's identity calling the operation and a specific Q App. This is
* useful to mark the Q App as a favorite for the user if the user doesn't own the Amazon Q App so they can
* still run it and see it in their inventory of Q Apps.
*
*
* @param associateQAppWithUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateQAppWithUser operation returned by the service.
* @sample AWSQAppsAsyncHandler.AssociateQAppWithUser
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateQAppWithUserAsync(AssociateQAppWithUserRequest associateQAppWithUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new library item for an Amazon Q App, allowing it to be discovered and used by other allowed users.
*
*
* @param createLibraryItemRequest
* @return A Java Future containing the result of the CreateLibraryItem operation returned by the service.
* @sample AWSQAppsAsync.CreateLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLibraryItemAsync(CreateLibraryItemRequest createLibraryItemRequest);
/**
*
* Creates a new library item for an Amazon Q App, allowing it to be discovered and used by other allowed users.
*
*
* @param createLibraryItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLibraryItem operation returned by the service.
* @sample AWSQAppsAsyncHandler.CreateLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLibraryItemAsync(CreateLibraryItemRequest createLibraryItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Q App based on the provided definition. The Q App definition specifies the cards and flow of
* the Q App. This operation also calculates the dependencies between the cards by inspecting the references in the
* prompts.
*
*
* @param createQAppRequest
* @return A Java Future containing the result of the CreateQApp operation returned by the service.
* @sample AWSQAppsAsync.CreateQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQAppAsync(CreateQAppRequest createQAppRequest);
/**
*
* Creates a new Amazon Q App based on the provided definition. The Q App definition specifies the cards and flow of
* the Q App. This operation also calculates the dependencies between the cards by inspecting the references in the
* prompts.
*
*
* @param createQAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQApp operation returned by the service.
* @sample AWSQAppsAsyncHandler.CreateQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQAppAsync(CreateQAppRequest createQAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a library item for an Amazon Q App, removing it from the library so it can no longer be discovered or
* used by other users.
*
*
* @param deleteLibraryItemRequest
* @return A Java Future containing the result of the DeleteLibraryItem operation returned by the service.
* @sample AWSQAppsAsync.DeleteLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLibraryItemAsync(DeleteLibraryItemRequest deleteLibraryItemRequest);
/**
*
* Deletes a library item for an Amazon Q App, removing it from the library so it can no longer be discovered or
* used by other users.
*
*
* @param deleteLibraryItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLibraryItem operation returned by the service.
* @sample AWSQAppsAsyncHandler.DeleteLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteLibraryItemAsync(DeleteLibraryItemRequest deleteLibraryItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Q App owned by the user. If the Q App was previously published to the library, it is also
* removed from the library.
*
*
* @param deleteQAppRequest
* @return A Java Future containing the result of the DeleteQApp operation returned by the service.
* @sample AWSQAppsAsync.DeleteQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteQAppAsync(DeleteQAppRequest deleteQAppRequest);
/**
*
* Deletes an Amazon Q App owned by the user. If the Q App was previously published to the library, it is also
* removed from the library.
*
*
* @param deleteQAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQApp operation returned by the service.
* @sample AWSQAppsAsyncHandler.DeleteQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteQAppAsync(DeleteQAppRequest deleteQAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a rating or review previously submitted by the user for a library item.
*
*
* @param disassociateLibraryItemReviewRequest
* @return A Java Future containing the result of the DisassociateLibraryItemReview operation returned by the
* service.
* @sample AWSQAppsAsync.DisassociateLibraryItemReview
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateLibraryItemReviewAsync(
DisassociateLibraryItemReviewRequest disassociateLibraryItemReviewRequest);
/**
*
* Removes a rating or review previously submitted by the user for a library item.
*
*
* @param disassociateLibraryItemReviewRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateLibraryItemReview operation returned by the
* service.
* @sample AWSQAppsAsyncHandler.DisassociateLibraryItemReview
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateLibraryItemReviewAsync(
DisassociateLibraryItemReviewRequest disassociateLibraryItemReviewRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a Q App from a user removing the user's access to run the Q App.
*
*
* @param disassociateQAppFromUserRequest
* @return A Java Future containing the result of the DisassociateQAppFromUser operation returned by the service.
* @sample AWSQAppsAsync.DisassociateQAppFromUser
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateQAppFromUserAsync(DisassociateQAppFromUserRequest disassociateQAppFromUserRequest);
/**
*
* Disassociates a Q App from a user removing the user's access to run the Q App.
*
*
* @param disassociateQAppFromUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateQAppFromUser operation returned by the service.
* @sample AWSQAppsAsyncHandler.DisassociateQAppFromUser
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disassociateQAppFromUserAsync(DisassociateQAppFromUserRequest disassociateQAppFromUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details about a library item for an Amazon Q App, including its metadata, categories, ratings, and
* usage statistics.
*
*
* @param getLibraryItemRequest
* @return A Java Future containing the result of the GetLibraryItem operation returned by the service.
* @sample AWSQAppsAsync.GetLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLibraryItemAsync(GetLibraryItemRequest getLibraryItemRequest);
/**
*
* Retrieves details about a library item for an Amazon Q App, including its metadata, categories, ratings, and
* usage statistics.
*
*
* @param getLibraryItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetLibraryItem operation returned by the service.
* @sample AWSQAppsAsyncHandler.GetLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getLibraryItemAsync(GetLibraryItemRequest getLibraryItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the full details of an Q App, including its definition specifying the cards and flow.
*
*
* @param getQAppRequest
* @return A Java Future containing the result of the GetQApp operation returned by the service.
* @sample AWSQAppsAsync.GetQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQAppAsync(GetQAppRequest getQAppRequest);
/**
*
* Retrieves the full details of an Q App, including its definition specifying the cards and flow.
*
*
* @param getQAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQApp operation returned by the service.
* @sample AWSQAppsAsyncHandler.GetQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQAppAsync(GetQAppRequest getQAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current state and results for an active session of an Amazon Q App.
*
*
* @param getQAppSessionRequest
* @return A Java Future containing the result of the GetQAppSession operation returned by the service.
* @sample AWSQAppsAsync.GetQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQAppSessionAsync(GetQAppSessionRequest getQAppSessionRequest);
/**
*
* Retrieves the current state and results for an active session of an Amazon Q App.
*
*
* @param getQAppSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQAppSession operation returned by the service.
* @sample AWSQAppsAsyncHandler.GetQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQAppSessionAsync(GetQAppSessionRequest getQAppSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads a file that can then be used either as a default in a FileUploadCard
from Q App definition
* or as a file that is used inside a single Q App run. The purpose of the document is determined by a scope
* parameter that indicates whether it is at the app definition level or at the app session level.
*
*
* @param importDocumentRequest
* @return A Java Future containing the result of the ImportDocument operation returned by the service.
* @sample AWSQAppsAsync.ImportDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importDocumentAsync(ImportDocumentRequest importDocumentRequest);
/**
*
* Uploads a file that can then be used either as a default in a FileUploadCard
from Q App definition
* or as a file that is used inside a single Q App run. The purpose of the document is determined by a scope
* parameter that indicates whether it is at the app definition level or at the app session level.
*
*
* @param importDocumentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportDocument operation returned by the service.
* @sample AWSQAppsAsyncHandler.ImportDocument
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importDocumentAsync(ImportDocumentRequest importDocumentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the library items for Amazon Q Apps that are published and available for users in your Amazon Web Services
* account.
*
*
* @param listLibraryItemsRequest
* @return A Java Future containing the result of the ListLibraryItems operation returned by the service.
* @sample AWSQAppsAsync.ListLibraryItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLibraryItemsAsync(ListLibraryItemsRequest listLibraryItemsRequest);
/**
*
* Lists the library items for Amazon Q Apps that are published and available for users in your Amazon Web Services
* account.
*
*
* @param listLibraryItemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLibraryItems operation returned by the service.
* @sample AWSQAppsAsyncHandler.ListLibraryItems
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listLibraryItemsAsync(ListLibraryItemsRequest listLibraryItemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon Q Apps owned by or associated with the user either because they created it or because they used
* it from the library in the past. The user identity is extracted from the credentials used to invoke this
* operation..
*
*
* @param listQAppsRequest
* @return A Java Future containing the result of the ListQApps operation returned by the service.
* @sample AWSQAppsAsync.ListQApps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQAppsAsync(ListQAppsRequest listQAppsRequest);
/**
*
* Lists the Amazon Q Apps owned by or associated with the user either because they created it or because they used
* it from the library in the past. The user identity is extracted from the credentials used to invoke this
* operation..
*
*
* @param listQAppsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQApps operation returned by the service.
* @sample AWSQAppsAsyncHandler.ListQApps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listQAppsAsync(ListQAppsRequest listQAppsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags associated with an Amazon Q Apps resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSQAppsAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags associated with an Amazon Q Apps resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSQAppsAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates an Amazon Q App definition based on either a conversation or a problem statement provided as input.The
* resulting app definition can be used to call CreateQApp
. This API doesn't create Amazon Q Apps
* directly.
*
*
* @param predictQAppRequest
* @return A Java Future containing the result of the PredictQApp operation returned by the service.
* @sample AWSQAppsAsync.PredictQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future predictQAppAsync(PredictQAppRequest predictQAppRequest);
/**
*
* Generates an Amazon Q App definition based on either a conversation or a problem statement provided as input.The
* resulting app definition can be used to call CreateQApp
. This API doesn't create Amazon Q Apps
* directly.
*
*
* @param predictQAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PredictQApp operation returned by the service.
* @sample AWSQAppsAsyncHandler.PredictQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future predictQAppAsync(PredictQAppRequest predictQAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a new session for an Amazon Q App, allowing inputs to be provided and the app to be run.
*
*
*
* Each Q App session will be condensed into a single conversation in the web experience.
*
*
*
* @param startQAppSessionRequest
* @return A Java Future containing the result of the StartQAppSession operation returned by the service.
* @sample AWSQAppsAsync.StartQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startQAppSessionAsync(StartQAppSessionRequest startQAppSessionRequest);
/**
*
* Starts a new session for an Amazon Q App, allowing inputs to be provided and the app to be run.
*
*
*
* Each Q App session will be condensed into a single conversation in the web experience.
*
*
*
* @param startQAppSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartQAppSession operation returned by the service.
* @sample AWSQAppsAsyncHandler.StartQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startQAppSessionAsync(StartQAppSessionRequest startQAppSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an active session for an Amazon Q App.This deletes all data related to the session and makes it invalid for
* future uses. The results of the session will be persisted as part of the conversation.
*
*
* @param stopQAppSessionRequest
* @return A Java Future containing the result of the StopQAppSession operation returned by the service.
* @sample AWSQAppsAsync.StopQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopQAppSessionAsync(StopQAppSessionRequest stopQAppSessionRequest);
/**
*
* Stops an active session for an Amazon Q App.This deletes all data related to the session and makes it invalid for
* future uses. The results of the session will be persisted as part of the conversation.
*
*
* @param stopQAppSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopQAppSession operation returned by the service.
* @sample AWSQAppsAsyncHandler.StopQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopQAppSessionAsync(StopQAppSessionRequest stopQAppSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates tags with an Amazon Q Apps resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSQAppsAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associates tags with an Amazon Q Apps resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSQAppsAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates tags from an Amazon Q Apps resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSQAppsAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Disassociates tags from an Amazon Q Apps resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSQAppsAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the metadata and status of a library item for an Amazon Q App.
*
*
* @param updateLibraryItemRequest
* @return A Java Future containing the result of the UpdateLibraryItem operation returned by the service.
* @sample AWSQAppsAsync.UpdateLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLibraryItemAsync(UpdateLibraryItemRequest updateLibraryItemRequest);
/**
*
* Updates the metadata and status of a library item for an Amazon Q App.
*
*
* @param updateLibraryItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateLibraryItem operation returned by the service.
* @sample AWSQAppsAsyncHandler.UpdateLibraryItem
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateLibraryItemAsync(UpdateLibraryItemRequest updateLibraryItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing Amazon Q App, allowing modifications to its title, description, and definition.
*
*
* @param updateQAppRequest
* @return A Java Future containing the result of the UpdateQApp operation returned by the service.
* @sample AWSQAppsAsync.UpdateQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateQAppAsync(UpdateQAppRequest updateQAppRequest);
/**
*
* Updates an existing Amazon Q App, allowing modifications to its title, description, and definition.
*
*
* @param updateQAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateQApp operation returned by the service.
* @sample AWSQAppsAsyncHandler.UpdateQApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateQAppAsync(UpdateQAppRequest updateQAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the session for a given Q App sessionId
. This is only valid when at least one card of the
* session is in the WAITING
state. Data for each WAITING
card can be provided as input.
* If inputs are not provided, the call will be accepted but session will not move forward. Inputs for cards that
* are not in the WAITING
status will be ignored.
*
*
* @param updateQAppSessionRequest
* @return A Java Future containing the result of the UpdateQAppSession operation returned by the service.
* @sample AWSQAppsAsync.UpdateQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateQAppSessionAsync(UpdateQAppSessionRequest updateQAppSessionRequest);
/**
*
* Updates the session for a given Q App sessionId
. This is only valid when at least one card of the
* session is in the WAITING
state. Data for each WAITING
card can be provided as input.
* If inputs are not provided, the call will be accepted but session will not move forward. Inputs for cards that
* are not in the WAITING
status will be ignored.
*
*
* @param updateQAppSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateQAppSession operation returned by the service.
* @sample AWSQAppsAsyncHandler.UpdateQAppSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateQAppSessionAsync(UpdateQAppSessionRequest updateQAppSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}