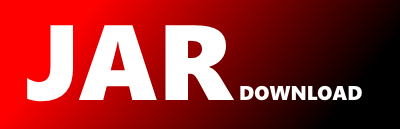
com.amazonaws.services.qconnect.model.CreateQuickResponseRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-qconnect Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.qconnect.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateQuickResponseRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Connect channels this quick response applies to.
*
*/
private java.util.List channels;
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided,
* the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries safe with
* idempotent APIs.
*
*/
private String clientToken;
/**
*
* The content of the quick response.
*
*/
private QuickResponseDataProvider content;
/**
*
* The media type of the quick response content.
*
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*
*/
private String contentType;
/**
*
* The description of the quick response.
*
*/
private String description;
/**
*
* The configuration information of the user groups that the quick response is accessible to.
*
*/
private GroupingConfiguration groupingConfiguration;
/**
*
* Whether the quick response is active.
*
*/
private Boolean isActive;
/**
*
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're storing
* Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*
*/
private String knowledgeBaseId;
/**
*
* The language code value for the language in which the quick response is written. The supported language codes
* include de_DE
, en_US
, es_ES
, fr_FR
, id_ID
,
* it_IT
, ja_JP
, ko_KR
, pt_BR
, zh_CN
,
* zh_TW
*
*/
private String language;
/**
*
* The name of the quick response.
*
*/
private String name;
/**
*
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*
*/
private String shortcutKey;
/**
*
* The tags used to organize, track, or control access for this resource.
*
*/
private java.util.Map tags;
/**
*
* The Amazon Connect channels this quick response applies to.
*
*
* @return The Amazon Connect channels this quick response applies to.
*/
public java.util.List getChannels() {
return channels;
}
/**
*
* The Amazon Connect channels this quick response applies to.
*
*
* @param channels
* The Amazon Connect channels this quick response applies to.
*/
public void setChannels(java.util.Collection channels) {
if (channels == null) {
this.channels = null;
return;
}
this.channels = new java.util.ArrayList(channels);
}
/**
*
* The Amazon Connect channels this quick response applies to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChannels(java.util.Collection)} or {@link #withChannels(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param channels
* The Amazon Connect channels this quick response applies to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withChannels(String... channels) {
if (this.channels == null) {
setChannels(new java.util.ArrayList(channels.length));
}
for (String ele : channels) {
this.channels.add(ele);
}
return this;
}
/**
*
* The Amazon Connect channels this quick response applies to.
*
*
* @param channels
* The Amazon Connect channels this quick response applies to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withChannels(java.util.Collection channels) {
setChannels(channels);
return this;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided,
* the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries safe with
* idempotent APIs.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not
* provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries
* safe with idempotent APIs.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided,
* the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries safe with
* idempotent APIs.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not
* provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see
* Making
* retries safe with idempotent APIs.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided,
* the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries safe with
* idempotent APIs.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not
* provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see Making retries
* safe with idempotent APIs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The content of the quick response.
*
*
* @param content
* The content of the quick response.
*/
public void setContent(QuickResponseDataProvider content) {
this.content = content;
}
/**
*
* The content of the quick response.
*
*
* @return The content of the quick response.
*/
public QuickResponseDataProvider getContent() {
return this.content;
}
/**
*
* The content of the quick response.
*
*
* @param content
* The content of the quick response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withContent(QuickResponseDataProvider content) {
setContent(content);
return this;
}
/**
*
* The media type of the quick response content.
*
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*
*
* @param contentType
* The media type of the quick response content.
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*/
public void setContentType(String contentType) {
this.contentType = contentType;
}
/**
*
* The media type of the quick response content.
*
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*
*
* @return The media type of the quick response content.
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*/
public String getContentType() {
return this.contentType;
}
/**
*
* The media type of the quick response content.
*
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
*
*
* @param contentType
* The media type of the quick response content.
*
* -
*
* Use application/x.quickresponse;format=plain
for a quick response written in plain text.
*
*
* -
*
* Use application/x.quickresponse;format=markdown
for a quick response written in richtext.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withContentType(String contentType) {
setContentType(contentType);
return this;
}
/**
*
* The description of the quick response.
*
*
* @param description
* The description of the quick response.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the quick response.
*
*
* @return The description of the quick response.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the quick response.
*
*
* @param description
* The description of the quick response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The configuration information of the user groups that the quick response is accessible to.
*
*
* @param groupingConfiguration
* The configuration information of the user groups that the quick response is accessible to.
*/
public void setGroupingConfiguration(GroupingConfiguration groupingConfiguration) {
this.groupingConfiguration = groupingConfiguration;
}
/**
*
* The configuration information of the user groups that the quick response is accessible to.
*
*
* @return The configuration information of the user groups that the quick response is accessible to.
*/
public GroupingConfiguration getGroupingConfiguration() {
return this.groupingConfiguration;
}
/**
*
* The configuration information of the user groups that the quick response is accessible to.
*
*
* @param groupingConfiguration
* The configuration information of the user groups that the quick response is accessible to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withGroupingConfiguration(GroupingConfiguration groupingConfiguration) {
setGroupingConfiguration(groupingConfiguration);
return this;
}
/**
*
* Whether the quick response is active.
*
*
* @param isActive
* Whether the quick response is active.
*/
public void setIsActive(Boolean isActive) {
this.isActive = isActive;
}
/**
*
* Whether the quick response is active.
*
*
* @return Whether the quick response is active.
*/
public Boolean getIsActive() {
return this.isActive;
}
/**
*
* Whether the quick response is active.
*
*
* @param isActive
* Whether the quick response is active.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withIsActive(Boolean isActive) {
setIsActive(isActive);
return this;
}
/**
*
* Whether the quick response is active.
*
*
* @return Whether the quick response is active.
*/
public Boolean isActive() {
return this.isActive;
}
/**
*
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're storing
* Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*
*
* @param knowledgeBaseId
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're
* storing Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*/
public void setKnowledgeBaseId(String knowledgeBaseId) {
this.knowledgeBaseId = knowledgeBaseId;
}
/**
*
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're storing
* Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*
*
* @return The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're
* storing Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*/
public String getKnowledgeBaseId() {
return this.knowledgeBaseId;
}
/**
*
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're storing
* Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
*
*
* @param knowledgeBaseId
* The identifier of the knowledge base. This should not be a QUICK_RESPONSES type knowledge base if you're
* storing Amazon Q Content resource to it. Can be either the ID or the ARN. URLs cannot contain the ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withKnowledgeBaseId(String knowledgeBaseId) {
setKnowledgeBaseId(knowledgeBaseId);
return this;
}
/**
*
* The language code value for the language in which the quick response is written. The supported language codes
* include de_DE
, en_US
, es_ES
, fr_FR
, id_ID
,
* it_IT
, ja_JP
, ko_KR
, pt_BR
, zh_CN
,
* zh_TW
*
*
* @param language
* The language code value for the language in which the quick response is written. The supported language
* codes include de_DE
, en_US
, es_ES
, fr_FR
,
* id_ID
, it_IT
, ja_JP
, ko_KR
, pt_BR
,
* zh_CN
, zh_TW
*/
public void setLanguage(String language) {
this.language = language;
}
/**
*
* The language code value for the language in which the quick response is written. The supported language codes
* include de_DE
, en_US
, es_ES
, fr_FR
, id_ID
,
* it_IT
, ja_JP
, ko_KR
, pt_BR
, zh_CN
,
* zh_TW
*
*
* @return The language code value for the language in which the quick response is written. The supported language
* codes include de_DE
, en_US
, es_ES
, fr_FR
,
* id_ID
, it_IT
, ja_JP
, ko_KR
, pt_BR
,
* zh_CN
, zh_TW
*/
public String getLanguage() {
return this.language;
}
/**
*
* The language code value for the language in which the quick response is written. The supported language codes
* include de_DE
, en_US
, es_ES
, fr_FR
, id_ID
,
* it_IT
, ja_JP
, ko_KR
, pt_BR
, zh_CN
,
* zh_TW
*
*
* @param language
* The language code value for the language in which the quick response is written. The supported language
* codes include de_DE
, en_US
, es_ES
, fr_FR
,
* id_ID
, it_IT
, ja_JP
, ko_KR
, pt_BR
,
* zh_CN
, zh_TW
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withLanguage(String language) {
setLanguage(language);
return this;
}
/**
*
* The name of the quick response.
*
*
* @param name
* The name of the quick response.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the quick response.
*
*
* @return The name of the quick response.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the quick response.
*
*
* @param name
* The name of the quick response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*
*
* @param shortcutKey
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*/
public void setShortcutKey(String shortcutKey) {
this.shortcutKey = shortcutKey;
}
/**
*
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*
*
* @return The shortcut key of the quick response. The value should be unique across the knowledge base.
*/
public String getShortcutKey() {
return this.shortcutKey;
}
/**
*
* The shortcut key of the quick response. The value should be unique across the knowledge base.
*
*
* @param shortcutKey
* The shortcut key of the quick response. The value should be unique across the knowledge base.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withShortcutKey(String shortcutKey) {
setShortcutKey(shortcutKey);
return this;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @return The tags used to organize, track, or control access for this resource.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @param tags
* The tags used to organize, track, or control access for this resource.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags used to organize, track, or control access for this resource.
*
*
* @param tags
* The tags used to organize, track, or control access for this resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateQuickResponseRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateQuickResponseRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChannels() != null)
sb.append("Channels: ").append("***Sensitive Data Redacted***").append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getContent() != null)
sb.append("Content: ").append(getContent()).append(",");
if (getContentType() != null)
sb.append("ContentType: ").append(getContentType()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getGroupingConfiguration() != null)
sb.append("GroupingConfiguration: ").append(getGroupingConfiguration()).append(",");
if (getIsActive() != null)
sb.append("IsActive: ").append(getIsActive()).append(",");
if (getKnowledgeBaseId() != null)
sb.append("KnowledgeBaseId: ").append(getKnowledgeBaseId()).append(",");
if (getLanguage() != null)
sb.append("Language: ").append(getLanguage()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getShortcutKey() != null)
sb.append("ShortcutKey: ").append(getShortcutKey()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateQuickResponseRequest == false)
return false;
CreateQuickResponseRequest other = (CreateQuickResponseRequest) obj;
if (other.getChannels() == null ^ this.getChannels() == null)
return false;
if (other.getChannels() != null && other.getChannels().equals(this.getChannels()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getContent() == null ^ this.getContent() == null)
return false;
if (other.getContent() != null && other.getContent().equals(this.getContent()) == false)
return false;
if (other.getContentType() == null ^ this.getContentType() == null)
return false;
if (other.getContentType() != null && other.getContentType().equals(this.getContentType()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getGroupingConfiguration() == null ^ this.getGroupingConfiguration() == null)
return false;
if (other.getGroupingConfiguration() != null && other.getGroupingConfiguration().equals(this.getGroupingConfiguration()) == false)
return false;
if (other.getIsActive() == null ^ this.getIsActive() == null)
return false;
if (other.getIsActive() != null && other.getIsActive().equals(this.getIsActive()) == false)
return false;
if (other.getKnowledgeBaseId() == null ^ this.getKnowledgeBaseId() == null)
return false;
if (other.getKnowledgeBaseId() != null && other.getKnowledgeBaseId().equals(this.getKnowledgeBaseId()) == false)
return false;
if (other.getLanguage() == null ^ this.getLanguage() == null)
return false;
if (other.getLanguage() != null && other.getLanguage().equals(this.getLanguage()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getShortcutKey() == null ^ this.getShortcutKey() == null)
return false;
if (other.getShortcutKey() != null && other.getShortcutKey().equals(this.getShortcutKey()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChannels() == null) ? 0 : getChannels().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getContent() == null) ? 0 : getContent().hashCode());
hashCode = prime * hashCode + ((getContentType() == null) ? 0 : getContentType().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getGroupingConfiguration() == null) ? 0 : getGroupingConfiguration().hashCode());
hashCode = prime * hashCode + ((getIsActive() == null) ? 0 : getIsActive().hashCode());
hashCode = prime * hashCode + ((getKnowledgeBaseId() == null) ? 0 : getKnowledgeBaseId().hashCode());
hashCode = prime * hashCode + ((getLanguage() == null) ? 0 : getLanguage().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getShortcutKey() == null) ? 0 : getShortcutKey().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateQuickResponseRequest clone() {
return (CreateQuickResponseRequest) super.clone();
}
}