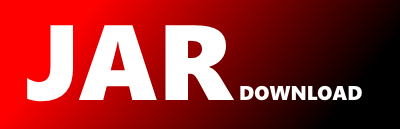
com.amazonaws.services.qconnect.AmazonQConnectAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-qconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.qconnect;
import javax.annotation.Generated;
import com.amazonaws.services.qconnect.model.*;
/**
* Interface for accessing Amazon Q Connect asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.qconnect.AbstractAmazonQConnectAsync} instead.
*
*
*
*
* Powered by Amazon Bedrock: Amazon Web Services implements automated abuse detection.
* Because Amazon Q in Connect is built on Amazon Bedrock, users can take full advantage of the controls implemented in
* Amazon Bedrock to enforce safety, security, and the responsible use of artificial intelligence (AI).
*
*
*
* Amazon Q in Connect is a generative AI customer service assistant. It is an LLM-enhanced evolution of Amazon Connect
* Wisdom that delivers real-time recommendations to help contact center agents resolve customer issues quickly and
* accurately.
*
*
* Amazon Q in Connect automatically detects customer intent during calls and chats using conversational analytics and
* natural language understanding (NLU). It then provides agents with immediate, real-time generative responses and
* suggested actions, and links to relevant documents and articles. Agents can also query Amazon Q in Connect directly
* using natural language or keywords to answer customer requests.
*
*
* Use the Amazon Q in Connect APIs to create an assistant and a knowledge base, for example, or manage content by
* uploading custom files.
*
*
* For more information, see Use
* Amazon Q in Connect for generative AI powered agent assistance in real-time in the Amazon Connect
* Administrator Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonQConnectAsync extends AmazonQConnect {
/**
*
* Creates an Amazon Q in Connect assistant.
*
*
* @param createAssistantRequest
* @return A Java Future containing the result of the CreateAssistant operation returned by the service.
* @sample AmazonQConnectAsync.CreateAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssistantAsync(CreateAssistantRequest createAssistantRequest);
/**
*
* Creates an Amazon Q in Connect assistant.
*
*
* @param createAssistantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssistant operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAssistantAsync(CreateAssistantRequest createAssistantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an association between an Amazon Q in Connect assistant and another resource. Currently, the only
* supported association is with a knowledge base. An assistant can have only a single association.
*
*
* @param createAssistantAssociationRequest
* @return A Java Future containing the result of the CreateAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsync.CreateAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssistantAssociationAsync(
CreateAssistantAssociationRequest createAssistantAssociationRequest);
/**
*
* Creates an association between an Amazon Q in Connect assistant and another resource. Currently, the only
* supported association is with a knowledge base. An assistant can have only a single association.
*
*
* @param createAssistantAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createAssistantAssociationAsync(
CreateAssistantAssociationRequest createAssistantAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates Amazon Q in Connect content. Before to calling this API, use StartContentUpload to upload an asset.
*
*
* @param createContentRequest
* @return A Java Future containing the result of the CreateContent operation returned by the service.
* @sample AmazonQConnectAsync.CreateContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContentAsync(CreateContentRequest createContentRequest);
/**
*
* Creates Amazon Q in Connect content. Before to calling this API, use StartContentUpload to upload an asset.
*
*
* @param createContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContent operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createContentAsync(CreateContentRequest createContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an association between a content resource in a knowledge base and step-by-step
* guides. Step-by-step guides offer instructions to agents for resolving common customer issues. You create a
* content association to integrate Amazon Q in Connect and step-by-step guides.
*
*
* After you integrate Amazon Q and step-by-step guides, when Amazon Q provides a recommendation to an agent based
* on the intent that it's detected, it also provides them with the option to start the step-by-step guide that you
* have associated with the content.
*
*
* Note the following limitations:
*
*
* -
*
* You can create only one content association for each content resource in a knowledge base.
*
*
* -
*
* You can associate a step-by-step guide with multiple content resources.
*
*
*
*
* For more information, see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param createContentAssociationRequest
* @return A Java Future containing the result of the CreateContentAssociation operation returned by the service.
* @sample AmazonQConnectAsync.CreateContentAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createContentAssociationAsync(CreateContentAssociationRequest createContentAssociationRequest);
/**
*
* Creates an association between a content resource in a knowledge base and step-by-step
* guides. Step-by-step guides offer instructions to agents for resolving common customer issues. You create a
* content association to integrate Amazon Q in Connect and step-by-step guides.
*
*
* After you integrate Amazon Q and step-by-step guides, when Amazon Q provides a recommendation to an agent based
* on the intent that it's detected, it also provides them with the option to start the step-by-step guide that you
* have associated with the content.
*
*
* Note the following limitations:
*
*
* -
*
* You can create only one content association for each content resource in a knowledge base.
*
*
* -
*
* You can associate a step-by-step guide with multiple content resources.
*
*
*
*
* For more information, see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param createContentAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateContentAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateContentAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createContentAssociationAsync(CreateContentAssociationRequest createContentAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a knowledge base.
*
*
*
* When using this API, you cannot reuse Amazon AppIntegrations
* DataIntegrations with external knowledge bases such as Salesforce and ServiceNow. If you do, you'll get an
* InvalidRequestException
error.
*
*
* For example, you're programmatically managing your external knowledge base, and you want to add or remove one of
* the fields that is being ingested from Salesforce. Do the following:
*
*
* -
*
* Call
* DeleteKnowledgeBase.
*
*
* -
*
* Call
* DeleteDataIntegration.
*
*
* -
*
* Call
* CreateDataIntegration to recreate the DataIntegration or a create different one.
*
*
* -
*
* Call CreateKnowledgeBase.
*
*
*
*
*
* @param createKnowledgeBaseRequest
* @return A Java Future containing the result of the CreateKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsync.CreateKnowledgeBase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createKnowledgeBaseAsync(CreateKnowledgeBaseRequest createKnowledgeBaseRequest);
/**
*
* Creates a knowledge base.
*
*
*
* When using this API, you cannot reuse Amazon AppIntegrations
* DataIntegrations with external knowledge bases such as Salesforce and ServiceNow. If you do, you'll get an
* InvalidRequestException
error.
*
*
* For example, you're programmatically managing your external knowledge base, and you want to add or remove one of
* the fields that is being ingested from Salesforce. Do the following:
*
*
* -
*
* Call
* DeleteKnowledgeBase.
*
*
* -
*
* Call
* DeleteDataIntegration.
*
*
* -
*
* Call
* CreateDataIntegration to recreate the DataIntegration or a create different one.
*
*
* -
*
* Call CreateKnowledgeBase.
*
*
*
*
*
* @param createKnowledgeBaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateKnowledgeBase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createKnowledgeBaseAsync(CreateKnowledgeBaseRequest createKnowledgeBaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Q in Connect quick response.
*
*
* @param createQuickResponseRequest
* @return A Java Future containing the result of the CreateQuickResponse operation returned by the service.
* @sample AmazonQConnectAsync.CreateQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createQuickResponseAsync(CreateQuickResponseRequest createQuickResponseRequest);
/**
*
* Creates an Amazon Q in Connect quick response.
*
*
* @param createQuickResponseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQuickResponse operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createQuickResponseAsync(CreateQuickResponseRequest createQuickResponseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* creates a new Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param createSessionRequest
* @return A Java Future containing the result of the CreateSession operation returned by the service.
* @sample AmazonQConnectAsync.CreateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSessionAsync(CreateSessionRequest createSessionRequest);
/**
*
* Creates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* creates a new Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param createSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSession operation returned by the service.
* @sample AmazonQConnectAsyncHandler.CreateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSessionAsync(CreateSessionRequest createSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an assistant.
*
*
* @param deleteAssistantRequest
* @return A Java Future containing the result of the DeleteAssistant operation returned by the service.
* @sample AmazonQConnectAsync.DeleteAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssistantAsync(DeleteAssistantRequest deleteAssistantRequest);
/**
*
* Deletes an assistant.
*
*
* @param deleteAssistantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssistant operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAssistantAsync(DeleteAssistantRequest deleteAssistantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an assistant association.
*
*
* @param deleteAssistantAssociationRequest
* @return A Java Future containing the result of the DeleteAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsync.DeleteAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssistantAssociationAsync(
DeleteAssistantAssociationRequest deleteAssistantAssociationRequest);
/**
*
* Deletes an assistant association.
*
*
* @param deleteAssistantAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAssistantAssociationAsync(
DeleteAssistantAssociationRequest deleteAssistantAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the content.
*
*
* @param deleteContentRequest
* @return A Java Future containing the result of the DeleteContent operation returned by the service.
* @sample AmazonQConnectAsync.DeleteContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContentAsync(DeleteContentRequest deleteContentRequest);
/**
*
* Deletes the content.
*
*
* @param deleteContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContent operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteContentAsync(DeleteContentRequest deleteContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param deleteContentAssociationRequest
* @return A Java Future containing the result of the DeleteContentAssociation operation returned by the service.
* @sample AmazonQConnectAsync.DeleteContentAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContentAssociationAsync(DeleteContentAssociationRequest deleteContentAssociationRequest);
/**
*
* Deletes the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param deleteContentAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteContentAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteContentAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteContentAssociationAsync(DeleteContentAssociationRequest deleteContentAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the quick response import job.
*
*
* @param deleteImportJobRequest
* @return A Java Future containing the result of the DeleteImportJob operation returned by the service.
* @sample AmazonQConnectAsync.DeleteImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteImportJobAsync(DeleteImportJobRequest deleteImportJobRequest);
/**
*
* Deletes the quick response import job.
*
*
* @param deleteImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteImportJob operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteImportJobAsync(DeleteImportJobRequest deleteImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the knowledge base.
*
*
*
* When you use this API to delete an external knowledge base such as Salesforce or ServiceNow, you must also delete
* the Amazon
* AppIntegrations DataIntegration. This is because you can't reuse the DataIntegration after it's been
* associated with an external knowledge base. However, you can delete and recreate it. See DeleteDataIntegration and CreateDataIntegration in the Amazon AppIntegrations API Reference.
*
*
*
* @param deleteKnowledgeBaseRequest
* @return A Java Future containing the result of the DeleteKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsync.DeleteKnowledgeBase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteKnowledgeBaseAsync(DeleteKnowledgeBaseRequest deleteKnowledgeBaseRequest);
/**
*
* Deletes the knowledge base.
*
*
*
* When you use this API to delete an external knowledge base such as Salesforce or ServiceNow, you must also delete
* the Amazon
* AppIntegrations DataIntegration. This is because you can't reuse the DataIntegration after it's been
* associated with an external knowledge base. However, you can delete and recreate it. See DeleteDataIntegration and CreateDataIntegration in the Amazon AppIntegrations API Reference.
*
*
*
* @param deleteKnowledgeBaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteKnowledgeBase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteKnowledgeBaseAsync(DeleteKnowledgeBaseRequest deleteKnowledgeBaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a quick response.
*
*
* @param deleteQuickResponseRequest
* @return A Java Future containing the result of the DeleteQuickResponse operation returned by the service.
* @sample AmazonQConnectAsync.DeleteQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteQuickResponseAsync(DeleteQuickResponseRequest deleteQuickResponseRequest);
/**
*
* Deletes a quick response.
*
*
* @param deleteQuickResponseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteQuickResponse operation returned by the service.
* @sample AmazonQConnectAsyncHandler.DeleteQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteQuickResponseAsync(DeleteQuickResponseRequest deleteQuickResponseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about an assistant.
*
*
* @param getAssistantRequest
* @return A Java Future containing the result of the GetAssistant operation returned by the service.
* @sample AmazonQConnectAsync.GetAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssistantAsync(GetAssistantRequest getAssistantRequest);
/**
*
* Retrieves information about an assistant.
*
*
* @param getAssistantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssistant operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetAssistant
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAssistantAsync(GetAssistantRequest getAssistantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about an assistant association.
*
*
* @param getAssistantAssociationRequest
* @return A Java Future containing the result of the GetAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsync.GetAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssistantAssociationAsync(GetAssistantAssociationRequest getAssistantAssociationRequest);
/**
*
* Retrieves information about an assistant association.
*
*
* @param getAssistantAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAssistantAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetAssistantAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future getAssistantAssociationAsync(GetAssistantAssociationRequest getAssistantAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves content, including a pre-signed URL to download the content.
*
*
* @param getContentRequest
* @return A Java Future containing the result of the GetContent operation returned by the service.
* @sample AmazonQConnectAsync.GetContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContentAsync(GetContentRequest getContentRequest);
/**
*
* Retrieves content, including a pre-signed URL to download the content.
*
*
* @param getContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContent operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContentAsync(GetContentRequest getContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param getContentAssociationRequest
* @return A Java Future containing the result of the GetContentAssociation operation returned by the service.
* @sample AmazonQConnectAsync.GetContentAssociation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContentAssociationAsync(GetContentAssociationRequest getContentAssociationRequest);
/**
*
* Returns the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param getContentAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContentAssociation operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetContentAssociation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getContentAssociationAsync(GetContentAssociationRequest getContentAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves summary information about the content.
*
*
* @param getContentSummaryRequest
* @return A Java Future containing the result of the GetContentSummary operation returned by the service.
* @sample AmazonQConnectAsync.GetContentSummary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContentSummaryAsync(GetContentSummaryRequest getContentSummaryRequest);
/**
*
* Retrieves summary information about the content.
*
*
* @param getContentSummaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetContentSummary operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetContentSummary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getContentSummaryAsync(GetContentSummaryRequest getContentSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the started import job.
*
*
* @param getImportJobRequest
* @return A Java Future containing the result of the GetImportJob operation returned by the service.
* @sample AmazonQConnectAsync.GetImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getImportJobAsync(GetImportJobRequest getImportJobRequest);
/**
*
* Retrieves the started import job.
*
*
* @param getImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetImportJob operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getImportJobAsync(GetImportJobRequest getImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information about the knowledge base.
*
*
* @param getKnowledgeBaseRequest
* @return A Java Future containing the result of the GetKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsync.GetKnowledgeBase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getKnowledgeBaseAsync(GetKnowledgeBaseRequest getKnowledgeBaseRequest);
/**
*
* Retrieves information about the knowledge base.
*
*
* @param getKnowledgeBaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetKnowledgeBase operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetKnowledgeBase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getKnowledgeBaseAsync(GetKnowledgeBaseRequest getKnowledgeBaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the quick response.
*
*
* @param getQuickResponseRequest
* @return A Java Future containing the result of the GetQuickResponse operation returned by the service.
* @sample AmazonQConnectAsync.GetQuickResponse
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuickResponseAsync(GetQuickResponseRequest getQuickResponseRequest);
/**
*
* Retrieves the quick response.
*
*
* @param getQuickResponseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQuickResponse operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetQuickResponse
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuickResponseAsync(GetQuickResponseRequest getQuickResponseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Retrieves recommendations for the specified session. To avoid retrieving the same recommendations in subsequent
* calls, use NotifyRecommendationsReceived. This API supports long-polling behavior with the waitTimeSeconds
* parameter. Short poll is the default behavior and only returns recommendations already available. To perform a
* manual query against an assistant, use QueryAssistant.
*
*
* @param getRecommendationsRequest
* @return A Java Future containing the result of the GetRecommendations operation returned by the service.
* @sample AmazonQConnectAsync.GetRecommendations
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future getRecommendationsAsync(GetRecommendationsRequest getRecommendationsRequest);
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Retrieves recommendations for the specified session. To avoid retrieving the same recommendations in subsequent
* calls, use NotifyRecommendationsReceived. This API supports long-polling behavior with the waitTimeSeconds
* parameter. Short poll is the default behavior and only returns recommendations already available. To perform a
* manual query against an assistant, use QueryAssistant.
*
*
* @param getRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetRecommendations operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetRecommendations
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future getRecommendationsAsync(GetRecommendationsRequest getRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves information for a specified session.
*
*
* @param getSessionRequest
* @return A Java Future containing the result of the GetSession operation returned by the service.
* @sample AmazonQConnectAsync.GetSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionAsync(GetSessionRequest getSessionRequest);
/**
*
* Retrieves information for a specified session.
*
*
* @param getSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSession operation returned by the service.
* @sample AmazonQConnectAsyncHandler.GetSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getSessionAsync(GetSessionRequest getSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about assistant associations.
*
*
* @param listAssistantAssociationsRequest
* @return A Java Future containing the result of the ListAssistantAssociations operation returned by the service.
* @sample AmazonQConnectAsync.ListAssistantAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssistantAssociationsAsync(
ListAssistantAssociationsRequest listAssistantAssociationsRequest);
/**
*
* Lists information about assistant associations.
*
*
* @param listAssistantAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssistantAssociations operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListAssistantAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAssistantAssociationsAsync(
ListAssistantAssociationsRequest listAssistantAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about assistants.
*
*
* @param listAssistantsRequest
* @return A Java Future containing the result of the ListAssistants operation returned by the service.
* @sample AmazonQConnectAsync.ListAssistants
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssistantsAsync(ListAssistantsRequest listAssistantsRequest);
/**
*
* Lists information about assistants.
*
*
* @param listAssistantsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAssistants operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListAssistants
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAssistantsAsync(ListAssistantsRequest listAssistantsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the content associations.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param listContentAssociationsRequest
* @return A Java Future containing the result of the ListContentAssociations operation returned by the service.
* @sample AmazonQConnectAsync.ListContentAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listContentAssociationsAsync(ListContentAssociationsRequest listContentAssociationsRequest);
/**
*
* Lists the content associations.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param listContentAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContentAssociations operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListContentAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listContentAssociationsAsync(ListContentAssociationsRequest listContentAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the content.
*
*
* @param listContentsRequest
* @return A Java Future containing the result of the ListContents operation returned by the service.
* @sample AmazonQConnectAsync.ListContents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContentsAsync(ListContentsRequest listContentsRequest);
/**
*
* Lists the content.
*
*
* @param listContentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContents operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListContents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listContentsAsync(ListContentsRequest listContentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about import jobs.
*
*
* @param listImportJobsRequest
* @return A Java Future containing the result of the ListImportJobs operation returned by the service.
* @sample AmazonQConnectAsync.ListImportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportJobsAsync(ListImportJobsRequest listImportJobsRequest);
/**
*
* Lists information about import jobs.
*
*
* @param listImportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImportJobs operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListImportJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportJobsAsync(ListImportJobsRequest listImportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the knowledge bases.
*
*
* @param listKnowledgeBasesRequest
* @return A Java Future containing the result of the ListKnowledgeBases operation returned by the service.
* @sample AmazonQConnectAsync.ListKnowledgeBases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listKnowledgeBasesAsync(ListKnowledgeBasesRequest listKnowledgeBasesRequest);
/**
*
* Lists the knowledge bases.
*
*
* @param listKnowledgeBasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListKnowledgeBases operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListKnowledgeBases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listKnowledgeBasesAsync(ListKnowledgeBasesRequest listKnowledgeBasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists information about quick response.
*
*
* @param listQuickResponsesRequest
* @return A Java Future containing the result of the ListQuickResponses operation returned by the service.
* @sample AmazonQConnectAsync.ListQuickResponses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listQuickResponsesAsync(ListQuickResponsesRequest listQuickResponsesRequest);
/**
*
* Lists information about quick response.
*
*
* @param listQuickResponsesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListQuickResponses operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListQuickResponses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listQuickResponsesAsync(ListQuickResponsesRequest listQuickResponsesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonQConnectAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonQConnectAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified recommendations from the specified assistant's queue of newly available recommendations.
* You can use this API in conjunction with GetRecommendations and a waitTimeSeconds
input for long-polling behavior and avoiding duplicate
* recommendations.
*
*
* @param notifyRecommendationsReceivedRequest
* @return A Java Future containing the result of the NotifyRecommendationsReceived operation returned by the
* service.
* @sample AmazonQConnectAsync.NotifyRecommendationsReceived
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyRecommendationsReceivedAsync(
NotifyRecommendationsReceivedRequest notifyRecommendationsReceivedRequest);
/**
*
* Removes the specified recommendations from the specified assistant's queue of newly available recommendations.
* You can use this API in conjunction with GetRecommendations and a waitTimeSeconds
input for long-polling behavior and avoiding duplicate
* recommendations.
*
*
* @param notifyRecommendationsReceivedRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the NotifyRecommendationsReceived operation returned by the
* service.
* @sample AmazonQConnectAsyncHandler.NotifyRecommendationsReceived
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyRecommendationsReceivedAsync(
NotifyRecommendationsReceivedRequest notifyRecommendationsReceivedRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides feedback against the specified assistant for the specified target. This API only supports generative
* targets.
*
*
* @param putFeedbackRequest
* @return A Java Future containing the result of the PutFeedback operation returned by the service.
* @sample AmazonQConnectAsync.PutFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putFeedbackAsync(PutFeedbackRequest putFeedbackRequest);
/**
*
* Provides feedback against the specified assistant for the specified target. This API only supports generative
* targets.
*
*
* @param putFeedbackRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutFeedback operation returned by the service.
* @sample AmazonQConnectAsyncHandler.PutFeedback
* @see AWS API
* Documentation
*/
java.util.concurrent.Future putFeedbackAsync(PutFeedbackRequest putFeedbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Performs a manual search against the specified assistant. To retrieve recommendations for an assistant, use
* GetRecommendations.
*
*
* @param queryAssistantRequest
* @return A Java Future containing the result of the QueryAssistant operation returned by the service.
* @sample AmazonQConnectAsync.QueryAssistant
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future queryAssistantAsync(QueryAssistantRequest queryAssistantRequest);
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Performs a manual search against the specified assistant. To retrieve recommendations for an assistant, use
* GetRecommendations.
*
*
* @param queryAssistantRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the QueryAssistant operation returned by the service.
* @sample AmazonQConnectAsyncHandler.QueryAssistant
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future queryAssistantAsync(QueryAssistantRequest queryAssistantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a URI template from a knowledge base.
*
*
* @param removeKnowledgeBaseTemplateUriRequest
* @return A Java Future containing the result of the RemoveKnowledgeBaseTemplateUri operation returned by the
* service.
* @sample AmazonQConnectAsync.RemoveKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
java.util.concurrent.Future removeKnowledgeBaseTemplateUriAsync(
RemoveKnowledgeBaseTemplateUriRequest removeKnowledgeBaseTemplateUriRequest);
/**
*
* Removes a URI template from a knowledge base.
*
*
* @param removeKnowledgeBaseTemplateUriRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveKnowledgeBaseTemplateUri operation returned by the
* service.
* @sample AmazonQConnectAsyncHandler.RemoveKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
java.util.concurrent.Future removeKnowledgeBaseTemplateUriAsync(
RemoveKnowledgeBaseTemplateUriRequest removeKnowledgeBaseTemplateUriRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for content in a specified knowledge base. Can be used to get a specific content resource by its name.
*
*
* @param searchContentRequest
* @return A Java Future containing the result of the SearchContent operation returned by the service.
* @sample AmazonQConnectAsync.SearchContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchContentAsync(SearchContentRequest searchContentRequest);
/**
*
* Searches for content in a specified knowledge base. Can be used to get a specific content resource by its name.
*
*
* @param searchContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchContent operation returned by the service.
* @sample AmazonQConnectAsyncHandler.SearchContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchContentAsync(SearchContentRequest searchContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches existing Amazon Q in Connect quick responses in an Amazon Q in Connect knowledge base.
*
*
* @param searchQuickResponsesRequest
* @return A Java Future containing the result of the SearchQuickResponses operation returned by the service.
* @sample AmazonQConnectAsync.SearchQuickResponses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchQuickResponsesAsync(SearchQuickResponsesRequest searchQuickResponsesRequest);
/**
*
* Searches existing Amazon Q in Connect quick responses in an Amazon Q in Connect knowledge base.
*
*
* @param searchQuickResponsesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchQuickResponses operation returned by the service.
* @sample AmazonQConnectAsyncHandler.SearchQuickResponses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future searchQuickResponsesAsync(SearchQuickResponsesRequest searchQuickResponsesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for sessions.
*
*
* @param searchSessionsRequest
* @return A Java Future containing the result of the SearchSessions operation returned by the service.
* @sample AmazonQConnectAsync.SearchSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchSessionsAsync(SearchSessionsRequest searchSessionsRequest);
/**
*
* Searches for sessions.
*
*
* @param searchSessionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchSessions operation returned by the service.
* @sample AmazonQConnectAsyncHandler.SearchSessions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchSessionsAsync(SearchSessionsRequest searchSessionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Get a URL to upload content to a knowledge base. To upload content, first make a PUT request to the returned URL
* with your file, making sure to include the required headers. Then use CreateContent
* to finalize the content creation process or UpdateContent
* to modify an existing resource. You can only upload content to a knowledge base of type CUSTOM.
*
*
* @param startContentUploadRequest
* @return A Java Future containing the result of the StartContentUpload operation returned by the service.
* @sample AmazonQConnectAsync.StartContentUpload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startContentUploadAsync(StartContentUploadRequest startContentUploadRequest);
/**
*
* Get a URL to upload content to a knowledge base. To upload content, first make a PUT request to the returned URL
* with your file, making sure to include the required headers. Then use CreateContent
* to finalize the content creation process or UpdateContent
* to modify an existing resource. You can only upload content to a knowledge base of type CUSTOM.
*
*
* @param startContentUploadRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartContentUpload operation returned by the service.
* @sample AmazonQConnectAsyncHandler.StartContentUpload
* @see AWS
* API Documentation
*/
java.util.concurrent.Future startContentUploadAsync(StartContentUploadRequest startContentUploadRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start an asynchronous job to import Amazon Q in Connect resources from an uploaded source file. Before calling
* this API, use StartContentUpload
* to upload an asset that contains the resource data.
*
*
* -
*
* For importing Amazon Q in Connect quick responses, you need to upload a csv file including the quick responses.
* For information about how to format the csv file for importing quick responses, see Import quick responses.
*
*
*
*
* @param startImportJobRequest
* @return A Java Future containing the result of the StartImportJob operation returned by the service.
* @sample AmazonQConnectAsync.StartImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startImportJobAsync(StartImportJobRequest startImportJobRequest);
/**
*
* Start an asynchronous job to import Amazon Q in Connect resources from an uploaded source file. Before calling
* this API, use StartContentUpload
* to upload an asset that contains the resource data.
*
*
* -
*
* For importing Amazon Q in Connect quick responses, you need to upload a csv file including the quick responses.
* For information about how to format the csv file for importing quick responses, see Import quick responses.
*
*
*
*
* @param startImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartImportJob operation returned by the service.
* @sample AmazonQConnectAsyncHandler.StartImportJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startImportJobAsync(StartImportJobRequest startImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonQConnectAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds the specified tags to the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonQConnectAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonQConnectAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from the specified resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonQConnectAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates information about the content.
*
*
* @param updateContentRequest
* @return A Java Future containing the result of the UpdateContent operation returned by the service.
* @sample AmazonQConnectAsync.UpdateContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContentAsync(UpdateContentRequest updateContentRequest);
/**
*
* Updates information about the content.
*
*
* @param updateContentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateContent operation returned by the service.
* @sample AmazonQConnectAsyncHandler.UpdateContent
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateContentAsync(UpdateContentRequest updateContentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the template URI of a knowledge base. This is only supported for knowledge bases of type EXTERNAL.
* Include a single variable in ${variable}
format; this interpolated by Amazon Q in Connect using
* ingested content. For example, if you ingest a Salesforce article, it has an Id
value, and you can
* set the template URI to
* https://myInstanceName.lightning.force.com/lightning/r/Knowledge__kav/*${Id}*/view
.
*
*
* @param updateKnowledgeBaseTemplateUriRequest
* @return A Java Future containing the result of the UpdateKnowledgeBaseTemplateUri operation returned by the
* service.
* @sample AmazonQConnectAsync.UpdateKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
java.util.concurrent.Future updateKnowledgeBaseTemplateUriAsync(
UpdateKnowledgeBaseTemplateUriRequest updateKnowledgeBaseTemplateUriRequest);
/**
*
* Updates the template URI of a knowledge base. This is only supported for knowledge bases of type EXTERNAL.
* Include a single variable in ${variable}
format; this interpolated by Amazon Q in Connect using
* ingested content. For example, if you ingest a Salesforce article, it has an Id
value, and you can
* set the template URI to
* https://myInstanceName.lightning.force.com/lightning/r/Knowledge__kav/*${Id}*/view
.
*
*
* @param updateKnowledgeBaseTemplateUriRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateKnowledgeBaseTemplateUri operation returned by the
* service.
* @sample AmazonQConnectAsyncHandler.UpdateKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
java.util.concurrent.Future updateKnowledgeBaseTemplateUriAsync(
UpdateKnowledgeBaseTemplateUriRequest updateKnowledgeBaseTemplateUriRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing Amazon Q in Connect quick response.
*
*
* @param updateQuickResponseRequest
* @return A Java Future containing the result of the UpdateQuickResponse operation returned by the service.
* @sample AmazonQConnectAsync.UpdateQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateQuickResponseAsync(UpdateQuickResponseRequest updateQuickResponseRequest);
/**
*
* Updates an existing Amazon Q in Connect quick response.
*
*
* @param updateQuickResponseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateQuickResponse operation returned by the service.
* @sample AmazonQConnectAsyncHandler.UpdateQuickResponse
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateQuickResponseAsync(UpdateQuickResponseRequest updateQuickResponseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* updates the existing Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param updateSessionRequest
* @return A Java Future containing the result of the UpdateSession operation returned by the service.
* @sample AmazonQConnectAsync.UpdateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSessionAsync(UpdateSessionRequest updateSessionRequest);
/**
*
* Updates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* updates the existing Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param updateSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSession operation returned by the service.
* @sample AmazonQConnectAsyncHandler.UpdateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateSessionAsync(UpdateSessionRequest updateSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}