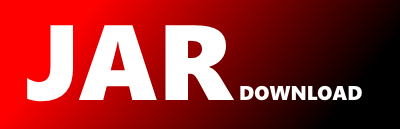
com.amazonaws.services.qconnect.AmazonQConnectClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-qconnect Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.qconnect;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.qconnect.AmazonQConnectClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.qconnect.model.*;
import com.amazonaws.services.qconnect.model.transform.*;
/**
* Client for accessing Amazon Q Connect. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
*
* Powered by Amazon Bedrock: Amazon Web Services implements automated abuse detection.
* Because Amazon Q in Connect is built on Amazon Bedrock, users can take full advantage of the controls implemented in
* Amazon Bedrock to enforce safety, security, and the responsible use of artificial intelligence (AI).
*
*
*
* Amazon Q in Connect is a generative AI customer service assistant. It is an LLM-enhanced evolution of Amazon Connect
* Wisdom that delivers real-time recommendations to help contact center agents resolve customer issues quickly and
* accurately.
*
*
* Amazon Q in Connect automatically detects customer intent during calls and chats using conversational analytics and
* natural language understanding (NLU). It then provides agents with immediate, real-time generative responses and
* suggested actions, and links to relevant documents and articles. Agents can also query Amazon Q in Connect directly
* using natural language or keywords to answer customer requests.
*
*
* Use the Amazon Q in Connect APIs to create an assistant and a knowledge base, for example, or manage content by
* uploading custom files.
*
*
* For more information, see Use
* Amazon Q in Connect for generative AI powered agent assistance in real-time in the Amazon Connect
* Administrator Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonQConnectClient extends AmazonWebServiceClient implements AmazonQConnect {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonQConnect.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "wisdom";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("PreconditionFailedException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.PreconditionFailedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("RequestTimeoutException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.RequestTimeoutExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.qconnect.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.qconnect.model.AmazonQConnectException.class));
public static AmazonQConnectClientBuilder builder() {
return AmazonQConnectClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Q Connect using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonQConnectClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Q Connect using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonQConnectClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("wisdom.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/qconnect/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/qconnect/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates an Amazon Q in Connect assistant.
*
*
* @param createAssistantRequest
* @return Result of the CreateAssistant operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonQConnect.CreateAssistant
* @see AWS API
* Documentation
*/
@Override
public CreateAssistantResult createAssistant(CreateAssistantRequest request) {
request = beforeClientExecution(request);
return executeCreateAssistant(request);
}
@SdkInternalApi
final CreateAssistantResult executeCreateAssistant(CreateAssistantRequest createAssistantRequest) {
ExecutionContext executionContext = createExecutionContext(createAssistantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssistantRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAssistantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAssistant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAssistantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an association between an Amazon Q in Connect assistant and another resource. Currently, the only
* supported association is with a knowledge base. An assistant can have only a single association.
*
*
* @param createAssistantAssociationRequest
* @return Result of the CreateAssistantAssociation operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.CreateAssistantAssociation
* @see AWS API Documentation
*/
@Override
public CreateAssistantAssociationResult createAssistantAssociation(CreateAssistantAssociationRequest request) {
request = beforeClientExecution(request);
return executeCreateAssistantAssociation(request);
}
@SdkInternalApi
final CreateAssistantAssociationResult executeCreateAssistantAssociation(CreateAssistantAssociationRequest createAssistantAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(createAssistantAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAssistantAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createAssistantAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAssistantAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAssistantAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates Amazon Q in Connect content. Before to calling this API, use StartContentUpload to upload an asset.
*
*
* @param createContentRequest
* @return Result of the CreateContent operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.CreateContent
* @see AWS API
* Documentation
*/
@Override
public CreateContentResult createContent(CreateContentRequest request) {
request = beforeClientExecution(request);
return executeCreateContent(request);
}
@SdkInternalApi
final CreateContentResult executeCreateContent(CreateContentRequest createContentRequest) {
ExecutionContext executionContext = createExecutionContext(createContentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateContentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createContentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateContent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateContentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an association between a content resource in a knowledge base and step-by-step
* guides. Step-by-step guides offer instructions to agents for resolving common customer issues. You create a
* content association to integrate Amazon Q in Connect and step-by-step guides.
*
*
* After you integrate Amazon Q and step-by-step guides, when Amazon Q provides a recommendation to an agent based
* on the intent that it's detected, it also provides them with the option to start the step-by-step guide that you
* have associated with the content.
*
*
* Note the following limitations:
*
*
* -
*
* You can create only one content association for each content resource in a knowledge base.
*
*
* -
*
* You can associate a step-by-step guide with multiple content resources.
*
*
*
*
* For more information, see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param createContentAssociationRequest
* @return Result of the CreateContentAssociation operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ThrottlingException
* The throttling limit has been exceeded.
* @sample AmazonQConnect.CreateContentAssociation
* @see AWS API Documentation
*/
@Override
public CreateContentAssociationResult createContentAssociation(CreateContentAssociationRequest request) {
request = beforeClientExecution(request);
return executeCreateContentAssociation(request);
}
@SdkInternalApi
final CreateContentAssociationResult executeCreateContentAssociation(CreateContentAssociationRequest createContentAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(createContentAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateContentAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createContentAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateContentAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateContentAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a knowledge base.
*
*
*
* When using this API, you cannot reuse Amazon AppIntegrations
* DataIntegrations with external knowledge bases such as Salesforce and ServiceNow. If you do, you'll get an
* InvalidRequestException
error.
*
*
* For example, you're programmatically managing your external knowledge base, and you want to add or remove one of
* the fields that is being ingested from Salesforce. Do the following:
*
*
* -
*
* Call
* DeleteKnowledgeBase.
*
*
* -
*
* Call
* DeleteDataIntegration.
*
*
* -
*
* Call
* CreateDataIntegration to recreate the DataIntegration or a create different one.
*
*
* -
*
* Call CreateKnowledgeBase.
*
*
*
*
*
* @param createKnowledgeBaseRequest
* @return Result of the CreateKnowledgeBase operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonQConnect.CreateKnowledgeBase
* @see AWS
* API Documentation
*/
@Override
public CreateKnowledgeBaseResult createKnowledgeBase(CreateKnowledgeBaseRequest request) {
request = beforeClientExecution(request);
return executeCreateKnowledgeBase(request);
}
@SdkInternalApi
final CreateKnowledgeBaseResult executeCreateKnowledgeBase(CreateKnowledgeBaseRequest createKnowledgeBaseRequest) {
ExecutionContext executionContext = createExecutionContext(createKnowledgeBaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateKnowledgeBaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createKnowledgeBaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateKnowledgeBase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateKnowledgeBaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Q in Connect quick response.
*
*
* @param createQuickResponseRequest
* @return Result of the CreateQuickResponse operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.CreateQuickResponse
* @see AWS
* API Documentation
*/
@Override
public CreateQuickResponseResult createQuickResponse(CreateQuickResponseRequest request) {
request = beforeClientExecution(request);
return executeCreateQuickResponse(request);
}
@SdkInternalApi
final CreateQuickResponseResult executeCreateQuickResponse(CreateQuickResponseRequest createQuickResponseRequest) {
ExecutionContext executionContext = createExecutionContext(createQuickResponseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateQuickResponseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createQuickResponseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateQuickResponse");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateQuickResponseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* creates a new Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param createSessionRequest
* @return Result of the CreateSession operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.CreateSession
* @see AWS API
* Documentation
*/
@Override
public CreateSessionResult createSession(CreateSessionRequest request) {
request = beforeClientExecution(request);
return executeCreateSession(request);
}
@SdkInternalApi
final CreateSessionResult executeCreateSession(CreateSessionRequest createSessionRequest) {
ExecutionContext executionContext = createExecutionContext(createSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an assistant.
*
*
* @param deleteAssistantRequest
* @return Result of the DeleteAssistant operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteAssistant
* @see AWS API
* Documentation
*/
@Override
public DeleteAssistantResult deleteAssistant(DeleteAssistantRequest request) {
request = beforeClientExecution(request);
return executeDeleteAssistant(request);
}
@SdkInternalApi
final DeleteAssistantResult executeDeleteAssistant(DeleteAssistantRequest deleteAssistantRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAssistantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAssistantRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAssistantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAssistant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAssistantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an assistant association.
*
*
* @param deleteAssistantAssociationRequest
* @return Result of the DeleteAssistantAssociation operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteAssistantAssociation
* @see AWS API Documentation
*/
@Override
public DeleteAssistantAssociationResult deleteAssistantAssociation(DeleteAssistantAssociationRequest request) {
request = beforeClientExecution(request);
return executeDeleteAssistantAssociation(request);
}
@SdkInternalApi
final DeleteAssistantAssociationResult executeDeleteAssistantAssociation(DeleteAssistantAssociationRequest deleteAssistantAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAssistantAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAssistantAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteAssistantAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAssistantAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAssistantAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the content.
*
*
* @param deleteContentRequest
* @return Result of the DeleteContent operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteContent
* @see AWS API
* Documentation
*/
@Override
public DeleteContentResult deleteContent(DeleteContentRequest request) {
request = beforeClientExecution(request);
return executeDeleteContent(request);
}
@SdkInternalApi
final DeleteContentResult executeDeleteContent(DeleteContentRequest deleteContentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteContentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteContentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteContentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteContent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteContentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param deleteContentAssociationRequest
* @return Result of the DeleteContentAssociation operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteContentAssociation
* @see AWS API Documentation
*/
@Override
public DeleteContentAssociationResult deleteContentAssociation(DeleteContentAssociationRequest request) {
request = beforeClientExecution(request);
return executeDeleteContentAssociation(request);
}
@SdkInternalApi
final DeleteContentAssociationResult executeDeleteContentAssociation(DeleteContentAssociationRequest deleteContentAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteContentAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteContentAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteContentAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteContentAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteContentAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the quick response import job.
*
*
* @param deleteImportJobRequest
* @return Result of the DeleteImportJob operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteImportJob
* @see AWS API
* Documentation
*/
@Override
public DeleteImportJobResult deleteImportJob(DeleteImportJobRequest request) {
request = beforeClientExecution(request);
return executeDeleteImportJob(request);
}
@SdkInternalApi
final DeleteImportJobResult executeDeleteImportJob(DeleteImportJobRequest deleteImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(deleteImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the knowledge base.
*
*
*
* When you use this API to delete an external knowledge base such as Salesforce or ServiceNow, you must also delete
* the Amazon
* AppIntegrations DataIntegration. This is because you can't reuse the DataIntegration after it's been
* associated with an external knowledge base. However, you can delete and recreate it. See DeleteDataIntegration and CreateDataIntegration in the Amazon AppIntegrations API Reference.
*
*
*
* @param deleteKnowledgeBaseRequest
* @return Result of the DeleteKnowledgeBase operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteKnowledgeBase
* @see AWS
* API Documentation
*/
@Override
public DeleteKnowledgeBaseResult deleteKnowledgeBase(DeleteKnowledgeBaseRequest request) {
request = beforeClientExecution(request);
return executeDeleteKnowledgeBase(request);
}
@SdkInternalApi
final DeleteKnowledgeBaseResult executeDeleteKnowledgeBase(DeleteKnowledgeBaseRequest deleteKnowledgeBaseRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKnowledgeBaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteKnowledgeBaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteKnowledgeBaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteKnowledgeBase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteKnowledgeBaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a quick response.
*
*
* @param deleteQuickResponseRequest
* @return Result of the DeleteQuickResponse operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.DeleteQuickResponse
* @see AWS
* API Documentation
*/
@Override
public DeleteQuickResponseResult deleteQuickResponse(DeleteQuickResponseRequest request) {
request = beforeClientExecution(request);
return executeDeleteQuickResponse(request);
}
@SdkInternalApi
final DeleteQuickResponseResult executeDeleteQuickResponse(DeleteQuickResponseRequest deleteQuickResponseRequest) {
ExecutionContext executionContext = createExecutionContext(deleteQuickResponseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteQuickResponseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteQuickResponseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteQuickResponse");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteQuickResponseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about an assistant.
*
*
* @param getAssistantRequest
* @return Result of the GetAssistant operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetAssistant
* @see AWS API
* Documentation
*/
@Override
public GetAssistantResult getAssistant(GetAssistantRequest request) {
request = beforeClientExecution(request);
return executeGetAssistant(request);
}
@SdkInternalApi
final GetAssistantResult executeGetAssistant(GetAssistantRequest getAssistantRequest) {
ExecutionContext executionContext = createExecutionContext(getAssistantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAssistantRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAssistantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAssistant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAssistantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about an assistant association.
*
*
* @param getAssistantAssociationRequest
* @return Result of the GetAssistantAssociation operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetAssistantAssociation
* @see AWS API Documentation
*/
@Override
public GetAssistantAssociationResult getAssistantAssociation(GetAssistantAssociationRequest request) {
request = beforeClientExecution(request);
return executeGetAssistantAssociation(request);
}
@SdkInternalApi
final GetAssistantAssociationResult executeGetAssistantAssociation(GetAssistantAssociationRequest getAssistantAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(getAssistantAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAssistantAssociationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAssistantAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAssistantAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAssistantAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves content, including a pre-signed URL to download the content.
*
*
* @param getContentRequest
* @return Result of the GetContent operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetContent
* @see AWS API
* Documentation
*/
@Override
public GetContentResult getContent(GetContentRequest request) {
request = beforeClientExecution(request);
return executeGetContent(request);
}
@SdkInternalApi
final GetContentResult executeGetContent(GetContentRequest getContentRequest) {
ExecutionContext executionContext = createExecutionContext(getContentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetContentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getContentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetContent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetContentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the content association.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param getContentAssociationRequest
* @return Result of the GetContentAssociation operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetContentAssociation
* @see AWS
* API Documentation
*/
@Override
public GetContentAssociationResult getContentAssociation(GetContentAssociationRequest request) {
request = beforeClientExecution(request);
return executeGetContentAssociation(request);
}
@SdkInternalApi
final GetContentAssociationResult executeGetContentAssociation(GetContentAssociationRequest getContentAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(getContentAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetContentAssociationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getContentAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetContentAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetContentAssociationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves summary information about the content.
*
*
* @param getContentSummaryRequest
* @return Result of the GetContentSummary operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetContentSummary
* @see AWS API
* Documentation
*/
@Override
public GetContentSummaryResult getContentSummary(GetContentSummaryRequest request) {
request = beforeClientExecution(request);
return executeGetContentSummary(request);
}
@SdkInternalApi
final GetContentSummaryResult executeGetContentSummary(GetContentSummaryRequest getContentSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getContentSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetContentSummaryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getContentSummaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetContentSummary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetContentSummaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the started import job.
*
*
* @param getImportJobRequest
* @return Result of the GetImportJob operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetImportJob
* @see AWS API
* Documentation
*/
@Override
public GetImportJobResult getImportJob(GetImportJobRequest request) {
request = beforeClientExecution(request);
return executeGetImportJob(request);
}
@SdkInternalApi
final GetImportJobResult executeGetImportJob(GetImportJobRequest getImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(getImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the knowledge base.
*
*
* @param getKnowledgeBaseRequest
* @return Result of the GetKnowledgeBase operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetKnowledgeBase
* @see AWS API
* Documentation
*/
@Override
public GetKnowledgeBaseResult getKnowledgeBase(GetKnowledgeBaseRequest request) {
request = beforeClientExecution(request);
return executeGetKnowledgeBase(request);
}
@SdkInternalApi
final GetKnowledgeBaseResult executeGetKnowledgeBase(GetKnowledgeBaseRequest getKnowledgeBaseRequest) {
ExecutionContext executionContext = createExecutionContext(getKnowledgeBaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetKnowledgeBaseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getKnowledgeBaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetKnowledgeBase");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetKnowledgeBaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the quick response.
*
*
* @param getQuickResponseRequest
* @return Result of the GetQuickResponse operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetQuickResponse
* @see AWS API
* Documentation
*/
@Override
public GetQuickResponseResult getQuickResponse(GetQuickResponseRequest request) {
request = beforeClientExecution(request);
return executeGetQuickResponse(request);
}
@SdkInternalApi
final GetQuickResponseResult executeGetQuickResponse(GetQuickResponseRequest getQuickResponseRequest) {
ExecutionContext executionContext = createExecutionContext(getQuickResponseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetQuickResponseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getQuickResponseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetQuickResponse");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetQuickResponseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Retrieves recommendations for the specified session. To avoid retrieving the same recommendations in subsequent
* calls, use NotifyRecommendationsReceived. This API supports long-polling behavior with the waitTimeSeconds
* parameter. Short poll is the default behavior and only returns recommendations already available. To perform a
* manual query against an assistant, use QueryAssistant.
*
*
* @param getRecommendationsRequest
* @return Result of the GetRecommendations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetRecommendations
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public GetRecommendationsResult getRecommendations(GetRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeGetRecommendations(request);
}
@SdkInternalApi
final GetRecommendationsResult executeGetRecommendations(GetRecommendationsRequest getRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(getRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information for a specified session.
*
*
* @param getSessionRequest
* @return Result of the GetSession operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.GetSession
* @see AWS API
* Documentation
*/
@Override
public GetSessionResult getSession(GetSessionRequest request) {
request = beforeClientExecution(request);
return executeGetSession(request);
}
@SdkInternalApi
final GetSessionResult executeGetSession(GetSessionRequest getSessionRequest) {
ExecutionContext executionContext = createExecutionContext(getSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists information about assistant associations.
*
*
* @param listAssistantAssociationsRequest
* @return Result of the ListAssistantAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.ListAssistantAssociations
* @see AWS API Documentation
*/
@Override
public ListAssistantAssociationsResult listAssistantAssociations(ListAssistantAssociationsRequest request) {
request = beforeClientExecution(request);
return executeListAssistantAssociations(request);
}
@SdkInternalApi
final ListAssistantAssociationsResult executeListAssistantAssociations(ListAssistantAssociationsRequest listAssistantAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(listAssistantAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssistantAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAssistantAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssistantAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAssistantAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists information about assistants.
*
*
* @param listAssistantsRequest
* @return Result of the ListAssistants operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonQConnect.ListAssistants
* @see AWS API
* Documentation
*/
@Override
public ListAssistantsResult listAssistants(ListAssistantsRequest request) {
request = beforeClientExecution(request);
return executeListAssistants(request);
}
@SdkInternalApi
final ListAssistantsResult executeListAssistants(ListAssistantsRequest listAssistantsRequest) {
ExecutionContext executionContext = createExecutionContext(listAssistantsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAssistantsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAssistantsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAssistants");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAssistantsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the content associations.
*
*
* For more information about content associations--what they are and when they are used--see Integrate Amazon Q in
* Connect with step-by-step guides in the Amazon Connect Administrator Guide.
*
*
* @param listContentAssociationsRequest
* @return Result of the ListContentAssociations operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.ListContentAssociations
* @see AWS API Documentation
*/
@Override
public ListContentAssociationsResult listContentAssociations(ListContentAssociationsRequest request) {
request = beforeClientExecution(request);
return executeListContentAssociations(request);
}
@SdkInternalApi
final ListContentAssociationsResult executeListContentAssociations(ListContentAssociationsRequest listContentAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(listContentAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListContentAssociationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listContentAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListContentAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListContentAssociationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the content.
*
*
* @param listContentsRequest
* @return Result of the ListContents operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.ListContents
* @see AWS API
* Documentation
*/
@Override
public ListContentsResult listContents(ListContentsRequest request) {
request = beforeClientExecution(request);
return executeListContents(request);
}
@SdkInternalApi
final ListContentsResult executeListContents(ListContentsRequest listContentsRequest) {
ExecutionContext executionContext = createExecutionContext(listContentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListContentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listContentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListContents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListContentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists information about import jobs.
*
*
* @param listImportJobsRequest
* @return Result of the ListImportJobs operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonQConnect.ListImportJobs
* @see AWS API
* Documentation
*/
@Override
public ListImportJobsResult listImportJobs(ListImportJobsRequest request) {
request = beforeClientExecution(request);
return executeListImportJobs(request);
}
@SdkInternalApi
final ListImportJobsResult executeListImportJobs(ListImportJobsRequest listImportJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listImportJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListImportJobsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listImportJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListImportJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListImportJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the knowledge bases.
*
*
* @param listKnowledgeBasesRequest
* @return Result of the ListKnowledgeBases operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AmazonQConnect.ListKnowledgeBases
* @see AWS
* API Documentation
*/
@Override
public ListKnowledgeBasesResult listKnowledgeBases(ListKnowledgeBasesRequest request) {
request = beforeClientExecution(request);
return executeListKnowledgeBases(request);
}
@SdkInternalApi
final ListKnowledgeBasesResult executeListKnowledgeBases(ListKnowledgeBasesRequest listKnowledgeBasesRequest) {
ExecutionContext executionContext = createExecutionContext(listKnowledgeBasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListKnowledgeBasesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listKnowledgeBasesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListKnowledgeBases");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListKnowledgeBasesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists information about quick response.
*
*
* @param listQuickResponsesRequest
* @return Result of the ListQuickResponses operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.ListQuickResponses
* @see AWS
* API Documentation
*/
@Override
public ListQuickResponsesResult listQuickResponses(ListQuickResponsesRequest request) {
request = beforeClientExecution(request);
return executeListQuickResponses(request);
}
@SdkInternalApi
final ListQuickResponsesResult executeListQuickResponses(ListQuickResponsesRequest listQuickResponsesRequest) {
ExecutionContext executionContext = createExecutionContext(listQuickResponsesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListQuickResponsesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listQuickResponsesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListQuickResponses");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListQuickResponsesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified recommendations from the specified assistant's queue of newly available recommendations.
* You can use this API in conjunction with GetRecommendations and a waitTimeSeconds
input for long-polling behavior and avoiding duplicate
* recommendations.
*
*
* @param notifyRecommendationsReceivedRequest
* @return Result of the NotifyRecommendationsReceived operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.NotifyRecommendationsReceived
* @see AWS API Documentation
*/
@Override
public NotifyRecommendationsReceivedResult notifyRecommendationsReceived(NotifyRecommendationsReceivedRequest request) {
request = beforeClientExecution(request);
return executeNotifyRecommendationsReceived(request);
}
@SdkInternalApi
final NotifyRecommendationsReceivedResult executeNotifyRecommendationsReceived(NotifyRecommendationsReceivedRequest notifyRecommendationsReceivedRequest) {
ExecutionContext executionContext = createExecutionContext(notifyRecommendationsReceivedRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new NotifyRecommendationsReceivedRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(notifyRecommendationsReceivedRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "NotifyRecommendationsReceived");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new NotifyRecommendationsReceivedResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Provides feedback against the specified assistant for the specified target. This API only supports generative
* targets.
*
*
* @param putFeedbackRequest
* @return Result of the PutFeedback operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.PutFeedback
* @see AWS API
* Documentation
*/
@Override
public PutFeedbackResult putFeedback(PutFeedbackRequest request) {
request = beforeClientExecution(request);
return executePutFeedback(request);
}
@SdkInternalApi
final PutFeedbackResult executePutFeedback(PutFeedbackRequest putFeedbackRequest) {
ExecutionContext executionContext = createExecutionContext(putFeedbackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutFeedbackRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putFeedbackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutFeedback");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutFeedbackResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This API will be discontinued starting June 1, 2024. To receive generative responses after March 1, 2024, you
* will need to create a new Assistant in the Amazon Connect console and integrate the Amazon Q in Connect
* JavaScript library (amazon-q-connectjs) into your applications.
*
*
*
* Performs a manual search against the specified assistant. To retrieve recommendations for an assistant, use
* GetRecommendations.
*
*
* @param queryAssistantRequest
* @return Result of the QueryAssistant operation returned by the service.
* @throws RequestTimeoutException
* The request reached the service more than 15 minutes after the date stamp on the request or more than 15
* minutes after the request expiration date (such as for pre-signed URLs), or the date stamp on the request
* is more than 15 minutes in the future.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.QueryAssistant
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public QueryAssistantResult queryAssistant(QueryAssistantRequest request) {
request = beforeClientExecution(request);
return executeQueryAssistant(request);
}
@SdkInternalApi
final QueryAssistantResult executeQueryAssistant(QueryAssistantRequest queryAssistantRequest) {
ExecutionContext executionContext = createExecutionContext(queryAssistantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new QueryAssistantRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(queryAssistantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "QueryAssistant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new QueryAssistantResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a URI template from a knowledge base.
*
*
* @param removeKnowledgeBaseTemplateUriRequest
* @return Result of the RemoveKnowledgeBaseTemplateUri operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.RemoveKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
@Override
public RemoveKnowledgeBaseTemplateUriResult removeKnowledgeBaseTemplateUri(RemoveKnowledgeBaseTemplateUriRequest request) {
request = beforeClientExecution(request);
return executeRemoveKnowledgeBaseTemplateUri(request);
}
@SdkInternalApi
final RemoveKnowledgeBaseTemplateUriResult executeRemoveKnowledgeBaseTemplateUri(RemoveKnowledgeBaseTemplateUriRequest removeKnowledgeBaseTemplateUriRequest) {
ExecutionContext executionContext = createExecutionContext(removeKnowledgeBaseTemplateUriRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveKnowledgeBaseTemplateUriRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(removeKnowledgeBaseTemplateUriRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveKnowledgeBaseTemplateUri");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RemoveKnowledgeBaseTemplateUriResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Searches for content in a specified knowledge base. Can be used to get a specific content resource by its name.
*
*
* @param searchContentRequest
* @return Result of the SearchContent operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.SearchContent
* @see AWS API
* Documentation
*/
@Override
public SearchContentResult searchContent(SearchContentRequest request) {
request = beforeClientExecution(request);
return executeSearchContent(request);
}
@SdkInternalApi
final SearchContentResult executeSearchContent(SearchContentRequest searchContentRequest) {
ExecutionContext executionContext = createExecutionContext(searchContentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchContentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchContentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchContent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchContentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Searches existing Amazon Q in Connect quick responses in an Amazon Q in Connect knowledge base.
*
*
* @param searchQuickResponsesRequest
* @return Result of the SearchQuickResponses operation returned by the service.
* @throws RequestTimeoutException
* The request reached the service more than 15 minutes after the date stamp on the request or more than 15
* minutes after the request expiration date (such as for pre-signed URLs), or the date stamp on the request
* is more than 15 minutes in the future.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.SearchQuickResponses
* @see AWS
* API Documentation
*/
@Override
public SearchQuickResponsesResult searchQuickResponses(SearchQuickResponsesRequest request) {
request = beforeClientExecution(request);
return executeSearchQuickResponses(request);
}
@SdkInternalApi
final SearchQuickResponsesResult executeSearchQuickResponses(SearchQuickResponsesRequest searchQuickResponsesRequest) {
ExecutionContext executionContext = createExecutionContext(searchQuickResponsesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchQuickResponsesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchQuickResponsesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchQuickResponses");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchQuickResponsesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Searches for sessions.
*
*
* @param searchSessionsRequest
* @return Result of the SearchSessions operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.SearchSessions
* @see AWS API
* Documentation
*/
@Override
public SearchSessionsResult searchSessions(SearchSessionsRequest request) {
request = beforeClientExecution(request);
return executeSearchSessions(request);
}
@SdkInternalApi
final SearchSessionsResult executeSearchSessions(SearchSessionsRequest searchSessionsRequest) {
ExecutionContext executionContext = createExecutionContext(searchSessionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchSessionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchSessionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchSessions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchSessionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Get a URL to upload content to a knowledge base. To upload content, first make a PUT request to the returned URL
* with your file, making sure to include the required headers. Then use CreateContent
* to finalize the content creation process or UpdateContent
* to modify an existing resource. You can only upload content to a knowledge base of type CUSTOM.
*
*
* @param startContentUploadRequest
* @return Result of the StartContentUpload operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.StartContentUpload
* @see AWS
* API Documentation
*/
@Override
public StartContentUploadResult startContentUpload(StartContentUploadRequest request) {
request = beforeClientExecution(request);
return executeStartContentUpload(request);
}
@SdkInternalApi
final StartContentUploadResult executeStartContentUpload(StartContentUploadRequest startContentUploadRequest) {
ExecutionContext executionContext = createExecutionContext(startContentUploadRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartContentUploadRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startContentUploadRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartContentUpload");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartContentUploadResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Start an asynchronous job to import Amazon Q in Connect resources from an uploaded source file. Before calling
* this API, use StartContentUpload
* to upload an asset that contains the resource data.
*
*
* -
*
* For importing Amazon Q in Connect quick responses, you need to upload a csv file including the quick responses.
* For information about how to format the csv file for importing quick responses, see Import quick responses.
*
*
*
*
* @param startImportJobRequest
* @return Result of the StartImportJob operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws ServiceQuotaExceededException
* You've exceeded your service quota. To perform the requested action, remove some of the relevant
* resources, or use service quotas to request a service quota increase.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.StartImportJob
* @see AWS API
* Documentation
*/
@Override
public StartImportJobResult startImportJob(StartImportJobRequest request) {
request = beforeClientExecution(request);
return executeStartImportJob(request);
}
@SdkInternalApi
final StartImportJobResult executeStartImportJob(StartImportJobRequest startImportJobRequest) {
ExecutionContext executionContext = createExecutionContext(startImportJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartImportJobRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startImportJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartImportJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartImportJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified tags to the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws TooManyTagsException
* Amazon Q in Connect throws this exception if you have too many tags in your tag set.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified tags from the specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates information about the content.
*
*
* @param updateContentRequest
* @return Result of the UpdateContent operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws PreconditionFailedException
* The provided revisionId
does not match, indicating the content has been modified since it
* was last read.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.UpdateContent
* @see AWS API
* Documentation
*/
@Override
public UpdateContentResult updateContent(UpdateContentRequest request) {
request = beforeClientExecution(request);
return executeUpdateContent(request);
}
@SdkInternalApi
final UpdateContentResult executeUpdateContent(UpdateContentRequest updateContentRequest) {
ExecutionContext executionContext = createExecutionContext(updateContentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateContentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateContentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateContent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateContentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the template URI of a knowledge base. This is only supported for knowledge bases of type EXTERNAL.
* Include a single variable in ${variable}
format; this interpolated by Amazon Q in Connect using
* ingested content. For example, if you ingest a Salesforce article, it has an Id
value, and you can
* set the template URI to
* https://myInstanceName.lightning.force.com/lightning/r/Knowledge__kav/*${Id}*/view
.
*
*
* @param updateKnowledgeBaseTemplateUriRequest
* @return Result of the UpdateKnowledgeBaseTemplateUri operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.UpdateKnowledgeBaseTemplateUri
* @see AWS API Documentation
*/
@Override
public UpdateKnowledgeBaseTemplateUriResult updateKnowledgeBaseTemplateUri(UpdateKnowledgeBaseTemplateUriRequest request) {
request = beforeClientExecution(request);
return executeUpdateKnowledgeBaseTemplateUri(request);
}
@SdkInternalApi
final UpdateKnowledgeBaseTemplateUriResult executeUpdateKnowledgeBaseTemplateUri(UpdateKnowledgeBaseTemplateUriRequest updateKnowledgeBaseTemplateUriRequest) {
ExecutionContext executionContext = createExecutionContext(updateKnowledgeBaseTemplateUriRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateKnowledgeBaseTemplateUriRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateKnowledgeBaseTemplateUriRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateKnowledgeBaseTemplateUri");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateKnowledgeBaseTemplateUriResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing Amazon Q in Connect quick response.
*
*
* @param updateQuickResponseRequest
* @return Result of the UpdateQuickResponse operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource. For example,
* if you're using a Create
API (such as CreateAssistant
) that accepts name, a
* conflicting resource (usually with the same name) is being created or mutated.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws PreconditionFailedException
* The provided revisionId
does not match, indicating the content has been modified since it
* was last read.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.UpdateQuickResponse
* @see AWS
* API Documentation
*/
@Override
public UpdateQuickResponseResult updateQuickResponse(UpdateQuickResponseRequest request) {
request = beforeClientExecution(request);
return executeUpdateQuickResponse(request);
}
@SdkInternalApi
final UpdateQuickResponseResult executeUpdateQuickResponse(UpdateQuickResponseRequest updateQuickResponseRequest) {
ExecutionContext executionContext = createExecutionContext(updateQuickResponseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateQuickResponseRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateQuickResponseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateQuickResponse");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateQuickResponseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a session. A session is a contextual container used for generating recommendations. Amazon Connect
* updates the existing Amazon Q in Connect session for each contact on which Amazon Q in Connect is enabled.
*
*
* @param updateSessionRequest
* @return Result of the UpdateSession operation returned by the service.
* @throws ValidationException
* The input fails to satisfy the constraints specified by a service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @sample AmazonQConnect.UpdateSession
* @see AWS API
* Documentation
*/
@Override
public UpdateSessionResult updateSession(UpdateSessionRequest request) {
request = beforeClientExecution(request);
return executeUpdateSession(request);
}
@SdkInternalApi
final UpdateSessionResult executeUpdateSession(UpdateSessionRequest updateSessionRequest) {
ExecutionContext executionContext = createExecutionContext(updateSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "QConnect");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}