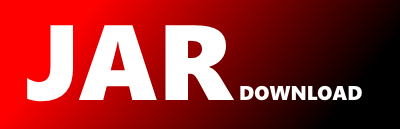
com.amazonaws.services.quicksight.AmazonQuickSightAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight;
import javax.annotation.Generated;
import com.amazonaws.services.quicksight.model.*;
/**
* Interface for accessing Amazon QuickSight asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.quicksight.AbstractAmazonQuickSightAsync} instead.
*
*
* Amazon QuickSight API Reference
*
* Amazon QuickSight is a fully managed, serverless business intelligence service for the AWS Cloud that makes it easy
* to extend data and insights to every user in your organization. This API reference contains documentation for a
* programming interface that you can use to manage Amazon QuickSight.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonQuickSightAsync extends AmazonQuickSight {
/**
*
* Cancels an ongoing ingestion of data into SPICE.
*
*
* @param cancelIngestionRequest
* @return A Java Future containing the result of the CancelIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.CancelIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelIngestionAsync(CancelIngestionRequest cancelIngestionRequest);
/**
*
* Cancels an ongoing ingestion of data into SPICE.
*
*
* @param cancelIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CancelIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelIngestionAsync(CancelIngestionRequest cancelIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dashboard from a template. To first create a template, see the CreateTemplate API.
*
*
* A dashboard is an entity in QuickSight which identifies Quicksight reports, created from analyses. QuickSight
* dashboards are sharable. With the right permissions, you can create scheduled email reports from them. The
* CreateDashboard
, DescribeDashboard
and ListDashboardsByUser
APIs act on
* the dashboard entity. If you have the correct permissions, you can create a dashboard from a template that exists
* in a different AWS account.
*
*
* @param createDashboardRequest
* @return A Java Future containing the result of the CreateDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDashboardAsync(CreateDashboardRequest createDashboardRequest);
/**
*
* Creates a dashboard from a template. To first create a template, see the CreateTemplate API.
*
*
* A dashboard is an entity in QuickSight which identifies Quicksight reports, created from analyses. QuickSight
* dashboards are sharable. With the right permissions, you can create scheduled email reports from them. The
* CreateDashboard
, DescribeDashboard
and ListDashboardsByUser
APIs act on
* the dashboard entity. If you have the correct permissions, you can create a dashboard from a template that exists
* in a different AWS account.
*
*
* @param createDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDashboardAsync(CreateDashboardRequest createDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dataset.
*
*
* @param createDataSetRequest
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest);
/**
*
* Creates a dataset.
*
*
* @param createDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data source.
*
*
* @param createDataSourceRequest
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a data source.
*
*
* @param createDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon QuickSight group.
*
*
* The permissions resource is
* arn:aws:quicksight:us-east-1:<relevant-aws-account-id>:group/default/<group-name>
* .
*
*
* The response is a group object.
*
*
* @param createGroupRequest
* The request object for this operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AmazonQuickSightAsync.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest);
/**
*
* Creates an Amazon QuickSight group.
*
*
* The permissions resource is
* arn:aws:quicksight:us-east-1:<relevant-aws-account-id>:group/default/<group-name>
* .
*
*
* The response is a group object.
*
*
* @param createGroupRequest
* The request object for this operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an Amazon QuickSight user to an Amazon QuickSight group.
*
*
* @param createGroupMembershipRequest
* @return A Java Future containing the result of the CreateGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsync.CreateGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createGroupMembershipAsync(CreateGroupMembershipRequest createGroupMembershipRequest);
/**
*
* Adds an Amazon QuickSight user to an Amazon QuickSight group.
*
*
* @param createGroupMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createGroupMembershipAsync(CreateGroupMembershipRequest createGroupMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an assignment with one specified IAM policy Amazon Resource Name (ARN) and will assigned to specified
* groups or users of QuickSight. Users and groups need to be in the same namespace.
*
*
* @param createIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the CreateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.CreateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future createIAMPolicyAssignmentAsync(
CreateIAMPolicyAssignmentRequest createIAMPolicyAssignmentRequest);
/**
*
* Creates an assignment with one specified IAM policy Amazon Resource Name (ARN) and will assigned to specified
* groups or users of QuickSight. Users and groups need to be in the same namespace.
*
*
* @param createIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future createIAMPolicyAssignmentAsync(
CreateIAMPolicyAssignmentRequest createIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and starts a new SPICE ingestion on a dataset
*
*
* Any ingestions operating on tagged datasets inherit the same tags automatically for use in access control. For an
* example, see How do I
* create an IAM policy to control access to Amazon EC2 resources using tags? in the AWS Knowledge Center. Tags
* are visible on the tagged dataset, but not on the ingestion resource.
*
*
* @param createIngestionRequest
* @return A Java Future containing the result of the CreateIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.CreateIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIngestionAsync(CreateIngestionRequest createIngestionRequest);
/**
*
* Creates and starts a new SPICE ingestion on a dataset
*
*
* Any ingestions operating on tagged datasets inherit the same tags automatically for use in access control. For an
* example, see How do I
* create an IAM policy to control access to Amazon EC2 resources using tags? in the AWS Knowledge Center. Tags
* are visible on the tagged dataset, but not on the ingestion resource.
*
*
* @param createIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIngestionAsync(CreateIngestionRequest createIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a template from an existing QuickSight analysis or template. The resulting template can be used to create
* a dashboard.
*
*
* A template is an entity in QuickSight which encapsulates the metadata required to create an analysis that can be
* used to create dashboard. It adds a layer of abstraction by use placeholders to replace the dataset associated
* with the analysis. You can use templates to create dashboards by replacing dataset placeholders with datasets
* which follow the same schema that was used to create the source analysis and template.
*
*
* @param createTemplateRequest
* @return A Java Future containing the result of the CreateTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTemplateAsync(CreateTemplateRequest createTemplateRequest);
/**
*
* Creates a template from an existing QuickSight analysis or template. The resulting template can be used to create
* a dashboard.
*
*
* A template is an entity in QuickSight which encapsulates the metadata required to create an analysis that can be
* used to create dashboard. It adds a layer of abstraction by use placeholders to replace the dataset associated
* with the analysis. You can use templates to create dashboards by replacing dataset placeholders with datasets
* which follow the same schema that was used to create the source analysis and template.
*
*
* @param createTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTemplateAsync(CreateTemplateRequest createTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a template alias for a template.
*
*
* @param createTemplateAliasRequest
* @return A Java Future containing the result of the CreateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTemplateAliasAsync(CreateTemplateAliasRequest createTemplateAliasRequest);
/**
*
* Creates a template alias for a template.
*
*
* @param createTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTemplateAliasAsync(CreateTemplateAliasRequest createTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dashboard.
*
*
* @param deleteDashboardRequest
* @return A Java Future containing the result of the DeleteDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDashboardAsync(DeleteDashboardRequest deleteDashboardRequest);
/**
*
* Deletes a dashboard.
*
*
* @param deleteDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDashboardAsync(DeleteDashboardRequest deleteDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dataset.
*
*
* @param deleteDataSetRequest
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest);
/**
*
* Deletes a dataset.
*
*
* @param deleteDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the data source permanently. This action breaks all the datasets that reference the deleted data source.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes the data source permanently. This action breaks all the datasets that reference the deleted data source.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a user group from Amazon QuickSight.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest);
/**
*
* Removes a user group from Amazon QuickSight.
*
*
* @param deleteGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a user from a group so that the user is no longer a member of the group.
*
*
* @param deleteGroupMembershipRequest
* @return A Java Future containing the result of the DeleteGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGroupMembershipAsync(DeleteGroupMembershipRequest deleteGroupMembershipRequest);
/**
*
* Removes a user from a group so that the user is no longer a member of the group.
*
*
* @param deleteGroupMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGroupMembershipAsync(DeleteGroupMembershipRequest deleteGroupMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing assignment.
*
*
* @param deleteIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the DeleteIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIAMPolicyAssignmentAsync(
DeleteIAMPolicyAssignmentRequest deleteIAMPolicyAssignmentRequest);
/**
*
* Deletes an existing assignment.
*
*
* @param deleteIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIAMPolicyAssignmentAsync(
DeleteIAMPolicyAssignmentRequest deleteIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a template.
*
*
* @param deleteTemplateRequest
* @return A Java Future containing the result of the DeleteTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTemplateAsync(DeleteTemplateRequest deleteTemplateRequest);
/**
*
* Deletes a template.
*
*
* @param deleteTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTemplateAsync(DeleteTemplateRequest deleteTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update template alias of given template.
*
*
* @param deleteTemplateAliasRequest
* @return A Java Future containing the result of the DeleteTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTemplateAliasAsync(DeleteTemplateAliasRequest deleteTemplateAliasRequest);
/**
*
* Update template alias of given template.
*
*
* @param deleteTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTemplateAliasAsync(DeleteTemplateAliasRequest deleteTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the Amazon QuickSight user that is associated with the identity of the AWS Identity and Access Management
* (IAM) user or role that's making the call. The IAM user isn't deleted as a result of this call.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest);
/**
*
* Deletes the Amazon QuickSight user that is associated with the identity of the AWS Identity and Access Management
* (IAM) user or role that's making the call. The IAM user isn't deleted as a result of this call.
*
*
* @param deleteUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a user identified by its principal ID.
*
*
* @param deleteUserByPrincipalIdRequest
* @return A Java Future containing the result of the DeleteUserByPrincipalId operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteUserByPrincipalId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserByPrincipalIdAsync(DeleteUserByPrincipalIdRequest deleteUserByPrincipalIdRequest);
/**
*
* Deletes a user identified by its principal ID.
*
*
* @param deleteUserByPrincipalIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUserByPrincipalId operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteUserByPrincipalId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserByPrincipalIdAsync(DeleteUserByPrincipalIdRequest deleteUserByPrincipalIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a summary for a dashboard.
*
*
* @param describeDashboardRequest
* @return A Java Future containing the result of the DescribeDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDashboard
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDashboardAsync(DescribeDashboardRequest describeDashboardRequest);
/**
*
* Provides a summary for a dashboard.
*
*
* @param describeDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboard
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDashboardAsync(DescribeDashboardRequest describeDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes read and write permissions on a dashboard.
*
*
* @param describeDashboardPermissionsRequest
* @return A Java Future containing the result of the DescribeDashboardPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardPermissionsAsync(
DescribeDashboardPermissionsRequest describeDashboardPermissionsRequest);
/**
*
* Describes read and write permissions on a dashboard.
*
*
* @param describeDashboardPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboardPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardPermissionsAsync(
DescribeDashboardPermissionsRequest describeDashboardPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a dataset.
*
*
* @param describeDataSetRequest
* @return A Java Future containing the result of the DescribeDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSetAsync(DescribeDataSetRequest describeDataSetRequest);
/**
*
* Describes a dataset.
*
*
* @param describeDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSetAsync(DescribeDataSetRequest describeDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param describeDataSetPermissionsRequest
* @return A Java Future containing the result of the DescribeDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetPermissionsAsync(
DescribeDataSetPermissionsRequest describeDataSetPermissionsRequest);
/**
*
* Describes the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param describeDataSetPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetPermissionsAsync(
DescribeDataSetPermissionsRequest describeDataSetPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a data source.
*
*
* @param describeDataSourceRequest
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest);
/**
*
* Describes a data source.
*
*
* @param describeDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the resource permissions for a data source.
*
*
* @param describeDataSourcePermissionsRequest
* @return A Java Future containing the result of the DescribeDataSourcePermissions operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSourcePermissionsAsync(
DescribeDataSourcePermissionsRequest describeDataSourcePermissionsRequest);
/**
*
* Describes the resource permissions for a data source.
*
*
* @param describeDataSourcePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSourcePermissions operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSourcePermissionsAsync(
DescribeDataSourcePermissionsRequest describeDataSourcePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an Amazon QuickSight group's description and Amazon Resource Name (ARN).
*
*
* @param describeGroupRequest
* @return A Java Future containing the result of the DescribeGroup operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeGroupAsync(DescribeGroupRequest describeGroupRequest);
/**
*
* Returns an Amazon QuickSight group's description and Amazon Resource Name (ARN).
*
*
* @param describeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeGroupAsync(DescribeGroupRequest describeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing IAMPolicy Assignment by specified assignment name.
*
*
* @param describeIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the DescribeIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIAMPolicyAssignmentAsync(
DescribeIAMPolicyAssignmentRequest describeIAMPolicyAssignmentRequest);
/**
*
* Describes an existing IAMPolicy Assignment by specified assignment name.
*
*
* @param describeIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIAMPolicyAssignmentAsync(
DescribeIAMPolicyAssignmentRequest describeIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a SPICE ingestion.
*
*
* @param describeIngestionRequest
* @return A Java Future containing the result of the DescribeIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeIngestion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIngestionAsync(DescribeIngestionRequest describeIngestionRequest);
/**
*
* Describes a SPICE ingestion.
*
*
* @param describeIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeIngestion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIngestionAsync(DescribeIngestionRequest describeIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a template's metadata.
*
*
* @param describeTemplateRequest
* @return A Java Future containing the result of the DescribeTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTemplateAsync(DescribeTemplateRequest describeTemplateRequest);
/**
*
* Describes a template's metadata.
*
*
* @param describeTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTemplateAsync(DescribeTemplateRequest describeTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the template aliases of a template.
*
*
* @param describeTemplateAliasRequest
* @return A Java Future containing the result of the DescribeTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeTemplateAlias
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplateAliasAsync(DescribeTemplateAliasRequest describeTemplateAliasRequest);
/**
*
* Describes the template aliases of a template.
*
*
* @param describeTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeTemplateAlias
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplateAliasAsync(DescribeTemplateAliasRequest describeTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes read and write permissions on a template.
*
*
* @param describeTemplatePermissionsRequest
* @return A Java Future containing the result of the DescribeTemplatePermissions operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeTemplatePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplatePermissionsAsync(
DescribeTemplatePermissionsRequest describeTemplatePermissionsRequest);
/**
*
* Describes read and write permissions on a template.
*
*
* @param describeTemplatePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTemplatePermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeTemplatePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplatePermissionsAsync(
DescribeTemplatePermissionsRequest describeTemplatePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a user, given the user name.
*
*
* @param describeUserRequest
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUserAsync(DescribeUserRequest describeUserRequest);
/**
*
* Returns information about a user, given the user name.
*
*
* @param describeUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUser operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeUserAsync(DescribeUserRequest describeUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Generates a server-side embeddable URL and authorization code. Before this can work properly, first you need to
* configure the dashboards and user permissions. For more information, see the Amazon QuickSight User Guide section
* on Embedding Amazon
* QuickSight Dashboards or see the Amazon QuickSight API Reference section on Embedding
* Amazon QuickSight Dashboards .
*
*
* Currently, you can use GetDashboardEmbedURL
only from the server, not from the user’s browser.
*
*
* @param getDashboardEmbedUrlRequest
* @return A Java Future containing the result of the GetDashboardEmbedUrl operation returned by the service.
* @sample AmazonQuickSightAsync.GetDashboardEmbedUrl
* @see AWS API Documentation
*/
java.util.concurrent.Future getDashboardEmbedUrlAsync(GetDashboardEmbedUrlRequest getDashboardEmbedUrlRequest);
/**
*
* Generates a server-side embeddable URL and authorization code. Before this can work properly, first you need to
* configure the dashboards and user permissions. For more information, see the Amazon QuickSight User Guide section
* on Embedding Amazon
* QuickSight Dashboards or see the Amazon QuickSight API Reference section on Embedding
* Amazon QuickSight Dashboards .
*
*
* Currently, you can use GetDashboardEmbedURL
only from the server, not from the user’s browser.
*
*
* @param getDashboardEmbedUrlRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDashboardEmbedUrl operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.GetDashboardEmbedUrl
* @see AWS API Documentation
*/
java.util.concurrent.Future getDashboardEmbedUrlAsync(GetDashboardEmbedUrlRequest getDashboardEmbedUrlRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the versions of the dashboards in the Quicksight subscription.
*
*
* @param listDashboardVersionsRequest
* @return A Java Future containing the result of the ListDashboardVersions operation returned by the service.
* @sample AmazonQuickSightAsync.ListDashboardVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDashboardVersionsAsync(ListDashboardVersionsRequest listDashboardVersionsRequest);
/**
*
* Lists all the versions of the dashboards in the Quicksight subscription.
*
*
* @param listDashboardVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDashboardVersions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListDashboardVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listDashboardVersionsAsync(ListDashboardVersionsRequest listDashboardVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists dashboards in the AWS account.
*
*
* @param listDashboardsRequest
* @return A Java Future containing the result of the ListDashboards operation returned by the service.
* @sample AmazonQuickSightAsync.ListDashboards
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDashboardsAsync(ListDashboardsRequest listDashboardsRequest);
/**
*
* Lists dashboards in the AWS account.
*
*
* @param listDashboardsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDashboards operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListDashboards
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDashboardsAsync(ListDashboardsRequest listDashboardsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the datasets belonging to this account in an AWS region.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/*
.
*
*
* @param listDataSetsRequest
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* @sample AmazonQuickSightAsync.ListDataSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSetsAsync(ListDataSetsRequest listDataSetsRequest);
/**
*
* Lists all of the datasets belonging to this account in an AWS region.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/*
.
*
*
* @param listDataSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSets operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListDataSets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSetsAsync(ListDataSetsRequest listDataSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists data sources in current AWS Region that belong to this AWS account.
*
*
* @param listDataSourcesRequest
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AmazonQuickSightAsync.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* Lists data sources in current AWS Region that belong to this AWS account.
*
*
* @param listDataSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDataSources operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListDataSources
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDataSourcesAsync(ListDataSourcesRequest listDataSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists member users in a group.
*
*
* @param listGroupMembershipsRequest
* @return A Java Future containing the result of the ListGroupMemberships operation returned by the service.
* @sample AmazonQuickSightAsync.ListGroupMemberships
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupMembershipsAsync(ListGroupMembershipsRequest listGroupMembershipsRequest);
/**
*
* Lists member users in a group.
*
*
* @param listGroupMembershipsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroupMemberships operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListGroupMemberships
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupMembershipsAsync(ListGroupMembershipsRequest listGroupMembershipsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all user groups in Amazon QuickSight.
*
*
* @param listGroupsRequest
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* @sample AmazonQuickSightAsync.ListGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGroupsAsync(ListGroupsRequest listGroupsRequest);
/**
*
* Lists all user groups in Amazon QuickSight.
*
*
* @param listGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGroupsAsync(ListGroupsRequest listGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists assignments in current QuickSight account.
*
*
* @param listIAMPolicyAssignmentsRequest
* @return A Java Future containing the result of the ListIAMPolicyAssignments operation returned by the service.
* @sample AmazonQuickSightAsync.ListIAMPolicyAssignments
* @see AWS API Documentation
*/
java.util.concurrent.Future listIAMPolicyAssignmentsAsync(ListIAMPolicyAssignmentsRequest listIAMPolicyAssignmentsRequest);
/**
*
* Lists assignments in current QuickSight account.
*
*
* @param listIAMPolicyAssignmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIAMPolicyAssignments operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListIAMPolicyAssignments
* @see AWS API Documentation
*/
java.util.concurrent.Future listIAMPolicyAssignmentsAsync(ListIAMPolicyAssignmentsRequest listIAMPolicyAssignmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the assignments and the Amazon Resource Names (ARNs) for the associated IAM policies assigned to the
* specified user and the group or groups that the user belongs to.
*
*
* @param listIAMPolicyAssignmentsForUserRequest
* @return A Java Future containing the result of the ListIAMPolicyAssignmentsForUser operation returned by the
* service.
* @sample AmazonQuickSightAsync.ListIAMPolicyAssignmentsForUser
* @see AWS API Documentation
*/
java.util.concurrent.Future listIAMPolicyAssignmentsForUserAsync(
ListIAMPolicyAssignmentsForUserRequest listIAMPolicyAssignmentsForUserRequest);
/**
*
* Lists all the assignments and the Amazon Resource Names (ARNs) for the associated IAM policies assigned to the
* specified user and the group or groups that the user belongs to.
*
*
* @param listIAMPolicyAssignmentsForUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIAMPolicyAssignmentsForUser operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.ListIAMPolicyAssignmentsForUser
* @see AWS API Documentation
*/
java.util.concurrent.Future listIAMPolicyAssignmentsForUserAsync(
ListIAMPolicyAssignmentsForUserRequest listIAMPolicyAssignmentsForUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the history of SPICE ingestions for a dataset.
*
*
* @param listIngestionsRequest
* @return A Java Future containing the result of the ListIngestions operation returned by the service.
* @sample AmazonQuickSightAsync.ListIngestions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIngestionsAsync(ListIngestionsRequest listIngestionsRequest);
/**
*
* Lists the history of SPICE ingestions for a dataset.
*
*
* @param listIngestionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListIngestions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListIngestions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listIngestionsAsync(ListIngestionsRequest listIngestionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags assigned to a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonQuickSightAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags assigned to a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the aliases of a template.
*
*
* @param listTemplateAliasesRequest
* @return A Java Future containing the result of the ListTemplateAliases operation returned by the service.
* @sample AmazonQuickSightAsync.ListTemplateAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTemplateAliasesAsync(ListTemplateAliasesRequest listTemplateAliasesRequest);
/**
*
* Lists all the aliases of a template.
*
*
* @param listTemplateAliasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTemplateAliases operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListTemplateAliases
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTemplateAliasesAsync(ListTemplateAliasesRequest listTemplateAliasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the versions of the templates in the Quicksight account.
*
*
* @param listTemplateVersionsRequest
* @return A Java Future containing the result of the ListTemplateVersions operation returned by the service.
* @sample AmazonQuickSightAsync.ListTemplateVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTemplateVersionsAsync(ListTemplateVersionsRequest listTemplateVersionsRequest);
/**
*
* Lists all the versions of the templates in the Quicksight account.
*
*
* @param listTemplateVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTemplateVersions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListTemplateVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listTemplateVersionsAsync(ListTemplateVersionsRequest listTemplateVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the templates in the QuickSight account.
*
*
* @param listTemplatesRequest
* @return A Java Future containing the result of the ListTemplates operation returned by the service.
* @sample AmazonQuickSightAsync.ListTemplates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTemplatesAsync(ListTemplatesRequest listTemplatesRequest);
/**
*
* Lists all the templates in the QuickSight account.
*
*
* @param listTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTemplates operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListTemplates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTemplatesAsync(ListTemplatesRequest listTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon QuickSight groups that an Amazon QuickSight user is a member of.
*
*
* @param listUserGroupsRequest
* @return A Java Future containing the result of the ListUserGroups operation returned by the service.
* @sample AmazonQuickSightAsync.ListUserGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUserGroupsAsync(ListUserGroupsRequest listUserGroupsRequest);
/**
*
* Lists the Amazon QuickSight groups that an Amazon QuickSight user is a member of.
*
*
* @param listUserGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUserGroups operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListUserGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUserGroupsAsync(ListUserGroupsRequest listUserGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all of the Amazon QuickSight users belonging to this account.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* @sample AmazonQuickSightAsync.ListUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUsersAsync(ListUsersRequest listUsersRequest);
/**
*
* Returns a list of all of the Amazon QuickSight users belonging to this account.
*
*
* @param listUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.ListUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUsersAsync(ListUsersRequest listUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon QuickSight user, whose identity is associated with the AWS Identity and Access Management (IAM)
* identity or role specified in the request.
*
*
* @param registerUserRequest
* @return A Java Future containing the result of the RegisterUser operation returned by the service.
* @sample AmazonQuickSightAsync.RegisterUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerUserAsync(RegisterUserRequest registerUserRequest);
/**
*
* Creates an Amazon QuickSight user, whose identity is associated with the AWS Identity and Access Management (IAM)
* identity or role specified in the request.
*
*
* @param registerUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterUser operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.RegisterUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future registerUserAsync(RegisterUserRequest registerUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more tags (key-value pairs) to the specified QuickSight resource.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only resources with certain tag values. You can use the
* TagResource
operation with a resource that already has tags. If you specify a new tag key for the
* resource, this tag is appended to the list of tags associated with the resource. If you specify a tag key that is
* already associated with the resource, the new tag value that you specify replaces the previous value for that
* tag.
*
*
* You can associate as many as 50 tags with a resource. QuickSight supports tagging on data set, data source,
* dashboard, and template.
*
*
* Tagging for QuickSight works in a similar way to tagging for other AWS services, except for the following:
*
*
* -
*
* You can't use tags to track AWS costs for QuickSight. This restriction is because QuickSight costs are based on
* users and SPICE capacity, which aren't taggable resources.
*
*
* -
*
* QuickSight doesn't currently support the Tag Editor for AWS Resource Groups.
*
*
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonQuickSightAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified QuickSight resource.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only resources with certain tag values. You can use the
* TagResource
operation with a resource that already has tags. If you specify a new tag key for the
* resource, this tag is appended to the list of tags associated with the resource. If you specify a tag key that is
* already associated with the resource, the new tag value that you specify replaces the previous value for that
* tag.
*
*
* You can associate as many as 50 tags with a resource. QuickSight supports tagging on data set, data source,
* dashboard, and template.
*
*
* Tagging for QuickSight works in a similar way to tagging for other AWS services, except for the following:
*
*
* -
*
* You can't use tags to track AWS costs for QuickSight. This restriction is because QuickSight costs are based on
* users and SPICE capacity, which aren't taggable resources.
*
*
* -
*
* QuickSight doesn't currently support the Tag Editor for AWS Resource Groups.
*
*
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a tag or tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonQuickSightAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes a tag or tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a dashboard in the AWS account.
*
*
* @param updateDashboardRequest
* @return A Java Future containing the result of the UpdateDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDashboardAsync(UpdateDashboardRequest updateDashboardRequest);
/**
*
* Updates a dashboard in the AWS account.
*
*
* @param updateDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDashboardAsync(UpdateDashboardRequest updateDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates read and write permissions on a dashboard.
*
*
* @param updateDashboardPermissionsRequest
* @return A Java Future containing the result of the UpdateDashboardPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDashboardPermissionsAsync(
UpdateDashboardPermissionsRequest updateDashboardPermissionsRequest);
/**
*
* Updates read and write permissions on a dashboard.
*
*
* @param updateDashboardPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDashboardPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDashboardPermissionsAsync(
UpdateDashboardPermissionsRequest updateDashboardPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the published version of a dashboard.
*
*
* @param updateDashboardPublishedVersionRequest
* @return A Java Future containing the result of the UpdateDashboardPublishedVersion operation returned by the
* service.
* @sample AmazonQuickSightAsync.UpdateDashboardPublishedVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDashboardPublishedVersionAsync(
UpdateDashboardPublishedVersionRequest updateDashboardPublishedVersionRequest);
/**
*
* Updates the published version of a dashboard.
*
*
* @param updateDashboardPublishedVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDashboardPublishedVersion operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.UpdateDashboardPublishedVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDashboardPublishedVersionAsync(
UpdateDashboardPublishedVersionRequest updateDashboardPublishedVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a dataset.
*
*
* @param updateDataSetRequest
* @return A Java Future containing the result of the UpdateDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSetAsync(UpdateDataSetRequest updateDataSetRequest);
/**
*
* Updates a dataset.
*
*
* @param updateDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDataSetAsync(UpdateDataSetRequest updateDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param updateDataSetPermissionsRequest
* @return A Java Future containing the result of the UpdateDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataSetPermissionsAsync(UpdateDataSetPermissionsRequest updateDataSetPermissionsRequest);
/**
*
* Updates the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param updateDataSetPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataSetPermissionsAsync(UpdateDataSetPermissionsRequest updateDataSetPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a data source.
*
*
* @param updateDataSourceRequest
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates a data source.
*
*
* @param updateDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateDataSourceAsync(UpdateDataSourceRequest updateDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the permissions to a data source.
*
*
* @param updateDataSourcePermissionsRequest
* @return A Java Future containing the result of the UpdateDataSourcePermissions operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataSourcePermissionsAsync(
UpdateDataSourcePermissionsRequest updateDataSourcePermissionsRequest);
/**
*
* Updates the permissions to a data source.
*
*
* @param updateDataSourcePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDataSourcePermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateDataSourcePermissionsAsync(
UpdateDataSourcePermissionsRequest updateDataSourcePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes a group description.
*
*
* @param updateGroupRequest
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest);
/**
*
* Changes a group description.
*
*
* @param updateGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing assignment. This operation updates only the optional parameter or parameters that are
* specified in the request.
*
*
* @param updateIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the UpdateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIAMPolicyAssignmentAsync(
UpdateIAMPolicyAssignmentRequest updateIAMPolicyAssignmentRequest);
/**
*
* Updates an existing assignment. This operation updates only the optional parameter or parameters that are
* specified in the request.
*
*
* @param updateIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future updateIAMPolicyAssignmentAsync(
UpdateIAMPolicyAssignmentRequest updateIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a template from an existing QuickSight analysis.
*
*
* @param updateTemplateRequest
* @return A Java Future containing the result of the UpdateTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTemplateAsync(UpdateTemplateRequest updateTemplateRequest);
/**
*
* Updates a template from an existing QuickSight analysis.
*
*
* @param updateTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateTemplateAsync(UpdateTemplateRequest updateTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the template alias of a template.
*
*
* @param updateTemplateAliasRequest
* @return A Java Future containing the result of the UpdateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateTemplateAliasAsync(UpdateTemplateAliasRequest updateTemplateAliasRequest);
/**
*
* Updates the template alias of a template.
*
*
* @param updateTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateTemplateAliasAsync(UpdateTemplateAliasRequest updateTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the permissions on a template.
*
*
* @param updateTemplatePermissionsRequest
* @return A Java Future containing the result of the UpdateTemplatePermissions operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateTemplatePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTemplatePermissionsAsync(
UpdateTemplatePermissionsRequest updateTemplatePermissionsRequest);
/**
*
* Updates the permissions on a template.
*
*
* @param updateTemplatePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTemplatePermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateTemplatePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTemplatePermissionsAsync(
UpdateTemplatePermissionsRequest updateTemplatePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an Amazon QuickSight user.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonQuickSightAsync.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest);
/**
*
* Updates an Amazon QuickSight user.
*
*
* @param updateUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}