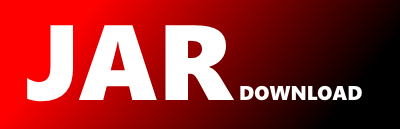
com.amazonaws.services.quicksight.model.Ingestion Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information on the SPICE ingestion for a dataset.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Ingestion implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource name (ARN) of the resource.
*
*/
private String arn;
/**
*
* Ingestion ID.
*
*/
private String ingestionId;
/**
*
* Ingestion status.
*
*/
private String ingestionStatus;
/**
*
* Error information for this ingestion.
*
*/
private ErrorInfo errorInfo;
private RowInfo rowInfo;
private QueueInfo queueInfo;
/**
*
* The time this ingestion started.
*
*/
private java.util.Date createdTime;
/**
*
* The time this ingestion took, measured in seconds.
*
*/
private Long ingestionTimeInSeconds;
/**
*
* Size of the data ingested in bytes.
*
*/
private Long ingestionSizeInBytes;
/**
*
* Event source for this ingestion.
*
*/
private String requestSource;
/**
*
* Type of this ingestion.
*
*/
private String requestType;
/**
*
* The Amazon Resource name (ARN) of the resource.
*
*
* @param arn
* The Amazon Resource name (ARN) of the resource.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource name (ARN) of the resource.
*
*
* @return The Amazon Resource name (ARN) of the resource.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource name (ARN) of the resource.
*
*
* @param arn
* The Amazon Resource name (ARN) of the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* Ingestion ID.
*
*
* @param ingestionId
* Ingestion ID.
*/
public void setIngestionId(String ingestionId) {
this.ingestionId = ingestionId;
}
/**
*
* Ingestion ID.
*
*
* @return Ingestion ID.
*/
public String getIngestionId() {
return this.ingestionId;
}
/**
*
* Ingestion ID.
*
*
* @param ingestionId
* Ingestion ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withIngestionId(String ingestionId) {
setIngestionId(ingestionId);
return this;
}
/**
*
* Ingestion status.
*
*
* @param ingestionStatus
* Ingestion status.
* @see IngestionStatus
*/
public void setIngestionStatus(String ingestionStatus) {
this.ingestionStatus = ingestionStatus;
}
/**
*
* Ingestion status.
*
*
* @return Ingestion status.
* @see IngestionStatus
*/
public String getIngestionStatus() {
return this.ingestionStatus;
}
/**
*
* Ingestion status.
*
*
* @param ingestionStatus
* Ingestion status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionStatus
*/
public Ingestion withIngestionStatus(String ingestionStatus) {
setIngestionStatus(ingestionStatus);
return this;
}
/**
*
* Ingestion status.
*
*
* @param ingestionStatus
* Ingestion status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionStatus
*/
public Ingestion withIngestionStatus(IngestionStatus ingestionStatus) {
this.ingestionStatus = ingestionStatus.toString();
return this;
}
/**
*
* Error information for this ingestion.
*
*
* @param errorInfo
* Error information for this ingestion.
*/
public void setErrorInfo(ErrorInfo errorInfo) {
this.errorInfo = errorInfo;
}
/**
*
* Error information for this ingestion.
*
*
* @return Error information for this ingestion.
*/
public ErrorInfo getErrorInfo() {
return this.errorInfo;
}
/**
*
* Error information for this ingestion.
*
*
* @param errorInfo
* Error information for this ingestion.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withErrorInfo(ErrorInfo errorInfo) {
setErrorInfo(errorInfo);
return this;
}
/**
* @param rowInfo
*/
public void setRowInfo(RowInfo rowInfo) {
this.rowInfo = rowInfo;
}
/**
* @return
*/
public RowInfo getRowInfo() {
return this.rowInfo;
}
/**
* @param rowInfo
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withRowInfo(RowInfo rowInfo) {
setRowInfo(rowInfo);
return this;
}
/**
* @param queueInfo
*/
public void setQueueInfo(QueueInfo queueInfo) {
this.queueInfo = queueInfo;
}
/**
* @return
*/
public QueueInfo getQueueInfo() {
return this.queueInfo;
}
/**
* @param queueInfo
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withQueueInfo(QueueInfo queueInfo) {
setQueueInfo(queueInfo);
return this;
}
/**
*
* The time this ingestion started.
*
*
* @param createdTime
* The time this ingestion started.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time this ingestion started.
*
*
* @return The time this ingestion started.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time this ingestion started.
*
*
* @param createdTime
* The time this ingestion started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The time this ingestion took, measured in seconds.
*
*
* @param ingestionTimeInSeconds
* The time this ingestion took, measured in seconds.
*/
public void setIngestionTimeInSeconds(Long ingestionTimeInSeconds) {
this.ingestionTimeInSeconds = ingestionTimeInSeconds;
}
/**
*
* The time this ingestion took, measured in seconds.
*
*
* @return The time this ingestion took, measured in seconds.
*/
public Long getIngestionTimeInSeconds() {
return this.ingestionTimeInSeconds;
}
/**
*
* The time this ingestion took, measured in seconds.
*
*
* @param ingestionTimeInSeconds
* The time this ingestion took, measured in seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withIngestionTimeInSeconds(Long ingestionTimeInSeconds) {
setIngestionTimeInSeconds(ingestionTimeInSeconds);
return this;
}
/**
*
* Size of the data ingested in bytes.
*
*
* @param ingestionSizeInBytes
* Size of the data ingested in bytes.
*/
public void setIngestionSizeInBytes(Long ingestionSizeInBytes) {
this.ingestionSizeInBytes = ingestionSizeInBytes;
}
/**
*
* Size of the data ingested in bytes.
*
*
* @return Size of the data ingested in bytes.
*/
public Long getIngestionSizeInBytes() {
return this.ingestionSizeInBytes;
}
/**
*
* Size of the data ingested in bytes.
*
*
* @param ingestionSizeInBytes
* Size of the data ingested in bytes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Ingestion withIngestionSizeInBytes(Long ingestionSizeInBytes) {
setIngestionSizeInBytes(ingestionSizeInBytes);
return this;
}
/**
*
* Event source for this ingestion.
*
*
* @param requestSource
* Event source for this ingestion.
* @see IngestionRequestSource
*/
public void setRequestSource(String requestSource) {
this.requestSource = requestSource;
}
/**
*
* Event source for this ingestion.
*
*
* @return Event source for this ingestion.
* @see IngestionRequestSource
*/
public String getRequestSource() {
return this.requestSource;
}
/**
*
* Event source for this ingestion.
*
*
* @param requestSource
* Event source for this ingestion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionRequestSource
*/
public Ingestion withRequestSource(String requestSource) {
setRequestSource(requestSource);
return this;
}
/**
*
* Event source for this ingestion.
*
*
* @param requestSource
* Event source for this ingestion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionRequestSource
*/
public Ingestion withRequestSource(IngestionRequestSource requestSource) {
this.requestSource = requestSource.toString();
return this;
}
/**
*
* Type of this ingestion.
*
*
* @param requestType
* Type of this ingestion.
* @see IngestionRequestType
*/
public void setRequestType(String requestType) {
this.requestType = requestType;
}
/**
*
* Type of this ingestion.
*
*
* @return Type of this ingestion.
* @see IngestionRequestType
*/
public String getRequestType() {
return this.requestType;
}
/**
*
* Type of this ingestion.
*
*
* @param requestType
* Type of this ingestion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionRequestType
*/
public Ingestion withRequestType(String requestType) {
setRequestType(requestType);
return this;
}
/**
*
* Type of this ingestion.
*
*
* @param requestType
* Type of this ingestion.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IngestionRequestType
*/
public Ingestion withRequestType(IngestionRequestType requestType) {
this.requestType = requestType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getIngestionId() != null)
sb.append("IngestionId: ").append(getIngestionId()).append(",");
if (getIngestionStatus() != null)
sb.append("IngestionStatus: ").append(getIngestionStatus()).append(",");
if (getErrorInfo() != null)
sb.append("ErrorInfo: ").append(getErrorInfo()).append(",");
if (getRowInfo() != null)
sb.append("RowInfo: ").append(getRowInfo()).append(",");
if (getQueueInfo() != null)
sb.append("QueueInfo: ").append(getQueueInfo()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getIngestionTimeInSeconds() != null)
sb.append("IngestionTimeInSeconds: ").append(getIngestionTimeInSeconds()).append(",");
if (getIngestionSizeInBytes() != null)
sb.append("IngestionSizeInBytes: ").append(getIngestionSizeInBytes()).append(",");
if (getRequestSource() != null)
sb.append("RequestSource: ").append(getRequestSource()).append(",");
if (getRequestType() != null)
sb.append("RequestType: ").append(getRequestType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Ingestion == false)
return false;
Ingestion other = (Ingestion) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getIngestionId() == null ^ this.getIngestionId() == null)
return false;
if (other.getIngestionId() != null && other.getIngestionId().equals(this.getIngestionId()) == false)
return false;
if (other.getIngestionStatus() == null ^ this.getIngestionStatus() == null)
return false;
if (other.getIngestionStatus() != null && other.getIngestionStatus().equals(this.getIngestionStatus()) == false)
return false;
if (other.getErrorInfo() == null ^ this.getErrorInfo() == null)
return false;
if (other.getErrorInfo() != null && other.getErrorInfo().equals(this.getErrorInfo()) == false)
return false;
if (other.getRowInfo() == null ^ this.getRowInfo() == null)
return false;
if (other.getRowInfo() != null && other.getRowInfo().equals(this.getRowInfo()) == false)
return false;
if (other.getQueueInfo() == null ^ this.getQueueInfo() == null)
return false;
if (other.getQueueInfo() != null && other.getQueueInfo().equals(this.getQueueInfo()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getIngestionTimeInSeconds() == null ^ this.getIngestionTimeInSeconds() == null)
return false;
if (other.getIngestionTimeInSeconds() != null && other.getIngestionTimeInSeconds().equals(this.getIngestionTimeInSeconds()) == false)
return false;
if (other.getIngestionSizeInBytes() == null ^ this.getIngestionSizeInBytes() == null)
return false;
if (other.getIngestionSizeInBytes() != null && other.getIngestionSizeInBytes().equals(this.getIngestionSizeInBytes()) == false)
return false;
if (other.getRequestSource() == null ^ this.getRequestSource() == null)
return false;
if (other.getRequestSource() != null && other.getRequestSource().equals(this.getRequestSource()) == false)
return false;
if (other.getRequestType() == null ^ this.getRequestType() == null)
return false;
if (other.getRequestType() != null && other.getRequestType().equals(this.getRequestType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getIngestionId() == null) ? 0 : getIngestionId().hashCode());
hashCode = prime * hashCode + ((getIngestionStatus() == null) ? 0 : getIngestionStatus().hashCode());
hashCode = prime * hashCode + ((getErrorInfo() == null) ? 0 : getErrorInfo().hashCode());
hashCode = prime * hashCode + ((getRowInfo() == null) ? 0 : getRowInfo().hashCode());
hashCode = prime * hashCode + ((getQueueInfo() == null) ? 0 : getQueueInfo().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getIngestionTimeInSeconds() == null) ? 0 : getIngestionTimeInSeconds().hashCode());
hashCode = prime * hashCode + ((getIngestionSizeInBytes() == null) ? 0 : getIngestionSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getRequestSource() == null) ? 0 : getRequestSource().hashCode());
hashCode = prime * hashCode + ((getRequestType() == null) ? 0 : getRequestType().hashCode());
return hashCode;
}
@Override
public Ingestion clone() {
try {
return (Ingestion) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.IngestionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}