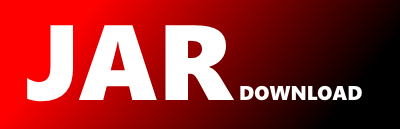
com.amazonaws.services.quicksight.AmazonQuickSight Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.quicksight.model.*;
/**
* Interface for accessing Amazon QuickSight.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.quicksight.AbstractAmazonQuickSight} instead.
*
*
* Amazon QuickSight API Reference
*
* Amazon QuickSight is a fully managed, serverless business intelligence service for the Amazon Web Services Cloud that
* makes it easy to extend data and insights to every user in your organization. This API reference contains
* documentation for a programming interface that you can use to manage Amazon QuickSight.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonQuickSight {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "quicksight";
/**
*
* Cancels an ongoing ingestion of data into SPICE.
*
*
* @param cancelIngestionRequest
* @return Result of the CancelIngestion operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CancelIngestion
* @see AWS API
* Documentation
*/
CancelIngestionResult cancelIngestion(CancelIngestionRequest cancelIngestionRequest);
/**
*
* Creates Amazon QuickSight customizations for the current Amazon Web Services Region. Currently, you can add a
* custom default theme by using the CreateAccountCustomization
or
* UpdateAccountCustomization
API operation. To further customize Amazon QuickSight by removing Amazon
* QuickSight sample assets and videos for all new users, see Customizing Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* You can create customizations for your Amazon Web Services account or, if you specify a namespace, for a
* QuickSight namespace instead. Customizations that apply to a namespace always override customizations that apply
* to an Amazon Web Services account. To find out which customizations apply, use the
* DescribeAccountCustomization
API operation.
*
*
* Before you use the CreateAccountCustomization
API operation to add a theme as the namespace default,
* make sure that you first share the theme with the namespace. If you don't share it with the namespace, the theme
* isn't visible to your users even if you make it the default theme. To check if the theme is shared, view the
* current permissions by using the
* DescribeThemePermissions
* API operation. To share the theme, grant permissions by using the
* UpdateThemePermissions
* API operation.
*
*
* @param createAccountCustomizationRequest
* @return Result of the CreateAccountCustomization operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.CreateAccountCustomization
* @see AWS API Documentation
*/
CreateAccountCustomizationResult createAccountCustomization(CreateAccountCustomizationRequest createAccountCustomizationRequest);
/**
*
* Creates an Amazon QuickSight account, or subscribes to Amazon QuickSight Q.
*
*
* The Amazon Web Services Region for the account is derived from what is configured in the CLI or SDK. This
* operation isn't supported in the US East (Ohio) Region, South America (Sao Paulo) Region, or Asia Pacific
* (Singapore) Region.
*
*
* Before you use this operation, make sure that you can connect to an existing Amazon Web Services account. If you
* don't have an Amazon Web Services account, see Sign up for Amazon Web
* Services in the Amazon QuickSight User Guide. The person who signs up for Amazon QuickSight needs to
* have the correct Identity and Access Management (IAM) permissions. For more information, see IAM Policy Examples for Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* If your IAM policy includes both the Subscribe
and CreateAccountSubscription
actions,
* make sure that both actions are set to Allow
. If either action is set to Deny
, the
* Deny
action prevails and your API call fails.
*
*
* You can't pass an existing IAM role to access other Amazon Web Services services using this API operation. To
* pass your existing IAM role to Amazon QuickSight, see Passing IAM roles to Amazon QuickSight in the Amazon QuickSight User Guide.
*
*
* You can't set default resource access on the new account from the Amazon QuickSight API. Instead, add default
* resource access from the Amazon QuickSight console. For more information about setting default resource access to
* Amazon Web Services services, see Setting default resource
* access to Amazon Web Services services in the Amazon QuickSight User Guide.
*
*
* @param createAccountSubscriptionRequest
* @return Result of the CreateAccountSubscription operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.CreateAccountSubscription
* @see AWS API Documentation
*/
CreateAccountSubscriptionResult createAccountSubscription(CreateAccountSubscriptionRequest createAccountSubscriptionRequest);
/**
*
* Creates an analysis in Amazon QuickSight. Analyses can be created either from a template or from an
* AnalysisDefinition
.
*
*
* @param createAnalysisRequest
* @return Result of the CreateAnalysis operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateAnalysis
* @see AWS API
* Documentation
*/
CreateAnalysisResult createAnalysis(CreateAnalysisRequest createAnalysisRequest);
/**
*
* Creates a dashboard from either a template or directly with a DashboardDefinition
. To first create a
* template, see the
* CreateTemplate
* API operation.
*
*
* A dashboard is an entity in Amazon QuickSight that identifies Amazon QuickSight reports, created from analyses.
* You can share Amazon QuickSight dashboards. With the right permissions, you can create scheduled email reports
* from them. If you have the correct permissions, you can create a dashboard from a template that exists in a
* different Amazon Web Services account.
*
*
* @param createDashboardRequest
* @return Result of the CreateDashboard operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateDashboard
* @see AWS API
* Documentation
*/
CreateDashboardResult createDashboard(CreateDashboardRequest createDashboardRequest);
/**
*
* Creates a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param createDataSetRequest
* @return Result of the CreateDataSet operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateDataSet
* @see AWS API
* Documentation
*/
CreateDataSetResult createDataSet(CreateDataSetRequest createDataSetRequest);
/**
*
* Creates a data source.
*
*
* @param createDataSourceRequest
* @return Result of the CreateDataSource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateDataSource
* @see AWS
* API Documentation
*/
CreateDataSourceResult createDataSource(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates an empty shared folder.
*
*
* @param createFolderRequest
* @return Result of the CreateFolder operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateFolder
* @see AWS API
* Documentation
*/
CreateFolderResult createFolder(CreateFolderRequest createFolderRequest);
/**
*
* Adds an asset, such as a dashboard, analysis, or dataset into a folder.
*
*
* @param createFolderMembershipRequest
* @return Result of the CreateFolderMembership operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ThrottlingException
* Access is throttled.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateFolderMembership
* @see AWS API Documentation
*/
CreateFolderMembershipResult createFolderMembership(CreateFolderMembershipRequest createFolderMembershipRequest);
/**
*
* Use the CreateGroup
operation to create a group in Amazon QuickSight. You can create up to 10,000
* groups in a namespace. If you want to create more than 10,000 groups in a namespace, contact AWS Support.
*
*
* The permissions resource is
* arn:aws:quicksight:<your-region>:<relevant-aws-account-id>:group/default/<group-name>
* .
*
*
* The response is a group object.
*
*
* @param createGroupRequest
* The request object for this operation.
* @return Result of the CreateGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.CreateGroup
* @see AWS API
* Documentation
*/
CreateGroupResult createGroup(CreateGroupRequest createGroupRequest);
/**
*
* Adds an Amazon QuickSight user to an Amazon QuickSight group.
*
*
* @param createGroupMembershipRequest
* @return Result of the CreateGroupMembership operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.CreateGroupMembership
* @see AWS API Documentation
*/
CreateGroupMembershipResult createGroupMembership(CreateGroupMembershipRequest createGroupMembershipRequest);
/**
*
* Creates an assignment with one specified IAM policy, identified by its Amazon Resource Name (ARN). This policy
* assignment is attached to the specified groups or users of Amazon QuickSight. Assignment names are unique per
* Amazon Web Services account. To avoid overwriting rules in other namespaces, use assignment names that are
* unique.
*
*
* @param createIAMPolicyAssignmentRequest
* @return Result of the CreateIAMPolicyAssignment operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConcurrentUpdatingException
* A resource is already in a state that indicates an operation is happening that must complete before a new
* update can be applied.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateIAMPolicyAssignment
* @see AWS API Documentation
*/
CreateIAMPolicyAssignmentResult createIAMPolicyAssignment(CreateIAMPolicyAssignmentRequest createIAMPolicyAssignmentRequest);
/**
*
* Creates and starts a new SPICE ingestion for a dataset. You can manually refresh datasets in an Enterprise
* edition account 32 times in a 24-hour period. You can manually refresh datasets in a Standard edition account 8
* times in a 24-hour period. Each 24-hour period is measured starting 24 hours before the current date and time.
*
*
* Any ingestions operating on tagged datasets inherit the same tags automatically for use in access control. For an
* example, see How do I
* create an IAM policy to control access to Amazon EC2 resources using tags? in the Amazon Web Services
* Knowledge Center. Tags are visible on the tagged dataset, but not on the ingestion resource.
*
*
* @param createIngestionRequest
* @return Result of the CreateIngestion operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateIngestion
* @see AWS API
* Documentation
*/
CreateIngestionResult createIngestion(CreateIngestionRequest createIngestionRequest);
/**
*
* (Enterprise edition only) Creates a new namespace for you to use with Amazon QuickSight.
*
*
* A namespace allows you to isolate the Amazon QuickSight users and groups that are registered for that namespace.
* Users that access the namespace can share assets only with other users or groups in the same namespace. They
* can't see users and groups in other namespaces. You can create a namespace after your Amazon Web Services account
* is subscribed to Amazon QuickSight. The namespace must be unique within the Amazon Web Services account. By
* default, there is a limit of 100 namespaces per Amazon Web Services account. To increase your limit, create a
* ticket with Amazon Web Services Support.
*
*
* @param createNamespaceRequest
* @return Result of the CreateNamespace operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.CreateNamespace
* @see AWS API
* Documentation
*/
CreateNamespaceResult createNamespace(CreateNamespaceRequest createNamespaceRequest);
/**
*
* Creates a template either from a TemplateDefinition
or from an existing Amazon QuickSight analysis
* or template. You can use the resulting template to create additional dashboards, templates, or analyses.
*
*
* A template is an entity in Amazon QuickSight that encapsulates the metadata required to create an analysis
* and that you can use to create s dashboard. A template adds a layer of abstraction by using placeholders to
* replace the dataset associated with the analysis. You can use templates to create dashboards by replacing dataset
* placeholders with datasets that follow the same schema that was used to create the source analysis and template.
*
*
* @param createTemplateRequest
* @return Result of the CreateTemplate operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateTemplate
* @see AWS API
* Documentation
*/
CreateTemplateResult createTemplate(CreateTemplateRequest createTemplateRequest);
/**
*
* Creates a template alias for a template.
*
*
* @param createTemplateAliasRequest
* @return Result of the CreateTemplateAlias operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateTemplateAlias
* @see AWS
* API Documentation
*/
CreateTemplateAliasResult createTemplateAlias(CreateTemplateAliasRequest createTemplateAliasRequest);
/**
*
* Creates a theme.
*
*
* A theme is set of configuration options for color and layout. Themes apply to analyses and dashboards. For
* more information, see Using Themes in Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* @param createThemeRequest
* @return Result of the CreateTheme operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateTheme
* @see AWS API
* Documentation
*/
CreateThemeResult createTheme(CreateThemeRequest createThemeRequest);
/**
*
* Creates a theme alias for a theme.
*
*
* @param createThemeAliasRequest
* @return Result of the CreateThemeAlias operation returned by the service.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.CreateThemeAlias
* @see AWS
* API Documentation
*/
CreateThemeAliasResult createThemeAlias(CreateThemeAliasRequest createThemeAliasRequest);
/**
*
* Deletes all Amazon QuickSight customizations in this Amazon Web Services Region for the specified Amazon Web
* Services account and Amazon QuickSight namespace.
*
*
* @param deleteAccountCustomizationRequest
* @return Result of the DeleteAccountCustomization operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteAccountCustomization
* @see AWS API Documentation
*/
DeleteAccountCustomizationResult deleteAccountCustomization(DeleteAccountCustomizationRequest deleteAccountCustomizationRequest);
/**
*
* Use the DeleteAccountSubscription
operation to delete an Amazon QuickSight account. This operation
* will result in an error message if you have configured your account termination protection settings to
* True
. To change this setting and delete your account, call the UpdateAccountSettings
* API and set the value of the TerminationProtectionEnabled
parameter to False
, then make
* another call to the DeleteAccountSubscription
API.
*
*
* @param deleteAccountSubscriptionRequest
* @return Result of the DeleteAccountSubscription operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteAccountSubscription
* @see AWS API Documentation
*/
DeleteAccountSubscriptionResult deleteAccountSubscription(DeleteAccountSubscriptionRequest deleteAccountSubscriptionRequest);
/**
*
* Deletes an analysis from Amazon QuickSight. You can optionally include a recovery window during which you can
* restore the analysis. If you don't specify a recovery window value, the operation defaults to 30 days. Amazon
* QuickSight attaches a DeletionTime
stamp to the response that specifies the end of the recovery
* window. At the end of the recovery window, Amazon QuickSight deletes the analysis permanently.
*
*
* At any time before recovery window ends, you can use the RestoreAnalysis
API operation to remove the
* DeletionTime
stamp and cancel the deletion of the analysis. The analysis remains visible in the API
* until it's deleted, so you can describe it but you can't make a template from it.
*
*
* An analysis that's scheduled for deletion isn't accessible in the Amazon QuickSight console. To access it in the
* console, restore it. Deleting an analysis doesn't delete the dashboards that you publish from it.
*
*
* @param deleteAnalysisRequest
* @return Result of the DeleteAnalysis operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteAnalysis
* @see AWS API
* Documentation
*/
DeleteAnalysisResult deleteAnalysis(DeleteAnalysisRequest deleteAnalysisRequest);
/**
*
* Deletes a dashboard.
*
*
* @param deleteDashboardRequest
* @return Result of the DeleteDashboard operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteDashboard
* @see AWS API
* Documentation
*/
DeleteDashboardResult deleteDashboard(DeleteDashboardRequest deleteDashboardRequest);
/**
*
* Deletes a dataset.
*
*
* @param deleteDataSetRequest
* @return Result of the DeleteDataSet operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteDataSet
* @see AWS API
* Documentation
*/
DeleteDataSetResult deleteDataSet(DeleteDataSetRequest deleteDataSetRequest);
/**
*
* Deletes the data source permanently. This operation breaks all the datasets that reference the deleted data
* source.
*
*
* @param deleteDataSourceRequest
* @return Result of the DeleteDataSource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteDataSource
* @see AWS
* API Documentation
*/
DeleteDataSourceResult deleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes an empty folder.
*
*
* @param deleteFolderRequest
* @return Result of the DeleteFolder operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteFolder
* @see AWS API
* Documentation
*/
DeleteFolderResult deleteFolder(DeleteFolderRequest deleteFolderRequest);
/**
*
* Removes an asset, such as a dashboard, analysis, or dataset, from a folder.
*
*
* @param deleteFolderMembershipRequest
* @return Result of the DeleteFolderMembership operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteFolderMembership
* @see AWS API Documentation
*/
DeleteFolderMembershipResult deleteFolderMembership(DeleteFolderMembershipRequest deleteFolderMembershipRequest);
/**
*
* Removes a user group from Amazon QuickSight.
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteGroup
* @see AWS API
* Documentation
*/
DeleteGroupResult deleteGroup(DeleteGroupRequest deleteGroupRequest);
/**
*
* Removes a user from a group so that the user is no longer a member of the group.
*
*
* @param deleteGroupMembershipRequest
* @return Result of the DeleteGroupMembership operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteGroupMembership
* @see AWS API Documentation
*/
DeleteGroupMembershipResult deleteGroupMembership(DeleteGroupMembershipRequest deleteGroupMembershipRequest);
/**
*
* Deletes an existing IAM policy assignment.
*
*
* @param deleteIAMPolicyAssignmentRequest
* @return Result of the DeleteIAMPolicyAssignment operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConcurrentUpdatingException
* A resource is already in a state that indicates an operation is happening that must complete before a new
* update can be applied.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteIAMPolicyAssignment
* @see AWS API Documentation
*/
DeleteIAMPolicyAssignmentResult deleteIAMPolicyAssignment(DeleteIAMPolicyAssignmentRequest deleteIAMPolicyAssignmentRequest);
/**
*
* Deletes a namespace and the users and groups that are associated with the namespace. This is an asynchronous
* process. Assets including dashboards, analyses, datasets and data sources are not deleted. To delete these
* assets, you use the API operations for the relevant asset.
*
*
* @param deleteNamespaceRequest
* @return Result of the DeleteNamespace operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteNamespace
* @see AWS API
* Documentation
*/
DeleteNamespaceResult deleteNamespace(DeleteNamespaceRequest deleteNamespaceRequest);
/**
*
* Deletes a template.
*
*
* @param deleteTemplateRequest
* @return Result of the DeleteTemplate operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteTemplate
* @see AWS API
* Documentation
*/
DeleteTemplateResult deleteTemplate(DeleteTemplateRequest deleteTemplateRequest);
/**
*
* Deletes the item that the specified template alias points to. If you provide a specific alias, you delete the
* version of the template that the alias points to.
*
*
* @param deleteTemplateAliasRequest
* @return Result of the DeleteTemplateAlias operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteTemplateAlias
* @see AWS
* API Documentation
*/
DeleteTemplateAliasResult deleteTemplateAlias(DeleteTemplateAliasRequest deleteTemplateAliasRequest);
/**
*
* Deletes a theme.
*
*
* @param deleteThemeRequest
* @return Result of the DeleteTheme operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteTheme
* @see AWS API
* Documentation
*/
DeleteThemeResult deleteTheme(DeleteThemeRequest deleteThemeRequest);
/**
*
* Deletes the version of the theme that the specified theme alias points to. If you provide a specific alias, you
* delete the version of the theme that the alias points to.
*
*
* @param deleteThemeAliasRequest
* @return Result of the DeleteThemeAlias operation returned by the service.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DeleteThemeAlias
* @see AWS
* API Documentation
*/
DeleteThemeAliasResult deleteThemeAlias(DeleteThemeAliasRequest deleteThemeAliasRequest);
/**
*
* Deletes the Amazon QuickSight user that is associated with the identity of the Identity and Access Management
* (IAM) user or role that's making the call. The IAM user isn't deleted as a result of this call.
*
*
* @param deleteUserRequest
* @return Result of the DeleteUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteUser
* @see AWS API
* Documentation
*/
DeleteUserResult deleteUser(DeleteUserRequest deleteUserRequest);
/**
*
* Deletes a user identified by its principal ID.
*
*
* @param deleteUserByPrincipalIdRequest
* @return Result of the DeleteUserByPrincipalId operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DeleteUserByPrincipalId
* @see AWS API Documentation
*/
DeleteUserByPrincipalIdResult deleteUserByPrincipalId(DeleteUserByPrincipalIdRequest deleteUserByPrincipalIdRequest);
/**
*
* Describes the customizations associated with the provided Amazon Web Services account and Amazon Amazon
* QuickSight namespace in an Amazon Web Services Region. The Amazon QuickSight console evaluates which
* customizations to apply by running this API operation with the Resolved
flag included.
*
*
* To determine what customizations display when you run this command, it can help to visualize the relationship of
* the entities involved.
*
*
* -
*
* Amazon Web Services account
- The Amazon Web Services account exists at the top of the hierarchy. It
* has the potential to use all of the Amazon Web Services Regions and Amazon Web Services Services. When you
* subscribe to Amazon QuickSight, you choose one Amazon Web Services Region to use as your home Region. That's
* where your free SPICE capacity is located. You can use Amazon QuickSight in any supported Amazon Web Services
* Region.
*
*
* -
*
* Amazon Web Services Region
- In each Amazon Web Services Region where you sign in to Amazon
* QuickSight at least once, Amazon QuickSight acts as a separate instance of the same service. If you have a user
* directory, it resides in us-east-1, which is the US East (N. Virginia). Generally speaking, these users have
* access to Amazon QuickSight in any Amazon Web Services Region, unless they are constrained to a namespace.
*
*
* To run the command in a different Amazon Web Services Region, you change your Region settings. If you're using
* the CLI, you can use one of the following options:
*
*
* -
*
* Use command line
* options.
*
*
* -
*
* Use named profiles.
*
*
* -
*
* Run aws configure
to change your default Amazon Web Services Region. Use Enter to key the same
* settings for your keys. For more information, see Configuring the CLI.
*
*
*
*
* -
*
* Namespace
- A QuickSight namespace is a partition that contains users and assets (data sources,
* datasets, dashboards, and so on). To access assets that are in a specific namespace, users and groups must also
* be part of the same namespace. People who share a namespace are completely isolated from users and assets in
* other namespaces, even if they are in the same Amazon Web Services account and Amazon Web Services Region.
*
*
* -
*
* Applied customizations
- Within an Amazon Web Services Region, a set of Amazon QuickSight
* customizations can apply to an Amazon Web Services account or to a namespace. Settings that you apply to a
* namespace override settings that you apply to an Amazon Web Services account. All settings are isolated to a
* single Amazon Web Services Region. To apply them in other Amazon Web Services Regions, run the
* CreateAccountCustomization
command in each Amazon Web Services Region where you want to apply the
* same customizations.
*
*
*
*
* @param describeAccountCustomizationRequest
* @return Result of the DescribeAccountCustomization operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeAccountCustomization
* @see AWS API Documentation
*/
DescribeAccountCustomizationResult describeAccountCustomization(DescribeAccountCustomizationRequest describeAccountCustomizationRequest);
/**
*
* Describes the settings that were used when your Amazon QuickSight subscription was first created in this Amazon
* Web Services account.
*
*
* @param describeAccountSettingsRequest
* @return Result of the DescribeAccountSettings operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeAccountSettings
* @see AWS API Documentation
*/
DescribeAccountSettingsResult describeAccountSettings(DescribeAccountSettingsRequest describeAccountSettingsRequest);
/**
*
* Use the DescribeAccountSubscription operation to receive a description of an Amazon QuickSight account's
* subscription. A successful API call returns an AccountInfo
object that includes an account's name,
* subscription status, authentication type, edition, and notification email address.
*
*
* @param describeAccountSubscriptionRequest
* @return Result of the DescribeAccountSubscription operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeAccountSubscription
* @see AWS API Documentation
*/
DescribeAccountSubscriptionResult describeAccountSubscription(DescribeAccountSubscriptionRequest describeAccountSubscriptionRequest);
/**
*
* Provides a summary of the metadata for an analysis.
*
*
* @param describeAnalysisRequest
* @return Result of the DescribeAnalysis operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeAnalysis
* @see AWS
* API Documentation
*/
DescribeAnalysisResult describeAnalysis(DescribeAnalysisRequest describeAnalysisRequest);
/**
*
* Provides a detailed description of the definition of an analysis.
*
*
*
* If you do not need to know details about the content of an Analysis, for instance if you are trying to check the
* status of a recently created or updated Analysis, use the
* DescribeAnalysis
instead.
*
*
*
* @param describeAnalysisDefinitionRequest
* @return Result of the DescribeAnalysisDefinition operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeAnalysisDefinition
* @see AWS API Documentation
*/
DescribeAnalysisDefinitionResult describeAnalysisDefinition(DescribeAnalysisDefinitionRequest describeAnalysisDefinitionRequest);
/**
*
* Provides the read and write permissions for an analysis.
*
*
* @param describeAnalysisPermissionsRequest
* @return Result of the DescribeAnalysisPermissions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeAnalysisPermissions
* @see AWS API Documentation
*/
DescribeAnalysisPermissionsResult describeAnalysisPermissions(DescribeAnalysisPermissionsRequest describeAnalysisPermissionsRequest);
/**
*
* Provides a summary for a dashboard.
*
*
* @param describeDashboardRequest
* @return Result of the DescribeDashboard operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDashboard
* @see AWS
* API Documentation
*/
DescribeDashboardResult describeDashboard(DescribeDashboardRequest describeDashboardRequest);
/**
*
* Provides a detailed description of the definition of a dashboard.
*
*
*
* If you do not need to know details about the content of a dashboard, for instance if you are trying to check the
* status of a recently created or updated dashboard, use the
* DescribeDashboard
instead.
*
*
*
* @param describeDashboardDefinitionRequest
* @return Result of the DescribeDashboardDefinition operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDashboardDefinition
* @see AWS API Documentation
*/
DescribeDashboardDefinitionResult describeDashboardDefinition(DescribeDashboardDefinitionRequest describeDashboardDefinitionRequest);
/**
*
* Describes read and write permissions for a dashboard.
*
*
* @param describeDashboardPermissionsRequest
* @return Result of the DescribeDashboardPermissions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDashboardPermissions
* @see AWS API Documentation
*/
DescribeDashboardPermissionsResult describeDashboardPermissions(DescribeDashboardPermissionsRequest describeDashboardPermissionsRequest);
/**
*
* Describes a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param describeDataSetRequest
* @return Result of the DescribeDataSet operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDataSet
* @see AWS API
* Documentation
*/
DescribeDataSetResult describeDataSet(DescribeDataSetRequest describeDataSetRequest);
/**
*
* Describes the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param describeDataSetPermissionsRequest
* @return Result of the DescribeDataSetPermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDataSetPermissions
* @see AWS API Documentation
*/
DescribeDataSetPermissionsResult describeDataSetPermissions(DescribeDataSetPermissionsRequest describeDataSetPermissionsRequest);
/**
*
* Describes a data source.
*
*
* @param describeDataSourceRequest
* @return Result of the DescribeDataSource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDataSource
* @see AWS
* API Documentation
*/
DescribeDataSourceResult describeDataSource(DescribeDataSourceRequest describeDataSourceRequest);
/**
*
* Describes the resource permissions for a data source.
*
*
* @param describeDataSourcePermissionsRequest
* @return Result of the DescribeDataSourcePermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeDataSourcePermissions
* @see AWS API Documentation
*/
DescribeDataSourcePermissionsResult describeDataSourcePermissions(DescribeDataSourcePermissionsRequest describeDataSourcePermissionsRequest);
/**
*
* Describes a folder.
*
*
* @param describeFolderRequest
* @return Result of the DescribeFolder operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeFolder
* @see AWS API
* Documentation
*/
DescribeFolderResult describeFolder(DescribeFolderRequest describeFolderRequest);
/**
*
* Describes permissions for a folder.
*
*
* @param describeFolderPermissionsRequest
* @return Result of the DescribeFolderPermissions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeFolderPermissions
* @see AWS API Documentation
*/
DescribeFolderPermissionsResult describeFolderPermissions(DescribeFolderPermissionsRequest describeFolderPermissionsRequest);
/**
*
* Describes the folder resolved permissions. Permissions consists of both folder direct permissions and the
* inherited permissions from the ancestor folders.
*
*
* @param describeFolderResolvedPermissionsRequest
* @return Result of the DescribeFolderResolvedPermissions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeFolderResolvedPermissions
* @see AWS API Documentation
*/
DescribeFolderResolvedPermissionsResult describeFolderResolvedPermissions(DescribeFolderResolvedPermissionsRequest describeFolderResolvedPermissionsRequest);
/**
*
* Returns an Amazon QuickSight group's description and Amazon Resource Name (ARN).
*
*
* @param describeGroupRequest
* @return Result of the DescribeGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeGroup
* @see AWS API
* Documentation
*/
DescribeGroupResult describeGroup(DescribeGroupRequest describeGroupRequest);
/**
*
* Use the DescribeGroupMembership
operation to determine if a user is a member of the specified group.
* If the user exists and is a member of the specified group, an associated GroupMember
object is
* returned.
*
*
* @param describeGroupMembershipRequest
* @return Result of the DescribeGroupMembership operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeGroupMembership
* @see AWS API Documentation
*/
DescribeGroupMembershipResult describeGroupMembership(DescribeGroupMembershipRequest describeGroupMembershipRequest);
/**
*
* Describes an existing IAM policy assignment, as specified by the assignment name.
*
*
* @param describeIAMPolicyAssignmentRequest
* @return Result of the DescribeIAMPolicyAssignment operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeIAMPolicyAssignment
* @see AWS API Documentation
*/
DescribeIAMPolicyAssignmentResult describeIAMPolicyAssignment(DescribeIAMPolicyAssignmentRequest describeIAMPolicyAssignmentRequest);
/**
*
* Describes a SPICE ingestion.
*
*
* @param describeIngestionRequest
* @return Result of the DescribeIngestion operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeIngestion
* @see AWS
* API Documentation
*/
DescribeIngestionResult describeIngestion(DescribeIngestionRequest describeIngestionRequest);
/**
*
* Provides a summary and status of IP rules.
*
*
* @param describeIpRestrictionRequest
* @return Result of the DescribeIpRestriction operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeIpRestriction
* @see AWS API Documentation
*/
DescribeIpRestrictionResult describeIpRestriction(DescribeIpRestrictionRequest describeIpRestrictionRequest);
/**
*
* Describes the current namespace.
*
*
* @param describeNamespaceRequest
* @return Result of the DescribeNamespace operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeNamespace
* @see AWS
* API Documentation
*/
DescribeNamespaceResult describeNamespace(DescribeNamespaceRequest describeNamespaceRequest);
/**
*
* Describes a template's metadata.
*
*
* @param describeTemplateRequest
* @return Result of the DescribeTemplate operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeTemplate
* @see AWS
* API Documentation
*/
DescribeTemplateResult describeTemplate(DescribeTemplateRequest describeTemplateRequest);
/**
*
* Describes the template alias for a template.
*
*
* @param describeTemplateAliasRequest
* @return Result of the DescribeTemplateAlias operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeTemplateAlias
* @see AWS API Documentation
*/
DescribeTemplateAliasResult describeTemplateAlias(DescribeTemplateAliasRequest describeTemplateAliasRequest);
/**
*
* Provides a detailed description of the definition of a template.
*
*
*
* If you do not need to know details about the content of a template, for instance if you are trying to check the
* status of a recently created or updated template, use the
* DescribeTemplate
instead.
*
*
*
* @param describeTemplateDefinitionRequest
* @return Result of the DescribeTemplateDefinition operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeTemplateDefinition
* @see AWS API Documentation
*/
DescribeTemplateDefinitionResult describeTemplateDefinition(DescribeTemplateDefinitionRequest describeTemplateDefinitionRequest);
/**
*
* Describes read and write permissions on a template.
*
*
* @param describeTemplatePermissionsRequest
* @return Result of the DescribeTemplatePermissions operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeTemplatePermissions
* @see AWS API Documentation
*/
DescribeTemplatePermissionsResult describeTemplatePermissions(DescribeTemplatePermissionsRequest describeTemplatePermissionsRequest);
/**
*
* Describes a theme.
*
*
* @param describeThemeRequest
* @return Result of the DescribeTheme operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeTheme
* @see AWS API
* Documentation
*/
DescribeThemeResult describeTheme(DescribeThemeRequest describeThemeRequest);
/**
*
* Describes the alias for a theme.
*
*
* @param describeThemeAliasRequest
* @return Result of the DescribeThemeAlias operation returned by the service.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeThemeAlias
* @see AWS
* API Documentation
*/
DescribeThemeAliasResult describeThemeAlias(DescribeThemeAliasRequest describeThemeAliasRequest);
/**
*
* Describes the read and write permissions for a theme.
*
*
* @param describeThemePermissionsRequest
* @return Result of the DescribeThemePermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.DescribeThemePermissions
* @see AWS API Documentation
*/
DescribeThemePermissionsResult describeThemePermissions(DescribeThemePermissionsRequest describeThemePermissionsRequest);
/**
*
* Returns information about a user, given the user name.
*
*
* @param describeUserRequest
* @return Result of the DescribeUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.DescribeUser
* @see AWS API
* Documentation
*/
DescribeUserResult describeUser(DescribeUserRequest describeUserRequest);
/**
*
* Generates an embed URL that you can use to embed an Amazon QuickSight dashboard or visual in your website,
* without having to register any reader users. Before you use this action, make sure that you have configured the
* dashboards and permissions.
*
*
* The following rules apply to the generated URL:
*
*
* -
*
* It contains a temporary bearer token. It is valid for 5 minutes after it is generated. Once redeemed within this
* period, it cannot be re-used again.
*
*
* -
*
* The URL validity period should not be confused with the actual session lifetime that can be customized using the
* SessionLifetimeInMinutes
* parameter. The resulting user session is valid for 15 minutes (minimum) to 10 hours (maximum). The default
* session duration is 10 hours.
*
*
* -
*
* You are charged only when the URL is used or there is interaction with Amazon QuickSight.
*
*
*
*
* For more information, see Embedded Analytics in the
* Amazon QuickSight User Guide.
*
*
* For more information about the high-level steps for embedding and for an interactive demo of the ways you can
* customize embedding, visit the Amazon QuickSight Developer
* Portal.
*
*
* @param generateEmbedUrlForAnonymousUserRequest
* @return Result of the GenerateEmbedUrlForAnonymousUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws SessionLifetimeInMinutesInvalidException
* The number of minutes specified for the lifetime of a session isn't valid. The session lifetime must be
* 15-600 minutes.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws UnsupportedPricingPlanException
* This error indicates that you are calling an embedding operation in Amazon QuickSight without the
* required pricing plan on your Amazon Web Services account. Before you can use embedding for anonymous
* users, a QuickSight administrator needs to add capacity pricing to Amazon QuickSight. You can do this on
* the Manage Amazon QuickSight page.
*
* After capacity pricing is added, you can use the
* GetDashboardEmbedUrl
* API operation with the --identity-type ANONYMOUS
option.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.GenerateEmbedUrlForAnonymousUser
* @see AWS API Documentation
*/
GenerateEmbedUrlForAnonymousUserResult generateEmbedUrlForAnonymousUser(GenerateEmbedUrlForAnonymousUserRequest generateEmbedUrlForAnonymousUserRequest);
/**
*
* Generates an embed URL that you can use to embed an Amazon QuickSight experience in your website. This action can
* be used for any type of user registered in an Amazon QuickSight account. Before you use this action, make sure
* that you have configured the relevant Amazon QuickSight resource and permissions.
*
*
* The following rules apply to the generated URL:
*
*
* -
*
* It contains a temporary bearer token. It is valid for 5 minutes after it is generated. Once redeemed within this
* period, it cannot be re-used again.
*
*
* -
*
* The URL validity period should not be confused with the actual session lifetime that can be customized using the
* SessionLifetimeInMinutes
* parameter.
*
*
* The resulting user session is valid for 15 minutes (minimum) to 10 hours (maximum). The default session duration
* is 10 hours.
*
*
* -
*
* You are charged only when the URL is used or there is interaction with Amazon QuickSight.
*
*
*
*
* For more information, see Embedded Analytics in the
* Amazon QuickSight User Guide.
*
*
* For more information about the high-level steps for embedding and for an interactive demo of the ways you can
* customize embedding, visit the Amazon QuickSight Developer
* Portal.
*
*
* @param generateEmbedUrlForRegisteredUserRequest
* @return Result of the GenerateEmbedUrlForRegisteredUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws QuickSightUserNotFoundException
* The user with the provided name isn't found. This error can happen in any operation that requires finding
* a user based on a provided user name, such as DeleteUser
, DescribeUser
, and so
* on.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws SessionLifetimeInMinutesInvalidException
* The number of minutes specified for the lifetime of a session isn't valid. The session lifetime must be
* 15-600 minutes.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws UnsupportedPricingPlanException
* This error indicates that you are calling an embedding operation in Amazon QuickSight without the
* required pricing plan on your Amazon Web Services account. Before you can use embedding for anonymous
* users, a QuickSight administrator needs to add capacity pricing to Amazon QuickSight. You can do this on
* the Manage Amazon QuickSight page.
*
* After capacity pricing is added, you can use the
* GetDashboardEmbedUrl
* API operation with the --identity-type ANONYMOUS
option.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.GenerateEmbedUrlForRegisteredUser
* @see AWS API Documentation
*/
GenerateEmbedUrlForRegisteredUserResult generateEmbedUrlForRegisteredUser(GenerateEmbedUrlForRegisteredUserRequest generateEmbedUrlForRegisteredUserRequest);
/**
*
* Generates a temporary session URL and authorization code(bearer token) that you can use to embed an Amazon
* QuickSight read-only dashboard in your website or application. Before you use this command, make sure that you
* have configured the dashboards and permissions.
*
*
* Currently, you can use GetDashboardEmbedURL
only from the server, not from the user's browser. The
* following rules apply to the generated URL:
*
*
* -
*
* They must be used together.
*
*
* -
*
* They can be used one time only.
*
*
* -
*
* They are valid for 5 minutes after you run this command.
*
*
* -
*
* You are charged only when the URL is used or there is interaction with Amazon QuickSight.
*
*
* -
*
* The resulting user session is valid for 15 minutes (default) up to 10 hours (maximum). You can use the optional
* SessionLifetimeInMinutes
parameter to customize session duration.
*
*
*
*
* For more information, see Embedding Analytics
* Using GetDashboardEmbedUrl in the Amazon QuickSight User Guide.
*
*
* For more information about the high-level steps for embedding and for an interactive demo of the ways you can
* customize embedding, visit the Amazon QuickSight Developer
* Portal.
*
*
* @param getDashboardEmbedUrlRequest
* @return Result of the GetDashboardEmbedUrl operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws DomainNotWhitelistedException
* The domain specified isn't on the allow list. All domains for embedded dashboards must be added to the
* approved list by an Amazon QuickSight admin.
* @throws QuickSightUserNotFoundException
* The user with the provided name isn't found. This error can happen in any operation that requires finding
* a user based on a provided user name, such as DeleteUser
, DescribeUser
, and so
* on.
* @throws IdentityTypeNotSupportedException
* The identity type specified isn't supported. Supported identity types include IAM
and
* QUICKSIGHT
.
* @throws SessionLifetimeInMinutesInvalidException
* The number of minutes specified for the lifetime of a session isn't valid. The session lifetime must be
* 15-600 minutes.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws UnsupportedPricingPlanException
* This error indicates that you are calling an embedding operation in Amazon QuickSight without the
* required pricing plan on your Amazon Web Services account. Before you can use embedding for anonymous
* users, a QuickSight administrator needs to add capacity pricing to Amazon QuickSight. You can do this on
* the Manage Amazon QuickSight page.
*
* After capacity pricing is added, you can use the
* GetDashboardEmbedUrl
* API operation with the --identity-type ANONYMOUS
option.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.GetDashboardEmbedUrl
* @see AWS API Documentation
*/
GetDashboardEmbedUrlResult getDashboardEmbedUrl(GetDashboardEmbedUrlRequest getDashboardEmbedUrlRequest);
/**
*
* Generates a session URL and authorization code that you can use to embed the Amazon Amazon QuickSight console in
* your web server code. Use GetSessionEmbedUrl
where you want to provide an authoring portal that
* allows users to create data sources, datasets, analyses, and dashboards. The users who access an embedded Amazon
* QuickSight console need belong to the author or admin security cohort. If you want to restrict permissions to
* some of these features, add a custom permissions profile to the user with the
* UpdateUser
* API operation. Use
* RegisterUser
* API operation to add a new user with a custom permission profile attached. For more information, see the
* following sections in the Amazon QuickSight User Guide:
*
*
* -
*
*
* -
*
*
*
*
* @param getSessionEmbedUrlRequest
* @return Result of the GetSessionEmbedUrl operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws QuickSightUserNotFoundException
* The user with the provided name isn't found. This error can happen in any operation that requires finding
* a user based on a provided user name, such as DeleteUser
, DescribeUser
, and so
* on.
* @throws SessionLifetimeInMinutesInvalidException
* The number of minutes specified for the lifetime of a session isn't valid. The session lifetime must be
* 15-600 minutes.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.GetSessionEmbedUrl
* @see AWS
* API Documentation
*/
GetSessionEmbedUrlResult getSessionEmbedUrl(GetSessionEmbedUrlRequest getSessionEmbedUrlRequest);
/**
*
* Lists Amazon QuickSight analyses that exist in the specified Amazon Web Services account.
*
*
* @param listAnalysesRequest
* @return Result of the ListAnalyses operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListAnalyses
* @see AWS API
* Documentation
*/
ListAnalysesResult listAnalyses(ListAnalysesRequest listAnalysesRequest);
/**
*
* Lists all the versions of the dashboards in the Amazon QuickSight subscription.
*
*
* @param listDashboardVersionsRequest
* @return Result of the ListDashboardVersions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListDashboardVersions
* @see AWS API Documentation
*/
ListDashboardVersionsResult listDashboardVersions(ListDashboardVersionsRequest listDashboardVersionsRequest);
/**
*
* Lists dashboards in an Amazon Web Services account.
*
*
* @param listDashboardsRequest
* @return Result of the ListDashboards operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListDashboards
* @see AWS API
* Documentation
*/
ListDashboardsResult listDashboards(ListDashboardsRequest listDashboardsRequest);
/**
*
* Lists all of the datasets belonging to the current Amazon Web Services account in an Amazon Web Services Region.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/*
.
*
*
* @param listDataSetsRequest
* @return Result of the ListDataSets operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListDataSets
* @see AWS API
* Documentation
*/
ListDataSetsResult listDataSets(ListDataSetsRequest listDataSetsRequest);
/**
*
* Lists data sources in current Amazon Web Services Region that belong to this Amazon Web Services account.
*
*
* @param listDataSourcesRequest
* @return Result of the ListDataSources operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListDataSources
* @see AWS API
* Documentation
*/
ListDataSourcesResult listDataSources(ListDataSourcesRequest listDataSourcesRequest);
/**
*
* List all assets (DASHBOARD
, ANALYSIS
, and DATASET
) in a folder.
*
*
* @param listFolderMembersRequest
* @return Result of the ListFolderMembers operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListFolderMembers
* @see AWS
* API Documentation
*/
ListFolderMembersResult listFolderMembers(ListFolderMembersRequest listFolderMembersRequest);
/**
*
* Lists all folders in an account.
*
*
* @param listFoldersRequest
* @return Result of the ListFolders operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListFolders
* @see AWS API
* Documentation
*/
ListFoldersResult listFolders(ListFoldersRequest listFoldersRequest);
/**
*
* Lists member users in a group.
*
*
* @param listGroupMembershipsRequest
* @return Result of the ListGroupMemberships operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.ListGroupMemberships
* @see AWS API Documentation
*/
ListGroupMembershipsResult listGroupMemberships(ListGroupMembershipsRequest listGroupMembershipsRequest);
/**
*
* Lists all user groups in Amazon QuickSight.
*
*
* @param listGroupsRequest
* @return Result of the ListGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.ListGroups
* @see AWS API
* Documentation
*/
ListGroupsResult listGroups(ListGroupsRequest listGroupsRequest);
/**
*
* Lists IAM policy assignments in the current Amazon QuickSight account.
*
*
* @param listIAMPolicyAssignmentsRequest
* @return Result of the ListIAMPolicyAssignments operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListIAMPolicyAssignments
* @see AWS API Documentation
*/
ListIAMPolicyAssignmentsResult listIAMPolicyAssignments(ListIAMPolicyAssignmentsRequest listIAMPolicyAssignmentsRequest);
/**
*
* Lists all the IAM policy assignments, including the Amazon Resource Names (ARNs) for the IAM policies assigned to
* the specified user and group or groups that the user belongs to.
*
*
* @param listIAMPolicyAssignmentsForUserRequest
* @return Result of the ListIAMPolicyAssignmentsForUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConcurrentUpdatingException
* A resource is already in a state that indicates an operation is happening that must complete before a new
* update can be applied.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListIAMPolicyAssignmentsForUser
* @see AWS API Documentation
*/
ListIAMPolicyAssignmentsForUserResult listIAMPolicyAssignmentsForUser(ListIAMPolicyAssignmentsForUserRequest listIAMPolicyAssignmentsForUserRequest);
/**
*
* Lists the history of SPICE ingestions for a dataset.
*
*
* @param listIngestionsRequest
* @return Result of the ListIngestions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListIngestions
* @see AWS API
* Documentation
*/
ListIngestionsResult listIngestions(ListIngestionsRequest listIngestionsRequest);
/**
*
* Lists the namespaces for the specified Amazon Web Services account. This operation doesn't list deleted
* namespaces.
*
*
* @param listNamespacesRequest
* @return Result of the ListNamespaces operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.ListNamespaces
* @see AWS API
* Documentation
*/
ListNamespacesResult listNamespaces(ListNamespacesRequest listNamespacesRequest);
/**
*
* Lists the tags assigned to a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all the aliases of a template.
*
*
* @param listTemplateAliasesRequest
* @return Result of the ListTemplateAliases operation returned by the service.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListTemplateAliases
* @see AWS
* API Documentation
*/
ListTemplateAliasesResult listTemplateAliases(ListTemplateAliasesRequest listTemplateAliasesRequest);
/**
*
* Lists all the versions of the templates in the current Amazon QuickSight account.
*
*
* @param listTemplateVersionsRequest
* @return Result of the ListTemplateVersions operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListTemplateVersions
* @see AWS API Documentation
*/
ListTemplateVersionsResult listTemplateVersions(ListTemplateVersionsRequest listTemplateVersionsRequest);
/**
*
* Lists all the templates in the current Amazon QuickSight account.
*
*
* @param listTemplatesRequest
* @return Result of the ListTemplates operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListTemplates
* @see AWS API
* Documentation
*/
ListTemplatesResult listTemplates(ListTemplatesRequest listTemplatesRequest);
/**
*
* Lists all the aliases of a theme.
*
*
* @param listThemeAliasesRequest
* @return Result of the ListThemeAliases operation returned by the service.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListThemeAliases
* @see AWS
* API Documentation
*/
ListThemeAliasesResult listThemeAliases(ListThemeAliasesRequest listThemeAliasesRequest);
/**
*
* Lists all the versions of the themes in the current Amazon Web Services account.
*
*
* @param listThemeVersionsRequest
* @return Result of the ListThemeVersions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListThemeVersions
* @see AWS
* API Documentation
*/
ListThemeVersionsResult listThemeVersions(ListThemeVersionsRequest listThemeVersionsRequest);
/**
*
* Lists all the themes in the current Amazon Web Services account.
*
*
* @param listThemesRequest
* @return Result of the ListThemes operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.ListThemes
* @see AWS API
* Documentation
*/
ListThemesResult listThemes(ListThemesRequest listThemesRequest);
/**
*
* Lists the Amazon QuickSight groups that an Amazon QuickSight user is a member of.
*
*
* @param listUserGroupsRequest
* @return Result of the ListUserGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.ListUserGroups
* @see AWS API
* Documentation
*/
ListUserGroupsResult listUserGroups(ListUserGroupsRequest listUserGroupsRequest);
/**
*
* Returns a list of all of the Amazon QuickSight users belonging to this account.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.ListUsers
* @see AWS API
* Documentation
*/
ListUsersResult listUsers(ListUsersRequest listUsersRequest);
/**
*
* Creates an Amazon QuickSight user whose identity is associated with the Identity and Access Management (IAM)
* identity or role specified in the request. When you register a new user from the Amazon QuickSight API, Amazon
* QuickSight generates a registration URL. The user accesses this registration URL to create their account. Amazon
* QuickSight doesn't send a registration email to users who are registered from the Amazon QuickSight API. If you
* want new users to receive a registration email, then add those users in the Amazon QuickSight console. For more
* information on registering a new user in the Amazon QuickSight console, see Inviting users to
* access Amazon QuickSight.
*
*
* @param registerUserRequest
* @return Result of the RegisterUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.RegisterUser
* @see AWS API
* Documentation
*/
RegisterUserResult registerUser(RegisterUserRequest registerUserRequest);
/**
*
* Restores an analysis.
*
*
* @param restoreAnalysisRequest
* @return Result of the RestoreAnalysis operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.RestoreAnalysis
* @see AWS API
* Documentation
*/
RestoreAnalysisResult restoreAnalysis(RestoreAnalysisRequest restoreAnalysisRequest);
/**
*
* Searches for analyses that belong to the user specified in the filter.
*
*
*
* This operation is eventually consistent. The results are best effort and may not reflect very recent updates and
* changes.
*
*
*
* @param searchAnalysesRequest
* @return Result of the SearchAnalyses operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.SearchAnalyses
* @see AWS API
* Documentation
*/
SearchAnalysesResult searchAnalyses(SearchAnalysesRequest searchAnalysesRequest);
/**
*
* Searches for dashboards that belong to a user.
*
*
*
* This operation is eventually consistent. The results are best effort and may not reflect very recent updates and
* changes.
*
*
*
* @param searchDashboardsRequest
* @return Result of the SearchDashboards operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.SearchDashboards
* @see AWS
* API Documentation
*/
SearchDashboardsResult searchDashboards(SearchDashboardsRequest searchDashboardsRequest);
/**
*
* Use the SearchDataSets
operation to search for datasets that belong to an account.
*
*
* @param searchDataSetsRequest
* @return Result of the SearchDataSets operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.SearchDataSets
* @see AWS API
* Documentation
*/
SearchDataSetsResult searchDataSets(SearchDataSetsRequest searchDataSetsRequest);
/**
*
* Use the SearchDataSources
operation to search for data sources that belong to an account.
*
*
* @param searchDataSourcesRequest
* @return Result of the SearchDataSources operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.SearchDataSources
* @see AWS
* API Documentation
*/
SearchDataSourcesResult searchDataSources(SearchDataSourcesRequest searchDataSourcesRequest);
/**
*
* Searches the subfolders in a folder.
*
*
* @param searchFoldersRequest
* @return Result of the SearchFolders operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InvalidRequestException
* You don't have this feature activated for your account. To fix this issue, contact Amazon Web Services
* support.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.SearchFolders
* @see AWS API
* Documentation
*/
SearchFoldersResult searchFolders(SearchFoldersRequest searchFoldersRequest);
/**
*
* Use the SearchGroups
operation to search groups in a specified Amazon QuickSight namespace using the
* supplied filters.
*
*
* @param searchGroupsRequest
* @return Result of the SearchGroups operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidNextTokenException
* The NextToken
value isn't valid.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.SearchGroups
* @see AWS API
* Documentation
*/
SearchGroupsResult searchGroups(SearchGroupsRequest searchGroupsRequest);
/**
*
* Assigns one or more tags (key-value pairs) to the specified Amazon QuickSight resource.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only resources with certain tag values. You can use the
* TagResource
operation with a resource that already has tags. If you specify a new tag key for the
* resource, this tag is appended to the list of tags associated with the resource. If you specify a tag key that is
* already associated with the resource, the new tag value that you specify replaces the previous value for that
* tag.
*
*
* You can associate as many as 50 tags with a resource. Amazon QuickSight supports tagging on data set, data
* source, dashboard, and template.
*
*
* Tagging for Amazon QuickSight works in a similar way to tagging for other Amazon Web Services services, except
* for the following:
*
*
* -
*
* You can't use tags to track costs for Amazon QuickSight. This isn't possible because you can't tag the resources
* that Amazon QuickSight costs are based on, for example Amazon QuickSight storage capacity (SPICE), number of
* users, type of users, and usage metrics.
*
*
* -
*
* Amazon QuickSight doesn't currently support the tag editor for Resource Groups.
*
*
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws LimitExceededException
* A limit is exceeded.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a tag or tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates Amazon QuickSight customizations for the current Amazon Web Services Region. Currently, the only
* customization that you can use is a theme.
*
*
* You can use customizations for your Amazon Web Services account or, if you specify a namespace, for a Amazon
* QuickSight namespace instead. Customizations that apply to a namespace override customizations that apply to an
* Amazon Web Services account. To find out which customizations apply, use the
* DescribeAccountCustomization
API operation.
*
*
* @param updateAccountCustomizationRequest
* @return Result of the UpdateAccountCustomization operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.UpdateAccountCustomization
* @see AWS API Documentation
*/
UpdateAccountCustomizationResult updateAccountCustomization(UpdateAccountCustomizationRequest updateAccountCustomizationRequest);
/**
*
* Updates the Amazon QuickSight settings in your Amazon Web Services account.
*
*
* @param updateAccountSettingsRequest
* @return Result of the UpdateAccountSettings operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.UpdateAccountSettings
* @see AWS API Documentation
*/
UpdateAccountSettingsResult updateAccountSettings(UpdateAccountSettingsRequest updateAccountSettingsRequest);
/**
*
* Updates an analysis in Amazon QuickSight
*
*
* @param updateAnalysisRequest
* @return Result of the UpdateAnalysis operation returned by the service.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateAnalysis
* @see AWS API
* Documentation
*/
UpdateAnalysisResult updateAnalysis(UpdateAnalysisRequest updateAnalysisRequest);
/**
*
* Updates the read and write permissions for an analysis.
*
*
* @param updateAnalysisPermissionsRequest
* @return Result of the UpdateAnalysisPermissions operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateAnalysisPermissions
* @see AWS API Documentation
*/
UpdateAnalysisPermissionsResult updateAnalysisPermissions(UpdateAnalysisPermissionsRequest updateAnalysisPermissionsRequest);
/**
*
* Updates a dashboard in an Amazon Web Services account.
*
*
*
* Updating a Dashboard creates a new dashboard version but does not immediately publish the new version. You can
* update the published version of a dashboard by using the
* UpdateDashboardPublishedVersion
* API operation.
*
*
*
* @param updateDashboardRequest
* @return Result of the UpdateDashboard operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDashboard
* @see AWS API
* Documentation
*/
UpdateDashboardResult updateDashboard(UpdateDashboardRequest updateDashboardRequest);
/**
*
* Updates read and write permissions on a dashboard.
*
*
* @param updateDashboardPermissionsRequest
* @return Result of the UpdateDashboardPermissions operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDashboardPermissions
* @see AWS API Documentation
*/
UpdateDashboardPermissionsResult updateDashboardPermissions(UpdateDashboardPermissionsRequest updateDashboardPermissionsRequest);
/**
*
* Updates the published version of a dashboard.
*
*
* @param updateDashboardPublishedVersionRequest
* @return Result of the UpdateDashboardPublishedVersion operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDashboardPublishedVersion
* @see AWS API Documentation
*/
UpdateDashboardPublishedVersionResult updateDashboardPublishedVersion(UpdateDashboardPublishedVersionRequest updateDashboardPublishedVersionRequest);
/**
*
* Updates a dataset. This operation doesn't support datasets that include uploaded files as a source. Partial
* updates are not supported by this operation.
*
*
* @param updateDataSetRequest
* @return Result of the UpdateDataSet operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDataSet
* @see AWS API
* Documentation
*/
UpdateDataSetResult updateDataSet(UpdateDataSetRequest updateDataSetRequest);
/**
*
* Updates the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param updateDataSetPermissionsRequest
* @return Result of the UpdateDataSetPermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDataSetPermissions
* @see AWS API Documentation
*/
UpdateDataSetPermissionsResult updateDataSetPermissions(UpdateDataSetPermissionsRequest updateDataSetPermissionsRequest);
/**
*
* Updates a data source.
*
*
* @param updateDataSourceRequest
* @return Result of the UpdateDataSource operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDataSource
* @see AWS
* API Documentation
*/
UpdateDataSourceResult updateDataSource(UpdateDataSourceRequest updateDataSourceRequest);
/**
*
* Updates the permissions to a data source.
*
*
* @param updateDataSourcePermissionsRequest
* @return Result of the UpdateDataSourcePermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateDataSourcePermissions
* @see AWS API Documentation
*/
UpdateDataSourcePermissionsResult updateDataSourcePermissions(UpdateDataSourcePermissionsRequest updateDataSourcePermissionsRequest);
/**
*
* Updates the name of a folder.
*
*
* @param updateFolderRequest
* @return Result of the UpdateFolder operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateFolder
* @see AWS API
* Documentation
*/
UpdateFolderResult updateFolder(UpdateFolderRequest updateFolderRequest);
/**
*
* Updates permissions of a folder.
*
*
* @param updateFolderPermissionsRequest
* @return Result of the UpdateFolderPermissions operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateFolderPermissions
* @see AWS API Documentation
*/
UpdateFolderPermissionsResult updateFolderPermissions(UpdateFolderPermissionsRequest updateFolderPermissionsRequest);
/**
*
* Changes a group description.
*
*
* @param updateGroupRequest
* @return Result of the UpdateGroup operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.UpdateGroup
* @see AWS API
* Documentation
*/
UpdateGroupResult updateGroup(UpdateGroupRequest updateGroupRequest);
/**
*
* Updates an existing IAM policy assignment. This operation updates only the optional parameter or parameters that
* are specified in the request. This overwrites all of the users included in Identities
.
*
*
* @param updateIAMPolicyAssignmentRequest
* @return Result of the UpdateIAMPolicyAssignment operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConcurrentUpdatingException
* A resource is already in a state that indicates an operation is happening that must complete before a new
* update can be applied.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateIAMPolicyAssignment
* @see AWS API Documentation
*/
UpdateIAMPolicyAssignmentResult updateIAMPolicyAssignment(UpdateIAMPolicyAssignmentRequest updateIAMPolicyAssignmentRequest);
/**
*
* Updates the content and status of IP rules. To use this operation, you need to provide the entire map of rules.
* You can use the DescribeIpRestriction
operation to get the current rule map.
*
*
* @param updateIpRestrictionRequest
* @return Result of the UpdateIpRestriction operation returned by the service.
* @throws LimitExceededException
* A limit is exceeded.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateIpRestriction
* @see AWS
* API Documentation
*/
UpdateIpRestrictionResult updateIpRestriction(UpdateIpRestrictionRequest updateIpRestrictionRequest);
/**
*
* Use the UpdatePublicSharingSettings
operation to turn on or turn off the public sharing settings of
* an Amazon QuickSight dashboard.
*
*
* To use this operation, turn on session capacity pricing for your Amazon QuickSight account.
*
*
* Before you can turn on public sharing on your account, make sure to give public sharing permissions to an
* administrative user in the Identity and Access Management (IAM) console. For more information on using IAM with
* Amazon QuickSight, see Using Amazon
* QuickSight with IAM in the Amazon QuickSight User Guide.
*
*
* @param updatePublicSharingSettingsRequest
* @return Result of the UpdatePublicSharingSettings operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedPricingPlanException
* This error indicates that you are calling an embedding operation in Amazon QuickSight without the
* required pricing plan on your Amazon Web Services account. Before you can use embedding for anonymous
* users, a QuickSight administrator needs to add capacity pricing to Amazon QuickSight. You can do this on
* the Manage Amazon QuickSight page.
*
* After capacity pricing is added, you can use the
* GetDashboardEmbedUrl
* API operation with the --identity-type ANONYMOUS
option.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdatePublicSharingSettings
* @see AWS API Documentation
*/
UpdatePublicSharingSettingsResult updatePublicSharingSettings(UpdatePublicSharingSettingsRequest updatePublicSharingSettingsRequest);
/**
*
* Updates a template from an existing Amazon QuickSight analysis or another template.
*
*
* @param updateTemplateRequest
* @return Result of the UpdateTemplate operation returned by the service.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws LimitExceededException
* A limit is exceeded.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateTemplate
* @see AWS API
* Documentation
*/
UpdateTemplateResult updateTemplate(UpdateTemplateRequest updateTemplateRequest);
/**
*
* Updates the template alias of a template.
*
*
* @param updateTemplateAliasRequest
* @return Result of the UpdateTemplateAlias operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateTemplateAlias
* @see AWS
* API Documentation
*/
UpdateTemplateAliasResult updateTemplateAlias(UpdateTemplateAliasRequest updateTemplateAliasRequest);
/**
*
* Updates the resource permissions for a template.
*
*
* @param updateTemplatePermissionsRequest
* @return Result of the UpdateTemplatePermissions operation returned by the service.
* @throws ThrottlingException
* Access is throttled.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateTemplatePermissions
* @see AWS API Documentation
*/
UpdateTemplatePermissionsResult updateTemplatePermissions(UpdateTemplatePermissionsRequest updateTemplatePermissionsRequest);
/**
*
* Updates a theme.
*
*
* @param updateThemeRequest
* @return Result of the UpdateTheme operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws LimitExceededException
* A limit is exceeded.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateTheme
* @see AWS API
* Documentation
*/
UpdateThemeResult updateTheme(UpdateThemeRequest updateThemeRequest);
/**
*
* Updates an alias of a theme.
*
*
* @param updateThemeAliasRequest
* @return Result of the UpdateThemeAlias operation returned by the service.
* @throws ConflictException
* Updating or deleting a resource can cause an inconsistent state.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceExistsException
* The resource specified already exists.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateThemeAlias
* @see AWS
* API Documentation
*/
UpdateThemeAliasResult updateThemeAlias(UpdateThemeAliasRequest updateThemeAliasRequest);
/**
*
* Updates the resource permissions for a theme. Permissions apply to the action to grant or revoke permissions on,
* for example "quicksight:DescribeTheme"
.
*
*
* Theme permissions apply in groupings. Valid groupings include the following for the three levels of permissions,
* which are user, owner, or no permissions:
*
*
* -
*
* User
*
*
* -
*
* "quicksight:DescribeTheme"
*
*
* -
*
* "quicksight:DescribeThemeAlias"
*
*
* -
*
* "quicksight:ListThemeAliases"
*
*
* -
*
* "quicksight:ListThemeVersions"
*
*
*
*
* -
*
* Owner
*
*
* -
*
* "quicksight:DescribeTheme"
*
*
* -
*
* "quicksight:DescribeThemeAlias"
*
*
* -
*
* "quicksight:ListThemeAliases"
*
*
* -
*
* "quicksight:ListThemeVersions"
*
*
* -
*
* "quicksight:DeleteTheme"
*
*
* -
*
* "quicksight:UpdateTheme"
*
*
* -
*
* "quicksight:CreateThemeAlias"
*
*
* -
*
* "quicksight:DeleteThemeAlias"
*
*
* -
*
* "quicksight:UpdateThemeAlias"
*
*
* -
*
* "quicksight:UpdateThemePermissions"
*
*
* -
*
* "quicksight:DescribeThemePermissions"
*
*
*
*
* -
*
* To specify no permissions, omit the permissions list.
*
*
*
*
* @param updateThemePermissionsRequest
* @return Result of the UpdateThemePermissions operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws UnsupportedUserEditionException
* This error indicates that you are calling an operation on an Amazon QuickSight subscription where the
* edition doesn't include support for that operation. Amazon Amazon QuickSight currently has Standard
* Edition and Enterprise Edition. Not every operation and capability is available in every edition.
* @throws LimitExceededException
* A limit is exceeded.
* @throws InternalFailureException
* An internal failure occurred.
* @sample AmazonQuickSight.UpdateThemePermissions
* @see AWS API Documentation
*/
UpdateThemePermissionsResult updateThemePermissions(UpdateThemePermissionsRequest updateThemePermissionsRequest);
/**
*
* Updates an Amazon QuickSight user.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws AccessDeniedException
* You don't have access to this item. The provided credentials couldn't be validated. You might not be
* authorized to carry out the request. Make sure that your account is authorized to use the Amazon
* QuickSight service, that your policies have the correct permissions, and that you are using the correct
* access keys.
* @throws InvalidParameterValueException
* One or more parameters has a value that isn't valid.
* @throws ResourceNotFoundException
* One or more resources can't be found.
* @throws ThrottlingException
* Access is throttled.
* @throws PreconditionNotMetException
* One or more preconditions aren't met.
* @throws InternalFailureException
* An internal failure occurred.
* @throws ResourceUnavailableException
* This resource is currently unavailable.
* @sample AmazonQuickSight.UpdateUser
* @see AWS API
* Documentation
*/
UpdateUserResult updateUser(UpdateUserRequest updateUserRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}