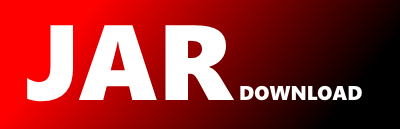
com.amazonaws.services.quicksight.model.DashboardPublishOptions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Dashboard publish options.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DashboardPublishOptions implements Serializable, Cloneable, StructuredPojo {
/**
*
* Ad hoc (one-time) filtering option.
*
*/
private AdHocFilteringOption adHocFilteringOption;
/**
*
* Export to .csv option.
*
*/
private ExportToCSVOption exportToCSVOption;
/**
*
* Sheet controls option.
*
*/
private SheetControlsOption sheetControlsOption;
/**
*
* The visual publish options of a visual in a dashboard.
*
*/
@Deprecated
private DashboardVisualPublishOptions visualPublishOptions;
/**
*
* The sheet layout maximization options of a dashbaord.
*
*/
private SheetLayoutElementMaximizationOption sheetLayoutElementMaximizationOption;
/**
*
* The menu options of a visual in a dashboard.
*
*/
private VisualMenuOption visualMenuOption;
/**
*
* The axis sort options of a dashboard.
*
*/
private VisualAxisSortOption visualAxisSortOption;
/**
*
* Determines if hidden fields are exported with a dashboard.
*
*/
private ExportWithHiddenFieldsOption exportWithHiddenFieldsOption;
/**
*
* The drill-down options of data points in a dashboard.
*
*/
private DataPointDrillUpDownOption dataPointDrillUpDownOption;
/**
*
* The data point menu label options of a dashboard.
*
*/
private DataPointMenuLabelOption dataPointMenuLabelOption;
/**
*
* The data point tool tip options of a dashboard.
*
*/
private DataPointTooltipOption dataPointTooltipOption;
/**
*
* Ad hoc (one-time) filtering option.
*
*
* @param adHocFilteringOption
* Ad hoc (one-time) filtering option.
*/
public void setAdHocFilteringOption(AdHocFilteringOption adHocFilteringOption) {
this.adHocFilteringOption = adHocFilteringOption;
}
/**
*
* Ad hoc (one-time) filtering option.
*
*
* @return Ad hoc (one-time) filtering option.
*/
public AdHocFilteringOption getAdHocFilteringOption() {
return this.adHocFilteringOption;
}
/**
*
* Ad hoc (one-time) filtering option.
*
*
* @param adHocFilteringOption
* Ad hoc (one-time) filtering option.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withAdHocFilteringOption(AdHocFilteringOption adHocFilteringOption) {
setAdHocFilteringOption(adHocFilteringOption);
return this;
}
/**
*
* Export to .csv option.
*
*
* @param exportToCSVOption
* Export to .csv option.
*/
public void setExportToCSVOption(ExportToCSVOption exportToCSVOption) {
this.exportToCSVOption = exportToCSVOption;
}
/**
*
* Export to .csv option.
*
*
* @return Export to .csv option.
*/
public ExportToCSVOption getExportToCSVOption() {
return this.exportToCSVOption;
}
/**
*
* Export to .csv option.
*
*
* @param exportToCSVOption
* Export to .csv option.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withExportToCSVOption(ExportToCSVOption exportToCSVOption) {
setExportToCSVOption(exportToCSVOption);
return this;
}
/**
*
* Sheet controls option.
*
*
* @param sheetControlsOption
* Sheet controls option.
*/
public void setSheetControlsOption(SheetControlsOption sheetControlsOption) {
this.sheetControlsOption = sheetControlsOption;
}
/**
*
* Sheet controls option.
*
*
* @return Sheet controls option.
*/
public SheetControlsOption getSheetControlsOption() {
return this.sheetControlsOption;
}
/**
*
* Sheet controls option.
*
*
* @param sheetControlsOption
* Sheet controls option.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withSheetControlsOption(SheetControlsOption sheetControlsOption) {
setSheetControlsOption(sheetControlsOption);
return this;
}
/**
*
* The visual publish options of a visual in a dashboard.
*
*
* @param visualPublishOptions
* The visual publish options of a visual in a dashboard.
*/
@Deprecated
public void setVisualPublishOptions(DashboardVisualPublishOptions visualPublishOptions) {
this.visualPublishOptions = visualPublishOptions;
}
/**
*
* The visual publish options of a visual in a dashboard.
*
*
* @return The visual publish options of a visual in a dashboard.
*/
@Deprecated
public DashboardVisualPublishOptions getVisualPublishOptions() {
return this.visualPublishOptions;
}
/**
*
* The visual publish options of a visual in a dashboard.
*
*
* @param visualPublishOptions
* The visual publish options of a visual in a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DashboardPublishOptions withVisualPublishOptions(DashboardVisualPublishOptions visualPublishOptions) {
setVisualPublishOptions(visualPublishOptions);
return this;
}
/**
*
* The sheet layout maximization options of a dashbaord.
*
*
* @param sheetLayoutElementMaximizationOption
* The sheet layout maximization options of a dashbaord.
*/
public void setSheetLayoutElementMaximizationOption(SheetLayoutElementMaximizationOption sheetLayoutElementMaximizationOption) {
this.sheetLayoutElementMaximizationOption = sheetLayoutElementMaximizationOption;
}
/**
*
* The sheet layout maximization options of a dashbaord.
*
*
* @return The sheet layout maximization options of a dashbaord.
*/
public SheetLayoutElementMaximizationOption getSheetLayoutElementMaximizationOption() {
return this.sheetLayoutElementMaximizationOption;
}
/**
*
* The sheet layout maximization options of a dashbaord.
*
*
* @param sheetLayoutElementMaximizationOption
* The sheet layout maximization options of a dashbaord.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withSheetLayoutElementMaximizationOption(SheetLayoutElementMaximizationOption sheetLayoutElementMaximizationOption) {
setSheetLayoutElementMaximizationOption(sheetLayoutElementMaximizationOption);
return this;
}
/**
*
* The menu options of a visual in a dashboard.
*
*
* @param visualMenuOption
* The menu options of a visual in a dashboard.
*/
public void setVisualMenuOption(VisualMenuOption visualMenuOption) {
this.visualMenuOption = visualMenuOption;
}
/**
*
* The menu options of a visual in a dashboard.
*
*
* @return The menu options of a visual in a dashboard.
*/
public VisualMenuOption getVisualMenuOption() {
return this.visualMenuOption;
}
/**
*
* The menu options of a visual in a dashboard.
*
*
* @param visualMenuOption
* The menu options of a visual in a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withVisualMenuOption(VisualMenuOption visualMenuOption) {
setVisualMenuOption(visualMenuOption);
return this;
}
/**
*
* The axis sort options of a dashboard.
*
*
* @param visualAxisSortOption
* The axis sort options of a dashboard.
*/
public void setVisualAxisSortOption(VisualAxisSortOption visualAxisSortOption) {
this.visualAxisSortOption = visualAxisSortOption;
}
/**
*
* The axis sort options of a dashboard.
*
*
* @return The axis sort options of a dashboard.
*/
public VisualAxisSortOption getVisualAxisSortOption() {
return this.visualAxisSortOption;
}
/**
*
* The axis sort options of a dashboard.
*
*
* @param visualAxisSortOption
* The axis sort options of a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withVisualAxisSortOption(VisualAxisSortOption visualAxisSortOption) {
setVisualAxisSortOption(visualAxisSortOption);
return this;
}
/**
*
* Determines if hidden fields are exported with a dashboard.
*
*
* @param exportWithHiddenFieldsOption
* Determines if hidden fields are exported with a dashboard.
*/
public void setExportWithHiddenFieldsOption(ExportWithHiddenFieldsOption exportWithHiddenFieldsOption) {
this.exportWithHiddenFieldsOption = exportWithHiddenFieldsOption;
}
/**
*
* Determines if hidden fields are exported with a dashboard.
*
*
* @return Determines if hidden fields are exported with a dashboard.
*/
public ExportWithHiddenFieldsOption getExportWithHiddenFieldsOption() {
return this.exportWithHiddenFieldsOption;
}
/**
*
* Determines if hidden fields are exported with a dashboard.
*
*
* @param exportWithHiddenFieldsOption
* Determines if hidden fields are exported with a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withExportWithHiddenFieldsOption(ExportWithHiddenFieldsOption exportWithHiddenFieldsOption) {
setExportWithHiddenFieldsOption(exportWithHiddenFieldsOption);
return this;
}
/**
*
* The drill-down options of data points in a dashboard.
*
*
* @param dataPointDrillUpDownOption
* The drill-down options of data points in a dashboard.
*/
public void setDataPointDrillUpDownOption(DataPointDrillUpDownOption dataPointDrillUpDownOption) {
this.dataPointDrillUpDownOption = dataPointDrillUpDownOption;
}
/**
*
* The drill-down options of data points in a dashboard.
*
*
* @return The drill-down options of data points in a dashboard.
*/
public DataPointDrillUpDownOption getDataPointDrillUpDownOption() {
return this.dataPointDrillUpDownOption;
}
/**
*
* The drill-down options of data points in a dashboard.
*
*
* @param dataPointDrillUpDownOption
* The drill-down options of data points in a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withDataPointDrillUpDownOption(DataPointDrillUpDownOption dataPointDrillUpDownOption) {
setDataPointDrillUpDownOption(dataPointDrillUpDownOption);
return this;
}
/**
*
* The data point menu label options of a dashboard.
*
*
* @param dataPointMenuLabelOption
* The data point menu label options of a dashboard.
*/
public void setDataPointMenuLabelOption(DataPointMenuLabelOption dataPointMenuLabelOption) {
this.dataPointMenuLabelOption = dataPointMenuLabelOption;
}
/**
*
* The data point menu label options of a dashboard.
*
*
* @return The data point menu label options of a dashboard.
*/
public DataPointMenuLabelOption getDataPointMenuLabelOption() {
return this.dataPointMenuLabelOption;
}
/**
*
* The data point menu label options of a dashboard.
*
*
* @param dataPointMenuLabelOption
* The data point menu label options of a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withDataPointMenuLabelOption(DataPointMenuLabelOption dataPointMenuLabelOption) {
setDataPointMenuLabelOption(dataPointMenuLabelOption);
return this;
}
/**
*
* The data point tool tip options of a dashboard.
*
*
* @param dataPointTooltipOption
* The data point tool tip options of a dashboard.
*/
public void setDataPointTooltipOption(DataPointTooltipOption dataPointTooltipOption) {
this.dataPointTooltipOption = dataPointTooltipOption;
}
/**
*
* The data point tool tip options of a dashboard.
*
*
* @return The data point tool tip options of a dashboard.
*/
public DataPointTooltipOption getDataPointTooltipOption() {
return this.dataPointTooltipOption;
}
/**
*
* The data point tool tip options of a dashboard.
*
*
* @param dataPointTooltipOption
* The data point tool tip options of a dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DashboardPublishOptions withDataPointTooltipOption(DataPointTooltipOption dataPointTooltipOption) {
setDataPointTooltipOption(dataPointTooltipOption);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdHocFilteringOption() != null)
sb.append("AdHocFilteringOption: ").append(getAdHocFilteringOption()).append(",");
if (getExportToCSVOption() != null)
sb.append("ExportToCSVOption: ").append(getExportToCSVOption()).append(",");
if (getSheetControlsOption() != null)
sb.append("SheetControlsOption: ").append(getSheetControlsOption()).append(",");
if (getVisualPublishOptions() != null)
sb.append("VisualPublishOptions: ").append(getVisualPublishOptions()).append(",");
if (getSheetLayoutElementMaximizationOption() != null)
sb.append("SheetLayoutElementMaximizationOption: ").append(getSheetLayoutElementMaximizationOption()).append(",");
if (getVisualMenuOption() != null)
sb.append("VisualMenuOption: ").append(getVisualMenuOption()).append(",");
if (getVisualAxisSortOption() != null)
sb.append("VisualAxisSortOption: ").append(getVisualAxisSortOption()).append(",");
if (getExportWithHiddenFieldsOption() != null)
sb.append("ExportWithHiddenFieldsOption: ").append(getExportWithHiddenFieldsOption()).append(",");
if (getDataPointDrillUpDownOption() != null)
sb.append("DataPointDrillUpDownOption: ").append(getDataPointDrillUpDownOption()).append(",");
if (getDataPointMenuLabelOption() != null)
sb.append("DataPointMenuLabelOption: ").append(getDataPointMenuLabelOption()).append(",");
if (getDataPointTooltipOption() != null)
sb.append("DataPointTooltipOption: ").append(getDataPointTooltipOption());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DashboardPublishOptions == false)
return false;
DashboardPublishOptions other = (DashboardPublishOptions) obj;
if (other.getAdHocFilteringOption() == null ^ this.getAdHocFilteringOption() == null)
return false;
if (other.getAdHocFilteringOption() != null && other.getAdHocFilteringOption().equals(this.getAdHocFilteringOption()) == false)
return false;
if (other.getExportToCSVOption() == null ^ this.getExportToCSVOption() == null)
return false;
if (other.getExportToCSVOption() != null && other.getExportToCSVOption().equals(this.getExportToCSVOption()) == false)
return false;
if (other.getSheetControlsOption() == null ^ this.getSheetControlsOption() == null)
return false;
if (other.getSheetControlsOption() != null && other.getSheetControlsOption().equals(this.getSheetControlsOption()) == false)
return false;
if (other.getVisualPublishOptions() == null ^ this.getVisualPublishOptions() == null)
return false;
if (other.getVisualPublishOptions() != null && other.getVisualPublishOptions().equals(this.getVisualPublishOptions()) == false)
return false;
if (other.getSheetLayoutElementMaximizationOption() == null ^ this.getSheetLayoutElementMaximizationOption() == null)
return false;
if (other.getSheetLayoutElementMaximizationOption() != null
&& other.getSheetLayoutElementMaximizationOption().equals(this.getSheetLayoutElementMaximizationOption()) == false)
return false;
if (other.getVisualMenuOption() == null ^ this.getVisualMenuOption() == null)
return false;
if (other.getVisualMenuOption() != null && other.getVisualMenuOption().equals(this.getVisualMenuOption()) == false)
return false;
if (other.getVisualAxisSortOption() == null ^ this.getVisualAxisSortOption() == null)
return false;
if (other.getVisualAxisSortOption() != null && other.getVisualAxisSortOption().equals(this.getVisualAxisSortOption()) == false)
return false;
if (other.getExportWithHiddenFieldsOption() == null ^ this.getExportWithHiddenFieldsOption() == null)
return false;
if (other.getExportWithHiddenFieldsOption() != null && other.getExportWithHiddenFieldsOption().equals(this.getExportWithHiddenFieldsOption()) == false)
return false;
if (other.getDataPointDrillUpDownOption() == null ^ this.getDataPointDrillUpDownOption() == null)
return false;
if (other.getDataPointDrillUpDownOption() != null && other.getDataPointDrillUpDownOption().equals(this.getDataPointDrillUpDownOption()) == false)
return false;
if (other.getDataPointMenuLabelOption() == null ^ this.getDataPointMenuLabelOption() == null)
return false;
if (other.getDataPointMenuLabelOption() != null && other.getDataPointMenuLabelOption().equals(this.getDataPointMenuLabelOption()) == false)
return false;
if (other.getDataPointTooltipOption() == null ^ this.getDataPointTooltipOption() == null)
return false;
if (other.getDataPointTooltipOption() != null && other.getDataPointTooltipOption().equals(this.getDataPointTooltipOption()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdHocFilteringOption() == null) ? 0 : getAdHocFilteringOption().hashCode());
hashCode = prime * hashCode + ((getExportToCSVOption() == null) ? 0 : getExportToCSVOption().hashCode());
hashCode = prime * hashCode + ((getSheetControlsOption() == null) ? 0 : getSheetControlsOption().hashCode());
hashCode = prime * hashCode + ((getVisualPublishOptions() == null) ? 0 : getVisualPublishOptions().hashCode());
hashCode = prime * hashCode + ((getSheetLayoutElementMaximizationOption() == null) ? 0 : getSheetLayoutElementMaximizationOption().hashCode());
hashCode = prime * hashCode + ((getVisualMenuOption() == null) ? 0 : getVisualMenuOption().hashCode());
hashCode = prime * hashCode + ((getVisualAxisSortOption() == null) ? 0 : getVisualAxisSortOption().hashCode());
hashCode = prime * hashCode + ((getExportWithHiddenFieldsOption() == null) ? 0 : getExportWithHiddenFieldsOption().hashCode());
hashCode = prime * hashCode + ((getDataPointDrillUpDownOption() == null) ? 0 : getDataPointDrillUpDownOption().hashCode());
hashCode = prime * hashCode + ((getDataPointMenuLabelOption() == null) ? 0 : getDataPointMenuLabelOption().hashCode());
hashCode = prime * hashCode + ((getDataPointTooltipOption() == null) ? 0 : getDataPointTooltipOption().hashCode());
return hashCode;
}
@Override
public DashboardPublishOptions clone() {
try {
return (DashboardPublishOptions) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.DashboardPublishOptionsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}