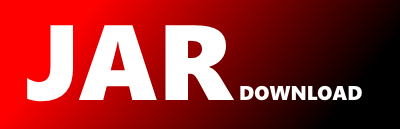
com.amazonaws.services.quicksight.model.DataSet Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Dataset.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataSet implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the resource.
*
*/
private String arn;
/**
*
* The ID of the dataset.
*
*/
private String dataSetId;
/**
*
* A display name for the dataset.
*
*/
private String name;
/**
*
* The time that this dataset was created.
*
*/
private java.util.Date createdTime;
/**
*
* The last time that this dataset was updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* Declares the physical tables that are available in the underlying data sources.
*
*/
private java.util.Map physicalTableMap;
/**
*
* Configures the combination and transformation of the data from the physical tables.
*
*/
private java.util.Map logicalTableMap;
/**
*
* The list of columns after all transforms. These columns are available in templates, analyses, and dashboards.
*
*/
private java.util.List outputColumns;
/**
*
* A value that indicates whether you want to import the data into SPICE.
*
*/
private String importMode;
/**
*
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*
*/
private Long consumedSpiceCapacityInBytes;
/**
*
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*
*/
private java.util.List columnGroups;
/**
*
* The folder that contains fields and nested subfolders for your dataset.
*
*/
private java.util.Map fieldFolders;
/**
*
* The row-level security configuration for the dataset.
*
*/
private RowLevelPermissionDataSet rowLevelPermissionDataSet;
/**
*
* The element you can use to define tags for row-level security.
*
*/
private RowLevelPermissionTagConfiguration rowLevelPermissionTagConfiguration;
/**
*
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*
*/
private java.util.List columnLevelPermissionRules;
/**
*
* The usage configuration to apply to child datasets that reference this dataset as a source.
*
*/
private DataSetUsageConfiguration dataSetUsageConfiguration;
/**
*
* The Amazon Resource Name (ARN) of the resource.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the resource.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the resource.
*
*
* @return The Amazon Resource Name (ARN) of the resource.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the resource.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The ID of the dataset.
*
*
* @param dataSetId
* The ID of the dataset.
*/
public void setDataSetId(String dataSetId) {
this.dataSetId = dataSetId;
}
/**
*
* The ID of the dataset.
*
*
* @return The ID of the dataset.
*/
public String getDataSetId() {
return this.dataSetId;
}
/**
*
* The ID of the dataset.
*
*
* @param dataSetId
* The ID of the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withDataSetId(String dataSetId) {
setDataSetId(dataSetId);
return this;
}
/**
*
* A display name for the dataset.
*
*
* @param name
* A display name for the dataset.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A display name for the dataset.
*
*
* @return A display name for the dataset.
*/
public String getName() {
return this.name;
}
/**
*
* A display name for the dataset.
*
*
* @param name
* A display name for the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withName(String name) {
setName(name);
return this;
}
/**
*
* The time that this dataset was created.
*
*
* @param createdTime
* The time that this dataset was created.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time that this dataset was created.
*
*
* @return The time that this dataset was created.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time that this dataset was created.
*
*
* @param createdTime
* The time that this dataset was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The last time that this dataset was updated.
*
*
* @param lastUpdatedTime
* The last time that this dataset was updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* The last time that this dataset was updated.
*
*
* @return The last time that this dataset was updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* The last time that this dataset was updated.
*
*
* @param lastUpdatedTime
* The last time that this dataset was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* Declares the physical tables that are available in the underlying data sources.
*
*
* @return Declares the physical tables that are available in the underlying data sources.
*/
public java.util.Map getPhysicalTableMap() {
return physicalTableMap;
}
/**
*
* Declares the physical tables that are available in the underlying data sources.
*
*
* @param physicalTableMap
* Declares the physical tables that are available in the underlying data sources.
*/
public void setPhysicalTableMap(java.util.Map physicalTableMap) {
this.physicalTableMap = physicalTableMap;
}
/**
*
* Declares the physical tables that are available in the underlying data sources.
*
*
* @param physicalTableMap
* Declares the physical tables that are available in the underlying data sources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withPhysicalTableMap(java.util.Map physicalTableMap) {
setPhysicalTableMap(physicalTableMap);
return this;
}
/**
* Add a single PhysicalTableMap entry
*
* @see DataSet#withPhysicalTableMap
* @returns a reference to this object so that method calls can be chained together.
*/
public DataSet addPhysicalTableMapEntry(String key, PhysicalTable value) {
if (null == this.physicalTableMap) {
this.physicalTableMap = new java.util.HashMap();
}
if (this.physicalTableMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.physicalTableMap.put(key, value);
return this;
}
/**
* Removes all the entries added into PhysicalTableMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet clearPhysicalTableMapEntries() {
this.physicalTableMap = null;
return this;
}
/**
*
* Configures the combination and transformation of the data from the physical tables.
*
*
* @return Configures the combination and transformation of the data from the physical tables.
*/
public java.util.Map getLogicalTableMap() {
return logicalTableMap;
}
/**
*
* Configures the combination and transformation of the data from the physical tables.
*
*
* @param logicalTableMap
* Configures the combination and transformation of the data from the physical tables.
*/
public void setLogicalTableMap(java.util.Map logicalTableMap) {
this.logicalTableMap = logicalTableMap;
}
/**
*
* Configures the combination and transformation of the data from the physical tables.
*
*
* @param logicalTableMap
* Configures the combination and transformation of the data from the physical tables.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withLogicalTableMap(java.util.Map logicalTableMap) {
setLogicalTableMap(logicalTableMap);
return this;
}
/**
* Add a single LogicalTableMap entry
*
* @see DataSet#withLogicalTableMap
* @returns a reference to this object so that method calls can be chained together.
*/
public DataSet addLogicalTableMapEntry(String key, LogicalTable value) {
if (null == this.logicalTableMap) {
this.logicalTableMap = new java.util.HashMap();
}
if (this.logicalTableMap.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.logicalTableMap.put(key, value);
return this;
}
/**
* Removes all the entries added into LogicalTableMap.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet clearLogicalTableMapEntries() {
this.logicalTableMap = null;
return this;
}
/**
*
* The list of columns after all transforms. These columns are available in templates, analyses, and dashboards.
*
*
* @return The list of columns after all transforms. These columns are available in templates, analyses, and
* dashboards.
*/
public java.util.List getOutputColumns() {
return outputColumns;
}
/**
*
* The list of columns after all transforms. These columns are available in templates, analyses, and dashboards.
*
*
* @param outputColumns
* The list of columns after all transforms. These columns are available in templates, analyses, and
* dashboards.
*/
public void setOutputColumns(java.util.Collection outputColumns) {
if (outputColumns == null) {
this.outputColumns = null;
return;
}
this.outputColumns = new java.util.ArrayList(outputColumns);
}
/**
*
* The list of columns after all transforms. These columns are available in templates, analyses, and dashboards.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOutputColumns(java.util.Collection)} or {@link #withOutputColumns(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param outputColumns
* The list of columns after all transforms. These columns are available in templates, analyses, and
* dashboards.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withOutputColumns(OutputColumn... outputColumns) {
if (this.outputColumns == null) {
setOutputColumns(new java.util.ArrayList(outputColumns.length));
}
for (OutputColumn ele : outputColumns) {
this.outputColumns.add(ele);
}
return this;
}
/**
*
* The list of columns after all transforms. These columns are available in templates, analyses, and dashboards.
*
*
* @param outputColumns
* The list of columns after all transforms. These columns are available in templates, analyses, and
* dashboards.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withOutputColumns(java.util.Collection outputColumns) {
setOutputColumns(outputColumns);
return this;
}
/**
*
* A value that indicates whether you want to import the data into SPICE.
*
*
* @param importMode
* A value that indicates whether you want to import the data into SPICE.
* @see DataSetImportMode
*/
public void setImportMode(String importMode) {
this.importMode = importMode;
}
/**
*
* A value that indicates whether you want to import the data into SPICE.
*
*
* @return A value that indicates whether you want to import the data into SPICE.
* @see DataSetImportMode
*/
public String getImportMode() {
return this.importMode;
}
/**
*
* A value that indicates whether you want to import the data into SPICE.
*
*
* @param importMode
* A value that indicates whether you want to import the data into SPICE.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSetImportMode
*/
public DataSet withImportMode(String importMode) {
setImportMode(importMode);
return this;
}
/**
*
* A value that indicates whether you want to import the data into SPICE.
*
*
* @param importMode
* A value that indicates whether you want to import the data into SPICE.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataSetImportMode
*/
public DataSet withImportMode(DataSetImportMode importMode) {
this.importMode = importMode.toString();
return this;
}
/**
*
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*
*
* @param consumedSpiceCapacityInBytes
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*/
public void setConsumedSpiceCapacityInBytes(Long consumedSpiceCapacityInBytes) {
this.consumedSpiceCapacityInBytes = consumedSpiceCapacityInBytes;
}
/**
*
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*
*
* @return The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*/
public Long getConsumedSpiceCapacityInBytes() {
return this.consumedSpiceCapacityInBytes;
}
/**
*
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
*
*
* @param consumedSpiceCapacityInBytes
* The amount of SPICE capacity used by this dataset. This is 0 if the dataset isn't imported into SPICE.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withConsumedSpiceCapacityInBytes(Long consumedSpiceCapacityInBytes) {
setConsumedSpiceCapacityInBytes(consumedSpiceCapacityInBytes);
return this;
}
/**
*
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*
*
* @return Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*/
public java.util.List getColumnGroups() {
return columnGroups;
}
/**
*
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*
*
* @param columnGroups
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*/
public void setColumnGroups(java.util.Collection columnGroups) {
if (columnGroups == null) {
this.columnGroups = null;
return;
}
this.columnGroups = new java.util.ArrayList(columnGroups);
}
/**
*
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setColumnGroups(java.util.Collection)} or {@link #withColumnGroups(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param columnGroups
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withColumnGroups(ColumnGroup... columnGroups) {
if (this.columnGroups == null) {
setColumnGroups(new java.util.ArrayList(columnGroups.length));
}
for (ColumnGroup ele : columnGroups) {
this.columnGroups.add(ele);
}
return this;
}
/**
*
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
*
*
* @param columnGroups
* Groupings of columns that work together in certain Amazon QuickSight features. Currently, only geospatial
* hierarchy is supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withColumnGroups(java.util.Collection columnGroups) {
setColumnGroups(columnGroups);
return this;
}
/**
*
* The folder that contains fields and nested subfolders for your dataset.
*
*
* @return The folder that contains fields and nested subfolders for your dataset.
*/
public java.util.Map getFieldFolders() {
return fieldFolders;
}
/**
*
* The folder that contains fields and nested subfolders for your dataset.
*
*
* @param fieldFolders
* The folder that contains fields and nested subfolders for your dataset.
*/
public void setFieldFolders(java.util.Map fieldFolders) {
this.fieldFolders = fieldFolders;
}
/**
*
* The folder that contains fields and nested subfolders for your dataset.
*
*
* @param fieldFolders
* The folder that contains fields and nested subfolders for your dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withFieldFolders(java.util.Map fieldFolders) {
setFieldFolders(fieldFolders);
return this;
}
/**
* Add a single FieldFolders entry
*
* @see DataSet#withFieldFolders
* @returns a reference to this object so that method calls can be chained together.
*/
public DataSet addFieldFoldersEntry(String key, FieldFolder value) {
if (null == this.fieldFolders) {
this.fieldFolders = new java.util.HashMap();
}
if (this.fieldFolders.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.fieldFolders.put(key, value);
return this;
}
/**
* Removes all the entries added into FieldFolders.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet clearFieldFoldersEntries() {
this.fieldFolders = null;
return this;
}
/**
*
* The row-level security configuration for the dataset.
*
*
* @param rowLevelPermissionDataSet
* The row-level security configuration for the dataset.
*/
public void setRowLevelPermissionDataSet(RowLevelPermissionDataSet rowLevelPermissionDataSet) {
this.rowLevelPermissionDataSet = rowLevelPermissionDataSet;
}
/**
*
* The row-level security configuration for the dataset.
*
*
* @return The row-level security configuration for the dataset.
*/
public RowLevelPermissionDataSet getRowLevelPermissionDataSet() {
return this.rowLevelPermissionDataSet;
}
/**
*
* The row-level security configuration for the dataset.
*
*
* @param rowLevelPermissionDataSet
* The row-level security configuration for the dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withRowLevelPermissionDataSet(RowLevelPermissionDataSet rowLevelPermissionDataSet) {
setRowLevelPermissionDataSet(rowLevelPermissionDataSet);
return this;
}
/**
*
* The element you can use to define tags for row-level security.
*
*
* @param rowLevelPermissionTagConfiguration
* The element you can use to define tags for row-level security.
*/
public void setRowLevelPermissionTagConfiguration(RowLevelPermissionTagConfiguration rowLevelPermissionTagConfiguration) {
this.rowLevelPermissionTagConfiguration = rowLevelPermissionTagConfiguration;
}
/**
*
* The element you can use to define tags for row-level security.
*
*
* @return The element you can use to define tags for row-level security.
*/
public RowLevelPermissionTagConfiguration getRowLevelPermissionTagConfiguration() {
return this.rowLevelPermissionTagConfiguration;
}
/**
*
* The element you can use to define tags for row-level security.
*
*
* @param rowLevelPermissionTagConfiguration
* The element you can use to define tags for row-level security.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withRowLevelPermissionTagConfiguration(RowLevelPermissionTagConfiguration rowLevelPermissionTagConfiguration) {
setRowLevelPermissionTagConfiguration(rowLevelPermissionTagConfiguration);
return this;
}
/**
*
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*
*
* @return A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*/
public java.util.List getColumnLevelPermissionRules() {
return columnLevelPermissionRules;
}
/**
*
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*
*
* @param columnLevelPermissionRules
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*/
public void setColumnLevelPermissionRules(java.util.Collection columnLevelPermissionRules) {
if (columnLevelPermissionRules == null) {
this.columnLevelPermissionRules = null;
return;
}
this.columnLevelPermissionRules = new java.util.ArrayList(columnLevelPermissionRules);
}
/**
*
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setColumnLevelPermissionRules(java.util.Collection)} or
* {@link #withColumnLevelPermissionRules(java.util.Collection)} if you want to override the existing values.
*
*
* @param columnLevelPermissionRules
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withColumnLevelPermissionRules(ColumnLevelPermissionRule... columnLevelPermissionRules) {
if (this.columnLevelPermissionRules == null) {
setColumnLevelPermissionRules(new java.util.ArrayList(columnLevelPermissionRules.length));
}
for (ColumnLevelPermissionRule ele : columnLevelPermissionRules) {
this.columnLevelPermissionRules.add(ele);
}
return this;
}
/**
*
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
*
*
* @param columnLevelPermissionRules
* A set of one or more definitions of a
* ColumnLevelPermissionRule
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withColumnLevelPermissionRules(java.util.Collection columnLevelPermissionRules) {
setColumnLevelPermissionRules(columnLevelPermissionRules);
return this;
}
/**
*
* The usage configuration to apply to child datasets that reference this dataset as a source.
*
*
* @param dataSetUsageConfiguration
* The usage configuration to apply to child datasets that reference this dataset as a source.
*/
public void setDataSetUsageConfiguration(DataSetUsageConfiguration dataSetUsageConfiguration) {
this.dataSetUsageConfiguration = dataSetUsageConfiguration;
}
/**
*
* The usage configuration to apply to child datasets that reference this dataset as a source.
*
*
* @return The usage configuration to apply to child datasets that reference this dataset as a source.
*/
public DataSetUsageConfiguration getDataSetUsageConfiguration() {
return this.dataSetUsageConfiguration;
}
/**
*
* The usage configuration to apply to child datasets that reference this dataset as a source.
*
*
* @param dataSetUsageConfiguration
* The usage configuration to apply to child datasets that reference this dataset as a source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataSet withDataSetUsageConfiguration(DataSetUsageConfiguration dataSetUsageConfiguration) {
setDataSetUsageConfiguration(dataSetUsageConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getDataSetId() != null)
sb.append("DataSetId: ").append(getDataSetId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getPhysicalTableMap() != null)
sb.append("PhysicalTableMap: ").append(getPhysicalTableMap()).append(",");
if (getLogicalTableMap() != null)
sb.append("LogicalTableMap: ").append(getLogicalTableMap()).append(",");
if (getOutputColumns() != null)
sb.append("OutputColumns: ").append(getOutputColumns()).append(",");
if (getImportMode() != null)
sb.append("ImportMode: ").append(getImportMode()).append(",");
if (getConsumedSpiceCapacityInBytes() != null)
sb.append("ConsumedSpiceCapacityInBytes: ").append(getConsumedSpiceCapacityInBytes()).append(",");
if (getColumnGroups() != null)
sb.append("ColumnGroups: ").append(getColumnGroups()).append(",");
if (getFieldFolders() != null)
sb.append("FieldFolders: ").append(getFieldFolders()).append(",");
if (getRowLevelPermissionDataSet() != null)
sb.append("RowLevelPermissionDataSet: ").append(getRowLevelPermissionDataSet()).append(",");
if (getRowLevelPermissionTagConfiguration() != null)
sb.append("RowLevelPermissionTagConfiguration: ").append(getRowLevelPermissionTagConfiguration()).append(",");
if (getColumnLevelPermissionRules() != null)
sb.append("ColumnLevelPermissionRules: ").append(getColumnLevelPermissionRules()).append(",");
if (getDataSetUsageConfiguration() != null)
sb.append("DataSetUsageConfiguration: ").append(getDataSetUsageConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataSet == false)
return false;
DataSet other = (DataSet) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getDataSetId() == null ^ this.getDataSetId() == null)
return false;
if (other.getDataSetId() != null && other.getDataSetId().equals(this.getDataSetId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getPhysicalTableMap() == null ^ this.getPhysicalTableMap() == null)
return false;
if (other.getPhysicalTableMap() != null && other.getPhysicalTableMap().equals(this.getPhysicalTableMap()) == false)
return false;
if (other.getLogicalTableMap() == null ^ this.getLogicalTableMap() == null)
return false;
if (other.getLogicalTableMap() != null && other.getLogicalTableMap().equals(this.getLogicalTableMap()) == false)
return false;
if (other.getOutputColumns() == null ^ this.getOutputColumns() == null)
return false;
if (other.getOutputColumns() != null && other.getOutputColumns().equals(this.getOutputColumns()) == false)
return false;
if (other.getImportMode() == null ^ this.getImportMode() == null)
return false;
if (other.getImportMode() != null && other.getImportMode().equals(this.getImportMode()) == false)
return false;
if (other.getConsumedSpiceCapacityInBytes() == null ^ this.getConsumedSpiceCapacityInBytes() == null)
return false;
if (other.getConsumedSpiceCapacityInBytes() != null && other.getConsumedSpiceCapacityInBytes().equals(this.getConsumedSpiceCapacityInBytes()) == false)
return false;
if (other.getColumnGroups() == null ^ this.getColumnGroups() == null)
return false;
if (other.getColumnGroups() != null && other.getColumnGroups().equals(this.getColumnGroups()) == false)
return false;
if (other.getFieldFolders() == null ^ this.getFieldFolders() == null)
return false;
if (other.getFieldFolders() != null && other.getFieldFolders().equals(this.getFieldFolders()) == false)
return false;
if (other.getRowLevelPermissionDataSet() == null ^ this.getRowLevelPermissionDataSet() == null)
return false;
if (other.getRowLevelPermissionDataSet() != null && other.getRowLevelPermissionDataSet().equals(this.getRowLevelPermissionDataSet()) == false)
return false;
if (other.getRowLevelPermissionTagConfiguration() == null ^ this.getRowLevelPermissionTagConfiguration() == null)
return false;
if (other.getRowLevelPermissionTagConfiguration() != null
&& other.getRowLevelPermissionTagConfiguration().equals(this.getRowLevelPermissionTagConfiguration()) == false)
return false;
if (other.getColumnLevelPermissionRules() == null ^ this.getColumnLevelPermissionRules() == null)
return false;
if (other.getColumnLevelPermissionRules() != null && other.getColumnLevelPermissionRules().equals(this.getColumnLevelPermissionRules()) == false)
return false;
if (other.getDataSetUsageConfiguration() == null ^ this.getDataSetUsageConfiguration() == null)
return false;
if (other.getDataSetUsageConfiguration() != null && other.getDataSetUsageConfiguration().equals(this.getDataSetUsageConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getDataSetId() == null) ? 0 : getDataSetId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getPhysicalTableMap() == null) ? 0 : getPhysicalTableMap().hashCode());
hashCode = prime * hashCode + ((getLogicalTableMap() == null) ? 0 : getLogicalTableMap().hashCode());
hashCode = prime * hashCode + ((getOutputColumns() == null) ? 0 : getOutputColumns().hashCode());
hashCode = prime * hashCode + ((getImportMode() == null) ? 0 : getImportMode().hashCode());
hashCode = prime * hashCode + ((getConsumedSpiceCapacityInBytes() == null) ? 0 : getConsumedSpiceCapacityInBytes().hashCode());
hashCode = prime * hashCode + ((getColumnGroups() == null) ? 0 : getColumnGroups().hashCode());
hashCode = prime * hashCode + ((getFieldFolders() == null) ? 0 : getFieldFolders().hashCode());
hashCode = prime * hashCode + ((getRowLevelPermissionDataSet() == null) ? 0 : getRowLevelPermissionDataSet().hashCode());
hashCode = prime * hashCode + ((getRowLevelPermissionTagConfiguration() == null) ? 0 : getRowLevelPermissionTagConfiguration().hashCode());
hashCode = prime * hashCode + ((getColumnLevelPermissionRules() == null) ? 0 : getColumnLevelPermissionRules().hashCode());
hashCode = prime * hashCode + ((getDataSetUsageConfiguration() == null) ? 0 : getDataSetUsageConfiguration().hashCode());
return hashCode;
}
@Override
public DataSet clone() {
try {
return (DataSet) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.DataSetMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}