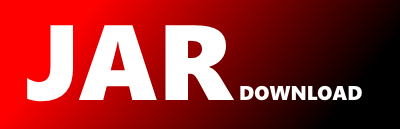
com.amazonaws.services.quicksight.model.GenerateEmbedUrlForAnonymousUserRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GenerateEmbedUrlForAnonymousUserRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*
*/
private String awsAccountId;
/**
*
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*
*/
private Long sessionLifetimeInMinutes;
/**
*
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an Amazon
* QuickSight custom namespace, set this to default
.
*
*/
private String namespace;
/**
*
* The session tags used for row-level security. Before you use this parameter, make sure that you have configured
* the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
parameter so that session
* tags can be used to provide row-level security.
*
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information, see Using Row-Level Security
* (RLS) with Tagsin the Amazon QuickSight User Guide.
*
*/
private java.util.List sessionTags;
/**
*
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access during
* the lifetime of the session. If you choose Dashboard
embedding experience, pass the list of
* dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass up to 25
* dashboard ARNs in each API call.
*
*/
private java.util.List authorizedResourceArns;
/**
*
* The configuration of the experience that you are embedding.
*
*/
private AnonymousUserEmbeddingExperienceConfiguration experienceConfiguration;
/**
*
* The domains that you want to add to the allow list for access to the generated URL that is then embedded. This
* optional parameter overrides the static domains that are configured in the Manage QuickSight menu in the Amazon
* QuickSight console. Instead, it allows only the domains that you include in this parameter. You can list up to
* three domains or subdomains in each API call.
*
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*
*/
private java.util.List allowedDomains;
/**
*
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*
*
* @param awsAccountId
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*
*
* @return The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
*
*
* @param awsAccountId
* The ID for the Amazon Web Services account that contains the dashboard that you're embedding.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*
*
* @param sessionLifetimeInMinutes
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*/
public void setSessionLifetimeInMinutes(Long sessionLifetimeInMinutes) {
this.sessionLifetimeInMinutes = sessionLifetimeInMinutes;
}
/**
*
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*
*
* @return How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*/
public Long getSessionLifetimeInMinutes() {
return this.sessionLifetimeInMinutes;
}
/**
*
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
*
*
* @param sessionLifetimeInMinutes
* How many minutes the session is valid. The session lifetime must be in [15-600] minutes range.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withSessionLifetimeInMinutes(Long sessionLifetimeInMinutes) {
setSessionLifetimeInMinutes(sessionLifetimeInMinutes);
return this;
}
/**
*
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an Amazon
* QuickSight custom namespace, set this to default
.
*
*
* @param namespace
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an
* Amazon QuickSight custom namespace, set this to default
.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
*
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an Amazon
* QuickSight custom namespace, set this to default
.
*
*
* @return The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an
* Amazon QuickSight custom namespace, set this to default
.
*/
public String getNamespace() {
return this.namespace;
}
/**
*
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an Amazon
* QuickSight custom namespace, set this to default
.
*
*
* @param namespace
* The Amazon QuickSight namespace that the anonymous user virtually belongs to. If you are not using an
* Amazon QuickSight custom namespace, set this to default
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withNamespace(String namespace) {
setNamespace(namespace);
return this;
}
/**
*
* The session tags used for row-level security. Before you use this parameter, make sure that you have configured
* the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
parameter so that session
* tags can be used to provide row-level security.
*
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information, see Using Row-Level Security
* (RLS) with Tagsin the Amazon QuickSight User Guide.
*
*
* @return The session tags used for row-level security. Before you use this parameter, make sure that you have
* configured the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
* parameter so that session tags can be used to provide row-level security.
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information,
* see Using
* Row-Level Security (RLS) with Tagsin the Amazon QuickSight User Guide.
*/
public java.util.List getSessionTags() {
return sessionTags;
}
/**
*
* The session tags used for row-level security. Before you use this parameter, make sure that you have configured
* the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
parameter so that session
* tags can be used to provide row-level security.
*
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information, see Using Row-Level Security
* (RLS) with Tagsin the Amazon QuickSight User Guide.
*
*
* @param sessionTags
* The session tags used for row-level security. Before you use this parameter, make sure that you have
* configured the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
* parameter so that session tags can be used to provide row-level security.
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information,
* see Using
* Row-Level Security (RLS) with Tagsin the Amazon QuickSight User Guide.
*/
public void setSessionTags(java.util.Collection sessionTags) {
if (sessionTags == null) {
this.sessionTags = null;
return;
}
this.sessionTags = new java.util.ArrayList(sessionTags);
}
/**
*
* The session tags used for row-level security. Before you use this parameter, make sure that you have configured
* the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
parameter so that session
* tags can be used to provide row-level security.
*
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information, see Using Row-Level Security
* (RLS) with Tagsin the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSessionTags(java.util.Collection)} or {@link #withSessionTags(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param sessionTags
* The session tags used for row-level security. Before you use this parameter, make sure that you have
* configured the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
* parameter so that session tags can be used to provide row-level security.
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information,
* see Using
* Row-Level Security (RLS) with Tagsin the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withSessionTags(SessionTag... sessionTags) {
if (this.sessionTags == null) {
setSessionTags(new java.util.ArrayList(sessionTags.length));
}
for (SessionTag ele : sessionTags) {
this.sessionTags.add(ele);
}
return this;
}
/**
*
* The session tags used for row-level security. Before you use this parameter, make sure that you have configured
* the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
parameter so that session
* tags can be used to provide row-level security.
*
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information, see Using Row-Level Security
* (RLS) with Tagsin the Amazon QuickSight User Guide.
*
*
* @param sessionTags
* The session tags used for row-level security. Before you use this parameter, make sure that you have
* configured the relevant datasets using the DataSet$RowLevelPermissionTagConfiguration
* parameter so that session tags can be used to provide row-level security.
*
* These are not the tags used for the Amazon Web Services resource tagging feature. For more information,
* see Using
* Row-Level Security (RLS) with Tagsin the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withSessionTags(java.util.Collection sessionTags) {
setSessionTags(sessionTags);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access during
* the lifetime of the session. If you choose Dashboard
embedding experience, pass the list of
* dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass up to 25
* dashboard ARNs in each API call.
*
*
* @return The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to
* access during the lifetime of the session. If you choose Dashboard
embedding experience,
* pass the list of dashboard ARNs in the account that you want the user to be able to view. Currently, you
* can pass up to 25 dashboard ARNs in each API call.
*/
public java.util.List getAuthorizedResourceArns() {
return authorizedResourceArns;
}
/**
*
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access during
* the lifetime of the session. If you choose Dashboard
embedding experience, pass the list of
* dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass up to 25
* dashboard ARNs in each API call.
*
*
* @param authorizedResourceArns
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access
* during the lifetime of the session. If you choose Dashboard
embedding experience, pass the
* list of dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass
* up to 25 dashboard ARNs in each API call.
*/
public void setAuthorizedResourceArns(java.util.Collection authorizedResourceArns) {
if (authorizedResourceArns == null) {
this.authorizedResourceArns = null;
return;
}
this.authorizedResourceArns = new java.util.ArrayList(authorizedResourceArns);
}
/**
*
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access during
* the lifetime of the session. If you choose Dashboard
embedding experience, pass the list of
* dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass up to 25
* dashboard ARNs in each API call.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAuthorizedResourceArns(java.util.Collection)} or
* {@link #withAuthorizedResourceArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param authorizedResourceArns
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access
* during the lifetime of the session. If you choose Dashboard
embedding experience, pass the
* list of dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass
* up to 25 dashboard ARNs in each API call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withAuthorizedResourceArns(String... authorizedResourceArns) {
if (this.authorizedResourceArns == null) {
setAuthorizedResourceArns(new java.util.ArrayList(authorizedResourceArns.length));
}
for (String ele : authorizedResourceArns) {
this.authorizedResourceArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access during
* the lifetime of the session. If you choose Dashboard
embedding experience, pass the list of
* dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass up to 25
* dashboard ARNs in each API call.
*
*
* @param authorizedResourceArns
* The Amazon Resource Names (ARNs) for the Amazon QuickSight resources that the user is authorized to access
* during the lifetime of the session. If you choose Dashboard
embedding experience, pass the
* list of dashboard ARNs in the account that you want the user to be able to view. Currently, you can pass
* up to 25 dashboard ARNs in each API call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withAuthorizedResourceArns(java.util.Collection authorizedResourceArns) {
setAuthorizedResourceArns(authorizedResourceArns);
return this;
}
/**
*
* The configuration of the experience that you are embedding.
*
*
* @param experienceConfiguration
* The configuration of the experience that you are embedding.
*/
public void setExperienceConfiguration(AnonymousUserEmbeddingExperienceConfiguration experienceConfiguration) {
this.experienceConfiguration = experienceConfiguration;
}
/**
*
* The configuration of the experience that you are embedding.
*
*
* @return The configuration of the experience that you are embedding.
*/
public AnonymousUserEmbeddingExperienceConfiguration getExperienceConfiguration() {
return this.experienceConfiguration;
}
/**
*
* The configuration of the experience that you are embedding.
*
*
* @param experienceConfiguration
* The configuration of the experience that you are embedding.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withExperienceConfiguration(AnonymousUserEmbeddingExperienceConfiguration experienceConfiguration) {
setExperienceConfiguration(experienceConfiguration);
return this;
}
/**
*
* The domains that you want to add to the allow list for access to the generated URL that is then embedded. This
* optional parameter overrides the static domains that are configured in the Manage QuickSight menu in the Amazon
* QuickSight console. Instead, it allows only the domains that you include in this parameter. You can list up to
* three domains or subdomains in each API call.
*
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*
*
* @return The domains that you want to add to the allow list for access to the generated URL that is then embedded.
* This optional parameter overrides the static domains that are configured in the Manage QuickSight menu in
* the Amazon QuickSight console. Instead, it allows only the domains that you include in this parameter.
* You can list up to three domains or subdomains in each API call.
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*/
public java.util.List getAllowedDomains() {
return allowedDomains;
}
/**
*
* The domains that you want to add to the allow list for access to the generated URL that is then embedded. This
* optional parameter overrides the static domains that are configured in the Manage QuickSight menu in the Amazon
* QuickSight console. Instead, it allows only the domains that you include in this parameter. You can list up to
* three domains or subdomains in each API call.
*
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*
*
* @param allowedDomains
* The domains that you want to add to the allow list for access to the generated URL that is then embedded.
* This optional parameter overrides the static domains that are configured in the Manage QuickSight menu in
* the Amazon QuickSight console. Instead, it allows only the domains that you include in this parameter. You
* can list up to three domains or subdomains in each API call.
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*/
public void setAllowedDomains(java.util.Collection allowedDomains) {
if (allowedDomains == null) {
this.allowedDomains = null;
return;
}
this.allowedDomains = new java.util.ArrayList(allowedDomains);
}
/**
*
* The domains that you want to add to the allow list for access to the generated URL that is then embedded. This
* optional parameter overrides the static domains that are configured in the Manage QuickSight menu in the Amazon
* QuickSight console. Instead, it allows only the domains that you include in this parameter. You can list up to
* three domains or subdomains in each API call.
*
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowedDomains(java.util.Collection)} or {@link #withAllowedDomains(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param allowedDomains
* The domains that you want to add to the allow list for access to the generated URL that is then embedded.
* This optional parameter overrides the static domains that are configured in the Manage QuickSight menu in
* the Amazon QuickSight console. Instead, it allows only the domains that you include in this parameter. You
* can list up to three domains or subdomains in each API call.
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withAllowedDomains(String... allowedDomains) {
if (this.allowedDomains == null) {
setAllowedDomains(new java.util.ArrayList(allowedDomains.length));
}
for (String ele : allowedDomains) {
this.allowedDomains.add(ele);
}
return this;
}
/**
*
* The domains that you want to add to the allow list for access to the generated URL that is then embedded. This
* optional parameter overrides the static domains that are configured in the Manage QuickSight menu in the Amazon
* QuickSight console. Instead, it allows only the domains that you include in this parameter. You can list up to
* three domains or subdomains in each API call.
*
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
*
*
* @param allowedDomains
* The domains that you want to add to the allow list for access to the generated URL that is then embedded.
* This optional parameter overrides the static domains that are configured in the Manage QuickSight menu in
* the Amazon QuickSight console. Instead, it allows only the domains that you include in this parameter. You
* can list up to three domains or subdomains in each API call.
*
* To include all subdomains under a specific domain to the allow list, use *
. For example,
* https://*.sapp.amazon.com
includes all subdomains under https://sapp.amazon.com
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GenerateEmbedUrlForAnonymousUserRequest withAllowedDomains(java.util.Collection allowedDomains) {
setAllowedDomains(allowedDomains);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getSessionLifetimeInMinutes() != null)
sb.append("SessionLifetimeInMinutes: ").append(getSessionLifetimeInMinutes()).append(",");
if (getNamespace() != null)
sb.append("Namespace: ").append(getNamespace()).append(",");
if (getSessionTags() != null)
sb.append("SessionTags: ").append(getSessionTags()).append(",");
if (getAuthorizedResourceArns() != null)
sb.append("AuthorizedResourceArns: ").append(getAuthorizedResourceArns()).append(",");
if (getExperienceConfiguration() != null)
sb.append("ExperienceConfiguration: ").append(getExperienceConfiguration()).append(",");
if (getAllowedDomains() != null)
sb.append("AllowedDomains: ").append(getAllowedDomains());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GenerateEmbedUrlForAnonymousUserRequest == false)
return false;
GenerateEmbedUrlForAnonymousUserRequest other = (GenerateEmbedUrlForAnonymousUserRequest) obj;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getSessionLifetimeInMinutes() == null ^ this.getSessionLifetimeInMinutes() == null)
return false;
if (other.getSessionLifetimeInMinutes() != null && other.getSessionLifetimeInMinutes().equals(this.getSessionLifetimeInMinutes()) == false)
return false;
if (other.getNamespace() == null ^ this.getNamespace() == null)
return false;
if (other.getNamespace() != null && other.getNamespace().equals(this.getNamespace()) == false)
return false;
if (other.getSessionTags() == null ^ this.getSessionTags() == null)
return false;
if (other.getSessionTags() != null && other.getSessionTags().equals(this.getSessionTags()) == false)
return false;
if (other.getAuthorizedResourceArns() == null ^ this.getAuthorizedResourceArns() == null)
return false;
if (other.getAuthorizedResourceArns() != null && other.getAuthorizedResourceArns().equals(this.getAuthorizedResourceArns()) == false)
return false;
if (other.getExperienceConfiguration() == null ^ this.getExperienceConfiguration() == null)
return false;
if (other.getExperienceConfiguration() != null && other.getExperienceConfiguration().equals(this.getExperienceConfiguration()) == false)
return false;
if (other.getAllowedDomains() == null ^ this.getAllowedDomains() == null)
return false;
if (other.getAllowedDomains() != null && other.getAllowedDomains().equals(this.getAllowedDomains()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getSessionLifetimeInMinutes() == null) ? 0 : getSessionLifetimeInMinutes().hashCode());
hashCode = prime * hashCode + ((getNamespace() == null) ? 0 : getNamespace().hashCode());
hashCode = prime * hashCode + ((getSessionTags() == null) ? 0 : getSessionTags().hashCode());
hashCode = prime * hashCode + ((getAuthorizedResourceArns() == null) ? 0 : getAuthorizedResourceArns().hashCode());
hashCode = prime * hashCode + ((getExperienceConfiguration() == null) ? 0 : getExperienceConfiguration().hashCode());
hashCode = prime * hashCode + ((getAllowedDomains() == null) ? 0 : getAllowedDomains().hashCode());
return hashCode;
}
@Override
public GenerateEmbedUrlForAnonymousUserRequest clone() {
return (GenerateEmbedUrlForAnonymousUserRequest) super.clone();
}
}