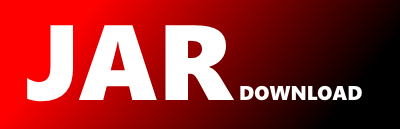
com.amazonaws.services.quicksight.model.RadarChartConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration of a RadarChartVisual
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RadarChartConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The field well configuration of a RadarChartVisual
.
*
*/
private RadarChartFieldWells fieldWells;
/**
*
* The sort configuration of a RadarChartVisual
.
*
*/
private RadarChartSortConfiguration sortConfiguration;
/**
*
* The shape of the radar chart.
*
*/
private String shape;
/**
*
* The base sreies settings of a radar chart.
*
*/
private RadarChartSeriesSettings baseSeriesSettings;
/**
*
* The start angle of a radar chart's axis.
*
*/
private Double startAngle;
/**
*
* The palette (chart color) display setup of the visual.
*
*/
private VisualPalette visualPalette;
/**
*
* Determines the visibility of the colors of alternatign bands in a radar chart.
*
*/
private String alternateBandColorsVisibility;
/**
*
* The color of the even-numbered alternate bands of a radar chart.
*
*/
private String alternateBandEvenColor;
/**
*
* The color of the odd-numbered alternate bands of a radar chart.
*
*/
private String alternateBandOddColor;
/**
*
* The category axis of a radar chart.
*
*/
private AxisDisplayOptions categoryAxis;
/**
*
* The category label options of a radar chart.
*
*/
private ChartAxisLabelOptions categoryLabelOptions;
/**
*
* The color axis of a radar chart.
*
*/
private AxisDisplayOptions colorAxis;
/**
*
* The color label options of a radar chart.
*
*/
private ChartAxisLabelOptions colorLabelOptions;
/**
*
* The legend display setup of the visual.
*
*/
private LegendOptions legend;
/**
*
* The field well configuration of a RadarChartVisual
.
*
*
* @param fieldWells
* The field well configuration of a RadarChartVisual
.
*/
public void setFieldWells(RadarChartFieldWells fieldWells) {
this.fieldWells = fieldWells;
}
/**
*
* The field well configuration of a RadarChartVisual
.
*
*
* @return The field well configuration of a RadarChartVisual
.
*/
public RadarChartFieldWells getFieldWells() {
return this.fieldWells;
}
/**
*
* The field well configuration of a RadarChartVisual
.
*
*
* @param fieldWells
* The field well configuration of a RadarChartVisual
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withFieldWells(RadarChartFieldWells fieldWells) {
setFieldWells(fieldWells);
return this;
}
/**
*
* The sort configuration of a RadarChartVisual
.
*
*
* @param sortConfiguration
* The sort configuration of a RadarChartVisual
.
*/
public void setSortConfiguration(RadarChartSortConfiguration sortConfiguration) {
this.sortConfiguration = sortConfiguration;
}
/**
*
* The sort configuration of a RadarChartVisual
.
*
*
* @return The sort configuration of a RadarChartVisual
.
*/
public RadarChartSortConfiguration getSortConfiguration() {
return this.sortConfiguration;
}
/**
*
* The sort configuration of a RadarChartVisual
.
*
*
* @param sortConfiguration
* The sort configuration of a RadarChartVisual
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withSortConfiguration(RadarChartSortConfiguration sortConfiguration) {
setSortConfiguration(sortConfiguration);
return this;
}
/**
*
* The shape of the radar chart.
*
*
* @param shape
* The shape of the radar chart.
* @see RadarChartShape
*/
public void setShape(String shape) {
this.shape = shape;
}
/**
*
* The shape of the radar chart.
*
*
* @return The shape of the radar chart.
* @see RadarChartShape
*/
public String getShape() {
return this.shape;
}
/**
*
* The shape of the radar chart.
*
*
* @param shape
* The shape of the radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RadarChartShape
*/
public RadarChartConfiguration withShape(String shape) {
setShape(shape);
return this;
}
/**
*
* The shape of the radar chart.
*
*
* @param shape
* The shape of the radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RadarChartShape
*/
public RadarChartConfiguration withShape(RadarChartShape shape) {
this.shape = shape.toString();
return this;
}
/**
*
* The base sreies settings of a radar chart.
*
*
* @param baseSeriesSettings
* The base sreies settings of a radar chart.
*/
public void setBaseSeriesSettings(RadarChartSeriesSettings baseSeriesSettings) {
this.baseSeriesSettings = baseSeriesSettings;
}
/**
*
* The base sreies settings of a radar chart.
*
*
* @return The base sreies settings of a radar chart.
*/
public RadarChartSeriesSettings getBaseSeriesSettings() {
return this.baseSeriesSettings;
}
/**
*
* The base sreies settings of a radar chart.
*
*
* @param baseSeriesSettings
* The base sreies settings of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withBaseSeriesSettings(RadarChartSeriesSettings baseSeriesSettings) {
setBaseSeriesSettings(baseSeriesSettings);
return this;
}
/**
*
* The start angle of a radar chart's axis.
*
*
* @param startAngle
* The start angle of a radar chart's axis.
*/
public void setStartAngle(Double startAngle) {
this.startAngle = startAngle;
}
/**
*
* The start angle of a radar chart's axis.
*
*
* @return The start angle of a radar chart's axis.
*/
public Double getStartAngle() {
return this.startAngle;
}
/**
*
* The start angle of a radar chart's axis.
*
*
* @param startAngle
* The start angle of a radar chart's axis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withStartAngle(Double startAngle) {
setStartAngle(startAngle);
return this;
}
/**
*
* The palette (chart color) display setup of the visual.
*
*
* @param visualPalette
* The palette (chart color) display setup of the visual.
*/
public void setVisualPalette(VisualPalette visualPalette) {
this.visualPalette = visualPalette;
}
/**
*
* The palette (chart color) display setup of the visual.
*
*
* @return The palette (chart color) display setup of the visual.
*/
public VisualPalette getVisualPalette() {
return this.visualPalette;
}
/**
*
* The palette (chart color) display setup of the visual.
*
*
* @param visualPalette
* The palette (chart color) display setup of the visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withVisualPalette(VisualPalette visualPalette) {
setVisualPalette(visualPalette);
return this;
}
/**
*
* Determines the visibility of the colors of alternatign bands in a radar chart.
*
*
* @param alternateBandColorsVisibility
* Determines the visibility of the colors of alternatign bands in a radar chart.
* @see Visibility
*/
public void setAlternateBandColorsVisibility(String alternateBandColorsVisibility) {
this.alternateBandColorsVisibility = alternateBandColorsVisibility;
}
/**
*
* Determines the visibility of the colors of alternatign bands in a radar chart.
*
*
* @return Determines the visibility of the colors of alternatign bands in a radar chart.
* @see Visibility
*/
public String getAlternateBandColorsVisibility() {
return this.alternateBandColorsVisibility;
}
/**
*
* Determines the visibility of the colors of alternatign bands in a radar chart.
*
*
* @param alternateBandColorsVisibility
* Determines the visibility of the colors of alternatign bands in a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public RadarChartConfiguration withAlternateBandColorsVisibility(String alternateBandColorsVisibility) {
setAlternateBandColorsVisibility(alternateBandColorsVisibility);
return this;
}
/**
*
* Determines the visibility of the colors of alternatign bands in a radar chart.
*
*
* @param alternateBandColorsVisibility
* Determines the visibility of the colors of alternatign bands in a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public RadarChartConfiguration withAlternateBandColorsVisibility(Visibility alternateBandColorsVisibility) {
this.alternateBandColorsVisibility = alternateBandColorsVisibility.toString();
return this;
}
/**
*
* The color of the even-numbered alternate bands of a radar chart.
*
*
* @param alternateBandEvenColor
* The color of the even-numbered alternate bands of a radar chart.
*/
public void setAlternateBandEvenColor(String alternateBandEvenColor) {
this.alternateBandEvenColor = alternateBandEvenColor;
}
/**
*
* The color of the even-numbered alternate bands of a radar chart.
*
*
* @return The color of the even-numbered alternate bands of a radar chart.
*/
public String getAlternateBandEvenColor() {
return this.alternateBandEvenColor;
}
/**
*
* The color of the even-numbered alternate bands of a radar chart.
*
*
* @param alternateBandEvenColor
* The color of the even-numbered alternate bands of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withAlternateBandEvenColor(String alternateBandEvenColor) {
setAlternateBandEvenColor(alternateBandEvenColor);
return this;
}
/**
*
* The color of the odd-numbered alternate bands of a radar chart.
*
*
* @param alternateBandOddColor
* The color of the odd-numbered alternate bands of a radar chart.
*/
public void setAlternateBandOddColor(String alternateBandOddColor) {
this.alternateBandOddColor = alternateBandOddColor;
}
/**
*
* The color of the odd-numbered alternate bands of a radar chart.
*
*
* @return The color of the odd-numbered alternate bands of a radar chart.
*/
public String getAlternateBandOddColor() {
return this.alternateBandOddColor;
}
/**
*
* The color of the odd-numbered alternate bands of a radar chart.
*
*
* @param alternateBandOddColor
* The color of the odd-numbered alternate bands of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withAlternateBandOddColor(String alternateBandOddColor) {
setAlternateBandOddColor(alternateBandOddColor);
return this;
}
/**
*
* The category axis of a radar chart.
*
*
* @param categoryAxis
* The category axis of a radar chart.
*/
public void setCategoryAxis(AxisDisplayOptions categoryAxis) {
this.categoryAxis = categoryAxis;
}
/**
*
* The category axis of a radar chart.
*
*
* @return The category axis of a radar chart.
*/
public AxisDisplayOptions getCategoryAxis() {
return this.categoryAxis;
}
/**
*
* The category axis of a radar chart.
*
*
* @param categoryAxis
* The category axis of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withCategoryAxis(AxisDisplayOptions categoryAxis) {
setCategoryAxis(categoryAxis);
return this;
}
/**
*
* The category label options of a radar chart.
*
*
* @param categoryLabelOptions
* The category label options of a radar chart.
*/
public void setCategoryLabelOptions(ChartAxisLabelOptions categoryLabelOptions) {
this.categoryLabelOptions = categoryLabelOptions;
}
/**
*
* The category label options of a radar chart.
*
*
* @return The category label options of a radar chart.
*/
public ChartAxisLabelOptions getCategoryLabelOptions() {
return this.categoryLabelOptions;
}
/**
*
* The category label options of a radar chart.
*
*
* @param categoryLabelOptions
* The category label options of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withCategoryLabelOptions(ChartAxisLabelOptions categoryLabelOptions) {
setCategoryLabelOptions(categoryLabelOptions);
return this;
}
/**
*
* The color axis of a radar chart.
*
*
* @param colorAxis
* The color axis of a radar chart.
*/
public void setColorAxis(AxisDisplayOptions colorAxis) {
this.colorAxis = colorAxis;
}
/**
*
* The color axis of a radar chart.
*
*
* @return The color axis of a radar chart.
*/
public AxisDisplayOptions getColorAxis() {
return this.colorAxis;
}
/**
*
* The color axis of a radar chart.
*
*
* @param colorAxis
* The color axis of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withColorAxis(AxisDisplayOptions colorAxis) {
setColorAxis(colorAxis);
return this;
}
/**
*
* The color label options of a radar chart.
*
*
* @param colorLabelOptions
* The color label options of a radar chart.
*/
public void setColorLabelOptions(ChartAxisLabelOptions colorLabelOptions) {
this.colorLabelOptions = colorLabelOptions;
}
/**
*
* The color label options of a radar chart.
*
*
* @return The color label options of a radar chart.
*/
public ChartAxisLabelOptions getColorLabelOptions() {
return this.colorLabelOptions;
}
/**
*
* The color label options of a radar chart.
*
*
* @param colorLabelOptions
* The color label options of a radar chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withColorLabelOptions(ChartAxisLabelOptions colorLabelOptions) {
setColorLabelOptions(colorLabelOptions);
return this;
}
/**
*
* The legend display setup of the visual.
*
*
* @param legend
* The legend display setup of the visual.
*/
public void setLegend(LegendOptions legend) {
this.legend = legend;
}
/**
*
* The legend display setup of the visual.
*
*
* @return The legend display setup of the visual.
*/
public LegendOptions getLegend() {
return this.legend;
}
/**
*
* The legend display setup of the visual.
*
*
* @param legend
* The legend display setup of the visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RadarChartConfiguration withLegend(LegendOptions legend) {
setLegend(legend);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFieldWells() != null)
sb.append("FieldWells: ").append(getFieldWells()).append(",");
if (getSortConfiguration() != null)
sb.append("SortConfiguration: ").append(getSortConfiguration()).append(",");
if (getShape() != null)
sb.append("Shape: ").append(getShape()).append(",");
if (getBaseSeriesSettings() != null)
sb.append("BaseSeriesSettings: ").append(getBaseSeriesSettings()).append(",");
if (getStartAngle() != null)
sb.append("StartAngle: ").append(getStartAngle()).append(",");
if (getVisualPalette() != null)
sb.append("VisualPalette: ").append(getVisualPalette()).append(",");
if (getAlternateBandColorsVisibility() != null)
sb.append("AlternateBandColorsVisibility: ").append(getAlternateBandColorsVisibility()).append(",");
if (getAlternateBandEvenColor() != null)
sb.append("AlternateBandEvenColor: ").append(getAlternateBandEvenColor()).append(",");
if (getAlternateBandOddColor() != null)
sb.append("AlternateBandOddColor: ").append(getAlternateBandOddColor()).append(",");
if (getCategoryAxis() != null)
sb.append("CategoryAxis: ").append(getCategoryAxis()).append(",");
if (getCategoryLabelOptions() != null)
sb.append("CategoryLabelOptions: ").append(getCategoryLabelOptions()).append(",");
if (getColorAxis() != null)
sb.append("ColorAxis: ").append(getColorAxis()).append(",");
if (getColorLabelOptions() != null)
sb.append("ColorLabelOptions: ").append(getColorLabelOptions()).append(",");
if (getLegend() != null)
sb.append("Legend: ").append(getLegend());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RadarChartConfiguration == false)
return false;
RadarChartConfiguration other = (RadarChartConfiguration) obj;
if (other.getFieldWells() == null ^ this.getFieldWells() == null)
return false;
if (other.getFieldWells() != null && other.getFieldWells().equals(this.getFieldWells()) == false)
return false;
if (other.getSortConfiguration() == null ^ this.getSortConfiguration() == null)
return false;
if (other.getSortConfiguration() != null && other.getSortConfiguration().equals(this.getSortConfiguration()) == false)
return false;
if (other.getShape() == null ^ this.getShape() == null)
return false;
if (other.getShape() != null && other.getShape().equals(this.getShape()) == false)
return false;
if (other.getBaseSeriesSettings() == null ^ this.getBaseSeriesSettings() == null)
return false;
if (other.getBaseSeriesSettings() != null && other.getBaseSeriesSettings().equals(this.getBaseSeriesSettings()) == false)
return false;
if (other.getStartAngle() == null ^ this.getStartAngle() == null)
return false;
if (other.getStartAngle() != null && other.getStartAngle().equals(this.getStartAngle()) == false)
return false;
if (other.getVisualPalette() == null ^ this.getVisualPalette() == null)
return false;
if (other.getVisualPalette() != null && other.getVisualPalette().equals(this.getVisualPalette()) == false)
return false;
if (other.getAlternateBandColorsVisibility() == null ^ this.getAlternateBandColorsVisibility() == null)
return false;
if (other.getAlternateBandColorsVisibility() != null
&& other.getAlternateBandColorsVisibility().equals(this.getAlternateBandColorsVisibility()) == false)
return false;
if (other.getAlternateBandEvenColor() == null ^ this.getAlternateBandEvenColor() == null)
return false;
if (other.getAlternateBandEvenColor() != null && other.getAlternateBandEvenColor().equals(this.getAlternateBandEvenColor()) == false)
return false;
if (other.getAlternateBandOddColor() == null ^ this.getAlternateBandOddColor() == null)
return false;
if (other.getAlternateBandOddColor() != null && other.getAlternateBandOddColor().equals(this.getAlternateBandOddColor()) == false)
return false;
if (other.getCategoryAxis() == null ^ this.getCategoryAxis() == null)
return false;
if (other.getCategoryAxis() != null && other.getCategoryAxis().equals(this.getCategoryAxis()) == false)
return false;
if (other.getCategoryLabelOptions() == null ^ this.getCategoryLabelOptions() == null)
return false;
if (other.getCategoryLabelOptions() != null && other.getCategoryLabelOptions().equals(this.getCategoryLabelOptions()) == false)
return false;
if (other.getColorAxis() == null ^ this.getColorAxis() == null)
return false;
if (other.getColorAxis() != null && other.getColorAxis().equals(this.getColorAxis()) == false)
return false;
if (other.getColorLabelOptions() == null ^ this.getColorLabelOptions() == null)
return false;
if (other.getColorLabelOptions() != null && other.getColorLabelOptions().equals(this.getColorLabelOptions()) == false)
return false;
if (other.getLegend() == null ^ this.getLegend() == null)
return false;
if (other.getLegend() != null && other.getLegend().equals(this.getLegend()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFieldWells() == null) ? 0 : getFieldWells().hashCode());
hashCode = prime * hashCode + ((getSortConfiguration() == null) ? 0 : getSortConfiguration().hashCode());
hashCode = prime * hashCode + ((getShape() == null) ? 0 : getShape().hashCode());
hashCode = prime * hashCode + ((getBaseSeriesSettings() == null) ? 0 : getBaseSeriesSettings().hashCode());
hashCode = prime * hashCode + ((getStartAngle() == null) ? 0 : getStartAngle().hashCode());
hashCode = prime * hashCode + ((getVisualPalette() == null) ? 0 : getVisualPalette().hashCode());
hashCode = prime * hashCode + ((getAlternateBandColorsVisibility() == null) ? 0 : getAlternateBandColorsVisibility().hashCode());
hashCode = prime * hashCode + ((getAlternateBandEvenColor() == null) ? 0 : getAlternateBandEvenColor().hashCode());
hashCode = prime * hashCode + ((getAlternateBandOddColor() == null) ? 0 : getAlternateBandOddColor().hashCode());
hashCode = prime * hashCode + ((getCategoryAxis() == null) ? 0 : getCategoryAxis().hashCode());
hashCode = prime * hashCode + ((getCategoryLabelOptions() == null) ? 0 : getCategoryLabelOptions().hashCode());
hashCode = prime * hashCode + ((getColorAxis() == null) ? 0 : getColorAxis().hashCode());
hashCode = prime * hashCode + ((getColorLabelOptions() == null) ? 0 : getColorLabelOptions().hashCode());
hashCode = prime * hashCode + ((getLegend() == null) ? 0 : getLegend().hashCode());
return hashCode;
}
@Override
public RadarChartConfiguration clone() {
try {
return (RadarChartConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.RadarChartConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}