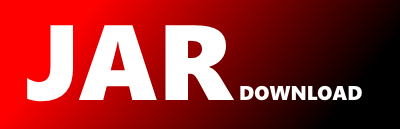
com.amazonaws.services.quicksight.model.UpdateUserRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateUserRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon QuickSight user name that you want to update.
*
*/
private String userName;
/**
*
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon Web
* Services account that contains your Amazon QuickSight account.
*
*/
private String awsAccountId;
/**
*
* The namespace. Currently, you should set this to default
.
*
*/
private String namespace;
/**
*
* The email address of the user that you want to update.
*
*/
private String email;
/**
*
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing with
* permissions.
*
*/
private String role;
/**
*
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following operations:
*
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create the
* profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the permissions
* typically granted by assigning Amazon QuickSight users to one of the default security cohorts in Amazon
* QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*
*/
private String customPermissionsName;
/**
*
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This parameter
* defaults to NULL and it doesn't accept any other value.
*
*/
private Boolean unapplyCustomPermissions;
/**
*
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external login
* provider can be one of the following.
*
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing the
* COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is only needed
* when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
type, use
* the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*
*/
private String externalLoginFederationProviderType;
/**
*
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only be used when
* ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
.
*
*/
private String customFederationProviderUrl;
/**
*
* The identity ID for a user in the external login provider.
*
*/
private String externalLoginId;
/**
*
* The Amazon QuickSight user name that you want to update.
*
*
* @param userName
* The Amazon QuickSight user name that you want to update.
*/
public void setUserName(String userName) {
this.userName = userName;
}
/**
*
* The Amazon QuickSight user name that you want to update.
*
*
* @return The Amazon QuickSight user name that you want to update.
*/
public String getUserName() {
return this.userName;
}
/**
*
* The Amazon QuickSight user name that you want to update.
*
*
* @param userName
* The Amazon QuickSight user name that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withUserName(String userName) {
setUserName(userName);
return this;
}
/**
*
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon Web
* Services account that contains your Amazon QuickSight account.
*
*
* @param awsAccountId
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon
* Web Services account that contains your Amazon QuickSight account.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon Web
* Services account that contains your Amazon QuickSight account.
*
*
* @return The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon
* Web Services account that contains your Amazon QuickSight account.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon Web
* Services account that contains your Amazon QuickSight account.
*
*
* @param awsAccountId
* The ID for the Amazon Web Services account that the user is in. Currently, you use the ID for the Amazon
* Web Services account that contains your Amazon QuickSight account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The namespace. Currently, you should set this to default
.
*
*
* @param namespace
* The namespace. Currently, you should set this to default
.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
*
* The namespace. Currently, you should set this to default
.
*
*
* @return The namespace. Currently, you should set this to default
.
*/
public String getNamespace() {
return this.namespace;
}
/**
*
* The namespace. Currently, you should set this to default
.
*
*
* @param namespace
* The namespace. Currently, you should set this to default
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withNamespace(String namespace) {
setNamespace(namespace);
return this;
}
/**
*
* The email address of the user that you want to update.
*
*
* @param email
* The email address of the user that you want to update.
*/
public void setEmail(String email) {
this.email = email;
}
/**
*
* The email address of the user that you want to update.
*
*
* @return The email address of the user that you want to update.
*/
public String getEmail() {
return this.email;
}
/**
*
* The email address of the user that you want to update.
*
*
* @param email
* The email address of the user that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withEmail(String email) {
setEmail(email);
return this;
}
/**
*
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing with
* permissions.
*
*
* @param role
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing
* with permissions.
* @see UserRole
*/
public void setRole(String role) {
this.role = role;
}
/**
*
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing with
* permissions.
*
*
* @return The Amazon QuickSight role of the user. The role can be one of the following default security
* cohorts:
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing
* with permissions.
* @see UserRole
*/
public String getRole() {
return this.role;
}
/**
*
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing with
* permissions.
*
*
* @param role
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing
* with permissions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UserRole
*/
public UpdateUserRequest withRole(String role) {
setRole(role);
return this;
}
/**
*
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing with
* permissions.
*
*
* @param role
* The Amazon QuickSight role of the user. The role can be one of the following default security cohorts:
*
* -
*
* READER
: A user who has read-only access to dashboards.
*
*
* -
*
* AUTHOR
: A user who can create data sources, datasets, analyses, and dashboards.
*
*
* -
*
* ADMIN
: A user who is an author, who can also manage Amazon QuickSight settings.
*
*
*
*
* The name of the Amazon QuickSight role is invisible to the user except for the console screens dealing
* with permissions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see UserRole
*/
public UpdateUserRequest withRole(UserRole role) {
this.role = role.toString();
return this;
}
/**
*
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following operations:
*
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create the
* profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the permissions
* typically granted by assigning Amazon QuickSight users to one of the default security cohorts in Amazon
* QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*
*
* @param customPermissionsName
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following
* operations:
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create
* the profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight
* user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the
* permissions typically granted by assigning Amazon QuickSight users to one of the default security cohorts
* in Amazon QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*/
public void setCustomPermissionsName(String customPermissionsName) {
this.customPermissionsName = customPermissionsName;
}
/**
*
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following operations:
*
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create the
* profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the permissions
* typically granted by assigning Amazon QuickSight users to one of the default security cohorts in Amazon
* QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*
*
* @return (Enterprise edition only) The name of the custom permissions profile that you want to assign to this
* user. Customized permissions allows you to control a user's access by restricting access the following
* operations:
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create
* the profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight
* user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the
* permissions typically granted by assigning Amazon QuickSight users to one of the default security cohorts
* in Amazon QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*/
public String getCustomPermissionsName() {
return this.customPermissionsName;
}
/**
*
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following operations:
*
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create the
* profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the permissions
* typically granted by assigning Amazon QuickSight users to one of the default security cohorts in Amazon
* QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
*
*
* @param customPermissionsName
* (Enterprise edition only) The name of the custom permissions profile that you want to assign to this user.
* Customized permissions allows you to control a user's access by restricting access the following
* operations:
*
* -
*
* Create and update data sources
*
*
* -
*
* Create and update datasets
*
*
* -
*
* Create and update email reports
*
*
* -
*
* Subscribe to email reports
*
*
*
*
* A set of custom permissions includes any combination of these restrictions. Currently, you need to create
* the profile names for custom permission sets by using the Amazon QuickSight console. Then, you use the
* RegisterUser
API operation to assign the named set of permissions to a Amazon QuickSight
* user.
*
*
* Amazon QuickSight custom permissions are applied through IAM policies. Therefore, they override the
* permissions typically granted by assigning Amazon QuickSight users to one of the default security cohorts
* in Amazon QuickSight (admin, author, reader).
*
*
* This feature is available only to Amazon QuickSight Enterprise edition subscriptions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withCustomPermissionsName(String customPermissionsName) {
setCustomPermissionsName(customPermissionsName);
return this;
}
/**
*
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This parameter
* defaults to NULL and it doesn't accept any other value.
*
*
* @param unapplyCustomPermissions
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This
* parameter defaults to NULL and it doesn't accept any other value.
*/
public void setUnapplyCustomPermissions(Boolean unapplyCustomPermissions) {
this.unapplyCustomPermissions = unapplyCustomPermissions;
}
/**
*
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This parameter
* defaults to NULL and it doesn't accept any other value.
*
*
* @return A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This
* parameter defaults to NULL and it doesn't accept any other value.
*/
public Boolean getUnapplyCustomPermissions() {
return this.unapplyCustomPermissions;
}
/**
*
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This parameter
* defaults to NULL and it doesn't accept any other value.
*
*
* @param unapplyCustomPermissions
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This
* parameter defaults to NULL and it doesn't accept any other value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withUnapplyCustomPermissions(Boolean unapplyCustomPermissions) {
setUnapplyCustomPermissions(unapplyCustomPermissions);
return this;
}
/**
*
* A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This parameter
* defaults to NULL and it doesn't accept any other value.
*
*
* @return A flag that you use to indicate that you want to remove all custom permissions from this user. Using this
* parameter resets the user to the state it was in before a custom permissions profile was applied. This
* parameter defaults to NULL and it doesn't accept any other value.
*/
public Boolean isUnapplyCustomPermissions() {
return this.unapplyCustomPermissions;
}
/**
*
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external login
* provider can be one of the following.
*
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing the
* COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is only needed
* when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
type, use
* the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*
*
* @param externalLoginFederationProviderType
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external
* login provider can be one of the following.
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing
* the COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is
* only needed when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
* type, use the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*/
public void setExternalLoginFederationProviderType(String externalLoginFederationProviderType) {
this.externalLoginFederationProviderType = externalLoginFederationProviderType;
}
/**
*
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external login
* provider can be one of the following.
*
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing the
* COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is only needed
* when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
type, use
* the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*
*
* @return The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external
* login provider can be one of the following.
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing
* the COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is
* only needed when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
* type, use the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*/
public String getExternalLoginFederationProviderType() {
return this.externalLoginFederationProviderType;
}
/**
*
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external login
* provider can be one of the following.
*
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing the
* COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is only needed
* when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
type, use
* the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
*
*
* @param externalLoginFederationProviderType
* The type of supported external login provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. The type of supported external
* login provider can be one of the following.
*
* -
*
* COGNITO
: Amazon Cognito. The provider URL is cognito-identity.amazonaws.com. When choosing
* the COGNITO
provider type, don’t use the "CustomFederationProviderUrl" parameter which is
* only needed when the external provider is custom.
*
*
* -
*
* CUSTOM_OIDC
: Custom OpenID Connect (OIDC) provider. When choosing CUSTOM_OIDC
* type, use the CustomFederationProviderUrl
parameter to provide the custom OIDC provider URL.
*
*
* -
*
* NONE
: This clears all the previously saved external login information for a user. Use the
* DescribeUser
* API operation to check the external login information.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withExternalLoginFederationProviderType(String externalLoginFederationProviderType) {
setExternalLoginFederationProviderType(externalLoginFederationProviderType);
return this;
}
/**
*
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only be used when
* ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
.
*
*
* @param customFederationProviderUrl
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into
* Amazon QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only
* be used when ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
* .
*/
public void setCustomFederationProviderUrl(String customFederationProviderUrl) {
this.customFederationProviderUrl = customFederationProviderUrl;
}
/**
*
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only be used when
* ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
.
*
*
* @return The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into
* Amazon QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only
* be used when ExternalLoginFederationProviderType
parameter is set to
* CUSTOM_OIDC
.
*/
public String getCustomFederationProviderUrl() {
return this.customFederationProviderUrl;
}
/**
*
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into Amazon
* QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only be used when
* ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
.
*
*
* @param customFederationProviderUrl
* The URL of the custom OpenID Connect (OIDC) provider that provides identity to let a user federate into
* Amazon QuickSight with an associated Identity and Access Management(IAM) role. This parameter should only
* be used when ExternalLoginFederationProviderType
parameter is set to CUSTOM_OIDC
* .
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withCustomFederationProviderUrl(String customFederationProviderUrl) {
setCustomFederationProviderUrl(customFederationProviderUrl);
return this;
}
/**
*
* The identity ID for a user in the external login provider.
*
*
* @param externalLoginId
* The identity ID for a user in the external login provider.
*/
public void setExternalLoginId(String externalLoginId) {
this.externalLoginId = externalLoginId;
}
/**
*
* The identity ID for a user in the external login provider.
*
*
* @return The identity ID for a user in the external login provider.
*/
public String getExternalLoginId() {
return this.externalLoginId;
}
/**
*
* The identity ID for a user in the external login provider.
*
*
* @param externalLoginId
* The identity ID for a user in the external login provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateUserRequest withExternalLoginId(String externalLoginId) {
setExternalLoginId(externalLoginId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUserName() != null)
sb.append("UserName: ").append(getUserName()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getNamespace() != null)
sb.append("Namespace: ").append(getNamespace()).append(",");
if (getEmail() != null)
sb.append("Email: ").append(getEmail()).append(",");
if (getRole() != null)
sb.append("Role: ").append(getRole()).append(",");
if (getCustomPermissionsName() != null)
sb.append("CustomPermissionsName: ").append(getCustomPermissionsName()).append(",");
if (getUnapplyCustomPermissions() != null)
sb.append("UnapplyCustomPermissions: ").append(getUnapplyCustomPermissions()).append(",");
if (getExternalLoginFederationProviderType() != null)
sb.append("ExternalLoginFederationProviderType: ").append(getExternalLoginFederationProviderType()).append(",");
if (getCustomFederationProviderUrl() != null)
sb.append("CustomFederationProviderUrl: ").append(getCustomFederationProviderUrl()).append(",");
if (getExternalLoginId() != null)
sb.append("ExternalLoginId: ").append(getExternalLoginId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateUserRequest == false)
return false;
UpdateUserRequest other = (UpdateUserRequest) obj;
if (other.getUserName() == null ^ this.getUserName() == null)
return false;
if (other.getUserName() != null && other.getUserName().equals(this.getUserName()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getNamespace() == null ^ this.getNamespace() == null)
return false;
if (other.getNamespace() != null && other.getNamespace().equals(this.getNamespace()) == false)
return false;
if (other.getEmail() == null ^ this.getEmail() == null)
return false;
if (other.getEmail() != null && other.getEmail().equals(this.getEmail()) == false)
return false;
if (other.getRole() == null ^ this.getRole() == null)
return false;
if (other.getRole() != null && other.getRole().equals(this.getRole()) == false)
return false;
if (other.getCustomPermissionsName() == null ^ this.getCustomPermissionsName() == null)
return false;
if (other.getCustomPermissionsName() != null && other.getCustomPermissionsName().equals(this.getCustomPermissionsName()) == false)
return false;
if (other.getUnapplyCustomPermissions() == null ^ this.getUnapplyCustomPermissions() == null)
return false;
if (other.getUnapplyCustomPermissions() != null && other.getUnapplyCustomPermissions().equals(this.getUnapplyCustomPermissions()) == false)
return false;
if (other.getExternalLoginFederationProviderType() == null ^ this.getExternalLoginFederationProviderType() == null)
return false;
if (other.getExternalLoginFederationProviderType() != null
&& other.getExternalLoginFederationProviderType().equals(this.getExternalLoginFederationProviderType()) == false)
return false;
if (other.getCustomFederationProviderUrl() == null ^ this.getCustomFederationProviderUrl() == null)
return false;
if (other.getCustomFederationProviderUrl() != null && other.getCustomFederationProviderUrl().equals(this.getCustomFederationProviderUrl()) == false)
return false;
if (other.getExternalLoginId() == null ^ this.getExternalLoginId() == null)
return false;
if (other.getExternalLoginId() != null && other.getExternalLoginId().equals(this.getExternalLoginId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUserName() == null) ? 0 : getUserName().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getNamespace() == null) ? 0 : getNamespace().hashCode());
hashCode = prime * hashCode + ((getEmail() == null) ? 0 : getEmail().hashCode());
hashCode = prime * hashCode + ((getRole() == null) ? 0 : getRole().hashCode());
hashCode = prime * hashCode + ((getCustomPermissionsName() == null) ? 0 : getCustomPermissionsName().hashCode());
hashCode = prime * hashCode + ((getUnapplyCustomPermissions() == null) ? 0 : getUnapplyCustomPermissions().hashCode());
hashCode = prime * hashCode + ((getExternalLoginFederationProviderType() == null) ? 0 : getExternalLoginFederationProviderType().hashCode());
hashCode = prime * hashCode + ((getCustomFederationProviderUrl() == null) ? 0 : getCustomFederationProviderUrl().hashCode());
hashCode = prime * hashCode + ((getExternalLoginId() == null) ? 0 : getExternalLoginId().hashCode());
return hashCode;
}
@Override
public UpdateUserRequest clone() {
return (UpdateUserRequest) super.clone();
}
}