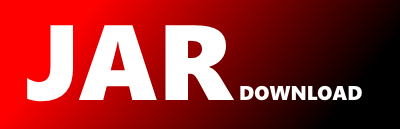
com.amazonaws.services.quicksight.model.CreateAccountSubscriptionRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAccountSubscriptionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
*
*/
private String edition;
/**
*
* The method that you want to use to authenticate your Amazon QuickSight account.
*
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your IAM
* Identity Center account.
*
*/
private String authenticationMethod;
/**
*
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight account.
*
*/
private String awsAccountId;
/**
*
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it appears
* only when users sign in. You can't change AccountName
value after the Amazon QuickSight account is
* created.
*
*/
private String accountName;
/**
*
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight
* account or Amazon QuickSight subscription.
*
*/
private String notificationEmail;
/**
*
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
*
*/
private String activeDirectoryName;
/**
*
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is required
* if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon QuickSight account.
*
*/
private String realm;
/**
*
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
*
*/
private String directoryId;
/**
*
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or the
* AdminProGroup
field is required if ACTIVE_DIRECTORY
or IAM_IDENTITY_CENTER
* is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List adminGroup;
/**
*
* The author group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List authorGroup;
/**
*
* The reader group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List readerGroup;
/**
*
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List adminProGroup;
/**
*
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List authorProGroup;
/**
*
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*/
private java.util.List readerProGroup;
/**
*
* The first name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*/
private String firstName;
/**
*
* The last name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*/
private String lastName;
/**
*
* The email address of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*/
private String emailAddress;
/**
*
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*/
private String contactNumber;
/**
*
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*/
private String iAMIdentityCenterInstanceArn;
/**
*
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
*
*
* @param edition
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
* @see Edition
*/
public void setEdition(String edition) {
this.edition = edition;
}
/**
*
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
*
*
* @return The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
* @see Edition
*/
public String getEdition() {
return this.edition;
}
/**
*
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
*
*
* @param edition
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Edition
*/
public CreateAccountSubscriptionRequest withEdition(String edition) {
setEdition(edition);
return this;
}
/**
*
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
*
*
* @param edition
* The edition of Amazon QuickSight that you want your account to have. Currently, you can choose from
* ENTERPRISE
or ENTERPRISE_AND_Q
.
*
* If you choose ENTERPRISE_AND_Q
, the following parameters are required:
*
*
* -
*
* FirstName
*
*
* -
*
* LastName
*
*
* -
*
* EmailAddress
*
*
* -
*
* ContactNumber
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Edition
*/
public CreateAccountSubscriptionRequest withEdition(Edition edition) {
this.edition = edition.toString();
return this;
}
/**
*
* The method that you want to use to authenticate your Amazon QuickSight account.
*
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your IAM
* Identity Center account.
*
*
* @param authenticationMethod
* The method that you want to use to authenticate your Amazon QuickSight account.
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your
* IAM Identity Center account.
* @see AuthenticationMethodOption
*/
public void setAuthenticationMethod(String authenticationMethod) {
this.authenticationMethod = authenticationMethod;
}
/**
*
* The method that you want to use to authenticate your Amazon QuickSight account.
*
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your IAM
* Identity Center account.
*
*
* @return The method that you want to use to authenticate your Amazon QuickSight account.
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your
* IAM Identity Center account.
* @see AuthenticationMethodOption
*/
public String getAuthenticationMethod() {
return this.authenticationMethod;
}
/**
*
* The method that you want to use to authenticate your Amazon QuickSight account.
*
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your IAM
* Identity Center account.
*
*
* @param authenticationMethod
* The method that you want to use to authenticate your Amazon QuickSight account.
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your
* IAM Identity Center account.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationMethodOption
*/
public CreateAccountSubscriptionRequest withAuthenticationMethod(String authenticationMethod) {
setAuthenticationMethod(authenticationMethod);
return this;
}
/**
*
* The method that you want to use to authenticate your Amazon QuickSight account.
*
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your IAM
* Identity Center account.
*
*
* @param authenticationMethod
* The method that you want to use to authenticate your Amazon QuickSight account.
*
* If you choose ACTIVE_DIRECTORY
, provide an ActiveDirectoryName
and an
* AdminGroup
associated with your Active Directory.
*
*
* If you choose IAM_IDENTITY_CENTER
, provide an AdminGroup
associated with your
* IAM Identity Center account.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthenticationMethodOption
*/
public CreateAccountSubscriptionRequest withAuthenticationMethod(AuthenticationMethodOption authenticationMethod) {
this.authenticationMethod = authenticationMethod.toString();
return this;
}
/**
*
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight account.
*
*
* @param awsAccountId
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight
* account.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight account.
*
*
* @return The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight
* account.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight account.
*
*
* @param awsAccountId
* The Amazon Web Services account ID of the account that you're using to create your Amazon QuickSight
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it appears
* only when users sign in. You can't change AccountName
value after the Amazon QuickSight account is
* created.
*
*
* @param accountName
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it
* appears only when users sign in. You can't change AccountName
value after the Amazon
* QuickSight account is created.
*/
public void setAccountName(String accountName) {
this.accountName = accountName;
}
/**
*
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it appears
* only when users sign in. You can't change AccountName
value after the Amazon QuickSight account is
* created.
*
*
* @return The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it
* appears only when users sign in. You can't change AccountName
value after the Amazon
* QuickSight account is created.
*/
public String getAccountName() {
return this.accountName;
}
/**
*
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it appears
* only when users sign in. You can't change AccountName
value after the Amazon QuickSight account is
* created.
*
*
* @param accountName
* The name of your Amazon QuickSight account. This name is unique over all of Amazon Web Services, and it
* appears only when users sign in. You can't change AccountName
value after the Amazon
* QuickSight account is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAccountName(String accountName) {
setAccountName(accountName);
return this;
}
/**
*
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight
* account or Amazon QuickSight subscription.
*
*
* @param notificationEmail
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon
* QuickSight account or Amazon QuickSight subscription.
*/
public void setNotificationEmail(String notificationEmail) {
this.notificationEmail = notificationEmail;
}
/**
*
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight
* account or Amazon QuickSight subscription.
*
*
* @return The email address that you want Amazon QuickSight to send notifications to regarding your Amazon
* QuickSight account or Amazon QuickSight subscription.
*/
public String getNotificationEmail() {
return this.notificationEmail;
}
/**
*
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon QuickSight
* account or Amazon QuickSight subscription.
*
*
* @param notificationEmail
* The email address that you want Amazon QuickSight to send notifications to regarding your Amazon
* QuickSight account or Amazon QuickSight subscription.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withNotificationEmail(String notificationEmail) {
setNotificationEmail(notificationEmail);
return this;
}
/**
*
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
*
*
* @param activeDirectoryName
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
*/
public void setActiveDirectoryName(String activeDirectoryName) {
this.activeDirectoryName = activeDirectoryName;
}
/**
*
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
*
*
* @return The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the
* selected authentication method of the new Amazon QuickSight account.
*/
public String getActiveDirectoryName() {
return this.activeDirectoryName;
}
/**
*
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
*
*
* @param activeDirectoryName
* The name of your Active Directory. This field is required if ACTIVE_DIRECTORY
is the selected
* authentication method of the new Amazon QuickSight account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withActiveDirectoryName(String activeDirectoryName) {
setActiveDirectoryName(activeDirectoryName);
return this;
}
/**
*
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is required
* if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon QuickSight account.
*
*
* @param realm
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is
* required if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon
* QuickSight account.
*/
public void setRealm(String realm) {
this.realm = realm;
}
/**
*
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is required
* if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon QuickSight account.
*
*
* @return The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is
* required if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon
* QuickSight account.
*/
public String getRealm() {
return this.realm;
}
/**
*
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is required
* if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon QuickSight account.
*
*
* @param realm
* The realm of the Active Directory that is associated with your Amazon QuickSight account. This field is
* required if ACTIVE_DIRECTORY
is the selected authentication method of the new Amazon
* QuickSight account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withRealm(String realm) {
setRealm(realm);
return this;
}
/**
*
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
*
*
* @param directoryId
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
*/
public void setDirectoryId(String directoryId) {
this.directoryId = directoryId;
}
/**
*
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
*
*
* @return The ID of the Active Directory that is associated with your Amazon QuickSight account.
*/
public String getDirectoryId() {
return this.directoryId;
}
/**
*
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
*
*
* @param directoryId
* The ID of the Active Directory that is associated with your Amazon QuickSight account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withDirectoryId(String directoryId) {
setDirectoryId(directoryId);
return this;
}
/**
*
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or the
* AdminProGroup
field is required if ACTIVE_DIRECTORY
or IAM_IDENTITY_CENTER
* is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The admin group associated with your Active Directory or IAM Identity Center account. Either this field
* or the AdminProGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getAdminGroup() {
return adminGroup;
}
/**
*
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or the
* AdminProGroup
field is required if ACTIVE_DIRECTORY
or IAM_IDENTITY_CENTER
* is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param adminGroup
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminProGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setAdminGroup(java.util.Collection adminGroup) {
if (adminGroup == null) {
this.adminGroup = null;
return;
}
this.adminGroup = new java.util.ArrayList(adminGroup);
}
/**
*
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or the
* AdminProGroup
field is required if ACTIVE_DIRECTORY
or IAM_IDENTITY_CENTER
* is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdminGroup(java.util.Collection)} or {@link #withAdminGroup(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param adminGroup
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminProGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAdminGroup(String... adminGroup) {
if (this.adminGroup == null) {
setAdminGroup(new java.util.ArrayList(adminGroup.length));
}
for (String ele : adminGroup) {
this.adminGroup.add(ele);
}
return this;
}
/**
*
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or the
* AdminProGroup
field is required if ACTIVE_DIRECTORY
or IAM_IDENTITY_CENTER
* is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param adminGroup
* The admin group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminProGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAdminGroup(java.util.Collection adminGroup) {
setAdminGroup(adminGroup);
return this;
}
/**
*
* The author group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The author group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getAuthorGroup() {
return authorGroup;
}
/**
*
* The author group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param authorGroup
* The author group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setAuthorGroup(java.util.Collection authorGroup) {
if (authorGroup == null) {
this.authorGroup = null;
return;
}
this.authorGroup = new java.util.ArrayList(authorGroup);
}
/**
*
* The author group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAuthorGroup(java.util.Collection)} or {@link #withAuthorGroup(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param authorGroup
* The author group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAuthorGroup(String... authorGroup) {
if (this.authorGroup == null) {
setAuthorGroup(new java.util.ArrayList(authorGroup.length));
}
for (String ele : authorGroup) {
this.authorGroup.add(ele);
}
return this;
}
/**
*
* The author group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param authorGroup
* The author group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAuthorGroup(java.util.Collection authorGroup) {
setAuthorGroup(authorGroup);
return this;
}
/**
*
* The reader group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The reader group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getReaderGroup() {
return readerGroup;
}
/**
*
* The reader group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param readerGroup
* The reader group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setReaderGroup(java.util.Collection readerGroup) {
if (readerGroup == null) {
this.readerGroup = null;
return;
}
this.readerGroup = new java.util.ArrayList(readerGroup);
}
/**
*
* The reader group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReaderGroup(java.util.Collection)} or {@link #withReaderGroup(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param readerGroup
* The reader group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withReaderGroup(String... readerGroup) {
if (this.readerGroup == null) {
setReaderGroup(new java.util.ArrayList(readerGroup.length));
}
for (String ele : readerGroup) {
this.readerGroup.add(ele);
}
return this;
}
/**
*
* The reader group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param readerGroup
* The reader group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withReaderGroup(java.util.Collection readerGroup) {
setReaderGroup(readerGroup);
return this;
}
/**
*
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The admin pro group associated with your Active Directory or IAM Identity Center account. Either this
* field or the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getAdminProGroup() {
return adminProGroup;
}
/**
*
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param adminProGroup
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this
* field or the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setAdminProGroup(java.util.Collection adminProGroup) {
if (adminProGroup == null) {
this.adminProGroup = null;
return;
}
this.adminProGroup = new java.util.ArrayList(adminProGroup);
}
/**
*
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdminProGroup(java.util.Collection)} or {@link #withAdminProGroup(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param adminProGroup
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this
* field or the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAdminProGroup(String... adminProGroup) {
if (this.adminProGroup == null) {
setAdminProGroup(new java.util.ArrayList(adminProGroup.length));
}
for (String ele : adminProGroup) {
this.adminProGroup.add(ele);
}
return this;
}
/**
*
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this field or
* the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param adminProGroup
* The admin pro group associated with your Active Directory or IAM Identity Center account. Either this
* field or the AdminGroup
field is required if ACTIVE_DIRECTORY
or
* IAM_IDENTITY_CENTER
is the selected authentication method of the new Amazon QuickSight
* account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAdminProGroup(java.util.Collection adminProGroup) {
setAdminProGroup(adminProGroup);
return this;
}
/**
*
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The author pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getAuthorProGroup() {
return authorProGroup;
}
/**
*
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param authorProGroup
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setAuthorProGroup(java.util.Collection authorProGroup) {
if (authorProGroup == null) {
this.authorProGroup = null;
return;
}
this.authorProGroup = new java.util.ArrayList(authorProGroup);
}
/**
*
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAuthorProGroup(java.util.Collection)} or {@link #withAuthorProGroup(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param authorProGroup
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAuthorProGroup(String... authorProGroup) {
if (this.authorProGroup == null) {
setAuthorProGroup(new java.util.ArrayList(authorProGroup.length));
}
for (String ele : authorProGroup) {
this.authorProGroup.add(ele);
}
return this;
}
/**
*
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param authorProGroup
* The author pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withAuthorProGroup(java.util.Collection authorProGroup) {
setAuthorProGroup(authorProGroup);
return this;
}
/**
*
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @return The reader pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public java.util.List getReaderProGroup() {
return readerProGroup;
}
/**
*
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param readerProGroup
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*/
public void setReaderProGroup(java.util.Collection readerProGroup) {
if (readerProGroup == null) {
this.readerProGroup = null;
return;
}
this.readerProGroup = new java.util.ArrayList(readerProGroup);
}
/**
*
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReaderProGroup(java.util.Collection)} or {@link #withReaderProGroup(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param readerProGroup
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withReaderProGroup(String... readerProGroup) {
if (this.readerProGroup == null) {
setReaderProGroup(new java.util.ArrayList(readerProGroup.length));
}
for (String ele : readerProGroup) {
this.readerProGroup.add(ele);
}
return this;
}
/**
*
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM
* Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide. For more
* information about using Active Directory in Amazon QuickSight, see Using Active Directory with
* Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
*
*
* @param readerProGroup
* The reader pro group associated with your Active Directory or IAM Identity Center account.
*
* For more information about using IAM Identity Center in Amazon QuickSight, see Using IAM Identity Center with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User
* Guide. For more information about using Active Directory in Amazon QuickSight, see Using Active
* Directory with Amazon QuickSight Enterprise Edition in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withReaderProGroup(java.util.Collection readerProGroup) {
setReaderProGroup(readerProGroup);
return this;
}
/**
*
* The first name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param firstName
* The first name of the author of the Amazon QuickSight account to use for future communications. This field
* is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
*
* The first name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @return The first name of the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public String getFirstName() {
return this.firstName;
}
/**
*
* The first name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param firstName
* The first name of the author of the Amazon QuickSight account to use for future communications. This field
* is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withFirstName(String firstName) {
setFirstName(firstName);
return this;
}
/**
*
* The last name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param lastName
* The last name of the author of the Amazon QuickSight account to use for future communications. This field
* is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
*
* The last name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @return The last name of the author of the Amazon QuickSight account to use for future communications. This field
* is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public String getLastName() {
return this.lastName;
}
/**
*
* The last name of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param lastName
* The last name of the author of the Amazon QuickSight account to use for future communications. This field
* is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withLastName(String lastName) {
setLastName(lastName);
return this;
}
/**
*
* The email address of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param emailAddress
* The email address of the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
/**
*
* The email address of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @return The email address of the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
*/
public String getEmailAddress() {
return this.emailAddress;
}
/**
*
* The email address of the author of the Amazon QuickSight account to use for future communications. This field is
* required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param emailAddress
* The email address of the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withEmailAddress(String emailAddress) {
setEmailAddress(emailAddress);
return this;
}
/**
*
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param contactNumber
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications.
* This field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon
* QuickSight account.
*/
public void setContactNumber(String contactNumber) {
this.contactNumber = contactNumber;
}
/**
*
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @return A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications.
* This field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon
* QuickSight account.
*/
public String getContactNumber() {
return this.contactNumber;
}
/**
*
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications. This
* field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon QuickSight account.
*
*
* @param contactNumber
* A 10-digit phone number for the author of the Amazon QuickSight account to use for future communications.
* This field is required if ENTERPPRISE_AND_Q
is the selected edition of the new Amazon
* QuickSight account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withContactNumber(String contactNumber) {
setContactNumber(contactNumber);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*
* @param iAMIdentityCenterInstanceArn
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*/
public void setIAMIdentityCenterInstanceArn(String iAMIdentityCenterInstanceArn) {
this.iAMIdentityCenterInstanceArn = iAMIdentityCenterInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*
* @return The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*/
public String getIAMIdentityCenterInstanceArn() {
return this.iAMIdentityCenterInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
*
*
* @param iAMIdentityCenterInstanceArn
* The Amazon Resource Name (ARN) for the IAM Identity Center instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccountSubscriptionRequest withIAMIdentityCenterInstanceArn(String iAMIdentityCenterInstanceArn) {
setIAMIdentityCenterInstanceArn(iAMIdentityCenterInstanceArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEdition() != null)
sb.append("Edition: ").append(getEdition()).append(",");
if (getAuthenticationMethod() != null)
sb.append("AuthenticationMethod: ").append(getAuthenticationMethod()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getAccountName() != null)
sb.append("AccountName: ").append(getAccountName()).append(",");
if (getNotificationEmail() != null)
sb.append("NotificationEmail: ").append(getNotificationEmail()).append(",");
if (getActiveDirectoryName() != null)
sb.append("ActiveDirectoryName: ").append(getActiveDirectoryName()).append(",");
if (getRealm() != null)
sb.append("Realm: ").append(getRealm()).append(",");
if (getDirectoryId() != null)
sb.append("DirectoryId: ").append(getDirectoryId()).append(",");
if (getAdminGroup() != null)
sb.append("AdminGroup: ").append(getAdminGroup()).append(",");
if (getAuthorGroup() != null)
sb.append("AuthorGroup: ").append(getAuthorGroup()).append(",");
if (getReaderGroup() != null)
sb.append("ReaderGroup: ").append(getReaderGroup()).append(",");
if (getAdminProGroup() != null)
sb.append("AdminProGroup: ").append(getAdminProGroup()).append(",");
if (getAuthorProGroup() != null)
sb.append("AuthorProGroup: ").append(getAuthorProGroup()).append(",");
if (getReaderProGroup() != null)
sb.append("ReaderProGroup: ").append(getReaderProGroup()).append(",");
if (getFirstName() != null)
sb.append("FirstName: ").append(getFirstName()).append(",");
if (getLastName() != null)
sb.append("LastName: ").append(getLastName()).append(",");
if (getEmailAddress() != null)
sb.append("EmailAddress: ").append(getEmailAddress()).append(",");
if (getContactNumber() != null)
sb.append("ContactNumber: ").append(getContactNumber()).append(",");
if (getIAMIdentityCenterInstanceArn() != null)
sb.append("IAMIdentityCenterInstanceArn: ").append(getIAMIdentityCenterInstanceArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAccountSubscriptionRequest == false)
return false;
CreateAccountSubscriptionRequest other = (CreateAccountSubscriptionRequest) obj;
if (other.getEdition() == null ^ this.getEdition() == null)
return false;
if (other.getEdition() != null && other.getEdition().equals(this.getEdition()) == false)
return false;
if (other.getAuthenticationMethod() == null ^ this.getAuthenticationMethod() == null)
return false;
if (other.getAuthenticationMethod() != null && other.getAuthenticationMethod().equals(this.getAuthenticationMethod()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getAccountName() == null ^ this.getAccountName() == null)
return false;
if (other.getAccountName() != null && other.getAccountName().equals(this.getAccountName()) == false)
return false;
if (other.getNotificationEmail() == null ^ this.getNotificationEmail() == null)
return false;
if (other.getNotificationEmail() != null && other.getNotificationEmail().equals(this.getNotificationEmail()) == false)
return false;
if (other.getActiveDirectoryName() == null ^ this.getActiveDirectoryName() == null)
return false;
if (other.getActiveDirectoryName() != null && other.getActiveDirectoryName().equals(this.getActiveDirectoryName()) == false)
return false;
if (other.getRealm() == null ^ this.getRealm() == null)
return false;
if (other.getRealm() != null && other.getRealm().equals(this.getRealm()) == false)
return false;
if (other.getDirectoryId() == null ^ this.getDirectoryId() == null)
return false;
if (other.getDirectoryId() != null && other.getDirectoryId().equals(this.getDirectoryId()) == false)
return false;
if (other.getAdminGroup() == null ^ this.getAdminGroup() == null)
return false;
if (other.getAdminGroup() != null && other.getAdminGroup().equals(this.getAdminGroup()) == false)
return false;
if (other.getAuthorGroup() == null ^ this.getAuthorGroup() == null)
return false;
if (other.getAuthorGroup() != null && other.getAuthorGroup().equals(this.getAuthorGroup()) == false)
return false;
if (other.getReaderGroup() == null ^ this.getReaderGroup() == null)
return false;
if (other.getReaderGroup() != null && other.getReaderGroup().equals(this.getReaderGroup()) == false)
return false;
if (other.getAdminProGroup() == null ^ this.getAdminProGroup() == null)
return false;
if (other.getAdminProGroup() != null && other.getAdminProGroup().equals(this.getAdminProGroup()) == false)
return false;
if (other.getAuthorProGroup() == null ^ this.getAuthorProGroup() == null)
return false;
if (other.getAuthorProGroup() != null && other.getAuthorProGroup().equals(this.getAuthorProGroup()) == false)
return false;
if (other.getReaderProGroup() == null ^ this.getReaderProGroup() == null)
return false;
if (other.getReaderProGroup() != null && other.getReaderProGroup().equals(this.getReaderProGroup()) == false)
return false;
if (other.getFirstName() == null ^ this.getFirstName() == null)
return false;
if (other.getFirstName() != null && other.getFirstName().equals(this.getFirstName()) == false)
return false;
if (other.getLastName() == null ^ this.getLastName() == null)
return false;
if (other.getLastName() != null && other.getLastName().equals(this.getLastName()) == false)
return false;
if (other.getEmailAddress() == null ^ this.getEmailAddress() == null)
return false;
if (other.getEmailAddress() != null && other.getEmailAddress().equals(this.getEmailAddress()) == false)
return false;
if (other.getContactNumber() == null ^ this.getContactNumber() == null)
return false;
if (other.getContactNumber() != null && other.getContactNumber().equals(this.getContactNumber()) == false)
return false;
if (other.getIAMIdentityCenterInstanceArn() == null ^ this.getIAMIdentityCenterInstanceArn() == null)
return false;
if (other.getIAMIdentityCenterInstanceArn() != null && other.getIAMIdentityCenterInstanceArn().equals(this.getIAMIdentityCenterInstanceArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEdition() == null) ? 0 : getEdition().hashCode());
hashCode = prime * hashCode + ((getAuthenticationMethod() == null) ? 0 : getAuthenticationMethod().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getAccountName() == null) ? 0 : getAccountName().hashCode());
hashCode = prime * hashCode + ((getNotificationEmail() == null) ? 0 : getNotificationEmail().hashCode());
hashCode = prime * hashCode + ((getActiveDirectoryName() == null) ? 0 : getActiveDirectoryName().hashCode());
hashCode = prime * hashCode + ((getRealm() == null) ? 0 : getRealm().hashCode());
hashCode = prime * hashCode + ((getDirectoryId() == null) ? 0 : getDirectoryId().hashCode());
hashCode = prime * hashCode + ((getAdminGroup() == null) ? 0 : getAdminGroup().hashCode());
hashCode = prime * hashCode + ((getAuthorGroup() == null) ? 0 : getAuthorGroup().hashCode());
hashCode = prime * hashCode + ((getReaderGroup() == null) ? 0 : getReaderGroup().hashCode());
hashCode = prime * hashCode + ((getAdminProGroup() == null) ? 0 : getAdminProGroup().hashCode());
hashCode = prime * hashCode + ((getAuthorProGroup() == null) ? 0 : getAuthorProGroup().hashCode());
hashCode = prime * hashCode + ((getReaderProGroup() == null) ? 0 : getReaderProGroup().hashCode());
hashCode = prime * hashCode + ((getFirstName() == null) ? 0 : getFirstName().hashCode());
hashCode = prime * hashCode + ((getLastName() == null) ? 0 : getLastName().hashCode());
hashCode = prime * hashCode + ((getEmailAddress() == null) ? 0 : getEmailAddress().hashCode());
hashCode = prime * hashCode + ((getContactNumber() == null) ? 0 : getContactNumber().hashCode());
hashCode = prime * hashCode + ((getIAMIdentityCenterInstanceArn() == null) ? 0 : getIAMIdentityCenterInstanceArn().hashCode());
return hashCode;
}
@Override
public CreateAccountSubscriptionRequest clone() {
return (CreateAccountSubscriptionRequest) super.clone();
}
}