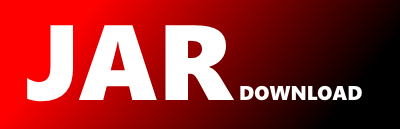
com.amazonaws.services.quicksight.model.DataLabelOptions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The options that determine the presentation of the data labels.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataLabelOptions implements Serializable, Cloneable, StructuredPojo {
/**
*
* Determines the visibility of the data labels.
*
*/
private String visibility;
/**
*
* Determines the visibility of the category field labels.
*
*/
private String categoryLabelVisibility;
/**
*
* Determines the visibility of the measure field labels.
*
*/
private String measureLabelVisibility;
/**
*
* The option that determines the data label type.
*
*/
private java.util.List dataLabelTypes;
/**
*
* Determines the position of the data labels.
*
*/
private String position;
/**
*
* Determines the content of the data labels.
*
*/
private String labelContent;
/**
*
* Determines the font configuration of the data labels.
*
*/
private FontConfiguration labelFontConfiguration;
/**
*
* Determines the color of the data labels.
*
*/
private String labelColor;
/**
*
* Determines whether overlap is enabled or disabled for the data labels.
*
*/
private String overlap;
/**
*
* Determines the visibility of the total.
*
*/
private String totalsVisibility;
/**
*
* Determines the visibility of the data labels.
*
*
* @param visibility
* Determines the visibility of the data labels.
* @see Visibility
*/
public void setVisibility(String visibility) {
this.visibility = visibility;
}
/**
*
* Determines the visibility of the data labels.
*
*
* @return Determines the visibility of the data labels.
* @see Visibility
*/
public String getVisibility() {
return this.visibility;
}
/**
*
* Determines the visibility of the data labels.
*
*
* @param visibility
* Determines the visibility of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withVisibility(String visibility) {
setVisibility(visibility);
return this;
}
/**
*
* Determines the visibility of the data labels.
*
*
* @param visibility
* Determines the visibility of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withVisibility(Visibility visibility) {
this.visibility = visibility.toString();
return this;
}
/**
*
* Determines the visibility of the category field labels.
*
*
* @param categoryLabelVisibility
* Determines the visibility of the category field labels.
* @see Visibility
*/
public void setCategoryLabelVisibility(String categoryLabelVisibility) {
this.categoryLabelVisibility = categoryLabelVisibility;
}
/**
*
* Determines the visibility of the category field labels.
*
*
* @return Determines the visibility of the category field labels.
* @see Visibility
*/
public String getCategoryLabelVisibility() {
return this.categoryLabelVisibility;
}
/**
*
* Determines the visibility of the category field labels.
*
*
* @param categoryLabelVisibility
* Determines the visibility of the category field labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withCategoryLabelVisibility(String categoryLabelVisibility) {
setCategoryLabelVisibility(categoryLabelVisibility);
return this;
}
/**
*
* Determines the visibility of the category field labels.
*
*
* @param categoryLabelVisibility
* Determines the visibility of the category field labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withCategoryLabelVisibility(Visibility categoryLabelVisibility) {
this.categoryLabelVisibility = categoryLabelVisibility.toString();
return this;
}
/**
*
* Determines the visibility of the measure field labels.
*
*
* @param measureLabelVisibility
* Determines the visibility of the measure field labels.
* @see Visibility
*/
public void setMeasureLabelVisibility(String measureLabelVisibility) {
this.measureLabelVisibility = measureLabelVisibility;
}
/**
*
* Determines the visibility of the measure field labels.
*
*
* @return Determines the visibility of the measure field labels.
* @see Visibility
*/
public String getMeasureLabelVisibility() {
return this.measureLabelVisibility;
}
/**
*
* Determines the visibility of the measure field labels.
*
*
* @param measureLabelVisibility
* Determines the visibility of the measure field labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withMeasureLabelVisibility(String measureLabelVisibility) {
setMeasureLabelVisibility(measureLabelVisibility);
return this;
}
/**
*
* Determines the visibility of the measure field labels.
*
*
* @param measureLabelVisibility
* Determines the visibility of the measure field labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withMeasureLabelVisibility(Visibility measureLabelVisibility) {
this.measureLabelVisibility = measureLabelVisibility.toString();
return this;
}
/**
*
* The option that determines the data label type.
*
*
* @return The option that determines the data label type.
*/
public java.util.List getDataLabelTypes() {
return dataLabelTypes;
}
/**
*
* The option that determines the data label type.
*
*
* @param dataLabelTypes
* The option that determines the data label type.
*/
public void setDataLabelTypes(java.util.Collection dataLabelTypes) {
if (dataLabelTypes == null) {
this.dataLabelTypes = null;
return;
}
this.dataLabelTypes = new java.util.ArrayList(dataLabelTypes);
}
/**
*
* The option that determines the data label type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataLabelTypes(java.util.Collection)} or {@link #withDataLabelTypes(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param dataLabelTypes
* The option that determines the data label type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataLabelOptions withDataLabelTypes(DataLabelType... dataLabelTypes) {
if (this.dataLabelTypes == null) {
setDataLabelTypes(new java.util.ArrayList(dataLabelTypes.length));
}
for (DataLabelType ele : dataLabelTypes) {
this.dataLabelTypes.add(ele);
}
return this;
}
/**
*
* The option that determines the data label type.
*
*
* @param dataLabelTypes
* The option that determines the data label type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataLabelOptions withDataLabelTypes(java.util.Collection dataLabelTypes) {
setDataLabelTypes(dataLabelTypes);
return this;
}
/**
*
* Determines the position of the data labels.
*
*
* @param position
* Determines the position of the data labels.
* @see DataLabelPosition
*/
public void setPosition(String position) {
this.position = position;
}
/**
*
* Determines the position of the data labels.
*
*
* @return Determines the position of the data labels.
* @see DataLabelPosition
*/
public String getPosition() {
return this.position;
}
/**
*
* Determines the position of the data labels.
*
*
* @param position
* Determines the position of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelPosition
*/
public DataLabelOptions withPosition(String position) {
setPosition(position);
return this;
}
/**
*
* Determines the position of the data labels.
*
*
* @param position
* Determines the position of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelPosition
*/
public DataLabelOptions withPosition(DataLabelPosition position) {
this.position = position.toString();
return this;
}
/**
*
* Determines the content of the data labels.
*
*
* @param labelContent
* Determines the content of the data labels.
* @see DataLabelContent
*/
public void setLabelContent(String labelContent) {
this.labelContent = labelContent;
}
/**
*
* Determines the content of the data labels.
*
*
* @return Determines the content of the data labels.
* @see DataLabelContent
*/
public String getLabelContent() {
return this.labelContent;
}
/**
*
* Determines the content of the data labels.
*
*
* @param labelContent
* Determines the content of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelContent
*/
public DataLabelOptions withLabelContent(String labelContent) {
setLabelContent(labelContent);
return this;
}
/**
*
* Determines the content of the data labels.
*
*
* @param labelContent
* Determines the content of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelContent
*/
public DataLabelOptions withLabelContent(DataLabelContent labelContent) {
this.labelContent = labelContent.toString();
return this;
}
/**
*
* Determines the font configuration of the data labels.
*
*
* @param labelFontConfiguration
* Determines the font configuration of the data labels.
*/
public void setLabelFontConfiguration(FontConfiguration labelFontConfiguration) {
this.labelFontConfiguration = labelFontConfiguration;
}
/**
*
* Determines the font configuration of the data labels.
*
*
* @return Determines the font configuration of the data labels.
*/
public FontConfiguration getLabelFontConfiguration() {
return this.labelFontConfiguration;
}
/**
*
* Determines the font configuration of the data labels.
*
*
* @param labelFontConfiguration
* Determines the font configuration of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataLabelOptions withLabelFontConfiguration(FontConfiguration labelFontConfiguration) {
setLabelFontConfiguration(labelFontConfiguration);
return this;
}
/**
*
* Determines the color of the data labels.
*
*
* @param labelColor
* Determines the color of the data labels.
*/
public void setLabelColor(String labelColor) {
this.labelColor = labelColor;
}
/**
*
* Determines the color of the data labels.
*
*
* @return Determines the color of the data labels.
*/
public String getLabelColor() {
return this.labelColor;
}
/**
*
* Determines the color of the data labels.
*
*
* @param labelColor
* Determines the color of the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataLabelOptions withLabelColor(String labelColor) {
setLabelColor(labelColor);
return this;
}
/**
*
* Determines whether overlap is enabled or disabled for the data labels.
*
*
* @param overlap
* Determines whether overlap is enabled or disabled for the data labels.
* @see DataLabelOverlap
*/
public void setOverlap(String overlap) {
this.overlap = overlap;
}
/**
*
* Determines whether overlap is enabled or disabled for the data labels.
*
*
* @return Determines whether overlap is enabled or disabled for the data labels.
* @see DataLabelOverlap
*/
public String getOverlap() {
return this.overlap;
}
/**
*
* Determines whether overlap is enabled or disabled for the data labels.
*
*
* @param overlap
* Determines whether overlap is enabled or disabled for the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelOverlap
*/
public DataLabelOptions withOverlap(String overlap) {
setOverlap(overlap);
return this;
}
/**
*
* Determines whether overlap is enabled or disabled for the data labels.
*
*
* @param overlap
* Determines whether overlap is enabled or disabled for the data labels.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataLabelOverlap
*/
public DataLabelOptions withOverlap(DataLabelOverlap overlap) {
this.overlap = overlap.toString();
return this;
}
/**
*
* Determines the visibility of the total.
*
*
* @param totalsVisibility
* Determines the visibility of the total.
* @see Visibility
*/
public void setTotalsVisibility(String totalsVisibility) {
this.totalsVisibility = totalsVisibility;
}
/**
*
* Determines the visibility of the total.
*
*
* @return Determines the visibility of the total.
* @see Visibility
*/
public String getTotalsVisibility() {
return this.totalsVisibility;
}
/**
*
* Determines the visibility of the total.
*
*
* @param totalsVisibility
* Determines the visibility of the total.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withTotalsVisibility(String totalsVisibility) {
setTotalsVisibility(totalsVisibility);
return this;
}
/**
*
* Determines the visibility of the total.
*
*
* @param totalsVisibility
* Determines the visibility of the total.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public DataLabelOptions withTotalsVisibility(Visibility totalsVisibility) {
this.totalsVisibility = totalsVisibility.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVisibility() != null)
sb.append("Visibility: ").append(getVisibility()).append(",");
if (getCategoryLabelVisibility() != null)
sb.append("CategoryLabelVisibility: ").append(getCategoryLabelVisibility()).append(",");
if (getMeasureLabelVisibility() != null)
sb.append("MeasureLabelVisibility: ").append(getMeasureLabelVisibility()).append(",");
if (getDataLabelTypes() != null)
sb.append("DataLabelTypes: ").append(getDataLabelTypes()).append(",");
if (getPosition() != null)
sb.append("Position: ").append(getPosition()).append(",");
if (getLabelContent() != null)
sb.append("LabelContent: ").append(getLabelContent()).append(",");
if (getLabelFontConfiguration() != null)
sb.append("LabelFontConfiguration: ").append(getLabelFontConfiguration()).append(",");
if (getLabelColor() != null)
sb.append("LabelColor: ").append(getLabelColor()).append(",");
if (getOverlap() != null)
sb.append("Overlap: ").append(getOverlap()).append(",");
if (getTotalsVisibility() != null)
sb.append("TotalsVisibility: ").append(getTotalsVisibility());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataLabelOptions == false)
return false;
DataLabelOptions other = (DataLabelOptions) obj;
if (other.getVisibility() == null ^ this.getVisibility() == null)
return false;
if (other.getVisibility() != null && other.getVisibility().equals(this.getVisibility()) == false)
return false;
if (other.getCategoryLabelVisibility() == null ^ this.getCategoryLabelVisibility() == null)
return false;
if (other.getCategoryLabelVisibility() != null && other.getCategoryLabelVisibility().equals(this.getCategoryLabelVisibility()) == false)
return false;
if (other.getMeasureLabelVisibility() == null ^ this.getMeasureLabelVisibility() == null)
return false;
if (other.getMeasureLabelVisibility() != null && other.getMeasureLabelVisibility().equals(this.getMeasureLabelVisibility()) == false)
return false;
if (other.getDataLabelTypes() == null ^ this.getDataLabelTypes() == null)
return false;
if (other.getDataLabelTypes() != null && other.getDataLabelTypes().equals(this.getDataLabelTypes()) == false)
return false;
if (other.getPosition() == null ^ this.getPosition() == null)
return false;
if (other.getPosition() != null && other.getPosition().equals(this.getPosition()) == false)
return false;
if (other.getLabelContent() == null ^ this.getLabelContent() == null)
return false;
if (other.getLabelContent() != null && other.getLabelContent().equals(this.getLabelContent()) == false)
return false;
if (other.getLabelFontConfiguration() == null ^ this.getLabelFontConfiguration() == null)
return false;
if (other.getLabelFontConfiguration() != null && other.getLabelFontConfiguration().equals(this.getLabelFontConfiguration()) == false)
return false;
if (other.getLabelColor() == null ^ this.getLabelColor() == null)
return false;
if (other.getLabelColor() != null && other.getLabelColor().equals(this.getLabelColor()) == false)
return false;
if (other.getOverlap() == null ^ this.getOverlap() == null)
return false;
if (other.getOverlap() != null && other.getOverlap().equals(this.getOverlap()) == false)
return false;
if (other.getTotalsVisibility() == null ^ this.getTotalsVisibility() == null)
return false;
if (other.getTotalsVisibility() != null && other.getTotalsVisibility().equals(this.getTotalsVisibility()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVisibility() == null) ? 0 : getVisibility().hashCode());
hashCode = prime * hashCode + ((getCategoryLabelVisibility() == null) ? 0 : getCategoryLabelVisibility().hashCode());
hashCode = prime * hashCode + ((getMeasureLabelVisibility() == null) ? 0 : getMeasureLabelVisibility().hashCode());
hashCode = prime * hashCode + ((getDataLabelTypes() == null) ? 0 : getDataLabelTypes().hashCode());
hashCode = prime * hashCode + ((getPosition() == null) ? 0 : getPosition().hashCode());
hashCode = prime * hashCode + ((getLabelContent() == null) ? 0 : getLabelContent().hashCode());
hashCode = prime * hashCode + ((getLabelFontConfiguration() == null) ? 0 : getLabelFontConfiguration().hashCode());
hashCode = prime * hashCode + ((getLabelColor() == null) ? 0 : getLabelColor().hashCode());
hashCode = prime * hashCode + ((getOverlap() == null) ? 0 : getOverlap().hashCode());
hashCode = prime * hashCode + ((getTotalsVisibility() == null) ? 0 : getTotalsVisibility().hashCode());
return hashCode;
}
@Override
public DataLabelOptions clone() {
try {
return (DataLabelOptions) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.DataLabelOptionsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}