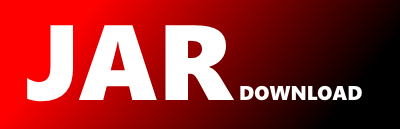
com.amazonaws.services.quicksight.model.TimeBasedForecastProperties Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The forecast properties setup of a forecast in the line chart.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TimeBasedForecastProperties implements Serializable, Cloneable, StructuredPojo {
/**
*
* The periods forward setup of a forecast computation.
*
*/
private Integer periodsForward;
/**
*
* The periods backward setup of a forecast computation.
*
*/
private Integer periodsBackward;
/**
*
* The upper boundary setup of a forecast computation.
*
*/
private Double upperBoundary;
/**
*
* The lower boundary setup of a forecast computation.
*
*/
private Double lowerBoundary;
/**
*
* The prediction interval setup of a forecast computation.
*
*/
private Integer predictionInterval;
/**
*
* The seasonality setup of a forecast computation. Choose one of the following options:
*
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*
*/
private Integer seasonality;
/**
*
* The periods forward setup of a forecast computation.
*
*
* @param periodsForward
* The periods forward setup of a forecast computation.
*/
public void setPeriodsForward(Integer periodsForward) {
this.periodsForward = periodsForward;
}
/**
*
* The periods forward setup of a forecast computation.
*
*
* @return The periods forward setup of a forecast computation.
*/
public Integer getPeriodsForward() {
return this.periodsForward;
}
/**
*
* The periods forward setup of a forecast computation.
*
*
* @param periodsForward
* The periods forward setup of a forecast computation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withPeriodsForward(Integer periodsForward) {
setPeriodsForward(periodsForward);
return this;
}
/**
*
* The periods backward setup of a forecast computation.
*
*
* @param periodsBackward
* The periods backward setup of a forecast computation.
*/
public void setPeriodsBackward(Integer periodsBackward) {
this.periodsBackward = periodsBackward;
}
/**
*
* The periods backward setup of a forecast computation.
*
*
* @return The periods backward setup of a forecast computation.
*/
public Integer getPeriodsBackward() {
return this.periodsBackward;
}
/**
*
* The periods backward setup of a forecast computation.
*
*
* @param periodsBackward
* The periods backward setup of a forecast computation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withPeriodsBackward(Integer periodsBackward) {
setPeriodsBackward(periodsBackward);
return this;
}
/**
*
* The upper boundary setup of a forecast computation.
*
*
* @param upperBoundary
* The upper boundary setup of a forecast computation.
*/
public void setUpperBoundary(Double upperBoundary) {
this.upperBoundary = upperBoundary;
}
/**
*
* The upper boundary setup of a forecast computation.
*
*
* @return The upper boundary setup of a forecast computation.
*/
public Double getUpperBoundary() {
return this.upperBoundary;
}
/**
*
* The upper boundary setup of a forecast computation.
*
*
* @param upperBoundary
* The upper boundary setup of a forecast computation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withUpperBoundary(Double upperBoundary) {
setUpperBoundary(upperBoundary);
return this;
}
/**
*
* The lower boundary setup of a forecast computation.
*
*
* @param lowerBoundary
* The lower boundary setup of a forecast computation.
*/
public void setLowerBoundary(Double lowerBoundary) {
this.lowerBoundary = lowerBoundary;
}
/**
*
* The lower boundary setup of a forecast computation.
*
*
* @return The lower boundary setup of a forecast computation.
*/
public Double getLowerBoundary() {
return this.lowerBoundary;
}
/**
*
* The lower boundary setup of a forecast computation.
*
*
* @param lowerBoundary
* The lower boundary setup of a forecast computation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withLowerBoundary(Double lowerBoundary) {
setLowerBoundary(lowerBoundary);
return this;
}
/**
*
* The prediction interval setup of a forecast computation.
*
*
* @param predictionInterval
* The prediction interval setup of a forecast computation.
*/
public void setPredictionInterval(Integer predictionInterval) {
this.predictionInterval = predictionInterval;
}
/**
*
* The prediction interval setup of a forecast computation.
*
*
* @return The prediction interval setup of a forecast computation.
*/
public Integer getPredictionInterval() {
return this.predictionInterval;
}
/**
*
* The prediction interval setup of a forecast computation.
*
*
* @param predictionInterval
* The prediction interval setup of a forecast computation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withPredictionInterval(Integer predictionInterval) {
setPredictionInterval(predictionInterval);
return this;
}
/**
*
* The seasonality setup of a forecast computation. Choose one of the following options:
*
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*
*
* @param seasonality
* The seasonality setup of a forecast computation. Choose one of the following options:
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*/
public void setSeasonality(Integer seasonality) {
this.seasonality = seasonality;
}
/**
*
* The seasonality setup of a forecast computation. Choose one of the following options:
*
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*
*
* @return The seasonality setup of a forecast computation. Choose one of the following options:
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*/
public Integer getSeasonality() {
return this.seasonality;
}
/**
*
* The seasonality setup of a forecast computation. Choose one of the following options:
*
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
*
*
* @param seasonality
* The seasonality setup of a forecast computation. Choose one of the following options:
*
* -
*
* NULL
: The input is set to NULL
.
*
*
* -
*
* NON_NULL
: The input is set to a custom value.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TimeBasedForecastProperties withSeasonality(Integer seasonality) {
setSeasonality(seasonality);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPeriodsForward() != null)
sb.append("PeriodsForward: ").append(getPeriodsForward()).append(",");
if (getPeriodsBackward() != null)
sb.append("PeriodsBackward: ").append(getPeriodsBackward()).append(",");
if (getUpperBoundary() != null)
sb.append("UpperBoundary: ").append(getUpperBoundary()).append(",");
if (getLowerBoundary() != null)
sb.append("LowerBoundary: ").append(getLowerBoundary()).append(",");
if (getPredictionInterval() != null)
sb.append("PredictionInterval: ").append(getPredictionInterval()).append(",");
if (getSeasonality() != null)
sb.append("Seasonality: ").append(getSeasonality());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TimeBasedForecastProperties == false)
return false;
TimeBasedForecastProperties other = (TimeBasedForecastProperties) obj;
if (other.getPeriodsForward() == null ^ this.getPeriodsForward() == null)
return false;
if (other.getPeriodsForward() != null && other.getPeriodsForward().equals(this.getPeriodsForward()) == false)
return false;
if (other.getPeriodsBackward() == null ^ this.getPeriodsBackward() == null)
return false;
if (other.getPeriodsBackward() != null && other.getPeriodsBackward().equals(this.getPeriodsBackward()) == false)
return false;
if (other.getUpperBoundary() == null ^ this.getUpperBoundary() == null)
return false;
if (other.getUpperBoundary() != null && other.getUpperBoundary().equals(this.getUpperBoundary()) == false)
return false;
if (other.getLowerBoundary() == null ^ this.getLowerBoundary() == null)
return false;
if (other.getLowerBoundary() != null && other.getLowerBoundary().equals(this.getLowerBoundary()) == false)
return false;
if (other.getPredictionInterval() == null ^ this.getPredictionInterval() == null)
return false;
if (other.getPredictionInterval() != null && other.getPredictionInterval().equals(this.getPredictionInterval()) == false)
return false;
if (other.getSeasonality() == null ^ this.getSeasonality() == null)
return false;
if (other.getSeasonality() != null && other.getSeasonality().equals(this.getSeasonality()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPeriodsForward() == null) ? 0 : getPeriodsForward().hashCode());
hashCode = prime * hashCode + ((getPeriodsBackward() == null) ? 0 : getPeriodsBackward().hashCode());
hashCode = prime * hashCode + ((getUpperBoundary() == null) ? 0 : getUpperBoundary().hashCode());
hashCode = prime * hashCode + ((getLowerBoundary() == null) ? 0 : getLowerBoundary().hashCode());
hashCode = prime * hashCode + ((getPredictionInterval() == null) ? 0 : getPredictionInterval().hashCode());
hashCode = prime * hashCode + ((getSeasonality() == null) ? 0 : getSeasonality().hashCode());
return hashCode;
}
@Override
public TimeBasedForecastProperties clone() {
try {
return (TimeBasedForecastProperties) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.TimeBasedForecastPropertiesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}