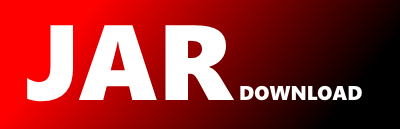
com.amazonaws.services.quicksight.model.TopicColumn Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Represents a column in a dataset.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TopicColumn implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the column.
*
*/
private String columnName;
/**
*
* A user-friendly name for the column.
*
*/
private String columnFriendlyName;
/**
*
* A description of the column and its contents.
*
*/
private String columnDescription;
/**
*
* The other names or aliases for the column.
*
*/
private java.util.List columnSynonyms;
/**
*
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
*
*/
private String columnDataRole;
/**
*
* The type of aggregation that is performed on the column data when it's queried.
*
*/
private String aggregation;
/**
*
* A Boolean value that indicates whether the column is included in the query results.
*
*/
private Boolean isIncludedInTopic;
/**
*
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*
*/
private Boolean disableIndexing;
/**
*
* The order in which data is displayed for the column when it's used in a comparative context.
*
*/
private ComparativeOrder comparativeOrder;
/**
*
* The semantic type of data contained in the column.
*
*/
private SemanticType semanticType;
/**
*
* The level of time precision that is used to aggregate DateTime
values.
*
*/
private String timeGranularity;
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*/
private java.util.List allowedAggregations;
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*/
private java.util.List notAllowedAggregations;
/**
*
* The default formatting used for values in the column.
*
*/
private DefaultFormatting defaultFormatting;
/**
*
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*
*/
private Boolean neverAggregateInFilter;
/**
*
* The other names or aliases for the column cell value.
*
*/
private java.util.List cellValueSynonyms;
/**
*
* The non additive value for the column.
*
*/
private Boolean nonAdditive;
/**
*
* The name of the column.
*
*
* @param columnName
* The name of the column.
*/
public void setColumnName(String columnName) {
this.columnName = columnName;
}
/**
*
* The name of the column.
*
*
* @return The name of the column.
*/
public String getColumnName() {
return this.columnName;
}
/**
*
* The name of the column.
*
*
* @param columnName
* The name of the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withColumnName(String columnName) {
setColumnName(columnName);
return this;
}
/**
*
* A user-friendly name for the column.
*
*
* @param columnFriendlyName
* A user-friendly name for the column.
*/
public void setColumnFriendlyName(String columnFriendlyName) {
this.columnFriendlyName = columnFriendlyName;
}
/**
*
* A user-friendly name for the column.
*
*
* @return A user-friendly name for the column.
*/
public String getColumnFriendlyName() {
return this.columnFriendlyName;
}
/**
*
* A user-friendly name for the column.
*
*
* @param columnFriendlyName
* A user-friendly name for the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withColumnFriendlyName(String columnFriendlyName) {
setColumnFriendlyName(columnFriendlyName);
return this;
}
/**
*
* A description of the column and its contents.
*
*
* @param columnDescription
* A description of the column and its contents.
*/
public void setColumnDescription(String columnDescription) {
this.columnDescription = columnDescription;
}
/**
*
* A description of the column and its contents.
*
*
* @return A description of the column and its contents.
*/
public String getColumnDescription() {
return this.columnDescription;
}
/**
*
* A description of the column and its contents.
*
*
* @param columnDescription
* A description of the column and its contents.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withColumnDescription(String columnDescription) {
setColumnDescription(columnDescription);
return this;
}
/**
*
* The other names or aliases for the column.
*
*
* @return The other names or aliases for the column.
*/
public java.util.List getColumnSynonyms() {
return columnSynonyms;
}
/**
*
* The other names or aliases for the column.
*
*
* @param columnSynonyms
* The other names or aliases for the column.
*/
public void setColumnSynonyms(java.util.Collection columnSynonyms) {
if (columnSynonyms == null) {
this.columnSynonyms = null;
return;
}
this.columnSynonyms = new java.util.ArrayList(columnSynonyms);
}
/**
*
* The other names or aliases for the column.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setColumnSynonyms(java.util.Collection)} or {@link #withColumnSynonyms(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param columnSynonyms
* The other names or aliases for the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withColumnSynonyms(String... columnSynonyms) {
if (this.columnSynonyms == null) {
setColumnSynonyms(new java.util.ArrayList(columnSynonyms.length));
}
for (String ele : columnSynonyms) {
this.columnSynonyms.add(ele);
}
return this;
}
/**
*
* The other names or aliases for the column.
*
*
* @param columnSynonyms
* The other names or aliases for the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withColumnSynonyms(java.util.Collection columnSynonyms) {
setColumnSynonyms(columnSynonyms);
return this;
}
/**
*
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
*
*
* @param columnDataRole
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
* @see ColumnDataRole
*/
public void setColumnDataRole(String columnDataRole) {
this.columnDataRole = columnDataRole;
}
/**
*
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
*
*
* @return The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
* @see ColumnDataRole
*/
public String getColumnDataRole() {
return this.columnDataRole;
}
/**
*
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
*
*
* @param columnDataRole
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ColumnDataRole
*/
public TopicColumn withColumnDataRole(String columnDataRole) {
setColumnDataRole(columnDataRole);
return this;
}
/**
*
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
*
*
* @param columnDataRole
* The role of the column in the data. Valid values are DIMENSION
and MEASURE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ColumnDataRole
*/
public TopicColumn withColumnDataRole(ColumnDataRole columnDataRole) {
this.columnDataRole = columnDataRole.toString();
return this;
}
/**
*
* The type of aggregation that is performed on the column data when it's queried.
*
*
* @param aggregation
* The type of aggregation that is performed on the column data when it's queried.
* @see DefaultAggregation
*/
public void setAggregation(String aggregation) {
this.aggregation = aggregation;
}
/**
*
* The type of aggregation that is performed on the column data when it's queried.
*
*
* @return The type of aggregation that is performed on the column data when it's queried.
* @see DefaultAggregation
*/
public String getAggregation() {
return this.aggregation;
}
/**
*
* The type of aggregation that is performed on the column data when it's queried.
*
*
* @param aggregation
* The type of aggregation that is performed on the column data when it's queried.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultAggregation
*/
public TopicColumn withAggregation(String aggregation) {
setAggregation(aggregation);
return this;
}
/**
*
* The type of aggregation that is performed on the column data when it's queried.
*
*
* @param aggregation
* The type of aggregation that is performed on the column data when it's queried.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultAggregation
*/
public TopicColumn withAggregation(DefaultAggregation aggregation) {
this.aggregation = aggregation.toString();
return this;
}
/**
*
* A Boolean value that indicates whether the column is included in the query results.
*
*
* @param isIncludedInTopic
* A Boolean value that indicates whether the column is included in the query results.
*/
public void setIsIncludedInTopic(Boolean isIncludedInTopic) {
this.isIncludedInTopic = isIncludedInTopic;
}
/**
*
* A Boolean value that indicates whether the column is included in the query results.
*
*
* @return A Boolean value that indicates whether the column is included in the query results.
*/
public Boolean getIsIncludedInTopic() {
return this.isIncludedInTopic;
}
/**
*
* A Boolean value that indicates whether the column is included in the query results.
*
*
* @param isIncludedInTopic
* A Boolean value that indicates whether the column is included in the query results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withIsIncludedInTopic(Boolean isIncludedInTopic) {
setIsIncludedInTopic(isIncludedInTopic);
return this;
}
/**
*
* A Boolean value that indicates whether the column is included in the query results.
*
*
* @return A Boolean value that indicates whether the column is included in the query results.
*/
public Boolean isIncludedInTopic() {
return this.isIncludedInTopic;
}
/**
*
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*
*
* @param disableIndexing
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*/
public void setDisableIndexing(Boolean disableIndexing) {
this.disableIndexing = disableIndexing;
}
/**
*
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*
*
* @return A Boolean value that indicates whether the column shows in the autocomplete functionality.
*/
public Boolean getDisableIndexing() {
return this.disableIndexing;
}
/**
*
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*
*
* @param disableIndexing
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withDisableIndexing(Boolean disableIndexing) {
setDisableIndexing(disableIndexing);
return this;
}
/**
*
* A Boolean value that indicates whether the column shows in the autocomplete functionality.
*
*
* @return A Boolean value that indicates whether the column shows in the autocomplete functionality.
*/
public Boolean isDisableIndexing() {
return this.disableIndexing;
}
/**
*
* The order in which data is displayed for the column when it's used in a comparative context.
*
*
* @param comparativeOrder
* The order in which data is displayed for the column when it's used in a comparative context.
*/
public void setComparativeOrder(ComparativeOrder comparativeOrder) {
this.comparativeOrder = comparativeOrder;
}
/**
*
* The order in which data is displayed for the column when it's used in a comparative context.
*
*
* @return The order in which data is displayed for the column when it's used in a comparative context.
*/
public ComparativeOrder getComparativeOrder() {
return this.comparativeOrder;
}
/**
*
* The order in which data is displayed for the column when it's used in a comparative context.
*
*
* @param comparativeOrder
* The order in which data is displayed for the column when it's used in a comparative context.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withComparativeOrder(ComparativeOrder comparativeOrder) {
setComparativeOrder(comparativeOrder);
return this;
}
/**
*
* The semantic type of data contained in the column.
*
*
* @param semanticType
* The semantic type of data contained in the column.
*/
public void setSemanticType(SemanticType semanticType) {
this.semanticType = semanticType;
}
/**
*
* The semantic type of data contained in the column.
*
*
* @return The semantic type of data contained in the column.
*/
public SemanticType getSemanticType() {
return this.semanticType;
}
/**
*
* The semantic type of data contained in the column.
*
*
* @param semanticType
* The semantic type of data contained in the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withSemanticType(SemanticType semanticType) {
setSemanticType(semanticType);
return this;
}
/**
*
* The level of time precision that is used to aggregate DateTime
values.
*
*
* @param timeGranularity
* The level of time precision that is used to aggregate DateTime
values.
* @see TopicTimeGranularity
*/
public void setTimeGranularity(String timeGranularity) {
this.timeGranularity = timeGranularity;
}
/**
*
* The level of time precision that is used to aggregate DateTime
values.
*
*
* @return The level of time precision that is used to aggregate DateTime
values.
* @see TopicTimeGranularity
*/
public String getTimeGranularity() {
return this.timeGranularity;
}
/**
*
* The level of time precision that is used to aggregate DateTime
values.
*
*
* @param timeGranularity
* The level of time precision that is used to aggregate DateTime
values.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TopicTimeGranularity
*/
public TopicColumn withTimeGranularity(String timeGranularity) {
setTimeGranularity(timeGranularity);
return this;
}
/**
*
* The level of time precision that is used to aggregate DateTime
values.
*
*
* @param timeGranularity
* The level of time precision that is used to aggregate DateTime
values.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TopicTimeGranularity
*/
public TopicColumn withTimeGranularity(TopicTimeGranularity timeGranularity) {
this.timeGranularity = timeGranularity.toString();
return this;
}
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @return The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @see AuthorSpecifiedAggregation
*/
public java.util.List getAllowedAggregations() {
return allowedAggregations;
}
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param allowedAggregations
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @see AuthorSpecifiedAggregation
*/
public void setAllowedAggregations(java.util.Collection allowedAggregations) {
if (allowedAggregations == null) {
this.allowedAggregations = null;
return;
}
this.allowedAggregations = new java.util.ArrayList(allowedAggregations);
}
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowedAggregations(java.util.Collection)} or {@link #withAllowedAggregations(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param allowedAggregations
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withAllowedAggregations(String... allowedAggregations) {
if (this.allowedAggregations == null) {
setAllowedAggregations(new java.util.ArrayList(allowedAggregations.length));
}
for (String ele : allowedAggregations) {
this.allowedAggregations.add(ele);
}
return this;
}
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param allowedAggregations
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withAllowedAggregations(java.util.Collection allowedAggregations) {
setAllowedAggregations(allowedAggregations);
return this;
}
/**
*
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param allowedAggregations
* The list of aggregation types that are allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withAllowedAggregations(AuthorSpecifiedAggregation... allowedAggregations) {
java.util.ArrayList allowedAggregationsCopy = new java.util.ArrayList(allowedAggregations.length);
for (AuthorSpecifiedAggregation value : allowedAggregations) {
allowedAggregationsCopy.add(value.toString());
}
if (getAllowedAggregations() == null) {
setAllowedAggregations(allowedAggregationsCopy);
} else {
getAllowedAggregations().addAll(allowedAggregationsCopy);
}
return this;
}
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @return The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @see AuthorSpecifiedAggregation
*/
public java.util.List getNotAllowedAggregations() {
return notAllowedAggregations;
}
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param notAllowedAggregations
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @see AuthorSpecifiedAggregation
*/
public void setNotAllowedAggregations(java.util.Collection notAllowedAggregations) {
if (notAllowedAggregations == null) {
this.notAllowedAggregations = null;
return;
}
this.notAllowedAggregations = new java.util.ArrayList(notAllowedAggregations);
}
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNotAllowedAggregations(java.util.Collection)} or
* {@link #withNotAllowedAggregations(java.util.Collection)} if you want to override the existing values.
*
*
* @param notAllowedAggregations
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withNotAllowedAggregations(String... notAllowedAggregations) {
if (this.notAllowedAggregations == null) {
setNotAllowedAggregations(new java.util.ArrayList(notAllowedAggregations.length));
}
for (String ele : notAllowedAggregations) {
this.notAllowedAggregations.add(ele);
}
return this;
}
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param notAllowedAggregations
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withNotAllowedAggregations(java.util.Collection notAllowedAggregations) {
setNotAllowedAggregations(notAllowedAggregations);
return this;
}
/**
*
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
*
*
* @param notAllowedAggregations
* The list of aggregation types that are not allowed for the column. Valid values for this structure are
* COUNT
, DISTINCT_COUNT
, MIN
, MAX
, MEDIAN
,
* SUM
, AVERAGE
, STDEV
, STDEVP
, VAR
,
* VARP
, and PERCENTILE
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorSpecifiedAggregation
*/
public TopicColumn withNotAllowedAggregations(AuthorSpecifiedAggregation... notAllowedAggregations) {
java.util.ArrayList notAllowedAggregationsCopy = new java.util.ArrayList(notAllowedAggregations.length);
for (AuthorSpecifiedAggregation value : notAllowedAggregations) {
notAllowedAggregationsCopy.add(value.toString());
}
if (getNotAllowedAggregations() == null) {
setNotAllowedAggregations(notAllowedAggregationsCopy);
} else {
getNotAllowedAggregations().addAll(notAllowedAggregationsCopy);
}
return this;
}
/**
*
* The default formatting used for values in the column.
*
*
* @param defaultFormatting
* The default formatting used for values in the column.
*/
public void setDefaultFormatting(DefaultFormatting defaultFormatting) {
this.defaultFormatting = defaultFormatting;
}
/**
*
* The default formatting used for values in the column.
*
*
* @return The default formatting used for values in the column.
*/
public DefaultFormatting getDefaultFormatting() {
return this.defaultFormatting;
}
/**
*
* The default formatting used for values in the column.
*
*
* @param defaultFormatting
* The default formatting used for values in the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withDefaultFormatting(DefaultFormatting defaultFormatting) {
setDefaultFormatting(defaultFormatting);
return this;
}
/**
*
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*
*
* @param neverAggregateInFilter
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*/
public void setNeverAggregateInFilter(Boolean neverAggregateInFilter) {
this.neverAggregateInFilter = neverAggregateInFilter;
}
/**
*
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*
*
* @return A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*/
public Boolean getNeverAggregateInFilter() {
return this.neverAggregateInFilter;
}
/**
*
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*
*
* @param neverAggregateInFilter
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withNeverAggregateInFilter(Boolean neverAggregateInFilter) {
setNeverAggregateInFilter(neverAggregateInFilter);
return this;
}
/**
*
* A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*
*
* @return A Boolean value that indicates whether to aggregate the column data when it's used in a filter context.
*/
public Boolean isNeverAggregateInFilter() {
return this.neverAggregateInFilter;
}
/**
*
* The other names or aliases for the column cell value.
*
*
* @return The other names or aliases for the column cell value.
*/
public java.util.List getCellValueSynonyms() {
return cellValueSynonyms;
}
/**
*
* The other names or aliases for the column cell value.
*
*
* @param cellValueSynonyms
* The other names or aliases for the column cell value.
*/
public void setCellValueSynonyms(java.util.Collection cellValueSynonyms) {
if (cellValueSynonyms == null) {
this.cellValueSynonyms = null;
return;
}
this.cellValueSynonyms = new java.util.ArrayList(cellValueSynonyms);
}
/**
*
* The other names or aliases for the column cell value.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCellValueSynonyms(java.util.Collection)} or {@link #withCellValueSynonyms(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param cellValueSynonyms
* The other names or aliases for the column cell value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withCellValueSynonyms(CellValueSynonym... cellValueSynonyms) {
if (this.cellValueSynonyms == null) {
setCellValueSynonyms(new java.util.ArrayList(cellValueSynonyms.length));
}
for (CellValueSynonym ele : cellValueSynonyms) {
this.cellValueSynonyms.add(ele);
}
return this;
}
/**
*
* The other names or aliases for the column cell value.
*
*
* @param cellValueSynonyms
* The other names or aliases for the column cell value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withCellValueSynonyms(java.util.Collection cellValueSynonyms) {
setCellValueSynonyms(cellValueSynonyms);
return this;
}
/**
*
* The non additive value for the column.
*
*
* @param nonAdditive
* The non additive value for the column.
*/
public void setNonAdditive(Boolean nonAdditive) {
this.nonAdditive = nonAdditive;
}
/**
*
* The non additive value for the column.
*
*
* @return The non additive value for the column.
*/
public Boolean getNonAdditive() {
return this.nonAdditive;
}
/**
*
* The non additive value for the column.
*
*
* @param nonAdditive
* The non additive value for the column.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicColumn withNonAdditive(Boolean nonAdditive) {
setNonAdditive(nonAdditive);
return this;
}
/**
*
* The non additive value for the column.
*
*
* @return The non additive value for the column.
*/
public Boolean isNonAdditive() {
return this.nonAdditive;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getColumnName() != null)
sb.append("ColumnName: ").append(getColumnName()).append(",");
if (getColumnFriendlyName() != null)
sb.append("ColumnFriendlyName: ").append(getColumnFriendlyName()).append(",");
if (getColumnDescription() != null)
sb.append("ColumnDescription: ").append(getColumnDescription()).append(",");
if (getColumnSynonyms() != null)
sb.append("ColumnSynonyms: ").append(getColumnSynonyms()).append(",");
if (getColumnDataRole() != null)
sb.append("ColumnDataRole: ").append(getColumnDataRole()).append(",");
if (getAggregation() != null)
sb.append("Aggregation: ").append(getAggregation()).append(",");
if (getIsIncludedInTopic() != null)
sb.append("IsIncludedInTopic: ").append(getIsIncludedInTopic()).append(",");
if (getDisableIndexing() != null)
sb.append("DisableIndexing: ").append(getDisableIndexing()).append(",");
if (getComparativeOrder() != null)
sb.append("ComparativeOrder: ").append(getComparativeOrder()).append(",");
if (getSemanticType() != null)
sb.append("SemanticType: ").append(getSemanticType()).append(",");
if (getTimeGranularity() != null)
sb.append("TimeGranularity: ").append(getTimeGranularity()).append(",");
if (getAllowedAggregations() != null)
sb.append("AllowedAggregations: ").append(getAllowedAggregations()).append(",");
if (getNotAllowedAggregations() != null)
sb.append("NotAllowedAggregations: ").append(getNotAllowedAggregations()).append(",");
if (getDefaultFormatting() != null)
sb.append("DefaultFormatting: ").append(getDefaultFormatting()).append(",");
if (getNeverAggregateInFilter() != null)
sb.append("NeverAggregateInFilter: ").append(getNeverAggregateInFilter()).append(",");
if (getCellValueSynonyms() != null)
sb.append("CellValueSynonyms: ").append(getCellValueSynonyms()).append(",");
if (getNonAdditive() != null)
sb.append("NonAdditive: ").append(getNonAdditive());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TopicColumn == false)
return false;
TopicColumn other = (TopicColumn) obj;
if (other.getColumnName() == null ^ this.getColumnName() == null)
return false;
if (other.getColumnName() != null && other.getColumnName().equals(this.getColumnName()) == false)
return false;
if (other.getColumnFriendlyName() == null ^ this.getColumnFriendlyName() == null)
return false;
if (other.getColumnFriendlyName() != null && other.getColumnFriendlyName().equals(this.getColumnFriendlyName()) == false)
return false;
if (other.getColumnDescription() == null ^ this.getColumnDescription() == null)
return false;
if (other.getColumnDescription() != null && other.getColumnDescription().equals(this.getColumnDescription()) == false)
return false;
if (other.getColumnSynonyms() == null ^ this.getColumnSynonyms() == null)
return false;
if (other.getColumnSynonyms() != null && other.getColumnSynonyms().equals(this.getColumnSynonyms()) == false)
return false;
if (other.getColumnDataRole() == null ^ this.getColumnDataRole() == null)
return false;
if (other.getColumnDataRole() != null && other.getColumnDataRole().equals(this.getColumnDataRole()) == false)
return false;
if (other.getAggregation() == null ^ this.getAggregation() == null)
return false;
if (other.getAggregation() != null && other.getAggregation().equals(this.getAggregation()) == false)
return false;
if (other.getIsIncludedInTopic() == null ^ this.getIsIncludedInTopic() == null)
return false;
if (other.getIsIncludedInTopic() != null && other.getIsIncludedInTopic().equals(this.getIsIncludedInTopic()) == false)
return false;
if (other.getDisableIndexing() == null ^ this.getDisableIndexing() == null)
return false;
if (other.getDisableIndexing() != null && other.getDisableIndexing().equals(this.getDisableIndexing()) == false)
return false;
if (other.getComparativeOrder() == null ^ this.getComparativeOrder() == null)
return false;
if (other.getComparativeOrder() != null && other.getComparativeOrder().equals(this.getComparativeOrder()) == false)
return false;
if (other.getSemanticType() == null ^ this.getSemanticType() == null)
return false;
if (other.getSemanticType() != null && other.getSemanticType().equals(this.getSemanticType()) == false)
return false;
if (other.getTimeGranularity() == null ^ this.getTimeGranularity() == null)
return false;
if (other.getTimeGranularity() != null && other.getTimeGranularity().equals(this.getTimeGranularity()) == false)
return false;
if (other.getAllowedAggregations() == null ^ this.getAllowedAggregations() == null)
return false;
if (other.getAllowedAggregations() != null && other.getAllowedAggregations().equals(this.getAllowedAggregations()) == false)
return false;
if (other.getNotAllowedAggregations() == null ^ this.getNotAllowedAggregations() == null)
return false;
if (other.getNotAllowedAggregations() != null && other.getNotAllowedAggregations().equals(this.getNotAllowedAggregations()) == false)
return false;
if (other.getDefaultFormatting() == null ^ this.getDefaultFormatting() == null)
return false;
if (other.getDefaultFormatting() != null && other.getDefaultFormatting().equals(this.getDefaultFormatting()) == false)
return false;
if (other.getNeverAggregateInFilter() == null ^ this.getNeverAggregateInFilter() == null)
return false;
if (other.getNeverAggregateInFilter() != null && other.getNeverAggregateInFilter().equals(this.getNeverAggregateInFilter()) == false)
return false;
if (other.getCellValueSynonyms() == null ^ this.getCellValueSynonyms() == null)
return false;
if (other.getCellValueSynonyms() != null && other.getCellValueSynonyms().equals(this.getCellValueSynonyms()) == false)
return false;
if (other.getNonAdditive() == null ^ this.getNonAdditive() == null)
return false;
if (other.getNonAdditive() != null && other.getNonAdditive().equals(this.getNonAdditive()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getColumnName() == null) ? 0 : getColumnName().hashCode());
hashCode = prime * hashCode + ((getColumnFriendlyName() == null) ? 0 : getColumnFriendlyName().hashCode());
hashCode = prime * hashCode + ((getColumnDescription() == null) ? 0 : getColumnDescription().hashCode());
hashCode = prime * hashCode + ((getColumnSynonyms() == null) ? 0 : getColumnSynonyms().hashCode());
hashCode = prime * hashCode + ((getColumnDataRole() == null) ? 0 : getColumnDataRole().hashCode());
hashCode = prime * hashCode + ((getAggregation() == null) ? 0 : getAggregation().hashCode());
hashCode = prime * hashCode + ((getIsIncludedInTopic() == null) ? 0 : getIsIncludedInTopic().hashCode());
hashCode = prime * hashCode + ((getDisableIndexing() == null) ? 0 : getDisableIndexing().hashCode());
hashCode = prime * hashCode + ((getComparativeOrder() == null) ? 0 : getComparativeOrder().hashCode());
hashCode = prime * hashCode + ((getSemanticType() == null) ? 0 : getSemanticType().hashCode());
hashCode = prime * hashCode + ((getTimeGranularity() == null) ? 0 : getTimeGranularity().hashCode());
hashCode = prime * hashCode + ((getAllowedAggregations() == null) ? 0 : getAllowedAggregations().hashCode());
hashCode = prime * hashCode + ((getNotAllowedAggregations() == null) ? 0 : getNotAllowedAggregations().hashCode());
hashCode = prime * hashCode + ((getDefaultFormatting() == null) ? 0 : getDefaultFormatting().hashCode());
hashCode = prime * hashCode + ((getNeverAggregateInFilter() == null) ? 0 : getNeverAggregateInFilter().hashCode());
hashCode = prime * hashCode + ((getCellValueSynonyms() == null) ? 0 : getCellValueSynonyms().hashCode());
hashCode = prime * hashCode + ((getNonAdditive() == null) ? 0 : getNonAdditive().hashCode());
return hashCode;
}
@Override
public TopicColumn clone() {
try {
return (TopicColumn) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.TopicColumnMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}