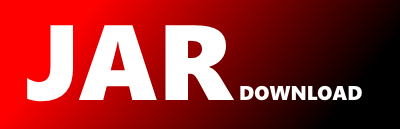
com.amazonaws.services.quicksight.model.TopicReviewedAnswer Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The deinition for a TopicReviewedAnswer
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TopicReviewedAnswer implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the reviewed answer.
*
*/
private String arn;
/**
*
* The answer ID of the reviewed answer.
*
*/
private String answerId;
/**
*
* The Dataset ARN for the TopicReviewedAnswer
.
*
*/
private String datasetArn;
/**
*
* The question for the TopicReviewedAnswer
.
*
*/
private String question;
/**
*
* The mir for the TopicReviewedAnswer
.
*
*/
private TopicIR mir;
/**
*
* The primary visual for the TopicReviewedAnswer
.
*
*/
private TopicVisual primaryVisual;
/**
*
* The template for the TopicReviewedAnswer
.
*
*/
private TopicTemplate template;
/**
*
* The Amazon Resource Name (ARN) of the reviewed answer.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the reviewed answer.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the reviewed answer.
*
*
* @return The Amazon Resource Name (ARN) of the reviewed answer.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the reviewed answer.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the reviewed answer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The answer ID of the reviewed answer.
*
*
* @param answerId
* The answer ID of the reviewed answer.
*/
public void setAnswerId(String answerId) {
this.answerId = answerId;
}
/**
*
* The answer ID of the reviewed answer.
*
*
* @return The answer ID of the reviewed answer.
*/
public String getAnswerId() {
return this.answerId;
}
/**
*
* The answer ID of the reviewed answer.
*
*
* @param answerId
* The answer ID of the reviewed answer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withAnswerId(String answerId) {
setAnswerId(answerId);
return this;
}
/**
*
* The Dataset ARN for the TopicReviewedAnswer
.
*
*
* @param datasetArn
* The Dataset ARN for the TopicReviewedAnswer
.
*/
public void setDatasetArn(String datasetArn) {
this.datasetArn = datasetArn;
}
/**
*
* The Dataset ARN for the TopicReviewedAnswer
.
*
*
* @return The Dataset ARN for the TopicReviewedAnswer
.
*/
public String getDatasetArn() {
return this.datasetArn;
}
/**
*
* The Dataset ARN for the TopicReviewedAnswer
.
*
*
* @param datasetArn
* The Dataset ARN for the TopicReviewedAnswer
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withDatasetArn(String datasetArn) {
setDatasetArn(datasetArn);
return this;
}
/**
*
* The question for the TopicReviewedAnswer
.
*
*
* @param question
* The question for the TopicReviewedAnswer
.
*/
public void setQuestion(String question) {
this.question = question;
}
/**
*
* The question for the TopicReviewedAnswer
.
*
*
* @return The question for the TopicReviewedAnswer
.
*/
public String getQuestion() {
return this.question;
}
/**
*
* The question for the TopicReviewedAnswer
.
*
*
* @param question
* The question for the TopicReviewedAnswer
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withQuestion(String question) {
setQuestion(question);
return this;
}
/**
*
* The mir for the TopicReviewedAnswer
.
*
*
* @param mir
* The mir for the TopicReviewedAnswer
.
*/
public void setMir(TopicIR mir) {
this.mir = mir;
}
/**
*
* The mir for the TopicReviewedAnswer
.
*
*
* @return The mir for the TopicReviewedAnswer
.
*/
public TopicIR getMir() {
return this.mir;
}
/**
*
* The mir for the TopicReviewedAnswer
.
*
*
* @param mir
* The mir for the TopicReviewedAnswer
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withMir(TopicIR mir) {
setMir(mir);
return this;
}
/**
*
* The primary visual for the TopicReviewedAnswer
.
*
*
* @param primaryVisual
* The primary visual for the TopicReviewedAnswer
.
*/
public void setPrimaryVisual(TopicVisual primaryVisual) {
this.primaryVisual = primaryVisual;
}
/**
*
* The primary visual for the TopicReviewedAnswer
.
*
*
* @return The primary visual for the TopicReviewedAnswer
.
*/
public TopicVisual getPrimaryVisual() {
return this.primaryVisual;
}
/**
*
* The primary visual for the TopicReviewedAnswer
.
*
*
* @param primaryVisual
* The primary visual for the TopicReviewedAnswer
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withPrimaryVisual(TopicVisual primaryVisual) {
setPrimaryVisual(primaryVisual);
return this;
}
/**
*
* The template for the TopicReviewedAnswer
.
*
*
* @param template
* The template for the TopicReviewedAnswer
.
*/
public void setTemplate(TopicTemplate template) {
this.template = template;
}
/**
*
* The template for the TopicReviewedAnswer
.
*
*
* @return The template for the TopicReviewedAnswer
.
*/
public TopicTemplate getTemplate() {
return this.template;
}
/**
*
* The template for the TopicReviewedAnswer
.
*
*
* @param template
* The template for the TopicReviewedAnswer
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TopicReviewedAnswer withTemplate(TopicTemplate template) {
setTemplate(template);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getAnswerId() != null)
sb.append("AnswerId: ").append(getAnswerId()).append(",");
if (getDatasetArn() != null)
sb.append("DatasetArn: ").append(getDatasetArn()).append(",");
if (getQuestion() != null)
sb.append("Question: ").append(getQuestion()).append(",");
if (getMir() != null)
sb.append("Mir: ").append(getMir()).append(",");
if (getPrimaryVisual() != null)
sb.append("PrimaryVisual: ").append(getPrimaryVisual()).append(",");
if (getTemplate() != null)
sb.append("Template: ").append(getTemplate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TopicReviewedAnswer == false)
return false;
TopicReviewedAnswer other = (TopicReviewedAnswer) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getAnswerId() == null ^ this.getAnswerId() == null)
return false;
if (other.getAnswerId() != null && other.getAnswerId().equals(this.getAnswerId()) == false)
return false;
if (other.getDatasetArn() == null ^ this.getDatasetArn() == null)
return false;
if (other.getDatasetArn() != null && other.getDatasetArn().equals(this.getDatasetArn()) == false)
return false;
if (other.getQuestion() == null ^ this.getQuestion() == null)
return false;
if (other.getQuestion() != null && other.getQuestion().equals(this.getQuestion()) == false)
return false;
if (other.getMir() == null ^ this.getMir() == null)
return false;
if (other.getMir() != null && other.getMir().equals(this.getMir()) == false)
return false;
if (other.getPrimaryVisual() == null ^ this.getPrimaryVisual() == null)
return false;
if (other.getPrimaryVisual() != null && other.getPrimaryVisual().equals(this.getPrimaryVisual()) == false)
return false;
if (other.getTemplate() == null ^ this.getTemplate() == null)
return false;
if (other.getTemplate() != null && other.getTemplate().equals(this.getTemplate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getAnswerId() == null) ? 0 : getAnswerId().hashCode());
hashCode = prime * hashCode + ((getDatasetArn() == null) ? 0 : getDatasetArn().hashCode());
hashCode = prime * hashCode + ((getQuestion() == null) ? 0 : getQuestion().hashCode());
hashCode = prime * hashCode + ((getMir() == null) ? 0 : getMir().hashCode());
hashCode = prime * hashCode + ((getPrimaryVisual() == null) ? 0 : getPrimaryVisual().hashCode());
hashCode = prime * hashCode + ((getTemplate() == null) ? 0 : getTemplate().hashCode());
return hashCode;
}
@Override
public TopicReviewedAnswer clone() {
try {
return (TopicReviewedAnswer) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.TopicReviewedAnswerMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}