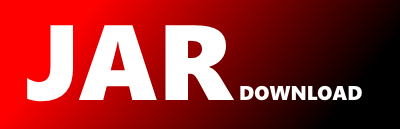
com.amazonaws.services.quicksight.model.UpdateDashboardRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateDashboardRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*
*/
private String awsAccountId;
/**
*
* The ID for the dashboard.
*
*/
private String dashboardId;
/**
*
* The display name of the dashboard.
*
*/
private String name;
/**
*
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you use a
* SourceTemplate
entity. If you need to update a dashboard from an analysis, first convert the
* analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source template.
* The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement datasets
* for the placeholders listed in the original. The schema in each dataset must match its placeholder.
*
*/
private DashboardSourceEntity sourceEntity;
/**
*
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard. A
* dashboard can have any type of parameters, and some parameters might accept multiple values.
*
*/
private Parameters parameters;
/**
*
* A description for the first version of the dashboard being created.
*
*/
private String versionDescription;
/**
*
* Options for publishing the dashboard when you create it:
*
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon QuickSight
* disables the left filter pane on the published dashboard, which can be used for ad hoc (one-time) filtering. This
* option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't enabled when
* this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*
*/
private DashboardPublishOptions dashboardPublishOptions;
/**
*
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for this
* field, it overrides the value that was originally associated with the entity. The theme ARN must exist in the
* same Amazon Web Services account where you create the dashboard.
*
*/
private String themeArn;
/**
*
* The definition of a dashboard.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*/
private DashboardVersionDefinition definition;
/**
*
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*
*/
private ValidationStrategy validationStrategy;
/**
*
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*
*
* @return The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account that contains the dashboard that you're updating.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The ID for the dashboard.
*
*
* @param dashboardId
* The ID for the dashboard.
*/
public void setDashboardId(String dashboardId) {
this.dashboardId = dashboardId;
}
/**
*
* The ID for the dashboard.
*
*
* @return The ID for the dashboard.
*/
public String getDashboardId() {
return this.dashboardId;
}
/**
*
* The ID for the dashboard.
*
*
* @param dashboardId
* The ID for the dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withDashboardId(String dashboardId) {
setDashboardId(dashboardId);
return this;
}
/**
*
* The display name of the dashboard.
*
*
* @param name
* The display name of the dashboard.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The display name of the dashboard.
*
*
* @return The display name of the dashboard.
*/
public String getName() {
return this.name;
}
/**
*
* The display name of the dashboard.
*
*
* @param name
* The display name of the dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you use a
* SourceTemplate
entity. If you need to update a dashboard from an analysis, first convert the
* analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source template.
* The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement datasets
* for the placeholders listed in the original. The schema in each dataset must match its placeholder.
*
*
* @param sourceEntity
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you
* use a SourceTemplate
entity. If you need to update a dashboard from an analysis, first
* convert the analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source
* template. The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement
* datasets for the placeholders listed in the original. The schema in each dataset must match its
* placeholder.
*/
public void setSourceEntity(DashboardSourceEntity sourceEntity) {
this.sourceEntity = sourceEntity;
}
/**
*
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you use a
* SourceTemplate
entity. If you need to update a dashboard from an analysis, first convert the
* analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source template.
* The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement datasets
* for the placeholders listed in the original. The schema in each dataset must match its placeholder.
*
*
* @return The entity that you are using as a source when you update the dashboard. In SourceEntity
,
* you specify the type of object you're using as source. You can only update a dashboard from a template,
* so you use a SourceTemplate
entity. If you need to update a dashboard from an analysis,
* first convert the analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source
* template. The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement
* datasets for the placeholders listed in the original. The schema in each dataset must match its
* placeholder.
*/
public DashboardSourceEntity getSourceEntity() {
return this.sourceEntity;
}
/**
*
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you use a
* SourceTemplate
entity. If you need to update a dashboard from an analysis, first convert the
* analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source template.
* The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement datasets
* for the placeholders listed in the original. The schema in each dataset must match its placeholder.
*
*
* @param sourceEntity
* The entity that you are using as a source when you update the dashboard. In SourceEntity
, you
* specify the type of object you're using as source. You can only update a dashboard from a template, so you
* use a SourceTemplate
entity. If you need to update a dashboard from an analysis, first
* convert the analysis to a template by using the
* CreateTemplate
* API operation. For SourceTemplate
, specify the Amazon Resource Name (ARN) of the source
* template. The SourceTemplate
ARN can contain any Amazon Web Services account and any Amazon
* QuickSight-supported Amazon Web Services Region.
*
* Use the DataSetReferences
entity within SourceTemplate
to list the replacement
* datasets for the placeholders listed in the original. The schema in each dataset must match its
* placeholder.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withSourceEntity(DashboardSourceEntity sourceEntity) {
setSourceEntity(sourceEntity);
return this;
}
/**
*
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard. A
* dashboard can have any type of parameters, and some parameters might accept multiple values.
*
*
* @param parameters
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard.
* A dashboard can have any type of parameters, and some parameters might accept multiple values.
*/
public void setParameters(Parameters parameters) {
this.parameters = parameters;
}
/**
*
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard. A
* dashboard can have any type of parameters, and some parameters might accept multiple values.
*
*
* @return A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard.
* A dashboard can have any type of parameters, and some parameters might accept multiple values.
*/
public Parameters getParameters() {
return this.parameters;
}
/**
*
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard. A
* dashboard can have any type of parameters, and some parameters might accept multiple values.
*
*
* @param parameters
* A structure that contains the parameters of the dashboard. These are parameter overrides for a dashboard.
* A dashboard can have any type of parameters, and some parameters might accept multiple values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withParameters(Parameters parameters) {
setParameters(parameters);
return this;
}
/**
*
* A description for the first version of the dashboard being created.
*
*
* @param versionDescription
* A description for the first version of the dashboard being created.
*/
public void setVersionDescription(String versionDescription) {
this.versionDescription = versionDescription;
}
/**
*
* A description for the first version of the dashboard being created.
*
*
* @return A description for the first version of the dashboard being created.
*/
public String getVersionDescription() {
return this.versionDescription;
}
/**
*
* A description for the first version of the dashboard being created.
*
*
* @param versionDescription
* A description for the first version of the dashboard being created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withVersionDescription(String versionDescription) {
setVersionDescription(versionDescription);
return this;
}
/**
*
* Options for publishing the dashboard when you create it:
*
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon QuickSight
* disables the left filter pane on the published dashboard, which can be used for ad hoc (one-time) filtering. This
* option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't enabled when
* this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*
*
* @param dashboardPublishOptions
* Options for publishing the dashboard when you create it:
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon
* QuickSight disables the left filter pane on the published dashboard, which can be used for ad hoc
* (one-time) filtering. This option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't
* enabled when this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*/
public void setDashboardPublishOptions(DashboardPublishOptions dashboardPublishOptions) {
this.dashboardPublishOptions = dashboardPublishOptions;
}
/**
*
* Options for publishing the dashboard when you create it:
*
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon QuickSight
* disables the left filter pane on the published dashboard, which can be used for ad hoc (one-time) filtering. This
* option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't enabled when
* this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*
*
* @return Options for publishing the dashboard when you create it:
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon
* QuickSight disables the left filter pane on the published dashboard, which can be used for ad hoc
* (one-time) filtering. This option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't
* enabled when this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*/
public DashboardPublishOptions getDashboardPublishOptions() {
return this.dashboardPublishOptions;
}
/**
*
* Options for publishing the dashboard when you create it:
*
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon QuickSight
* disables the left filter pane on the published dashboard, which can be used for ad hoc (one-time) filtering. This
* option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't enabled when
* this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
*
*
* @param dashboardPublishOptions
* Options for publishing the dashboard when you create it:
*
* -
*
* AvailabilityStatus
for AdHocFilteringOption
- This status can be either
* ENABLED
or DISABLED
. When this is set to DISABLED
, Amazon
* QuickSight disables the left filter pane on the published dashboard, which can be used for ad hoc
* (one-time) filtering. This option is ENABLED
by default.
*
*
* -
*
* AvailabilityStatus
for ExportToCSVOption
- This status can be either
* ENABLED
or DISABLED
. The visual option to export data to .CSV format isn't
* enabled when this is set to DISABLED
. This option is ENABLED
by default.
*
*
* -
*
* VisibilityState
for SheetControlsOption
- This visibility state can be either
* COLLAPSED
or EXPANDED
. This option is COLLAPSED
by default.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withDashboardPublishOptions(DashboardPublishOptions dashboardPublishOptions) {
setDashboardPublishOptions(dashboardPublishOptions);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for this
* field, it overrides the value that was originally associated with the entity. The theme ARN must exist in the
* same Amazon Web Services account where you create the dashboard.
*
*
* @param themeArn
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for
* this field, it overrides the value that was originally associated with the entity. The theme ARN must
* exist in the same Amazon Web Services account where you create the dashboard.
*/
public void setThemeArn(String themeArn) {
this.themeArn = themeArn;
}
/**
*
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for this
* field, it overrides the value that was originally associated with the entity. The theme ARN must exist in the
* same Amazon Web Services account where you create the dashboard.
*
*
* @return The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for
* this field, it overrides the value that was originally associated with the entity. The theme ARN must
* exist in the same Amazon Web Services account where you create the dashboard.
*/
public String getThemeArn() {
return this.themeArn;
}
/**
*
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for this
* field, it overrides the value that was originally associated with the entity. The theme ARN must exist in the
* same Amazon Web Services account where you create the dashboard.
*
*
* @param themeArn
* The Amazon Resource Name (ARN) of the theme that is being used for this dashboard. If you add a value for
* this field, it overrides the value that was originally associated with the entity. The theme ARN must
* exist in the same Amazon Web Services account where you create the dashboard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withThemeArn(String themeArn) {
setThemeArn(themeArn);
return this;
}
/**
*
* The definition of a dashboard.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* @param definition
* The definition of a dashboard.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*/
public void setDefinition(DashboardVersionDefinition definition) {
this.definition = definition;
}
/**
*
* The definition of a dashboard.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* @return The definition of a dashboard.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*/
public DashboardVersionDefinition getDefinition() {
return this.definition;
}
/**
*
* The definition of a dashboard.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* @param definition
* The definition of a dashboard.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withDefinition(DashboardVersionDefinition definition) {
setDefinition(definition);
return this;
}
/**
*
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*
*
* @param validationStrategy
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*/
public void setValidationStrategy(ValidationStrategy validationStrategy) {
this.validationStrategy = validationStrategy;
}
/**
*
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*
*
* @return The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*/
public ValidationStrategy getValidationStrategy() {
return this.validationStrategy;
}
/**
*
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
*
*
* @param validationStrategy
* The option to relax the validation needed to update a dashboard with definition objects. This skips the
* validation step for specific errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDashboardRequest withValidationStrategy(ValidationStrategy validationStrategy) {
setValidationStrategy(validationStrategy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getDashboardId() != null)
sb.append("DashboardId: ").append(getDashboardId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getSourceEntity() != null)
sb.append("SourceEntity: ").append(getSourceEntity()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getVersionDescription() != null)
sb.append("VersionDescription: ").append(getVersionDescription()).append(",");
if (getDashboardPublishOptions() != null)
sb.append("DashboardPublishOptions: ").append(getDashboardPublishOptions()).append(",");
if (getThemeArn() != null)
sb.append("ThemeArn: ").append(getThemeArn()).append(",");
if (getDefinition() != null)
sb.append("Definition: ").append(getDefinition()).append(",");
if (getValidationStrategy() != null)
sb.append("ValidationStrategy: ").append(getValidationStrategy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateDashboardRequest == false)
return false;
UpdateDashboardRequest other = (UpdateDashboardRequest) obj;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getDashboardId() == null ^ this.getDashboardId() == null)
return false;
if (other.getDashboardId() != null && other.getDashboardId().equals(this.getDashboardId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getSourceEntity() == null ^ this.getSourceEntity() == null)
return false;
if (other.getSourceEntity() != null && other.getSourceEntity().equals(this.getSourceEntity()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getVersionDescription() == null ^ this.getVersionDescription() == null)
return false;
if (other.getVersionDescription() != null && other.getVersionDescription().equals(this.getVersionDescription()) == false)
return false;
if (other.getDashboardPublishOptions() == null ^ this.getDashboardPublishOptions() == null)
return false;
if (other.getDashboardPublishOptions() != null && other.getDashboardPublishOptions().equals(this.getDashboardPublishOptions()) == false)
return false;
if (other.getThemeArn() == null ^ this.getThemeArn() == null)
return false;
if (other.getThemeArn() != null && other.getThemeArn().equals(this.getThemeArn()) == false)
return false;
if (other.getDefinition() == null ^ this.getDefinition() == null)
return false;
if (other.getDefinition() != null && other.getDefinition().equals(this.getDefinition()) == false)
return false;
if (other.getValidationStrategy() == null ^ this.getValidationStrategy() == null)
return false;
if (other.getValidationStrategy() != null && other.getValidationStrategy().equals(this.getValidationStrategy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getDashboardId() == null) ? 0 : getDashboardId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getSourceEntity() == null) ? 0 : getSourceEntity().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getVersionDescription() == null) ? 0 : getVersionDescription().hashCode());
hashCode = prime * hashCode + ((getDashboardPublishOptions() == null) ? 0 : getDashboardPublishOptions().hashCode());
hashCode = prime * hashCode + ((getThemeArn() == null) ? 0 : getThemeArn().hashCode());
hashCode = prime * hashCode + ((getDefinition() == null) ? 0 : getDefinition().hashCode());
hashCode = prime * hashCode + ((getValidationStrategy() == null) ? 0 : getValidationStrategy().hashCode());
return hashCode;
}
@Override
public UpdateDashboardRequest clone() {
return (UpdateDashboardRequest) super.clone();
}
}