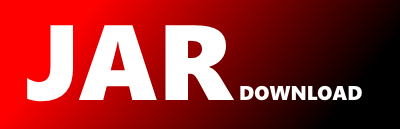
com.amazonaws.services.quicksight.model.Visual Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A visual displayed on a sheet in an analysis, dashboard, or template.
*
*
* This is a union type structure. For this structure to be valid, only one of the attributes can be defined.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Visual implements Serializable, Cloneable, StructuredPojo {
/**
*
* A table visual.
*
*
* For more information, see Using tables
* as visuals in the Amazon QuickSight User Guide.
*
*/
private TableVisual tableVisual;
/**
*
* A pivot table.
*
*
* For more information, see Using
* pivot tables in the Amazon QuickSight User Guide.
*
*/
private PivotTableVisual pivotTableVisual;
/**
*
* A bar chart.
*
*
* For more information, see Using bar
* charts in the Amazon QuickSight User Guide.
*
*/
private BarChartVisual barChartVisual;
/**
*
* A key performance indicator (KPI).
*
*
* For more information, see Using KPIs in
* the Amazon QuickSight User Guide.
*
*/
private KPIVisual kPIVisual;
/**
*
* A pie or donut chart.
*
*
* For more information, see Using pie
* charts in the Amazon QuickSight User Guide.
*
*/
private PieChartVisual pieChartVisual;
/**
*
* A gauge chart.
*
*
* For more information, see Using
* gauge charts in the Amazon QuickSight User Guide.
*
*/
private GaugeChartVisual gaugeChartVisual;
/**
*
* A line chart.
*
*
* For more information, see Using
* line charts in the Amazon QuickSight User Guide.
*
*/
private LineChartVisual lineChartVisual;
/**
*
* A heat map.
*
*
* For more information, see Using heat
* maps in the Amazon QuickSight User Guide.
*
*/
private HeatMapVisual heatMapVisual;
/**
*
* A tree map.
*
*
* For more information, see Using tree
* maps in the Amazon QuickSight User Guide.
*
*/
private TreeMapVisual treeMapVisual;
/**
*
* A geospatial map or a points on map visual.
*
*
* For more information, see Creating
* point maps in the Amazon QuickSight User Guide.
*
*/
private GeospatialMapVisual geospatialMapVisual;
/**
*
* A filled map.
*
*
* For more information, see Creating
* filled maps in the Amazon QuickSight User Guide.
*
*/
private FilledMapVisual filledMapVisual;
/**
*
* A funnel chart.
*
*
* For more information, see Using funnel charts in
* the Amazon QuickSight User Guide.
*
*/
private FunnelChartVisual funnelChartVisual;
/**
*
* A scatter plot.
*
*
* For more information, see Using
* scatter plots in the Amazon QuickSight User Guide.
*
*/
private ScatterPlotVisual scatterPlotVisual;
/**
*
* A combo chart.
*
*
* For more information, see Using
* combo charts in the Amazon QuickSight User Guide.
*
*/
private ComboChartVisual comboChartVisual;
/**
*
* A box plot.
*
*
* For more information, see Using box
* plots in the Amazon QuickSight User Guide.
*
*/
private BoxPlotVisual boxPlotVisual;
/**
*
* A waterfall chart.
*
*
* For more information, see Using
* waterfall charts in the Amazon QuickSight User Guide.
*
*/
private WaterfallVisual waterfallVisual;
/**
*
* A histogram.
*
*
* For more information, see Using histograms in the
* Amazon QuickSight User Guide.
*
*/
private HistogramVisual histogramVisual;
/**
*
* A word cloud.
*
*
* For more information, see Using word
* clouds in the Amazon QuickSight User Guide.
*
*/
private WordCloudVisual wordCloudVisual;
/**
*
* An insight visual.
*
*
* For more information, see Working with insights
* in the Amazon QuickSight User Guide.
*
*/
private InsightVisual insightVisual;
/**
*
* A sankey diagram.
*
*
* For more information, see Using
* Sankey diagrams in the Amazon QuickSight User Guide.
*
*/
private SankeyDiagramVisual sankeyDiagramVisual;
/**
*
* A visual that contains custom content.
*
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*
*/
private CustomContentVisual customContentVisual;
/**
*
* An empty visual.
*
*/
private EmptyVisual emptyVisual;
/**
*
* A radar chart visual.
*
*
* For more information, see Using
* radar charts in the Amazon QuickSight User Guide.
*
*/
private RadarChartVisual radarChartVisual;
/**
*
* A table visual.
*
*
* For more information, see Using tables
* as visuals in the Amazon QuickSight User Guide.
*
*
* @param tableVisual
* A table visual.
*
* For more information, see Using
* tables as visuals in the Amazon QuickSight User Guide.
*/
public void setTableVisual(TableVisual tableVisual) {
this.tableVisual = tableVisual;
}
/**
*
* A table visual.
*
*
* For more information, see Using tables
* as visuals in the Amazon QuickSight User Guide.
*
*
* @return A table visual.
*
* For more information, see Using
* tables as visuals in the Amazon QuickSight User Guide.
*/
public TableVisual getTableVisual() {
return this.tableVisual;
}
/**
*
* A table visual.
*
*
* For more information, see Using tables
* as visuals in the Amazon QuickSight User Guide.
*
*
* @param tableVisual
* A table visual.
*
* For more information, see Using
* tables as visuals in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withTableVisual(TableVisual tableVisual) {
setTableVisual(tableVisual);
return this;
}
/**
*
* A pivot table.
*
*
* For more information, see Using
* pivot tables in the Amazon QuickSight User Guide.
*
*
* @param pivotTableVisual
* A pivot table.
*
* For more information, see Using pivot tables in the
* Amazon QuickSight User Guide.
*/
public void setPivotTableVisual(PivotTableVisual pivotTableVisual) {
this.pivotTableVisual = pivotTableVisual;
}
/**
*
* A pivot table.
*
*
* For more information, see Using
* pivot tables in the Amazon QuickSight User Guide.
*
*
* @return A pivot table.
*
* For more information, see Using pivot tables in the
* Amazon QuickSight User Guide.
*/
public PivotTableVisual getPivotTableVisual() {
return this.pivotTableVisual;
}
/**
*
* A pivot table.
*
*
* For more information, see Using
* pivot tables in the Amazon QuickSight User Guide.
*
*
* @param pivotTableVisual
* A pivot table.
*
* For more information, see Using pivot tables in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withPivotTableVisual(PivotTableVisual pivotTableVisual) {
setPivotTableVisual(pivotTableVisual);
return this;
}
/**
*
* A bar chart.
*
*
* For more information, see Using bar
* charts in the Amazon QuickSight User Guide.
*
*
* @param barChartVisual
* A bar chart.
*
* For more information, see Using bar charts in the
* Amazon QuickSight User Guide.
*/
public void setBarChartVisual(BarChartVisual barChartVisual) {
this.barChartVisual = barChartVisual;
}
/**
*
* A bar chart.
*
*
* For more information, see Using bar
* charts in the Amazon QuickSight User Guide.
*
*
* @return A bar chart.
*
* For more information, see Using bar charts in the
* Amazon QuickSight User Guide.
*/
public BarChartVisual getBarChartVisual() {
return this.barChartVisual;
}
/**
*
* A bar chart.
*
*
* For more information, see Using bar
* charts in the Amazon QuickSight User Guide.
*
*
* @param barChartVisual
* A bar chart.
*
* For more information, see Using bar charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withBarChartVisual(BarChartVisual barChartVisual) {
setBarChartVisual(barChartVisual);
return this;
}
/**
*
* A key performance indicator (KPI).
*
*
* For more information, see Using KPIs in
* the Amazon QuickSight User Guide.
*
*
* @param kPIVisual
* A key performance indicator (KPI).
*
* For more information, see Using
* KPIs in the Amazon QuickSight User Guide.
*/
public void setKPIVisual(KPIVisual kPIVisual) {
this.kPIVisual = kPIVisual;
}
/**
*
* A key performance indicator (KPI).
*
*
* For more information, see Using KPIs in
* the Amazon QuickSight User Guide.
*
*
* @return A key performance indicator (KPI).
*
* For more information, see Using
* KPIs in the Amazon QuickSight User Guide.
*/
public KPIVisual getKPIVisual() {
return this.kPIVisual;
}
/**
*
* A key performance indicator (KPI).
*
*
* For more information, see Using KPIs in
* the Amazon QuickSight User Guide.
*
*
* @param kPIVisual
* A key performance indicator (KPI).
*
* For more information, see Using
* KPIs in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withKPIVisual(KPIVisual kPIVisual) {
setKPIVisual(kPIVisual);
return this;
}
/**
*
* A pie or donut chart.
*
*
* For more information, see Using pie
* charts in the Amazon QuickSight User Guide.
*
*
* @param pieChartVisual
* A pie or donut chart.
*
* For more information, see Using pie charts in the
* Amazon QuickSight User Guide.
*/
public void setPieChartVisual(PieChartVisual pieChartVisual) {
this.pieChartVisual = pieChartVisual;
}
/**
*
* A pie or donut chart.
*
*
* For more information, see Using pie
* charts in the Amazon QuickSight User Guide.
*
*
* @return A pie or donut chart.
*
* For more information, see Using pie charts in the
* Amazon QuickSight User Guide.
*/
public PieChartVisual getPieChartVisual() {
return this.pieChartVisual;
}
/**
*
* A pie or donut chart.
*
*
* For more information, see Using pie
* charts in the Amazon QuickSight User Guide.
*
*
* @param pieChartVisual
* A pie or donut chart.
*
* For more information, see Using pie charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withPieChartVisual(PieChartVisual pieChartVisual) {
setPieChartVisual(pieChartVisual);
return this;
}
/**
*
* A gauge chart.
*
*
* For more information, see Using
* gauge charts in the Amazon QuickSight User Guide.
*
*
* @param gaugeChartVisual
* A gauge chart.
*
* For more information, see Using gauge charts in the
* Amazon QuickSight User Guide.
*/
public void setGaugeChartVisual(GaugeChartVisual gaugeChartVisual) {
this.gaugeChartVisual = gaugeChartVisual;
}
/**
*
* A gauge chart.
*
*
* For more information, see Using
* gauge charts in the Amazon QuickSight User Guide.
*
*
* @return A gauge chart.
*
* For more information, see Using gauge charts in the
* Amazon QuickSight User Guide.
*/
public GaugeChartVisual getGaugeChartVisual() {
return this.gaugeChartVisual;
}
/**
*
* A gauge chart.
*
*
* For more information, see Using
* gauge charts in the Amazon QuickSight User Guide.
*
*
* @param gaugeChartVisual
* A gauge chart.
*
* For more information, see Using gauge charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withGaugeChartVisual(GaugeChartVisual gaugeChartVisual) {
setGaugeChartVisual(gaugeChartVisual);
return this;
}
/**
*
* A line chart.
*
*
* For more information, see Using
* line charts in the Amazon QuickSight User Guide.
*
*
* @param lineChartVisual
* A line chart.
*
* For more information, see Using line charts in the
* Amazon QuickSight User Guide.
*/
public void setLineChartVisual(LineChartVisual lineChartVisual) {
this.lineChartVisual = lineChartVisual;
}
/**
*
* A line chart.
*
*
* For more information, see Using
* line charts in the Amazon QuickSight User Guide.
*
*
* @return A line chart.
*
* For more information, see Using line charts in the
* Amazon QuickSight User Guide.
*/
public LineChartVisual getLineChartVisual() {
return this.lineChartVisual;
}
/**
*
* A line chart.
*
*
* For more information, see Using
* line charts in the Amazon QuickSight User Guide.
*
*
* @param lineChartVisual
* A line chart.
*
* For more information, see Using line charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withLineChartVisual(LineChartVisual lineChartVisual) {
setLineChartVisual(lineChartVisual);
return this;
}
/**
*
* A heat map.
*
*
* For more information, see Using heat
* maps in the Amazon QuickSight User Guide.
*
*
* @param heatMapVisual
* A heat map.
*
* For more information, see Using
* heat maps in the Amazon QuickSight User Guide.
*/
public void setHeatMapVisual(HeatMapVisual heatMapVisual) {
this.heatMapVisual = heatMapVisual;
}
/**
*
* A heat map.
*
*
* For more information, see Using heat
* maps in the Amazon QuickSight User Guide.
*
*
* @return A heat map.
*
* For more information, see Using heat maps in the
* Amazon QuickSight User Guide.
*/
public HeatMapVisual getHeatMapVisual() {
return this.heatMapVisual;
}
/**
*
* A heat map.
*
*
* For more information, see Using heat
* maps in the Amazon QuickSight User Guide.
*
*
* @param heatMapVisual
* A heat map.
*
* For more information, see Using
* heat maps in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withHeatMapVisual(HeatMapVisual heatMapVisual) {
setHeatMapVisual(heatMapVisual);
return this;
}
/**
*
* A tree map.
*
*
* For more information, see Using tree
* maps in the Amazon QuickSight User Guide.
*
*
* @param treeMapVisual
* A tree map.
*
* For more information, see Using
* tree maps in the Amazon QuickSight User Guide.
*/
public void setTreeMapVisual(TreeMapVisual treeMapVisual) {
this.treeMapVisual = treeMapVisual;
}
/**
*
* A tree map.
*
*
* For more information, see Using tree
* maps in the Amazon QuickSight User Guide.
*
*
* @return A tree map.
*
* For more information, see Using tree maps in the
* Amazon QuickSight User Guide.
*/
public TreeMapVisual getTreeMapVisual() {
return this.treeMapVisual;
}
/**
*
* A tree map.
*
*
* For more information, see Using tree
* maps in the Amazon QuickSight User Guide.
*
*
* @param treeMapVisual
* A tree map.
*
* For more information, see Using
* tree maps in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withTreeMapVisual(TreeMapVisual treeMapVisual) {
setTreeMapVisual(treeMapVisual);
return this;
}
/**
*
* A geospatial map or a points on map visual.
*
*
* For more information, see Creating
* point maps in the Amazon QuickSight User Guide.
*
*
* @param geospatialMapVisual
* A geospatial map or a points on map visual.
*
* For more information, see Creating point maps in the
* Amazon QuickSight User Guide.
*/
public void setGeospatialMapVisual(GeospatialMapVisual geospatialMapVisual) {
this.geospatialMapVisual = geospatialMapVisual;
}
/**
*
* A geospatial map or a points on map visual.
*
*
* For more information, see Creating
* point maps in the Amazon QuickSight User Guide.
*
*
* @return A geospatial map or a points on map visual.
*
* For more information, see Creating point maps in the
* Amazon QuickSight User Guide.
*/
public GeospatialMapVisual getGeospatialMapVisual() {
return this.geospatialMapVisual;
}
/**
*
* A geospatial map or a points on map visual.
*
*
* For more information, see Creating
* point maps in the Amazon QuickSight User Guide.
*
*
* @param geospatialMapVisual
* A geospatial map or a points on map visual.
*
* For more information, see Creating point maps in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withGeospatialMapVisual(GeospatialMapVisual geospatialMapVisual) {
setGeospatialMapVisual(geospatialMapVisual);
return this;
}
/**
*
* A filled map.
*
*
* For more information, see Creating
* filled maps in the Amazon QuickSight User Guide.
*
*
* @param filledMapVisual
* A filled map.
*
* For more information, see Creating filled maps in the
* Amazon QuickSight User Guide.
*/
public void setFilledMapVisual(FilledMapVisual filledMapVisual) {
this.filledMapVisual = filledMapVisual;
}
/**
*
* A filled map.
*
*
* For more information, see Creating
* filled maps in the Amazon QuickSight User Guide.
*
*
* @return A filled map.
*
* For more information, see Creating filled maps in
* the Amazon QuickSight User Guide.
*/
public FilledMapVisual getFilledMapVisual() {
return this.filledMapVisual;
}
/**
*
* A filled map.
*
*
* For more information, see Creating
* filled maps in the Amazon QuickSight User Guide.
*
*
* @param filledMapVisual
* A filled map.
*
* For more information, see Creating filled maps in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withFilledMapVisual(FilledMapVisual filledMapVisual) {
setFilledMapVisual(filledMapVisual);
return this;
}
/**
*
* A funnel chart.
*
*
* For more information, see Using funnel charts in
* the Amazon QuickSight User Guide.
*
*
* @param funnelChartVisual
* A funnel chart.
*
* For more information, see Using funnel
* charts in the Amazon QuickSight User Guide.
*/
public void setFunnelChartVisual(FunnelChartVisual funnelChartVisual) {
this.funnelChartVisual = funnelChartVisual;
}
/**
*
* A funnel chart.
*
*
* For more information, see Using funnel charts in
* the Amazon QuickSight User Guide.
*
*
* @return A funnel chart.
*
* For more information, see Using funnel
* charts in the Amazon QuickSight User Guide.
*/
public FunnelChartVisual getFunnelChartVisual() {
return this.funnelChartVisual;
}
/**
*
* A funnel chart.
*
*
* For more information, see Using funnel charts in
* the Amazon QuickSight User Guide.
*
*
* @param funnelChartVisual
* A funnel chart.
*
* For more information, see Using funnel
* charts in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withFunnelChartVisual(FunnelChartVisual funnelChartVisual) {
setFunnelChartVisual(funnelChartVisual);
return this;
}
/**
*
* A scatter plot.
*
*
* For more information, see Using
* scatter plots in the Amazon QuickSight User Guide.
*
*
* @param scatterPlotVisual
* A scatter plot.
*
* For more information, see Using scatter plots in the
* Amazon QuickSight User Guide.
*/
public void setScatterPlotVisual(ScatterPlotVisual scatterPlotVisual) {
this.scatterPlotVisual = scatterPlotVisual;
}
/**
*
* A scatter plot.
*
*
* For more information, see Using
* scatter plots in the Amazon QuickSight User Guide.
*
*
* @return A scatter plot.
*
* For more information, see Using scatter plots in
* the Amazon QuickSight User Guide.
*/
public ScatterPlotVisual getScatterPlotVisual() {
return this.scatterPlotVisual;
}
/**
*
* A scatter plot.
*
*
* For more information, see Using
* scatter plots in the Amazon QuickSight User Guide.
*
*
* @param scatterPlotVisual
* A scatter plot.
*
* For more information, see Using scatter plots in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withScatterPlotVisual(ScatterPlotVisual scatterPlotVisual) {
setScatterPlotVisual(scatterPlotVisual);
return this;
}
/**
*
* A combo chart.
*
*
* For more information, see Using
* combo charts in the Amazon QuickSight User Guide.
*
*
* @param comboChartVisual
* A combo chart.
*
* For more information, see Using combo charts in the
* Amazon QuickSight User Guide.
*/
public void setComboChartVisual(ComboChartVisual comboChartVisual) {
this.comboChartVisual = comboChartVisual;
}
/**
*
* A combo chart.
*
*
* For more information, see Using
* combo charts in the Amazon QuickSight User Guide.
*
*
* @return A combo chart.
*
* For more information, see Using combo charts in the
* Amazon QuickSight User Guide.
*/
public ComboChartVisual getComboChartVisual() {
return this.comboChartVisual;
}
/**
*
* A combo chart.
*
*
* For more information, see Using
* combo charts in the Amazon QuickSight User Guide.
*
*
* @param comboChartVisual
* A combo chart.
*
* For more information, see Using combo charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withComboChartVisual(ComboChartVisual comboChartVisual) {
setComboChartVisual(comboChartVisual);
return this;
}
/**
*
* A box plot.
*
*
* For more information, see Using box
* plots in the Amazon QuickSight User Guide.
*
*
* @param boxPlotVisual
* A box plot.
*
* For more information, see Using box plots in the
* Amazon QuickSight User Guide.
*/
public void setBoxPlotVisual(BoxPlotVisual boxPlotVisual) {
this.boxPlotVisual = boxPlotVisual;
}
/**
*
* A box plot.
*
*
* For more information, see Using box
* plots in the Amazon QuickSight User Guide.
*
*
* @return A box plot.
*
* For more information, see Using box plots in the
* Amazon QuickSight User Guide.
*/
public BoxPlotVisual getBoxPlotVisual() {
return this.boxPlotVisual;
}
/**
*
* A box plot.
*
*
* For more information, see Using box
* plots in the Amazon QuickSight User Guide.
*
*
* @param boxPlotVisual
* A box plot.
*
* For more information, see Using box plots in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withBoxPlotVisual(BoxPlotVisual boxPlotVisual) {
setBoxPlotVisual(boxPlotVisual);
return this;
}
/**
*
* A waterfall chart.
*
*
* For more information, see Using
* waterfall charts in the Amazon QuickSight User Guide.
*
*
* @param waterfallVisual
* A waterfall chart.
*
* For more information, see Using waterfall charts
* in the Amazon QuickSight User Guide.
*/
public void setWaterfallVisual(WaterfallVisual waterfallVisual) {
this.waterfallVisual = waterfallVisual;
}
/**
*
* A waterfall chart.
*
*
* For more information, see Using
* waterfall charts in the Amazon QuickSight User Guide.
*
*
* @return A waterfall chart.
*
* For more information, see Using waterfall charts
* in the Amazon QuickSight User Guide.
*/
public WaterfallVisual getWaterfallVisual() {
return this.waterfallVisual;
}
/**
*
* A waterfall chart.
*
*
* For more information, see Using
* waterfall charts in the Amazon QuickSight User Guide.
*
*
* @param waterfallVisual
* A waterfall chart.
*
* For more information, see Using waterfall charts
* in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withWaterfallVisual(WaterfallVisual waterfallVisual) {
setWaterfallVisual(waterfallVisual);
return this;
}
/**
*
* A histogram.
*
*
* For more information, see Using histograms in the
* Amazon QuickSight User Guide.
*
*
* @param histogramVisual
* A histogram.
*
* For more information, see Using histograms in
* the Amazon QuickSight User Guide.
*/
public void setHistogramVisual(HistogramVisual histogramVisual) {
this.histogramVisual = histogramVisual;
}
/**
*
* A histogram.
*
*
* For more information, see Using histograms in the
* Amazon QuickSight User Guide.
*
*
* @return A histogram.
*
* For more information, see Using histograms in
* the Amazon QuickSight User Guide.
*/
public HistogramVisual getHistogramVisual() {
return this.histogramVisual;
}
/**
*
* A histogram.
*
*
* For more information, see Using histograms in the
* Amazon QuickSight User Guide.
*
*
* @param histogramVisual
* A histogram.
*
* For more information, see Using histograms in
* the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withHistogramVisual(HistogramVisual histogramVisual) {
setHistogramVisual(histogramVisual);
return this;
}
/**
*
* A word cloud.
*
*
* For more information, see Using word
* clouds in the Amazon QuickSight User Guide.
*
*
* @param wordCloudVisual
* A word cloud.
*
* For more information, see Using word clouds in the
* Amazon QuickSight User Guide.
*/
public void setWordCloudVisual(WordCloudVisual wordCloudVisual) {
this.wordCloudVisual = wordCloudVisual;
}
/**
*
* A word cloud.
*
*
* For more information, see Using word
* clouds in the Amazon QuickSight User Guide.
*
*
* @return A word cloud.
*
* For more information, see Using word clouds in the
* Amazon QuickSight User Guide.
*/
public WordCloudVisual getWordCloudVisual() {
return this.wordCloudVisual;
}
/**
*
* A word cloud.
*
*
* For more information, see Using word
* clouds in the Amazon QuickSight User Guide.
*
*
* @param wordCloudVisual
* A word cloud.
*
* For more information, see Using word clouds in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withWordCloudVisual(WordCloudVisual wordCloudVisual) {
setWordCloudVisual(wordCloudVisual);
return this;
}
/**
*
* An insight visual.
*
*
* For more information, see Working with insights
* in the Amazon QuickSight User Guide.
*
*
* @param insightVisual
* An insight visual.
*
* For more information, see Working with
* insights in the Amazon QuickSight User Guide.
*/
public void setInsightVisual(InsightVisual insightVisual) {
this.insightVisual = insightVisual;
}
/**
*
* An insight visual.
*
*
* For more information, see Working with insights
* in the Amazon QuickSight User Guide.
*
*
* @return An insight visual.
*
* For more information, see Working with
* insights in the Amazon QuickSight User Guide.
*/
public InsightVisual getInsightVisual() {
return this.insightVisual;
}
/**
*
* An insight visual.
*
*
* For more information, see Working with insights
* in the Amazon QuickSight User Guide.
*
*
* @param insightVisual
* An insight visual.
*
* For more information, see Working with
* insights in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withInsightVisual(InsightVisual insightVisual) {
setInsightVisual(insightVisual);
return this;
}
/**
*
* A sankey diagram.
*
*
* For more information, see Using
* Sankey diagrams in the Amazon QuickSight User Guide.
*
*
* @param sankeyDiagramVisual
* A sankey diagram.
*
* For more information, see Using Sankey diagrams in
* the Amazon QuickSight User Guide.
*/
public void setSankeyDiagramVisual(SankeyDiagramVisual sankeyDiagramVisual) {
this.sankeyDiagramVisual = sankeyDiagramVisual;
}
/**
*
* A sankey diagram.
*
*
* For more information, see Using
* Sankey diagrams in the Amazon QuickSight User Guide.
*
*
* @return A sankey diagram.
*
* For more information, see Using Sankey diagrams
* in the Amazon QuickSight User Guide.
*/
public SankeyDiagramVisual getSankeyDiagramVisual() {
return this.sankeyDiagramVisual;
}
/**
*
* A sankey diagram.
*
*
* For more information, see Using
* Sankey diagrams in the Amazon QuickSight User Guide.
*
*
* @param sankeyDiagramVisual
* A sankey diagram.
*
* For more information, see Using Sankey diagrams in
* the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withSankeyDiagramVisual(SankeyDiagramVisual sankeyDiagramVisual) {
setSankeyDiagramVisual(sankeyDiagramVisual);
return this;
}
/**
*
* A visual that contains custom content.
*
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*
*
* @param customContentVisual
* A visual that contains custom content.
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*/
public void setCustomContentVisual(CustomContentVisual customContentVisual) {
this.customContentVisual = customContentVisual;
}
/**
*
* A visual that contains custom content.
*
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*
*
* @return A visual that contains custom content.
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*/
public CustomContentVisual getCustomContentVisual() {
return this.customContentVisual;
}
/**
*
* A visual that contains custom content.
*
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
*
*
* @param customContentVisual
* A visual that contains custom content.
*
* For more information, see Using custom visual
* content in the Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withCustomContentVisual(CustomContentVisual customContentVisual) {
setCustomContentVisual(customContentVisual);
return this;
}
/**
*
* An empty visual.
*
*
* @param emptyVisual
* An empty visual.
*/
public void setEmptyVisual(EmptyVisual emptyVisual) {
this.emptyVisual = emptyVisual;
}
/**
*
* An empty visual.
*
*
* @return An empty visual.
*/
public EmptyVisual getEmptyVisual() {
return this.emptyVisual;
}
/**
*
* An empty visual.
*
*
* @param emptyVisual
* An empty visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withEmptyVisual(EmptyVisual emptyVisual) {
setEmptyVisual(emptyVisual);
return this;
}
/**
*
* A radar chart visual.
*
*
* For more information, see Using
* radar charts in the Amazon QuickSight User Guide.
*
*
* @param radarChartVisual
* A radar chart visual.
*
* For more information, see Using radar charts in the
* Amazon QuickSight User Guide.
*/
public void setRadarChartVisual(RadarChartVisual radarChartVisual) {
this.radarChartVisual = radarChartVisual;
}
/**
*
* A radar chart visual.
*
*
* For more information, see Using
* radar charts in the Amazon QuickSight User Guide.
*
*
* @return A radar chart visual.
*
* For more information, see Using radar charts in the
* Amazon QuickSight User Guide.
*/
public RadarChartVisual getRadarChartVisual() {
return this.radarChartVisual;
}
/**
*
* A radar chart visual.
*
*
* For more information, see Using
* radar charts in the Amazon QuickSight User Guide.
*
*
* @param radarChartVisual
* A radar chart visual.
*
* For more information, see Using radar charts in the
* Amazon QuickSight User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Visual withRadarChartVisual(RadarChartVisual radarChartVisual) {
setRadarChartVisual(radarChartVisual);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTableVisual() != null)
sb.append("TableVisual: ").append(getTableVisual()).append(",");
if (getPivotTableVisual() != null)
sb.append("PivotTableVisual: ").append(getPivotTableVisual()).append(",");
if (getBarChartVisual() != null)
sb.append("BarChartVisual: ").append(getBarChartVisual()).append(",");
if (getKPIVisual() != null)
sb.append("KPIVisual: ").append(getKPIVisual()).append(",");
if (getPieChartVisual() != null)
sb.append("PieChartVisual: ").append(getPieChartVisual()).append(",");
if (getGaugeChartVisual() != null)
sb.append("GaugeChartVisual: ").append(getGaugeChartVisual()).append(",");
if (getLineChartVisual() != null)
sb.append("LineChartVisual: ").append(getLineChartVisual()).append(",");
if (getHeatMapVisual() != null)
sb.append("HeatMapVisual: ").append(getHeatMapVisual()).append(",");
if (getTreeMapVisual() != null)
sb.append("TreeMapVisual: ").append(getTreeMapVisual()).append(",");
if (getGeospatialMapVisual() != null)
sb.append("GeospatialMapVisual: ").append(getGeospatialMapVisual()).append(",");
if (getFilledMapVisual() != null)
sb.append("FilledMapVisual: ").append(getFilledMapVisual()).append(",");
if (getFunnelChartVisual() != null)
sb.append("FunnelChartVisual: ").append(getFunnelChartVisual()).append(",");
if (getScatterPlotVisual() != null)
sb.append("ScatterPlotVisual: ").append(getScatterPlotVisual()).append(",");
if (getComboChartVisual() != null)
sb.append("ComboChartVisual: ").append(getComboChartVisual()).append(",");
if (getBoxPlotVisual() != null)
sb.append("BoxPlotVisual: ").append(getBoxPlotVisual()).append(",");
if (getWaterfallVisual() != null)
sb.append("WaterfallVisual: ").append(getWaterfallVisual()).append(",");
if (getHistogramVisual() != null)
sb.append("HistogramVisual: ").append(getHistogramVisual()).append(",");
if (getWordCloudVisual() != null)
sb.append("WordCloudVisual: ").append(getWordCloudVisual()).append(",");
if (getInsightVisual() != null)
sb.append("InsightVisual: ").append(getInsightVisual()).append(",");
if (getSankeyDiagramVisual() != null)
sb.append("SankeyDiagramVisual: ").append(getSankeyDiagramVisual()).append(",");
if (getCustomContentVisual() != null)
sb.append("CustomContentVisual: ").append(getCustomContentVisual()).append(",");
if (getEmptyVisual() != null)
sb.append("EmptyVisual: ").append(getEmptyVisual()).append(",");
if (getRadarChartVisual() != null)
sb.append("RadarChartVisual: ").append(getRadarChartVisual());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Visual == false)
return false;
Visual other = (Visual) obj;
if (other.getTableVisual() == null ^ this.getTableVisual() == null)
return false;
if (other.getTableVisual() != null && other.getTableVisual().equals(this.getTableVisual()) == false)
return false;
if (other.getPivotTableVisual() == null ^ this.getPivotTableVisual() == null)
return false;
if (other.getPivotTableVisual() != null && other.getPivotTableVisual().equals(this.getPivotTableVisual()) == false)
return false;
if (other.getBarChartVisual() == null ^ this.getBarChartVisual() == null)
return false;
if (other.getBarChartVisual() != null && other.getBarChartVisual().equals(this.getBarChartVisual()) == false)
return false;
if (other.getKPIVisual() == null ^ this.getKPIVisual() == null)
return false;
if (other.getKPIVisual() != null && other.getKPIVisual().equals(this.getKPIVisual()) == false)
return false;
if (other.getPieChartVisual() == null ^ this.getPieChartVisual() == null)
return false;
if (other.getPieChartVisual() != null && other.getPieChartVisual().equals(this.getPieChartVisual()) == false)
return false;
if (other.getGaugeChartVisual() == null ^ this.getGaugeChartVisual() == null)
return false;
if (other.getGaugeChartVisual() != null && other.getGaugeChartVisual().equals(this.getGaugeChartVisual()) == false)
return false;
if (other.getLineChartVisual() == null ^ this.getLineChartVisual() == null)
return false;
if (other.getLineChartVisual() != null && other.getLineChartVisual().equals(this.getLineChartVisual()) == false)
return false;
if (other.getHeatMapVisual() == null ^ this.getHeatMapVisual() == null)
return false;
if (other.getHeatMapVisual() != null && other.getHeatMapVisual().equals(this.getHeatMapVisual()) == false)
return false;
if (other.getTreeMapVisual() == null ^ this.getTreeMapVisual() == null)
return false;
if (other.getTreeMapVisual() != null && other.getTreeMapVisual().equals(this.getTreeMapVisual()) == false)
return false;
if (other.getGeospatialMapVisual() == null ^ this.getGeospatialMapVisual() == null)
return false;
if (other.getGeospatialMapVisual() != null && other.getGeospatialMapVisual().equals(this.getGeospatialMapVisual()) == false)
return false;
if (other.getFilledMapVisual() == null ^ this.getFilledMapVisual() == null)
return false;
if (other.getFilledMapVisual() != null && other.getFilledMapVisual().equals(this.getFilledMapVisual()) == false)
return false;
if (other.getFunnelChartVisual() == null ^ this.getFunnelChartVisual() == null)
return false;
if (other.getFunnelChartVisual() != null && other.getFunnelChartVisual().equals(this.getFunnelChartVisual()) == false)
return false;
if (other.getScatterPlotVisual() == null ^ this.getScatterPlotVisual() == null)
return false;
if (other.getScatterPlotVisual() != null && other.getScatterPlotVisual().equals(this.getScatterPlotVisual()) == false)
return false;
if (other.getComboChartVisual() == null ^ this.getComboChartVisual() == null)
return false;
if (other.getComboChartVisual() != null && other.getComboChartVisual().equals(this.getComboChartVisual()) == false)
return false;
if (other.getBoxPlotVisual() == null ^ this.getBoxPlotVisual() == null)
return false;
if (other.getBoxPlotVisual() != null && other.getBoxPlotVisual().equals(this.getBoxPlotVisual()) == false)
return false;
if (other.getWaterfallVisual() == null ^ this.getWaterfallVisual() == null)
return false;
if (other.getWaterfallVisual() != null && other.getWaterfallVisual().equals(this.getWaterfallVisual()) == false)
return false;
if (other.getHistogramVisual() == null ^ this.getHistogramVisual() == null)
return false;
if (other.getHistogramVisual() != null && other.getHistogramVisual().equals(this.getHistogramVisual()) == false)
return false;
if (other.getWordCloudVisual() == null ^ this.getWordCloudVisual() == null)
return false;
if (other.getWordCloudVisual() != null && other.getWordCloudVisual().equals(this.getWordCloudVisual()) == false)
return false;
if (other.getInsightVisual() == null ^ this.getInsightVisual() == null)
return false;
if (other.getInsightVisual() != null && other.getInsightVisual().equals(this.getInsightVisual()) == false)
return false;
if (other.getSankeyDiagramVisual() == null ^ this.getSankeyDiagramVisual() == null)
return false;
if (other.getSankeyDiagramVisual() != null && other.getSankeyDiagramVisual().equals(this.getSankeyDiagramVisual()) == false)
return false;
if (other.getCustomContentVisual() == null ^ this.getCustomContentVisual() == null)
return false;
if (other.getCustomContentVisual() != null && other.getCustomContentVisual().equals(this.getCustomContentVisual()) == false)
return false;
if (other.getEmptyVisual() == null ^ this.getEmptyVisual() == null)
return false;
if (other.getEmptyVisual() != null && other.getEmptyVisual().equals(this.getEmptyVisual()) == false)
return false;
if (other.getRadarChartVisual() == null ^ this.getRadarChartVisual() == null)
return false;
if (other.getRadarChartVisual() != null && other.getRadarChartVisual().equals(this.getRadarChartVisual()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTableVisual() == null) ? 0 : getTableVisual().hashCode());
hashCode = prime * hashCode + ((getPivotTableVisual() == null) ? 0 : getPivotTableVisual().hashCode());
hashCode = prime * hashCode + ((getBarChartVisual() == null) ? 0 : getBarChartVisual().hashCode());
hashCode = prime * hashCode + ((getKPIVisual() == null) ? 0 : getKPIVisual().hashCode());
hashCode = prime * hashCode + ((getPieChartVisual() == null) ? 0 : getPieChartVisual().hashCode());
hashCode = prime * hashCode + ((getGaugeChartVisual() == null) ? 0 : getGaugeChartVisual().hashCode());
hashCode = prime * hashCode + ((getLineChartVisual() == null) ? 0 : getLineChartVisual().hashCode());
hashCode = prime * hashCode + ((getHeatMapVisual() == null) ? 0 : getHeatMapVisual().hashCode());
hashCode = prime * hashCode + ((getTreeMapVisual() == null) ? 0 : getTreeMapVisual().hashCode());
hashCode = prime * hashCode + ((getGeospatialMapVisual() == null) ? 0 : getGeospatialMapVisual().hashCode());
hashCode = prime * hashCode + ((getFilledMapVisual() == null) ? 0 : getFilledMapVisual().hashCode());
hashCode = prime * hashCode + ((getFunnelChartVisual() == null) ? 0 : getFunnelChartVisual().hashCode());
hashCode = prime * hashCode + ((getScatterPlotVisual() == null) ? 0 : getScatterPlotVisual().hashCode());
hashCode = prime * hashCode + ((getComboChartVisual() == null) ? 0 : getComboChartVisual().hashCode());
hashCode = prime * hashCode + ((getBoxPlotVisual() == null) ? 0 : getBoxPlotVisual().hashCode());
hashCode = prime * hashCode + ((getWaterfallVisual() == null) ? 0 : getWaterfallVisual().hashCode());
hashCode = prime * hashCode + ((getHistogramVisual() == null) ? 0 : getHistogramVisual().hashCode());
hashCode = prime * hashCode + ((getWordCloudVisual() == null) ? 0 : getWordCloudVisual().hashCode());
hashCode = prime * hashCode + ((getInsightVisual() == null) ? 0 : getInsightVisual().hashCode());
hashCode = prime * hashCode + ((getSankeyDiagramVisual() == null) ? 0 : getSankeyDiagramVisual().hashCode());
hashCode = prime * hashCode + ((getCustomContentVisual() == null) ? 0 : getCustomContentVisual().hashCode());
hashCode = prime * hashCode + ((getEmptyVisual() == null) ? 0 : getEmptyVisual().hashCode());
hashCode = prime * hashCode + ((getRadarChartVisual() == null) ? 0 : getRadarChartVisual().hashCode());
return hashCode;
}
@Override
public Visual clone() {
try {
return (Visual) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.VisualMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}