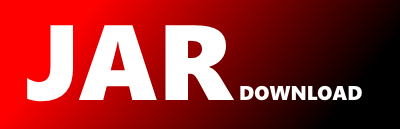
com.amazonaws.services.quicksight.AmazonQuickSightAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight;
import javax.annotation.Generated;
import com.amazonaws.services.quicksight.model.*;
/**
* Interface for accessing Amazon QuickSight asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.quicksight.AbstractAmazonQuickSightAsync} instead.
*
*
* Amazon QuickSight API Reference
*
* Amazon QuickSight is a fully managed, serverless business intelligence service for the Amazon Web Services Cloud that
* makes it easy to extend data and insights to every user in your organization. This API reference contains
* documentation for a programming interface that you can use to manage Amazon QuickSight.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonQuickSightAsync extends AmazonQuickSight {
/**
*
* Creates new reviewed answers for a Q Topic.
*
*
* @param batchCreateTopicReviewedAnswerRequest
* @return A Java Future containing the result of the BatchCreateTopicReviewedAnswer operation returned by the
* service.
* @sample AmazonQuickSightAsync.BatchCreateTopicReviewedAnswer
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateTopicReviewedAnswerAsync(
BatchCreateTopicReviewedAnswerRequest batchCreateTopicReviewedAnswerRequest);
/**
*
* Creates new reviewed answers for a Q Topic.
*
*
* @param batchCreateTopicReviewedAnswerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateTopicReviewedAnswer operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.BatchCreateTopicReviewedAnswer
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateTopicReviewedAnswerAsync(
BatchCreateTopicReviewedAnswerRequest batchCreateTopicReviewedAnswerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes reviewed answers for Q Topic.
*
*
* @param batchDeleteTopicReviewedAnswerRequest
* @return A Java Future containing the result of the BatchDeleteTopicReviewedAnswer operation returned by the
* service.
* @sample AmazonQuickSightAsync.BatchDeleteTopicReviewedAnswer
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteTopicReviewedAnswerAsync(
BatchDeleteTopicReviewedAnswerRequest batchDeleteTopicReviewedAnswerRequest);
/**
*
* Deletes reviewed answers for Q Topic.
*
*
* @param batchDeleteTopicReviewedAnswerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteTopicReviewedAnswer operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.BatchDeleteTopicReviewedAnswer
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteTopicReviewedAnswerAsync(
BatchDeleteTopicReviewedAnswerRequest batchDeleteTopicReviewedAnswerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an ongoing ingestion of data into SPICE.
*
*
* @param cancelIngestionRequest
* @return A Java Future containing the result of the CancelIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.CancelIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelIngestionAsync(CancelIngestionRequest cancelIngestionRequest);
/**
*
* Cancels an ongoing ingestion of data into SPICE.
*
*
* @param cancelIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CancelIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelIngestionAsync(CancelIngestionRequest cancelIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates Amazon QuickSight customizations for the current Amazon Web Services Region. Currently, you can add a
* custom default theme by using the CreateAccountCustomization
or
* UpdateAccountCustomization
API operation. To further customize Amazon QuickSight by removing Amazon
* QuickSight sample assets and videos for all new users, see Customizing Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* You can create customizations for your Amazon Web Services account or, if you specify a namespace, for a
* QuickSight namespace instead. Customizations that apply to a namespace always override customizations that apply
* to an Amazon Web Services account. To find out which customizations apply, use the
* DescribeAccountCustomization
API operation.
*
*
* Before you use the CreateAccountCustomization
API operation to add a theme as the namespace default,
* make sure that you first share the theme with the namespace. If you don't share it with the namespace, the theme
* isn't visible to your users even if you make it the default theme. To check if the theme is shared, view the
* current permissions by using the
* DescribeThemePermissions
* API operation. To share the theme, grant permissions by using the
* UpdateThemePermissions
* API operation.
*
*
* @param createAccountCustomizationRequest
* @return A Java Future containing the result of the CreateAccountCustomization operation returned by the service.
* @sample AmazonQuickSightAsync.CreateAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccountCustomizationAsync(
CreateAccountCustomizationRequest createAccountCustomizationRequest);
/**
*
* Creates Amazon QuickSight customizations for the current Amazon Web Services Region. Currently, you can add a
* custom default theme by using the CreateAccountCustomization
or
* UpdateAccountCustomization
API operation. To further customize Amazon QuickSight by removing Amazon
* QuickSight sample assets and videos for all new users, see Customizing Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* You can create customizations for your Amazon Web Services account or, if you specify a namespace, for a
* QuickSight namespace instead. Customizations that apply to a namespace always override customizations that apply
* to an Amazon Web Services account. To find out which customizations apply, use the
* DescribeAccountCustomization
API operation.
*
*
* Before you use the CreateAccountCustomization
API operation to add a theme as the namespace default,
* make sure that you first share the theme with the namespace. If you don't share it with the namespace, the theme
* isn't visible to your users even if you make it the default theme. To check if the theme is shared, view the
* current permissions by using the
* DescribeThemePermissions
* API operation. To share the theme, grant permissions by using the
* UpdateThemePermissions
* API operation.
*
*
* @param createAccountCustomizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccountCustomization operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccountCustomizationAsync(
CreateAccountCustomizationRequest createAccountCustomizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon QuickSight account, or subscribes to Amazon QuickSight Q.
*
*
* The Amazon Web Services Region for the account is derived from what is configured in the CLI or SDK.
*
*
* Before you use this operation, make sure that you can connect to an existing Amazon Web Services account. If you
* don't have an Amazon Web Services account, see Sign up for Amazon Web
* Services in the Amazon QuickSight User Guide. The person who signs up for Amazon QuickSight needs to
* have the correct Identity and Access Management (IAM) permissions. For more information, see IAM Policy Examples for Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* If your IAM policy includes both the Subscribe
and CreateAccountSubscription
actions,
* make sure that both actions are set to Allow
. If either action is set to Deny
, the
* Deny
action prevails and your API call fails.
*
*
* You can't pass an existing IAM role to access other Amazon Web Services services using this API operation. To
* pass your existing IAM role to Amazon QuickSight, see Passing IAM roles to Amazon QuickSight in the Amazon QuickSight User Guide.
*
*
* You can't set default resource access on the new account from the Amazon QuickSight API. Instead, add default
* resource access from the Amazon QuickSight console. For more information about setting default resource access to
* Amazon Web Services services, see Setting default resource
* access to Amazon Web Services services in the Amazon QuickSight User Guide.
*
*
* @param createAccountSubscriptionRequest
* @return A Java Future containing the result of the CreateAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsync.CreateAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccountSubscriptionAsync(
CreateAccountSubscriptionRequest createAccountSubscriptionRequest);
/**
*
* Creates an Amazon QuickSight account, or subscribes to Amazon QuickSight Q.
*
*
* The Amazon Web Services Region for the account is derived from what is configured in the CLI or SDK.
*
*
* Before you use this operation, make sure that you can connect to an existing Amazon Web Services account. If you
* don't have an Amazon Web Services account, see Sign up for Amazon Web
* Services in the Amazon QuickSight User Guide. The person who signs up for Amazon QuickSight needs to
* have the correct Identity and Access Management (IAM) permissions. For more information, see IAM Policy Examples for Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* If your IAM policy includes both the Subscribe
and CreateAccountSubscription
actions,
* make sure that both actions are set to Allow
. If either action is set to Deny
, the
* Deny
action prevails and your API call fails.
*
*
* You can't pass an existing IAM role to access other Amazon Web Services services using this API operation. To
* pass your existing IAM role to Amazon QuickSight, see Passing IAM roles to Amazon QuickSight in the Amazon QuickSight User Guide.
*
*
* You can't set default resource access on the new account from the Amazon QuickSight API. Instead, add default
* resource access from the Amazon QuickSight console. For more information about setting default resource access to
* Amazon Web Services services, see Setting default resource
* access to Amazon Web Services services in the Amazon QuickSight User Guide.
*
*
* @param createAccountSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createAccountSubscriptionAsync(
CreateAccountSubscriptionRequest createAccountSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an analysis in Amazon QuickSight. Analyses can be created either from a template or from an
* AnalysisDefinition
.
*
*
* @param createAnalysisRequest
* @return A Java Future containing the result of the CreateAnalysis operation returned by the service.
* @sample AmazonQuickSightAsync.CreateAnalysis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAnalysisAsync(CreateAnalysisRequest createAnalysisRequest);
/**
*
* Creates an analysis in Amazon QuickSight. Analyses can be created either from a template or from an
* AnalysisDefinition
.
*
*
* @param createAnalysisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAnalysis operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateAnalysis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAnalysisAsync(CreateAnalysisRequest createAnalysisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dashboard from either a template or directly with a DashboardDefinition
. To first create a
* template, see the
* CreateTemplate
* API operation.
*
*
* A dashboard is an entity in Amazon QuickSight that identifies Amazon QuickSight reports, created from analyses.
* You can share Amazon QuickSight dashboards. With the right permissions, you can create scheduled email reports
* from them. If you have the correct permissions, you can create a dashboard from a template that exists in a
* different Amazon Web Services account.
*
*
* @param createDashboardRequest
* @return A Java Future containing the result of the CreateDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDashboardAsync(CreateDashboardRequest createDashboardRequest);
/**
*
* Creates a dashboard from either a template or directly with a DashboardDefinition
. To first create a
* template, see the
* CreateTemplate
* API operation.
*
*
* A dashboard is an entity in Amazon QuickSight that identifies Amazon QuickSight reports, created from analyses.
* You can share Amazon QuickSight dashboards. With the right permissions, you can create scheduled email reports
* from them. If you have the correct permissions, you can create a dashboard from a template that exists in a
* different Amazon Web Services account.
*
*
* @param createDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDashboardAsync(CreateDashboardRequest createDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param createDataSetRequest
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest);
/**
*
* Creates a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param createDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataSetAsync(CreateDataSetRequest createDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data source.
*
*
* @param createDataSourceRequest
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.CreateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest);
/**
*
* Creates a data source.
*
*
* @param createDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDataSourceAsync(CreateDataSourceRequest createDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an empty shared folder.
*
*
* @param createFolderRequest
* @return A Java Future containing the result of the CreateFolder operation returned by the service.
* @sample AmazonQuickSightAsync.CreateFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFolderAsync(CreateFolderRequest createFolderRequest);
/**
*
* Creates an empty shared folder.
*
*
* @param createFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFolder operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFolderAsync(CreateFolderRequest createFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an asset, such as a dashboard, analysis, or dataset into a folder.
*
*
* @param createFolderMembershipRequest
* @return A Java Future containing the result of the CreateFolderMembership operation returned by the service.
* @sample AmazonQuickSightAsync.CreateFolderMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createFolderMembershipAsync(CreateFolderMembershipRequest createFolderMembershipRequest);
/**
*
* Adds an asset, such as a dashboard, analysis, or dataset into a folder.
*
*
* @param createFolderMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFolderMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateFolderMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createFolderMembershipAsync(CreateFolderMembershipRequest createFolderMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the CreateGroup
operation to create a group in Amazon QuickSight. You can create up to 10,000
* groups in a namespace. If you want to create more than 10,000 groups in a namespace, contact Amazon Web Services
* Support.
*
*
* The permissions resource is
* arn:aws:quicksight:<your-region>:<relevant-aws-account-id>:group/default/<group-name>
* .
*
*
* The response is a group object.
*
*
* @param createGroupRequest
* The request object for this operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AmazonQuickSightAsync.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest);
/**
*
* Use the CreateGroup
operation to create a group in Amazon QuickSight. You can create up to 10,000
* groups in a namespace. If you want to create more than 10,000 groups in a namespace, contact Amazon Web Services
* Support.
*
*
* The permissions resource is
* arn:aws:quicksight:<your-region>:<relevant-aws-account-id>:group/default/<group-name>
* .
*
*
* The response is a group object.
*
*
* @param createGroupRequest
* The request object for this operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an Amazon QuickSight user to an Amazon QuickSight group.
*
*
* @param createGroupMembershipRequest
* @return A Java Future containing the result of the CreateGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsync.CreateGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createGroupMembershipAsync(CreateGroupMembershipRequest createGroupMembershipRequest);
/**
*
* Adds an Amazon QuickSight user to an Amazon QuickSight group.
*
*
* @param createGroupMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createGroupMembershipAsync(CreateGroupMembershipRequest createGroupMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an assignment with one specified IAM policy, identified by its Amazon Resource Name (ARN). This policy
* assignment is attached to the specified groups or users of Amazon QuickSight. Assignment names are unique per
* Amazon Web Services account. To avoid overwriting rules in other namespaces, use assignment names that are
* unique.
*
*
* @param createIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the CreateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.CreateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future createIAMPolicyAssignmentAsync(
CreateIAMPolicyAssignmentRequest createIAMPolicyAssignmentRequest);
/**
*
* Creates an assignment with one specified IAM policy, identified by its Amazon Resource Name (ARN). This policy
* assignment is attached to the specified groups or users of Amazon QuickSight. Assignment names are unique per
* Amazon Web Services account. To avoid overwriting rules in other namespaces, use assignment names that are
* unique.
*
*
* @param createIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future createIAMPolicyAssignmentAsync(
CreateIAMPolicyAssignmentRequest createIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates and starts a new SPICE ingestion for a dataset. You can manually refresh datasets in an Enterprise
* edition account 32 times in a 24-hour period. You can manually refresh datasets in a Standard edition account 8
* times in a 24-hour period. Each 24-hour period is measured starting 24 hours before the current date and time.
*
*
* Any ingestions operating on tagged datasets inherit the same tags automatically for use in access control. For an
* example, see How do I
* create an IAM policy to control access to Amazon EC2 resources using tags? in the Amazon Web Services
* Knowledge Center. Tags are visible on the tagged dataset, but not on the ingestion resource.
*
*
* @param createIngestionRequest
* @return A Java Future containing the result of the CreateIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.CreateIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIngestionAsync(CreateIngestionRequest createIngestionRequest);
/**
*
* Creates and starts a new SPICE ingestion for a dataset. You can manually refresh datasets in an Enterprise
* edition account 32 times in a 24-hour period. You can manually refresh datasets in a Standard edition account 8
* times in a 24-hour period. Each 24-hour period is measured starting 24 hours before the current date and time.
*
*
* Any ingestions operating on tagged datasets inherit the same tags automatically for use in access control. For an
* example, see How do I
* create an IAM policy to control access to Amazon EC2 resources using tags? in the Amazon Web Services
* Knowledge Center. Tags are visible on the tagged dataset, but not on the ingestion resource.
*
*
* @param createIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateIngestion
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIngestionAsync(CreateIngestionRequest createIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* (Enterprise edition only) Creates a new namespace for you to use with Amazon QuickSight.
*
*
* A namespace allows you to isolate the Amazon QuickSight users and groups that are registered for that namespace.
* Users that access the namespace can share assets only with other users or groups in the same namespace. They
* can't see users and groups in other namespaces. You can create a namespace after your Amazon Web Services account
* is subscribed to Amazon QuickSight. The namespace must be unique within the Amazon Web Services account. By
* default, there is a limit of 100 namespaces per Amazon Web Services account. To increase your limit, create a
* ticket with Amazon Web Services Support.
*
*
* @param createNamespaceRequest
* @return A Java Future containing the result of the CreateNamespace operation returned by the service.
* @sample AmazonQuickSightAsync.CreateNamespace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNamespaceAsync(CreateNamespaceRequest createNamespaceRequest);
/**
*
* (Enterprise edition only) Creates a new namespace for you to use with Amazon QuickSight.
*
*
* A namespace allows you to isolate the Amazon QuickSight users and groups that are registered for that namespace.
* Users that access the namespace can share assets only with other users or groups in the same namespace. They
* can't see users and groups in other namespaces. You can create a namespace after your Amazon Web Services account
* is subscribed to Amazon QuickSight. The namespace must be unique within the Amazon Web Services account. By
* default, there is a limit of 100 namespaces per Amazon Web Services account. To increase your limit, create a
* ticket with Amazon Web Services Support.
*
*
* @param createNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNamespace operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateNamespace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNamespaceAsync(CreateNamespaceRequest createNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a refresh schedule for a dataset. You can create up to 5 different schedules for a single dataset.
*
*
* @param createRefreshScheduleRequest
* @return A Java Future containing the result of the CreateRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsync.CreateRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createRefreshScheduleAsync(CreateRefreshScheduleRequest createRefreshScheduleRequest);
/**
*
* Creates a refresh schedule for a dataset. You can create up to 5 different schedules for a single dataset.
*
*
* @param createRefreshScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createRefreshScheduleAsync(CreateRefreshScheduleRequest createRefreshScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use CreateRoleMembership
to add an existing Amazon QuickSight group to an existing role.
*
*
* @param createRoleMembershipRequest
* @return A Java Future containing the result of the CreateRoleMembership operation returned by the service.
* @sample AmazonQuickSightAsync.CreateRoleMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createRoleMembershipAsync(CreateRoleMembershipRequest createRoleMembershipRequest);
/**
*
* Use CreateRoleMembership
to add an existing Amazon QuickSight group to an existing role.
*
*
* @param createRoleMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRoleMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateRoleMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createRoleMembershipAsync(CreateRoleMembershipRequest createRoleMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a template either from a TemplateDefinition
or from an existing Amazon QuickSight analysis
* or template. You can use the resulting template to create additional dashboards, templates, or analyses.
*
*
* A template is an entity in Amazon QuickSight that encapsulates the metadata required to create an analysis
* and that you can use to create s dashboard. A template adds a layer of abstraction by using placeholders to
* replace the dataset associated with the analysis. You can use templates to create dashboards by replacing dataset
* placeholders with datasets that follow the same schema that was used to create the source analysis and template.
*
*
* @param createTemplateRequest
* @return A Java Future containing the result of the CreateTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTemplateAsync(CreateTemplateRequest createTemplateRequest);
/**
*
* Creates a template either from a TemplateDefinition
or from an existing Amazon QuickSight analysis
* or template. You can use the resulting template to create additional dashboards, templates, or analyses.
*
*
* A template is an entity in Amazon QuickSight that encapsulates the metadata required to create an analysis
* and that you can use to create s dashboard. A template adds a layer of abstraction by using placeholders to
* replace the dataset associated with the analysis. You can use templates to create dashboards by replacing dataset
* placeholders with datasets that follow the same schema that was used to create the source analysis and template.
*
*
* @param createTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTemplateAsync(CreateTemplateRequest createTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a template alias for a template.
*
*
* @param createTemplateAliasRequest
* @return A Java Future containing the result of the CreateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTemplateAliasAsync(CreateTemplateAliasRequest createTemplateAliasRequest);
/**
*
* Creates a template alias for a template.
*
*
* @param createTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTemplateAliasAsync(CreateTemplateAliasRequest createTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a theme.
*
*
* A theme is set of configuration options for color and layout. Themes apply to analyses and dashboards. For
* more information, see Using Themes in Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* @param createThemeRequest
* @return A Java Future containing the result of the CreateTheme operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTheme
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createThemeAsync(CreateThemeRequest createThemeRequest);
/**
*
* Creates a theme.
*
*
* A theme is set of configuration options for color and layout. Themes apply to analyses and dashboards. For
* more information, see Using Themes in Amazon
* QuickSight in the Amazon QuickSight User Guide.
*
*
* @param createThemeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTheme operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTheme
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createThemeAsync(CreateThemeRequest createThemeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a theme alias for a theme.
*
*
* @param createThemeAliasRequest
* @return A Java Future containing the result of the CreateThemeAlias operation returned by the service.
* @sample AmazonQuickSightAsync.CreateThemeAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createThemeAliasAsync(CreateThemeAliasRequest createThemeAliasRequest);
/**
*
* Creates a theme alias for a theme.
*
*
* @param createThemeAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateThemeAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateThemeAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createThemeAliasAsync(CreateThemeAliasRequest createThemeAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Q topic.
*
*
* @param createTopicRequest
* @return A Java Future containing the result of the CreateTopic operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTopicAsync(CreateTopicRequest createTopicRequest);
/**
*
* Creates a new Q topic.
*
*
* @param createTopicRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTopic operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTopicAsync(CreateTopicRequest createTopicRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a topic refresh schedule.
*
*
* @param createTopicRefreshScheduleRequest
* @return A Java Future containing the result of the CreateTopicRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsync.CreateTopicRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createTopicRefreshScheduleAsync(
CreateTopicRefreshScheduleRequest createTopicRefreshScheduleRequest);
/**
*
* Creates a topic refresh schedule.
*
*
* @param createTopicRefreshScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTopicRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateTopicRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createTopicRefreshScheduleAsync(
CreateTopicRefreshScheduleRequest createTopicRefreshScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new VPC connection.
*
*
* @param createVPCConnectionRequest
* @return A Java Future containing the result of the CreateVPCConnection operation returned by the service.
* @sample AmazonQuickSightAsync.CreateVPCConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVPCConnectionAsync(CreateVPCConnectionRequest createVPCConnectionRequest);
/**
*
* Creates a new VPC connection.
*
*
* @param createVPCConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVPCConnection operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.CreateVPCConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVPCConnectionAsync(CreateVPCConnectionRequest createVPCConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all Amazon QuickSight customizations in this Amazon Web Services Region for the specified Amazon Web
* Services account and Amazon QuickSight namespace.
*
*
* @param deleteAccountCustomizationRequest
* @return A Java Future containing the result of the DeleteAccountCustomization operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountCustomizationAsync(
DeleteAccountCustomizationRequest deleteAccountCustomizationRequest);
/**
*
* Deletes all Amazon QuickSight customizations in this Amazon Web Services Region for the specified Amazon Web
* Services account and Amazon QuickSight namespace.
*
*
* @param deleteAccountCustomizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccountCustomization operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountCustomizationAsync(
DeleteAccountCustomizationRequest deleteAccountCustomizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the DeleteAccountSubscription
operation to delete an Amazon QuickSight account. This operation
* will result in an error message if you have configured your account termination protection settings to
* True
. To change this setting and delete your account, call the UpdateAccountSettings
* API and set the value of the TerminationProtectionEnabled
parameter to False
, then make
* another call to the DeleteAccountSubscription
API.
*
*
* @param deleteAccountSubscriptionRequest
* @return A Java Future containing the result of the DeleteAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountSubscriptionAsync(
DeleteAccountSubscriptionRequest deleteAccountSubscriptionRequest);
/**
*
* Use the DeleteAccountSubscription
operation to delete an Amazon QuickSight account. This operation
* will result in an error message if you have configured your account termination protection settings to
* True
. To change this setting and delete your account, call the UpdateAccountSettings
* API and set the value of the TerminationProtectionEnabled
parameter to False
, then make
* another call to the DeleteAccountSubscription
API.
*
*
* @param deleteAccountSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAccountSubscriptionAsync(
DeleteAccountSubscriptionRequest deleteAccountSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an analysis from Amazon QuickSight. You can optionally include a recovery window during which you can
* restore the analysis. If you don't specify a recovery window value, the operation defaults to 30 days. Amazon
* QuickSight attaches a DeletionTime
stamp to the response that specifies the end of the recovery
* window. At the end of the recovery window, Amazon QuickSight deletes the analysis permanently.
*
*
* At any time before recovery window ends, you can use the RestoreAnalysis
API operation to remove the
* DeletionTime
stamp and cancel the deletion of the analysis. The analysis remains visible in the API
* until it's deleted, so you can describe it but you can't make a template from it.
*
*
* An analysis that's scheduled for deletion isn't accessible in the Amazon QuickSight console. To access it in the
* console, restore it. Deleting an analysis doesn't delete the dashboards that you publish from it.
*
*
* @param deleteAnalysisRequest
* @return A Java Future containing the result of the DeleteAnalysis operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteAnalysis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAnalysisAsync(DeleteAnalysisRequest deleteAnalysisRequest);
/**
*
* Deletes an analysis from Amazon QuickSight. You can optionally include a recovery window during which you can
* restore the analysis. If you don't specify a recovery window value, the operation defaults to 30 days. Amazon
* QuickSight attaches a DeletionTime
stamp to the response that specifies the end of the recovery
* window. At the end of the recovery window, Amazon QuickSight deletes the analysis permanently.
*
*
* At any time before recovery window ends, you can use the RestoreAnalysis
API operation to remove the
* DeletionTime
stamp and cancel the deletion of the analysis. The analysis remains visible in the API
* until it's deleted, so you can describe it but you can't make a template from it.
*
*
* An analysis that's scheduled for deletion isn't accessible in the Amazon QuickSight console. To access it in the
* console, restore it. Deleting an analysis doesn't delete the dashboards that you publish from it.
*
*
* @param deleteAnalysisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAnalysis operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteAnalysis
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAnalysisAsync(DeleteAnalysisRequest deleteAnalysisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dashboard.
*
*
* @param deleteDashboardRequest
* @return A Java Future containing the result of the DeleteDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDashboardAsync(DeleteDashboardRequest deleteDashboardRequest);
/**
*
* Deletes a dashboard.
*
*
* @param deleteDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDashboard
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDashboardAsync(DeleteDashboardRequest deleteDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a dataset.
*
*
* @param deleteDataSetRequest
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest);
/**
*
* Deletes a dataset.
*
*
* @param deleteDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataSetAsync(DeleteDataSetRequest deleteDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the dataset refresh properties of the dataset.
*
*
* @param deleteDataSetRefreshPropertiesRequest
* @return A Java Future containing the result of the DeleteDataSetRefreshProperties operation returned by the
* service.
* @sample AmazonQuickSightAsync.DeleteDataSetRefreshProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataSetRefreshPropertiesAsync(
DeleteDataSetRefreshPropertiesRequest deleteDataSetRefreshPropertiesRequest);
/**
*
* Deletes the dataset refresh properties of the dataset.
*
*
* @param deleteDataSetRefreshPropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSetRefreshProperties operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DeleteDataSetRefreshProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDataSetRefreshPropertiesAsync(
DeleteDataSetRefreshPropertiesRequest deleteDataSetRefreshPropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the data source permanently. This operation breaks all the datasets that reference the deleted data
* source.
*
*
* @param deleteDataSourceRequest
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest);
/**
*
* Deletes the data source permanently. This operation breaks all the datasets that reference the deleted data
* source.
*
*
* @param deleteDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDataSourceAsync(DeleteDataSourceRequest deleteDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an empty folder.
*
*
* @param deleteFolderRequest
* @return A Java Future containing the result of the DeleteFolder operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFolderAsync(DeleteFolderRequest deleteFolderRequest);
/**
*
* Deletes an empty folder.
*
*
* @param deleteFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFolder operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFolderAsync(DeleteFolderRequest deleteFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes an asset, such as a dashboard, analysis, or dataset, from a folder.
*
*
* @param deleteFolderMembershipRequest
* @return A Java Future containing the result of the DeleteFolderMembership operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteFolderMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFolderMembershipAsync(DeleteFolderMembershipRequest deleteFolderMembershipRequest);
/**
*
* Removes an asset, such as a dashboard, analysis, or dataset, from a folder.
*
*
* @param deleteFolderMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFolderMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteFolderMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFolderMembershipAsync(DeleteFolderMembershipRequest deleteFolderMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a user group from Amazon QuickSight.
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest);
/**
*
* Removes a user group from Amazon QuickSight.
*
*
* @param deleteGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a user from a group so that the user is no longer a member of the group.
*
*
* @param deleteGroupMembershipRequest
* @return A Java Future containing the result of the DeleteGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGroupMembershipAsync(DeleteGroupMembershipRequest deleteGroupMembershipRequest);
/**
*
* Removes a user from a group so that the user is no longer a member of the group.
*
*
* @param deleteGroupMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteGroupMembershipAsync(DeleteGroupMembershipRequest deleteGroupMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing IAM policy assignment.
*
*
* @param deleteIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the DeleteIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIAMPolicyAssignmentAsync(
DeleteIAMPolicyAssignmentRequest deleteIAMPolicyAssignmentRequest);
/**
*
* Deletes an existing IAM policy assignment.
*
*
* @param deleteIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIAMPolicyAssignmentAsync(
DeleteIAMPolicyAssignmentRequest deleteIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes all access scopes and authorized targets that are associated with a service from the Amazon QuickSight
* IAM Identity Center application.
*
*
* This operation is only supported for Amazon QuickSight accounts that use IAM Identity Center.
*
*
* @param deleteIdentityPropagationConfigRequest
* @return A Java Future containing the result of the DeleteIdentityPropagationConfig operation returned by the
* service.
* @sample AmazonQuickSightAsync.DeleteIdentityPropagationConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdentityPropagationConfigAsync(
DeleteIdentityPropagationConfigRequest deleteIdentityPropagationConfigRequest);
/**
*
* Deletes all access scopes and authorized targets that are associated with a service from the Amazon QuickSight
* IAM Identity Center application.
*
*
* This operation is only supported for Amazon QuickSight accounts that use IAM Identity Center.
*
*
* @param deleteIdentityPropagationConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteIdentityPropagationConfig operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DeleteIdentityPropagationConfig
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteIdentityPropagationConfigAsync(
DeleteIdentityPropagationConfigRequest deleteIdentityPropagationConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a namespace and the users and groups that are associated with the namespace. This is an asynchronous
* process. Assets including dashboards, analyses, datasets and data sources are not deleted. To delete these
* assets, you use the API operations for the relevant asset.
*
*
* @param deleteNamespaceRequest
* @return A Java Future containing the result of the DeleteNamespace operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteNamespace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteNamespaceAsync(DeleteNamespaceRequest deleteNamespaceRequest);
/**
*
* Deletes a namespace and the users and groups that are associated with the namespace. This is an asynchronous
* process. Assets including dashboards, analyses, datasets and data sources are not deleted. To delete these
* assets, you use the API operations for the relevant asset.
*
*
* @param deleteNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteNamespace operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteNamespace
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteNamespaceAsync(DeleteNamespaceRequest deleteNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a refresh schedule from a dataset.
*
*
* @param deleteRefreshScheduleRequest
* @return A Java Future containing the result of the DeleteRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRefreshScheduleAsync(DeleteRefreshScheduleRequest deleteRefreshScheduleRequest);
/**
*
* Deletes a refresh schedule from a dataset.
*
*
* @param deleteRefreshScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRefreshScheduleAsync(DeleteRefreshScheduleRequest deleteRefreshScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes custom permissions from the role.
*
*
* @param deleteRoleCustomPermissionRequest
* @return A Java Future containing the result of the DeleteRoleCustomPermission operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteRoleCustomPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRoleCustomPermissionAsync(
DeleteRoleCustomPermissionRequest deleteRoleCustomPermissionRequest);
/**
*
* Removes custom permissions from the role.
*
*
* @param deleteRoleCustomPermissionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRoleCustomPermission operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteRoleCustomPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRoleCustomPermissionAsync(
DeleteRoleCustomPermissionRequest deleteRoleCustomPermissionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a group from a role.
*
*
* @param deleteRoleMembershipRequest
* @return A Java Future containing the result of the DeleteRoleMembership operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteRoleMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRoleMembershipAsync(DeleteRoleMembershipRequest deleteRoleMembershipRequest);
/**
*
* Removes a group from a role.
*
*
* @param deleteRoleMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRoleMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteRoleMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRoleMembershipAsync(DeleteRoleMembershipRequest deleteRoleMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a template.
*
*
* @param deleteTemplateRequest
* @return A Java Future containing the result of the DeleteTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTemplateAsync(DeleteTemplateRequest deleteTemplateRequest);
/**
*
* Deletes a template.
*
*
* @param deleteTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTemplateAsync(DeleteTemplateRequest deleteTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the item that the specified template alias points to. If you provide a specific alias, you delete the
* version of the template that the alias points to.
*
*
* @param deleteTemplateAliasRequest
* @return A Java Future containing the result of the DeleteTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTemplateAliasAsync(DeleteTemplateAliasRequest deleteTemplateAliasRequest);
/**
*
* Deletes the item that the specified template alias points to. If you provide a specific alias, you delete the
* version of the template that the alias points to.
*
*
* @param deleteTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTemplateAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTemplateAliasAsync(DeleteTemplateAliasRequest deleteTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a theme.
*
*
* @param deleteThemeRequest
* @return A Java Future containing the result of the DeleteTheme operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTheme
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteThemeAsync(DeleteThemeRequest deleteThemeRequest);
/**
*
* Deletes a theme.
*
*
* @param deleteThemeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTheme operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTheme
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteThemeAsync(DeleteThemeRequest deleteThemeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the version of the theme that the specified theme alias points to. If you provide a specific alias, you
* delete the version of the theme that the alias points to.
*
*
* @param deleteThemeAliasRequest
* @return A Java Future containing the result of the DeleteThemeAlias operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteThemeAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteThemeAliasAsync(DeleteThemeAliasRequest deleteThemeAliasRequest);
/**
*
* Deletes the version of the theme that the specified theme alias points to. If you provide a specific alias, you
* delete the version of the theme that the alias points to.
*
*
* @param deleteThemeAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteThemeAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteThemeAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteThemeAliasAsync(DeleteThemeAliasRequest deleteThemeAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a topic.
*
*
* @param deleteTopicRequest
* @return A Java Future containing the result of the DeleteTopic operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTopicAsync(DeleteTopicRequest deleteTopicRequest);
/**
*
* Deletes a topic.
*
*
* @param deleteTopicRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTopic operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTopic
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTopicAsync(DeleteTopicRequest deleteTopicRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a topic refresh schedule.
*
*
* @param deleteTopicRefreshScheduleRequest
* @return A Java Future containing the result of the DeleteTopicRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteTopicRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTopicRefreshScheduleAsync(
DeleteTopicRefreshScheduleRequest deleteTopicRefreshScheduleRequest);
/**
*
* Deletes a topic refresh schedule.
*
*
* @param deleteTopicRefreshScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTopicRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteTopicRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTopicRefreshScheduleAsync(
DeleteTopicRefreshScheduleRequest deleteTopicRefreshScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the Amazon QuickSight user that is associated with the identity of the IAM user or role that's making the
* call. The IAM user isn't deleted as a result of this call.
*
*
* @param deleteUserRequest
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest);
/**
*
* Deletes the Amazon QuickSight user that is associated with the identity of the IAM user or role that's making the
* call. The IAM user isn't deleted as a result of this call.
*
*
* @param deleteUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUser operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUserAsync(DeleteUserRequest deleteUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a user identified by its principal ID.
*
*
* @param deleteUserByPrincipalIdRequest
* @return A Java Future containing the result of the DeleteUserByPrincipalId operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteUserByPrincipalId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserByPrincipalIdAsync(DeleteUserByPrincipalIdRequest deleteUserByPrincipalIdRequest);
/**
*
* Deletes a user identified by its principal ID.
*
*
* @param deleteUserByPrincipalIdRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUserByPrincipalId operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteUserByPrincipalId
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteUserByPrincipalIdAsync(DeleteUserByPrincipalIdRequest deleteUserByPrincipalIdRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a VPC connection.
*
*
* @param deleteVPCConnectionRequest
* @return A Java Future containing the result of the DeleteVPCConnection operation returned by the service.
* @sample AmazonQuickSightAsync.DeleteVPCConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVPCConnectionAsync(DeleteVPCConnectionRequest deleteVPCConnectionRequest);
/**
*
* Deletes a VPC connection.
*
*
* @param deleteVPCConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVPCConnection operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DeleteVPCConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVPCConnectionAsync(DeleteVPCConnectionRequest deleteVPCConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the customizations associated with the provided Amazon Web Services account and Amazon Amazon
* QuickSight namespace in an Amazon Web Services Region. The Amazon QuickSight console evaluates which
* customizations to apply by running this API operation with the Resolved
flag included.
*
*
* To determine what customizations display when you run this command, it can help to visualize the relationship of
* the entities involved.
*
*
* -
*
* Amazon Web Services account
- The Amazon Web Services account exists at the top of the hierarchy. It
* has the potential to use all of the Amazon Web Services Regions and Amazon Web Services Services. When you
* subscribe to Amazon QuickSight, you choose one Amazon Web Services Region to use as your home Region. That's
* where your free SPICE capacity is located. You can use Amazon QuickSight in any supported Amazon Web Services
* Region.
*
*
* -
*
* Amazon Web Services Region
- In each Amazon Web Services Region where you sign in to Amazon
* QuickSight at least once, Amazon QuickSight acts as a separate instance of the same service. If you have a user
* directory, it resides in us-east-1, which is the US East (N. Virginia). Generally speaking, these users have
* access to Amazon QuickSight in any Amazon Web Services Region, unless they are constrained to a namespace.
*
*
* To run the command in a different Amazon Web Services Region, you change your Region settings. If you're using
* the CLI, you can use one of the following options:
*
*
* -
*
* Use command line
* options.
*
*
* -
*
* Use named profiles.
*
*
* -
*
* Run aws configure
to change your default Amazon Web Services Region. Use Enter to key the same
* settings for your keys. For more information, see Configuring the CLI.
*
*
*
*
* -
*
* Namespace
- A QuickSight namespace is a partition that contains users and assets (data sources,
* datasets, dashboards, and so on). To access assets that are in a specific namespace, users and groups must also
* be part of the same namespace. People who share a namespace are completely isolated from users and assets in
* other namespaces, even if they are in the same Amazon Web Services account and Amazon Web Services Region.
*
*
* -
*
* Applied customizations
- Within an Amazon Web Services Region, a set of Amazon QuickSight
* customizations can apply to an Amazon Web Services account or to a namespace. Settings that you apply to a
* namespace override settings that you apply to an Amazon Web Services account. All settings are isolated to a
* single Amazon Web Services Region. To apply them in other Amazon Web Services Regions, run the
* CreateAccountCustomization
command in each Amazon Web Services Region where you want to apply the
* same customizations.
*
*
*
*
* @param describeAccountCustomizationRequest
* @return A Java Future containing the result of the DescribeAccountCustomization operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountCustomizationAsync(
DescribeAccountCustomizationRequest describeAccountCustomizationRequest);
/**
*
* Describes the customizations associated with the provided Amazon Web Services account and Amazon Amazon
* QuickSight namespace in an Amazon Web Services Region. The Amazon QuickSight console evaluates which
* customizations to apply by running this API operation with the Resolved
flag included.
*
*
* To determine what customizations display when you run this command, it can help to visualize the relationship of
* the entities involved.
*
*
* -
*
* Amazon Web Services account
- The Amazon Web Services account exists at the top of the hierarchy. It
* has the potential to use all of the Amazon Web Services Regions and Amazon Web Services Services. When you
* subscribe to Amazon QuickSight, you choose one Amazon Web Services Region to use as your home Region. That's
* where your free SPICE capacity is located. You can use Amazon QuickSight in any supported Amazon Web Services
* Region.
*
*
* -
*
* Amazon Web Services Region
- In each Amazon Web Services Region where you sign in to Amazon
* QuickSight at least once, Amazon QuickSight acts as a separate instance of the same service. If you have a user
* directory, it resides in us-east-1, which is the US East (N. Virginia). Generally speaking, these users have
* access to Amazon QuickSight in any Amazon Web Services Region, unless they are constrained to a namespace.
*
*
* To run the command in a different Amazon Web Services Region, you change your Region settings. If you're using
* the CLI, you can use one of the following options:
*
*
* -
*
* Use command line
* options.
*
*
* -
*
* Use named profiles.
*
*
* -
*
* Run aws configure
to change your default Amazon Web Services Region. Use Enter to key the same
* settings for your keys. For more information, see Configuring the CLI.
*
*
*
*
* -
*
* Namespace
- A QuickSight namespace is a partition that contains users and assets (data sources,
* datasets, dashboards, and so on). To access assets that are in a specific namespace, users and groups must also
* be part of the same namespace. People who share a namespace are completely isolated from users and assets in
* other namespaces, even if they are in the same Amazon Web Services account and Amazon Web Services Region.
*
*
* -
*
* Applied customizations
- Within an Amazon Web Services Region, a set of Amazon QuickSight
* customizations can apply to an Amazon Web Services account or to a namespace. Settings that you apply to a
* namespace override settings that you apply to an Amazon Web Services account. All settings are isolated to a
* single Amazon Web Services Region. To apply them in other Amazon Web Services Regions, run the
* CreateAccountCustomization
command in each Amazon Web Services Region where you want to apply the
* same customizations.
*
*
*
*
* @param describeAccountCustomizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountCustomization operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeAccountCustomization
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountCustomizationAsync(
DescribeAccountCustomizationRequest describeAccountCustomizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the settings that were used when your Amazon QuickSight subscription was first created in this Amazon
* Web Services account.
*
*
* @param describeAccountSettingsRequest
* @return A Java Future containing the result of the DescribeAccountSettings operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountSettingsAsync(DescribeAccountSettingsRequest describeAccountSettingsRequest);
/**
*
* Describes the settings that were used when your Amazon QuickSight subscription was first created in this Amazon
* Web Services account.
*
*
* @param describeAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountSettings operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountSettingsAsync(DescribeAccountSettingsRequest describeAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the DescribeAccountSubscription operation to receive a description of an Amazon QuickSight account's
* subscription. A successful API call returns an AccountInfo
object that includes an account's name,
* subscription status, authentication type, edition, and notification email address.
*
*
* @param describeAccountSubscriptionRequest
* @return A Java Future containing the result of the DescribeAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountSubscriptionAsync(
DescribeAccountSubscriptionRequest describeAccountSubscriptionRequest);
/**
*
* Use the DescribeAccountSubscription operation to receive a description of an Amazon QuickSight account's
* subscription. A successful API call returns an AccountInfo
object that includes an account's name,
* subscription status, authentication type, edition, and notification email address.
*
*
* @param describeAccountSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountSubscription operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeAccountSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountSubscriptionAsync(
DescribeAccountSubscriptionRequest describeAccountSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a summary of the metadata for an analysis.
*
*
* @param describeAnalysisRequest
* @return A Java Future containing the result of the DescribeAnalysis operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeAnalysis
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAnalysisAsync(DescribeAnalysisRequest describeAnalysisRequest);
/**
*
* Provides a summary of the metadata for an analysis.
*
*
* @param describeAnalysisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnalysis operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeAnalysis
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAnalysisAsync(DescribeAnalysisRequest describeAnalysisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a detailed description of the definition of an analysis.
*
*
*
* If you do not need to know details about the content of an Analysis, for instance if you are trying to check the
* status of a recently created or updated Analysis, use the
* DescribeAnalysis
instead.
*
*
*
* @param describeAnalysisDefinitionRequest
* @return A Java Future containing the result of the DescribeAnalysisDefinition operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeAnalysisDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnalysisDefinitionAsync(
DescribeAnalysisDefinitionRequest describeAnalysisDefinitionRequest);
/**
*
* Provides a detailed description of the definition of an analysis.
*
*
*
* If you do not need to know details about the content of an Analysis, for instance if you are trying to check the
* status of a recently created or updated Analysis, use the
* DescribeAnalysis
instead.
*
*
*
* @param describeAnalysisDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnalysisDefinition operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeAnalysisDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnalysisDefinitionAsync(
DescribeAnalysisDefinitionRequest describeAnalysisDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides the read and write permissions for an analysis.
*
*
* @param describeAnalysisPermissionsRequest
* @return A Java Future containing the result of the DescribeAnalysisPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeAnalysisPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnalysisPermissionsAsync(
DescribeAnalysisPermissionsRequest describeAnalysisPermissionsRequest);
/**
*
* Provides the read and write permissions for an analysis.
*
*
* @param describeAnalysisPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAnalysisPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeAnalysisPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAnalysisPermissionsAsync(
DescribeAnalysisPermissionsRequest describeAnalysisPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing export job.
*
*
* Poll job descriptions after a job starts to know the status of the job. When a job succeeds, a URL is provided to
* download the exported assets' data from. Download URLs are valid for five minutes after they are generated. You
* can call the DescribeAssetBundleExportJob
API for a new download URL as needed.
*
*
* Job descriptions are available for 14 days after the job starts.
*
*
* @param describeAssetBundleExportJobRequest
* @return A Java Future containing the result of the DescribeAssetBundleExportJob operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeAssetBundleExportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssetBundleExportJobAsync(
DescribeAssetBundleExportJobRequest describeAssetBundleExportJobRequest);
/**
*
* Describes an existing export job.
*
*
* Poll job descriptions after a job starts to know the status of the job. When a job succeeds, a URL is provided to
* download the exported assets' data from. Download URLs are valid for five minutes after they are generated. You
* can call the DescribeAssetBundleExportJob
API for a new download URL as needed.
*
*
* Job descriptions are available for 14 days after the job starts.
*
*
* @param describeAssetBundleExportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAssetBundleExportJob operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeAssetBundleExportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssetBundleExportJobAsync(
DescribeAssetBundleExportJobRequest describeAssetBundleExportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing import job.
*
*
* Poll job descriptions after starting a job to know when it has succeeded or failed. Job descriptions are
* available for 14 days after job starts.
*
*
* @param describeAssetBundleImportJobRequest
* @return A Java Future containing the result of the DescribeAssetBundleImportJob operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeAssetBundleImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssetBundleImportJobAsync(
DescribeAssetBundleImportJobRequest describeAssetBundleImportJobRequest);
/**
*
* Describes an existing import job.
*
*
* Poll job descriptions after starting a job to know when it has succeeded or failed. Job descriptions are
* available for 14 days after job starts.
*
*
* @param describeAssetBundleImportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAssetBundleImportJob operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeAssetBundleImportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAssetBundleImportJobAsync(
DescribeAssetBundleImportJobRequest describeAssetBundleImportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a summary for a dashboard.
*
*
* @param describeDashboardRequest
* @return A Java Future containing the result of the DescribeDashboard operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDashboard
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDashboardAsync(DescribeDashboardRequest describeDashboardRequest);
/**
*
* Provides a summary for a dashboard.
*
*
* @param describeDashboardRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboard operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboard
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDashboardAsync(DescribeDashboardRequest describeDashboardRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a detailed description of the definition of a dashboard.
*
*
*
* If you do not need to know details about the content of a dashboard, for instance if you are trying to check the
* status of a recently created or updated dashboard, use the
* DescribeDashboard
instead.
*
*
*
* @param describeDashboardDefinitionRequest
* @return A Java Future containing the result of the DescribeDashboardDefinition operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDashboardDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardDefinitionAsync(
DescribeDashboardDefinitionRequest describeDashboardDefinitionRequest);
/**
*
* Provides a detailed description of the definition of a dashboard.
*
*
*
* If you do not need to know details about the content of a dashboard, for instance if you are trying to check the
* status of a recently created or updated dashboard, use the
* DescribeDashboard
instead.
*
*
*
* @param describeDashboardDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboardDefinition operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboardDefinition
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardDefinitionAsync(
DescribeDashboardDefinitionRequest describeDashboardDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes read and write permissions for a dashboard.
*
*
* @param describeDashboardPermissionsRequest
* @return A Java Future containing the result of the DescribeDashboardPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardPermissionsAsync(
DescribeDashboardPermissionsRequest describeDashboardPermissionsRequest);
/**
*
* Describes read and write permissions for a dashboard.
*
*
* @param describeDashboardPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboardPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboardPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardPermissionsAsync(
DescribeDashboardPermissionsRequest describeDashboardPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing snapshot job.
*
*
* Poll job descriptions after a job starts to know the status of the job. For information on available status
* codes, see JobStatus
.
*
*
* @param describeDashboardSnapshotJobRequest
* @return A Java Future containing the result of the DescribeDashboardSnapshotJob operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDashboardSnapshotJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardSnapshotJobAsync(
DescribeDashboardSnapshotJobRequest describeDashboardSnapshotJobRequest);
/**
*
* Describes an existing snapshot job.
*
*
* Poll job descriptions after a job starts to know the status of the job. For information on available status
* codes, see JobStatus
.
*
*
* @param describeDashboardSnapshotJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboardSnapshotJob operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboardSnapshotJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardSnapshotJobAsync(
DescribeDashboardSnapshotJobRequest describeDashboardSnapshotJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the result of an existing snapshot job that has finished running.
*
*
* A finished snapshot job will return a COMPLETED
or FAILED
status when you poll the job
* with a DescribeDashboardSnapshotJob
API call.
*
*
* If the job has not finished running, this operation returns a message that says
* Dashboard Snapshot Job with id <SnapshotjobId> has not reached a terminal state.
.
*
*
* @param describeDashboardSnapshotJobResultRequest
* @return A Java Future containing the result of the DescribeDashboardSnapshotJobResult operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDashboardSnapshotJobResult
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardSnapshotJobResultAsync(
DescribeDashboardSnapshotJobResultRequest describeDashboardSnapshotJobResultRequest);
/**
*
* Describes the result of an existing snapshot job that has finished running.
*
*
* A finished snapshot job will return a COMPLETED
or FAILED
status when you poll the job
* with a DescribeDashboardSnapshotJob
API call.
*
*
* If the job has not finished running, this operation returns a message that says
* Dashboard Snapshot Job with id <SnapshotjobId> has not reached a terminal state.
.
*
*
* @param describeDashboardSnapshotJobResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDashboardSnapshotJobResult operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDashboardSnapshotJobResult
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDashboardSnapshotJobResultAsync(
DescribeDashboardSnapshotJobResultRequest describeDashboardSnapshotJobResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param describeDataSetRequest
* @return A Java Future containing the result of the DescribeDataSet operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSetAsync(DescribeDataSetRequest describeDataSetRequest);
/**
*
* Describes a dataset. This operation doesn't support datasets that include uploaded files as a source.
*
*
* @param describeDataSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSet operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataSetAsync(DescribeDataSetRequest describeDataSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param describeDataSetPermissionsRequest
* @return A Java Future containing the result of the DescribeDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetPermissionsAsync(
DescribeDataSetPermissionsRequest describeDataSetPermissionsRequest);
/**
*
* Describes the permissions on a dataset.
*
*
* The permissions resource is arn:aws:quicksight:region:aws-account-id:dataset/data-set-id
.
*
*
* @param describeDataSetPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSetPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSetPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetPermissionsAsync(
DescribeDataSetPermissionsRequest describeDataSetPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the refresh properties of a dataset.
*
*
* @param describeDataSetRefreshPropertiesRequest
* @return A Java Future containing the result of the DescribeDataSetRefreshProperties operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDataSetRefreshProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetRefreshPropertiesAsync(
DescribeDataSetRefreshPropertiesRequest describeDataSetRefreshPropertiesRequest);
/**
*
* Describes the refresh properties of a dataset.
*
*
* @param describeDataSetRefreshPropertiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSetRefreshProperties operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSetRefreshProperties
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSetRefreshPropertiesAsync(
DescribeDataSetRefreshPropertiesRequest describeDataSetRefreshPropertiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a data source.
*
*
* @param describeDataSourceRequest
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest);
/**
*
* Describes a data source.
*
*
* @param describeDataSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSource operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSourceAsync(DescribeDataSourceRequest describeDataSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the resource permissions for a data source.
*
*
* @param describeDataSourcePermissionsRequest
* @return A Java Future containing the result of the DescribeDataSourcePermissions operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSourcePermissionsAsync(
DescribeDataSourcePermissionsRequest describeDataSourcePermissionsRequest);
/**
*
* Describes the resource permissions for a data source.
*
*
* @param describeDataSourcePermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSourcePermissions operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeDataSourcePermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSourcePermissionsAsync(
DescribeDataSourcePermissionsRequest describeDataSourcePermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a folder.
*
*
* @param describeFolderRequest
* @return A Java Future containing the result of the DescribeFolder operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFolderAsync(DescribeFolderRequest describeFolderRequest);
/**
*
* Describes a folder.
*
*
* @param describeFolderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFolder operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeFolder
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFolderAsync(DescribeFolderRequest describeFolderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes permissions for a folder.
*
*
* @param describeFolderPermissionsRequest
* @return A Java Future containing the result of the DescribeFolderPermissions operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeFolderPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFolderPermissionsAsync(
DescribeFolderPermissionsRequest describeFolderPermissionsRequest);
/**
*
* Describes permissions for a folder.
*
*
* @param describeFolderPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFolderPermissions operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeFolderPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFolderPermissionsAsync(
DescribeFolderPermissionsRequest describeFolderPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the folder resolved permissions. Permissions consists of both folder direct permissions and the
* inherited permissions from the ancestor folders.
*
*
* @param describeFolderResolvedPermissionsRequest
* @return A Java Future containing the result of the DescribeFolderResolvedPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeFolderResolvedPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFolderResolvedPermissionsAsync(
DescribeFolderResolvedPermissionsRequest describeFolderResolvedPermissionsRequest);
/**
*
* Describes the folder resolved permissions. Permissions consists of both folder direct permissions and the
* inherited permissions from the ancestor folders.
*
*
* @param describeFolderResolvedPermissionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFolderResolvedPermissions operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeFolderResolvedPermissions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFolderResolvedPermissionsAsync(
DescribeFolderResolvedPermissionsRequest describeFolderResolvedPermissionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an Amazon QuickSight group's description and Amazon Resource Name (ARN).
*
*
* @param describeGroupRequest
* @return A Java Future containing the result of the DescribeGroup operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeGroupAsync(DescribeGroupRequest describeGroupRequest);
/**
*
* Returns an Amazon QuickSight group's description and Amazon Resource Name (ARN).
*
*
* @param describeGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGroup operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeGroupAsync(DescribeGroupRequest describeGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Use the DescribeGroupMembership
operation to determine if a user is a member of the specified group.
* If the user exists and is a member of the specified group, an associated GroupMember
object is
* returned.
*
*
* @param describeGroupMembershipRequest
* @return A Java Future containing the result of the DescribeGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGroupMembershipAsync(DescribeGroupMembershipRequest describeGroupMembershipRequest);
/**
*
* Use the DescribeGroupMembership
operation to determine if a user is a member of the specified group.
* If the user exists and is a member of the specified group, an associated GroupMember
object is
* returned.
*
*
* @param describeGroupMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGroupMembership operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeGroupMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGroupMembershipAsync(DescribeGroupMembershipRequest describeGroupMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an existing IAM policy assignment, as specified by the assignment name.
*
*
* @param describeIAMPolicyAssignmentRequest
* @return A Java Future containing the result of the DescribeIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIAMPolicyAssignmentAsync(
DescribeIAMPolicyAssignmentRequest describeIAMPolicyAssignmentRequest);
/**
*
* Describes an existing IAM policy assignment, as specified by the assignment name.
*
*
* @param describeIAMPolicyAssignmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIAMPolicyAssignment operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeIAMPolicyAssignment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIAMPolicyAssignmentAsync(
DescribeIAMPolicyAssignmentRequest describeIAMPolicyAssignmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a SPICE ingestion.
*
*
* @param describeIngestionRequest
* @return A Java Future containing the result of the DescribeIngestion operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeIngestion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIngestionAsync(DescribeIngestionRequest describeIngestionRequest);
/**
*
* Describes a SPICE ingestion.
*
*
* @param describeIngestionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIngestion operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeIngestion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeIngestionAsync(DescribeIngestionRequest describeIngestionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a summary and status of IP rules.
*
*
* @param describeIpRestrictionRequest
* @return A Java Future containing the result of the DescribeIpRestriction operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeIpRestriction
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIpRestrictionAsync(DescribeIpRestrictionRequest describeIpRestrictionRequest);
/**
*
* Provides a summary and status of IP rules.
*
*
* @param describeIpRestrictionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeIpRestriction operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeIpRestriction
* @see AWS API Documentation
*/
java.util.concurrent.Future describeIpRestrictionAsync(DescribeIpRestrictionRequest describeIpRestrictionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes all customer managed key registrations in a Amazon QuickSight account.
*
*
* @param describeKeyRegistrationRequest
* @return A Java Future containing the result of the DescribeKeyRegistration operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeKeyRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeyRegistrationAsync(DescribeKeyRegistrationRequest describeKeyRegistrationRequest);
/**
*
* Describes all customer managed key registrations in a Amazon QuickSight account.
*
*
* @param describeKeyRegistrationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeKeyRegistration operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeKeyRegistration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeKeyRegistrationAsync(DescribeKeyRegistrationRequest describeKeyRegistrationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the current namespace.
*
*
* @param describeNamespaceRequest
* @return A Java Future containing the result of the DescribeNamespace operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeNamespace
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeNamespaceAsync(DescribeNamespaceRequest describeNamespaceRequest);
/**
*
* Describes the current namespace.
*
*
* @param describeNamespaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeNamespace operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeNamespace
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeNamespaceAsync(DescribeNamespaceRequest describeNamespaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a summary of a refresh schedule.
*
*
* @param describeRefreshScheduleRequest
* @return A Java Future containing the result of the DescribeRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshScheduleAsync(DescribeRefreshScheduleRequest describeRefreshScheduleRequest);
/**
*
* Provides a summary of a refresh schedule.
*
*
* @param describeRefreshScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRefreshSchedule operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeRefreshSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshScheduleAsync(DescribeRefreshScheduleRequest describeRefreshScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes all custom permissions that are mapped to a role.
*
*
* @param describeRoleCustomPermissionRequest
* @return A Java Future containing the result of the DescribeRoleCustomPermission operation returned by the
* service.
* @sample AmazonQuickSightAsync.DescribeRoleCustomPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRoleCustomPermissionAsync(
DescribeRoleCustomPermissionRequest describeRoleCustomPermissionRequest);
/**
*
* Describes all custom permissions that are mapped to a role.
*
*
* @param describeRoleCustomPermissionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRoleCustomPermission operation returned by the
* service.
* @sample AmazonQuickSightAsyncHandler.DescribeRoleCustomPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRoleCustomPermissionAsync(
DescribeRoleCustomPermissionRequest describeRoleCustomPermissionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a template's metadata.
*
*
* @param describeTemplateRequest
* @return A Java Future containing the result of the DescribeTemplate operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTemplateAsync(DescribeTemplateRequest describeTemplateRequest);
/**
*
* Describes a template's metadata.
*
*
* @param describeTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTemplate operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTemplateAsync(DescribeTemplateRequest describeTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the template alias for a template.
*
*
* @param describeTemplateAliasRequest
* @return A Java Future containing the result of the DescribeTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsync.DescribeTemplateAlias
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplateAliasAsync(DescribeTemplateAliasRequest describeTemplateAliasRequest);
/**
*
* Describes the template alias for a template.
*
*
* @param describeTemplateAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTemplateAlias operation returned by the service.
* @sample AmazonQuickSightAsyncHandler.DescribeTemplateAlias
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTemplateAliasAsync(DescribeTemplateAliasRequest describeTemplateAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a detailed description of the definition of a template.
*
*
*