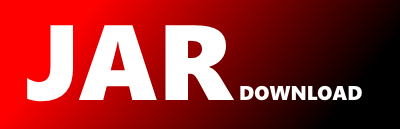
com.amazonaws.services.quicksight.model.CreateAnalysisRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAnalysisRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the Amazon Web Services account where you are creating an analysis.
*
*/
private String awsAccountId;
/**
*
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*
*/
private String analysisId;
/**
*
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the Amazon
* QuickSight console.
*
*/
private String name;
/**
*
* The parameter names and override values that you want to use. An analysis can have any parameter type, and some
* parameters might accept multiple values.
*
*/
private Parameters parameters;
/**
*
* A structure that describes the principals and the resource-level permissions on an analysis. You can use the
* Permissions
structure to grant permissions by providing a list of Identity and Access Management
* (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
*
* To specify no permissions, omit Permissions
.
*
*/
private java.util.List permissions;
/**
*
* A source entity to use for the analysis that you're creating. This metadata structure contains details that
* describe a source template and one or more datasets.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*/
private AnalysisSourceEntity sourceEntity;
/**
*
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon QuickSight
* console, make sure that you have access to it.
*
*/
private String themeArn;
/**
*
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*
*/
private java.util.List tags;
/**
*
* The definition of an analysis.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*/
private AnalysisDefinition definition;
/**
*
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*
*/
private ValidationStrategy validationStrategy;
/**
*
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*
*/
private java.util.List folderArns;
/**
*
* The ID of the Amazon Web Services account where you are creating an analysis.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account where you are creating an analysis.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account where you are creating an analysis.
*
*
* @return The ID of the Amazon Web Services account where you are creating an analysis.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account where you are creating an analysis.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account where you are creating an analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*
*
* @param analysisId
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*/
public void setAnalysisId(String analysisId) {
this.analysisId = analysisId;
}
/**
*
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*
*
* @return The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*/
public String getAnalysisId() {
return this.analysisId;
}
/**
*
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
*
*
* @param analysisId
* The ID for the analysis that you're creating. This ID displays in the URL of the analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withAnalysisId(String analysisId) {
setAnalysisId(analysisId);
return this;
}
/**
*
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the Amazon
* QuickSight console.
*
*
* @param name
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the
* Amazon QuickSight console.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the Amazon
* QuickSight console.
*
*
* @return A descriptive name for the analysis that you're creating. This name displays for the analysis in the
* Amazon QuickSight console.
*/
public String getName() {
return this.name;
}
/**
*
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the Amazon
* QuickSight console.
*
*
* @param name
* A descriptive name for the analysis that you're creating. This name displays for the analysis in the
* Amazon QuickSight console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The parameter names and override values that you want to use. An analysis can have any parameter type, and some
* parameters might accept multiple values.
*
*
* @param parameters
* The parameter names and override values that you want to use. An analysis can have any parameter type, and
* some parameters might accept multiple values.
*/
public void setParameters(Parameters parameters) {
this.parameters = parameters;
}
/**
*
* The parameter names and override values that you want to use. An analysis can have any parameter type, and some
* parameters might accept multiple values.
*
*
* @return The parameter names and override values that you want to use. An analysis can have any parameter type,
* and some parameters might accept multiple values.
*/
public Parameters getParameters() {
return this.parameters;
}
/**
*
* The parameter names and override values that you want to use. An analysis can have any parameter type, and some
* parameters might accept multiple values.
*
*
* @param parameters
* The parameter names and override values that you want to use. An analysis can have any parameter type, and
* some parameters might accept multiple values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withParameters(Parameters parameters) {
setParameters(parameters);
return this;
}
/**
*
* A structure that describes the principals and the resource-level permissions on an analysis. You can use the
* Permissions
structure to grant permissions by providing a list of Identity and Access Management
* (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
*
* To specify no permissions, omit Permissions
.
*
*
* @return A structure that describes the principals and the resource-level permissions on an analysis. You can use
* the Permissions
structure to grant permissions by providing a list of Identity and Access
* Management (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
* To specify no permissions, omit Permissions
.
*/
public java.util.List getPermissions() {
return permissions;
}
/**
*
* A structure that describes the principals and the resource-level permissions on an analysis. You can use the
* Permissions
structure to grant permissions by providing a list of Identity and Access Management
* (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
*
* To specify no permissions, omit Permissions
.
*
*
* @param permissions
* A structure that describes the principals and the resource-level permissions on an analysis. You can use
* the Permissions
structure to grant permissions by providing a list of Identity and Access
* Management (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
* To specify no permissions, omit Permissions
.
*/
public void setPermissions(java.util.Collection permissions) {
if (permissions == null) {
this.permissions = null;
return;
}
this.permissions = new java.util.ArrayList(permissions);
}
/**
*
* A structure that describes the principals and the resource-level permissions on an analysis. You can use the
* Permissions
structure to grant permissions by providing a list of Identity and Access Management
* (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
*
* To specify no permissions, omit Permissions
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPermissions(java.util.Collection)} or {@link #withPermissions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param permissions
* A structure that describes the principals and the resource-level permissions on an analysis. You can use
* the Permissions
structure to grant permissions by providing a list of Identity and Access
* Management (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
* To specify no permissions, omit Permissions
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withPermissions(ResourcePermission... permissions) {
if (this.permissions == null) {
setPermissions(new java.util.ArrayList(permissions.length));
}
for (ResourcePermission ele : permissions) {
this.permissions.add(ele);
}
return this;
}
/**
*
* A structure that describes the principals and the resource-level permissions on an analysis. You can use the
* Permissions
structure to grant permissions by providing a list of Identity and Access Management
* (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
*
* To specify no permissions, omit Permissions
.
*
*
* @param permissions
* A structure that describes the principals and the resource-level permissions on an analysis. You can use
* the Permissions
structure to grant permissions by providing a list of Identity and Access
* Management (IAM) action information for each principal listed by Amazon Resource Name (ARN).
*
* To specify no permissions, omit Permissions
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withPermissions(java.util.Collection permissions) {
setPermissions(permissions);
return this;
}
/**
*
* A source entity to use for the analysis that you're creating. This metadata structure contains details that
* describe a source template and one or more datasets.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @param sourceEntity
* A source entity to use for the analysis that you're creating. This metadata structure contains details
* that describe a source template and one or more datasets.
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
*/
public void setSourceEntity(AnalysisSourceEntity sourceEntity) {
this.sourceEntity = sourceEntity;
}
/**
*
* A source entity to use for the analysis that you're creating. This metadata structure contains details that
* describe a source template and one or more datasets.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @return A source entity to use for the analysis that you're creating. This metadata structure contains details
* that describe a source template and one or more datasets.
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
*/
public AnalysisSourceEntity getSourceEntity() {
return this.sourceEntity;
}
/**
*
* A source entity to use for the analysis that you're creating. This metadata structure contains details that
* describe a source template and one or more datasets.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @param sourceEntity
* A source entity to use for the analysis that you're creating. This metadata structure contains details
* that describe a source template and one or more datasets.
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withSourceEntity(AnalysisSourceEntity sourceEntity) {
setSourceEntity(sourceEntity);
return this;
}
/**
*
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon QuickSight
* console, make sure that you have access to it.
*
*
* @param themeArn
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon
* QuickSight console, make sure that you have access to it.
*/
public void setThemeArn(String themeArn) {
this.themeArn = themeArn;
}
/**
*
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon QuickSight
* console, make sure that you have access to it.
*
*
* @return The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon
* QuickSight console, make sure that you have access to it.
*/
public String getThemeArn() {
return this.themeArn;
}
/**
*
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon QuickSight
* console, make sure that you have access to it.
*
*
* @param themeArn
* The ARN for the theme to apply to the analysis that you're creating. To see the theme in the Amazon
* QuickSight console, make sure that you have access to it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withThemeArn(String themeArn) {
setThemeArn(themeArn);
return this;
}
/**
*
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*
*
* @return Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*
*
* @param tags
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
*
*
* @param tags
* Contains a map of the key-value pairs for the resource tag or tags assigned to the analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The definition of an analysis.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @param definition
* The definition of an analysis.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
*/
public void setDefinition(AnalysisDefinition definition) {
this.definition = definition;
}
/**
*
* The definition of an analysis.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @return The definition of an analysis.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
*/
public AnalysisDefinition getDefinition() {
return this.definition;
}
/**
*
* The definition of an analysis.
*
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request to be
* valid.
*
*
* @param definition
* The definition of an analysis.
*
* A definition is the data model of all features in a Dashboard, Template, or Analysis.
*
*
* Either a SourceEntity
or a Definition
must be provided in order for the request
* to be valid.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withDefinition(AnalysisDefinition definition) {
setDefinition(definition);
return this;
}
/**
*
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*
*
* @param validationStrategy
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*/
public void setValidationStrategy(ValidationStrategy validationStrategy) {
this.validationStrategy = validationStrategy;
}
/**
*
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*
*
* @return The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*/
public ValidationStrategy getValidationStrategy() {
return this.validationStrategy;
}
/**
*
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
*
*
* @param validationStrategy
* The option to relax the validation needed to create an analysis with definition objects. This skips the
* validation step for specific errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withValidationStrategy(ValidationStrategy validationStrategy) {
setValidationStrategy(validationStrategy);
return this;
}
/**
*
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*
*
* @return When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*/
public java.util.List getFolderArns() {
return folderArns;
}
/**
*
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*
*
* @param folderArns
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*/
public void setFolderArns(java.util.Collection folderArns) {
if (folderArns == null) {
this.folderArns = null;
return;
}
this.folderArns = new java.util.ArrayList(folderArns);
}
/**
*
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFolderArns(java.util.Collection)} or {@link #withFolderArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param folderArns
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withFolderArns(String... folderArns) {
if (this.folderArns == null) {
setFolderArns(new java.util.ArrayList(folderArns.length));
}
for (String ele : folderArns) {
this.folderArns.add(ele);
}
return this;
}
/**
*
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
*
*
* @param folderArns
* When you create the analysis, Amazon QuickSight adds the analysis to these folders.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAnalysisRequest withFolderArns(java.util.Collection folderArns) {
setFolderArns(folderArns);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getAnalysisId() != null)
sb.append("AnalysisId: ").append(getAnalysisId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getPermissions() != null)
sb.append("Permissions: ").append(getPermissions()).append(",");
if (getSourceEntity() != null)
sb.append("SourceEntity: ").append(getSourceEntity()).append(",");
if (getThemeArn() != null)
sb.append("ThemeArn: ").append(getThemeArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getDefinition() != null)
sb.append("Definition: ").append(getDefinition()).append(",");
if (getValidationStrategy() != null)
sb.append("ValidationStrategy: ").append(getValidationStrategy()).append(",");
if (getFolderArns() != null)
sb.append("FolderArns: ").append(getFolderArns());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAnalysisRequest == false)
return false;
CreateAnalysisRequest other = (CreateAnalysisRequest) obj;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getAnalysisId() == null ^ this.getAnalysisId() == null)
return false;
if (other.getAnalysisId() != null && other.getAnalysisId().equals(this.getAnalysisId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getPermissions() == null ^ this.getPermissions() == null)
return false;
if (other.getPermissions() != null && other.getPermissions().equals(this.getPermissions()) == false)
return false;
if (other.getSourceEntity() == null ^ this.getSourceEntity() == null)
return false;
if (other.getSourceEntity() != null && other.getSourceEntity().equals(this.getSourceEntity()) == false)
return false;
if (other.getThemeArn() == null ^ this.getThemeArn() == null)
return false;
if (other.getThemeArn() != null && other.getThemeArn().equals(this.getThemeArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getDefinition() == null ^ this.getDefinition() == null)
return false;
if (other.getDefinition() != null && other.getDefinition().equals(this.getDefinition()) == false)
return false;
if (other.getValidationStrategy() == null ^ this.getValidationStrategy() == null)
return false;
if (other.getValidationStrategy() != null && other.getValidationStrategy().equals(this.getValidationStrategy()) == false)
return false;
if (other.getFolderArns() == null ^ this.getFolderArns() == null)
return false;
if (other.getFolderArns() != null && other.getFolderArns().equals(this.getFolderArns()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getAnalysisId() == null) ? 0 : getAnalysisId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getPermissions() == null) ? 0 : getPermissions().hashCode());
hashCode = prime * hashCode + ((getSourceEntity() == null) ? 0 : getSourceEntity().hashCode());
hashCode = prime * hashCode + ((getThemeArn() == null) ? 0 : getThemeArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getDefinition() == null) ? 0 : getDefinition().hashCode());
hashCode = prime * hashCode + ((getValidationStrategy() == null) ? 0 : getValidationStrategy().hashCode());
hashCode = prime * hashCode + ((getFolderArns() == null) ? 0 : getFolderArns().hashCode());
return hashCode;
}
@Override
public CreateAnalysisRequest clone() {
return (CreateAnalysisRequest) super.clone();
}
}