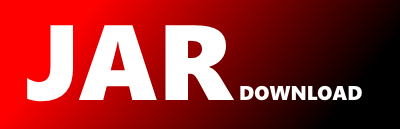
com.amazonaws.services.quicksight.model.DescribeAssetBundleImportJobResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeAssetBundleImportJobResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Indicates the status of a job through its queuing and execution.
*
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the following
* values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
*
*/
private String jobStatus;
/**
*
* An array of error records that describes any failures that occurred during the export job processing.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*/
private java.util.List errors;
/**
*
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*/
private java.util.List rollbackErrors;
/**
*
* The Amazon Resource Name (ARN) for the import job.
*
*/
private String arn;
/**
*
* The time that the import job was created.
*
*/
private java.util.Date createdTime;
/**
*
* The ID of the job. The job ID is set when you start a new job with a StartAssetBundleImportJob
API
* call.
*
*/
private String assetBundleImportJobId;
/**
*
* The ID of the Amazon Web Services account the import job was executed in.
*
*/
private String awsAccountId;
/**
*
* The source of the asset bundle zip file that contains the data that is imported by the job.
*
*/
private AssetBundleImportSourceDescription assetBundleImportSource;
/**
*
* Optional overrides that are applied to the resource configuration before import.
*
*/
private AssetBundleImportJobOverrideParameters overrideParameters;
/**
*
* The failure action for the import job.
*
*/
private String failureAction;
/**
*
* The Amazon Web Services request ID for this operation.
*
*/
private String requestId;
/**
*
* The HTTP status of the response.
*
*/
private Integer status;
/**
*
* Optional permission overrides that are applied to the resource configuration before import.
*
*/
private AssetBundleImportJobOverridePermissions overridePermissions;
/**
*
* Optional tag overrides that are applied to the resource configuration before import.
*
*/
private AssetBundleImportJobOverrideTags overrideTags;
/**
*
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*
*/
private AssetBundleImportJobOverrideValidationStrategy overrideValidationStrategy;
/**
*
* An array of warning records that describe all permitted errors that are encountered during the import job.
*
*/
private java.util.List warnings;
/**
*
* Indicates the status of a job through its queuing and execution.
*
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the following
* values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
*
*
* @param jobStatus
* Indicates the status of a job through its queuing and execution.
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the
* following values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
* @see AssetBundleImportJobStatus
*/
public void setJobStatus(String jobStatus) {
this.jobStatus = jobStatus;
}
/**
*
* Indicates the status of a job through its queuing and execution.
*
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the following
* values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
*
*
* @return Indicates the status of a job through its queuing and execution.
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the
* following values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
* @see AssetBundleImportJobStatus
*/
public String getJobStatus() {
return this.jobStatus;
}
/**
*
* Indicates the status of a job through its queuing and execution.
*
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the following
* values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
*
*
* @param jobStatus
* Indicates the status of a job through its queuing and execution.
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the
* following values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleImportJobStatus
*/
public DescribeAssetBundleImportJobResult withJobStatus(String jobStatus) {
setJobStatus(jobStatus);
return this;
}
/**
*
* Indicates the status of a job through its queuing and execution.
*
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the following
* values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
*
*
* @param jobStatus
* Indicates the status of a job through its queuing and execution.
*
* Poll the DescribeAssetBundleImport
API until JobStatus
returns one of the
* following values:
*
*
* -
*
* SUCCESSFUL
*
*
* -
*
* FAILED
*
*
* -
*
* FAILED_ROLLBACK_COMPLETED
*
*
* -
*
* FAILED_ROLLBACK_ERROR
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleImportJobStatus
*/
public DescribeAssetBundleImportJobResult withJobStatus(AssetBundleImportJobStatus jobStatus) {
this.jobStatus = jobStatus.toString();
return this;
}
/**
*
* An array of error records that describes any failures that occurred during the export job processing.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @return An array of error records that describes any failures that occurred during the export job processing.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
*/
public java.util.List getErrors() {
return errors;
}
/**
*
* An array of error records that describes any failures that occurred during the export job processing.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @param errors
* An array of error records that describes any failures that occurred during the export job processing.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
*/
public void setErrors(java.util.Collection errors) {
if (errors == null) {
this.errors = null;
return;
}
this.errors = new java.util.ArrayList(errors);
}
/**
*
* An array of error records that describes any failures that occurred during the export job processing.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setErrors(java.util.Collection)} or {@link #withErrors(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param errors
* An array of error records that describes any failures that occurred during the export job processing.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withErrors(AssetBundleImportJobError... errors) {
if (this.errors == null) {
setErrors(new java.util.ArrayList(errors.length));
}
for (AssetBundleImportJobError ele : errors) {
this.errors.add(ele);
}
return this;
}
/**
*
* An array of error records that describes any failures that occurred during the export job processing.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @param errors
* An array of error records that describes any failures that occurred during the export job processing.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withErrors(java.util.Collection errors) {
setErrors(errors);
return this;
}
/**
*
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @return An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
*/
public java.util.List getRollbackErrors() {
return rollbackErrors;
}
/**
*
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @param rollbackErrors
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
*/
public void setRollbackErrors(java.util.Collection rollbackErrors) {
if (rollbackErrors == null) {
this.rollbackErrors = null;
return;
}
this.rollbackErrors = new java.util.ArrayList(rollbackErrors);
}
/**
*
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRollbackErrors(java.util.Collection)} or {@link #withRollbackErrors(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param rollbackErrors
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withRollbackErrors(AssetBundleImportJobError... rollbackErrors) {
if (this.rollbackErrors == null) {
setRollbackErrors(new java.util.ArrayList(rollbackErrors.length));
}
for (AssetBundleImportJobError ele : rollbackErrors) {
this.rollbackErrors.add(ele);
}
return this;
}
/**
*
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
*
* Error records accumulate while the job is still running. The complete set of error records is available after the
* job has completed and failed.
*
*
* @param rollbackErrors
* An array of error records that describes any failures that occurred while an import job was attempting a
* rollback.
*
* Error records accumulate while the job is still running. The complete set of error records is available
* after the job has completed and failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withRollbackErrors(java.util.Collection rollbackErrors) {
setRollbackErrors(rollbackErrors);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the import job.
*
*
* @param arn
* The Amazon Resource Name (ARN) for the import job.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) for the import job.
*
*
* @return The Amazon Resource Name (ARN) for the import job.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) for the import job.
*
*
* @param arn
* The Amazon Resource Name (ARN) for the import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The time that the import job was created.
*
*
* @param createdTime
* The time that the import job was created.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time that the import job was created.
*
*
* @return The time that the import job was created.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time that the import job was created.
*
*
* @param createdTime
* The time that the import job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The ID of the job. The job ID is set when you start a new job with a StartAssetBundleImportJob
API
* call.
*
*
* @param assetBundleImportJobId
* The ID of the job. The job ID is set when you start a new job with a
* StartAssetBundleImportJob
API call.
*/
public void setAssetBundleImportJobId(String assetBundleImportJobId) {
this.assetBundleImportJobId = assetBundleImportJobId;
}
/**
*
* The ID of the job. The job ID is set when you start a new job with a StartAssetBundleImportJob
API
* call.
*
*
* @return The ID of the job. The job ID is set when you start a new job with a
* StartAssetBundleImportJob
API call.
*/
public String getAssetBundleImportJobId() {
return this.assetBundleImportJobId;
}
/**
*
* The ID of the job. The job ID is set when you start a new job with a StartAssetBundleImportJob
API
* call.
*
*
* @param assetBundleImportJobId
* The ID of the job. The job ID is set when you start a new job with a
* StartAssetBundleImportJob
API call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withAssetBundleImportJobId(String assetBundleImportJobId) {
setAssetBundleImportJobId(assetBundleImportJobId);
return this;
}
/**
*
* The ID of the Amazon Web Services account the import job was executed in.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account the import job was executed in.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account the import job was executed in.
*
*
* @return The ID of the Amazon Web Services account the import job was executed in.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account the import job was executed in.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account the import job was executed in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The source of the asset bundle zip file that contains the data that is imported by the job.
*
*
* @param assetBundleImportSource
* The source of the asset bundle zip file that contains the data that is imported by the job.
*/
public void setAssetBundleImportSource(AssetBundleImportSourceDescription assetBundleImportSource) {
this.assetBundleImportSource = assetBundleImportSource;
}
/**
*
* The source of the asset bundle zip file that contains the data that is imported by the job.
*
*
* @return The source of the asset bundle zip file that contains the data that is imported by the job.
*/
public AssetBundleImportSourceDescription getAssetBundleImportSource() {
return this.assetBundleImportSource;
}
/**
*
* The source of the asset bundle zip file that contains the data that is imported by the job.
*
*
* @param assetBundleImportSource
* The source of the asset bundle zip file that contains the data that is imported by the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withAssetBundleImportSource(AssetBundleImportSourceDescription assetBundleImportSource) {
setAssetBundleImportSource(assetBundleImportSource);
return this;
}
/**
*
* Optional overrides that are applied to the resource configuration before import.
*
*
* @param overrideParameters
* Optional overrides that are applied to the resource configuration before import.
*/
public void setOverrideParameters(AssetBundleImportJobOverrideParameters overrideParameters) {
this.overrideParameters = overrideParameters;
}
/**
*
* Optional overrides that are applied to the resource configuration before import.
*
*
* @return Optional overrides that are applied to the resource configuration before import.
*/
public AssetBundleImportJobOverrideParameters getOverrideParameters() {
return this.overrideParameters;
}
/**
*
* Optional overrides that are applied to the resource configuration before import.
*
*
* @param overrideParameters
* Optional overrides that are applied to the resource configuration before import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withOverrideParameters(AssetBundleImportJobOverrideParameters overrideParameters) {
setOverrideParameters(overrideParameters);
return this;
}
/**
*
* The failure action for the import job.
*
*
* @param failureAction
* The failure action for the import job.
* @see AssetBundleImportFailureAction
*/
public void setFailureAction(String failureAction) {
this.failureAction = failureAction;
}
/**
*
* The failure action for the import job.
*
*
* @return The failure action for the import job.
* @see AssetBundleImportFailureAction
*/
public String getFailureAction() {
return this.failureAction;
}
/**
*
* The failure action for the import job.
*
*
* @param failureAction
* The failure action for the import job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleImportFailureAction
*/
public DescribeAssetBundleImportJobResult withFailureAction(String failureAction) {
setFailureAction(failureAction);
return this;
}
/**
*
* The failure action for the import job.
*
*
* @param failureAction
* The failure action for the import job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleImportFailureAction
*/
public DescribeAssetBundleImportJobResult withFailureAction(AssetBundleImportFailureAction failureAction) {
this.failureAction = failureAction.toString();
return this;
}
/**
*
* The Amazon Web Services request ID for this operation.
*
*
* @param requestId
* The Amazon Web Services request ID for this operation.
*/
public void setRequestId(String requestId) {
this.requestId = requestId;
}
/**
*
* The Amazon Web Services request ID for this operation.
*
*
* @return The Amazon Web Services request ID for this operation.
*/
public String getRequestId() {
return this.requestId;
}
/**
*
* The Amazon Web Services request ID for this operation.
*
*
* @param requestId
* The Amazon Web Services request ID for this operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withRequestId(String requestId) {
setRequestId(requestId);
return this;
}
/**
*
* The HTTP status of the response.
*
*
* @param status
* The HTTP status of the response.
*/
public void setStatus(Integer status) {
this.status = status;
}
/**
*
* The HTTP status of the response.
*
*
* @return The HTTP status of the response.
*/
public Integer getStatus() {
return this.status;
}
/**
*
* The HTTP status of the response.
*
*
* @param status
* The HTTP status of the response.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withStatus(Integer status) {
setStatus(status);
return this;
}
/**
*
* Optional permission overrides that are applied to the resource configuration before import.
*
*
* @param overridePermissions
* Optional permission overrides that are applied to the resource configuration before import.
*/
public void setOverridePermissions(AssetBundleImportJobOverridePermissions overridePermissions) {
this.overridePermissions = overridePermissions;
}
/**
*
* Optional permission overrides that are applied to the resource configuration before import.
*
*
* @return Optional permission overrides that are applied to the resource configuration before import.
*/
public AssetBundleImportJobOverridePermissions getOverridePermissions() {
return this.overridePermissions;
}
/**
*
* Optional permission overrides that are applied to the resource configuration before import.
*
*
* @param overridePermissions
* Optional permission overrides that are applied to the resource configuration before import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withOverridePermissions(AssetBundleImportJobOverridePermissions overridePermissions) {
setOverridePermissions(overridePermissions);
return this;
}
/**
*
* Optional tag overrides that are applied to the resource configuration before import.
*
*
* @param overrideTags
* Optional tag overrides that are applied to the resource configuration before import.
*/
public void setOverrideTags(AssetBundleImportJobOverrideTags overrideTags) {
this.overrideTags = overrideTags;
}
/**
*
* Optional tag overrides that are applied to the resource configuration before import.
*
*
* @return Optional tag overrides that are applied to the resource configuration before import.
*/
public AssetBundleImportJobOverrideTags getOverrideTags() {
return this.overrideTags;
}
/**
*
* Optional tag overrides that are applied to the resource configuration before import.
*
*
* @param overrideTags
* Optional tag overrides that are applied to the resource configuration before import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withOverrideTags(AssetBundleImportJobOverrideTags overrideTags) {
setOverrideTags(overrideTags);
return this;
}
/**
*
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*
*
* @param overrideValidationStrategy
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*/
public void setOverrideValidationStrategy(AssetBundleImportJobOverrideValidationStrategy overrideValidationStrategy) {
this.overrideValidationStrategy = overrideValidationStrategy;
}
/**
*
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*
*
* @return An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*/
public AssetBundleImportJobOverrideValidationStrategy getOverrideValidationStrategy() {
return this.overrideValidationStrategy;
}
/**
*
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
*
*
* @param overrideValidationStrategy
* An optional validation strategy override for all analyses and dashboards to be applied to the resource
* configuration before import.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withOverrideValidationStrategy(AssetBundleImportJobOverrideValidationStrategy overrideValidationStrategy) {
setOverrideValidationStrategy(overrideValidationStrategy);
return this;
}
/**
*
* An array of warning records that describe all permitted errors that are encountered during the import job.
*
*
* @return An array of warning records that describe all permitted errors that are encountered during the import
* job.
*/
public java.util.List getWarnings() {
return warnings;
}
/**
*
* An array of warning records that describe all permitted errors that are encountered during the import job.
*
*
* @param warnings
* An array of warning records that describe all permitted errors that are encountered during the import job.
*/
public void setWarnings(java.util.Collection warnings) {
if (warnings == null) {
this.warnings = null;
return;
}
this.warnings = new java.util.ArrayList(warnings);
}
/**
*
* An array of warning records that describe all permitted errors that are encountered during the import job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setWarnings(java.util.Collection)} or {@link #withWarnings(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param warnings
* An array of warning records that describe all permitted errors that are encountered during the import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withWarnings(AssetBundleImportJobWarning... warnings) {
if (this.warnings == null) {
setWarnings(new java.util.ArrayList(warnings.length));
}
for (AssetBundleImportJobWarning ele : warnings) {
this.warnings.add(ele);
}
return this;
}
/**
*
* An array of warning records that describe all permitted errors that are encountered during the import job.
*
*
* @param warnings
* An array of warning records that describe all permitted errors that are encountered during the import job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeAssetBundleImportJobResult withWarnings(java.util.Collection warnings) {
setWarnings(warnings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobStatus() != null)
sb.append("JobStatus: ").append(getJobStatus()).append(",");
if (getErrors() != null)
sb.append("Errors: ").append(getErrors()).append(",");
if (getRollbackErrors() != null)
sb.append("RollbackErrors: ").append(getRollbackErrors()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getAssetBundleImportJobId() != null)
sb.append("AssetBundleImportJobId: ").append(getAssetBundleImportJobId()).append(",");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getAssetBundleImportSource() != null)
sb.append("AssetBundleImportSource: ").append(getAssetBundleImportSource()).append(",");
if (getOverrideParameters() != null)
sb.append("OverrideParameters: ").append(getOverrideParameters()).append(",");
if (getFailureAction() != null)
sb.append("FailureAction: ").append(getFailureAction()).append(",");
if (getRequestId() != null)
sb.append("RequestId: ").append(getRequestId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getOverridePermissions() != null)
sb.append("OverridePermissions: ").append(getOverridePermissions()).append(",");
if (getOverrideTags() != null)
sb.append("OverrideTags: ").append(getOverrideTags()).append(",");
if (getOverrideValidationStrategy() != null)
sb.append("OverrideValidationStrategy: ").append(getOverrideValidationStrategy()).append(",");
if (getWarnings() != null)
sb.append("Warnings: ").append(getWarnings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeAssetBundleImportJobResult == false)
return false;
DescribeAssetBundleImportJobResult other = (DescribeAssetBundleImportJobResult) obj;
if (other.getJobStatus() == null ^ this.getJobStatus() == null)
return false;
if (other.getJobStatus() != null && other.getJobStatus().equals(this.getJobStatus()) == false)
return false;
if (other.getErrors() == null ^ this.getErrors() == null)
return false;
if (other.getErrors() != null && other.getErrors().equals(this.getErrors()) == false)
return false;
if (other.getRollbackErrors() == null ^ this.getRollbackErrors() == null)
return false;
if (other.getRollbackErrors() != null && other.getRollbackErrors().equals(this.getRollbackErrors()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getAssetBundleImportJobId() == null ^ this.getAssetBundleImportJobId() == null)
return false;
if (other.getAssetBundleImportJobId() != null && other.getAssetBundleImportJobId().equals(this.getAssetBundleImportJobId()) == false)
return false;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getAssetBundleImportSource() == null ^ this.getAssetBundleImportSource() == null)
return false;
if (other.getAssetBundleImportSource() != null && other.getAssetBundleImportSource().equals(this.getAssetBundleImportSource()) == false)
return false;
if (other.getOverrideParameters() == null ^ this.getOverrideParameters() == null)
return false;
if (other.getOverrideParameters() != null && other.getOverrideParameters().equals(this.getOverrideParameters()) == false)
return false;
if (other.getFailureAction() == null ^ this.getFailureAction() == null)
return false;
if (other.getFailureAction() != null && other.getFailureAction().equals(this.getFailureAction()) == false)
return false;
if (other.getRequestId() == null ^ this.getRequestId() == null)
return false;
if (other.getRequestId() != null && other.getRequestId().equals(this.getRequestId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getOverridePermissions() == null ^ this.getOverridePermissions() == null)
return false;
if (other.getOverridePermissions() != null && other.getOverridePermissions().equals(this.getOverridePermissions()) == false)
return false;
if (other.getOverrideTags() == null ^ this.getOverrideTags() == null)
return false;
if (other.getOverrideTags() != null && other.getOverrideTags().equals(this.getOverrideTags()) == false)
return false;
if (other.getOverrideValidationStrategy() == null ^ this.getOverrideValidationStrategy() == null)
return false;
if (other.getOverrideValidationStrategy() != null && other.getOverrideValidationStrategy().equals(this.getOverrideValidationStrategy()) == false)
return false;
if (other.getWarnings() == null ^ this.getWarnings() == null)
return false;
if (other.getWarnings() != null && other.getWarnings().equals(this.getWarnings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobStatus() == null) ? 0 : getJobStatus().hashCode());
hashCode = prime * hashCode + ((getErrors() == null) ? 0 : getErrors().hashCode());
hashCode = prime * hashCode + ((getRollbackErrors() == null) ? 0 : getRollbackErrors().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getAssetBundleImportJobId() == null) ? 0 : getAssetBundleImportJobId().hashCode());
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getAssetBundleImportSource() == null) ? 0 : getAssetBundleImportSource().hashCode());
hashCode = prime * hashCode + ((getOverrideParameters() == null) ? 0 : getOverrideParameters().hashCode());
hashCode = prime * hashCode + ((getFailureAction() == null) ? 0 : getFailureAction().hashCode());
hashCode = prime * hashCode + ((getRequestId() == null) ? 0 : getRequestId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getOverridePermissions() == null) ? 0 : getOverridePermissions().hashCode());
hashCode = prime * hashCode + ((getOverrideTags() == null) ? 0 : getOverrideTags().hashCode());
hashCode = prime * hashCode + ((getOverrideValidationStrategy() == null) ? 0 : getOverrideValidationStrategy().hashCode());
hashCode = prime * hashCode + ((getWarnings() == null) ? 0 : getWarnings().hashCode());
return hashCode;
}
@Override
public DescribeAssetBundleImportJobResult clone() {
try {
return (DescribeAssetBundleImportJobResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}