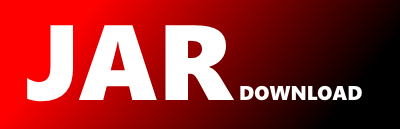
com.amazonaws.services.quicksight.model.HeatMapConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration of a heat map.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HeatMapConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The field wells of the visual.
*
*/
private HeatMapFieldWells fieldWells;
/**
*
* The sort configuration of a heat map.
*
*/
private HeatMapSortConfiguration sortConfiguration;
/**
*
* The label options of the row that is displayed in a heat map
.
*
*/
private ChartAxisLabelOptions rowLabelOptions;
/**
*
* The label options of the column that is displayed in a heat map.
*
*/
private ChartAxisLabelOptions columnLabelOptions;
/**
*
* The color options (gradient color, point of divergence) in a heat map.
*
*/
private ColorScale colorScale;
/**
*
* The legend display setup of the visual.
*
*/
private LegendOptions legend;
/**
*
* The options that determine if visual data labels are displayed.
*
*/
private DataLabelOptions dataLabels;
/**
*
* The tooltip display setup of the visual.
*
*/
private TooltipOptions tooltip;
/**
*
* The general visual interactions setup for a visual.
*
*/
private VisualInteractionOptions interactions;
/**
*
* The field wells of the visual.
*
*
* @param fieldWells
* The field wells of the visual.
*/
public void setFieldWells(HeatMapFieldWells fieldWells) {
this.fieldWells = fieldWells;
}
/**
*
* The field wells of the visual.
*
*
* @return The field wells of the visual.
*/
public HeatMapFieldWells getFieldWells() {
return this.fieldWells;
}
/**
*
* The field wells of the visual.
*
*
* @param fieldWells
* The field wells of the visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withFieldWells(HeatMapFieldWells fieldWells) {
setFieldWells(fieldWells);
return this;
}
/**
*
* The sort configuration of a heat map.
*
*
* @param sortConfiguration
* The sort configuration of a heat map.
*/
public void setSortConfiguration(HeatMapSortConfiguration sortConfiguration) {
this.sortConfiguration = sortConfiguration;
}
/**
*
* The sort configuration of a heat map.
*
*
* @return The sort configuration of a heat map.
*/
public HeatMapSortConfiguration getSortConfiguration() {
return this.sortConfiguration;
}
/**
*
* The sort configuration of a heat map.
*
*
* @param sortConfiguration
* The sort configuration of a heat map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withSortConfiguration(HeatMapSortConfiguration sortConfiguration) {
setSortConfiguration(sortConfiguration);
return this;
}
/**
*
* The label options of the row that is displayed in a heat map
.
*
*
* @param rowLabelOptions
* The label options of the row that is displayed in a heat map
.
*/
public void setRowLabelOptions(ChartAxisLabelOptions rowLabelOptions) {
this.rowLabelOptions = rowLabelOptions;
}
/**
*
* The label options of the row that is displayed in a heat map
.
*
*
* @return The label options of the row that is displayed in a heat map
.
*/
public ChartAxisLabelOptions getRowLabelOptions() {
return this.rowLabelOptions;
}
/**
*
* The label options of the row that is displayed in a heat map
.
*
*
* @param rowLabelOptions
* The label options of the row that is displayed in a heat map
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withRowLabelOptions(ChartAxisLabelOptions rowLabelOptions) {
setRowLabelOptions(rowLabelOptions);
return this;
}
/**
*
* The label options of the column that is displayed in a heat map.
*
*
* @param columnLabelOptions
* The label options of the column that is displayed in a heat map.
*/
public void setColumnLabelOptions(ChartAxisLabelOptions columnLabelOptions) {
this.columnLabelOptions = columnLabelOptions;
}
/**
*
* The label options of the column that is displayed in a heat map.
*
*
* @return The label options of the column that is displayed in a heat map.
*/
public ChartAxisLabelOptions getColumnLabelOptions() {
return this.columnLabelOptions;
}
/**
*
* The label options of the column that is displayed in a heat map.
*
*
* @param columnLabelOptions
* The label options of the column that is displayed in a heat map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withColumnLabelOptions(ChartAxisLabelOptions columnLabelOptions) {
setColumnLabelOptions(columnLabelOptions);
return this;
}
/**
*
* The color options (gradient color, point of divergence) in a heat map.
*
*
* @param colorScale
* The color options (gradient color, point of divergence) in a heat map.
*/
public void setColorScale(ColorScale colorScale) {
this.colorScale = colorScale;
}
/**
*
* The color options (gradient color, point of divergence) in a heat map.
*
*
* @return The color options (gradient color, point of divergence) in a heat map.
*/
public ColorScale getColorScale() {
return this.colorScale;
}
/**
*
* The color options (gradient color, point of divergence) in a heat map.
*
*
* @param colorScale
* The color options (gradient color, point of divergence) in a heat map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withColorScale(ColorScale colorScale) {
setColorScale(colorScale);
return this;
}
/**
*
* The legend display setup of the visual.
*
*
* @param legend
* The legend display setup of the visual.
*/
public void setLegend(LegendOptions legend) {
this.legend = legend;
}
/**
*
* The legend display setup of the visual.
*
*
* @return The legend display setup of the visual.
*/
public LegendOptions getLegend() {
return this.legend;
}
/**
*
* The legend display setup of the visual.
*
*
* @param legend
* The legend display setup of the visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withLegend(LegendOptions legend) {
setLegend(legend);
return this;
}
/**
*
* The options that determine if visual data labels are displayed.
*
*
* @param dataLabels
* The options that determine if visual data labels are displayed.
*/
public void setDataLabels(DataLabelOptions dataLabels) {
this.dataLabels = dataLabels;
}
/**
*
* The options that determine if visual data labels are displayed.
*
*
* @return The options that determine if visual data labels are displayed.
*/
public DataLabelOptions getDataLabels() {
return this.dataLabels;
}
/**
*
* The options that determine if visual data labels are displayed.
*
*
* @param dataLabels
* The options that determine if visual data labels are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withDataLabels(DataLabelOptions dataLabels) {
setDataLabels(dataLabels);
return this;
}
/**
*
* The tooltip display setup of the visual.
*
*
* @param tooltip
* The tooltip display setup of the visual.
*/
public void setTooltip(TooltipOptions tooltip) {
this.tooltip = tooltip;
}
/**
*
* The tooltip display setup of the visual.
*
*
* @return The tooltip display setup of the visual.
*/
public TooltipOptions getTooltip() {
return this.tooltip;
}
/**
*
* The tooltip display setup of the visual.
*
*
* @param tooltip
* The tooltip display setup of the visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withTooltip(TooltipOptions tooltip) {
setTooltip(tooltip);
return this;
}
/**
*
* The general visual interactions setup for a visual.
*
*
* @param interactions
* The general visual interactions setup for a visual.
*/
public void setInteractions(VisualInteractionOptions interactions) {
this.interactions = interactions;
}
/**
*
* The general visual interactions setup for a visual.
*
*
* @return The general visual interactions setup for a visual.
*/
public VisualInteractionOptions getInteractions() {
return this.interactions;
}
/**
*
* The general visual interactions setup for a visual.
*
*
* @param interactions
* The general visual interactions setup for a visual.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HeatMapConfiguration withInteractions(VisualInteractionOptions interactions) {
setInteractions(interactions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFieldWells() != null)
sb.append("FieldWells: ").append(getFieldWells()).append(",");
if (getSortConfiguration() != null)
sb.append("SortConfiguration: ").append(getSortConfiguration()).append(",");
if (getRowLabelOptions() != null)
sb.append("RowLabelOptions: ").append(getRowLabelOptions()).append(",");
if (getColumnLabelOptions() != null)
sb.append("ColumnLabelOptions: ").append(getColumnLabelOptions()).append(",");
if (getColorScale() != null)
sb.append("ColorScale: ").append(getColorScale()).append(",");
if (getLegend() != null)
sb.append("Legend: ").append(getLegend()).append(",");
if (getDataLabels() != null)
sb.append("DataLabels: ").append(getDataLabels()).append(",");
if (getTooltip() != null)
sb.append("Tooltip: ").append(getTooltip()).append(",");
if (getInteractions() != null)
sb.append("Interactions: ").append(getInteractions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HeatMapConfiguration == false)
return false;
HeatMapConfiguration other = (HeatMapConfiguration) obj;
if (other.getFieldWells() == null ^ this.getFieldWells() == null)
return false;
if (other.getFieldWells() != null && other.getFieldWells().equals(this.getFieldWells()) == false)
return false;
if (other.getSortConfiguration() == null ^ this.getSortConfiguration() == null)
return false;
if (other.getSortConfiguration() != null && other.getSortConfiguration().equals(this.getSortConfiguration()) == false)
return false;
if (other.getRowLabelOptions() == null ^ this.getRowLabelOptions() == null)
return false;
if (other.getRowLabelOptions() != null && other.getRowLabelOptions().equals(this.getRowLabelOptions()) == false)
return false;
if (other.getColumnLabelOptions() == null ^ this.getColumnLabelOptions() == null)
return false;
if (other.getColumnLabelOptions() != null && other.getColumnLabelOptions().equals(this.getColumnLabelOptions()) == false)
return false;
if (other.getColorScale() == null ^ this.getColorScale() == null)
return false;
if (other.getColorScale() != null && other.getColorScale().equals(this.getColorScale()) == false)
return false;
if (other.getLegend() == null ^ this.getLegend() == null)
return false;
if (other.getLegend() != null && other.getLegend().equals(this.getLegend()) == false)
return false;
if (other.getDataLabels() == null ^ this.getDataLabels() == null)
return false;
if (other.getDataLabels() != null && other.getDataLabels().equals(this.getDataLabels()) == false)
return false;
if (other.getTooltip() == null ^ this.getTooltip() == null)
return false;
if (other.getTooltip() != null && other.getTooltip().equals(this.getTooltip()) == false)
return false;
if (other.getInteractions() == null ^ this.getInteractions() == null)
return false;
if (other.getInteractions() != null && other.getInteractions().equals(this.getInteractions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFieldWells() == null) ? 0 : getFieldWells().hashCode());
hashCode = prime * hashCode + ((getSortConfiguration() == null) ? 0 : getSortConfiguration().hashCode());
hashCode = prime * hashCode + ((getRowLabelOptions() == null) ? 0 : getRowLabelOptions().hashCode());
hashCode = prime * hashCode + ((getColumnLabelOptions() == null) ? 0 : getColumnLabelOptions().hashCode());
hashCode = prime * hashCode + ((getColorScale() == null) ? 0 : getColorScale().hashCode());
hashCode = prime * hashCode + ((getLegend() == null) ? 0 : getLegend().hashCode());
hashCode = prime * hashCode + ((getDataLabels() == null) ? 0 : getDataLabels().hashCode());
hashCode = prime * hashCode + ((getTooltip() == null) ? 0 : getTooltip().hashCode());
hashCode = prime * hashCode + ((getInteractions() == null) ? 0 : getInteractions().hashCode());
return hashCode;
}
@Override
public HeatMapConfiguration clone() {
try {
return (HeatMapConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.HeatMapConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}