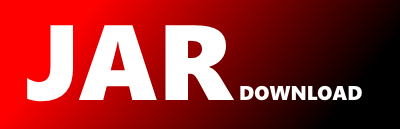
com.amazonaws.services.quicksight.model.LineChartSortConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The sort configuration of a line chart.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LineChartSortConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The sort configuration of the category fields.
*
*/
private java.util.List categorySort;
/**
*
* The limit on the number of categories that are displayed in a line chart.
*
*/
private ItemsLimitConfiguration categoryItemsLimitConfiguration;
/**
*
* The limit on the number of lines that are displayed in a line chart.
*
*/
private ItemsLimitConfiguration colorItemsLimitConfiguration;
/**
*
* The sort configuration of the small multiples field.
*
*/
private java.util.List smallMultiplesSort;
/**
*
* The limit on the number of small multiples panels that are displayed.
*
*/
private ItemsLimitConfiguration smallMultiplesLimitConfiguration;
/**
*
* The sort configuration of the category fields.
*
*
* @return The sort configuration of the category fields.
*/
public java.util.List getCategorySort() {
return categorySort;
}
/**
*
* The sort configuration of the category fields.
*
*
* @param categorySort
* The sort configuration of the category fields.
*/
public void setCategorySort(java.util.Collection categorySort) {
if (categorySort == null) {
this.categorySort = null;
return;
}
this.categorySort = new java.util.ArrayList(categorySort);
}
/**
*
* The sort configuration of the category fields.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCategorySort(java.util.Collection)} or {@link #withCategorySort(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param categorySort
* The sort configuration of the category fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withCategorySort(FieldSortOptions... categorySort) {
if (this.categorySort == null) {
setCategorySort(new java.util.ArrayList(categorySort.length));
}
for (FieldSortOptions ele : categorySort) {
this.categorySort.add(ele);
}
return this;
}
/**
*
* The sort configuration of the category fields.
*
*
* @param categorySort
* The sort configuration of the category fields.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withCategorySort(java.util.Collection categorySort) {
setCategorySort(categorySort);
return this;
}
/**
*
* The limit on the number of categories that are displayed in a line chart.
*
*
* @param categoryItemsLimitConfiguration
* The limit on the number of categories that are displayed in a line chart.
*/
public void setCategoryItemsLimitConfiguration(ItemsLimitConfiguration categoryItemsLimitConfiguration) {
this.categoryItemsLimitConfiguration = categoryItemsLimitConfiguration;
}
/**
*
* The limit on the number of categories that are displayed in a line chart.
*
*
* @return The limit on the number of categories that are displayed in a line chart.
*/
public ItemsLimitConfiguration getCategoryItemsLimitConfiguration() {
return this.categoryItemsLimitConfiguration;
}
/**
*
* The limit on the number of categories that are displayed in a line chart.
*
*
* @param categoryItemsLimitConfiguration
* The limit on the number of categories that are displayed in a line chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withCategoryItemsLimitConfiguration(ItemsLimitConfiguration categoryItemsLimitConfiguration) {
setCategoryItemsLimitConfiguration(categoryItemsLimitConfiguration);
return this;
}
/**
*
* The limit on the number of lines that are displayed in a line chart.
*
*
* @param colorItemsLimitConfiguration
* The limit on the number of lines that are displayed in a line chart.
*/
public void setColorItemsLimitConfiguration(ItemsLimitConfiguration colorItemsLimitConfiguration) {
this.colorItemsLimitConfiguration = colorItemsLimitConfiguration;
}
/**
*
* The limit on the number of lines that are displayed in a line chart.
*
*
* @return The limit on the number of lines that are displayed in a line chart.
*/
public ItemsLimitConfiguration getColorItemsLimitConfiguration() {
return this.colorItemsLimitConfiguration;
}
/**
*
* The limit on the number of lines that are displayed in a line chart.
*
*
* @param colorItemsLimitConfiguration
* The limit on the number of lines that are displayed in a line chart.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withColorItemsLimitConfiguration(ItemsLimitConfiguration colorItemsLimitConfiguration) {
setColorItemsLimitConfiguration(colorItemsLimitConfiguration);
return this;
}
/**
*
* The sort configuration of the small multiples field.
*
*
* @return The sort configuration of the small multiples field.
*/
public java.util.List getSmallMultiplesSort() {
return smallMultiplesSort;
}
/**
*
* The sort configuration of the small multiples field.
*
*
* @param smallMultiplesSort
* The sort configuration of the small multiples field.
*/
public void setSmallMultiplesSort(java.util.Collection smallMultiplesSort) {
if (smallMultiplesSort == null) {
this.smallMultiplesSort = null;
return;
}
this.smallMultiplesSort = new java.util.ArrayList(smallMultiplesSort);
}
/**
*
* The sort configuration of the small multiples field.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSmallMultiplesSort(java.util.Collection)} or {@link #withSmallMultiplesSort(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param smallMultiplesSort
* The sort configuration of the small multiples field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withSmallMultiplesSort(FieldSortOptions... smallMultiplesSort) {
if (this.smallMultiplesSort == null) {
setSmallMultiplesSort(new java.util.ArrayList(smallMultiplesSort.length));
}
for (FieldSortOptions ele : smallMultiplesSort) {
this.smallMultiplesSort.add(ele);
}
return this;
}
/**
*
* The sort configuration of the small multiples field.
*
*
* @param smallMultiplesSort
* The sort configuration of the small multiples field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withSmallMultiplesSort(java.util.Collection smallMultiplesSort) {
setSmallMultiplesSort(smallMultiplesSort);
return this;
}
/**
*
* The limit on the number of small multiples panels that are displayed.
*
*
* @param smallMultiplesLimitConfiguration
* The limit on the number of small multiples panels that are displayed.
*/
public void setSmallMultiplesLimitConfiguration(ItemsLimitConfiguration smallMultiplesLimitConfiguration) {
this.smallMultiplesLimitConfiguration = smallMultiplesLimitConfiguration;
}
/**
*
* The limit on the number of small multiples panels that are displayed.
*
*
* @return The limit on the number of small multiples panels that are displayed.
*/
public ItemsLimitConfiguration getSmallMultiplesLimitConfiguration() {
return this.smallMultiplesLimitConfiguration;
}
/**
*
* The limit on the number of small multiples panels that are displayed.
*
*
* @param smallMultiplesLimitConfiguration
* The limit on the number of small multiples panels that are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LineChartSortConfiguration withSmallMultiplesLimitConfiguration(ItemsLimitConfiguration smallMultiplesLimitConfiguration) {
setSmallMultiplesLimitConfiguration(smallMultiplesLimitConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCategorySort() != null)
sb.append("CategorySort: ").append(getCategorySort()).append(",");
if (getCategoryItemsLimitConfiguration() != null)
sb.append("CategoryItemsLimitConfiguration: ").append(getCategoryItemsLimitConfiguration()).append(",");
if (getColorItemsLimitConfiguration() != null)
sb.append("ColorItemsLimitConfiguration: ").append(getColorItemsLimitConfiguration()).append(",");
if (getSmallMultiplesSort() != null)
sb.append("SmallMultiplesSort: ").append(getSmallMultiplesSort()).append(",");
if (getSmallMultiplesLimitConfiguration() != null)
sb.append("SmallMultiplesLimitConfiguration: ").append(getSmallMultiplesLimitConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LineChartSortConfiguration == false)
return false;
LineChartSortConfiguration other = (LineChartSortConfiguration) obj;
if (other.getCategorySort() == null ^ this.getCategorySort() == null)
return false;
if (other.getCategorySort() != null && other.getCategorySort().equals(this.getCategorySort()) == false)
return false;
if (other.getCategoryItemsLimitConfiguration() == null ^ this.getCategoryItemsLimitConfiguration() == null)
return false;
if (other.getCategoryItemsLimitConfiguration() != null
&& other.getCategoryItemsLimitConfiguration().equals(this.getCategoryItemsLimitConfiguration()) == false)
return false;
if (other.getColorItemsLimitConfiguration() == null ^ this.getColorItemsLimitConfiguration() == null)
return false;
if (other.getColorItemsLimitConfiguration() != null && other.getColorItemsLimitConfiguration().equals(this.getColorItemsLimitConfiguration()) == false)
return false;
if (other.getSmallMultiplesSort() == null ^ this.getSmallMultiplesSort() == null)
return false;
if (other.getSmallMultiplesSort() != null && other.getSmallMultiplesSort().equals(this.getSmallMultiplesSort()) == false)
return false;
if (other.getSmallMultiplesLimitConfiguration() == null ^ this.getSmallMultiplesLimitConfiguration() == null)
return false;
if (other.getSmallMultiplesLimitConfiguration() != null
&& other.getSmallMultiplesLimitConfiguration().equals(this.getSmallMultiplesLimitConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCategorySort() == null) ? 0 : getCategorySort().hashCode());
hashCode = prime * hashCode + ((getCategoryItemsLimitConfiguration() == null) ? 0 : getCategoryItemsLimitConfiguration().hashCode());
hashCode = prime * hashCode + ((getColorItemsLimitConfiguration() == null) ? 0 : getColorItemsLimitConfiguration().hashCode());
hashCode = prime * hashCode + ((getSmallMultiplesSort() == null) ? 0 : getSmallMultiplesSort().hashCode());
hashCode = prime * hashCode + ((getSmallMultiplesLimitConfiguration() == null) ? 0 : getSmallMultiplesLimitConfiguration().hashCode());
return hashCode;
}
@Override
public LineChartSortConfiguration clone() {
try {
return (LineChartSortConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.LineChartSortConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}