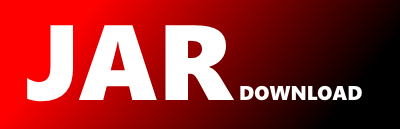
com.amazonaws.services.quicksight.model.StartAssetBundleExportJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartAssetBundleExportJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the Amazon Web Services account to export assets from.
*
*/
private String awsAccountId;
/**
*
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse this ID
* for another job.
*
*/
private String assetBundleExportJobId;
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*/
private java.util.List resourceArns;
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*/
private Boolean includeAllDependencies;
/**
*
* The export data format.
*
*/
private String exportFormat;
/**
*
* An optional collection of structures that generate CloudFormation parameters to override the existing resource
* property values when the resource is exported to a new CloudFormation template.
*
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
API call
* is set to CLOUDFORMATION_JSON
.
*
*/
private AssetBundleCloudFormationOverridePropertyConfiguration cloudFormationOverridePropertyConfiguration;
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*/
private Boolean includePermissions;
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*/
private Boolean includeTags;
/**
*
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown as
* warnings. The default value for StrictModeForAllResources
is FALSE
.
*
*/
private AssetBundleExportJobValidationStrategy validationStrategy;
/**
*
* The ID of the Amazon Web Services account to export assets from.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account to export assets from.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account to export assets from.
*
*
* @return The ID of the Amazon Web Services account to export assets from.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The ID of the Amazon Web Services account to export assets from.
*
*
* @param awsAccountId
* The ID of the Amazon Web Services account to export assets from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse this ID
* for another job.
*
*
* @param assetBundleExportJobId
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse
* this ID for another job.
*/
public void setAssetBundleExportJobId(String assetBundleExportJobId) {
this.assetBundleExportJobId = assetBundleExportJobId;
}
/**
*
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse this ID
* for another job.
*
*
* @return The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse
* this ID for another job.
*/
public String getAssetBundleExportJobId() {
return this.assetBundleExportJobId;
}
/**
*
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse this ID
* for another job.
*
*
* @param assetBundleExportJobId
* The ID of the job. This ID is unique while the job is running. After the job is completed, you can reuse
* this ID for another job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withAssetBundleExportJobId(String assetBundleExportJobId) {
setAssetBundleExportJobId(assetBundleExportJobId);
return this;
}
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*
* @return An array of resource ARNs to export. The following resources are supported.
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the
* resources can be exported.
*/
public java.util.List getResourceArns() {
return resourceArns;
}
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*
* @param resourceArns
* An array of resource ARNs to export. The following resources are supported.
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the
* resources can be exported.
*/
public void setResourceArns(java.util.Collection resourceArns) {
if (resourceArns == null) {
this.resourceArns = null;
return;
}
this.resourceArns = new java.util.ArrayList(resourceArns);
}
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceArns(java.util.Collection)} or {@link #withResourceArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param resourceArns
* An array of resource ARNs to export. The following resources are supported.
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the
* resources can be exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withResourceArns(String... resourceArns) {
if (this.resourceArns == null) {
setResourceArns(new java.util.ArrayList(resourceArns.length));
}
for (String ele : resourceArns) {
this.resourceArns.add(ele);
}
return this;
}
/**
*
* An array of resource ARNs to export. The following resources are supported.
*
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the resources
* can be exported.
*
*
* @param resourceArns
* An array of resource ARNs to export. The following resources are supported.
*
* -
*
* Analysis
*
*
* -
*
* Dashboard
*
*
* -
*
* DataSet
*
*
* -
*
* DataSource
*
*
* -
*
* RefreshSchedule
*
*
* -
*
* Theme
*
*
* -
*
* VPCConnection
*
*
*
*
* The API caller must have the necessary permissions in their IAM role to access each resource before the
* resources can be exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withResourceArns(java.util.Collection resourceArns) {
setResourceArns(resourceArns);
return this;
}
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*
* @param includeAllDependencies
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the
* job. For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource
* that is a dependency of the dashboard is also exported.
*/
public void setIncludeAllDependencies(Boolean includeAllDependencies) {
this.includeAllDependencies = includeAllDependencies;
}
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*
* @return A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the
* job. For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource
* that is a dependency of the dashboard is also exported.
*/
public Boolean getIncludeAllDependencies() {
return this.includeAllDependencies;
}
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*
* @param includeAllDependencies
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the
* job. For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource
* that is a dependency of the dashboard is also exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withIncludeAllDependencies(Boolean includeAllDependencies) {
setIncludeAllDependencies(includeAllDependencies);
return this;
}
/**
*
* A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the job.
* For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource that is a
* dependency of the dashboard is also exported.
*
*
* @return A Boolean that determines whether all dependencies of each resource ARN are recursively exported with the
* job. For example, say you provided a Dashboard ARN to the ResourceArns
parameter. If you set
* IncludeAllDependencies
to TRUE
, any theme, dataset, and data source resource
* that is a dependency of the dashboard is also exported.
*/
public Boolean isIncludeAllDependencies() {
return this.includeAllDependencies;
}
/**
*
* The export data format.
*
*
* @param exportFormat
* The export data format.
* @see AssetBundleExportFormat
*/
public void setExportFormat(String exportFormat) {
this.exportFormat = exportFormat;
}
/**
*
* The export data format.
*
*
* @return The export data format.
* @see AssetBundleExportFormat
*/
public String getExportFormat() {
return this.exportFormat;
}
/**
*
* The export data format.
*
*
* @param exportFormat
* The export data format.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleExportFormat
*/
public StartAssetBundleExportJobRequest withExportFormat(String exportFormat) {
setExportFormat(exportFormat);
return this;
}
/**
*
* The export data format.
*
*
* @param exportFormat
* The export data format.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetBundleExportFormat
*/
public StartAssetBundleExportJobRequest withExportFormat(AssetBundleExportFormat exportFormat) {
this.exportFormat = exportFormat.toString();
return this;
}
/**
*
* An optional collection of structures that generate CloudFormation parameters to override the existing resource
* property values when the resource is exported to a new CloudFormation template.
*
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
API call
* is set to CLOUDFORMATION_JSON
.
*
*
* @param cloudFormationOverridePropertyConfiguration
* An optional collection of structures that generate CloudFormation parameters to override the existing
* resource property values when the resource is exported to a new CloudFormation template.
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
* API call is set to CLOUDFORMATION_JSON
.
*/
public void setCloudFormationOverridePropertyConfiguration(
AssetBundleCloudFormationOverridePropertyConfiguration cloudFormationOverridePropertyConfiguration) {
this.cloudFormationOverridePropertyConfiguration = cloudFormationOverridePropertyConfiguration;
}
/**
*
* An optional collection of structures that generate CloudFormation parameters to override the existing resource
* property values when the resource is exported to a new CloudFormation template.
*
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
API call
* is set to CLOUDFORMATION_JSON
.
*
*
* @return An optional collection of structures that generate CloudFormation parameters to override the existing
* resource property values when the resource is exported to a new CloudFormation template.
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
* API call is set to CLOUDFORMATION_JSON
.
*/
public AssetBundleCloudFormationOverridePropertyConfiguration getCloudFormationOverridePropertyConfiguration() {
return this.cloudFormationOverridePropertyConfiguration;
}
/**
*
* An optional collection of structures that generate CloudFormation parameters to override the existing resource
* property values when the resource is exported to a new CloudFormation template.
*
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
API call
* is set to CLOUDFORMATION_JSON
.
*
*
* @param cloudFormationOverridePropertyConfiguration
* An optional collection of structures that generate CloudFormation parameters to override the existing
* resource property values when the resource is exported to a new CloudFormation template.
*
* Use this field if the ExportFormat
field of a StartAssetBundleExportJobRequest
* API call is set to CLOUDFORMATION_JSON
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withCloudFormationOverridePropertyConfiguration(
AssetBundleCloudFormationOverridePropertyConfiguration cloudFormationOverridePropertyConfiguration) {
setCloudFormationOverridePropertyConfiguration(cloudFormationOverridePropertyConfiguration);
return this;
}
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*
* @param includePermissions
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you
* set IncludePermissions
to TRUE
, any permissions associated with each resource
* are exported.
*/
public void setIncludePermissions(Boolean includePermissions) {
this.includePermissions = includePermissions;
}
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*
* @return A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you
* set IncludePermissions
to TRUE
, any permissions associated with each resource
* are exported.
*/
public Boolean getIncludePermissions() {
return this.includePermissions;
}
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*
* @param includePermissions
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you
* set IncludePermissions
to TRUE
, any permissions associated with each resource
* are exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withIncludePermissions(Boolean includePermissions) {
setIncludePermissions(includePermissions);
return this;
}
/**
*
* A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you set
* IncludePermissions
to TRUE
, any permissions associated with each resource are exported.
*
*
* @return A Boolean that determines whether all permissions for each resource ARN are exported with the job. If you
* set IncludePermissions
to TRUE
, any permissions associated with each resource
* are exported.
*/
public Boolean isIncludePermissions() {
return this.includePermissions;
}
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*
* @param includeTags
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*/
public void setIncludeTags(Boolean includeTags) {
this.includeTags = includeTags;
}
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*
* @return A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*/
public Boolean getIncludeTags() {
return this.includeTags;
}
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*
* @param includeTags
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withIncludeTags(Boolean includeTags) {
setIncludeTags(includeTags);
return this;
}
/**
*
* A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*
*
* @return A Boolean that determines whether all tags for each resource ARN are exported with the job. If you set
* IncludeTags
to TRUE
, any tags associated with each resource are exported.
*/
public Boolean isIncludeTags() {
return this.includeTags;
}
/**
*
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown as
* warnings. The default value for StrictModeForAllResources
is FALSE
.
*
*
* @param validationStrategy
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown
* as warnings. The default value for StrictModeForAllResources
is FALSE
.
*/
public void setValidationStrategy(AssetBundleExportJobValidationStrategy validationStrategy) {
this.validationStrategy = validationStrategy;
}
/**
*
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown as
* warnings. The default value for StrictModeForAllResources
is FALSE
.
*
*
* @return An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown
* as warnings. The default value for StrictModeForAllResources
is FALSE
.
*/
public AssetBundleExportJobValidationStrategy getValidationStrategy() {
return this.validationStrategy;
}
/**
*
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown as
* warnings. The default value for StrictModeForAllResources
is FALSE
.
*
*
* @param validationStrategy
* An optional parameter that determines which validation strategy to use for the export job. If
* StrictModeForAllResources
is set to TRUE
, strict validation for every error is
* enforced. If it is set to FALSE
, validation is skipped for specific UI errors that are shown
* as warnings. The default value for StrictModeForAllResources
is FALSE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartAssetBundleExportJobRequest withValidationStrategy(AssetBundleExportJobValidationStrategy validationStrategy) {
setValidationStrategy(validationStrategy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getAssetBundleExportJobId() != null)
sb.append("AssetBundleExportJobId: ").append(getAssetBundleExportJobId()).append(",");
if (getResourceArns() != null)
sb.append("ResourceArns: ").append(getResourceArns()).append(",");
if (getIncludeAllDependencies() != null)
sb.append("IncludeAllDependencies: ").append(getIncludeAllDependencies()).append(",");
if (getExportFormat() != null)
sb.append("ExportFormat: ").append(getExportFormat()).append(",");
if (getCloudFormationOverridePropertyConfiguration() != null)
sb.append("CloudFormationOverridePropertyConfiguration: ").append(getCloudFormationOverridePropertyConfiguration()).append(",");
if (getIncludePermissions() != null)
sb.append("IncludePermissions: ").append(getIncludePermissions()).append(",");
if (getIncludeTags() != null)
sb.append("IncludeTags: ").append(getIncludeTags()).append(",");
if (getValidationStrategy() != null)
sb.append("ValidationStrategy: ").append(getValidationStrategy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartAssetBundleExportJobRequest == false)
return false;
StartAssetBundleExportJobRequest other = (StartAssetBundleExportJobRequest) obj;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getAssetBundleExportJobId() == null ^ this.getAssetBundleExportJobId() == null)
return false;
if (other.getAssetBundleExportJobId() != null && other.getAssetBundleExportJobId().equals(this.getAssetBundleExportJobId()) == false)
return false;
if (other.getResourceArns() == null ^ this.getResourceArns() == null)
return false;
if (other.getResourceArns() != null && other.getResourceArns().equals(this.getResourceArns()) == false)
return false;
if (other.getIncludeAllDependencies() == null ^ this.getIncludeAllDependencies() == null)
return false;
if (other.getIncludeAllDependencies() != null && other.getIncludeAllDependencies().equals(this.getIncludeAllDependencies()) == false)
return false;
if (other.getExportFormat() == null ^ this.getExportFormat() == null)
return false;
if (other.getExportFormat() != null && other.getExportFormat().equals(this.getExportFormat()) == false)
return false;
if (other.getCloudFormationOverridePropertyConfiguration() == null ^ this.getCloudFormationOverridePropertyConfiguration() == null)
return false;
if (other.getCloudFormationOverridePropertyConfiguration() != null
&& other.getCloudFormationOverridePropertyConfiguration().equals(this.getCloudFormationOverridePropertyConfiguration()) == false)
return false;
if (other.getIncludePermissions() == null ^ this.getIncludePermissions() == null)
return false;
if (other.getIncludePermissions() != null && other.getIncludePermissions().equals(this.getIncludePermissions()) == false)
return false;
if (other.getIncludeTags() == null ^ this.getIncludeTags() == null)
return false;
if (other.getIncludeTags() != null && other.getIncludeTags().equals(this.getIncludeTags()) == false)
return false;
if (other.getValidationStrategy() == null ^ this.getValidationStrategy() == null)
return false;
if (other.getValidationStrategy() != null && other.getValidationStrategy().equals(this.getValidationStrategy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getAssetBundleExportJobId() == null) ? 0 : getAssetBundleExportJobId().hashCode());
hashCode = prime * hashCode + ((getResourceArns() == null) ? 0 : getResourceArns().hashCode());
hashCode = prime * hashCode + ((getIncludeAllDependencies() == null) ? 0 : getIncludeAllDependencies().hashCode());
hashCode = prime * hashCode + ((getExportFormat() == null) ? 0 : getExportFormat().hashCode());
hashCode = prime * hashCode
+ ((getCloudFormationOverridePropertyConfiguration() == null) ? 0 : getCloudFormationOverridePropertyConfiguration().hashCode());
hashCode = prime * hashCode + ((getIncludePermissions() == null) ? 0 : getIncludePermissions().hashCode());
hashCode = prime * hashCode + ((getIncludeTags() == null) ? 0 : getIncludeTags().hashCode());
hashCode = prime * hashCode + ((getValidationStrategy() == null) ? 0 : getValidationStrategy().hashCode());
return hashCode;
}
@Override
public StartAssetBundleExportJobRequest clone() {
return (StartAssetBundleExportJobRequest) super.clone();
}
}