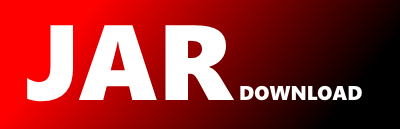
com.amazonaws.services.quicksight.model.SubtotalOptions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The subtotal options.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SubtotalOptions implements Serializable, Cloneable, StructuredPojo {
/**
*
* The visibility configuration for the subtotal cells.
*
*/
private String totalsVisibility;
/**
*
* The custom label string for the subtotal cells.
*
*/
private String customLabel;
/**
*
* The field level (all, custom, last) for the subtotal cells.
*
*/
private String fieldLevel;
/**
*
* The optional configuration of subtotal cells.
*
*/
private java.util.List fieldLevelOptions;
/**
*
* The cell styling options for the subtotal cells.
*
*/
private TableCellStyle totalCellStyle;
/**
*
* The cell styling options for the subtotals of value cells.
*
*/
private TableCellStyle valueCellStyle;
/**
*
* The cell styling options for the subtotals of header cells.
*
*/
private TableCellStyle metricHeaderCellStyle;
/**
*
* The style targets options for subtotals.
*
*/
private java.util.List styleTargets;
/**
*
* The visibility configuration for the subtotal cells.
*
*
* @param totalsVisibility
* The visibility configuration for the subtotal cells.
* @see Visibility
*/
public void setTotalsVisibility(String totalsVisibility) {
this.totalsVisibility = totalsVisibility;
}
/**
*
* The visibility configuration for the subtotal cells.
*
*
* @return The visibility configuration for the subtotal cells.
* @see Visibility
*/
public String getTotalsVisibility() {
return this.totalsVisibility;
}
/**
*
* The visibility configuration for the subtotal cells.
*
*
* @param totalsVisibility
* The visibility configuration for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public SubtotalOptions withTotalsVisibility(String totalsVisibility) {
setTotalsVisibility(totalsVisibility);
return this;
}
/**
*
* The visibility configuration for the subtotal cells.
*
*
* @param totalsVisibility
* The visibility configuration for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Visibility
*/
public SubtotalOptions withTotalsVisibility(Visibility totalsVisibility) {
this.totalsVisibility = totalsVisibility.toString();
return this;
}
/**
*
* The custom label string for the subtotal cells.
*
*
* @param customLabel
* The custom label string for the subtotal cells.
*/
public void setCustomLabel(String customLabel) {
this.customLabel = customLabel;
}
/**
*
* The custom label string for the subtotal cells.
*
*
* @return The custom label string for the subtotal cells.
*/
public String getCustomLabel() {
return this.customLabel;
}
/**
*
* The custom label string for the subtotal cells.
*
*
* @param customLabel
* The custom label string for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withCustomLabel(String customLabel) {
setCustomLabel(customLabel);
return this;
}
/**
*
* The field level (all, custom, last) for the subtotal cells.
*
*
* @param fieldLevel
* The field level (all, custom, last) for the subtotal cells.
* @see PivotTableSubtotalLevel
*/
public void setFieldLevel(String fieldLevel) {
this.fieldLevel = fieldLevel;
}
/**
*
* The field level (all, custom, last) for the subtotal cells.
*
*
* @return The field level (all, custom, last) for the subtotal cells.
* @see PivotTableSubtotalLevel
*/
public String getFieldLevel() {
return this.fieldLevel;
}
/**
*
* The field level (all, custom, last) for the subtotal cells.
*
*
* @param fieldLevel
* The field level (all, custom, last) for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PivotTableSubtotalLevel
*/
public SubtotalOptions withFieldLevel(String fieldLevel) {
setFieldLevel(fieldLevel);
return this;
}
/**
*
* The field level (all, custom, last) for the subtotal cells.
*
*
* @param fieldLevel
* The field level (all, custom, last) for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PivotTableSubtotalLevel
*/
public SubtotalOptions withFieldLevel(PivotTableSubtotalLevel fieldLevel) {
this.fieldLevel = fieldLevel.toString();
return this;
}
/**
*
* The optional configuration of subtotal cells.
*
*
* @return The optional configuration of subtotal cells.
*/
public java.util.List getFieldLevelOptions() {
return fieldLevelOptions;
}
/**
*
* The optional configuration of subtotal cells.
*
*
* @param fieldLevelOptions
* The optional configuration of subtotal cells.
*/
public void setFieldLevelOptions(java.util.Collection fieldLevelOptions) {
if (fieldLevelOptions == null) {
this.fieldLevelOptions = null;
return;
}
this.fieldLevelOptions = new java.util.ArrayList(fieldLevelOptions);
}
/**
*
* The optional configuration of subtotal cells.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFieldLevelOptions(java.util.Collection)} or {@link #withFieldLevelOptions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param fieldLevelOptions
* The optional configuration of subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withFieldLevelOptions(PivotTableFieldSubtotalOptions... fieldLevelOptions) {
if (this.fieldLevelOptions == null) {
setFieldLevelOptions(new java.util.ArrayList(fieldLevelOptions.length));
}
for (PivotTableFieldSubtotalOptions ele : fieldLevelOptions) {
this.fieldLevelOptions.add(ele);
}
return this;
}
/**
*
* The optional configuration of subtotal cells.
*
*
* @param fieldLevelOptions
* The optional configuration of subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withFieldLevelOptions(java.util.Collection fieldLevelOptions) {
setFieldLevelOptions(fieldLevelOptions);
return this;
}
/**
*
* The cell styling options for the subtotal cells.
*
*
* @param totalCellStyle
* The cell styling options for the subtotal cells.
*/
public void setTotalCellStyle(TableCellStyle totalCellStyle) {
this.totalCellStyle = totalCellStyle;
}
/**
*
* The cell styling options for the subtotal cells.
*
*
* @return The cell styling options for the subtotal cells.
*/
public TableCellStyle getTotalCellStyle() {
return this.totalCellStyle;
}
/**
*
* The cell styling options for the subtotal cells.
*
*
* @param totalCellStyle
* The cell styling options for the subtotal cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withTotalCellStyle(TableCellStyle totalCellStyle) {
setTotalCellStyle(totalCellStyle);
return this;
}
/**
*
* The cell styling options for the subtotals of value cells.
*
*
* @param valueCellStyle
* The cell styling options for the subtotals of value cells.
*/
public void setValueCellStyle(TableCellStyle valueCellStyle) {
this.valueCellStyle = valueCellStyle;
}
/**
*
* The cell styling options for the subtotals of value cells.
*
*
* @return The cell styling options for the subtotals of value cells.
*/
public TableCellStyle getValueCellStyle() {
return this.valueCellStyle;
}
/**
*
* The cell styling options for the subtotals of value cells.
*
*
* @param valueCellStyle
* The cell styling options for the subtotals of value cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withValueCellStyle(TableCellStyle valueCellStyle) {
setValueCellStyle(valueCellStyle);
return this;
}
/**
*
* The cell styling options for the subtotals of header cells.
*
*
* @param metricHeaderCellStyle
* The cell styling options for the subtotals of header cells.
*/
public void setMetricHeaderCellStyle(TableCellStyle metricHeaderCellStyle) {
this.metricHeaderCellStyle = metricHeaderCellStyle;
}
/**
*
* The cell styling options for the subtotals of header cells.
*
*
* @return The cell styling options for the subtotals of header cells.
*/
public TableCellStyle getMetricHeaderCellStyle() {
return this.metricHeaderCellStyle;
}
/**
*
* The cell styling options for the subtotals of header cells.
*
*
* @param metricHeaderCellStyle
* The cell styling options for the subtotals of header cells.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withMetricHeaderCellStyle(TableCellStyle metricHeaderCellStyle) {
setMetricHeaderCellStyle(metricHeaderCellStyle);
return this;
}
/**
*
* The style targets options for subtotals.
*
*
* @return The style targets options for subtotals.
*/
public java.util.List getStyleTargets() {
return styleTargets;
}
/**
*
* The style targets options for subtotals.
*
*
* @param styleTargets
* The style targets options for subtotals.
*/
public void setStyleTargets(java.util.Collection styleTargets) {
if (styleTargets == null) {
this.styleTargets = null;
return;
}
this.styleTargets = new java.util.ArrayList(styleTargets);
}
/**
*
* The style targets options for subtotals.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStyleTargets(java.util.Collection)} or {@link #withStyleTargets(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param styleTargets
* The style targets options for subtotals.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withStyleTargets(TableStyleTarget... styleTargets) {
if (this.styleTargets == null) {
setStyleTargets(new java.util.ArrayList(styleTargets.length));
}
for (TableStyleTarget ele : styleTargets) {
this.styleTargets.add(ele);
}
return this;
}
/**
*
* The style targets options for subtotals.
*
*
* @param styleTargets
* The style targets options for subtotals.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtotalOptions withStyleTargets(java.util.Collection styleTargets) {
setStyleTargets(styleTargets);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTotalsVisibility() != null)
sb.append("TotalsVisibility: ").append(getTotalsVisibility()).append(",");
if (getCustomLabel() != null)
sb.append("CustomLabel: ").append(getCustomLabel()).append(",");
if (getFieldLevel() != null)
sb.append("FieldLevel: ").append(getFieldLevel()).append(",");
if (getFieldLevelOptions() != null)
sb.append("FieldLevelOptions: ").append(getFieldLevelOptions()).append(",");
if (getTotalCellStyle() != null)
sb.append("TotalCellStyle: ").append(getTotalCellStyle()).append(",");
if (getValueCellStyle() != null)
sb.append("ValueCellStyle: ").append(getValueCellStyle()).append(",");
if (getMetricHeaderCellStyle() != null)
sb.append("MetricHeaderCellStyle: ").append(getMetricHeaderCellStyle()).append(",");
if (getStyleTargets() != null)
sb.append("StyleTargets: ").append(getStyleTargets());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SubtotalOptions == false)
return false;
SubtotalOptions other = (SubtotalOptions) obj;
if (other.getTotalsVisibility() == null ^ this.getTotalsVisibility() == null)
return false;
if (other.getTotalsVisibility() != null && other.getTotalsVisibility().equals(this.getTotalsVisibility()) == false)
return false;
if (other.getCustomLabel() == null ^ this.getCustomLabel() == null)
return false;
if (other.getCustomLabel() != null && other.getCustomLabel().equals(this.getCustomLabel()) == false)
return false;
if (other.getFieldLevel() == null ^ this.getFieldLevel() == null)
return false;
if (other.getFieldLevel() != null && other.getFieldLevel().equals(this.getFieldLevel()) == false)
return false;
if (other.getFieldLevelOptions() == null ^ this.getFieldLevelOptions() == null)
return false;
if (other.getFieldLevelOptions() != null && other.getFieldLevelOptions().equals(this.getFieldLevelOptions()) == false)
return false;
if (other.getTotalCellStyle() == null ^ this.getTotalCellStyle() == null)
return false;
if (other.getTotalCellStyle() != null && other.getTotalCellStyle().equals(this.getTotalCellStyle()) == false)
return false;
if (other.getValueCellStyle() == null ^ this.getValueCellStyle() == null)
return false;
if (other.getValueCellStyle() != null && other.getValueCellStyle().equals(this.getValueCellStyle()) == false)
return false;
if (other.getMetricHeaderCellStyle() == null ^ this.getMetricHeaderCellStyle() == null)
return false;
if (other.getMetricHeaderCellStyle() != null && other.getMetricHeaderCellStyle().equals(this.getMetricHeaderCellStyle()) == false)
return false;
if (other.getStyleTargets() == null ^ this.getStyleTargets() == null)
return false;
if (other.getStyleTargets() != null && other.getStyleTargets().equals(this.getStyleTargets()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTotalsVisibility() == null) ? 0 : getTotalsVisibility().hashCode());
hashCode = prime * hashCode + ((getCustomLabel() == null) ? 0 : getCustomLabel().hashCode());
hashCode = prime * hashCode + ((getFieldLevel() == null) ? 0 : getFieldLevel().hashCode());
hashCode = prime * hashCode + ((getFieldLevelOptions() == null) ? 0 : getFieldLevelOptions().hashCode());
hashCode = prime * hashCode + ((getTotalCellStyle() == null) ? 0 : getTotalCellStyle().hashCode());
hashCode = prime * hashCode + ((getValueCellStyle() == null) ? 0 : getValueCellStyle().hashCode());
hashCode = prime * hashCode + ((getMetricHeaderCellStyle() == null) ? 0 : getMetricHeaderCellStyle().hashCode());
hashCode = prime * hashCode + ((getStyleTargets() == null) ? 0 : getStyleTargets().hashCode());
return hashCode;
}
@Override
public SubtotalOptions clone() {
try {
return (SubtotalOptions) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.quicksight.model.transform.SubtotalOptionsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}