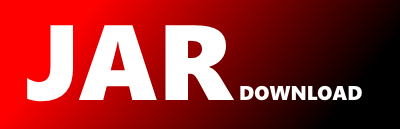
com.amazonaws.services.quicksight.model.UpdateDataSourceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-quicksight Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.quicksight.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateDataSourceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Web Services account ID.
*
*/
private String awsAccountId;
/**
*
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services account.
*
*/
private String dataSourceId;
/**
*
* A display name for the data source.
*
*/
private String name;
/**
*
* The parameters that Amazon QuickSight uses to connect to your underlying source.
*
*/
private DataSourceParameters dataSourceParameters;
/**
*
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*
*/
private DataSourceCredentials credentials;
/**
*
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*
*/
private VpcConnectionProperties vpcConnectionProperties;
/**
*
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
*
*/
private SslProperties sslProperties;
/**
*
* The Amazon Web Services account ID.
*
*
* @param awsAccountId
* The Amazon Web Services account ID.
*/
public void setAwsAccountId(String awsAccountId) {
this.awsAccountId = awsAccountId;
}
/**
*
* The Amazon Web Services account ID.
*
*
* @return The Amazon Web Services account ID.
*/
public String getAwsAccountId() {
return this.awsAccountId;
}
/**
*
* The Amazon Web Services account ID.
*
*
* @param awsAccountId
* The Amazon Web Services account ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withAwsAccountId(String awsAccountId) {
setAwsAccountId(awsAccountId);
return this;
}
/**
*
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services account.
*
*
* @param dataSourceId
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services
* account.
*/
public void setDataSourceId(String dataSourceId) {
this.dataSourceId = dataSourceId;
}
/**
*
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services account.
*
*
* @return The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services
* account.
*/
public String getDataSourceId() {
return this.dataSourceId;
}
/**
*
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services account.
*
*
* @param dataSourceId
* The ID of the data source. This ID is unique per Amazon Web Services Region for each Amazon Web Services
* account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withDataSourceId(String dataSourceId) {
setDataSourceId(dataSourceId);
return this;
}
/**
*
* A display name for the data source.
*
*
* @param name
* A display name for the data source.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A display name for the data source.
*
*
* @return A display name for the data source.
*/
public String getName() {
return this.name;
}
/**
*
* A display name for the data source.
*
*
* @param name
* A display name for the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The parameters that Amazon QuickSight uses to connect to your underlying source.
*
*
* @param dataSourceParameters
* The parameters that Amazon QuickSight uses to connect to your underlying source.
*/
public void setDataSourceParameters(DataSourceParameters dataSourceParameters) {
this.dataSourceParameters = dataSourceParameters;
}
/**
*
* The parameters that Amazon QuickSight uses to connect to your underlying source.
*
*
* @return The parameters that Amazon QuickSight uses to connect to your underlying source.
*/
public DataSourceParameters getDataSourceParameters() {
return this.dataSourceParameters;
}
/**
*
* The parameters that Amazon QuickSight uses to connect to your underlying source.
*
*
* @param dataSourceParameters
* The parameters that Amazon QuickSight uses to connect to your underlying source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withDataSourceParameters(DataSourceParameters dataSourceParameters) {
setDataSourceParameters(dataSourceParameters);
return this;
}
/**
*
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*
*
* @param credentials
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*/
public void setCredentials(DataSourceCredentials credentials) {
this.credentials = credentials;
}
/**
*
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*
*
* @return The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*/
public DataSourceCredentials getCredentials() {
return this.credentials;
}
/**
*
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
*
*
* @param credentials
* The credentials that Amazon QuickSight that uses to connect to your underlying source. Currently, only
* credentials based on user name and password are supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withCredentials(DataSourceCredentials credentials) {
setCredentials(credentials);
return this;
}
/**
*
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*
*
* @param vpcConnectionProperties
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*/
public void setVpcConnectionProperties(VpcConnectionProperties vpcConnectionProperties) {
this.vpcConnectionProperties = vpcConnectionProperties;
}
/**
*
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*
*
* @return Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*/
public VpcConnectionProperties getVpcConnectionProperties() {
return this.vpcConnectionProperties;
}
/**
*
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
*
*
* @param vpcConnectionProperties
* Use this parameter only when you want Amazon QuickSight to use a VPC connection when connecting to your
* underlying source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withVpcConnectionProperties(VpcConnectionProperties vpcConnectionProperties) {
setVpcConnectionProperties(vpcConnectionProperties);
return this;
}
/**
*
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
*
*
* @param sslProperties
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
*/
public void setSslProperties(SslProperties sslProperties) {
this.sslProperties = sslProperties;
}
/**
*
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
*
*
* @return Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying
* source.
*/
public SslProperties getSslProperties() {
return this.sslProperties;
}
/**
*
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
*
*
* @param sslProperties
* Secure Socket Layer (SSL) properties that apply when Amazon QuickSight connects to your underlying source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDataSourceRequest withSslProperties(SslProperties sslProperties) {
setSslProperties(sslProperties);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAwsAccountId() != null)
sb.append("AwsAccountId: ").append(getAwsAccountId()).append(",");
if (getDataSourceId() != null)
sb.append("DataSourceId: ").append(getDataSourceId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDataSourceParameters() != null)
sb.append("DataSourceParameters: ").append(getDataSourceParameters()).append(",");
if (getCredentials() != null)
sb.append("Credentials: ").append("***Sensitive Data Redacted***").append(",");
if (getVpcConnectionProperties() != null)
sb.append("VpcConnectionProperties: ").append(getVpcConnectionProperties()).append(",");
if (getSslProperties() != null)
sb.append("SslProperties: ").append(getSslProperties());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateDataSourceRequest == false)
return false;
UpdateDataSourceRequest other = (UpdateDataSourceRequest) obj;
if (other.getAwsAccountId() == null ^ this.getAwsAccountId() == null)
return false;
if (other.getAwsAccountId() != null && other.getAwsAccountId().equals(this.getAwsAccountId()) == false)
return false;
if (other.getDataSourceId() == null ^ this.getDataSourceId() == null)
return false;
if (other.getDataSourceId() != null && other.getDataSourceId().equals(this.getDataSourceId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDataSourceParameters() == null ^ this.getDataSourceParameters() == null)
return false;
if (other.getDataSourceParameters() != null && other.getDataSourceParameters().equals(this.getDataSourceParameters()) == false)
return false;
if (other.getCredentials() == null ^ this.getCredentials() == null)
return false;
if (other.getCredentials() != null && other.getCredentials().equals(this.getCredentials()) == false)
return false;
if (other.getVpcConnectionProperties() == null ^ this.getVpcConnectionProperties() == null)
return false;
if (other.getVpcConnectionProperties() != null && other.getVpcConnectionProperties().equals(this.getVpcConnectionProperties()) == false)
return false;
if (other.getSslProperties() == null ^ this.getSslProperties() == null)
return false;
if (other.getSslProperties() != null && other.getSslProperties().equals(this.getSslProperties()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAwsAccountId() == null) ? 0 : getAwsAccountId().hashCode());
hashCode = prime * hashCode + ((getDataSourceId() == null) ? 0 : getDataSourceId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDataSourceParameters() == null) ? 0 : getDataSourceParameters().hashCode());
hashCode = prime * hashCode + ((getCredentials() == null) ? 0 : getCredentials().hashCode());
hashCode = prime * hashCode + ((getVpcConnectionProperties() == null) ? 0 : getVpcConnectionProperties().hashCode());
hashCode = prime * hashCode + ((getSslProperties() == null) ? 0 : getSslProperties().hashCode());
return hashCode;
}
@Override
public UpdateDataSourceRequest clone() {
return (UpdateDataSourceRequest) super.clone();
}
}