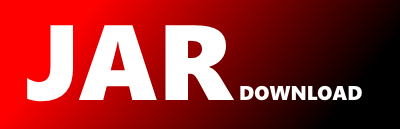
com.amazonaws.services.ram.AWSRAM Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.ram.model.*;
/**
* Interface for accessing RAM.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ram.AbstractAWSRAM} instead.
*
*
*
* This is the Resource Access Manager API Reference. This documentation provides descriptions and syntax for
* each of the actions and data types in RAM. RAM is a service that helps you securely share your Amazon Web Services
* resources to other Amazon Web Services accounts. If you use Organizations to manage your accounts, then you can share
* your resources with your entire organization or to organizational units (OUs). For supported resource types, you can
* also share resources with individual Identity and Access Management (IAM) roles and users.
*
*
* To learn more about RAM, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSRAM {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "ram";
/**
*
* Accepts an invitation to a resource share from another Amazon Web Services account. After you accept the
* invitation, the resources included in the resource share are available to interact with in the relevant Amazon
* Web Services Management Consoles and tools.
*
*
* @param acceptResourceShareInvitationRequest
* @return Result of the AcceptResourceShareInvitation operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ResourceShareInvitationArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) for an invitation was not found.
* @throws ResourceShareInvitationAlreadyAcceptedException
* The operation failed because the specified invitation was already accepted.
* @throws ResourceShareInvitationAlreadyRejectedException
* The operation failed because the specified invitation was already rejected.
* @throws ResourceShareInvitationExpiredException
* The operation failed because the specified invitation is past its expiration date and time.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.AcceptResourceShareInvitation
* @see AWS API Documentation
*/
AcceptResourceShareInvitationResult acceptResourceShareInvitation(AcceptResourceShareInvitationRequest acceptResourceShareInvitationRequest);
/**
*
* Adds the specified list of principals and list of resources to a resource share. Principals that already have
* access to this resource share immediately receive access to the added resources. Newly added principals
* immediately receive access to the resources shared in this resource share.
*
*
* @param associateResourceShareRequest
* @return Result of the AssociateResourceShare operation returned by the service.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws ResourceShareLimitExceededException
* The operation failed because it would exceed the limit for resource shares for your account. To view the
* limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws ThrottlingException
* The operation failed because it exceeded the rate at which you are allowed to perform this operation.
* Please try again later.
* @sample AWSRAM.AssociateResourceShare
* @see AWS API
* Documentation
*/
AssociateResourceShareResult associateResourceShare(AssociateResourceShareRequest associateResourceShareRequest);
/**
*
* Adds or replaces the RAM permission for a resource type included in a resource share. You can have exactly one
* permission associated with each resource type in the resource share. You can add a new RAM permission only if
* there are currently no resources of that resource type currently in the resource share.
*
*
* @param associateResourceSharePermissionRequest
* @return Result of the AssociateResourceSharePermission operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @sample AWSRAM.AssociateResourceSharePermission
* @see AWS API Documentation
*/
AssociateResourceSharePermissionResult associateResourceSharePermission(AssociateResourceSharePermissionRequest associateResourceSharePermissionRequest);
/**
*
* Creates a customer managed permission for a specified resource type that you can attach to resource shares. It is
* created in the Amazon Web Services Region in which you call the operation.
*
*
* @param createPermissionRequest
* @return Result of the CreatePermission operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws InvalidPolicyException
* The operation failed because a policy you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws PermissionAlreadyExistsException
* The operation failed because a permission with the specified name already exists in the requested Amazon
* Web Services Region. Choose a different name.
* @throws MalformedPolicyTemplateException
* The operation failed because the policy template that you provided isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws PermissionLimitExceededException
* The operation failed because it would exceed the maximum number of permissions you can create in each
* Amazon Web Services Region. To view the limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.CreatePermission
* @see AWS API
* Documentation
*/
CreatePermissionResult createPermission(CreatePermissionRequest createPermissionRequest);
/**
*
* Creates a new version of the specified customer managed permission. The new version is automatically set as the
* default version of the customer managed permission. New resource shares automatically use the default permission.
* Existing resource shares continue to use their original permission versions, but you can use
* ReplacePermissionAssociations to update them.
*
*
* If the specified customer managed permission already has the maximum of 5 versions, then you must delete one of
* the existing versions before you can create a new one.
*
*
* @param createPermissionVersionRequest
* @return Result of the CreatePermissionVersion operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws InvalidPolicyException
* The operation failed because a policy you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedPolicyTemplateException
* The operation failed because the policy template that you provided isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws PermissionVersionsLimitExceededException
* The operation failed because it would exceed the limit for the number of versions you can have for a
* permission. To view the limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @sample AWSRAM.CreatePermissionVersion
* @see AWS
* API Documentation
*/
CreatePermissionVersionResult createPermissionVersion(CreatePermissionVersionRequest createPermissionVersionRequest);
/**
*
* Creates a resource share. You can provide a list of the Amazon Resource Names
* (ARNs) for the resources that you want to share, a list of principals you want to share the resources with,
* and the permissions to grant those principals.
*
*
*
* Sharing a resource makes it available for use by principals outside of the Amazon Web Services account that
* created the resource. Sharing doesn't change any permissions or quotas that apply to the resource in the account
* that created it.
*
*
*
* @param createResourceShareRequest
* @return Result of the CreateResourceShare operation returned by the service.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ResourceShareLimitExceededException
* The operation failed because it would exceed the limit for resource shares for your account. To view the
* limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @throws TagPolicyViolationException
* The operation failed because the specified tag key is a reserved word and can't be used.
* @throws TagLimitExceededException
* The operation failed because it would exceed the limit for tags for your Amazon Web Services account.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.CreateResourceShare
* @see AWS API
* Documentation
*/
CreateResourceShareResult createResourceShare(CreateResourceShareRequest createResourceShareRequest);
/**
*
* Deletes the specified customer managed permission in the Amazon Web Services Region in which you call this
* operation. You can delete a customer managed permission only if it isn't attached to any resource share. The
* operation deletes all versions associated with the customer managed permission.
*
*
* @param deletePermissionRequest
* @return Result of the DeletePermission operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.DeletePermission
* @see AWS API
* Documentation
*/
DeletePermissionResult deletePermission(DeletePermissionRequest deletePermissionRequest);
/**
*
* Deletes one version of a customer managed permission. The version you specify must not be attached to any
* resource share and must not be the default version for the permission.
*
*
* If a customer managed permission has the maximum of 5 versions, then you must delete at least one version before
* you can create another.
*
*
* @param deletePermissionVersionRequest
* @return Result of the DeletePermissionVersion operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.DeletePermissionVersion
* @see AWS
* API Documentation
*/
DeletePermissionVersionResult deletePermissionVersion(DeletePermissionVersionRequest deletePermissionVersionRequest);
/**
*
* Deletes the specified resource share.
*
*
*
* This doesn't delete any of the resources that were associated with the resource share; it only stops the sharing
* of those resources through this resource share.
*
*
*
* @param deleteResourceShareRequest
* @return Result of the DeleteResourceShare operation returned by the service.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.DeleteResourceShare
* @see AWS API
* Documentation
*/
DeleteResourceShareResult deleteResourceShare(DeleteResourceShareRequest deleteResourceShareRequest);
/**
*
* Removes the specified principals or resources from participating in the specified resource share.
*
*
* @param disassociateResourceShareRequest
* @return Result of the DisassociateResourceShare operation returned by the service.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws ResourceShareLimitExceededException
* The operation failed because it would exceed the limit for resource shares for your account. To view the
* limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @sample AWSRAM.DisassociateResourceShare
* @see AWS
* API Documentation
*/
DisassociateResourceShareResult disassociateResourceShare(DisassociateResourceShareRequest disassociateResourceShareRequest);
/**
*
* Removes a managed permission from a resource share. Permission changes take effect immediately. You can remove a
* managed permission from a resource share only if there are currently no resources of the relevant resource type
* currently attached to the resource share.
*
*
* @param disassociateResourceSharePermissionRequest
* @return Result of the DisassociateResourceSharePermission operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @sample AWSRAM.DisassociateResourceSharePermission
* @see AWS API Documentation
*/
DisassociateResourceSharePermissionResult disassociateResourceSharePermission(
DisassociateResourceSharePermissionRequest disassociateResourceSharePermissionRequest);
/**
*
* Enables resource sharing within your organization in Organizations. This operation creates a service-linked role
* called AWSServiceRoleForResourceAccessManager
that has the IAM managed policy named
* AWSResourceAccessManagerServiceRolePolicy attached. This role permits RAM to retrieve information about the
* organization and its structure. This lets you share resources with all of the accounts in the calling account's
* organization by specifying the organization ID, or all of the accounts in an organizational unit (OU) by
* specifying the OU ID. Until you enable sharing within the organization, you can specify only individual Amazon
* Web Services accounts, or for supported resource types, IAM roles and users.
*
*
* You must call this operation from an IAM role or user in the organization's management account.
*
*
*
* @param enableSharingWithAwsOrganizationRequest
* @return Result of the EnableSharingWithAwsOrganization operation returned by the service.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.EnableSharingWithAwsOrganization
* @see AWS API Documentation
*/
EnableSharingWithAwsOrganizationResult enableSharingWithAwsOrganization(EnableSharingWithAwsOrganizationRequest enableSharingWithAwsOrganizationRequest);
/**
*
* Retrieves the contents of a managed permission in JSON format.
*
*
* @param getPermissionRequest
* @return Result of the GetPermission operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @sample AWSRAM.GetPermission
* @see AWS API
* Documentation
*/
GetPermissionResult getPermission(GetPermissionRequest getPermissionRequest);
/**
*
* Retrieves the resource policies for the specified resources that you own and have shared.
*
*
* @param getResourcePoliciesRequest
* @return Result of the GetResourcePolicies operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ResourceArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) was not found.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.GetResourcePolicies
* @see AWS API
* Documentation
*/
GetResourcePoliciesResult getResourcePolicies(GetResourcePoliciesRequest getResourcePoliciesRequest);
/**
*
* Retrieves the lists of resources and principals that associated for resource shares that you own.
*
*
* @param getResourceShareAssociationsRequest
* @return Result of the GetResourceShareAssociations operation returned by the service.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.GetResourceShareAssociations
* @see AWS API Documentation
*/
GetResourceShareAssociationsResult getResourceShareAssociations(GetResourceShareAssociationsRequest getResourceShareAssociationsRequest);
/**
*
* Retrieves details about invitations that you have received for resource shares.
*
*
* @param getResourceShareInvitationsRequest
* @return Result of the GetResourceShareInvitations operation returned by the service.
* @throws ResourceShareInvitationArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) for an invitation was not found.
* @throws InvalidMaxResultsException
* The operation failed because the specified value for MaxResults
isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.GetResourceShareInvitations
* @see AWS API Documentation
*/
GetResourceShareInvitationsResult getResourceShareInvitations(GetResourceShareInvitationsRequest getResourceShareInvitationsRequest);
/**
*
* Retrieves details about the resource shares that you own or that are shared with you.
*
*
* @param getResourceSharesRequest
* @return Result of the GetResourceShares operation returned by the service.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.GetResourceShares
* @see AWS API
* Documentation
*/
GetResourceSharesResult getResourceShares(GetResourceSharesRequest getResourceSharesRequest);
/**
*
* Lists the resources in a resource share that is shared with you but for which the invitation is still
* PENDING
. That means that you haven't accepted or rejected the invitation and the invitation hasn't
* expired.
*
*
* @param listPendingInvitationResourcesRequest
* @return Result of the ListPendingInvitationResources operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws ResourceShareInvitationArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) for an invitation was not found.
* @throws MissingRequiredParameterException
* The operation failed because a required input parameter is missing.
* @throws ResourceShareInvitationAlreadyRejectedException
* The operation failed because the specified invitation was already rejected.
* @throws ResourceShareInvitationExpiredException
* The operation failed because the specified invitation is past its expiration date and time.
* @sample AWSRAM.ListPendingInvitationResources
* @see AWS API Documentation
*/
ListPendingInvitationResourcesResult listPendingInvitationResources(ListPendingInvitationResourcesRequest listPendingInvitationResourcesRequest);
/**
*
* Lists information about the managed permission and its associations to any resource shares that use this managed
* permission. This lets you see which resource shares use which versions of the specified managed permission.
*
*
* @param listPermissionAssociationsRequest
* @return Result of the ListPermissionAssociations operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.ListPermissionAssociations
* @see AWS
* API Documentation
*/
ListPermissionAssociationsResult listPermissionAssociations(ListPermissionAssociationsRequest listPermissionAssociationsRequest);
/**
*
* Lists the available versions of the specified RAM permission.
*
*
* @param listPermissionVersionsRequest
* @return Result of the ListPermissionVersions operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @sample AWSRAM.ListPermissionVersions
* @see AWS API
* Documentation
*/
ListPermissionVersionsResult listPermissionVersions(ListPermissionVersionsRequest listPermissionVersionsRequest);
/**
*
* Retrieves a list of available RAM permissions that you can use for the supported resource types.
*
*
* @param listPermissionsRequest
* @return Result of the ListPermissions operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @sample AWSRAM.ListPermissions
* @see AWS API
* Documentation
*/
ListPermissionsResult listPermissions(ListPermissionsRequest listPermissionsRequest);
/**
*
* Lists the principals that you are sharing resources with or that are sharing resources with you.
*
*
* @param listPrincipalsRequest
* @return Result of the ListPrincipals operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.ListPrincipals
* @see AWS API
* Documentation
*/
ListPrincipalsResult listPrincipals(ListPrincipalsRequest listPrincipalsRequest);
/**
*
* Retrieves the current status of the asynchronous tasks performed by RAM when you perform the
* ReplacePermissionAssociationsWork operation.
*
*
* @param listReplacePermissionAssociationsWorkRequest
* @return Result of the ListReplacePermissionAssociationsWork operation returned by the service.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @sample AWSRAM.ListReplacePermissionAssociationsWork
* @see AWS API Documentation
*/
ListReplacePermissionAssociationsWorkResult listReplacePermissionAssociationsWork(
ListReplacePermissionAssociationsWorkRequest listReplacePermissionAssociationsWorkRequest);
/**
*
* Lists the RAM permissions that are associated with a resource share.
*
*
* @param listResourceSharePermissionsRequest
* @return Result of the ListResourceSharePermissions operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @sample AWSRAM.ListResourceSharePermissions
* @see AWS API Documentation
*/
ListResourceSharePermissionsResult listResourceSharePermissions(ListResourceSharePermissionsRequest listResourceSharePermissionsRequest);
/**
*
* Lists the resource types that can be shared by RAM.
*
*
* @param listResourceTypesRequest
* @return Result of the ListResourceTypes operation returned by the service.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.ListResourceTypes
* @see AWS API
* Documentation
*/
ListResourceTypesResult listResourceTypes(ListResourceTypesRequest listResourceTypesRequest);
/**
*
* Lists the resources that you added to a resource share or the resources that are shared with you.
*
*
* @param listResourcesRequest
* @return Result of the ListResources operation returned by the service.
* @throws InvalidResourceTypeException
* The operation failed because the specified resource type isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidNextTokenException
* The operation failed because the specified value for NextToken
isn't valid. You must specify
* a value you received in the NextToken
response of a previous call to this operation.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.ListResources
* @see AWS API
* Documentation
*/
ListResourcesResult listResources(ListResourcesRequest listResourcesRequest);
/**
*
* When you attach a resource-based policy to a resource, RAM automatically creates a resource share of
* featureSet
=CREATED_FROM_POLICY
with a managed permission that has the same IAM
* permissions as the original resource-based policy. However, this type of managed permission is visible to only
* the resource share owner, and the associated resource share can't be modified by using RAM.
*
*
* This operation creates a separate, fully manageable customer managed permission that has the same IAM permissions
* as the original resource-based policy. You can associate this customer managed permission to any resource shares.
*
*
* Before you use PromoteResourceShareCreatedFromPolicy, you should first run this operation to ensure that
* you have an appropriate customer managed permission that can be associated with the promoted resource share.
*
*
*
* -
*
* The original CREATED_FROM_POLICY
policy isn't deleted, and resource shares using that original
* policy aren't automatically updated.
*
*
* -
*
* You can't modify a CREATED_FROM_POLICY
resource share so you can't associate the new customer
* managed permission by using ReplacePermsissionAssociations
. However, if you use
* PromoteResourceShareCreatedFromPolicy, that operation automatically associates the fully manageable
* customer managed permission to the newly promoted STANDARD
resource share.
*
*
* -
*
* After you promote a resource share, if the original CREATED_FROM_POLICY
managed permission has no
* other associations to A resource share, then RAM automatically deletes it.
*
*
*
*
*
* @param promotePermissionCreatedFromPolicyRequest
* @return Result of the PromotePermissionCreatedFromPolicy operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MissingRequiredParameterException
* The operation failed because a required input parameter is missing.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @sample AWSRAM.PromotePermissionCreatedFromPolicy
* @see AWS API Documentation
*/
PromotePermissionCreatedFromPolicyResult promotePermissionCreatedFromPolicy(
PromotePermissionCreatedFromPolicyRequest promotePermissionCreatedFromPolicyRequest);
/**
*
* When you attach a resource-based policy to a resource, RAM automatically creates a resource share of
* featureSet
=CREATED_FROM_POLICY
with a managed permission that has the same IAM
* permissions as the original resource-based policy. However, this type of managed permission is visible to only
* the resource share owner, and the associated resource share can't be modified by using RAM.
*
*
* This operation promotes the resource share to a STANDARD
resource share that is fully manageable in
* RAM. When you promote a resource share, you can then manage the resource share in RAM and it becomes visible to
* all of the principals you shared it with.
*
*
*
* Before you perform this operation, you should first run PromotePermissionCreatedFromPolicyto ensure that
* you have an appropriate customer managed permission that can be associated with this resource share after its is
* promoted. If this operation can't find a managed permission that exactly matches the existing
* CREATED_FROM_POLICY
permission, then this operation fails.
*
*
*
* @param promoteResourceShareCreatedFromPolicyRequest
* @return Result of the PromoteResourceShareCreatedFromPolicy operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws ResourceShareLimitExceededException
* The operation failed because it would exceed the limit for resource shares for your account. To view the
* limits for your Amazon Web Services account, see the RAM page in the Service
* Quotas console.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MissingRequiredParameterException
* The operation failed because a required input parameter is missing.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidStateTransitionException
* The operation failed because the requested operation isn't valid for the resource share in its current
* state.
* @throws UnmatchedPolicyPermissionException
* There isn't an existing managed permission defined in RAM that has the same IAM permissions as the
* resource-based policy attached to the resource. You should first run
* PromotePermissionCreatedFromPolicy to create that managed permission.
* @sample AWSRAM.PromoteResourceShareCreatedFromPolicy
* @see AWS API Documentation
*/
PromoteResourceShareCreatedFromPolicyResult promoteResourceShareCreatedFromPolicy(
PromoteResourceShareCreatedFromPolicyRequest promoteResourceShareCreatedFromPolicyRequest);
/**
*
* Rejects an invitation to a resource share from another Amazon Web Services account.
*
*
* @param rejectResourceShareInvitationRequest
* @return Result of the RejectResourceShareInvitation operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ResourceShareInvitationArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) for an invitation was not found.
* @throws ResourceShareInvitationAlreadyAcceptedException
* The operation failed because the specified invitation was already accepted.
* @throws ResourceShareInvitationAlreadyRejectedException
* The operation failed because the specified invitation was already rejected.
* @throws ResourceShareInvitationExpiredException
* The operation failed because the specified invitation is past its expiration date and time.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.RejectResourceShareInvitation
* @see AWS API Documentation
*/
RejectResourceShareInvitationResult rejectResourceShareInvitation(RejectResourceShareInvitationRequest rejectResourceShareInvitationRequest);
/**
*
* Updates all resource shares that use a managed permission to a different managed permission. This operation
* always applies the default version of the target managed permission. You can optionally specify that the update
* applies to only resource shares that currently use a specified version. This enables you to update to the latest
* version, without changing the which managed permission is used.
*
*
* You can use this operation to update all of your resource shares to use the current default version of the
* permission by specifying the same value for the fromPermissionArn
and toPermissionArn
* parameters.
*
*
* You can use the optional fromPermissionVersion
parameter to update only those resources that use a
* specified version of the managed permission to the new managed permission.
*
*
*
* To successfully perform this operation, you must have permission to update the resource-based policy on all
* affected resource types.
*
*
*
* @param replacePermissionAssociationsRequest
* @return Result of the ReplacePermissionAssociations operation returned by the service.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.ReplacePermissionAssociations
* @see AWS API Documentation
*/
ReplacePermissionAssociationsResult replacePermissionAssociations(ReplacePermissionAssociationsRequest replacePermissionAssociationsRequest);
/**
*
* Designates the specified version number as the default version for the specified customer managed permission. New
* resource shares automatically use this new default permission. Existing resource shares continue to use their
* original permission version, but you can use ReplacePermissionAssociations to update them.
*
*
* @param setDefaultPermissionVersionRequest
* @return Result of the SetDefaultPermissionVersion operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @sample AWSRAM.SetDefaultPermissionVersion
* @see AWS API Documentation
*/
SetDefaultPermissionVersionResult setDefaultPermissionVersion(SetDefaultPermissionVersionRequest setDefaultPermissionVersionRequest);
/**
*
* Adds the specified tag keys and values to a resource share or managed permission. If you choose a resource share,
* the tags are attached to only the resource share, not to the resources that are in the resource share.
*
*
* The tags on a managed permission are the same for all versions of the managed permission.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws TagLimitExceededException
* The operation failed because it would exceed the limit for tags for your Amazon Web Services account.
* @throws ResourceArnNotFoundException
* The operation failed because the specified Amazon Resource Name
* (ARN) was not found.
* @throws TagPolicyViolationException
* The operation failed because the specified tag key is a reserved word and can't be used.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes the specified tag key and value pairs from the specified resource share or managed permission.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Modifies some of the properties of the specified resource share.
*
*
* @param updateResourceShareRequest
* @return Result of the UpdateResourceShare operation returned by the service.
* @throws IdempotentParameterMismatchException
* The operation failed because the client token input parameter matched one that was used with a previous
* call to the operation, but at least one of the other input parameters is different from the previous
* call.
* @throws MissingRequiredParameterException
* The operation failed because a required input parameter is missing.
* @throws UnknownResourceException
* The operation failed because a specified resource couldn't be found.
* @throws MalformedArnException
* The operation failed because the specified Amazon Resource Name
* (ARN) has a format that isn't valid.
* @throws InvalidClientTokenException
* The operation failed because the specified client token isn't valid.
* @throws InvalidParameterException
* The operation failed because a parameter you specified isn't valid.
* @throws OperationNotPermittedException
* The operation failed because the requested operation isn't permitted.
* @throws ServerInternalException
* The operation failed because the service could not respond to the request due to an internal problem. Try
* again later.
* @throws ServiceUnavailableException
* The operation failed because the service isn't available. Try again later.
* @sample AWSRAM.UpdateResourceShare
* @see AWS API
* Documentation
*/
UpdateResourceShareResult updateResourceShare(UpdateResourceShareRequest updateResourceShareRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}