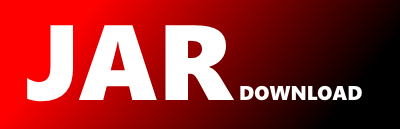
com.amazonaws.services.ram.model.AssociateResourceSharePermissionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AssociateResourceSharePermissionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*
*/
private String resourceShareArn;
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM console and
* then choose the name of the permission. The ARN is displayed on the detail page.
*
*/
private String permissionArn;
/**
*
* Specifies whether the specified permission should replace the existing permission associated with the resource
* share. Use true
to replace the current permissions. Use false
to add the permission to
* a resource share that currently doesn't have a permission. The default value is false
.
*
*
*
* A resource share can have only one permission per resource type. If a resource share already has a permission for
* the specified resource type and you don't set replace
to true
then the operation
* returns an error. This helps prevent accidental overwriting of a permission.
*
*
*/
private Boolean replace;
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*/
private String clientToken;
/**
*
* Specifies the version of the RAM permission to associate with the resource share. You can specify only the
* version that is currently set as the default version for the permission. If you also set the replace
* pararameter to true
, then this operation updates an outdated version of the permission to the
* current default version.
*
*
*
* You don't need to specify this parameter because the default behavior is to use the version that is currently set
* as the default version for the permission. This parameter is supported for backwards compatibility.
*
*
*/
private Integer permissionVersion;
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*
*
* @param resourceShareArn
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*/
public void setResourceShareArn(String resourceShareArn) {
this.resourceShareArn = resourceShareArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*
*
* @return Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*/
public String getResourceShareArn() {
return this.resourceShareArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
*
*
* @param resourceShareArn
* Specifies the Amazon
* Resource Name (ARN) of the resource share to which you want to add or replace permissions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceSharePermissionRequest withResourceShareArn(String resourceShareArn) {
setResourceShareArn(resourceShareArn);
return this;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM console and
* then choose the name of the permission. The ARN is displayed on the detail page.
*
*
* @param permissionArn
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM
* console and then choose the name of the permission. The ARN is displayed on the detail page.
*/
public void setPermissionArn(String permissionArn) {
this.permissionArn = permissionArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM console and
* then choose the name of the permission. The ARN is displayed on the detail page.
*
*
* @return Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM
* console and then choose the name of the permission. The ARN is displayed on the detail page.
*/
public String getPermissionArn() {
return this.permissionArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM console and
* then choose the name of the permission. The ARN is displayed on the detail page.
*
*
* @param permissionArn
* Specifies the Amazon
* Resource Name (ARN) of the RAM permission to associate with the resource share. To find the ARN for a
* permission, use either the ListPermissions operation or go to the Permissions library page in the RAM
* console and then choose the name of the permission. The ARN is displayed on the detail page.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceSharePermissionRequest withPermissionArn(String permissionArn) {
setPermissionArn(permissionArn);
return this;
}
/**
*
* Specifies whether the specified permission should replace the existing permission associated with the resource
* share. Use true
to replace the current permissions. Use false
to add the permission to
* a resource share that currently doesn't have a permission. The default value is false
.
*
*
*
* A resource share can have only one permission per resource type. If a resource share already has a permission for
* the specified resource type and you don't set replace
to true
then the operation
* returns an error. This helps prevent accidental overwriting of a permission.
*
*
*
* @param replace
* Specifies whether the specified permission should replace the existing permission associated with the
* resource share. Use true
to replace the current permissions. Use false
to add
* the permission to a resource share that currently doesn't have a permission. The default value is
* false
.
*
* A resource share can have only one permission per resource type. If a resource share already has a
* permission for the specified resource type and you don't set replace
to true
* then the operation returns an error. This helps prevent accidental overwriting of a permission.
*
*/
public void setReplace(Boolean replace) {
this.replace = replace;
}
/**
*
* Specifies whether the specified permission should replace the existing permission associated with the resource
* share. Use true
to replace the current permissions. Use false
to add the permission to
* a resource share that currently doesn't have a permission. The default value is false
.
*
*
*
* A resource share can have only one permission per resource type. If a resource share already has a permission for
* the specified resource type and you don't set replace
to true
then the operation
* returns an error. This helps prevent accidental overwriting of a permission.
*
*
*
* @return Specifies whether the specified permission should replace the existing permission associated with the
* resource share. Use true
to replace the current permissions. Use false
to add
* the permission to a resource share that currently doesn't have a permission. The default value is
* false
.
*
* A resource share can have only one permission per resource type. If a resource share already has a
* permission for the specified resource type and you don't set replace
to true
* then the operation returns an error. This helps prevent accidental overwriting of a permission.
*
*/
public Boolean getReplace() {
return this.replace;
}
/**
*
* Specifies whether the specified permission should replace the existing permission associated with the resource
* share. Use true
to replace the current permissions. Use false
to add the permission to
* a resource share that currently doesn't have a permission. The default value is false
.
*
*
*
* A resource share can have only one permission per resource type. If a resource share already has a permission for
* the specified resource type and you don't set replace
to true
then the operation
* returns an error. This helps prevent accidental overwriting of a permission.
*
*
*
* @param replace
* Specifies whether the specified permission should replace the existing permission associated with the
* resource share. Use true
to replace the current permissions. Use false
to add
* the permission to a resource share that currently doesn't have a permission. The default value is
* false
.
*
* A resource share can have only one permission per resource type. If a resource share already has a
* permission for the specified resource type and you don't set replace
to true
* then the operation returns an error. This helps prevent accidental overwriting of a permission.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceSharePermissionRequest withReplace(Boolean replace) {
setReplace(replace);
return this;
}
/**
*
* Specifies whether the specified permission should replace the existing permission associated with the resource
* share. Use true
to replace the current permissions. Use false
to add the permission to
* a resource share that currently doesn't have a permission. The default value is false
.
*
*
*
* A resource share can have only one permission per resource type. If a resource share already has a permission for
* the specified resource type and you don't set replace
to true
then the operation
* returns an error. This helps prevent accidental overwriting of a permission.
*
*
*
* @return Specifies whether the specified permission should replace the existing permission associated with the
* resource share. Use true
to replace the current permissions. Use false
to add
* the permission to a resource share that currently doesn't have a permission. The default value is
* false
.
*
* A resource share can have only one permission per resource type. If a resource share already has a
* permission for the specified resource type and you don't set replace
to true
* then the operation returns an error. This helps prevent accidental overwriting of a permission.
*
*/
public Boolean isReplace() {
return this.replace;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @param clientToken
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @return Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @param clientToken
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceSharePermissionRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Specifies the version of the RAM permission to associate with the resource share. You can specify only the
* version that is currently set as the default version for the permission. If you also set the replace
* pararameter to true
, then this operation updates an outdated version of the permission to the
* current default version.
*
*
*
* You don't need to specify this parameter because the default behavior is to use the version that is currently set
* as the default version for the permission. This parameter is supported for backwards compatibility.
*
*
*
* @param permissionVersion
* Specifies the version of the RAM permission to associate with the resource share. You can specify
* only the version that is currently set as the default version for the permission. If you also set
* the replace
pararameter to true
, then this operation updates an outdated version
* of the permission to the current default version.
*
* You don't need to specify this parameter because the default behavior is to use the version that is
* currently set as the default version for the permission. This parameter is supported for backwards
* compatibility.
*
*/
public void setPermissionVersion(Integer permissionVersion) {
this.permissionVersion = permissionVersion;
}
/**
*
* Specifies the version of the RAM permission to associate with the resource share. You can specify only the
* version that is currently set as the default version for the permission. If you also set the replace
* pararameter to true
, then this operation updates an outdated version of the permission to the
* current default version.
*
*
*
* You don't need to specify this parameter because the default behavior is to use the version that is currently set
* as the default version for the permission. This parameter is supported for backwards compatibility.
*
*
*
* @return Specifies the version of the RAM permission to associate with the resource share. You can specify
* only the version that is currently set as the default version for the permission. If you also set
* the replace
pararameter to true
, then this operation updates an outdated
* version of the permission to the current default version.
*
* You don't need to specify this parameter because the default behavior is to use the version that is
* currently set as the default version for the permission. This parameter is supported for backwards
* compatibility.
*
*/
public Integer getPermissionVersion() {
return this.permissionVersion;
}
/**
*
* Specifies the version of the RAM permission to associate with the resource share. You can specify only the
* version that is currently set as the default version for the permission. If you also set the replace
* pararameter to true
, then this operation updates an outdated version of the permission to the
* current default version.
*
*
*
* You don't need to specify this parameter because the default behavior is to use the version that is currently set
* as the default version for the permission. This parameter is supported for backwards compatibility.
*
*
*
* @param permissionVersion
* Specifies the version of the RAM permission to associate with the resource share. You can specify
* only the version that is currently set as the default version for the permission. If you also set
* the replace
pararameter to true
, then this operation updates an outdated version
* of the permission to the current default version.
*
* You don't need to specify this parameter because the default behavior is to use the version that is
* currently set as the default version for the permission. This parameter is supported for backwards
* compatibility.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceSharePermissionRequest withPermissionVersion(Integer permissionVersion) {
setPermissionVersion(permissionVersion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceShareArn() != null)
sb.append("ResourceShareArn: ").append(getResourceShareArn()).append(",");
if (getPermissionArn() != null)
sb.append("PermissionArn: ").append(getPermissionArn()).append(",");
if (getReplace() != null)
sb.append("Replace: ").append(getReplace()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getPermissionVersion() != null)
sb.append("PermissionVersion: ").append(getPermissionVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AssociateResourceSharePermissionRequest == false)
return false;
AssociateResourceSharePermissionRequest other = (AssociateResourceSharePermissionRequest) obj;
if (other.getResourceShareArn() == null ^ this.getResourceShareArn() == null)
return false;
if (other.getResourceShareArn() != null && other.getResourceShareArn().equals(this.getResourceShareArn()) == false)
return false;
if (other.getPermissionArn() == null ^ this.getPermissionArn() == null)
return false;
if (other.getPermissionArn() != null && other.getPermissionArn().equals(this.getPermissionArn()) == false)
return false;
if (other.getReplace() == null ^ this.getReplace() == null)
return false;
if (other.getReplace() != null && other.getReplace().equals(this.getReplace()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getPermissionVersion() == null ^ this.getPermissionVersion() == null)
return false;
if (other.getPermissionVersion() != null && other.getPermissionVersion().equals(this.getPermissionVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceShareArn() == null) ? 0 : getResourceShareArn().hashCode());
hashCode = prime * hashCode + ((getPermissionArn() == null) ? 0 : getPermissionArn().hashCode());
hashCode = prime * hashCode + ((getReplace() == null) ? 0 : getReplace().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getPermissionVersion() == null) ? 0 : getPermissionVersion().hashCode());
return hashCode;
}
@Override
public AssociateResourceSharePermissionRequest clone() {
return (AssociateResourceSharePermissionRequest) super.clone();
}
}