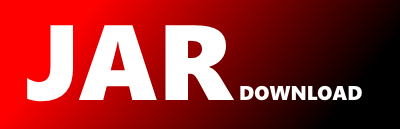
com.amazonaws.services.ram.model.ListPermissionsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListPermissionsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies that you want to list only those permissions that apply to the specified resource type. This parameter
* is not case sensitive.
*
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
. You can
* use the ListResourceTypes operation to get the specific string required.
*
*/
private String resourceType;
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*/
private String nextToken;
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*/
private Integer maxResults;
/**
*
* Specifies that you want to list only permissions of this type:
*
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
*
*/
private String permissionType;
/**
*
* Specifies that you want to list only those permissions that apply to the specified resource type. This parameter
* is not case sensitive.
*
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
. You can
* use the ListResourceTypes operation to get the specific string required.
*
*
* @param resourceType
* Specifies that you want to list only those permissions that apply to the specified resource type. This
* parameter is not case sensitive.
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
.
* You can use the ListResourceTypes operation to get the specific string required.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* Specifies that you want to list only those permissions that apply to the specified resource type. This parameter
* is not case sensitive.
*
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
. You can
* use the ListResourceTypes operation to get the specific string required.
*
*
* @return Specifies that you want to list only those permissions that apply to the specified resource type. This
* parameter is not case sensitive.
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
.
* You can use the ListResourceTypes operation to get the specific string required.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* Specifies that you want to list only those permissions that apply to the specified resource type. This parameter
* is not case sensitive.
*
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
. You can
* use the ListResourceTypes operation to get the specific string required.
*
*
* @param resourceType
* Specifies that you want to list only those permissions that apply to the specified resource type. This
* parameter is not case sensitive.
*
* For example, to list only permissions that apply to Amazon EC2 subnets, specify ec2:subnet
.
* You can use the ListResourceTypes operation to get the specific string required.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPermissionsRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @return Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
* response to request the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPermissionsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @return Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
* after every operation to ensure that you receive all of the results.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPermissionsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* Specifies that you want to list only permissions of this type:
*
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
*
*
* @param permissionType
* Specifies that you want to list only permissions of this type:
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
* @see PermissionTypeFilter
*/
public void setPermissionType(String permissionType) {
this.permissionType = permissionType;
}
/**
*
* Specifies that you want to list only permissions of this type:
*
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
*
*
* @return Specifies that you want to list only permissions of this type:
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
* @see PermissionTypeFilter
*/
public String getPermissionType() {
return this.permissionType;
}
/**
*
* Specifies that you want to list only permissions of this type:
*
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
*
*
* @param permissionType
* Specifies that you want to list only permissions of this type:
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionTypeFilter
*/
public ListPermissionsRequest withPermissionType(String permissionType) {
setPermissionType(permissionType);
return this;
}
/**
*
* Specifies that you want to list only permissions of this type:
*
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
*
*
* @param permissionType
* Specifies that you want to list only permissions of this type:
*
* -
*
* AWS
– returns only Amazon Web Services managed permissions.
*
*
* -
*
* LOCAL
– returns only customer managed permissions
*
*
* -
*
* ALL
– returns both Amazon Web Services managed permissions and customer managed permissions.
*
*
*
*
* If you don't specify this parameter, the default is All
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionTypeFilter
*/
public ListPermissionsRequest withPermissionType(PermissionTypeFilter permissionType) {
this.permissionType = permissionType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getPermissionType() != null)
sb.append("PermissionType: ").append(getPermissionType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListPermissionsRequest == false)
return false;
ListPermissionsRequest other = (ListPermissionsRequest) obj;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getPermissionType() == null ^ this.getPermissionType() == null)
return false;
if (other.getPermissionType() != null && other.getPermissionType().equals(this.getPermissionType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getPermissionType() == null) ? 0 : getPermissionType().hashCode());
return hashCode;
}
@Override
public ListPermissionsRequest clone() {
return (ListPermissionsRequest) super.clone();
}
}