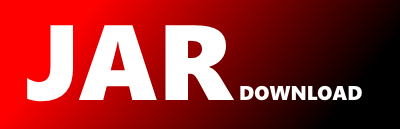
com.amazonaws.services.ram.model.ListPrincipalsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListPrincipalsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies that you want to list information for only resource shares that match the following:
*
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
*
*/
private String resourceOwner;
/**
*
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name (ARN).
*
*/
private String resourceArn;
/**
*
* Specifies that you want to list information for only the listed principals.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*/
private java.util.List principals;
/**
*
* Specifies that you want to list information for only principals associated with resource shares that include the
* specified resource type.
*
*
* For a list of valid values, query the ListResourceTypes operation.
*
*/
private String resourceType;
/**
*
* Specifies that you want to list information for only principals associated with the resource shares specified by
* a list the Amazon Resource
* Names (ARNs).
*
*/
private java.util.List resourceShareArns;
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*/
private String nextToken;
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*/
private Integer maxResults;
/**
*
* Specifies that you want to list information for only resource shares that match the following:
*
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to list information for only resource shares that match the following:
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
* @see ResourceOwner
*/
public void setResourceOwner(String resourceOwner) {
this.resourceOwner = resourceOwner;
}
/**
*
* Specifies that you want to list information for only resource shares that match the following:
*
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
*
*
* @return Specifies that you want to list information for only resource shares that match the following:
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
* @see ResourceOwner
*/
public String getResourceOwner() {
return this.resourceOwner;
}
/**
*
* Specifies that you want to list information for only resource shares that match the following:
*
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to list information for only resource shares that match the following:
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public ListPrincipalsRequest withResourceOwner(String resourceOwner) {
setResourceOwner(resourceOwner);
return this;
}
/**
*
* Specifies that you want to list information for only resource shares that match the following:
*
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to list information for only resource shares that match the following:
*
* -
*
* SELF
– principals that your account is sharing resources with
*
*
* -
*
* OTHER-ACCOUNTS
– principals that are sharing resources with your account
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public ListPrincipalsRequest withResourceOwner(ResourceOwner resourceOwner) {
this.resourceOwner = resourceOwner.toString();
return this;
}
/**
*
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name (ARN).
*
*
* @param resourceArn
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name
* (ARN).
*/
public void setResourceArn(String resourceArn) {
this.resourceArn = resourceArn;
}
/**
*
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name (ARN).
*
*
* @return Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name
* (ARN).
*/
public String getResourceArn() {
return this.resourceArn;
}
/**
*
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name (ARN).
*
*
* @param resourceArn
* Specifies that you want to list principal information for the resource share with the specified Amazon Resource Name
* (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withResourceArn(String resourceArn) {
setResourceArn(resourceArn);
return this;
}
/**
*
* Specifies that you want to list information for only the listed principals.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @return Specifies that you want to list information for only the listed principals.
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*/
public java.util.List getPrincipals() {
return principals;
}
/**
*
* Specifies that you want to list information for only the listed principals.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @param principals
* Specifies that you want to list information for only the listed principals.
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*/
public void setPrincipals(java.util.Collection principals) {
if (principals == null) {
this.principals = null;
return;
}
this.principals = new java.util.ArrayList(principals);
}
/**
*
* Specifies that you want to list information for only the listed principals.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPrincipals(java.util.Collection)} or {@link #withPrincipals(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param principals
* Specifies that you want to list information for only the listed principals.
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withPrincipals(String... principals) {
if (this.principals == null) {
setPrincipals(new java.util.ArrayList(principals.length));
}
for (String ele : principals) {
this.principals.add(ele);
}
return this;
}
/**
*
* Specifies that you want to list information for only the listed principals.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @param principals
* Specifies that you want to list information for only the listed principals.
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withPrincipals(java.util.Collection principals) {
setPrincipals(principals);
return this;
}
/**
*
* Specifies that you want to list information for only principals associated with resource shares that include the
* specified resource type.
*
*
* For a list of valid values, query the ListResourceTypes operation.
*
*
* @param resourceType
* Specifies that you want to list information for only principals associated with resource shares that
* include the specified resource type.
*
* For a list of valid values, query the ListResourceTypes operation.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* Specifies that you want to list information for only principals associated with resource shares that include the
* specified resource type.
*
*
* For a list of valid values, query the ListResourceTypes operation.
*
*
* @return Specifies that you want to list information for only principals associated with resource shares that
* include the specified resource type.
*
* For a list of valid values, query the ListResourceTypes operation.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* Specifies that you want to list information for only principals associated with resource shares that include the
* specified resource type.
*
*
* For a list of valid values, query the ListResourceTypes operation.
*
*
* @param resourceType
* Specifies that you want to list information for only principals associated with resource shares that
* include the specified resource type.
*
* For a list of valid values, query the ListResourceTypes operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* Specifies that you want to list information for only principals associated with the resource shares specified by
* a list the Amazon Resource
* Names (ARNs).
*
*
* @return Specifies that you want to list information for only principals associated with the resource shares
* specified by a list the Amazon Resource Names
* (ARNs).
*/
public java.util.List getResourceShareArns() {
return resourceShareArns;
}
/**
*
* Specifies that you want to list information for only principals associated with the resource shares specified by
* a list the Amazon Resource
* Names (ARNs).
*
*
* @param resourceShareArns
* Specifies that you want to list information for only principals associated with the resource shares
* specified by a list the Amazon Resource Names
* (ARNs).
*/
public void setResourceShareArns(java.util.Collection resourceShareArns) {
if (resourceShareArns == null) {
this.resourceShareArns = null;
return;
}
this.resourceShareArns = new java.util.ArrayList(resourceShareArns);
}
/**
*
* Specifies that you want to list information for only principals associated with the resource shares specified by
* a list the Amazon Resource
* Names (ARNs).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceShareArns(java.util.Collection)} or {@link #withResourceShareArns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param resourceShareArns
* Specifies that you want to list information for only principals associated with the resource shares
* specified by a list the Amazon Resource Names
* (ARNs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withResourceShareArns(String... resourceShareArns) {
if (this.resourceShareArns == null) {
setResourceShareArns(new java.util.ArrayList(resourceShareArns.length));
}
for (String ele : resourceShareArns) {
this.resourceShareArns.add(ele);
}
return this;
}
/**
*
* Specifies that you want to list information for only principals associated with the resource shares specified by
* a list the Amazon Resource
* Names (ARNs).
*
*
* @param resourceShareArns
* Specifies that you want to list information for only principals associated with the resource shares
* specified by a list the Amazon Resource Names
* (ARNs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withResourceShareArns(java.util.Collection resourceShareArns) {
setResourceShareArns(resourceShareArns);
return this;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @return Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
* response to request the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @return Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
* after every operation to ensure that you receive all of the results.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListPrincipalsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceOwner() != null)
sb.append("ResourceOwner: ").append(getResourceOwner()).append(",");
if (getResourceArn() != null)
sb.append("ResourceArn: ").append(getResourceArn()).append(",");
if (getPrincipals() != null)
sb.append("Principals: ").append(getPrincipals()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getResourceShareArns() != null)
sb.append("ResourceShareArns: ").append(getResourceShareArns()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListPrincipalsRequest == false)
return false;
ListPrincipalsRequest other = (ListPrincipalsRequest) obj;
if (other.getResourceOwner() == null ^ this.getResourceOwner() == null)
return false;
if (other.getResourceOwner() != null && other.getResourceOwner().equals(this.getResourceOwner()) == false)
return false;
if (other.getResourceArn() == null ^ this.getResourceArn() == null)
return false;
if (other.getResourceArn() != null && other.getResourceArn().equals(this.getResourceArn()) == false)
return false;
if (other.getPrincipals() == null ^ this.getPrincipals() == null)
return false;
if (other.getPrincipals() != null && other.getPrincipals().equals(this.getPrincipals()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getResourceShareArns() == null ^ this.getResourceShareArns() == null)
return false;
if (other.getResourceShareArns() != null && other.getResourceShareArns().equals(this.getResourceShareArns()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceOwner() == null) ? 0 : getResourceOwner().hashCode());
hashCode = prime * hashCode + ((getResourceArn() == null) ? 0 : getResourceArn().hashCode());
hashCode = prime * hashCode + ((getPrincipals() == null) ? 0 : getPrincipals().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getResourceShareArns() == null) ? 0 : getResourceShareArns().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public ListPrincipalsRequest clone() {
return (ListPrincipalsRequest) super.clone();
}
}