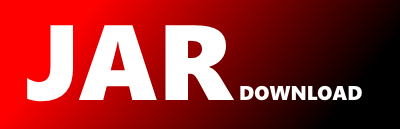
com.amazonaws.services.ram.model.ResourceShareInvitation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an invitation for an Amazon Web Services account to join a resource share.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceShareInvitation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name
* (ARN) of the invitation.
*
*/
private String resourceShareInvitationArn;
/**
*
* The name of the resource share.
*
*/
private String resourceShareName;
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*/
private String resourceShareArn;
/**
*
* The ID of the Amazon Web Services account that sent the invitation.
*
*/
private String senderAccountId;
/**
*
* The ID of the Amazon Web Services account that received the invitation.
*
*/
private String receiverAccountId;
/**
*
* The date and time when the invitation was sent.
*
*/
private java.util.Date invitationTimestamp;
/**
*
* The current status of the invitation.
*
*/
private String status;
/**
*
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*
*/
@Deprecated
private java.util.List resourceShareAssociations;
/**
*
* The Amazon Resource Name
* (ARN) of the IAM user or role that received the invitation.
*
*/
private String receiverArn;
/**
*
* The Amazon Resource Name
* (ARN) of the invitation.
*
*
* @param resourceShareInvitationArn
* The Amazon Resource
* Name (ARN) of the invitation.
*/
public void setResourceShareInvitationArn(String resourceShareInvitationArn) {
this.resourceShareInvitationArn = resourceShareInvitationArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the invitation.
*
*
* @return The Amazon Resource
* Name (ARN) of the invitation.
*/
public String getResourceShareInvitationArn() {
return this.resourceShareInvitationArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the invitation.
*
*
* @param resourceShareInvitationArn
* The Amazon Resource
* Name (ARN) of the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withResourceShareInvitationArn(String resourceShareInvitationArn) {
setResourceShareInvitationArn(resourceShareInvitationArn);
return this;
}
/**
*
* The name of the resource share.
*
*
* @param resourceShareName
* The name of the resource share.
*/
public void setResourceShareName(String resourceShareName) {
this.resourceShareName = resourceShareName;
}
/**
*
* The name of the resource share.
*
*
* @return The name of the resource share.
*/
public String getResourceShareName() {
return this.resourceShareName;
}
/**
*
* The name of the resource share.
*
*
* @param resourceShareName
* The name of the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withResourceShareName(String resourceShareName) {
setResourceShareName(resourceShareName);
return this;
}
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @param resourceShareArn
* The Amazon Resource
* Name (ARN) of the resource share
*/
public void setResourceShareArn(String resourceShareArn) {
this.resourceShareArn = resourceShareArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @return The Amazon Resource
* Name (ARN) of the resource share
*/
public String getResourceShareArn() {
return this.resourceShareArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @param resourceShareArn
* The Amazon Resource
* Name (ARN) of the resource share
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withResourceShareArn(String resourceShareArn) {
setResourceShareArn(resourceShareArn);
return this;
}
/**
*
* The ID of the Amazon Web Services account that sent the invitation.
*
*
* @param senderAccountId
* The ID of the Amazon Web Services account that sent the invitation.
*/
public void setSenderAccountId(String senderAccountId) {
this.senderAccountId = senderAccountId;
}
/**
*
* The ID of the Amazon Web Services account that sent the invitation.
*
*
* @return The ID of the Amazon Web Services account that sent the invitation.
*/
public String getSenderAccountId() {
return this.senderAccountId;
}
/**
*
* The ID of the Amazon Web Services account that sent the invitation.
*
*
* @param senderAccountId
* The ID of the Amazon Web Services account that sent the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withSenderAccountId(String senderAccountId) {
setSenderAccountId(senderAccountId);
return this;
}
/**
*
* The ID of the Amazon Web Services account that received the invitation.
*
*
* @param receiverAccountId
* The ID of the Amazon Web Services account that received the invitation.
*/
public void setReceiverAccountId(String receiverAccountId) {
this.receiverAccountId = receiverAccountId;
}
/**
*
* The ID of the Amazon Web Services account that received the invitation.
*
*
* @return The ID of the Amazon Web Services account that received the invitation.
*/
public String getReceiverAccountId() {
return this.receiverAccountId;
}
/**
*
* The ID of the Amazon Web Services account that received the invitation.
*
*
* @param receiverAccountId
* The ID of the Amazon Web Services account that received the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withReceiverAccountId(String receiverAccountId) {
setReceiverAccountId(receiverAccountId);
return this;
}
/**
*
* The date and time when the invitation was sent.
*
*
* @param invitationTimestamp
* The date and time when the invitation was sent.
*/
public void setInvitationTimestamp(java.util.Date invitationTimestamp) {
this.invitationTimestamp = invitationTimestamp;
}
/**
*
* The date and time when the invitation was sent.
*
*
* @return The date and time when the invitation was sent.
*/
public java.util.Date getInvitationTimestamp() {
return this.invitationTimestamp;
}
/**
*
* The date and time when the invitation was sent.
*
*
* @param invitationTimestamp
* The date and time when the invitation was sent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withInvitationTimestamp(java.util.Date invitationTimestamp) {
setInvitationTimestamp(invitationTimestamp);
return this;
}
/**
*
* The current status of the invitation.
*
*
* @param status
* The current status of the invitation.
* @see ResourceShareInvitationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the invitation.
*
*
* @return The current status of the invitation.
* @see ResourceShareInvitationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the invitation.
*
*
* @param status
* The current status of the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareInvitationStatus
*/
public ResourceShareInvitation withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the invitation.
*
*
* @param status
* The current status of the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareInvitationStatus
*/
public ResourceShareInvitation withStatus(ResourceShareInvitationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*
*
* @return To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*/
@Deprecated
public java.util.List getResourceShareAssociations() {
return resourceShareAssociations;
}
/**
*
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*
*
* @param resourceShareAssociations
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*/
@Deprecated
public void setResourceShareAssociations(java.util.Collection resourceShareAssociations) {
if (resourceShareAssociations == null) {
this.resourceShareAssociations = null;
return;
}
this.resourceShareAssociations = new java.util.ArrayList(resourceShareAssociations);
}
/**
*
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceShareAssociations(java.util.Collection)} or
* {@link #withResourceShareAssociations(java.util.Collection)} if you want to override the existing values.
*
*
* @param resourceShareAssociations
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ResourceShareInvitation withResourceShareAssociations(ResourceShareAssociation... resourceShareAssociations) {
if (this.resourceShareAssociations == null) {
setResourceShareAssociations(new java.util.ArrayList(resourceShareAssociations.length));
}
for (ResourceShareAssociation ele : resourceShareAssociations) {
this.resourceShareAssociations.add(ele);
}
return this;
}
/**
*
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
*
*
* @param resourceShareAssociations
* To view the resources associated with a pending resource share invitation, use
* ListPendingInvitationResources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public ResourceShareInvitation withResourceShareAssociations(java.util.Collection resourceShareAssociations) {
setResourceShareAssociations(resourceShareAssociations);
return this;
}
/**
*
* The Amazon Resource Name
* (ARN) of the IAM user or role that received the invitation.
*
*
* @param receiverArn
* The Amazon Resource
* Name (ARN) of the IAM user or role that received the invitation.
*/
public void setReceiverArn(String receiverArn) {
this.receiverArn = receiverArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the IAM user or role that received the invitation.
*
*
* @return The Amazon Resource
* Name (ARN) of the IAM user or role that received the invitation.
*/
public String getReceiverArn() {
return this.receiverArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the IAM user or role that received the invitation.
*
*
* @param receiverArn
* The Amazon Resource
* Name (ARN) of the IAM user or role that received the invitation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShareInvitation withReceiverArn(String receiverArn) {
setReceiverArn(receiverArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceShareInvitationArn() != null)
sb.append("ResourceShareInvitationArn: ").append(getResourceShareInvitationArn()).append(",");
if (getResourceShareName() != null)
sb.append("ResourceShareName: ").append(getResourceShareName()).append(",");
if (getResourceShareArn() != null)
sb.append("ResourceShareArn: ").append(getResourceShareArn()).append(",");
if (getSenderAccountId() != null)
sb.append("SenderAccountId: ").append(getSenderAccountId()).append(",");
if (getReceiverAccountId() != null)
sb.append("ReceiverAccountId: ").append(getReceiverAccountId()).append(",");
if (getInvitationTimestamp() != null)
sb.append("InvitationTimestamp: ").append(getInvitationTimestamp()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getResourceShareAssociations() != null)
sb.append("ResourceShareAssociations: ").append(getResourceShareAssociations()).append(",");
if (getReceiverArn() != null)
sb.append("ReceiverArn: ").append(getReceiverArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceShareInvitation == false)
return false;
ResourceShareInvitation other = (ResourceShareInvitation) obj;
if (other.getResourceShareInvitationArn() == null ^ this.getResourceShareInvitationArn() == null)
return false;
if (other.getResourceShareInvitationArn() != null && other.getResourceShareInvitationArn().equals(this.getResourceShareInvitationArn()) == false)
return false;
if (other.getResourceShareName() == null ^ this.getResourceShareName() == null)
return false;
if (other.getResourceShareName() != null && other.getResourceShareName().equals(this.getResourceShareName()) == false)
return false;
if (other.getResourceShareArn() == null ^ this.getResourceShareArn() == null)
return false;
if (other.getResourceShareArn() != null && other.getResourceShareArn().equals(this.getResourceShareArn()) == false)
return false;
if (other.getSenderAccountId() == null ^ this.getSenderAccountId() == null)
return false;
if (other.getSenderAccountId() != null && other.getSenderAccountId().equals(this.getSenderAccountId()) == false)
return false;
if (other.getReceiverAccountId() == null ^ this.getReceiverAccountId() == null)
return false;
if (other.getReceiverAccountId() != null && other.getReceiverAccountId().equals(this.getReceiverAccountId()) == false)
return false;
if (other.getInvitationTimestamp() == null ^ this.getInvitationTimestamp() == null)
return false;
if (other.getInvitationTimestamp() != null && other.getInvitationTimestamp().equals(this.getInvitationTimestamp()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getResourceShareAssociations() == null ^ this.getResourceShareAssociations() == null)
return false;
if (other.getResourceShareAssociations() != null && other.getResourceShareAssociations().equals(this.getResourceShareAssociations()) == false)
return false;
if (other.getReceiverArn() == null ^ this.getReceiverArn() == null)
return false;
if (other.getReceiverArn() != null && other.getReceiverArn().equals(this.getReceiverArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceShareInvitationArn() == null) ? 0 : getResourceShareInvitationArn().hashCode());
hashCode = prime * hashCode + ((getResourceShareName() == null) ? 0 : getResourceShareName().hashCode());
hashCode = prime * hashCode + ((getResourceShareArn() == null) ? 0 : getResourceShareArn().hashCode());
hashCode = prime * hashCode + ((getSenderAccountId() == null) ? 0 : getSenderAccountId().hashCode());
hashCode = prime * hashCode + ((getReceiverAccountId() == null) ? 0 : getReceiverAccountId().hashCode());
hashCode = prime * hashCode + ((getInvitationTimestamp() == null) ? 0 : getInvitationTimestamp().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getResourceShareAssociations() == null) ? 0 : getResourceShareAssociations().hashCode());
hashCode = prime * hashCode + ((getReceiverArn() == null) ? 0 : getReceiverArn().hashCode());
return hashCode;
}
@Override
public ResourceShareInvitation clone() {
try {
return (ResourceShareInvitation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ram.model.transform.ResourceShareInvitationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}