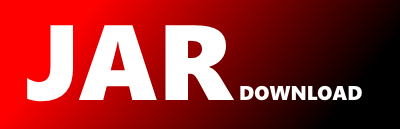
com.amazonaws.services.ram.model.AssociateResourceShareRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AssociateResourceShareRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*
*/
private String resourceShareArn;
/**
*
* Specifies a list of Amazon
* Resource Names (ARNs) of the resources that you want to share. This can be null
if you want to
* add only principals.
*
*/
private java.util.List resourceArns;
/**
*
* Specifies a list of principals to whom you want to the resource share. This can be null
if you want
* to add only resources.
*
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*/
private java.util.List principals;
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*/
private String clientToken;
/**
*
* Specifies from which source accounts the service principal has access to the resources in this resource share.
*
*/
private java.util.List sources;
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*
*
* @param resourceShareArn
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*/
public void setResourceShareArn(String resourceShareArn) {
this.resourceShareArn = resourceShareArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*
*
* @return Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*/
public String getResourceShareArn() {
return this.resourceShareArn;
}
/**
*
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
*
*
* @param resourceShareArn
* Specifies the Amazon
* Resource Name (ARN) of the resource share that you want to add principals or resources to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withResourceShareArn(String resourceShareArn) {
setResourceShareArn(resourceShareArn);
return this;
}
/**
*
* Specifies a list of Amazon
* Resource Names (ARNs) of the resources that you want to share. This can be null
if you want to
* add only principals.
*
*
* @return Specifies a list of Amazon Resource Names
* (ARNs) of the resources that you want to share. This can be null
if you want to add only
* principals.
*/
public java.util.List getResourceArns() {
return resourceArns;
}
/**
*
* Specifies a list of Amazon
* Resource Names (ARNs) of the resources that you want to share. This can be null
if you want to
* add only principals.
*
*
* @param resourceArns
* Specifies a list of Amazon Resource Names
* (ARNs) of the resources that you want to share. This can be null
if you want to add only
* principals.
*/
public void setResourceArns(java.util.Collection resourceArns) {
if (resourceArns == null) {
this.resourceArns = null;
return;
}
this.resourceArns = new java.util.ArrayList(resourceArns);
}
/**
*
* Specifies a list of Amazon
* Resource Names (ARNs) of the resources that you want to share. This can be null
if you want to
* add only principals.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceArns(java.util.Collection)} or {@link #withResourceArns(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param resourceArns
* Specifies a list of Amazon Resource Names
* (ARNs) of the resources that you want to share. This can be null
if you want to add only
* principals.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withResourceArns(String... resourceArns) {
if (this.resourceArns == null) {
setResourceArns(new java.util.ArrayList(resourceArns.length));
}
for (String ele : resourceArns) {
this.resourceArns.add(ele);
}
return this;
}
/**
*
* Specifies a list of Amazon
* Resource Names (ARNs) of the resources that you want to share. This can be null
if you want to
* add only principals.
*
*
* @param resourceArns
* Specifies a list of Amazon Resource Names
* (ARNs) of the resources that you want to share. This can be null
if you want to add only
* principals.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withResourceArns(java.util.Collection resourceArns) {
setResourceArns(resourceArns);
return this;
}
/**
*
* Specifies a list of principals to whom you want to the resource share. This can be null
if you want
* to add only resources.
*
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @return Specifies a list of principals to whom you want to the resource share. This can be null
if
* you want to add only resources.
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*/
public java.util.List getPrincipals() {
return principals;
}
/**
*
* Specifies a list of principals to whom you want to the resource share. This can be null
if you want
* to add only resources.
*
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @param principals
* Specifies a list of principals to whom you want to the resource share. This can be null
if
* you want to add only resources.
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*/
public void setPrincipals(java.util.Collection principals) {
if (principals == null) {
this.principals = null;
return;
}
this.principals = new java.util.ArrayList(principals);
}
/**
*
* Specifies a list of principals to whom you want to the resource share. This can be null
if you want
* to add only resources.
*
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPrincipals(java.util.Collection)} or {@link #withPrincipals(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param principals
* Specifies a list of principals to whom you want to the resource share. This can be null
if
* you want to add only resources.
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withPrincipals(String... principals) {
if (this.principals == null) {
setPrincipals(new java.util.ArrayList(principals.length));
}
for (String ele : principals) {
this.principals.add(ele);
}
return this;
}
/**
*
* Specifies a list of principals to whom you want to the resource share. This can be null
if you want
* to add only resources.
*
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource Name
* (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
*
*
* @param principals
* Specifies a list of principals to whom you want to the resource share. This can be null
if
* you want to add only resources.
*
* What the principals can do with the resources in the share is determined by the RAM permissions that you
* associate with the resource share. See AssociateResourceSharePermission.
*
*
* You can include the following values:
*
*
* -
*
* An Amazon Web Services account ID, for example: 123456789012
*
*
* -
*
* An Amazon Resource
* Name (ARN) of an organization in Organizations, for example:
* organizations::123456789012:organization/o-exampleorgid
*
*
* -
*
* An ARN of an organizational unit (OU) in Organizations, for example:
* organizations::123456789012:ou/o-exampleorgid/ou-examplerootid-exampleouid123
*
*
* -
*
* An ARN of an IAM role, for example: iam::123456789012:role/rolename
*
*
* -
*
* An ARN of an IAM user, for example: iam::123456789012user/username
*
*
*
*
*
* Not all resource types can be shared with IAM roles and users. For more information, see Sharing with IAM roles and users in the Resource Access Manager User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withPrincipals(java.util.Collection principals) {
setPrincipals(principals);
return this;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @param clientToken
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @return Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request. This
* lets you safely retry the request without accidentally performing the same operation a second time. Passing the
* same value to a later call to an operation requires that you also pass the same value for all other parameters.
* We recommend that you use a UUID type of
* value..
*
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the retry fails
* with an IdempotentParameterMismatch
error.
*
*
* @param clientToken
* Specifies a unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* This lets you safely retry the request without accidentally performing the same operation a second time.
* Passing the same value to a later call to an operation requires that you also pass the same value for all
* other parameters. We recommend that you use a UUID type of value..
*
* If you don't provide this value, then Amazon Web Services generates a random one for you.
*
*
* If you retry the operation with the same ClientToken
, but with different parameters, the
* retry fails with an IdempotentParameterMismatch
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Specifies from which source accounts the service principal has access to the resources in this resource share.
*
*
* @return Specifies from which source accounts the service principal has access to the resources in this resource
* share.
*/
public java.util.List getSources() {
return sources;
}
/**
*
* Specifies from which source accounts the service principal has access to the resources in this resource share.
*
*
* @param sources
* Specifies from which source accounts the service principal has access to the resources in this resource
* share.
*/
public void setSources(java.util.Collection sources) {
if (sources == null) {
this.sources = null;
return;
}
this.sources = new java.util.ArrayList(sources);
}
/**
*
* Specifies from which source accounts the service principal has access to the resources in this resource share.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSources(java.util.Collection)} or {@link #withSources(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param sources
* Specifies from which source accounts the service principal has access to the resources in this resource
* share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withSources(String... sources) {
if (this.sources == null) {
setSources(new java.util.ArrayList(sources.length));
}
for (String ele : sources) {
this.sources.add(ele);
}
return this;
}
/**
*
* Specifies from which source accounts the service principal has access to the resources in this resource share.
*
*
* @param sources
* Specifies from which source accounts the service principal has access to the resources in this resource
* share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AssociateResourceShareRequest withSources(java.util.Collection sources) {
setSources(sources);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceShareArn() != null)
sb.append("ResourceShareArn: ").append(getResourceShareArn()).append(",");
if (getResourceArns() != null)
sb.append("ResourceArns: ").append(getResourceArns()).append(",");
if (getPrincipals() != null)
sb.append("Principals: ").append(getPrincipals()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getSources() != null)
sb.append("Sources: ").append(getSources());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AssociateResourceShareRequest == false)
return false;
AssociateResourceShareRequest other = (AssociateResourceShareRequest) obj;
if (other.getResourceShareArn() == null ^ this.getResourceShareArn() == null)
return false;
if (other.getResourceShareArn() != null && other.getResourceShareArn().equals(this.getResourceShareArn()) == false)
return false;
if (other.getResourceArns() == null ^ this.getResourceArns() == null)
return false;
if (other.getResourceArns() != null && other.getResourceArns().equals(this.getResourceArns()) == false)
return false;
if (other.getPrincipals() == null ^ this.getPrincipals() == null)
return false;
if (other.getPrincipals() != null && other.getPrincipals().equals(this.getPrincipals()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getSources() == null ^ this.getSources() == null)
return false;
if (other.getSources() != null && other.getSources().equals(this.getSources()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceShareArn() == null) ? 0 : getResourceShareArn().hashCode());
hashCode = prime * hashCode + ((getResourceArns() == null) ? 0 : getResourceArns().hashCode());
hashCode = prime * hashCode + ((getPrincipals() == null) ? 0 : getPrincipals().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getSources() == null) ? 0 : getSources().hashCode());
return hashCode;
}
@Override
public AssociateResourceShareRequest clone() {
return (AssociateResourceShareRequest) super.clone();
}
}