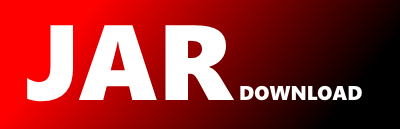
com.amazonaws.services.ram.model.GetResourceSharesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetResourceSharesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*
*/
private java.util.List resourceShareArns;
/**
*
* Specifies that you want to retrieve details of only those resource shares that have this status.
*
*/
private String resourceShareStatus;
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
*
*/
private String resourceOwner;
/**
*
* Specifies the name of an individual resource share that you want to retrieve details about.
*
*/
private String name;
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the specified tag keys and
* values.
*
*/
private java.util.List tagFilters;
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*/
private String nextToken;
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*/
private Integer maxResults;
/**
*
* Specifies that you want to retrieve details of only those resource shares that use the managed permission with
* this Amazon Resource Name
* (ARN).
*
*/
private String permissionArn;
/**
*
* Specifies that you want to retrieve details for only those resource shares that use the specified version of the
* managed permission.
*
*/
private Integer permissionVersion;
/**
*
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*
*
* @return Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*/
public java.util.List getResourceShareArns() {
return resourceShareArns;
}
/**
*
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*
*
* @param resourceShareArns
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*/
public void setResourceShareArns(java.util.Collection resourceShareArns) {
if (resourceShareArns == null) {
this.resourceShareArns = null;
return;
}
this.resourceShareArns = new java.util.ArrayList(resourceShareArns);
}
/**
*
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceShareArns(java.util.Collection)} or {@link #withResourceShareArns(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param resourceShareArns
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withResourceShareArns(String... resourceShareArns) {
if (this.resourceShareArns == null) {
setResourceShareArns(new java.util.ArrayList(resourceShareArns.length));
}
for (String ele : resourceShareArns) {
this.resourceShareArns.add(ele);
}
return this;
}
/**
*
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
*
*
* @param resourceShareArns
* Specifies the Amazon
* Resource Names (ARNs) of individual resource shares that you want information about.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withResourceShareArns(java.util.Collection resourceShareArns) {
setResourceShareArns(resourceShareArns);
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that have this status.
*
*
* @param resourceShareStatus
* Specifies that you want to retrieve details of only those resource shares that have this status.
* @see ResourceShareStatus
*/
public void setResourceShareStatus(String resourceShareStatus) {
this.resourceShareStatus = resourceShareStatus;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that have this status.
*
*
* @return Specifies that you want to retrieve details of only those resource shares that have this status.
* @see ResourceShareStatus
*/
public String getResourceShareStatus() {
return this.resourceShareStatus;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that have this status.
*
*
* @param resourceShareStatus
* Specifies that you want to retrieve details of only those resource shares that have this status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareStatus
*/
public GetResourceSharesRequest withResourceShareStatus(String resourceShareStatus) {
setResourceShareStatus(resourceShareStatus);
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that have this status.
*
*
* @param resourceShareStatus
* Specifies that you want to retrieve details of only those resource shares that have this status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareStatus
*/
public GetResourceSharesRequest withResourceShareStatus(ResourceShareStatus resourceShareStatus) {
this.resourceShareStatus = resourceShareStatus.toString();
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
* @see ResourceOwner
*/
public void setResourceOwner(String resourceOwner) {
this.resourceOwner = resourceOwner;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
*
*
* @return Specifies that you want to retrieve details of only those resource shares that match the following:
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
* @see ResourceOwner
*/
public String getResourceOwner() {
return this.resourceOwner;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public GetResourceSharesRequest withResourceOwner(String resourceOwner) {
setResourceOwner(resourceOwner);
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
*
*
* @param resourceOwner
* Specifies that you want to retrieve details of only those resource shares that match the following:
*
* -
*
* SELF
– resource shares that your account shares with other accounts
*
*
* -
*
* OTHER-ACCOUNTS
– resource shares that other accounts share with your account
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceOwner
*/
public GetResourceSharesRequest withResourceOwner(ResourceOwner resourceOwner) {
this.resourceOwner = resourceOwner.toString();
return this;
}
/**
*
* Specifies the name of an individual resource share that you want to retrieve details about.
*
*
* @param name
* Specifies the name of an individual resource share that you want to retrieve details about.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* Specifies the name of an individual resource share that you want to retrieve details about.
*
*
* @return Specifies the name of an individual resource share that you want to retrieve details about.
*/
public String getName() {
return this.name;
}
/**
*
* Specifies the name of an individual resource share that you want to retrieve details about.
*
*
* @param name
* Specifies the name of an individual resource share that you want to retrieve details about.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the specified tag keys and
* values.
*
*
* @return Specifies that you want to retrieve details of only those resource shares that match the specified tag
* keys and values.
*/
public java.util.List getTagFilters() {
return tagFilters;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the specified tag keys and
* values.
*
*
* @param tagFilters
* Specifies that you want to retrieve details of only those resource shares that match the specified tag
* keys and values.
*/
public void setTagFilters(java.util.Collection tagFilters) {
if (tagFilters == null) {
this.tagFilters = null;
return;
}
this.tagFilters = new java.util.ArrayList(tagFilters);
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the specified tag keys and
* values.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagFilters(java.util.Collection)} or {@link #withTagFilters(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tagFilters
* Specifies that you want to retrieve details of only those resource shares that match the specified tag
* keys and values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withTagFilters(TagFilter... tagFilters) {
if (this.tagFilters == null) {
setTagFilters(new java.util.ArrayList(tagFilters.length));
}
for (TagFilter ele : tagFilters) {
this.tagFilters.add(ele);
}
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that match the specified tag keys and
* values.
*
*
* @param tagFilters
* Specifies that you want to retrieve details of only those resource shares that match the specified tag
* keys and values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withTagFilters(java.util.Collection tagFilters) {
setTagFilters(tagFilters);
return this;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @return Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
* response to request the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* Specifies that you want to receive the next page of results. Valid only if you received a NextToken
* response in the previous request. If you did, it indicates that more output is available. Set this parameter to
* the value provided by the previous call's NextToken
response to request the next page of results.
*
*
* @param nextToken
* Specifies that you want to receive the next page of results. Valid only if you received a
* NextToken
response in the previous request. If you did, it indicates that more output is
* available. Set this parameter to the value provided by the previous call's NextToken
response
* to request the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @return Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
* after every operation to ensure that you receive all of the results.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* Specifies the total number of results that you want included on each page of the response. If you do not include
* this parameter, it defaults to a value that is specific to the operation. If additional items exist beyond the
* number you specify, the NextToken
response element is returned with a value (not null). Include the
* specified value as the NextToken
request parameter in the next call to the operation to get the next
* part of the results. Note that the service might return fewer results than the maximum even when there are more
* results available. You should check NextToken
after every operation to ensure that you receive all
* of the results.
*
*
* @param maxResults
* Specifies the total number of results that you want included on each page of the response. If you do not
* include this parameter, it defaults to a value that is specific to the operation. If additional items
* exist beyond the number you specify, the NextToken
response element is returned with a value
* (not null). Include the specified value as the NextToken
request parameter in the next call
* to the operation to get the next part of the results. Note that the service might return fewer results
* than the maximum even when there are more results available. You should check NextToken
after
* every operation to ensure that you receive all of the results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that use the managed permission with
* this Amazon Resource Name
* (ARN).
*
*
* @param permissionArn
* Specifies that you want to retrieve details of only those resource shares that use the managed permission
* with this Amazon
* Resource Name (ARN).
*/
public void setPermissionArn(String permissionArn) {
this.permissionArn = permissionArn;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that use the managed permission with
* this Amazon Resource Name
* (ARN).
*
*
* @return Specifies that you want to retrieve details of only those resource shares that use the managed permission
* with this Amazon
* Resource Name (ARN).
*/
public String getPermissionArn() {
return this.permissionArn;
}
/**
*
* Specifies that you want to retrieve details of only those resource shares that use the managed permission with
* this Amazon Resource Name
* (ARN).
*
*
* @param permissionArn
* Specifies that you want to retrieve details of only those resource shares that use the managed permission
* with this Amazon
* Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withPermissionArn(String permissionArn) {
setPermissionArn(permissionArn);
return this;
}
/**
*
* Specifies that you want to retrieve details for only those resource shares that use the specified version of the
* managed permission.
*
*
* @param permissionVersion
* Specifies that you want to retrieve details for only those resource shares that use the specified version
* of the managed permission.
*/
public void setPermissionVersion(Integer permissionVersion) {
this.permissionVersion = permissionVersion;
}
/**
*
* Specifies that you want to retrieve details for only those resource shares that use the specified version of the
* managed permission.
*
*
* @return Specifies that you want to retrieve details for only those resource shares that use the specified version
* of the managed permission.
*/
public Integer getPermissionVersion() {
return this.permissionVersion;
}
/**
*
* Specifies that you want to retrieve details for only those resource shares that use the specified version of the
* managed permission.
*
*
* @param permissionVersion
* Specifies that you want to retrieve details for only those resource shares that use the specified version
* of the managed permission.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetResourceSharesRequest withPermissionVersion(Integer permissionVersion) {
setPermissionVersion(permissionVersion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceShareArns() != null)
sb.append("ResourceShareArns: ").append(getResourceShareArns()).append(",");
if (getResourceShareStatus() != null)
sb.append("ResourceShareStatus: ").append(getResourceShareStatus()).append(",");
if (getResourceOwner() != null)
sb.append("ResourceOwner: ").append(getResourceOwner()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getTagFilters() != null)
sb.append("TagFilters: ").append(getTagFilters()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getPermissionArn() != null)
sb.append("PermissionArn: ").append(getPermissionArn()).append(",");
if (getPermissionVersion() != null)
sb.append("PermissionVersion: ").append(getPermissionVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetResourceSharesRequest == false)
return false;
GetResourceSharesRequest other = (GetResourceSharesRequest) obj;
if (other.getResourceShareArns() == null ^ this.getResourceShareArns() == null)
return false;
if (other.getResourceShareArns() != null && other.getResourceShareArns().equals(this.getResourceShareArns()) == false)
return false;
if (other.getResourceShareStatus() == null ^ this.getResourceShareStatus() == null)
return false;
if (other.getResourceShareStatus() != null && other.getResourceShareStatus().equals(this.getResourceShareStatus()) == false)
return false;
if (other.getResourceOwner() == null ^ this.getResourceOwner() == null)
return false;
if (other.getResourceOwner() != null && other.getResourceOwner().equals(this.getResourceOwner()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getTagFilters() == null ^ this.getTagFilters() == null)
return false;
if (other.getTagFilters() != null && other.getTagFilters().equals(this.getTagFilters()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getPermissionArn() == null ^ this.getPermissionArn() == null)
return false;
if (other.getPermissionArn() != null && other.getPermissionArn().equals(this.getPermissionArn()) == false)
return false;
if (other.getPermissionVersion() == null ^ this.getPermissionVersion() == null)
return false;
if (other.getPermissionVersion() != null && other.getPermissionVersion().equals(this.getPermissionVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceShareArns() == null) ? 0 : getResourceShareArns().hashCode());
hashCode = prime * hashCode + ((getResourceShareStatus() == null) ? 0 : getResourceShareStatus().hashCode());
hashCode = prime * hashCode + ((getResourceOwner() == null) ? 0 : getResourceOwner().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getTagFilters() == null) ? 0 : getTagFilters().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getPermissionArn() == null) ? 0 : getPermissionArn().hashCode());
hashCode = prime * hashCode + ((getPermissionVersion() == null) ? 0 : getPermissionVersion().hashCode());
return hashCode;
}
@Override
public GetResourceSharesRequest clone() {
return (GetResourceSharesRequest) super.clone();
}
}