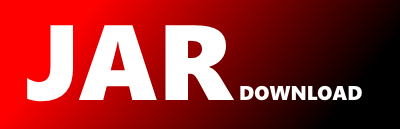
com.amazonaws.services.ram.model.ResourceShare Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ram Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ram.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a resource share in RAM.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceShare implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*/
private String resourceShareArn;
/**
*
* The name of the resource share.
*
*/
private String name;
/**
*
* The ID of the Amazon Web Services account that owns the resource share.
*
*/
private String owningAccountId;
/**
*
* Indicates whether principals outside your organization in Organizations can be associated with a resource share.
*
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the account
* that owns the resource share.
*
*
*
*/
private Boolean allowExternalPrincipals;
/**
*
* The current status of the resource share.
*
*/
private String status;
/**
*
* A message about the status of the resource share.
*
*/
private String statusMessage;
/**
*
* The tag key and value pairs attached to the resource share.
*
*/
private java.util.List tags;
/**
*
* The date and time when the resource share was created.
*
*/
private java.util.Date creationTime;
/**
*
* The date and time when the resource share was last updated.
*
*/
private java.util.Date lastUpdatedTime;
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*/
private String featureSet;
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @param resourceShareArn
* The Amazon Resource
* Name (ARN) of the resource share
*/
public void setResourceShareArn(String resourceShareArn) {
this.resourceShareArn = resourceShareArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @return The Amazon Resource
* Name (ARN) of the resource share
*/
public String getResourceShareArn() {
return this.resourceShareArn;
}
/**
*
* The Amazon Resource Name
* (ARN) of the resource share
*
*
* @param resourceShareArn
* The Amazon Resource
* Name (ARN) of the resource share
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withResourceShareArn(String resourceShareArn) {
setResourceShareArn(resourceShareArn);
return this;
}
/**
*
* The name of the resource share.
*
*
* @param name
* The name of the resource share.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the resource share.
*
*
* @return The name of the resource share.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the resource share.
*
*
* @param name
* The name of the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withName(String name) {
setName(name);
return this;
}
/**
*
* The ID of the Amazon Web Services account that owns the resource share.
*
*
* @param owningAccountId
* The ID of the Amazon Web Services account that owns the resource share.
*/
public void setOwningAccountId(String owningAccountId) {
this.owningAccountId = owningAccountId;
}
/**
*
* The ID of the Amazon Web Services account that owns the resource share.
*
*
* @return The ID of the Amazon Web Services account that owns the resource share.
*/
public String getOwningAccountId() {
return this.owningAccountId;
}
/**
*
* The ID of the Amazon Web Services account that owns the resource share.
*
*
* @param owningAccountId
* The ID of the Amazon Web Services account that owns the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withOwningAccountId(String owningAccountId) {
setOwningAccountId(owningAccountId);
return this;
}
/**
*
* Indicates whether principals outside your organization in Organizations can be associated with a resource share.
*
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the account
* that owns the resource share.
*
*
*
*
* @param allowExternalPrincipals
* Indicates whether principals outside your organization in Organizations can be associated with a resource
* share.
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the
* account that owns the resource share.
*
*
*/
public void setAllowExternalPrincipals(Boolean allowExternalPrincipals) {
this.allowExternalPrincipals = allowExternalPrincipals;
}
/**
*
* Indicates whether principals outside your organization in Organizations can be associated with a resource share.
*
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the account
* that owns the resource share.
*
*
*
*
* @return Indicates whether principals outside your organization in Organizations can be associated with a resource
* share.
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the
* account that owns the resource share.
*
*
*/
public Boolean getAllowExternalPrincipals() {
return this.allowExternalPrincipals;
}
/**
*
* Indicates whether principals outside your organization in Organizations can be associated with a resource share.
*
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the account
* that owns the resource share.
*
*
*
*
* @param allowExternalPrincipals
* Indicates whether principals outside your organization in Organizations can be associated with a resource
* share.
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the
* account that owns the resource share.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withAllowExternalPrincipals(Boolean allowExternalPrincipals) {
setAllowExternalPrincipals(allowExternalPrincipals);
return this;
}
/**
*
* Indicates whether principals outside your organization in Organizations can be associated with a resource share.
*
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the account
* that owns the resource share.
*
*
*
*
* @return Indicates whether principals outside your organization in Organizations can be associated with a resource
* share.
*
* -
*
* True
– the resource share can be shared with any Amazon Web Services account.
*
*
* -
*
* False
– the resource share can be shared with only accounts in the same organization as the
* account that owns the resource share.
*
*
*/
public Boolean isAllowExternalPrincipals() {
return this.allowExternalPrincipals;
}
/**
*
* The current status of the resource share.
*
*
* @param status
* The current status of the resource share.
* @see ResourceShareStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the resource share.
*
*
* @return The current status of the resource share.
* @see ResourceShareStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the resource share.
*
*
* @param status
* The current status of the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareStatus
*/
public ResourceShare withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the resource share.
*
*
* @param status
* The current status of the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareStatus
*/
public ResourceShare withStatus(ResourceShareStatus status) {
this.status = status.toString();
return this;
}
/**
*
* A message about the status of the resource share.
*
*
* @param statusMessage
* A message about the status of the resource share.
*/
public void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
/**
*
* A message about the status of the resource share.
*
*
* @return A message about the status of the resource share.
*/
public String getStatusMessage() {
return this.statusMessage;
}
/**
*
* A message about the status of the resource share.
*
*
* @param statusMessage
* A message about the status of the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withStatusMessage(String statusMessage) {
setStatusMessage(statusMessage);
return this;
}
/**
*
* The tag key and value pairs attached to the resource share.
*
*
* @return The tag key and value pairs attached to the resource share.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tag key and value pairs attached to the resource share.
*
*
* @param tags
* The tag key and value pairs attached to the resource share.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tag key and value pairs attached to the resource share.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tag key and value pairs attached to the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tag key and value pairs attached to the resource share.
*
*
* @param tags
* The tag key and value pairs attached to the resource share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The date and time when the resource share was created.
*
*
* @param creationTime
* The date and time when the resource share was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The date and time when the resource share was created.
*
*
* @return The date and time when the resource share was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The date and time when the resource share was created.
*
*
* @param creationTime
* The date and time when the resource share was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The date and time when the resource share was last updated.
*
*
* @param lastUpdatedTime
* The date and time when the resource share was last updated.
*/
public void setLastUpdatedTime(java.util.Date lastUpdatedTime) {
this.lastUpdatedTime = lastUpdatedTime;
}
/**
*
* The date and time when the resource share was last updated.
*
*
* @return The date and time when the resource share was last updated.
*/
public java.util.Date getLastUpdatedTime() {
return this.lastUpdatedTime;
}
/**
*
* The date and time when the resource share was last updated.
*
*
* @param lastUpdatedTime
* The date and time when the resource share was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceShare withLastUpdatedTime(java.util.Date lastUpdatedTime) {
setLastUpdatedTime(lastUpdatedTime);
return this;
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* @param featureSet
* Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy.
* That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress.
* This value changes to STANDARD
when complete.
*
*
* @see ResourceShareFeatureSet
*/
public void setFeatureSet(String featureSet) {
this.featureSet = featureSet;
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* @return Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based
* policy. That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in
* progress. This value changes to STANDARD
when complete.
*
*
* @see ResourceShareFeatureSet
*/
public String getFeatureSet() {
return this.featureSet;
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* @param featureSet
* Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy.
* That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress.
* This value changes to STANDARD
when complete.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareFeatureSet
*/
public ResourceShare withFeatureSet(String featureSet) {
setFeatureSet(featureSet);
return this;
}
/**
*
* Indicates what features are available for this resource share. This parameter can have one of the following
* values:
*
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to all
* principals you share the resource share with. You can modify these resource shares in RAM using the console or
* APIs. This resource share might have been created by RAM, or it might have been CREATED_FROM_POLICY and
* then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy. That
* policy did not match any existing managed permissions, so RAM created this customer managed permission
* automatically on the customer's behalf based on the attached policy document. This type of resource share is
* visible only to the Amazon Web Services account that created it. You can't modify it in RAM unless you promote
* it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but the
* customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress. This value
* changes to STANDARD
when complete.
*
*
*
*
* @param featureSet
* Indicates what features are available for this resource share. This parameter can have one of the
* following values:
*
* -
*
* STANDARD – A resource share that supports all functionality. These resource shares are visible to
* all principals you share the resource share with. You can modify these resource shares in RAM using the
* console or APIs. This resource share might have been created by RAM, or it might have been
* CREATED_FROM_POLICY and then promoted.
*
*
* -
*
* CREATED_FROM_POLICY – The customer manually shared a resource by attaching a resource-based policy.
* That policy did not match any existing managed permissions, so RAM created this customer managed
* permission automatically on the customer's behalf based on the attached policy document. This type of
* resource share is visible only to the Amazon Web Services account that created it. You can't modify it in
* RAM unless you promote it. For more information, see PromoteResourceShareCreatedFromPolicy.
*
*
* -
*
* PROMOTING_TO_STANDARD – This resource share was originally CREATED_FROM_POLICY
, but
* the customer ran the PromoteResourceShareCreatedFromPolicy and that operation is still in progress.
* This value changes to STANDARD
when complete.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceShareFeatureSet
*/
public ResourceShare withFeatureSet(ResourceShareFeatureSet featureSet) {
this.featureSet = featureSet.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getResourceShareArn() != null)
sb.append("ResourceShareArn: ").append(getResourceShareArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getOwningAccountId() != null)
sb.append("OwningAccountId: ").append(getOwningAccountId()).append(",");
if (getAllowExternalPrincipals() != null)
sb.append("AllowExternalPrincipals: ").append(getAllowExternalPrincipals()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusMessage() != null)
sb.append("StatusMessage: ").append(getStatusMessage()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastUpdatedTime() != null)
sb.append("LastUpdatedTime: ").append(getLastUpdatedTime()).append(",");
if (getFeatureSet() != null)
sb.append("FeatureSet: ").append(getFeatureSet());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceShare == false)
return false;
ResourceShare other = (ResourceShare) obj;
if (other.getResourceShareArn() == null ^ this.getResourceShareArn() == null)
return false;
if (other.getResourceShareArn() != null && other.getResourceShareArn().equals(this.getResourceShareArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getOwningAccountId() == null ^ this.getOwningAccountId() == null)
return false;
if (other.getOwningAccountId() != null && other.getOwningAccountId().equals(this.getOwningAccountId()) == false)
return false;
if (other.getAllowExternalPrincipals() == null ^ this.getAllowExternalPrincipals() == null)
return false;
if (other.getAllowExternalPrincipals() != null && other.getAllowExternalPrincipals().equals(this.getAllowExternalPrincipals()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusMessage() == null ^ this.getStatusMessage() == null)
return false;
if (other.getStatusMessage() != null && other.getStatusMessage().equals(this.getStatusMessage()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastUpdatedTime() == null ^ this.getLastUpdatedTime() == null)
return false;
if (other.getLastUpdatedTime() != null && other.getLastUpdatedTime().equals(this.getLastUpdatedTime()) == false)
return false;
if (other.getFeatureSet() == null ^ this.getFeatureSet() == null)
return false;
if (other.getFeatureSet() != null && other.getFeatureSet().equals(this.getFeatureSet()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getResourceShareArn() == null) ? 0 : getResourceShareArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getOwningAccountId() == null) ? 0 : getOwningAccountId().hashCode());
hashCode = prime * hashCode + ((getAllowExternalPrincipals() == null) ? 0 : getAllowExternalPrincipals().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusMessage() == null) ? 0 : getStatusMessage().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastUpdatedTime() == null) ? 0 : getLastUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getFeatureSet() == null) ? 0 : getFeatureSet().hashCode());
return hashCode;
}
@Override
public ResourceShare clone() {
try {
return (ResourceShare) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ram.model.transform.ResourceShareMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}