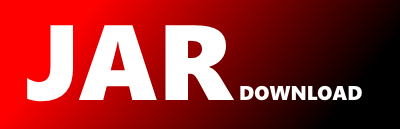
com.amazonaws.services.rds.AmazonRDSAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds;
import javax.annotation.Generated;
import com.amazonaws.services.rds.model.*;
/**
* Interface for accessing Amazon RDS asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.rds.AbstractAmazonRDSAsync} instead.
*
*
* Amazon Relational Database Service
*
*
*
* Amazon Relational Database Service (Amazon RDS) is a web service that makes it easier to set up, operate, and scale a
* relational database in the cloud. It provides cost-efficient, resizeable capacity for an industry-standard relational
* database and manages common database administration tasks, freeing up developers to focus on what makes their
* applications and businesses unique.
*
*
* Amazon RDS gives you access to the capabilities of a MySQL, MariaDB, PostgreSQL, Microsoft SQL Server, Oracle, or
* Amazon Aurora database server. These capabilities mean that the code, applications, and tools you already use today
* with your existing databases work with Amazon RDS without modification. Amazon RDS automatically backs up your
* database and maintains the database software that powers your DB instance. Amazon RDS is flexible: you can scale your
* DB instance's compute resources and storage capacity to meet your application's demand. As with all Amazon Web
* Services, there are no up-front investments, and you pay only for the resources you use.
*
*
* This interface reference for Amazon RDS contains documentation for a programming or command line interface you can
* use to manage Amazon RDS. Amazon RDS is asynchronous, which means that some interfaces might require techniques such
* as polling or callback functions to determine when a command has been applied. In this reference, the parameter
* descriptions indicate whether a command is applied immediately, on the next instance reboot, or during the
* maintenance window. The reference structure is as follows, and we list following some related topics from the user
* guide.
*
*
* Amazon RDS API Reference
*
*
* -
*
* For the alphabetical list of API actions, see API Actions.
*
*
* -
*
* For the alphabetical list of data types, see Data Types.
*
*
* -
*
* For a list of common query parameters, see Common Parameters.
*
*
* -
*
* For descriptions of the error codes, see Common Errors.
*
*
*
*
* Amazon RDS User Guide
*
*
* -
*
* For a summary of the Amazon RDS interfaces, see Available RDS
* Interfaces.
*
*
* -
*
* For more information about how to use the Query API, see Using the Query API.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonRDSAsync extends AmazonRDS {
/**
*
* Associates an Identity and Access Management (IAM) role from an Amazon Aurora DB cluster. For more information,
* see Authorizing Amazon Aurora MySQL to Access Other Amazon Web Services Services on Your Behalf in the Amazon
* Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param addRoleToDBClusterRequest
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* @sample AmazonRDSAsync.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBClusterAsync(AddRoleToDBClusterRequest addRoleToDBClusterRequest);
/**
*
* Associates an Identity and Access Management (IAM) role from an Amazon Aurora DB cluster. For more information,
* see Authorizing Amazon Aurora MySQL to Access Other Amazon Web Services Services on Your Behalf in the Amazon
* Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param addRoleToDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddRoleToDBCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBClusterAsync(AddRoleToDBClusterRequest addRoleToDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an Amazon Web Services Identity and Access Management (IAM) role with a DB instance.
*
*
*
* To add a role to a DB instance, the status of the DB instance must be available
.
*
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param addRoleToDBInstanceRequest
* @return A Java Future containing the result of the AddRoleToDBInstance operation returned by the service.
* @sample AmazonRDSAsync.AddRoleToDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBInstanceAsync(AddRoleToDBInstanceRequest addRoleToDBInstanceRequest);
/**
*
* Associates an Amazon Web Services Identity and Access Management (IAM) role with a DB instance.
*
*
*
* To add a role to a DB instance, the status of the DB instance must be available
.
*
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param addRoleToDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddRoleToDBInstance operation returned by the service.
* @sample AmazonRDSAsyncHandler.AddRoleToDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addRoleToDBInstanceAsync(AddRoleToDBInstanceRequest addRoleToDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a source identifier to an existing RDS event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* @sample AmazonRDSAsync.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future addSourceIdentifierToSubscriptionAsync(
AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest);
/**
*
* Adds a source identifier to an existing RDS event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddSourceIdentifierToSubscription operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future addSourceIdentifierToSubscriptionAsync(
AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be used with cost allocation reporting to track
* cost associated with Amazon RDS resources, or used in a Condition statement in an IAM policy for Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see Tagging Amazon RDS
* Resources.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AmazonRDSAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be used with cost allocation reporting to track
* cost associated with Amazon RDS resources, or used in a Condition statement in an IAM policy for Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see Tagging Amazon RDS
* Resources.
*
*
* @param addTagsToResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AmazonRDSAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AmazonRDSAsync.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest);
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of authorization. First, EC2 or VPC security groups
* can be added to the DBSecurityGroup if the application using the database is running on EC2 or VPC instances.
* Second, IP ranges are available if the application accessing your database is running on the Internet. Required
* parameters for this API are one of CIDR range, EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
*
* You can't authorize ingress from an EC2 security group in one Amazon Web Services Region to an Amazon RDS DB
* instance in another. You can't authorize ingress from a VPC security group in one VPC to an Amazon RDS DB
* instance in another.
*
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param authorizeDBSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeDBSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRDSAsync.AuthorizeDBSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeDBSecurityGroupIngressAsync(
AuthorizeDBSecurityGroupIngressRequest authorizeDBSecurityGroupIngressRequest);
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of authorization. First, EC2 or VPC security groups
* can be added to the DBSecurityGroup if the application using the database is running on EC2 or VPC instances.
* Second, IP ranges are available if the application accessing your database is running on the Internet. Required
* parameters for this API are one of CIDR range, EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
*
* You can't authorize ingress from an EC2 security group in one Amazon Web Services Region to an Amazon RDS DB
* instance in another. You can't authorize ingress from a VPC security group in one VPC to an Amazon RDS DB
* instance in another.
*
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param authorizeDBSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeDBSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.AuthorizeDBSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeDBSecurityGroupIngressAsync(
AuthorizeDBSecurityGroupIngressRequest authorizeDBSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Backtracks a DB cluster to a specific time, without creating a new DB cluster.
*
*
* For more information on backtracking, see
* Backtracking an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param backtrackDBClusterRequest
* @return A Java Future containing the result of the BacktrackDBCluster operation returned by the service.
* @sample AmazonRDSAsync.BacktrackDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future backtrackDBClusterAsync(BacktrackDBClusterRequest backtrackDBClusterRequest);
/**
*
* Backtracks a DB cluster to a specific time, without creating a new DB cluster.
*
*
* For more information on backtracking, see
* Backtracking an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param backtrackDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BacktrackDBCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.BacktrackDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future backtrackDBClusterAsync(BacktrackDBClusterRequest backtrackDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an export task in progress that is exporting a snapshot to Amazon S3. Any data that has already been
* written to the S3 bucket isn't removed.
*
*
* @param cancelExportTaskRequest
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonRDSAsync.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest);
/**
*
* Cancels an export task in progress that is exporting a snapshot to Amazon S3. Any data that has already been
* written to the S3 bucket isn't removed.
*
*
* @param cancelExportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonRDSAsyncHandler.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified DB cluster parameter group.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterParameterGroupRequest
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* @sample AmazonRDSAsync.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future copyDBClusterParameterGroupAsync(CopyDBClusterParameterGroupRequest copyDBClusterParameterGroupRequest);
/**
*
* Copies the specified DB cluster parameter group.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBClusterParameterGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future copyDBClusterParameterGroupAsync(
CopyDBClusterParameterGroupRequest copyDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* You can copy an encrypted DB cluster snapshot from another Amazon Web Services Region. In that case, the Amazon
* Web Services Region where you call the CopyDBClusterSnapshot
action is the destination Amazon Web
* Services Region for the encrypted DB cluster snapshot to be copied to. To copy an encrypted DB cluster snapshot
* from another Amazon Web Services Region, you must provide the following values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier
* for the key to use to encrypt the copy of the DB cluster snapshot in the destination Amazon Web Services Region.
*
*
* -
*
* PreSignedUrl
- A URL that contains a Signature Version 4 signed request for the
* CopyDBClusterSnapshot
action to be called in the source Amazon Web Services Region where the DB
* cluster snapshot is copied from. The pre-signed URL must be a valid request for the
* CopyDBClusterSnapshot
API action that can be executed in the source Amazon Web Services Region that
* contains the encrypted DB cluster snapshot to be copied.
*
*
* The pre-signed URL request must contain the following parameter values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB cluster snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBClusterSnapshot
action that is called in the destination Amazon Web Services Region, and the
* action contained in the pre-signed URL.
*
*
* -
*
* DestinationRegion
- The name of the Amazon Web Services Region that the DB cluster snapshot is to be
* created in.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB cluster snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBClusterSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:cluster-snapshot:aurora-cluster1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
* -
*
* TargetDBClusterSnapshotIdentifier
- The identifier for the new copy of the DB cluster snapshot in
* the destination Amazon Web Services Region.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the ARN format for the source Amazon Web Services Region and is
* the same value as the SourceDBClusterSnapshotIdentifier
in the pre-signed URL.
*
*
*
*
* To cancel the copy operation once it is in progress, delete the target DB cluster snapshot identified by
* TargetDBClusterSnapshotIdentifier
while that DB cluster snapshot is in "copying" status.
*
*
* For more information on copying encrypted DB cluster snapshots from one Amazon Web Services Region to another,
* see Copying a
* Snapshot in the Amazon Aurora User Guide.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterSnapshotRequest
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsync.CopyDBClusterSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBClusterSnapshotAsync(CopyDBClusterSnapshotRequest copyDBClusterSnapshotRequest);
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* You can copy an encrypted DB cluster snapshot from another Amazon Web Services Region. In that case, the Amazon
* Web Services Region where you call the CopyDBClusterSnapshot
action is the destination Amazon Web
* Services Region for the encrypted DB cluster snapshot to be copied to. To copy an encrypted DB cluster snapshot
* from another Amazon Web Services Region, you must provide the following values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier
* for the key to use to encrypt the copy of the DB cluster snapshot in the destination Amazon Web Services Region.
*
*
* -
*
* PreSignedUrl
- A URL that contains a Signature Version 4 signed request for the
* CopyDBClusterSnapshot
action to be called in the source Amazon Web Services Region where the DB
* cluster snapshot is copied from. The pre-signed URL must be a valid request for the
* CopyDBClusterSnapshot
API action that can be executed in the source Amazon Web Services Region that
* contains the encrypted DB cluster snapshot to be copied.
*
*
* The pre-signed URL request must contain the following parameter values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB cluster snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBClusterSnapshot
action that is called in the destination Amazon Web Services Region, and the
* action contained in the pre-signed URL.
*
*
* -
*
* DestinationRegion
- The name of the Amazon Web Services Region that the DB cluster snapshot is to be
* created in.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB cluster snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBClusterSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:cluster-snapshot:aurora-cluster1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
* -
*
* TargetDBClusterSnapshotIdentifier
- The identifier for the new copy of the DB cluster snapshot in
* the destination Amazon Web Services Region.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the ARN format for the source Amazon Web Services Region and is
* the same value as the SourceDBClusterSnapshotIdentifier
in the pre-signed URL.
*
*
*
*
* To cancel the copy operation once it is in progress, delete the target DB cluster snapshot identified by
* TargetDBClusterSnapshotIdentifier
while that DB cluster snapshot is in "copying" status.
*
*
* For more information on copying encrypted DB cluster snapshots from one Amazon Web Services Region to another,
* see Copying a
* Snapshot in the Amazon Aurora User Guide.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.CopyDBClusterSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBClusterSnapshotAsync(CopyDBClusterSnapshotRequest copyDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsync.CopyDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBParameterGroupAsync(CopyDBParameterGroupRequest copyDBParameterGroupRequest);
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CopyDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBParameterGroupAsync(CopyDBParameterGroupRequest copyDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified DB snapshot. The source DB snapshot must be in the available
state.
*
*
* You can copy a snapshot from one Amazon Web Services Region to another. In that case, the Amazon Web Services
* Region where you call the CopyDBSnapshot
action is the destination Amazon Web Services Region for
* the DB snapshot copy.
*
*
* This command doesn't apply to RDS Custom.
*
*
* For more information about copying snapshots, see Copying
* a DB Snapshot in the Amazon RDS User Guide.
*
*
* @param copyDBSnapshotRequest
* @return A Java Future containing the result of the CopyDBSnapshot operation returned by the service.
* @sample AmazonRDSAsync.CopyDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBSnapshotAsync(CopyDBSnapshotRequest copyDBSnapshotRequest);
/**
*
* Copies the specified DB snapshot. The source DB snapshot must be in the available
state.
*
*
* You can copy a snapshot from one Amazon Web Services Region to another. In that case, the Amazon Web Services
* Region where you call the CopyDBSnapshot
action is the destination Amazon Web Services Region for
* the DB snapshot copy.
*
*
* This command doesn't apply to RDS Custom.
*
*
* For more information about copying snapshots, see Copying
* a DB Snapshot in the Amazon RDS User Guide.
*
*
* @param copyDBSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyDBSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.CopyDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyDBSnapshotAsync(CopyDBSnapshotRequest copyDBSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified option group.
*
*
* @param copyOptionGroupRequest
* @return A Java Future containing the result of the CopyOptionGroup operation returned by the service.
* @sample AmazonRDSAsync.CopyOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyOptionGroupAsync(CopyOptionGroupRequest copyOptionGroupRequest);
/**
*
* Copies the specified option group.
*
*
* @param copyOptionGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyOptionGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CopyOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyOptionGroupAsync(CopyOptionGroupRequest copyOptionGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param createCustomAvailabilityZoneRequest
* @return A Java Future containing the result of the CreateCustomAvailabilityZone operation returned by the
* service.
* @sample AmazonRDSAsync.CreateCustomAvailabilityZone
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomAvailabilityZoneAsync(
CreateCustomAvailabilityZoneRequest createCustomAvailabilityZoneRequest);
/**
*
* Creates a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param createCustomAvailabilityZoneRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomAvailabilityZone operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.CreateCustomAvailabilityZone
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomAvailabilityZoneAsync(
CreateCustomAvailabilityZoneRequest createCustomAvailabilityZoneRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom DB engine version (CEV). A CEV is a binary volume snapshot of a database engine and specific
* AMI. The only supported engine is Oracle Database 19c Enterprise Edition with the January 2021 or later RU/RUR.
* For more information, see
* Amazon RDS Custom requirements and limitations in the Amazon RDS User Guide.
*
*
* Amazon RDS, which is a fully managed service, supplies the Amazon Machine Image (AMI) and database software. The
* Amazon RDS database software is preinstalled, so you need only select a DB engine and version, and create your
* database. With Amazon RDS Custom, you upload your database installation files in Amazon S3. For more information,
* see
* Preparing to create a CEV in the Amazon RDS User Guide.
*
*
* When you create a custom engine version, you specify the files in a JSON document called a CEV manifest. This
* document describes installation .zip files stored in Amazon S3. RDS Custom creates your CEV from the installation
* files that you provided. This service model is called Bring Your Own Media (BYOM).
*
*
* Creation takes approximately two hours. If creation fails, RDS Custom issues RDS-EVENT-0196
with the
* message Creation failed for custom engine version
, and includes details about the failure. For
* example, the event prints missing files.
*
*
* After you create the CEV, it is available for use. You can create multiple CEVs, and create multiple RDS Custom
* instances from any CEV. You can also change the status of a CEV to make it available or inactive.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* CreateCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* CreateCustomDbEngineVersion
event.
*
*
*
* For more information, see Creating a
* CEV in the Amazon RDS User Guide.
*
*
* @param createCustomDBEngineVersionRequest
* @return A Java Future containing the result of the CreateCustomDBEngineVersion operation returned by the service.
* @sample AmazonRDSAsync.CreateCustomDBEngineVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDBEngineVersionAsync(
CreateCustomDBEngineVersionRequest createCustomDBEngineVersionRequest);
/**
*
* Creates a custom DB engine version (CEV). A CEV is a binary volume snapshot of a database engine and specific
* AMI. The only supported engine is Oracle Database 19c Enterprise Edition with the January 2021 or later RU/RUR.
* For more information, see
* Amazon RDS Custom requirements and limitations in the Amazon RDS User Guide.
*
*
* Amazon RDS, which is a fully managed service, supplies the Amazon Machine Image (AMI) and database software. The
* Amazon RDS database software is preinstalled, so you need only select a DB engine and version, and create your
* database. With Amazon RDS Custom, you upload your database installation files in Amazon S3. For more information,
* see
* Preparing to create a CEV in the Amazon RDS User Guide.
*
*
* When you create a custom engine version, you specify the files in a JSON document called a CEV manifest. This
* document describes installation .zip files stored in Amazon S3. RDS Custom creates your CEV from the installation
* files that you provided. This service model is called Bring Your Own Media (BYOM).
*
*
* Creation takes approximately two hours. If creation fails, RDS Custom issues RDS-EVENT-0196
with the
* message Creation failed for custom engine version
, and includes details about the failure. For
* example, the event prints missing files.
*
*
* After you create the CEV, it is available for use. You can create multiple CEVs, and create multiple RDS Custom
* instances from any CEV. You can also change the status of a CEV to make it available or inactive.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* CreateCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* CreateCustomDbEngineVersion
event.
*
*
*
* For more information, see Creating a
* CEV in the Amazon RDS User Guide.
*
*
* @param createCustomDBEngineVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomDBEngineVersion operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateCustomDBEngineVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDBEngineVersionAsync(
CreateCustomDBEngineVersionRequest createCustomDBEngineVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Aurora DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a read replica of
* another DB cluster or Amazon RDS MySQL or PostgreSQL DB instance. For cross-region replication where the DB
* cluster identified by ReplicationSourceIdentifier
is encrypted, you must also specify the
* PreSignedUrl
parameter.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterRequest
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* @sample AmazonRDSAsync.CreateDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBClusterAsync(CreateDBClusterRequest createDBClusterRequest);
/**
*
* Creates a new Amazon Aurora DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a read replica of
* another DB cluster or Amazon RDS MySQL or PostgreSQL DB instance. For cross-region replication where the DB
* cluster identified by ReplicationSourceIdentifier
is encrypted, you must also specify the
* PreSignedUrl
parameter.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBClusterAsync(CreateDBClusterRequest createDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom endpoint and associates it with an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterEndpointRequest
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* @sample AmazonRDSAsync.CreateDBClusterEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBClusterEndpointAsync(CreateDBClusterEndpointRequest createDBClusterEndpointRequest);
/**
*
* Creates a new custom endpoint and associates it with an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterEndpoint operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBClusterEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBClusterEndpointAsync(CreateDBClusterEndpointRequest createDBClusterEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup
. Once you've created a DB cluster parameter group,
* you need to associate it with your DB cluster using ModifyDBCluster
. When you associate a new DB
* cluster parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without
* failover for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon RDS to fully
* complete the create action before the DB cluster parameter group is used as the default for a new DB cluster.
* This is especially important for parameters that are critical when creating the default database for a DB
* cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon RDS console or the DescribeDBClusterParameters
* action to verify that your DB cluster parameter group has been created or modified.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterParameterGroupRequest
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonRDSAsync.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterParameterGroupAsync(
CreateDBClusterParameterGroupRequest createDBClusterParameterGroupRequest);
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup
. Once you've created a DB cluster parameter group,
* you need to associate it with your DB cluster using ModifyDBCluster
. When you associate a new DB
* cluster parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without
* failover for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon RDS to fully
* complete the create action before the DB cluster parameter group is used as the default for a new DB cluster.
* This is especially important for parameters that are critical when creating the default database for a DB
* cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon RDS console or the DescribeDBClusterParameters
* action to verify that your DB cluster parameter group has been created or modified.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBClusterParameterGroupAsync(
CreateDBClusterParameterGroupRequest createDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of a DB cluster. For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterSnapshotRequest
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsync.CreateDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBClusterSnapshotAsync(CreateDBClusterSnapshotRequest createDBClusterSnapshotRequest);
/**
*
* Creates a snapshot of a DB cluster. For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createDBClusterSnapshotAsync(CreateDBClusterSnapshotRequest createDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* @sample AmazonRDSAsync.CreateDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBInstanceAsync(CreateDBInstanceRequest createDBInstanceRequest);
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBInstance operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBInstanceAsync(CreateDBInstanceRequest createDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB instance that acts as a read replica for an existing source DB instance. You can create a read
* replica for a DB instance running MySQL, MariaDB, Oracle, PostgreSQL, or SQL Server. For more information, see Working with Read Replicas
* in the Amazon RDS User Guide.
*
*
* Amazon Aurora doesn't support this action. Call the CreateDBInstance
action to create a DB instance
* for an Aurora DB cluster.
*
*
* All read replica DB instances are created with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source DB instance, except as specified.
*
*
*
* Your source DB instance must have backup retention enabled.
*
*
*
* @param createDBInstanceReadReplicaRequest
* @return A Java Future containing the result of the CreateDBInstanceReadReplica operation returned by the service.
* @sample AmazonRDSAsync.CreateDBInstanceReadReplica
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBInstanceReadReplicaAsync(CreateDBInstanceReadReplicaRequest createDBInstanceReadReplicaRequest);
/**
*
* Creates a new DB instance that acts as a read replica for an existing source DB instance. You can create a read
* replica for a DB instance running MySQL, MariaDB, Oracle, PostgreSQL, or SQL Server. For more information, see Working with Read Replicas
* in the Amazon RDS User Guide.
*
*
* Amazon Aurora doesn't support this action. Call the CreateDBInstance
action to create a DB instance
* for an Aurora DB cluster.
*
*
* All read replica DB instances are created with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source DB instance, except as specified.
*
*
*
* Your source DB instance must have backup retention enabled.
*
*
*
* @param createDBInstanceReadReplicaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBInstanceReadReplica operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBInstanceReadReplica
* @see AWS API Documentation
*/
java.util.concurrent.Future createDBInstanceReadReplicaAsync(CreateDBInstanceReadReplicaRequest createDBInstanceReadReplicaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup
. Once you've created a DB parameter group, you need to associate it with your
* DB instance using ModifyDBInstance
. When you associate a new DB parameter group with a running DB
* instance, you need to reboot the DB instance without failover for the new DB parameter group and associated
* settings to take effect.
*
*
* This command doesn't apply to RDS Custom.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon RDS to fully complete the
* create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter group has been created or modified.
*
*
*
* @param createDBParameterGroupRequest
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsync.CreateDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBParameterGroupAsync(CreateDBParameterGroupRequest createDBParameterGroupRequest);
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup
. Once you've created a DB parameter group, you need to associate it with your
* DB instance using ModifyDBInstance
. When you associate a new DB parameter group with a running DB
* instance, you need to reboot the DB instance without failover for the new DB parameter group and associated
* settings to take effect.
*
*
* This command doesn't apply to RDS Custom.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon RDS to fully complete the
* create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter group has been created or modified.
*
*
*
* @param createDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBParameterGroupAsync(CreateDBParameterGroupRequest createDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB proxy.
*
*
* @param createDBProxyRequest
* @return A Java Future containing the result of the CreateDBProxy operation returned by the service.
* @sample AmazonRDSAsync.CreateDBProxy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBProxyAsync(CreateDBProxyRequest createDBProxyRequest);
/**
*
* Creates a new DB proxy.
*
*
* @param createDBProxyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBProxy operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBProxy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBProxyAsync(CreateDBProxyRequest createDBProxyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a DBProxyEndpoint
. Only applies to proxies that are associated with Aurora DB clusters. You
* can use DB proxy endpoints to specify read/write or read-only access to the DB cluster. You can also use DB proxy
* endpoints to access a DB proxy through a different VPC than the proxy's default VPC.
*
*
* @param createDBProxyEndpointRequest
* @return A Java Future containing the result of the CreateDBProxyEndpoint operation returned by the service.
* @sample AmazonRDSAsync.CreateDBProxyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBProxyEndpointAsync(CreateDBProxyEndpointRequest createDBProxyEndpointRequest);
/**
*
* Creates a DBProxyEndpoint
. Only applies to proxies that are associated with Aurora DB clusters. You
* can use DB proxy endpoints to specify read/write or read-only access to the DB cluster. You can also use DB proxy
* endpoints to access a DB proxy through a different VPC than the proxy's default VPC.
*
*
* @param createDBProxyEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBProxyEndpoint operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBProxyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBProxyEndpointAsync(CreateDBProxyEndpointRequest createDBProxyEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB security group. DB security groups control access to a DB instance.
*
*
*
* A DB security group controls access to EC2-Classic DB instances that are not in a VPC.
*
*
*
* @param createDBSecurityGroupRequest
* @return A Java Future containing the result of the CreateDBSecurityGroup operation returned by the service.
* @sample AmazonRDSAsync.CreateDBSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSecurityGroupAsync(CreateDBSecurityGroupRequest createDBSecurityGroupRequest);
/**
*
* Creates a new DB security group. DB security groups control access to a DB instance.
*
*
*
* A DB security group controls access to EC2-Classic DB instances that are not in a VPC.
*
*
*
* @param createDBSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBSecurityGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSecurityGroupAsync(CreateDBSecurityGroupRequest createDBSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of a DB instance. The source DB instance must be in the available
or
* storage-optimization
state.
*
*
* @param createDBSnapshotRequest
* @return A Java Future containing the result of the CreateDBSnapshot operation returned by the service.
* @sample AmazonRDSAsync.CreateDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSnapshotAsync(CreateDBSnapshotRequest createDBSnapshotRequest);
/**
*
* Creates a snapshot of a DB instance. The source DB instance must be in the available
or
* storage-optimization
state.
*
*
* @param createDBSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSnapshotAsync(CreateDBSnapshotRequest createDBSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the
* Amazon Web Services Region.
*
*
* @param createDBSubnetGroupRequest
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* @sample AmazonRDSAsync.CreateDBSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSubnetGroupAsync(CreateDBSubnetGroupRequest createDBSubnetGroupRequest);
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the
* Amazon Web Services Region.
*
*
* @param createDBSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDBSubnetGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateDBSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDBSubnetGroupAsync(CreateDBSubnetGroupRequest createDBSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an RDS event notification subscription. This action requires a topic Amazon Resource Name (ARN) created
* by either the RDS console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType
) that you want to be notified of and provide a list
* of RDS sources (SourceIds
) that triggers the events. You can also provide a list of event categories
* (EventCategories
) for events that you want to be notified of. For example, you can specify
* SourceType
= db-instance
, SourceIds
= mydbinstance1
,
* mydbinstance2
and EventCategories
= Availability
, Backup
.
*
*
* If you specify both the SourceType
and SourceIds
, such as SourceType
=
* db-instance
and SourceIdentifier
= myDBInstance1
, you are notified of all
* the db-instance
events for the specified source. If you specify a SourceType
but do not
* specify a SourceIdentifier
, you receive notice of the events for that source type for all your RDS
* sources. If you don't specify either the SourceType or the SourceIdentifier
, you are notified of
* events generated from all RDS sources belonging to your customer account.
*
*
*
* RDS event notification is only available for unencrypted SNS topics. If you specify an encrypted SNS topic, event
* notifications aren't sent for the topic.
*
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonRDSAsync.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an RDS event notification subscription. This action requires a topic Amazon Resource Name (ARN) created
* by either the RDS console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType
) that you want to be notified of and provide a list
* of RDS sources (SourceIds
) that triggers the events. You can also provide a list of event categories
* (EventCategories
) for events that you want to be notified of. For example, you can specify
* SourceType
= db-instance
, SourceIds
= mydbinstance1
,
* mydbinstance2
and EventCategories
= Availability
, Backup
.
*
*
* If you specify both the SourceType
and SourceIds
, such as SourceType
=
* db-instance
and SourceIdentifier
= myDBInstance1
, you are notified of all
* the db-instance
events for the specified source. If you specify a SourceType
but do not
* specify a SourceIdentifier
, you receive notice of the events for that source type for all your RDS
* sources. If you don't specify either the SourceType or the SourceIdentifier
, you are notified of
* events generated from all RDS sources belonging to your customer account.
*
*
*
* RDS event notification is only available for unencrypted SNS topics. If you specify an encrypted SNS topic, event
* notifications aren't sent for the topic.
*
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Aurora global database spread across multiple Amazon Web Services Regions. The global database
* contains a single primary cluster with read-write capability, and a read-only secondary cluster that receives
* data from the primary cluster through high-speed replication performed by the Aurora storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and a secondary cluster
* to it. Or you can specify an existing Aurora cluster during the create operation, and this cluster becomes the
* primary cluster of the global database.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createGlobalClusterRequest
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* @sample AmazonRDSAsync.CreateGlobalCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGlobalClusterAsync(CreateGlobalClusterRequest createGlobalClusterRequest);
/**
*
* Creates an Aurora global database spread across multiple Amazon Web Services Regions. The global database
* contains a single primary cluster with read-write capability, and a read-only secondary cluster that receives
* data from the primary cluster through high-speed replication performed by the Aurora storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and a secondary cluster
* to it. Or you can specify an existing Aurora cluster during the create operation, and this cluster becomes the
* primary cluster of the global database.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGlobalCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateGlobalCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createGlobalClusterAsync(CreateGlobalClusterRequest createGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param createOptionGroupRequest
* @return A Java Future containing the result of the CreateOptionGroup operation returned by the service.
* @sample AmazonRDSAsync.CreateOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOptionGroupAsync(CreateOptionGroupRequest createOptionGroupRequest);
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param createOptionGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateOptionGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.CreateOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createOptionGroupAsync(CreateOptionGroupRequest createOptionGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param deleteCustomAvailabilityZoneRequest
* @return A Java Future containing the result of the DeleteCustomAvailabilityZone operation returned by the
* service.
* @sample AmazonRDSAsync.DeleteCustomAvailabilityZone
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomAvailabilityZoneAsync(
DeleteCustomAvailabilityZoneRequest deleteCustomAvailabilityZoneRequest);
/**
*
* Deletes a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param deleteCustomAvailabilityZoneRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomAvailabilityZone operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DeleteCustomAvailabilityZone
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomAvailabilityZoneAsync(
DeleteCustomAvailabilityZoneRequest deleteCustomAvailabilityZoneRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom engine version. To run this command, make sure you meet the following prerequisites:
*
*
* -
*
* The CEV must not be the default for RDS Custom. If it is, change the default before running this command.
*
*
* -
*
* The CEV must not be associated with an RDS Custom DB instance, RDS Custom instance snapshot, or automated backup
* of your RDS Custom instance.
*
*
*
*
* Typically, deletion takes a few minutes.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* DeleteCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* DeleteCustomDbEngineVersion
event.
*
*
*
* For more information, see Deleting a
* CEV in the Amazon RDS User Guide.
*
*
* @param deleteCustomDBEngineVersionRequest
* @return A Java Future containing the result of the DeleteCustomDBEngineVersion operation returned by the service.
* @sample AmazonRDSAsync.DeleteCustomDBEngineVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDBEngineVersionAsync(
DeleteCustomDBEngineVersionRequest deleteCustomDBEngineVersionRequest);
/**
*
* Deletes a custom engine version. To run this command, make sure you meet the following prerequisites:
*
*
* -
*
* The CEV must not be the default for RDS Custom. If it is, change the default before running this command.
*
*
* -
*
* The CEV must not be associated with an RDS Custom DB instance, RDS Custom instance snapshot, or automated backup
* of your RDS Custom instance.
*
*
*
*
* Typically, deletion takes a few minutes.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* DeleteCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* DeleteCustomDbEngineVersion
event.
*
*
*
* For more information, see Deleting a
* CEV in the Amazon RDS User Guide.
*
*
* @param deleteCustomDBEngineVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomDBEngineVersion operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteCustomDBEngineVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDBEngineVersionAsync(
DeleteCustomDBEngineVersionRequest deleteCustomDBEngineVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterRequest
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBClusterAsync(DeleteDBClusterRequest deleteDBClusterRequest);
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBClusterAsync(DeleteDBClusterRequest deleteDBClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom endpoint and removes it from an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterEndpointRequest
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBClusterEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBClusterEndpointAsync(DeleteDBClusterEndpointRequest deleteDBClusterEndpointRequest);
/**
*
* Deletes a custom endpoint and removes it from an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterEndpoint operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBClusterEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBClusterEndpointAsync(DeleteDBClusterEndpointRequest deleteDBClusterEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonRDSAsync.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterParameterGroupAsync(
DeleteDBClusterParameterGroupRequest deleteDBClusterParameterGroupRequest);
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterParameterGroup operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBClusterParameterGroupAsync(
DeleteDBClusterParameterGroupRequest deleteDBClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBClusterSnapshotAsync(DeleteDBClusterSnapshotRequest deleteDBClusterSnapshotRequest);
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBClusterSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteDBClusterSnapshotAsync(DeleteDBClusterSnapshotRequest deleteDBClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon RDS DB instance is deleting
until the DB
* snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* When a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when you skip
* creation of the final snapshot with the SkipFinalSnapshot
parameter.
*
*
* If the specified DB instance is part of an Amazon Aurora DB cluster, you can't delete the DB instance if both of
* the following conditions are true:
*
*
* -
*
* The DB cluster is a read replica of another Amazon Aurora DB cluster.
*
*
* -
*
* The DB instance is the only instance in the DB cluster.
*
*
*
*
* To delete a DB instance in this case, first call the PromoteReadReplicaDBCluster
API action to
* promote the DB cluster so it's no longer a read replica. After the promotion completes, then call the
* DeleteDBInstance
API action to delete the final instance in the DB cluster.
*
*
* @param deleteDBInstanceRequest
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBInstanceAsync(DeleteDBInstanceRequest deleteDBInstanceRequest);
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon RDS DB instance is deleting
until the DB
* snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* When a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when you skip
* creation of the final snapshot with the SkipFinalSnapshot
parameter.
*
*
* If the specified DB instance is part of an Amazon Aurora DB cluster, you can't delete the DB instance if both of
* the following conditions are true:
*
*
* -
*
* The DB cluster is a read replica of another Amazon Aurora DB cluster.
*
*
* -
*
* The DB instance is the only instance in the DB cluster.
*
*
*
*
* To delete a DB instance in this case, first call the PromoteReadReplicaDBCluster
API action to
* promote the DB cluster so it's no longer a read replica. After the promotion completes, then call the
* DeleteDBInstance
API action to delete the final instance in the DB cluster.
*
*
* @param deleteDBInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBInstance operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBInstanceAsync(DeleteDBInstanceRequest deleteDBInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes automated backups using the DbiResourceId
value of the source DB instance or the Amazon
* Resource Name (ARN) of the automated backups.
*
*
* @param deleteDBInstanceAutomatedBackupRequest
* Parameter input for the DeleteDBInstanceAutomatedBackup
operation.
* @return A Java Future containing the result of the DeleteDBInstanceAutomatedBackup operation returned by the
* service.
* @sample AmazonRDSAsync.DeleteDBInstanceAutomatedBackup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBInstanceAutomatedBackupAsync(
DeleteDBInstanceAutomatedBackupRequest deleteDBInstanceAutomatedBackupRequest);
/**
*
* Deletes automated backups using the DbiResourceId
value of the source DB instance or the Amazon
* Resource Name (ARN) of the automated backups.
*
*
* @param deleteDBInstanceAutomatedBackupRequest
* Parameter input for the DeleteDBInstanceAutomatedBackup
operation.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBInstanceAutomatedBackup operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DeleteDBInstanceAutomatedBackup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteDBInstanceAutomatedBackupAsync(
DeleteDBInstanceAutomatedBackupRequest deleteDBInstanceAutomatedBackupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified DB parameter group. The DB parameter group to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDBParameterGroupRequest
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBParameterGroupAsync(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest);
/**
*
* Deletes a specified DB parameter group. The DB parameter group to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDBParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBParameterGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBParameterGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBParameterGroupAsync(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing DB proxy.
*
*
* @param deleteDBProxyRequest
* @return A Java Future containing the result of the DeleteDBProxy operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBProxy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBProxyAsync(DeleteDBProxyRequest deleteDBProxyRequest);
/**
*
* Deletes an existing DB proxy.
*
*
* @param deleteDBProxyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBProxy operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBProxy
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBProxyAsync(DeleteDBProxyRequest deleteDBProxyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DBProxyEndpoint
. Doing so removes the ability to access the DB proxy using the endpoint
* that you defined. The endpoint that you delete might have provided capabilities such as read/write or read-only
* operations, or using a different VPC than the DB proxy's default VPC.
*
*
* @param deleteDBProxyEndpointRequest
* @return A Java Future containing the result of the DeleteDBProxyEndpoint operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBProxyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBProxyEndpointAsync(DeleteDBProxyEndpointRequest deleteDBProxyEndpointRequest);
/**
*
* Deletes a DBProxyEndpoint
. Doing so removes the ability to access the DB proxy using the endpoint
* that you defined. The endpoint that you delete might have provided capabilities such as read/write or read-only
* operations, or using a different VPC than the DB proxy's default VPC.
*
*
* @param deleteDBProxyEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBProxyEndpoint operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBProxyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBProxyEndpointAsync(DeleteDBProxyEndpointRequest deleteDBProxyEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB security group.
*
*
*
* The specified DB security group must not be associated with any DB instances.
*
*
*
* @param deleteDBSecurityGroupRequest
* @return A Java Future containing the result of the DeleteDBSecurityGroup operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSecurityGroupAsync(DeleteDBSecurityGroupRequest deleteDBSecurityGroupRequest);
/**
*
* Deletes a DB security group.
*
*
*
* The specified DB security group must not be associated with any DB instances.
*
*
*
* @param deleteDBSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBSecurityGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSecurityGroupAsync(DeleteDBSecurityGroupRequest deleteDBSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDBSnapshotRequest
* @return A Java Future containing the result of the DeleteDBSnapshot operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSnapshotAsync(DeleteDBSnapshotRequest deleteDBSnapshotRequest);
/**
*
* Deletes a DB snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDBSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBSnapshot operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSnapshotAsync(DeleteDBSnapshotRequest deleteDBSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDBSubnetGroupRequest
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* @sample AmazonRDSAsync.DeleteDBSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSubnetGroupAsync(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest);
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDBSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDBSubnetGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteDBSubnetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDBSubnetGroupAsync(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an RDS event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonRDSAsync.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an RDS event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a global database cluster. The primary and secondary clusters must already be detached or destroyed
* first.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteGlobalClusterRequest
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* @sample AmazonRDSAsync.DeleteGlobalCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGlobalClusterAsync(DeleteGlobalClusterRequest deleteGlobalClusterRequest);
/**
*
* Deletes a global database cluster. The primary and secondary clusters must already be detached or destroyed
* first.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteGlobalClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGlobalCluster operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteGlobalCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteGlobalClusterAsync(DeleteGlobalClusterRequest deleteGlobalClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the installation medium for a DB engine that requires an on-premises customer provided license, such as
* Microsoft SQL Server.
*
*
* @param deleteInstallationMediaRequest
* @return A Java Future containing the result of the DeleteInstallationMedia operation returned by the service.
* @sample AmazonRDSAsync.DeleteInstallationMedia
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteInstallationMediaAsync(DeleteInstallationMediaRequest deleteInstallationMediaRequest);
/**
*
* Deletes the installation medium for a DB engine that requires an on-premises customer provided license, such as
* Microsoft SQL Server.
*
*
* @param deleteInstallationMediaRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInstallationMedia operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteInstallationMedia
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteInstallationMediaAsync(DeleteInstallationMediaRequest deleteInstallationMediaRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an existing option group.
*
*
* @param deleteOptionGroupRequest
* @return A Java Future containing the result of the DeleteOptionGroup operation returned by the service.
* @sample AmazonRDSAsync.DeleteOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOptionGroupAsync(DeleteOptionGroupRequest deleteOptionGroupRequest);
/**
*
* Deletes an existing option group.
*
*
* @param deleteOptionGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteOptionGroup operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeleteOptionGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteOptionGroupAsync(DeleteOptionGroupRequest deleteOptionGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove the association between one or more DBProxyTarget
data structures and a
* DBProxyTargetGroup
.
*
*
* @param deregisterDBProxyTargetsRequest
* @return A Java Future containing the result of the DeregisterDBProxyTargets operation returned by the service.
* @sample AmazonRDSAsync.DeregisterDBProxyTargets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterDBProxyTargetsAsync(DeregisterDBProxyTargetsRequest deregisterDBProxyTargetsRequest);
/**
*
* Remove the association between one or more DBProxyTarget
data structures and a
* DBProxyTargetGroup
.
*
*
* @param deregisterDBProxyTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterDBProxyTargets operation returned by the service.
* @sample AmazonRDSAsyncHandler.DeregisterDBProxyTargets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deregisterDBProxyTargetsAsync(DeregisterDBProxyTargetsRequest deregisterDBProxyTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRDSAsync.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
* @param describeAccountAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeAccountAttributes operation.
*
* @see #describeAccountAttributesAsync(DescribeAccountAttributesRequest)
*/
java.util.concurrent.Future describeAccountAttributesAsync();
/**
* Simplified method form for invoking the DescribeAccountAttributes operation with an AsyncHandler.
*
* @see #describeAccountAttributesAsync(DescribeAccountAttributesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeAccountAttributesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this Amazon Web Services account.
*
*
* @param describeCertificatesRequest
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AmazonRDSAsync.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest);
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this Amazon Web Services account.
*
*
* @param describeCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCertificates operation.
*
* @see #describeCertificatesAsync(DescribeCertificatesRequest)
*/
java.util.concurrent.Future describeCertificatesAsync();
/**
* Simplified method form for invoking the DescribeCertificates operation with an AsyncHandler.
*
* @see #describeCertificatesAsync(DescribeCertificatesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCertificatesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param describeCustomAvailabilityZonesRequest
* @return A Java Future containing the result of the DescribeCustomAvailabilityZones operation returned by the
* service.
* @sample AmazonRDSAsync.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomAvailabilityZonesAsync(
DescribeCustomAvailabilityZonesRequest describeCustomAvailabilityZonesRequest);
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param describeCustomAvailabilityZonesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCustomAvailabilityZones operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomAvailabilityZonesAsync(
DescribeCustomAvailabilityZonesRequest describeCustomAvailabilityZonesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param describeDBClusterBacktracksRequest
* @return A Java Future containing the result of the DescribeDBClusterBacktracks operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterBacktracksAsync(
DescribeDBClusterBacktracksRequest describeDBClusterBacktracksRequest);
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param describeDBClusterBacktracksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterBacktracks operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterBacktracksAsync(
DescribeDBClusterBacktracksRequest describeDBClusterBacktracksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterEndpointsRequest
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBClusterEndpointsAsync(
DescribeDBClusterEndpointsRequest describeDBClusterEndpointsRequest);
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterEndpoints operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBClusterEndpointsAsync(
DescribeDBClusterEndpointsRequest describeDBClusterEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* @sample AmazonRDSAsync.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync(
DescribeDBClusterParameterGroupsRequest describeDBClusterParameterGroupsRequest);
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterParameterGroups operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync(
DescribeDBClusterParameterGroupsRequest describeDBClusterParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBClusterParameterGroups operation.
*
* @see #describeDBClusterParameterGroupsAsync(DescribeDBClusterParameterGroupsRequest)
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync();
/**
* Simplified method form for invoking the DescribeDBClusterParameterGroups operation with an AsyncHandler.
*
* @see #describeDBClusterParameterGroupsAsync(DescribeDBClusterParameterGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBClusterParameterGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParametersRequest
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParametersAsync(
DescribeDBClusterParametersRequest describeDBClusterParametersRequest);
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterParameters operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterParametersAsync(
DescribeDBClusterParametersRequest describeDBClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other Amazon Web Services accounts, DescribeDBClusterSnapshotAttributes
* returns the restore
attribute and a list of IDs for the Amazon Web Services accounts that are
* authorized to copy or restore the manual DB cluster snapshot. If all
is included in the list of
* values for the restore
attribute, then the manual DB cluster snapshot is public and can be copied or
* restored by all Amazon Web Services accounts.
*
*
* To add or remove access for an Amazon Web Services account to copy or restore a manual DB cluster snapshot, or to
* make the manual DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute
API
* action.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotAttributesRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* @sample AmazonRDSAsync.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotAttributesAsync(
DescribeDBClusterSnapshotAttributesRequest describeDBClusterSnapshotAttributesRequest);
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other Amazon Web Services accounts, DescribeDBClusterSnapshotAttributes
* returns the restore
attribute and a list of IDs for the Amazon Web Services accounts that are
* authorized to copy or restore the manual DB cluster snapshot. If all
is included in the list of
* values for the restore
attribute, then the manual DB cluster snapshot is public and can be copied or
* restored by all Amazon Web Services accounts.
*
*
* To add or remove access for an Amazon Web Services account to copy or restore a manual DB cluster snapshot, or to
* make the manual DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute
API
* action.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterSnapshotAttributes operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotAttributesAsync(
DescribeDBClusterSnapshotAttributesRequest describeDBClusterSnapshotAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotsRequest
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync(
DescribeDBClusterSnapshotsRequest describeDBClusterSnapshotsRequest);
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusterSnapshots operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync(
DescribeDBClusterSnapshotsRequest describeDBClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBClusterSnapshots operation.
*
* @see #describeDBClusterSnapshotsAsync(DescribeDBClusterSnapshotsRequest)
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeDBClusterSnapshots operation with an AsyncHandler.
*
* @see #describeDBClusterSnapshotsAsync(DescribeDBClusterSnapshotsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBClusterSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBClustersRequest
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBClustersAsync(DescribeDBClustersRequest describeDBClustersRequest);
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBClusters operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBClustersAsync(DescribeDBClustersRequest describeDBClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBClusters operation.
*
* @see #describeDBClustersAsync(DescribeDBClustersRequest)
*/
java.util.concurrent.Future describeDBClustersAsync();
/**
* Simplified method form for invoking the DescribeDBClusters operation with an AsyncHandler.
*
* @see #describeDBClustersAsync(DescribeDBClustersRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest);
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBEngineVersions operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBEngineVersions operation.
*
* @see #describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest)
*/
java.util.concurrent.Future describeDBEngineVersionsAsync();
/**
* Simplified method form for invoking the DescribeDBEngineVersions operation with an AsyncHandler.
*
* @see #describeDBEngineVersionsAsync(DescribeDBEngineVersionsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBEngineVersionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
* @param describeDBInstanceAutomatedBackupsRequest
* Parameter input for DescribeDBInstanceAutomatedBackups.
* @return A Java Future containing the result of the DescribeDBInstanceAutomatedBackups operation returned by the
* service.
* @sample AmazonRDSAsync.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBInstanceAutomatedBackupsAsync(
DescribeDBInstanceAutomatedBackupsRequest describeDBInstanceAutomatedBackupsRequest);
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
* @param describeDBInstanceAutomatedBackupsRequest
* Parameter input for DescribeDBInstanceAutomatedBackups.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBInstanceAutomatedBackups operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBInstanceAutomatedBackupsAsync(
DescribeDBInstanceAutomatedBackupsRequest describeDBInstanceAutomatedBackupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBInstancesRequest
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBInstances
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBInstancesAsync(DescribeDBInstancesRequest describeDBInstancesRequest);
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBInstances operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBInstances
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBInstancesAsync(DescribeDBInstancesRequest describeDBInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBInstances operation.
*
* @see #describeDBInstancesAsync(DescribeDBInstancesRequest)
*/
java.util.concurrent.Future describeDBInstancesAsync();
/**
* Simplified method form for invoking the DescribeDBInstances operation with an AsyncHandler.
*
* @see #describeDBInstancesAsync(DescribeDBInstancesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DB log files for the DB instance.
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param describeDBLogFilesRequest
* @return A Java Future containing the result of the DescribeDBLogFiles operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBLogFilesAsync(DescribeDBLogFilesRequest describeDBLogFilesRequest);
/**
*
* Returns a list of DB log files for the DB instance.
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param describeDBLogFilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBLogFiles operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBLogFiles
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBLogFilesAsync(DescribeDBLogFilesRequest describeDBLogFilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @param describeDBParameterGroupsRequest
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBParameterGroupsAsync(
DescribeDBParameterGroupsRequest describeDBParameterGroupsRequest);
/**
*
* Returns a list of DBParameterGroup
descriptions. If a DBParameterGroupName
is
* specified, the list will contain only the description of the specified DB parameter group.
*
*
* @param describeDBParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBParameterGroups operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBParameterGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBParameterGroupsAsync(
DescribeDBParameterGroupsRequest describeDBParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBParameterGroups operation.
*
* @see #describeDBParameterGroupsAsync(DescribeDBParameterGroupsRequest)
*/
java.util.concurrent.Future describeDBParameterGroupsAsync();
/**
* Simplified method form for invoking the DescribeDBParameterGroups operation with an AsyncHandler.
*
* @see #describeDBParameterGroupsAsync(DescribeDBParameterGroupsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBParameterGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
* @param describeDBParametersRequest
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBParametersAsync(DescribeDBParametersRequest describeDBParametersRequest);
/**
*
* Returns the detailed parameter list for a particular DB parameter group.
*
*
* @param describeDBParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBParameters operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBParameters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBParametersAsync(DescribeDBParametersRequest describeDBParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB proxies.
*
*
* @param describeDBProxiesRequest
* @return A Java Future containing the result of the DescribeDBProxies operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBProxies
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBProxiesAsync(DescribeDBProxiesRequest describeDBProxiesRequest);
/**
*
* Returns information about DB proxies.
*
*
* @param describeDBProxiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBProxies operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBProxies
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBProxiesAsync(DescribeDBProxiesRequest describeDBProxiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB proxy endpoints.
*
*
* @param describeDBProxyEndpointsRequest
* @return A Java Future containing the result of the DescribeDBProxyEndpoints operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBProxyEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBProxyEndpointsAsync(DescribeDBProxyEndpointsRequest describeDBProxyEndpointsRequest);
/**
*
* Returns information about DB proxy endpoints.
*
*
* @param describeDBProxyEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBProxyEndpoints operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBProxyEndpoints
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBProxyEndpointsAsync(DescribeDBProxyEndpointsRequest describeDBProxyEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
* @param describeDBProxyTargetGroupsRequest
* @return A Java Future containing the result of the DescribeDBProxyTargetGroups operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBProxyTargetGroupsAsync(
DescribeDBProxyTargetGroupsRequest describeDBProxyTargetGroupsRequest);
/**
*
* Returns information about DB proxy target groups, represented by DBProxyTargetGroup
data structures.
*
*
* @param describeDBProxyTargetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBProxyTargetGroups operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBProxyTargetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBProxyTargetGroupsAsync(
DescribeDBProxyTargetGroupsRequest describeDBProxyTargetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
* @param describeDBProxyTargetsRequest
* @return A Java Future containing the result of the DescribeDBProxyTargets operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBProxyTargetsAsync(DescribeDBProxyTargetsRequest describeDBProxyTargetsRequest);
/**
*
* Returns information about DBProxyTarget
objects. This API supports pagination.
*
*
* @param describeDBProxyTargetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBProxyTargets operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBProxyTargets
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBProxyTargetsAsync(DescribeDBProxyTargetsRequest describeDBProxyTargetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
* @param describeDBSecurityGroupsRequest
* @return A Java Future containing the result of the DescribeDBSecurityGroups operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBSecurityGroupsAsync(DescribeDBSecurityGroupsRequest describeDBSecurityGroupsRequest);
/**
*
* Returns a list of DBSecurityGroup
descriptions. If a DBSecurityGroupName
is specified,
* the list will contain only the descriptions of the specified DB security group.
*
*
* @param describeDBSecurityGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBSecurityGroups operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBSecurityGroups
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDBSecurityGroupsAsync(DescribeDBSecurityGroupsRequest describeDBSecurityGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBSecurityGroups operation.
*
* @see #describeDBSecurityGroupsAsync(DescribeDBSecurityGroupsRequest)
*/
java.util.concurrent.Future describeDBSecurityGroupsAsync();
/**
* Simplified method form for invoking the DescribeDBSecurityGroups operation with an AsyncHandler.
*
* @see #describeDBSecurityGroupsAsync(DescribeDBSecurityGroupsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBSecurityGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DB snapshot attribute names and values for a manual DB snapshot.
*
*
* When sharing snapshots with other Amazon Web Services accounts, DescribeDBSnapshotAttributes
returns
* the restore
attribute and a list of IDs for the Amazon Web Services accounts that are authorized to
* copy or restore the manual DB snapshot. If all
is included in the list of values for the
* restore
attribute, then the manual DB snapshot is public and can be copied or restored by all Amazon
* Web Services accounts.
*
*
* To add or remove access for an Amazon Web Services account to copy or restore a manual DB snapshot, or to make
* the manual DB snapshot public or private, use the ModifyDBSnapshotAttribute
API action.
*
*
* @param describeDBSnapshotAttributesRequest
* @return A Java Future containing the result of the DescribeDBSnapshotAttributes operation returned by the
* service.
* @sample AmazonRDSAsync.DescribeDBSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBSnapshotAttributesAsync(
DescribeDBSnapshotAttributesRequest describeDBSnapshotAttributesRequest);
/**
*
* Returns a list of DB snapshot attribute names and values for a manual DB snapshot.
*
*
* When sharing snapshots with other Amazon Web Services accounts, DescribeDBSnapshotAttributes
returns
* the restore
attribute and a list of IDs for the Amazon Web Services accounts that are authorized to
* copy or restore the manual DB snapshot. If all
is included in the list of values for the
* restore
attribute, then the manual DB snapshot is public and can be copied or restored by all Amazon
* Web Services accounts.
*
*
* To add or remove access for an Amazon Web Services account to copy or restore a manual DB snapshot, or to make
* the manual DB snapshot public or private, use the ModifyDBSnapshotAttribute
API action.
*
*
* @param describeDBSnapshotAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBSnapshotAttributes operation returned by the
* service.
* @sample AmazonRDSAsyncHandler.DescribeDBSnapshotAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDBSnapshotAttributesAsync(
DescribeDBSnapshotAttributesRequest describeDBSnapshotAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBSnapshotAttributes operation.
*
* @see #describeDBSnapshotAttributesAsync(DescribeDBSnapshotAttributesRequest)
*/
java.util.concurrent.Future describeDBSnapshotAttributesAsync();
/**
* Simplified method form for invoking the DescribeDBSnapshotAttributes operation with an AsyncHandler.
*
* @see #describeDBSnapshotAttributesAsync(DescribeDBSnapshotAttributesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBSnapshotAttributesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
* @param describeDBSnapshotsRequest
* @return A Java Future containing the result of the DescribeDBSnapshots operation returned by the service.
* @sample AmazonRDSAsync.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBSnapshotsAsync(DescribeDBSnapshotsRequest describeDBSnapshotsRequest);
/**
*
* Returns information about DB snapshots. This API action supports pagination.
*
*
* @param describeDBSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDBSnapshots operation returned by the service.
* @sample AmazonRDSAsyncHandler.DescribeDBSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDBSnapshotsAsync(DescribeDBSnapshotsRequest describeDBSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeDBSnapshots operation.
*
* @see #describeDBSnapshotsAsync(DescribeDBSnapshotsRequest)
*/
java.util.concurrent.Future describeDBSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeDBSnapshots operation with an AsyncHandler.
*
* @see #describeDBSnapshotsAsync(DescribeDBSnapshotsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeDBSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of DBSubnetGroup descriptions. If a DBSubnetGroupName is specified, the list will contain only the
* descriptions of the specified DBSubnetGroup.
*
*
* For an overview of CIDR ranges, go to the